1 | # Angular Froala WYSIWYG Editor - [Demo](https://www.froala.com/wysiwyg-editor)
|
2 |
|
3 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
4 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
5 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
6 |
|
7 | >Angular 2, Angular 4, Angular 5, Angular 6 and Angular 7 bindings for Froala WYSIWYG Editor.
|
8 |
|
9 | 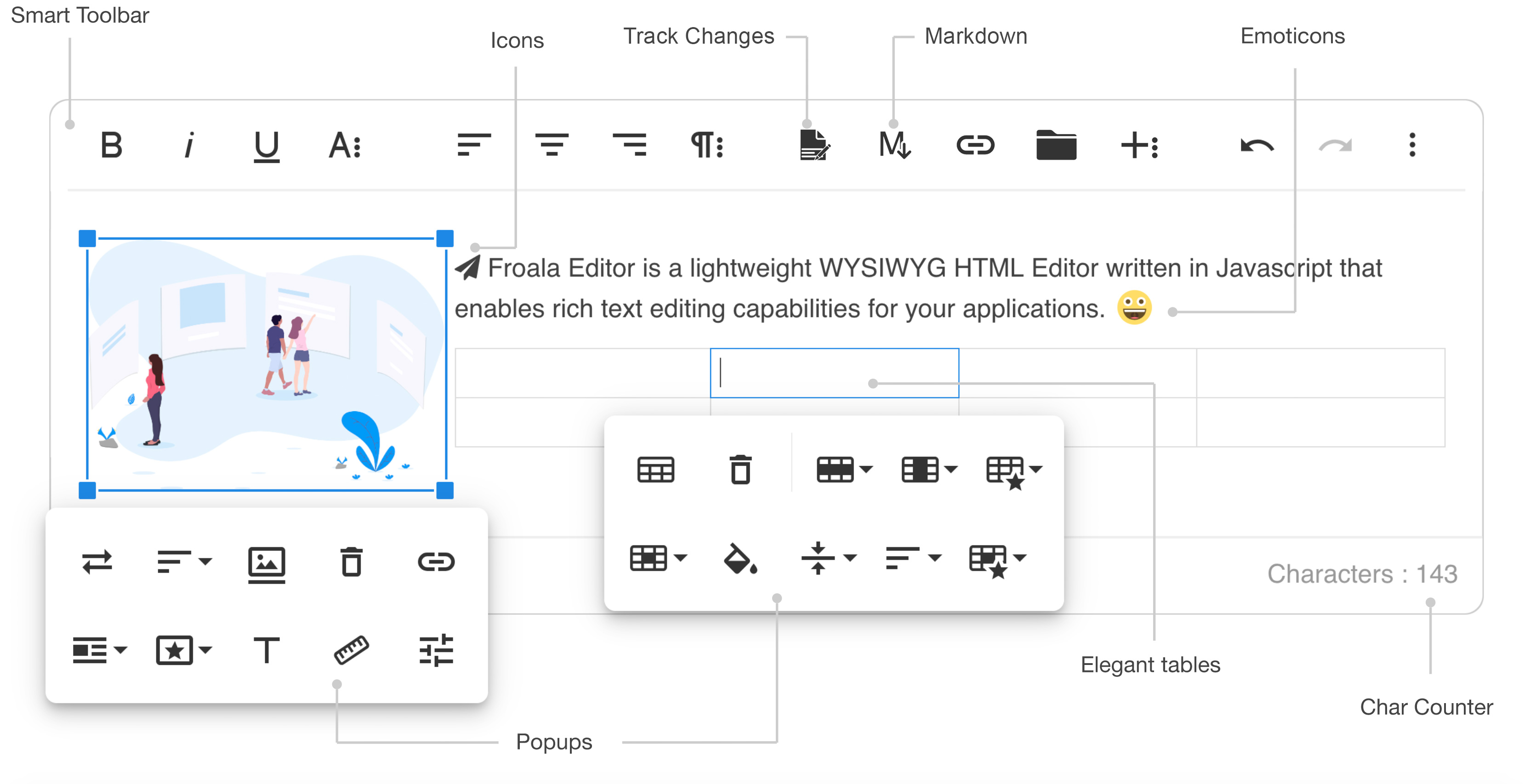
|
10 |
|
11 | ## Table of contents
|
12 | 1. [Installation instructions](#installation-instructions)
|
13 | 2. [Update editor instructions](#update-editor-instructions)
|
14 | 3. [Integration](#integration)
|
15 | - [angular-cli](#use-with-angular-cli)
|
16 | - [ionic v2 or v3](#use-with-ionic-v2-or-v3)
|
17 | - [webpack](#use-with-webpack)
|
18 | - [angular-starter](#use-with-webpack)
|
19 | - [angular-seed](#use-with-angular-seed)
|
20 | - [System.js and JIT](#use-with-systemjs-and-jit)
|
21 | - [AOT](#use-with-aot)
|
22 | 4. [Usage](#usage)
|
23 | 5. [Manual Initialization](#manual-initialization)
|
24 | 6. [Displaying HTML](#displaying-html)
|
25 | 7. [License](#license)
|
26 | 8. [Development environment setup](#development-environment-setup)
|
27 |
|
28 | ## Installation instructions
|
29 |
|
30 | Install `angular-froala-wysiwyg` from `npm`
|
31 |
|
32 | ```bash
|
33 | npm install angular-froala-wysiwyg
|
34 | ```
|
35 |
|
36 | You will need CSS styles and Font Awesome
|
37 |
|
38 | ```html
|
39 | <!-- index.html -->
|
40 | <link href="node_modules/froala-editor/css/froala_editor.pkgd.min.css" rel="stylesheet">
|
41 | <link href="node_modules/font-awesome/css/font-awesome.min.css" rel="stylesheet">
|
42 | ```
|
43 |
|
44 | ## Update editor instructions
|
45 |
|
46 | ```bash
|
47 | npm update froala-editor --save
|
48 | ```
|
49 |
|
50 | ## Integration
|
51 |
|
52 | ### Use with Angular CLI
|
53 |
|
54 | #### Installing @angular/cli
|
55 |
|
56 | *Note*: you can skip this part if you already have application generated.
