1 | # Angular Froala WYSIWYG Editor - [Demo](https://www.froala.com/wysiwyg-editor)
|
2 |
|
3 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
4 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
5 | [](https://www.npmjs.com/package/angular-froala-wysiwyg)
|
6 |
|
7 | >Angular 2, Angular 4, Angular 5, Angular 6 and Angular 7 bindings for Froala WYSIWYG Editor.
|
8 |
|
9 | 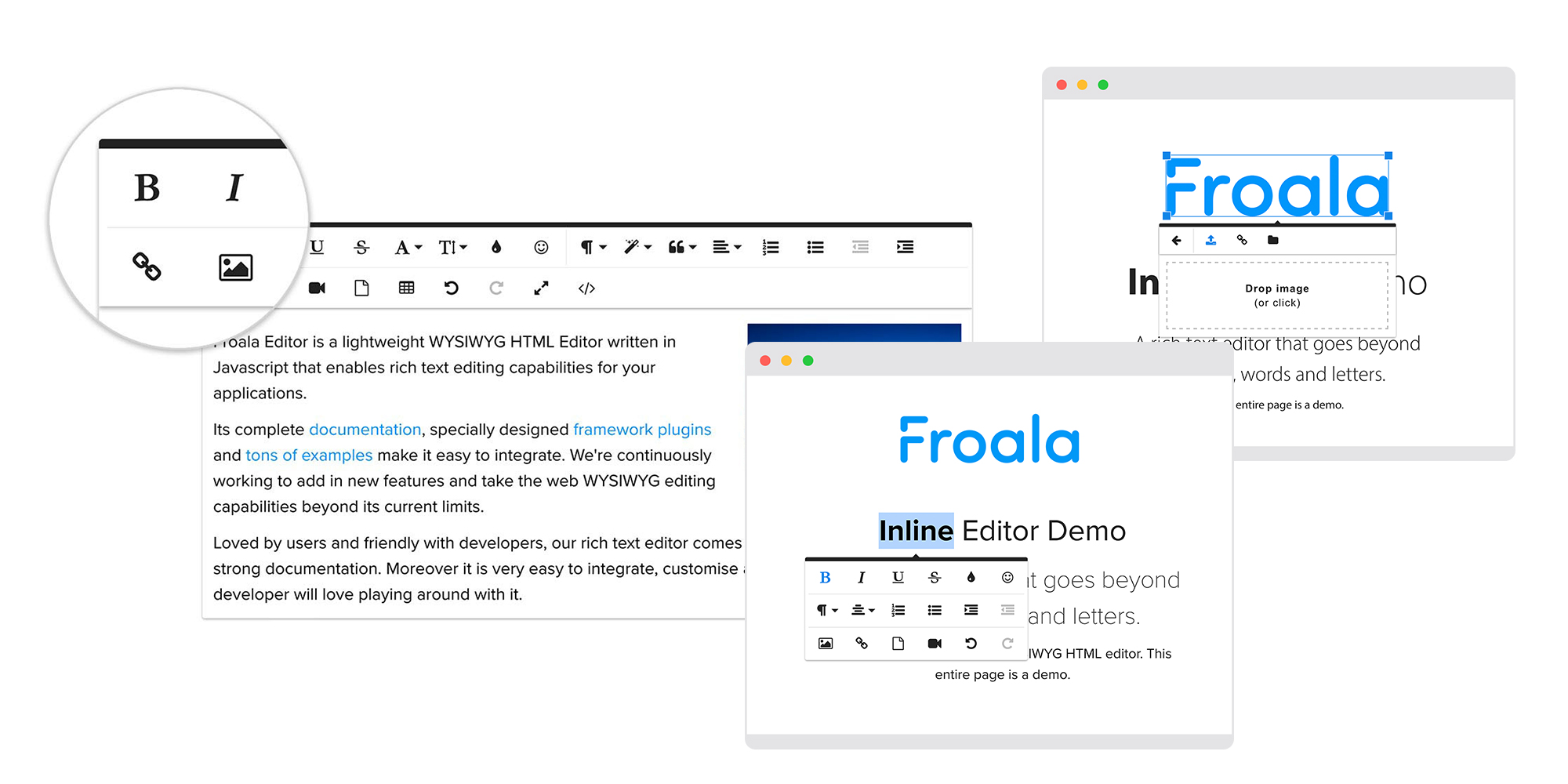
|
10 |
|
11 | ## Table of contents
|
12 | 1. [Installation instructions](#installation-instructions)
|
13 | 2. [Update editor instructions](#update-editor-instructions)
|
14 | 3. [Integration](#integration)
|
15 | - [angular-cli](#use-with-angular-cli)
|
16 | - [ionic v2 or v3](#use-with-ionic-v2-or-v3)
|
17 | - [webpack](#use-with-webpack)
|
18 | - [angular-starter](#use-with-webpack)
|
19 | - [angular-seed](#use-with-angular-seed)
|
20 | - [System.js and JIT](#use-with-systemjs-and-jit)
|
21 | - [AOT](#use-with-aot)
|
22 | 4. [Usage](#usage)
|
23 | 5. [Manual Initialization](#manual-initialization)
|
24 | 6. [Displaying HTML](#displaying-html)
|
25 | 7. [License](#license)
|
26 | 8. [Development environment setup](#development-environment-setup)
|
27 |
|
28 | ## Installation instructions
|
29 |
|
30 | Install `angular-froala-wysiwyg` from `npm`
|
31 |
|
32 | ```bash
|
33 | npm install angular-froala-wysiwyg
|
34 | ```
|
35 |
|
36 | You will need CSS styles
|
37 |
|
38 | ```html
|
39 | <!-- index.html -->
|
40 | <link href="node_modules/froala-editor/css/froala_editor.pkgd.min.css" rel="stylesheet">
|
41 | ```
|
42 |
|
43 | ## Update editor instructions
|
44 |
|
45 | ```bash
|
46 | npm update froala-editor --save
|
47 | ```
|
48 |
|
49 | ## Integration
|
50 |
|
51 | ### Use with Angular CLI
|
52 |
|
53 | #### Installing @angular/cli
|
54 |
|
55 | *Note*: you can skip this part if you already have application generated.
|
56 |
|
57 | ```bash
|
