1 | 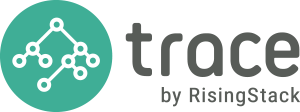
|
2 |
|
3 | [](https://circleci.com/gh/RisingStack/trace-nodejs)
|
4 | [](https://www.npmjs.com/package/@risingstack/trace)
|
5 | [](https://trace.risingstack.com)
|
6 | [](https://trace-slack.risingstack.com)
|
7 | [](http://standardjs.com/)
|
8 | [](https://twitter.com/TraceAPM)
|
9 | ***
|
10 | [App](https://trace.risingstack.com/app) | [Documentation](https://trace-docs.risingstack.com/) | [Status page](https://trace-status.risingstack.com/) | [Case study](https://blog.risingstack.com/case-study-node-js-memory-leak-in-ghost/)
|
11 | ***
|
12 |
|
13 | ## ⚠️ Breaking change
|
14 |
|
15 | With version 3.x.x we have dropped support for Node v0.10. This means that
|
16 | future releases under this major version might contain code changes that are
|
17 | incompatible with Node.js v0.10 to an extent of crashing your application.
|
18 | Please consider updating to a newer runtime, especially that the
|
19 | [maintenance of v0.10 has already ended](node-lts). See our compatibility table
|
20 | below.
|
21 |
|
22 | Also, since 3.1.0 we switched to a different API backend for collecting traces
|
23 | which is incompatible with the old one. The old endpoint is still supported, but
|
24 | we would like it to be phased out eventually. Please update your agents to
|
25 | 3.1.0 or newer.
|
26 |
|
27 | ## Installation and usage
|
28 |
|
29 | As Trace uses scoped packages, be sure to use npm version greater than 2.7.0.
|
30 |
|
31 | ```
|
32 | npm install --save @risingstack/trace
|
33 | ```
|
34 |
|
35 | *If you can't update to npm@2.7.0 for whatever reason, you can still install Trace using `npm i risingstack/trace-nodejs`.*
|
36 |
|
37 | After you installed Trace as a dependency, you just require it at the beginning of your main file.
|
38 | ```javascript
|
39 | var trace = require('@risingstack/trace');
|
40 | ```
|
41 |
|
42 | ### Configuration
|
43 |
|
44 | You can specify the configuration two ways. Configuration options can be set via environment variables or using a config module. We look for a config module named `trace.config.js` at your current working directory by default, which can be overridden with the `TRACE_CONFIG_PATH` environment variable. Having a config module is optional, but some options may be set only with it. In order to use our service, you need to specify an api key and and a service name at minimum. The corresponding environment variables are: `TRACE_API_KEY` and `TRACE_SERVICE_NAME`.
|
45 |
|
46 | An example for how to start your app with environment variables:
|
47 |
|
48 | ```
|
49 | node TRACE_SERVICE_NAME=MyApp TRACE_API_KEY=1 index.js
|
50 | ```
|
51 |
|
52 | An example with a custom config file using the Trace servers:
|
53 |
|
54 | ```
|
55 | node TRACE_CONFIG_PATH=/path/to/my/config.js index.js
|
56 | ```
|
57 |
|
58 | or simply
|
59 |
|
60 |
|
61 | ```
|
62 | node index.js
|
63 | ```
|
64 |
|
65 | if it's in the current working directory.
|
66 |
|
67 | ```javascript
|
68 | /**
|
69 | * Your Trace configuration file at /path/to/my/config.js
|
70 | */
|
71 |
|
72 | module.exports = {
|
73 | serviceName: 'your-awesome-app',
|
74 | apiKey: 'KEEP_ME_SECRET',
|
75 | ignoreHeaders: {
|
76 | 'user-agent': ['007']
|
77 | },
|
78 | ignorePaths: [
|
79 | '/healthcheck'
|
80 | ],
|
81 | ignoreStatusCodes: [
|
82 | 401,
|
83 | 404
|
84 | ],
|
85 | ignoreOutgoingHosts: [
|
86 | 'google.com'
|
87 | ],
|
88 | disableInstrumentations: [
|
89 | 'mongodb'
|
90 | ],
|
91 | proxy: 'http://168.63.76.32:3128',
|
92 | keepQueryParams: true
|
93 | }
|
94 | ```
|
95 |
|
96 | For the complete set of configuration options, visit the [docs](https://trace-docs.risingstack.com/docs/advanced-usage#section-available-options).
|
97 |
|
98 | *Note: Custom reporters are no longer supported in trace 2.x*
|
99 |
|
100 | *Note: If you are running your app with NODE_ENV=test, Trace won't start*
|
101 |
|
102 | ## API
|
103 |
|
104 | ### trace.report(String, [Object])
|
105 |
|
106 | This method can be use to report additional data to the Trace servers which later on helps with debugging.
|
107 |
|
108 | ```javascript
|
109 | trace.report('name', {
|
110 | userId: 10
|
111 | });
|
112 | ```
|
113 |
|
114 | Throws an error if first parameter is not a String.
|
115 | Throws an error if second parameter is not an Object.