|
57 |
|
58 | ```bash
|
59 | npm install -g @angular/cli
|
60 | ng new my-app
|
61 | cd my-app
|
62 | ```
|
63 |
|
64 | #### Add angular-froala-wysiwyg
|
65 |
|
66 | - install `angular-froala-wysiwyg`
|
67 |
|
68 | ```bash
|
69 | npm install angular-froala-wysiwyg --save
|
70 | ```
|
71 |
|
72 | - open `src/app/app.module.ts` and add
|
73 |
|
74 | ```typescript
|
75 | // Import Angular plugin.
|
76 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
77 | ...
|
78 |
|
79 | @NgModule({
|
80 | ...
|
81 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
|
82 | ...
|
83 | })
|
84 | ```
|
85 |
|
86 | - open `angular.json` file and insert a new entry into the `styles` array
|
87 |
|
88 | ```json
|
89 | "styles": [
|
90 | "styles.css",
|
91 | "./node_modules/froala-editor/css/froala_editor.pkgd.min.css",
|
92 | "./node_modules/froala-editor/css/froala_style.min.css",
|
93 | "./node_modules/font-awesome/css/font-awesome.css"
|
94 | ]
|
95 | ```
|
96 |
|
97 | - in `angular.json` file insert a new entry into the `scripts` array
|
98 |
|
99 | ```json
|
100 | "scripts": [
|
101 | "./node_modules/jquery/dist/jquery.min.js",
|
102 | "./node_modules/froala-editor/js/froala_editor.pkgd.min.js"
|
103 | ]
|
104 | ```
|
105 |
|
106 | - open `src/app/app.component.html` and add
|
107 |
|
108 | ```html
|
109 | <div [froalaEditor]>Hello, Froala!</div>
|
110 | ```
|
111 |
|
112 | #### Run angular-cli
|
113 | ```bash
|
114 | ng serve
|
115 | ```
|
116 |
|
117 |
|
118 |
|
119 | ### Use with `ionic v2 or v3`
|
120 |
|
121 | #### Create Ionic app
|
122 |
|
123 | *Note*: you can skip this part if you already have application generated.
|
124 |
|
125 | ```bash
|
126 | npm install -g cordova ionic
|
127 | ionic start myApp blank
|
128 | cd myApp
|
129 | ```
|
130 |
|
131 |
|
132 |
|
133 | #### Add angular-froala-wysiwyg
|
134 |
|
135 | For v3 make sure that you use the latest version of ionic and also the latest version of angular.
