1 | # G6: A Graph Visualization Framework in TypeScript
|
2 |
|
3 | 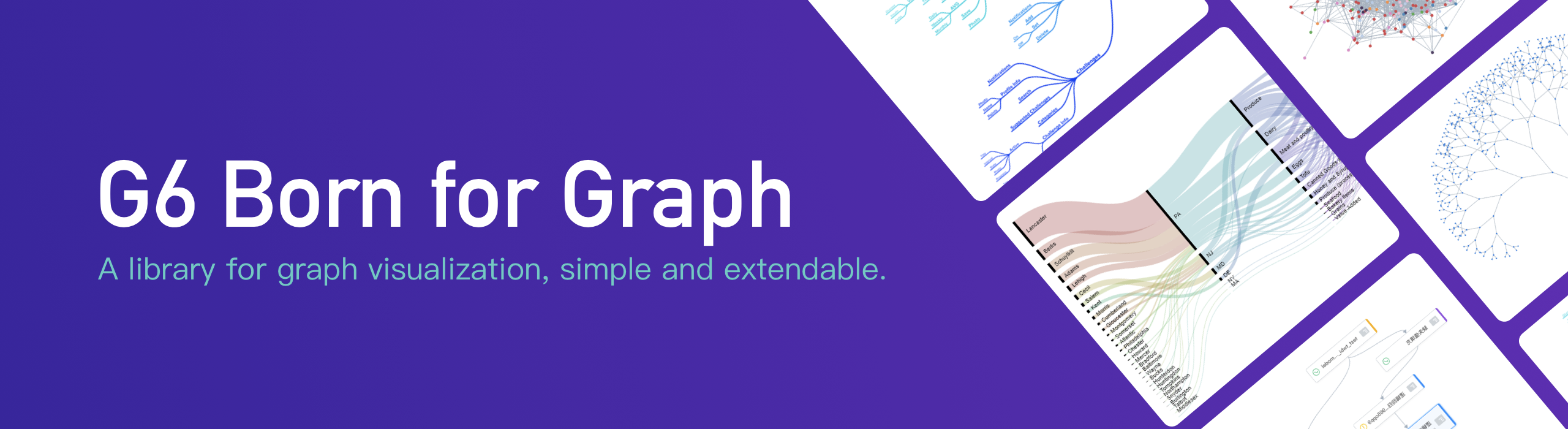
|
4 |
|
5 | [](https://travis-ci.org/antvis/g6) [](https://codecov.io/gh/antvis/G6)   [](https://www.npmjs.com/package/@antv/g6) [](https://npmjs.org/package/@antv/g6) [](http://isitmaintained.com/project/antvis/g6 'Percentage of issues still open')
|
6 |
|
7 | [中文 README](README.md)
|
8 |
|
9 | ## What is G6
|
10 |
|
11 | [G6](https://github.com/antvis/g6) is a graph visualization engine, which provides a set of basic mechanisms, including rendering, layout, analysis, interaction, animation, and other auxiliary tools. G6 aims to simplify the relationships, and help people to obtain the insight of relational data.
|
12 |
|
13 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*zTjwQaXokeQAAAAAAAAAAABkARQnAQ' width=550 alt='' />
|
14 |
|
15 | Developers are able to build graph visualization **analysis** applications or graph visualization **modeling** applications easily.
|
16 |
|
17 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*zau8QJcVpDQAAAAAAAAAAABkARQnAQ' height=200 alt='' /><img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*RIlETY_S6IoAAAAAAAAAAABkARQnAQ' height=200 alt='' />
|
18 |
|
19 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*cDzXR4jIWr8AAAAAAAAAAABkARQnAQ' height=150 alt='' /><img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*DifbSahOblAAAAAAAAAAAABkARQnAQ' height=150 alt='' /><img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*HTasSJGC4koAAAAAAAAAAABkARQnAQ' height=150 alt='' />
|
20 |
|
21 | > Powerful Animation and Interactions
|
22 |
|
23 | <img src="https://user-images.githubusercontent.com/6113694/44995293-02858600-afd5-11e8-840c-349e4730d63d.gif" height=150 alt='' /><img src="https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*I9OdTbXJIi0AAAAAAAAAAABkARQnAQ" height=150 alt='' /><img src="https://user-images.githubusercontent.com/6113694/44995332-2ba61680-afd5-11e8-8cab-db0e9d08ceb7.gif" height=150 alt='' />
|
24 |
|
25 | <img src="https://gw.alipayobjects.com/zos/rmsportal/HQxYguinFOMIXrGQOABY.gif" height=150 alt='' /><img src="https://gw.alipayobjects.com/zos/rmsportal/nAugyFgrbrUWPmDIDiQm.gif" height=150 alt='' /><img src="https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*xoufSYcjK2AAAAAAAAAAAABkARQnAQ" height=150 alt='' />
|
26 |
|
27 | > Powerful Layouts
|
28 |
|
29 | ## Features
|
30 |
|
31 | - Abundant Built-in Items: Nodes and edges with free configurations;
|
32 | - Steerable Interactions: More than 10 basic interaction behaviors ;
|
33 | - Powerful Layout: More than 10 layout algorithms;
|
34 | - Convenient Components: Outstanding ability and performance;
|
35 | - Friendly User Experience: Complete documents for different levels of user requirements. TypeScript supported.
|
36 |
|
37 | G6 concentrates on the principle of 'good by default'. In addition, the custom mechanism of the item, interation behavior, and layout satisfies the customazation requirements.
