1 | <h1 align="center">
|
2 | <br>
|
3 | clasp
|
4 | <br>
|
5 | </h1>
|
6 |
|
7 | <p align="center"><a href="https://travis-ci.org/google/clasp"><img src="https://travis-ci.org/google/clasp.svg?branch=master" alt="Build Status"></a> <a href="https://coveralls.io/github/google/clasp?branch=master"><img src="https://coveralls.io/repos/github/google/clasp/badge.svg?branch=master" alt="Coverage Status"></a> <a href="https://www.npmjs.com/package/@google/clasp"><img src="https://img.shields.io/npm/v/@google/clasp.svg" alt="npm Version"></a> <a href="https://npmcharts.com/compare/@google/clasp?minimal=true"><img src="https://img.shields.io/npm/dw/@google/clasp.svg" alt="npm Downloads"></a> <a href="http://packagequality.com/#?package=%40google%2Fclasp"><img src="http://npm.packagequality.com/shield/%40google%2Fclasp.svg" alt="Package Quality"></a> <a href="https://david-dm.org/google/clasp" title="dependencies status"><img src="https://david-dm.org/google/clasp/status.svg"/></a></p>
|
8 |
|
9 | > Develop [Apps Script](https://developers.google.com/apps-script/) projects locally using clasp (*C*ommand *L*ine *A*pps *S*cript *P*rojects).
|
10 |
|
11 |
|
12 |
|
13 |
|
14 | clasp create "Hello"
|
15 | ls
|
16 | echo 'function hello() {
|
17 | Logger.log("Hello, Apps Script!");
|
18 | }' >> hello.js
|
19 | clasp push
|
20 | clasp deploy
|
21 | rm .clasp.json appsscript.json hello.js
|
22 | clear
|
23 | -->
|
24 | 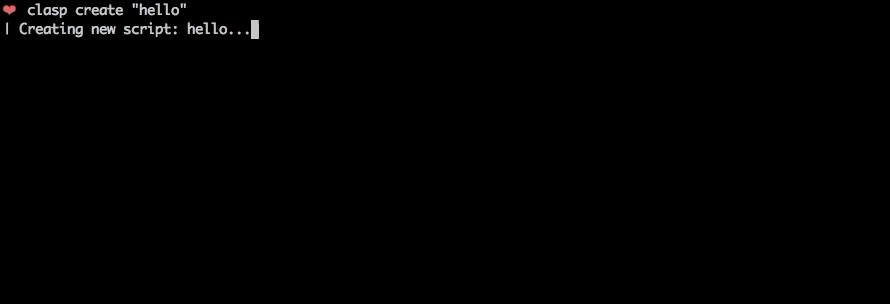
|
25 |
|
26 | **To get started, try out the [codelab](https://g.co/codelabs/clasp)!**
|
27 |
|
28 | ## Features
|
29 |
|
30 | **πΊοΈ Develop Locally:** `clasp` allows you to develop your Apps Script projects locally. That means you can check-in your code into source control, collaborate with other developers, and use your favorite tools to develop Apps Script.
|
31 |
|
32 | **π’ Manage Deployment Versions:** Create, update, and view your multiple deployments of your project.
|
33 |
|
34 | **π Structure Code:** `clasp` automatically converts your flat project on [script.google.com](https://script.google.com) into **folders**. For example:
|
35 |
|
36 | - _On script.google.com_:
|
37 | - `tests/slides.gs`
|
38 | - `tests/sheets.gs`
|
39 | - _locally_:
|
40 | - `tests/`
|
41 | - `slides.js`
|
42 | - `sheets.js`
|
43 |
|
44 | **π· Write Apps Script in TypeScript:** Write your Apps Script projects using TypeScript features:
|
45 |
|
46 | - Arrow functions
|
47 | - Optional structural typing
|
48 | - Classes
|
49 | - Type inference
|
50 | - Interfaces
|
51 | - [And more...](docs/typescript.md)
|
52 |
|
53 | **β‘οΈ Run Apps Script:** Execute your Apps Script from the command line. Features:
|
54 |
|
55 | - _Instant_ deployment.