|
136 |
|
137 | Installing Froala Wysiwyg Editor in Ionic is fairly easy, it can be done using npm:
|
138 | ```bash
|
139 | npm install angular-froala-wysiwyg --save
|
140 | ```
|
141 | - open `src/app/app.module.ts` and add
|
142 |
|
143 | ```typescript
|
144 | // Import Froala Editor.
|
145 | import "froala-editor/js/froala_editor.pkgd.min.js";
|
146 |
|
147 | // Import Angular2 plugin.
|
148 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
149 | ...
|
150 |
|
151 | @NgModule({
|
152 | ...
|
153 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
|
154 | ...
|
155 | })
|
156 | ```
|
157 | - open `src/app/main.ts` and add
|
158 | ```javascript
|
159 | import * as $ from 'jquery';
|
160 | window["$"] = $;
|
161 | window["jQuery"] = $;
|
162 | ```
|
163 | - In `package.json` add the following:
|
164 |
|
165 | ```json
|
166 | "config": {
|
167 | "ionic_copy": "./config/copy.config.js"
|
168 | }
|
169 | ```
|
170 | - Run the following commands
|
171 |
|
172 | ```bash
|
173 | mkdir config
|
174 | cp node_modules/@ionic/app-scripts/config/copy.config.js ./config/
|
175 | ```
|
176 |
|
177 | - Open `config/copy.config.js` file and add the following at the beginning of `module.exports`
|
178 |
|
179 | ```javascript
|
180 | module.exports = {
|
181 | copyFroalaEditorCss: {
|
182 | src: ['{{ROOT}}/node_modules/froala-editor/css/froala_editor.pkgd.min.css', '{{ROOT}}/node_modules/froala-editor/css/froala_style.min.css'],
|
183 | dest: '{{BUILD}}'
|
184 | },
|
185 | copyFontAwesome: {
|
186 | src: '{{ROOT}}/node_modules/font-awesome/css/font-awesome.min.css',
|
187 | dest: '{{BUILD}}'
|
188 | },
|
189 | copyFontsAwesomeFonts: {
|
190 | src: '{{ROOT}}/node_modules/font-awesome/fonts/*',
|
191 | dest: '{{WWW}}/fonts'
|
192 | },
|
193 | ...
|
194 | }
|
195 | ```
|
196 |
|
197 | - Open `src/index.html` file and add in the `<head>` tag:
|
198 |
|
199 | ```html
|
200 | <link rel="stylesheet" href="build/font-awesome.min.css">
|
201 | <link rel="stylesheet" href="build/froala_editor.pkgd.min.css">
|
202 | <link rel="stylesheet" href="build/froala_style.min.css">
|
203 | ```
|
204 | In your desired view add the Froala Editor like this:
|
205 |
|
206 | ```html
|
207 | <div [froalaEditor]>Hello, Froala!</div>
|
208 | ```
|
209 |
|
210 | #### Run your App
|
211 |
|
212 | ```bash
|
213 | ionic serve
|
214 | ```
|
215 |
|
216 |
|
217 |
|
218 | ### Use with `webpack`
|
219 |
|
220 | #### Create webpack app
|
221 |
|
222 | *Note*: you can skip this part if you already have application generated.
|
223 |
|
224 | ```bash
|
225 | git clone --depth 1 https://github.com/AngularClass/angular-starter.git
|
226 | cd angular-starter
|
227 | npm install
|
228 | ```
|
229 |
|
230 | #### Add angular-froala-wysiwyg
|
231 |
|
232 | - install `angular-froala-wysiwyg`
|
233 |
|
234 | ```bash
|
235 | npm install angular-froala-wysiwyg --save
|
236 | ```
|
237 |
|
238 | - open `src/app/app.module.ts` and add
|
239 |
|
240 | ```typescript
|
241 | // Import the Froala Editor plugin.
|
242 | import "froala-editor/js/froala_editor.pkgd.min.js";
|
243 |
|
244 | // Import Angular plugin.
|
245 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
246 | ...
|
247 |
|
248 | @NgModule({
|
249 | ...
|
250 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
251 | ...
