1 | # Microsoft Graph JavaScript Client Library
|
2 |
|
3 | [](https://www.npmjs.com/package/@microsoft/microsoft-graph-client) [](https://snyk.io/test/github/microsoftgraph/msgraph-sdk-javascript) [](https://github.com/microsoftgraph/msgraph-sdk-javascript) [](https://github.com/microsoftgraph/msgraph-sdk-javascript) [](https://www.npmjs.com/package/@microsoft/microsoft-graph-client)
|
4 |
|
5 | The Microsoft Graph JavaScript client library is a lightweight wrapper around the Microsoft Graph API that can be used server-side and in the browser.
|
6 |
|
7 | - [Microsoft Graph JavaScript Client Library](#microsoft-graph-javascript-client-library)
|
8 | - [Installation](#installation)
|
9 | - [Via npm](#via-npm)
|
10 | - [Via Script Tag](#via-script-tag)
|
11 | - [Getting started](#getting-started)
|
12 | - [1. Register your application](#1-register-your-application)
|
13 | - [2. Create a Client Instance](#2-create-a-client-instance)
|
14 | - [3. Make requests to the graph](#3-make-requests-to-the-graph)
|
15 | - Documentation
|
16 | - [HTTP Actions](docs/Actions.md)
|
17 | - [Chained APIs to call Microsoft Graph](docs/CallingPattern.md)
|
18 | - [OData system query options - Query Parameters](docs/QueryParameters.md)
|
19 | - [Batch multiple requests into single HTTP request](docs/content/Batching.md)
|
20 | - [Cancel a HTTP request](docs/CancellingAHTTPRequest.md)
|
21 | - [Configurations to your request](docs/OtherAPIs.md)
|
22 | - [Query](docs/OtherAPIs.md#QUERY)
|
23 | - [Version](docs/OtherAPIs.md#VERSION)
|
24 | - [Headers](docs/OtherAPIs.md#HEADER-AND-HEADERS)
|
25 | - [Options](docs/OtherAPIs.md#OPTION-AND-OPTIONS)
|
26 | - [MiddlewareOptions](docs/OtherAPIs.md#MIDDLEWAREOPTIONS)
|
27 | - [ResponseType](docs/OtherAPIs.md#RESPONSETYPE)
|
28 | - [Upload large files to OneDrive, Outlook, Print API](docs/tasks/LargeFileUploadTask.md)
|
29 | - [Page Iteration](docs/tasks/PageIterator.md)
|
30 | - [Getting Raw Response](docs/GettingRawResponse.md)
|
31 | - [Creating an instance of TokenCredentialAuthenticationProvider](docs/TokenCredentialAuthenticationProvider.md)
|
32 | - [Samples and tutorials](#samples-and-tutorials)
|
33 | - Step-by-step training exercises on creating a basic application using the Microsoft Graph JavaScript SDK:
|
34 | - [Build Angular single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/angular)
|
35 | - [Build Node.js Express apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/node)
|
36 | - [Build React Native apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/react-native)
|
37 | - [Build React single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/react)
|
38 | - [Build JavaScript single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/javascript)
|
39 | - [Explore Microsoft Graph scenarios for JavaScript development](https://docs.microsoft.com/learn/paths/m365-msgraph-scenarios/)
|
40 | - Samples using `TokenCredentialAuthenticationProvider` with the `@azure/identity` library:
|
41 | - [TokenCredentialAuthenticationProvider Samples](samples/)
|
42 | - Samples using `LargeFileUploadTask` and `OneDriveLargeFileTask`:
|
43 | - [LargeFileUploadTask Samples](samples/)
|
44 | - Samples to learn more about authentication using `MSAL`libraries:
|
45 | - [Azure-Sample Vanilla JS SPA using MSAL Browser and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
46 | - [ Azure-Sample Angular SPA using MSAL Angular and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-angular-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
47 | - [ Azure-Sample React SPA using MSAL React and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-react-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
48 | - [Questions and comments](#questions-and-comments)
|
49 | - [Contributing](#contributing)
|
50 | - [Additional resources](#additional-resources)
|
51 | - [Third Party Notices](#third-party-notices)
|
52 | - [Security Reporting](#security-reporting)
|
53 | - [License](#license)
|
54 | - [We Value and Adhere to the Microsoft Open Source Code of Conduct](#we-value-and-adhere-to-the-microsoft-open-source-code-of-conduct)
|
55 |
|
56 | **Looking for IntelliSense on models (Users, Groups, etc.)? Check out the Microsoft Graph Types [v1.0](https://github.com/microsoftgraph/msgraph-typescript-typings) and [beta](https://github.com/microsoftgraph/msgraph-beta-typescript-typings)!!**
|
57 |
|
58 | [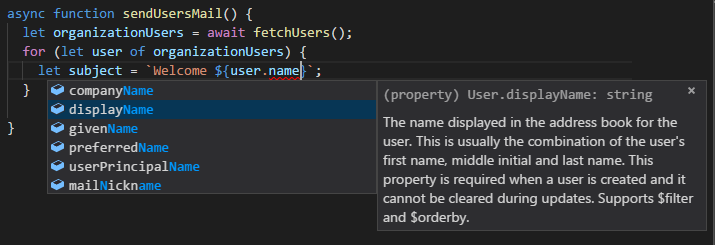](https://github.com/microsoftgraph/msgraph-typescript-typings)
|
59 |
|
60 | ## Node version requirement
|
61 |
|
62 | Node.js 12 LTS or higher. The active Long Term Service (LTS) version of Node.js is used for on-going testing of existing and upcoming product features.