|
56 | - Suggested functions Autocomplete (Fuzzy)
|
57 | - Easily add custom Google OAuth scopes
|
58 | - [And more...](docs/run.md)
|
59 |
|
60 | ## Install
|
61 |
|
62 | First download `clasp`:
|
63 |
|
64 | ```sh
|
65 | npm install -g @google/clasp
|
66 | # Alternatively: sudo npm i -g grpc @google/clasp --unsafe-perm
|
67 | ```
|
68 |
|
69 | Then enable the Google Apps Script API: https://script.google.com/home/usersettings
|
70 |
|
71 | 
|
72 |
|
73 | ## Commands
|
74 |
|
75 | The following command provide basic Apps Script project management.
|
76 |
|
77 | > Note: Most of them require you to `clasp login` and `clasp create/clone` before using the rest of the commands.
|
78 |
|
79 | ```sh
|
80 | clasp
|
81 | ```
|
82 |
|
83 | - [`clasp login [--no-localhost] [--creds <file>] [--status]`](#login)
|
84 | - [`clasp logout`](#logout)
|
85 | - [`clasp create [--title <title>] [--type <type>] [--rootDir <dir>] [--parentId <id>]`](#create)
|
86 | - [`clasp clone <scriptId | scriptURL> [versionNumber] [--rootDir <dir>]`](#clone)
|
87 | - [`clasp pull [--versionNumber]`](#pull)
|
88 | - [`clasp push [--watch] [--force]`](#push)
|
89 | - [`clasp status [--json]`](#status)
|
90 | - [`clasp open [scriptId] [--webapp] [--creds]`](#open)
|
91 | - [`clasp deployments`](#deployments)
|
92 | - [`clasp deploy [--versionNumber <version>] [--description <description>] [--deploymentId <id>]`](#deploy)
|
93 | - [`clasp undeploy [deploymentId] [--all]`](#undeploy)
|
94 | - [`clasp version [description]`](#version)
|
95 | - [`clasp versions`](#versions)
|
96 | - [`clasp list`](#list)
|
97 |
|
98 | ### Advanced Commands
|
99 |
|
100 | > **NOTE**: These commands require you to add your [Project ID](#projectid-optional).
|
101 |
|
102 | - [`clasp logs [--json] [--open] [--setup] [--watch] [--simplified]`](#logs)
|
103 | - [`clasp apis list`](#apis)
|
104 | - [`clasp apis enable <api>`](#apis)
|
105 | - [`clasp apis disable <api>`](#apis)
|
106 | - [`clasp setting <key> [value]`](#setting)
|
107 |
|
108 | #### Clasp Run
|
109 |
|
110 | > **NOTE**: This command requires you to [bring your own Google API credentials](/docs/run.md).
|
111 |
|
112 | - [`clasp run [functionName] [--nondev] [--params <StringArray>]`](#run)
|
113 |
|
114 | ## Reference
|
115 |
|
116 | ### Login
|
117 |
|
118 | Logs the user in. Saves the client credentials to a `.clasprc.json` file.
|
119 |
|
120 | #### Options
|
121 |
|
122 | - `--no-localhost`: Do not run a local server, manually enter code instead.
|
123 | - `--creds <file>`: Use custom credentials used for `clasp run`. Saves a `.clasprc.json` file to current working directory. This file should be private!
|
124 | - `--status`: Print who you are currently logged in as, if anyone.
|
125 |
|
126 | #### Examples
|
127 |
|
128 | - `clasp login --no-localhost`
|
129 | - `clasp login --creds creds.json`
|
130 | - `clasp login --status`
|
131 |
|
132 | ### Logout
|
133 |
|
134 | Logs out the user by deleting client credentials.
|
135 |
|
136 | #### Examples
|
137 |
|
138 | - `clasp logout`
|
139 |
|
140 | ### Create
|
141 |
|
142 | Creates a new script project. Prompts the user for the script type if not specified.
|
143 |
|
144 | #### Options
|
145 |
|
146 | - `--type [docs/sheets/slides/forms]`: If specified, creates a new add-on attached to a Document, Spreadsheet, Presentation, or Form. If `--parentId` is specified, this value is ignored.
|
147 | - `--title <title>`: A project title.
|
148 | - `--rootDir <dir>`: Local directory in which clasp will store your project files. If not specified, clasp will default to the current directory.
|
149 | - `--parentId <id>`: A project parent Id.
|
150 | - The Drive ID of a parent file that the created script project is bound to. This is usually the ID of a Google Doc, Google Sheet, Google Form, or Google Slides file. If not set, a standalone script project is created.