|
252 | })
|
253 | ```
|
254 |
|
255 | - open `src/app/app.component.ts` and add to the template
|
256 |
|
257 | ```html
|
258 | <div [froalaEditor]>Hello, Froala!</div>
|
259 | ```
|
260 |
|
261 | - open `config/webpack.common.js` and add the following to `CopyWebpackPlugin`
|
262 |
|
263 | ```javascript
|
264 | {
|
265 | from: 'node_modules/froala-editor/css/',
|
266 | to: 'assets/froala-editor/css/',
|
267 | },
|
268 | {
|
269 | from: 'node_modules/font-awesome/css/font-awesome.min.css',
|
270 | to: 'assets/font-awesome/css/font-awesome.min.css',
|
271 | },
|
272 | {
|
273 | from: 'node_modules/font-awesome/fonts',
|
274 | to: 'assets/font-awesome/fonts'
|
275 | }
|
276 | ```
|
277 |
|
278 | - in `config/webpack.common.js` copy the following to `plugins`
|
279 |
|
280 | ```javascript
|
281 | new webpack.ProvidePlugin({
|
282 | $: "jquery",
|
283 | jQuery: "jquery"
|
284 | })
|
285 | ```
|
286 |
|
287 | - open `config/head-config.common.js` and add a new entry to link
|
288 |
|
289 | ```javascript
|
290 | { rel: 'stylesheet', href: '/assets/font-awesome/css/font-awesome.min.css' },
|
291 | { rel: 'stylesheet', href: '/assets/froala-editor/css/froala_editor.pkgd.min.css' },
|
292 | { rel: 'stylesheet', href: '/assets/froala-editor/css/froala_style.min.css' }
|
293 | ```
|
294 |
|
295 | #### Run webpack app
|
296 |
|
297 | ```bash
|
298 | npm run start
|
299 | ```
|
300 |
|
301 |
|
302 |
|
303 | ### Use with `angular-seed`
|
304 |
|
305 | #### Create angular-seed app
|
306 |
|
307 | *Note*: you can skip this part if you already have application generated. For more details please also read: https://github.com/mgechev/angular-seed.
|
308 |
|
309 | ```bash
|
310 | git clone --depth 1 https://github.com/mgechev/angular-seed.git
|
311 | cd angular-seed
|
312 | npm install
|
313 | ```
|
314 |
|
315 | #### Add angular-froala-wysiwyg
|
316 |
|
317 | - install `angular-froala-wysiwyg`
|
318 |
|
319 | ```bash
|
320 | npm install angular-froala-wysiwyg --save
|
321 | ```
|
322 |
|
323 | - open `tools/config/project.config.ts` file and **uncomment** the following line from the top of the file
|
324 |
|
325 | ```typescript
|
326 | import { ExtendPackages } from './seed.config.interfaces';
|
327 | ```
|
328 |
|
329 | - in `tools/config/project.config.ts` file add
|
330 |
|
331 | ```typescript
|
332 | ...
|
333 |
|
334 | this.NPM_DEPENDENCIES = [
|
335 | ...this.NPM_DEPENDENCIES,
|
336 | { src: 'jquery/dist/jquery.min.js', inject: 'libs'},
|
337 | { src: 'froala-editor/js/froala_editor.pkgd.min.js', inject: 'libs' },
|
338 | { src: 'font-awesome/css/font-awesome.min.css', inject: true },
|
339 | { src: 'froala-editor/css/froala_editor.pkgd.min.css', inject: true },
|
340 | { src: 'froala-editor/css/froala_style.min.css', inject: true }
|
341 | ];
|
342 |
|
343 | ...
|
344 |
|
345 | let additionalPackages: ExtendPackages[] = [
|
346 | // required for dev build
|
347 | {
|
348 | name:'angular-froala-wysiwyg',
|
349 | path:'node_modules/angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.min.js'
|
350 | },
|
351 |
|
352 | // required for prod build
|
353 | {
|
354 | name:'angular-froala-wysiwyg/*',
|
355 | path:'node_modules/angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.min.js'
|
356 | }
|
357 | ]
|
358 |
|
359 | this.addPackagesBundles(additionalPackages);
|
360 | ```
|
361 |
|
362 | - open `src/client/app/home/home.module.ts` and add
|
363 |
|
364 | ```typescript
|
365 | // Import Angular2 plugin.
|
366 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
367 | ...
|
368 |
|
369 | @NgModule({
|
370 | ...
|
371 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
|
372 | ...