|
63 |
|
64 | For Node.js 18 users, it is recommended to disable the experimental `fetch` feature by supplying the `--no-experimental-fetch` command-line flag while using the Microsoft Graph JavaScript client library.
|
65 |
|
66 | ## Installation
|
67 |
|
68 | ### Via npm
|
69 |
|
70 | ```cmd
|
71 | npm install @microsoft/microsoft-graph-client
|
72 | ```
|
73 |
|
74 | import `@microsoft/microsoft-graph-client` into your module.
|
75 |
|
76 | Also, you will need to import any fetch polyfill which suits your requirements. Following are some fetch polyfills -
|
77 |
|
78 | - [isomorphic-fetch](https://www.npmjs.com/package/isomorphic-fetch).
|
79 | - [cross-fetch](https://www.npmjs.com/package/cross-fetch)
|
80 | - [whatwg-fetch](https://www.npmjs.com/package/whatwg-fetch)
|
81 |
|
82 | ```typescript
|
83 | import "isomorphic-fetch"; // or import the fetch polyfill you installed
|
84 | import { Client } from "@microsoft/microsoft-graph-client";
|
85 | ```
|
86 |
|
87 | ### Via Script Tag
|
88 |
|
89 | Include [graph-js-sdk.js](https://cdn.jsdelivr.net/npm/@microsoft/microsoft-graph-client/lib/graph-js-sdk.js) in your HTML page.
|
90 |
|
91 | ```HTML
|
92 | <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/@microsoft/microsoft-graph-client/lib/graph-js-sdk.js"></script>
|
93 | ```
|
94 |
|
95 | In case your browser doesn't have support for [Fetch](https://developer.mozilla.org/docs/Web/API/Fetch_API) [[support](https://developer.mozilla.org/docs/Web/API/Fetch_API#Browser_compatibility)] or [Promise](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise) [[support](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise#Browser_compatibility)], you need to use polyfills like [github/fetch](https://github.com/github/fetch) for fetch and [es6-promise](https://github.com/stefanpenner/es6-promise) for promise.
|
96 |
|
97 | ```HTML
|
98 | <!-- polyfilling promise -->
|
99 | <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/es6-promise/dist/es6-promise.auto.min.js"></script>
|
100 |
|
101 | <!-- polyfilling fetch -->
|
102 | <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/whatwg-fetch/dist/fetch.umd.min.js"></script>
|
103 |
|
104 | <!-- depending on your browser you might wanna include babel polyfill -->
|
105 | <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/@babel/polyfill@7.4.4/dist/polyfill.min.js"></script>
|
106 | ```
|
107 |
|
108 | ## Getting started
|
109 |
|
110 | ### 1. Register your application
|
111 |
|
112 | To call Microsoft Graph, your app must acquire an access token from the Microsoft identity platform. Learn more about this -
|
113 |
|
114 | - [Authentication and authorization basics for Microsoft Graph](https://docs.microsoft.com/graph/auth/auth-concepts)
|
115 | - [Register your app with the Microsoft identity platform](https://docs.microsoft.com/graph/auth-register-app-v2)
|
116 |
|
117 | ### 2. Create a Client Instance
|
118 |
|
119 | The Microsoft Graph client is designed to make it simple to make calls to Microsoft Graph. You can use a single client instance for the lifetime of the application.
|
120 |
|
121 | For information on how to create a client instance, see [Creating Client Instance](./docs/CreatingClientInstance.md)
|
122 |
|
123 | ### 3. Make requests to the graph
|
124 |
|
125 | Once you have authentication setup and an instance of Client, you can begin to make calls to the service. All requests should start with `client.api(path)` and end with an [action](./docs/Actions.md).
|
126 |
|
127 | Example of getting user details:
|
128 |
|
129 | ```typescript
|
130 | try {
|
131 | let userDetails = await client.api("/me").get();
|
132 | console.log(userDetails);
|
133 | } catch (error) {
|
134 | throw error;
|
135 | }
|
136 | ```
|
137 |
|
138 | Example of sending an email to the recipients:
|
139 |
|
140 | ```typescript
|
141 | // Construct email object
|
142 | const mail = {
|
143 | subject: "Microsoft Graph JavaScript Sample",
|
144 | toRecipients: [
|
145 | {
|
146 | emailAddress: {
|
147 | address: "example@example.com",
|
148 | },
|
149 | },
|
150 | ],
|
151 | body: {
|
152 | content: "<h1>MicrosoftGraph JavaScript Sample</h1>Check out https://github.com/microsoftgraph/msgraph-sdk-javascript",
|
153 | contentType: "html",
|
154 | },
|
155 | };
|
156 | try {
|
157 | let response = await client.api("/me/sendMail").post({ message: mail });
|
158 | console.log(response);
|
159 | } catch (error) {
|
160 | throw error;
|
161 | }
|
162 | ```
|
163 |
|
164 | For more information, refer: [Calling Pattern](docs/CallingPattern.md), [Actions](docs/Actions.md), [Query Params](docs/QueryParameters.md), [API Methods](docs/OtherAPIs.md) and [more](docs/).