|
116 |
|
117 | ### trace.reportError(String, Error)
|
118 |
|
119 | This method can be used to send errors to the Trace servers - note that transactions that use
|
120 | this method are not subject to sampling, so it will be collected all the time.
|
121 |
|
122 | ```javascript
|
123 | trace.reportError('mysql_error', new Error('connection refused'));
|
124 | ```
|
125 |
|
126 | Throws an error if first parameter is not a String.
|
127 |
|
128 | ### trace.getTransactionId()
|
129 |
|
130 | This method can be use to get the current transactionId. It can be useful if you want to integrate trace with your
|
131 | current logging systems.
|
132 |
|
133 | ```javascript
|
134 | var transactionId = trace.getTransactionId();
|
135 | ```
|
136 |
|
137 | ### trace.recordMetric(name, value)
|
138 |
|
139 | This method can be used to record custom metrics values.
|
140 |
|
141 | ```javascript
|
142 | trace.recordMetric('user/imageUpload', 6)
|
143 | ```
|
144 |
|
145 | The name must have the following format: `<Category>/<Name>`
|
146 | The value must be a number.
|
147 |
|
148 | ### trace.incrementMetric(name, [amount])
|
149 |
|
150 | This method can be used to record increment-only type of metrics.
|
151 |
|
152 | ```javascript
|
153 | trace.incrementMetric('user/signup')
|
154 | ```
|
155 |
|
156 | The name must have the following format: `<Category>/<Name>`
|
157 |
|
158 | ### trace.stop()
|
159 |
|
160 | This method gracefully stops trace.
|
161 |
|
162 | ```javascript
|
163 | trace.stop(cb)
|
164 | ```
|
165 | Accepts a node-style callback to be called when trace stopped.
|
166 |
|
167 | Note: There is no way to restart trace after calling this method. You should end your process after calling this
|
168 | method.
|
169 |
|
170 | ## Troubleshooting
|
171 |
|
172 | ### Debug logs
|
173 | If you have problems using Trace, e.g it doesn't seem to report anything you can
|
174 | turn on logging. We use the lightweight
|
175 | [debug](https://github.com/visionmedia/debug). If you are not familiar with it
|
176 | yet, please read its documentation to learn how it works.
|
177 |
|
178 |
|
179 | #### Quickstart
|
180 | To turn it only for Trace start your app with the `DEBUG`
|
181 | environment variable set to `risingstack/trace*`.
|
182 |
|
183 | ```
|
184 | DEBUG=risingstack/trace* node my_app.js
|
185 | ```
|
186 |
|
187 | #### Configure logging
|
188 |
|
189 | To make it possible to filter severities and components in Trace we use
|
190 | subnamespaces. The namespace will start with `trace/risingstack` then a `:` then
|
191 | a mandatory severity specifier,
|
192 | - `error`,
|
193 | - `warn` or
|
194 | - `info`.
|
195 |
|
196 | Then come zero or more namespaces led by colons. The namespaces are
|
197 | hierarchically organized according to components inside of Trace.
|
198 |
|
199 | Currently these namespaces (and their subnamespaces) are used:
|
200 |
|
201 | - `config`
|
202 | - `instrumentation`
|
203 | - `agent`
|
204 | - `agent:tracer`
|
205 | - `agent:metrics`
|
206 | - `agent:profiler`
|
207 | - `agent:security`
|
208 | - `api`
|
209 |
|
210 | As they can have subnamespaces, always append an `*` to them to get all
|
211 | messages.
|
212 |
|
213 | Examples:
|
214 |
|
215 | - get all error messages: `DEBUG=risingstack/trace:error*`
|
216 |
|
217 | - get all messages from agents: `DEBUG=risingstack/trace:*:agent*`
|
218 |
|
219 | - get all error messages and all messages from agents: `DEBUG=risingstack/trace:error*,risingstack/trace:*:agent*`
|
220 |
|
221 |
|
222 | ## Compatibility with Node versions
|
223 |
|
224 | * node v0.12@latest
|
225 | * node v4@latest
|
226 | * node v5@latest
|
227 | * node v6@latest
|
228 | * node v7@latest
|
229 |
|
230 | ## Migrating from previous versions
|
231 |
|
232 | ### Versions below 2.x
|
233 |
|
234 | The `trace.config.js` file changed, and has the following format:
|
235 |
|
236 | ```
|
237 | module.exports = {
|
238 | serviceName: 'your-awesome-app',
|
239 | apiKey: 'KEEP_ME_SECRET'
|
240 | }
|
241 | ```
|
242 |
|
243 | Also, from `2.x` you can specify these values using only environment variables: `TRACE_SERVICE_NAME` and `TRACE_API_KEY`.
|
244 |
|
245 | ### Versions below 3.x
|
246 |
|
247 | We dropped support for Node v0.10. [Update your runtime](node) to a more recent version to continue using Trace.
|
248 |
|
249 | [node]: https://nodejs.org/en/
|
250 | [node-lts]: https://github.com/nodejs/LTS#lts-schedule
|