|
373 | })
|
374 | ```
|
375 |
|
376 | - open `src/client/app/home/home.component.html` and add
|
377 |
|
378 | ```html
|
379 | <div [froalaEditor]>Hello, Froala!</div>
|
380 | ```
|
381 |
|
382 | #### Run webpack app
|
383 |
|
384 | ```bash
|
385 | npm run start
|
386 | ```
|
387 |
|
388 |
|
389 |
|
390 | ### Use with `system.js` and `JIT`
|
391 |
|
392 | #### Create Angular app
|
393 |
|
394 | *Note*: you can skip this part if you already have application generated.
|
395 |
|
396 | ```bash
|
397 | git clone https://github.com/angular/quickstart.git angular-quickstart
|
398 | cd angular-quickstart
|
399 | npm install
|
400 | ```
|
401 |
|
402 | #### Add angular-froala-wysiwyg
|
403 |
|
404 | - install `angular-froala-wysiwyg`
|
405 |
|
406 | ```bash
|
407 | npm install angular-froala-wysiwyg --save
|
408 | ```
|
409 |
|
410 | - open `src/index.html` and add
|
411 |
|
412 | ```html
|
413 | <link rel="stylesheet" href="node_modules/font-awesome/css/font-awesome.min.css">
|
414 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_editor.pkgd.min.css">
|
415 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_style.min.css">
|
416 |
|
417 | <script src="node_modules/jquery/dist/jquery.min.js"></script>
|
418 | <script src="node_modules/froala-editor/js/froala_editor.pkgd.min.js"></script>
|
419 | ```
|
420 |
|
421 | - open `src/app/app.module.ts` and add
|
422 |
|
423 | ```typescript
|
424 | // Import Angular2 plugin.
|
425 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
426 | ...
|
427 |
|
428 | @NgModule({
|
429 | ...
|
430 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
431 | ...
|
432 | })
|
433 | ```
|
434 |
|
435 | - open `src/app/app.component.ts` file and add to the template
|
436 |
|
437 | ```html
|
438 | <div [froalaEditor]>Hello, Froala!</div>
|
439 | ```
|
440 |
|
441 | - open `src/systemjs.config.js` file and add to map
|
442 |
|
443 | ```javascript
|
444 | map: {
|
445 | ...
|
446 | 'angular-froala-wysiwyg': 'npm:angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.js',
|
447 | ...
|
448 | }
|
449 | ```
|
450 |
|
451 | ```html
|
452 | <div [froalaEditor]>Hello, Froala!</div>
|
453 | ```
|
454 |
|
455 | -
|
456 |
|
457 | #### Run app
|
458 |
|
459 | ```bash
|
460 | npm run start
|
461 | ```
|
462 |
|
463 |
|
464 |
|
465 | ### Use with `aot`
|
466 |
|
467 | #### Create Angular app
|
468 |
|
469 | *Note*: you can skip this part if you already have application generated.
|
470 |
|
471 | ```bash
|
472 | git clone https://github.com/angular/quickstart.git angular-quickstart
|
473 | cd angular-quickstart
|
474 | npm install
|
475 | ```
|
476 |
|
477 | Install additional dependencies. *Make sure that there is no UNMET PEER DEPENDENCY.*
|
478 |
|
479 | ```bash
|
480 | npm install @angular/compiler-cli @angular/platform-server --save
|
481 | npm install rollup rollup-plugin-node-resolve rollup-plugin-commonjs rollup-plugin-uglify --save-dev
|
482 | npm install lite-server --save-dev
|
483 | ```
|
484 |
|
485 | Copy `scr/tsconfig.json` to a new file called `tsconfig-aot.json` in the root of the project, then modify it as follows.
|
486 |
|
487 | ```json
|
488 | {
|
489 | "compilerOptions": {
|
490 | "target": "es5",
|
491 | "module": "es2015",
|
492 | "moduleResolution": "node",
|
493 | "sourceMap": true,
|
494 | "emitDecoratorMetadata": true,
|
495 | "experimentalDecorators": true,
|
496 | "lib": ["es2015", "dom"],
|
497 | "noImplicitAny": true,
|
498 | "suppressImplicitAnyIndexErrors": true,
|
499 | "typeRoots": [
|
500 | "./node_modules/@types/"
|
501 | ]
|
502 | },
|
503 |
|
504 | "files": [
|
505 | "src/app/app.module.ts",
|
506 | "src/main.ts"
|
507 | ],
|
508 |
|
509 | "angularCompilerOptions": {
|
510 | "genDir": "aot",
|
511 | "skipMetadataEmit" : true
|
512 | }
|
513 | }
|
514 | ```
|
515 |
|
516 | Create a configuration file (`rollup-config.js`) in the project root directory to tell Rollup how to process the application.