58 | npm install -g @angular/cli
|
59 | ng new my-app
|
60 | cd my-app
|
61 | ```
|
62 |
|
63 | #### Add angular-froala-wysiwyg
|
64 |
|
65 | - install `angular-froala-wysiwyg`
|
66 |
|
67 | ```bash
|
68 | npm install angular-froala-wysiwyg --save
|
69 | ```
|
70 |
|
71 | - open `src/app/app.module.ts` and add
|
72 |
|
73 | ```typescript
|
74 | // Import all Froala Editor plugins.
|
75 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
76 |
|
77 | // Import a single Froala Editor plugin.
|
78 | // import 'froala-editor/js/plugins/align.min.js';
|
79 |
|
80 | // Import a Froala Editor language file.
|
81 | // import 'froala-editor/js/languages/de.js';
|
82 |
|
83 | // Import Angular plugin.
|
84 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
85 | ...
|
86 |
|
87 | @NgModule({
|
88 | ...
|
89 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
|
90 | ...
|
91 | })
|
92 | ```
|
93 |
|
94 | - open `angular.json` file and insert a new entry into the `styles` array
|
95 |
|
96 | ```json
|
97 | "styles": [
|
98 | "styles.css",
|
99 | "./node_modules/froala-editor/css/froala_editor.pkgd.min.css",
|
100 | "./node_modules/froala-editor/css/froala_style.min.css",
|
101 | ]
|
102 | ```
|
103 |
|
104 | - open `src/app/app.component.html` and add
|
105 |
|
106 | ```html
|
107 | <div [froalaEditor]>Hello, Froala!</div>
|
108 | ```
|
109 |
|
110 | #### Run angular-cli
|
111 | ```bash
|
112 | ng serve
|
113 | ```
|
114 |
|
115 |
|
116 |
|
117 | ### Use with `ionic v2 or v3`
|
118 |
|
119 | #### Create Ionic app
|
120 |
|
121 | *Note*: you can skip this part if you already have application generated.
|
122 |
|
123 | ```bash
|
124 | npm install -g cordova ionic
|
125 | ionic start sample blank
|
126 | cd sample
|
127 | ```
|
128 |
|
129 | #### Add angular-froala-wysiwyg
|
130 |
|
131 | For v3 make sure that you use the latest version of ionic and also the latest version of angular.