|
151 | - i.e. `https://docs.google.com/presentation/d/{id}/edit`
|
152 |
|
153 | #### Examples
|
154 |
|
155 | - `clasp create`
|
156 | - `clasp create --type standalone` (default)
|
157 | - `clasp create --type docs`
|
158 | - `clasp create --type sheets`
|
159 | - `clasp create --type slides`
|
160 | - `clasp create --type forms`
|
161 | - `clasp create --type webapp`
|
162 | - `clasp create --type api`
|
163 | - `clasp create --title "My Script"`
|
164 | - `clasp create --rootDir ./dist`
|
165 | - `clasp create --parentId "1D_Gxyv*****************************NXO7o"`
|
166 |
|
167 | These options can be combined like so:
|
168 |
|
169 | - `clasp create --title "My Script" --parentId "1D_Gxyv*****************************NXO7o" --rootDir ./dist`
|
170 |
|
171 | ### Clone
|
172 |
|
173 | Clones the script project from script.google.com.
|
174 |
|
175 | #### Options
|
176 |
|
177 | - `scriptId | scriptURL`: The script ID _or_ script URL to clone.
|
178 | - `--versionNumber <number>`: The version of the script to clone.
|
179 | - `--rootDir <dir>`: Local directory in which clasp will store your project files. If not specified, clasp will default to the current directory.
|
180 |
|
181 | #### Examples
|
182 |
|
183 | - `clasp clone "15ImUCpyi1Jsd8yF8Z6wey_7cw793CymWTLxOqwMka3P1CzE5hQun6qiC"`
|
184 | - `clasp clone "https://script.google.com/d/15ImUCpyi1Jsd8yF8Z6wey_7cw793CymWTLxOqwMka3P1CzE5hQun6qiC/edit"`
|
185 | - `clasp clone "15ImUCpyi1Jsd8yF8Z6wey_7cw793CymWTLxOqwMka3P1CzE5hQun6qiC" --rootDir ./src`
|
186 |
|
187 | ### Pull
|
188 |
|
189 | Fetches a project from either a provided or saved script ID.
|
190 | Updates local files with Apps Script project.
|
191 |
|
192 | #### Options
|
193 |
|
194 | - `--versionNumber <number>`: The version number of the project to retrieve.
|
195 |
|
196 | #### Examples
|
197 |
|
198 | - `clasp pull`
|
199 | - `clasp pull --versionNumber 23`
|
200 |
|
201 | ### Push
|
202 |
|
203 | Force writes all local files to script.google.com.
|
204 |
|
205 | Ignores files:
|
206 |
|
207 | - That start with a `.`
|
208 | - That don't have an accepted file extension
|
209 | - That are ignored (filename matches a glob pattern in the `.claspignore` file)
|
210 |
|
211 | #### Options
|
212 |
|
213 | - `-f` `--force`: Forcibly overwrites the remote manifest.
|
214 | - `-w` `--watch`: Watches local file changes. Pushes files every few seconds.
|
215 |
|
216 | #### Examples
|
217 |
|
218 | - `clasp push`
|
219 | - `clasp push -f`
|
220 | - `clasp push --watch`
|
221 |
|
222 | ### Status
|
223 |
|
224 | Lists files that will be written to the server on `push`.
|
225 |
|
226 | Ignores files:
|
227 |
|
228 | - That start with a `.`
|
229 | - That don't have an accepted file extension
|
230 | - That are ignored (filename matches a glob pattern in the ignore file)
|
231 |
|
232 | #### Options
|
233 |
|
234 | - `--json`: Show status in JSON form.
|
235 |
|
236 | #### Examples
|
237 |
|
238 | - `clasp status`
|
239 | - `clasp status --json`
|
240 |
|
241 | ### Open
|
242 |
|
243 | Opens the current directory's `clasp` project on script.google.com. Provide a `scriptId` to open a different script. Can also open web apps.
|
244 |
|
245 | #### Options
|
246 |
|
247 | - `[scriptId]`: The optional script project to open.
|
248 | - `--webapp`: open web application in a browser.
|
249 | - `--creds`: Open the URL to create credentials.
|
250 |
|
251 | #### Examples
|
252 |
|
253 | - `clasp open`
|
254 | - `clasp open "15ImUCpyi1Jsd8yF8Z6wey_7cw793CymWTLxOqwMka3P1CzE5hQun6qiC"`
|
255 | - `clasp open --webapp`
|
256 | - `clasp open --creds`
|
257 |
|
258 | ### Deployments
|
259 |
|
260 | List deployments of a script.