|
517 |
|
518 | ```javascript
|
519 | import nodeResolve from 'rollup-plugin-node-resolve';
|
520 | import commonjs from 'rollup-plugin-commonjs';
|
521 | import uglify from 'rollup-plugin-uglify';
|
522 |
|
523 | export default {
|
524 | entry: 'src/main.js',
|
525 | dest: 'src/build.js', // output a single application bundle
|
526 | sourceMap: false,
|
527 | format: 'iife',
|
528 | onwarn: function(warning) {
|
529 | // Skip certain warnings
|
530 |
|
531 | // should intercept ... but doesn't in some rollup versions
|
532 | if ( warning.code === 'THIS_IS_UNDEFINED' ) { return; }
|
533 |
|
534 | // console.warn everything else
|
535 | console.warn( warning.message );
|
536 | },
|
537 | plugins: [
|
538 | nodeResolve({jsnext: true, module: true}),
|
539 | commonjs({
|
540 | include: 'node_modules/rxjs/**',
|
541 | }),
|
542 | uglify()
|
543 | ]
|
544 | };
|
545 | ```
|
546 |
|
547 | Update `src/main.ts` file for AOT:
|
548 |
|
549 | ```typescript
|
550 | import { platformBrowser } from '@angular/platform-browser';
|
551 | import { AppModuleNgFactory } from '../aot/src/app/app.module.ngfactory';
|
552 |
|
553 | console.log('Running AOT compiled');
|
554 | platformBrowser().bootstrapModuleFactory(AppModuleNgFactory);
|
555 | ```
|
556 |
|
557 | #### Add angular-froala-wysiwyg
|
558 |
|
559 | - install `angular-froala-wysiwyg`
|
560 |
|
561 | ```bash
|
562 | npm install angular-froala-wysiwyg --save
|
563 | ```
|
564 |
|
565 | - open `src/index.html` and add
|
566 |
|
567 | ```html
|
568 | <link rel="stylesheet" href="node_modules/font-awesome/css/font-awesome.min.css">
|
569 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_editor.pkgd.min.css">
|
570 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_style.min.css">
|
571 |
|
572 | <script src="node_modules/jquery/dist/jquery.min.js"></script>
|
573 | <script src="node_modules/froala-editor/js/froala_editor.pkgd.min.js"></script>
|
574 | ```
|
575 |
|
576 | - open `src/app/app.module.ts` and add
|
577 |
|
578 | ```typescript
|
579 | // Import Angular2 plugin.
|
580 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
581 | ...
|
582 |
|
583 | @NgModule({
|
584 | ...
|
585 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
586 | ...
|
587 | })
|
588 | ```
|
589 |
|
590 | - open `src/app/app.component.ts` file and add to the template
|
591 |
|
592 | ```html
|
593 | <div [froalaEditor]>Hello, Froala!</div>
|
594 | ```
|
595 |
|
596 | - open `rollup-config.js` and add the following
|
597 |
|
598 | ```javascript
|
599 | //paths are relative to the execution path
|
600 | export default {
|
601 | ...
|
602 | plugins: [
|
603 | ...
|
604 | commonjs({
|
605 | include: [
|
606 | 'node_modules/rxjs/**',
|
607 | 'node_modules/angular-froala-wysiwyg/**'
|
608 | ]
|
609 | }),
|
610 | ...
|
611 | ]
|
612 | }
|
613 | ```
|
614 |
|
615 | #### Run app
|
616 |
|
617 | ```bash
|
618 | node_modules/.bin/ngc -p tsconfig-aot.json
|
619 | node_modules/.bin/rollup -c rollup-config.js -c rollup-config.js
|
620 | lite-server
|
621 | ```
|
622 |
|
623 |
|
624 |
|
625 | ## Usage
|
626 |
|
627 | ### Options
|
628 |
|
629 | You can pass editor options as Input (optional).