|
132 |
|
133 | Installing Froala Wysiwyg Editor in Ionic is fairly easy, it can be done using npm:
|
134 | ```bash
|
135 | npm install angular-froala-wysiwyg --save
|
136 | ```
|
137 |
|
138 | - Inside `src/app/app.component.html` add
|
139 |
|
140 | ```html
|
141 | <ion-app>
|
142 | <ion-router-outlet></ion-router-outlet>
|
143 | <div [froalaEditor]>Hello, Froala!</div>
|
144 | </ion-app>
|
145 | ```
|
146 |
|
147 |
|
148 | - open `src/app/app.module.ts` and add
|
149 |
|
150 | ```typescript
|
151 | // Import all Froala Editor plugins.
|
152 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
153 |
|
154 | // Import a single Froala Editor plugin.
|
155 | // import 'froala-editor/js/plugins/align.min.js';
|
156 |
|
157 | // Import a Froala Editor language file.
|
158 | // import 'froala-editor/js/languages/de.js';
|
159 |
|
160 | // Import Angular2 plugin.
|
161 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
162 | ...
|
163 |
|
164 | ```
|
165 | Replace
|
166 | ```
|
167 | imports: [BrowserModule, IonicModule.forRoot(), AppRoutingModule]
|
168 | ```
|
169 | with
|
170 | ```
|
171 | imports: [BrowserModule, IonicModule.forRoot(), AppRoutingModule,FroalaEditorModule.forRoot(), FroalaViewModule.forRoot()]
|
172 | ```
|
173 |
|
174 | - Inside `src/app/app-routing.module.ts` remove the line
|
175 | ```
|
176 | { path: '', redirectTo: 'home', pathMatch: 'full' }
|
177 | ```
|
178 |
|
179 | - Inside `src/index.html`
|
180 |
|
181 | ```html
|
182 | <link rel="stylesheet" href="assets/css/font-awesome.min.css">
|
183 | <link rel="stylesheet" href="assets/css/froala_editor.pkgd.min.css">
|
184 | <link rel="stylesheet" href="assets/css/froala_style.min.css">
|
185 | ```
|
186 |
|
187 | - In `angular.json` change outpath of build to "outputPath": "src/assets" and insert following inside assets of build:
|
188 | ```javascript
|
189 | {
|
190 | "glob": "**/*",
|
191 | "input": "node_modules/froala-editor/css",
|
192 | "output": "css"
|
193 | },
|
194 | {
|
195 | "glob": "**/*",
|
196 | "input": "node_modules/font-awesome/css",
|
197 | "output": "css"
|
198 | },
|
199 | {
|
200 | "glob": "**/*",
|
201 | "input": "node_modules/font-awesome/fonts",
|
202 | "output": "fonts"
|
203 | },
|
204 | {
|
205 | "glob": "**/*",
|
206 | "input": "node_modules/froala-editor/js",
|
207 | "output": "js"
|
208 | }
|
209 | ```
|
210 |
|
211 | #### Run your App
|
212 |
|
213 | ```bash
|
214 | ionic build
|
215 | ionic serve
|
216 | ```
|
217 |
|
218 |
|
219 |
|
220 | ### Use with `webpack`
|
221 |
|
222 | #### Create webpack app
|
223 |
|
224 | *Note*: you can skip this part if you already have application generated.
|
225 |
|
226 | ```bash
|
227 | git clone --depth 1 https://github.com/AngularClass/angular-starter.git
|
228 | cd angular-starter
|
229 | npm install
|
230 | npm install rxjs@6.0.0 --save
|
231 | npm install @types/node@10.1.4
|
232 | ```
|
233 |
|
234 | #### Add angular-froala-wysiwyg
|
235 |
|
236 | - install `angular-froala-wysiwyg`
|
237 |
|
238 | ```bash
|
239 | npm install angular-froala-wysiwyg --save
|
240 | ```
|
241 |
|
242 | - open `src/app/app.module.ts` and add
|
243 |
|
244 | ```typescript
|
245 | // Import all Froala Editor plugins.
|
246 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
247 |
|
248 | // Import a single Froala Editor plugin.
|
249 | // import 'froala-editor/js/plugins/align.min.js';
|
250 |
|
251 | // Import a Froala Editor language file.