|
261 |
|
262 | #### Examples
|
263 |
|
264 | - `clasp deployments`
|
265 |
|
266 | ### Deploy
|
267 |
|
268 | Creates a version and deploys a script.
|
269 | The response gives the version of the deployment.
|
270 |
|
271 | #### Options
|
272 |
|
273 | - `-V <version>` `--versionNumber <version>`: The project version to deploy at.
|
274 | - `-d <description>` `--description <description>`: The deployment description.
|
275 | - `-i <id>` `--deploymentId <id>`: The deployment ID to redeploy.
|
276 |
|
277 | #### Examples
|
278 |
|
279 | - `clasp deploy` (create new deployment and new version)
|
280 | - `clasp deploy --versionNumber 4` (create new deployment)
|
281 | - `clasp deploy --description "Updates sidebar logo."` (deploy with description)
|
282 | - `clasp deploy --deploymentId 123` (create new version)
|
283 | - `clasp deploy -V 7 -d "Updates sidebar logo." -i 456`
|
284 |
|
285 | ### Undeploy
|
286 |
|
287 | Undeploys a deployment of a script.
|
288 |
|
289 | #### Options
|
290 |
|
291 | - `[deploymentId]`: An optional deployment ID.
|
292 | - `-a` `--all`: Undeploy all deployments.
|
293 |
|
294 | #### Examples
|
295 |
|
296 | - `clasp undeploy` (undeploy the last deployment.)
|
297 | - `clasp undeploy "123"`
|
298 | - `clasp undeploy --all`
|
299 |
|
300 | ### Version
|
301 |
|
302 | Creates an immutable version of the script.
|
303 |
|
304 | #### Options
|
305 |
|
306 | - `description`: description The description of the script version.
|
307 |
|
308 | #### Examples
|
309 |
|
310 | - `clasp version`
|
311 | - `clasp version "Bump the version."`
|
312 |
|
313 | ### Versions
|
314 |
|
315 | List versions of a script.
|
316 |
|
317 | #### Examples
|
318 |
|
319 | - `clasp versions`
|
320 |
|
321 | ### List
|
322 |
|
323 | Lists your most recent Apps Script projects.
|
324 |
|
325 | #### Examples
|
326 |
|
327 | - `clasp list`: Prints `helloworld1 β xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ...`
|
328 |
|
329 | ## Advanced Commands
|
330 |
|
331 | > **NOTE**: These commands require Project ID/credentials setup (see below).
|
332 |
|
333 | ### Logs
|
334 |
|
335 | Prints out most recent the _StackDriver logs_. These are logs from `console.log`, not `Logger.log`.
|
336 |
|
337 | #### Options
|
338 |
|
339 | - `--json`: Output logs in json format.
|
340 | - `--open`: Open StackDriver logs in a browser.
|
341 | - `--setup`: Setup StackDriver logs.
|
342 | - `--watch`: Retrieves the newest logs every 5 seconds.
|
343 | - `--simplified`: Removes timestamps from the logs.
|
344 |
|
345 | #### Examples
|
346 |
|
347 | ```text
|
348 | clasp logs
|
349 | ERROR Sat Apr 07 2019 10:58:31 GMT-0700 (PDT) myFunction my log error
|
350 | INFO Sat Apr 07 2019 10:58:31 GMT-0700 (PDT) myFunction info message
|
351 | ```
|
352 |
|
353 | - `clasp logs --json`
|
354 | - `clasp logs --open`
|
355 | - `clasp logs --watch`
|
356 | - `clasp logs --simplified`
|
357 |
|
358 | ### Run
|
359 |
|
360 | Remotely executes an Apps Script function.
|
361 |
|
362 | The complete step-by-step information on how to use `clasp run` is available here: [Run](/docs/run.md)
|
363 | Below is a short summary:
|
364 |
|
365 | 1. Log in with your credentials (`clasp login --creds creds.json`), see: [Run - Prerequisites](/docs/run.md#prerequisites)
|
366 | 1. Deploy the Script as an API executable (Easiest done via GUI at the moment).