|
630 |
|
631 | `[froalaEditor]='options'`
|
632 |
|
633 | You can pass any existing Froala option. Consult the [Froala documentation](https://www.froala.com/wysiwyg-editor/docs/options) to view the list of all the available options:
|
634 |
|
635 | ```typescript
|
636 | public options: Object = {
|
637 | placeholderText: 'Edit Your Content Here!',
|
638 | charCounterCount: false
|
639 | }
|
640 | ```
|
641 |
|
642 | Aditional option is used:
|
643 | * **immediateAngularModelUpdate**: (default: false) This option synchronizes the angular model as soon as a key is released in the editor. Note that it may affect performances.
|
644 |
|
645 |
|
646 |
|
647 | ### Events and Methods
|
648 |
|
649 | Events can be passed in with the options, with a key events and object where the key is the event name and the value is the callback function.
|
650 |
|
651 | ```typescript
|
652 | public options: Object = {
|
653 | placeholder: "Edit Me",
|
654 | events : {
|
655 | 'froalaEditor.focus' : function(e, editor) {
|
656 | console.log(editor.selection.get());
|
657 | }
|
658 | }
|
659 | }
|
660 | ```
|
661 |
|
662 | Using the editor instance from the arguments of the callback you can call editor methods as described in the [method docs](http://froala.com/wysiwyg-editor/docs/methods).
|
663 |
|
664 | Froala events are described in the [events docs](https://froala.com/wysiwyg-editor/docs/events).
|
665 |
|
666 |
|
667 |
|
668 | ### Model
|
669 |
|
670 | The WYSIWYG HTML editor content model.
|
671 |
|
672 | `[(froalaModel)]="editorContent"`
|
673 |
|
674 | Pass initial content:
|
675 |
|
676 | ```typescript
|
677 | public editorContent: string = 'My Document\'s Title'
|
678 | ```
|
679 |
|
680 | Use the content in other places:
|
681 |
|
682 | ```html
|
683 | <input [ngModel]="editorContent"/>
|
684 | <input [(ngModel)]="editorContent"/> <!-- For two way binding -->
|
685 | ```
|
686 |
|
687 | Other two way binding example:
|
688 |
|
689 | ```html
|
690 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
691 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
692 | ```
|
693 |
|
694 | Use it with reactive forms:
|
695 |
|
696 | ```html
|
697 | <form [formGroup]="form" (ngSubmit)="onSubmit()">
|
698 | <textarea [froalaEditor] formControlName="formModel"></textarea>
|
699 | <button type="submit">Submit</button>
|
700 | </form>
|
701 | ```
|
702 |
|
703 | If you want to use two-way binding to display the form model in other places you must include `[(froalaModel)]`:
|
704 |
|
705 | ```html
|
706 | <form [formGroup]="form" (ngSubmit)="onSubmit()">
|
707 | <textarea [froalaEditor] formControlName="formModel" [(froalaModel)]="form.formModel"></textarea>
|
708 | <div [froalaView]="form.formModel"></div>
|
709 | <button type="submit">Submit</button>
|
710 | </form>
|
711 | ```
|
712 |
|
713 | If you want to wrap froalaEditor directive into a component that supports reactive forms please see [froala.component.ts](https://github.com/froala/angular-froala-wysiwyg/blob/master/demo/src/app/froala.component.ts) from demo.
|
714 |
|
715 | ### Extend functionality
|
716 |
|
717 | You can extend the functionality by adding a custom button like bellow:
|
718 |
|
719 | ```typescript
|
720 |
|
721 | // We will make usage of the Init hook and make the implementation there.
|
722 | import { Component, OnInit } from '@angular/core';
|
723 | declare var $ :any;
|
724 |
|
725 | @Component({
|
726 | selector: 'app-demo',
|
727 | template: `<div class="sample">
|
728 | <h2>Sample 11: Add Custom Button</h2>
|
729 | <div [froalaEditor]="options" [(froalaModel)]="content" ></div>
|
730 | </div>`,
|
731 |
|
732 |
|
733 | export class AppComponent implements OnInit{
|
734 |
|
735 | ngOnInit () {
|
736 | $.FroalaEditor.DefineIcon('alert', {NAME: 'info'});
|
737 | $.FroalaEditor.RegisterCommand('alert', {
|
738 | title: 'Hello',
|
739 | focus: false,
|
740 | undo: false,
|
741 | refreshAfterCallback: false,
|
742 |
|
743 | callback: () => {
|
744 | alert('Hello!', this);
|
745 | }
|
746 | });
|
747 | }
|
748 |
|
749 | public options: Object = {
|
750 | charCounterCount: true,
|
751 | toolbarButtons: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
752 | toolbarButtonsXS: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
753 | toolbarButtonsSM: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
754 | toolbarButtonsMD: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
755 | };
|
756 | }
|
757 | ```
|
758 |
|
759 |
|
760 | ### Special tags
|
761 |
|
762 | You can also use the editor on **img**, **button**, **input** and **a** tags:
|
763 |
|
764 | ```html
|
765 | <img [froalaEditor] [(froalaModel)]="imgObj"/>
|
766 | ```
|
767 |
|
768 | The model must be an object containing the attributes for your special tags. Example:
|
769 |
|
770 | ```typescript
|
771 | public imgObj: Object = {
|
772 | src: 'path/to/image.jpg'
|
773 | };
|
774 | ```
|
775 |
|
776 | The froalaModel will change as the attributes change during usage.