|
38 |
|
39 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*Y0c6S7cxjVkAAAAAAAAAAABkARQnAQ' width=800 height=200 alt='' />
|
40 |
|
41 | > Abundant Built-in Items
|
42 |
|
43 | ## Installation
|
44 |
|
45 | ```bash
|
46 | $ npm install @antv/g6
|
47 | ```
|
48 |
|
49 | ## Usage
|
50 |
|
51 | <img src="https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*khbvSrptr0kAAAAAAAAAAABkARQnAQ" width=437 height=148 alt='' />
|
52 |
|
53 | ```js
|
54 | import G6 from '@antv/g6';
|
55 |
|
56 | const data = {
|
57 | nodes: [
|
58 | {
|
59 | id: 'node1',
|
60 | label: 'Circle1',
|
61 | x: 150,
|
62 | y: 150,
|
63 | },
|
64 | {
|
65 | id: 'node2',
|
66 | label: 'Circle2',
|
67 | x: 400,
|
68 | y: 150,
|
69 | },
|
70 | ],
|
71 | edges: [
|
72 | {
|
73 | source: 'node1',
|
74 | target: 'node2',
|
75 | },
|
76 | ],
|
77 | };
|
78 |
|
79 | const graph = new G6.Graph({
|
80 | container: 'container',
|
81 | width: 500,
|
82 | height: 500,
|
83 | defaultNode: {
|
84 | type: 'circle',
|
85 | size: [100],
|
86 | color: '#5B8FF9',
|
87 | style: {
|
88 | fill: '#9EC9FF',
|
89 | lineWidth: 3,
|
90 | },
|
91 | labelCfg: {
|
92 | style: {
|
93 | fill: '#fff',
|
94 | fontSize: 20,
|
95 | },
|
96 | },
|
97 | },
|
98 | defaultEdge: {
|
99 | style: {
|
100 | stroke: '#e2e2e2',
|
101 | },
|
102 | },
|
103 | });
|
104 |
|
105 | graph.data(data);
|
106 | graph.render();
|
107 | ```
|
108 |
|
109 | [](https://codesandbox.io/s/compassionate-lalande-5lxm7?fontsize=14&hidenavigation=1&theme=dark)
|
110 |
|
111 | For more information of the usage, please refer to [Getting Started](https://g6.antv.antgroup.com/en/manual/getting-started).
|
112 |
|
113 | ## Development
|
114 |
|
115 | ```bash
|
116 | $ npm install
|
117 |
|
118 | # lerna bootstrap for multiple packages
|
119 | $ npm run bootstrap
|
120 |
|
121 | # build the packages
|
122 | $ npm run build:all
|
123 |
|
124 | # if you wanna watch one of the packages, e.g. packages/core
|
125 | $ cd ./packages/core
|
126 | $ npm run watch
|
127 |
|
128 | # run test case
|
129 | $ npm test
|
130 |
|
131 | # run test case in watch mode
|
132 | npm test -- --watch ./tests/unit/algorithm/find-path-spec
|
133 | DEBUG_MODE=1 npm test -- --watch ./tests/unit/algorithm/find-path-spec
|
134 | ```
|
135 |
|
136 | ## Documents
|
137 |
|
138 | - <a href='https://g6.antv.antgroup.com/en/manual/tutorial/preface' target='_blank'>Tutorial</a>
|
139 | - <a href='https://g6.antv.antgroup.com/en/manual/middle/overview' target='_blank'>Middle Guides</a>
|
140 | - <a href='https://g6.antv.antgroup.com/en/manual/advanced/coordinate-system' target='_blank'>Further Reading</a>
|
141 | - <a href='https://g6.antv.antgroup.com/en/api/Graph' target='_blank'>API Reference</a>
|
142 |
|
143 | ## React project integration
|
144 |
|
145 | For React project integration, we have an independent product recommendation: [Graphin](https://graphin.antv.vision), which is a toolkit based on G6 and React, that focuses on relational visual analysis. It's simple, efficient, out of the box.
|
146 |
|
147 | At present, Graphin has good practices in business graph analysis projects. For details, see [《Who uses Graphin》](https://github.com/antvis/Graphin/issues/212)
|
148 |
|
149 | ## G6 Communication Group
|
150 |
|
151 | Welcome to join the **G6 Communication Group** or **G6 Communication Group-2** (DingTalk groups). We also welcome the github issues.
|
152 |
|
153 | <p>
|
154 | <a href="https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*-RO_R7SCvroAAAAAAAAAAAAAARQnAQ" >
|
155 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*-RO_R7SCvroAAAAAAAAAAAAAARQnAQ' style='width:250px;display:inline-block;vertical-align:top;' alt='' />
|
156 | </a>
|
157 | <a href="https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*hzfaSrAj0jkAAAAAAAAAAABkARQnAQ" >
|
158 | <img src='https://gw.alipayobjects.com/mdn/rms_f8c6a0/afts/img/A*hzfaSrAj0jkAAAAAAAAAAABkARQnAQ' style='width:250px;display:inline-block;vertical-align:top;' alt='' />
|
159 | </a>
|
160 | <a href="https://graphin.antv.vision/" >
|
161 | <img src='https://camo.githubusercontent.com/5e6624abcdde991f9fd89fce4933ad133a48d8fb603d1852c670da329df73ef7/68747470733a2f2f67772e616c697061796f626a656374732e636f6d2f6d646e2f726d735f3430326331612f616674732f696d672f412a2d717a6f54704c672d3163414141414141414141414141414152516e4151' style='width:250px;display:inline-block;vertical-align: top;' alt='' />
|
162 | </a>
|
163 | </p>
|
164 | ## How to Contribute
|
165 |
|
166 | Please let us know what you are you going to help. Do check out [issues](https://github.com/antvis/g6/issues) for bug reports or suggestions first.
|
167 |
|
168 | ## License
|
169 |
|
170 | [MIT license](./LICENSE).
|