|
165 |
|
166 | ## Samples and tutorials
|
167 |
|
168 | Step-by-step training exercises that guide you through creating a basic application that accesses data via the Microsoft Graph:
|
169 |
|
170 | - [Build Angular single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/angular)
|
171 | - [Build Node.js Express apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/node)
|
172 | - [Build React Native apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/react-native)
|
173 | - [Build React single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/react)
|
174 | - [Build JavaScript single-page apps with Microsoft Graph](https://docs.microsoft.com/graph/tutorials/javascript)
|
175 | - [Explore Microsoft Graph scenarios for JavaScript development](https://docs.microsoft.com/learn/paths/m365-msgraph-scenarios/)
|
176 |
|
177 | The Microsoft Graph JavaScript SDK provides a `TokenCredentialAuthenticationProvider` to authenticate using the `@azure/identity` auth library. Learn more:
|
178 |
|
179 | - [Documentation for creating an instance of TokenCredentialAuthenticationProvider](docs/TokenCredentialAuthenticationProvider.md)
|
180 | - [TokenCredentialAuthenticationProvider Samples](samples/)
|
181 |
|
182 | The Microsoft Graph JavaScript SDK provides a `LargeFileUploadTask` to upload large files to OneDrive, Outlook and Print API:
|
183 |
|
184 | - [LargeFileUploadTask documentation](docs/tasks/LargeFileUploadTask.md)
|
185 | - [Samples using `LargeFileUploadTask` and `OneDriveLargeFileTask`](samples/) The following `MSAL` samples provide information on authentication using `MSAL` libraries and how to use the Microsoft Graph JavaScript SDK client with MSAL as a custom authentication provider to query the Graph API:
|
186 |
|
187 | - [Azure-Sample Vanilla JS SPA using MSAL Browser and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
188 | - [Azure-Sample Angular SPA using MSAL Angular and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-angular-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
189 | - [Azure-Sample React SPA using MSAL React and Microsoft Graph JavaScript SDK](https://github.com/Azure-Samples/ms-identity-javascript-react-tutorial/tree/main/2-Authorization-I/1-call-graph)
|
190 |
|
191 | ## Questions and comments
|
192 |
|
193 | We'd love to get your feedback about the Microsoft Graph JavaScript client library. You can send your questions and suggestions to us in the [Issues](https://github.com/microsoftgraph/msgraph-sdk-javascript/issues) section of this repository.
|
194 |
|
195 | ## Contributing
|
196 |
|
197 | Please see the [contributing guidelines](CONTRIBUTING.md).
|
198 |
|
199 | ## Additional resources
|
200 |
|
201 | - [Microsoft Graph website](https://graph.microsoft.io)
|
202 | - The Microsoft Graph TypeScript definitions enable editors to provide intellisense on Microsoft Graph objects including users, messages, and groups.
|
203 | - [@microsoft/microsoft-graph-types](https://www.npmjs.com/package/@microsoft/microsoft-graph-types) or [@types/microsoft-graph](https://www.npmjs.com/package/@types/microsoft-graph)
|
204 | - [@microsoft/microsoft-graph-types-beta](https://www.npmjs.com/package/@microsoft/microsoft-graph-types-beta)
|
205 | - [Microsoft Graph Toolkit: UI Components and Authentication Providers for Microsoft Graph](https://docs.microsoft.com/graph/toolkit/overview)
|
206 | - [Office Dev Center](http://dev.office.com/)
|
207 |
|
208 | ## Third Party Notices
|
209 |
|
210 | See [Third Party Notices](./THIRD%20PARTY%20NOTICES) for information on the packages that are included in the [package.json](./package.json)
|
211 |
|
212 | ## Security Reporting
|
213 |
|
214 | If you find a security issue with our libraries or services please report it to [secure@microsoft.com](mailto:secure@microsoft.com) with as much detail as possible. Your submission may be eligible for a bounty through the [Microsoft Bounty](http://aka.ms/bugbounty) program. Please do not post security issues to GitHub Issues or any other public site. We will contact you shortly upon receiving the information. We encourage you to get notifications of when security incidents occur by visiting [this page](https://technet.microsoft.com/security/dd252948) and subscribing to Security Advisory Alerts.
|
215 |
|
216 | ## License
|
217 |
|
218 | Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License (the "[License](./LICENSE)");
|
219 |
|
220 | ## We Value and Adhere to the Microsoft Open Source Code of Conduct
|
221 |
|
222 | This project has adopted the [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/). For more information see the [Code of Conduct FAQ](https://opensource.microsoft.com/codeofconduct/faq/) or contact [opencode@microsoft.com](mailto:opencode@microsoft.com) with any additional questions or comments.
|