|
777 |
|
778 | * froalaModel can contain a special attribute named **innerHTML** which inserts innerHTML in the element: If you are using 'button' tag, you can specify the button text like this:
|
779 |
|
780 | ```typescript
|
781 | public buttonModel: Object = {
|
782 | innerHTML: 'Click Me'
|
783 | };
|
784 | ```
|
785 | As the button text is modified by the editor, the **innerHTML** attribute from buttonModel model will be modified too.
|
786 |
|
787 |
|
788 |
|
789 | ### Specific option for special tags
|
790 |
|
791 | * **angularIgnoreAttrs**: (default: null) This option is an array of attributes that you want to ignore when the editor updates the froalaModel:
|
792 |
|
793 | ```typescript
|
794 | public inputOptions: Object = {
|
795 | angularIgnoreAttrs: ['class', 'id']
|
796 | };
|
797 | ```
|
798 |
|
799 |
|
800 |
|
801 | ## Manual Initialization
|
802 |
|
803 | Gets the functionality to operate on the editor: create, destroy and get editor instance. Use it if you want to manually initialize the editor.
|
804 |
|
805 | `(froalaInit)="initialize($event)"`
|
806 |
|
807 | Where `initialize` is the name of a function in your component which will receive an object with different methods to control the editor initialization process.
|
808 |
|
809 | ```typescript
|
810 | public initialize(initControls) {
|
811 | this.initControls = initControls;
|
812 | this.deleteAll = function() {
|
813 | this.initControls.getEditor()('html.set', '');
|
814 | };
|
815 | }
|
816 | ```
|
817 |
|
818 | The object received by the function will contain the following methods:
|
819 |
|
820 | - **initialize**: Call this method to initialize the Froala Editor
|
821 | - **destroy**: Call this method to destroy the Froala Editor
|
822 | - **getEditor**: Call this method to retrieve the editor that was created. This method will return *null* if the editor was not yet created
|
823 |
|
824 |
|
825 |
|
826 |
|
827 | ## Displaying HTML
|
828 |
|
829 | To display content created with the froala editor use the froalaView directive.
|
830 |
|
831 | `[froalaView]="editorContent"`
|
832 |
|
833 | ```html
|
834 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
835 | <div [froalaView]="editorContent"></div>
|
836 | ```
|
837 |
|
838 |
|
839 |
|
840 | ## License
|
841 |
|
842 | The `angular-froala-wyswiyg` project is under MIT license. However, in order to use Froala WYSIWYG HTML Editor plugin you should purchase a license for it.
|
843 |
|
844 | Froala Editor has [3 different licenses](http://froala.com/wysiwyg-editor/pricing) for commercial use.
|
845 | For details please see [License Agreement](http://froala.com/wysiwyg-editor/terms).
|
846 |
|
847 |
|
848 |
|
849 | ## Development environment setup
|
850 |
|
851 | If you want to contribute to angular-froala-wyswiyg, you will first need to install the required tools to get the project going.
|
852 |
|
853 | #### Prerequisites
|
854 |
|
855 | * [Node Package Manager](https://npmjs.org/) (NPM)
|
856 | * [Git](http://git-scm.com/)
|
857 |
|
858 | #### Install dependencies
|
859 |
|
860 | $ npm install
|
861 |
|
862 | #### Build
|
863 |
|
864 | $ npm run demo.build
|
865 |
|
866 | #### Run Demo
|
867 |
|
868 | $ npm run start
|