|
367 | 1. Enable any APIs that are used by the script, see: [Run - Function with Scopes](/docs/run.md#run-a-function-that-requires-scopes)
|
368 | 1. Have the following in your `appsscript.json`. Be sure it's pushed:
|
369 |
|
370 | ```json
|
371 | "executionApi": {
|
372 | "access": "ANYONE"
|
373 | }
|
374 | ```
|
375 |
|
376 | #### Options
|
377 |
|
378 | - `<functionName>`: The name of the function in the script that you want to run.
|
379 | - `--nondev`: If true, runs the function in non-devMode.
|
380 | - `-p <paramString>` `--params <paramString>`: A JSON string array of parameters to pass to the function
|
381 |
|
382 | #### Examples
|
383 |
|
384 | - `clasp run 'sendEmail'`
|
385 | - `clasp run 'addOptions' -p '["string", 123, {"test": "for"}, true]'`
|
386 |
|
387 | ### List/Enable/Disable Google APIs
|
388 |
|
389 | List available APIs. Enables and disables Google APIs.
|
390 |
|
391 | #### List APIs
|
392 |
|
393 | Lists Google APIs that can be enabled as [Advanced Services](https://developers.google.com/apps-script/guides/services/advanced).
|
394 |
|
395 | - `clasp apis`
|
396 | - `clasp apis list`
|
397 |
|
398 | #### Enable/Disable APIs
|
399 |
|
400 | Enables or disables APIs with the Google Cloud project. These APIs are used via services like GmailApp and Advanced Services like BigQuery.
|
401 |
|
402 | The API name can be found using `clasp apis list`.
|
403 |
|
404 | - `clasp apis enable drive`
|
405 | - `clasp apis disable drive`
|
406 |
|
407 | #### Open APIs Console
|
408 |
|
409 | Open the Google Cloud Console where you can view and manage API access.
|
410 |
|
411 | - `clasp apis --open`
|
412 |
|
413 | ### Help
|
414 |
|
415 | Displays the help function.
|
416 |
|
417 | #### Examples
|
418 |
|
419 | - `clasp`
|
420 | - `clasp help`
|
421 |
|
422 | ### Setting
|
423 |
|
424 | Update `.clasp.json` settings file.
|
425 |
|
426 | If `settingKey` is omitted it prints the current settings.
|
427 | If `newValue` is omitted it returns the current setting value.
|
428 |
|
429 | #### Options
|
430 |
|
431 | - `settingKey`: settingKey They key in `.clasp.json` you want to change
|
432 | - `newValue`: newValue The new value for the setting
|
433 |
|
434 | #### Examples
|
435 |
|
436 | - `clasp setting`
|
437 | - `clasp setting scriptId`
|
438 | - `clasp setting scriptId new-id`
|
439 |
|
440 | ## Guides
|
441 |
|
442 | ### Ignore File (`.claspignore`)
|
443 |
|
444 | Like `.gitignore`, `.claspignore` allows you to ignore files that you do not wish to not upload on `clasp push`. Steps:
|
445 |
|
446 | 1. Create a file called `.claspignore` in your project's root directory.
|
447 | 1. Add patterns to be excluded from `clasp push`. _Note_: The `.claspignore` patterns are applied by [multimatch](https://github.com/sindresorhus/multimatch), which is different from `.gitignore`, especially for directories. To ignore a directory, use syntax like `**/node_modules/**`.
|
448 |
|
449 | A sample `.claspignore` ignoring everything except the manifest and `build/main.js`:
|
450 |
|
451 | ```text
|
452 | **/**
|
453 | !build/main.js
|
454 | !appsscript.json
|
455 | ```
|
456 |
|
457 | _Note_: The `.claspignore` patterns are applied relative from the `rootDir`.
|
458 |
|
459 | If no `.claspignore` is specified, a default set of patterns is applied. This default set will only consider the `appsscript.json` manifest and any JavaScript, TypeScript and `.html` source files within the `rootDir` folder. Child folders other than `.git` and `node_modules` are processed.
|
460 |
|
461 | ```text
|
462 | # ignore all files...
|
463 | **/**
|
464 |
|
465 | # except the extensions...
|
466 | !appsscript.json
|
467 | !**/*.gs
|
468 | !**/*.js
|
469 | !**/*.ts
|
470 | !**/*.html
|
471 |
|
472 | # ignore even valid files if in...
