1 | # Clock component
|
2 |
|
3 | The clock component is, in reality, a wrapper function, which wraps the component, which you supply and provides all the props you need to create your own look and feel for the clock :)
|
4 |
|
5 | 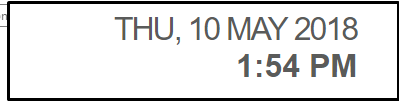
|
6 |
|
7 | [Live examples here](https://adactivesas.github.io/adsum-react-components/packages/adsum-clock/examples/index.html)
|
8 |
|
9 | ## Getting started
|
10 |
|
11 | ```javascript
|
12 | npm i --save @adactive/arc-clock
|
13 | ```
|
14 | OR
|
15 | ```javascript
|
16 | yarn add @adactive/arc-clock
|
17 | ```
|
18 |
|
19 | ```javascript
|
20 | import AdsumClock from "@adactive/arc-clock"
|
21 | ...
|
22 | // Your own stateless UI component for the clock
|
23 | // You will be provided with props, which are described below
|
24 | const ClockUi = (props) => (
|
25 | <div role="presentation" className="adsum-clock-wrapper">
|
26 | <div className="adsum-clock">
|
27 | <div className="day-date">{props.dateStr}</div>
|
28 | <div className="time">{props.timeStr}</div>
|
29 | </div>
|
30 | </div>
|
31 | );
|
32 |
|
33 |
|
34 | // The actual wrapping of your component with AdsumClock wrapper
|
35 | const Clock = AdsumClock(ClockUi);
|
36 |
|
37 | // Usage of the wrapped component
|
38 | <Clock lang="en" timeFormat="12hrs" />
|
39 | ```
|
40 |
|
41 | ### Props
|
42 |
|
43 | ```javascript
|
44 | static defaultProps = {
|
45 | lang: 'en',
|
46 | timeFormat: '24hrs',
|
47 | };
|
48 |
|
49 | type AdsumClockPropsType = {
|
50 | lang: LangType,
|
51 | timeFormat: TimeFormatType
|
52 | };
|
53 | ```
|
54 |
|
55 | ### Additional props, which will be passed to the provided ClockUi component:
|
56 |
|
57 | ```javascript
|
58 |
|
59 | {
|
60 | +year: string,
|
61 | +month: string,
|
62 | +day: string,
|
63 | +hours: string,
|
64 | +minutes: string,
|
65 | +dateStr: string,
|
66 | +timeStr: string
|
67 | };
|
68 |
|
69 | ```
|
70 |
|
71 | ```javascript
|
72 | type LangType = 'en' | 'zh' | 'fr';
|
73 | type TimeFormatType = '24hrs' | '12hrs';
|
74 | type AdsumClockPropsType = {
|
75 | lang: LangType,
|
76 | timeFormat: TimeFormatType
|
77 | };
|
78 | ```
|
79 |
|
80 |
|
81 | ## Copy component inside your project src folder
|
82 |
|
83 | ### Less only
|
84 | `npx @adactive/arc-clock copy --less-only`
|
85 |
|
86 | ### Full copy
|
87 | `npx @adactive/arc-clock copy`
|