|
473 | .git/**
|
474 | node_modules/**
|
475 | ```
|
476 |
|
477 | ## Project Settings File (`.clasp.json`)
|
478 |
|
479 | When running `clone` or `create`, a file named `.clasp.json` is created in the current directory to describe `clasp`'s configuration for the current project. Example `.clasp.json`:
|
480 |
|
481 | ```json
|
482 | {
|
483 | "scriptId": "",
|
484 | "rootDir": "build/",
|
485 | "projectId": "project-id-xxxxxxxxxxxxxxxxxxx",
|
486 | "fileExtension": "ts",
|
487 | "filePushOrder": ["file1.ts", "file2.ts"]
|
488 | }
|
489 | ```
|
490 |
|
491 | The following configuration values can be used:
|
492 |
|
493 | ### `scriptId` (required)
|
494 |
|
495 | Specifies the id of the Google Script project that clasp will target. It is the part located inbetween `/d/` and `/edit` in your project's URL: `https://script.google.com/d/<SCRIPT_ID>/edit`.
|
496 |
|
497 | ### `rootDir` (optional)
|
498 |
|
499 | Specifies the **local** directory in which clasp will store your project files. If not specified, clasp will default to the current directory.
|
500 |
|
501 | ### `projectId` (optional)
|
502 |
|
503 | Specifies the id of the Google Cloud Platform project that clasp will target.
|
504 | You must [associate Google Script project with Google Cloud Platform](https://github.com/google/clasp/blob/master/docs/run.md#setup-instructions) beforehand.
|
505 |
|
506 | 1. Run `clasp open`.
|
507 | 1. Click `Resources > Cloud Platform project...`.
|
508 | 1. Specify the project ID `project-id-xxxxxxxxxxxxxxxxxxx`.
|
509 |
|
510 | Even if you do not set this manually, clasp will ask this via a prompt to you at the required time.
|
511 |
|
512 | ### `fileExtension` (optional)
|
513 |
|
514 | Specifies the file extension for **local** script files in your Apps Script project.
|
515 |
|
516 | ### `filePushOrder` (optional)
|
517 |
|
518 | Specifies the files that should be pushed first, useful for scripts that rely on order of execution. All other files are pushed after this list of files.
|
519 |
|
520 | ## Troubleshooting
|
521 |
|
522 | ### Node Version
|
523 |
|
524 | The library requires **Node version >= 8.2.1**. Use this script to check your version and **upgrade Node if necessary**:
|
525 |
|
526 | ```sh
|
527 | node -v # Check Node version
|
528 | npm install -g npm # Update npm and npx
|
529 | npx n latest # use the n package to update node
|
530 | ```
|
531 |
|
532 | ### Using a Proxy
|
533 |
|
534 | Clasp supports proxies via the Google APIs Node Module.
|
535 | See ["Using a Proxy"](https://github.com/googleapis/google-api-nodejs-client#using-a-proxy) and [this discussion](https://github.com/google/clasp/issues/8#issuecomment-427560737) for details on how to use the proxy.
|
536 | This requires using the environment variables `HTTP_PROXY` / `HTTPS_PROXY`.
|
537 |
|
538 | ## README Badge
|
539 |
|
540 | Using clasp for your project? Add a README badge to show it off: [](https://github.com/google/clasp)
|
541 |
|
542 | ```md
|
543 | [](https://github.com/google/clasp)
|
544 | ```
|
545 |
|
546 | ## Develop clasp
|
547 |
|
548 | See [the develop guide](docs/develop.md) for instructions on how to build `clasp`. It's not that hard!
|
549 |
|
550 | ## Contributing
|
551 |
|
552 | The main purpose of this tool is to enable local Apps Script development.
|
553 | If you have a core feature or use-case you'd like to see, find a GitHub issue or
|
554 | create a detailed proposal of the use-case.
|
555 | PRs are very welcome! See the [issues](https://github.com/google/clasp/issues) (especially **good first issue** and **help wanted**).
|
556 |
|
557 | ### How to Submit a Pull Request
|
558 |
|
559 | 1. Look over the test cases in `tests/test.ts`, try cases that the PR may affect.
|
560 | 1. Run [tslint](https://palantir.github.io/tslint/): `npm run lint`.
|
561 | 1. Submit a pull request after testing your feature to make sure it works.
|
562 |
|
563 | β‘ Powered by the [Apps Script API](https://developers.google.com/apps-script/api/).
|