|
252 | // import 'froala-editor/js/languages/de.js';
|
253 |
|
254 | // Import Angular plugin.
|
255 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
256 | ...
|
257 |
|
258 | @NgModule({
|
259 | ...
|
260 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
261 | ...
|
262 | })
|
263 | ```
|
264 |
|
265 | - open `src/app/app.component.ts` and add to the template
|
266 |
|
267 | ```html
|
268 | <div [froalaEditor]>Hello, Froala!</div>
|
269 | ```
|
270 |
|
271 | - open `config/webpack.common.js`
|
272 |
|
273 | ```javascript
|
274 | var webpack = require('webpack');
|
275 | ```
|
276 |
|
277 |
|
278 | - open `config/webpack.common.js` and add the following to `CopyWebpackPlugin`
|
279 |
|
280 | ```javascript
|
281 | {
|
282 | from: 'node_modules/froala-editor/css/',
|
283 | to: 'assets/froala-editor/css/',
|
284 | },
|
285 | ```
|
286 |
|
287 | - open `config/head-config.common.js` and add a new entry to link
|
288 |
|
289 | ```javascript
|
290 | { rel: 'stylesheet', href: '/assets/froala-editor/css/froala_editor.pkgd.min.css' },
|
291 | { rel: 'stylesheet', href: '/assets/froala-editor/css/froala_style.min.css' }
|
292 | ```
|
293 |
|
294 | #### Run webpack app
|
295 |
|
296 | ```bash
|
297 | npm run start
|
298 | ```
|
299 |
|
300 |
|
301 |
|
302 | ### Use with `angular-seed`
|
303 |
|
304 | #### Create angular-seed app
|
305 |
|
306 | *Note*: you can skip this part if you already have application generated. For more details please also read: https://github.com/mgechev/angular-seed.
|
307 |
|
308 | ```bash
|
309 | git clone --depth 1 https://github.com/mgechev/angular-seed.git
|
310 | cd angular-seed
|
311 | npm install
|
312 | ```
|
313 |
|
314 | #### Add angular-froala-wysiwyg
|
315 |
|
316 | - install `angular-froala-wysiwyg`
|
317 |
|
318 | ```bash
|
319 | npm install angular-froala-wysiwyg --save
|
320 | ```
|
321 |
|
322 | - open `tools/config/project.config.ts` file and **uncomment** the following line from the top of the file
|
323 |
|
324 | ```typescript
|
325 | import { ExtendPackages } from './seed.config.interfaces';
|
326 | ```
|
327 |
|
328 | - in `tools/config/project.config.ts` file add
|
329 |
|
330 | ```typescript
|
331 | ...
|
332 |
|
333 | this.NPM_DEPENDENCIES = [
|
334 | ...this.NPM_DEPENDENCIES,
|
335 | { src: 'froala-editor/css/froala_editor.pkgd.min.css', inject: true },
|
336 | { src: 'froala-editor/css/froala_style.min.css', inject: true }
|
337 | ];
|
338 |
|
339 | ...
|
340 |
|
341 | let additionalPackages: ExtendPackages[] = [
|
342 | // required for dev build
|
343 | {
|
344 | name:'angular-froala-wysiwyg',
|
345 | path:'node_modules/angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.min.js'
|
346 | },
|
347 |
|
348 | // required for prod build
|
349 | {
|
350 | name:'angular-froala-wysiwyg/*',
|
351 | path:'node_modules/angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.min.js'
|
352 | }
|
353 | ]
|
354 |
|
355 | this.addPackagesBundles(additionalPackages);
|
356 | ```
|
357 |
|
358 | - open `src/client/app/home/home.module.ts` and add
|
359 |
|
360 | ```typescript
|
361 | // Import all Froala Editor plugins.
|
362 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
363 |
|
364 | // Import a single Froala Editor plugin.
|
365 | // import 'froala-editor/js/plugins/align.min.js';
|
366 |
|
367 | // Import a Froala Editor language file.
|
368 | // import 'froala-editor/js/languages/de.js';
|
369 |
|
370 | // Import Angular2 plugin.
|
371 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
372 | ...
|
373 |
|
374 | @NgModule({
|
375 | ...
|
376 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
|
377 | ...
|
378 | })
|
379 | ```
|
380 |
|
381 | - open `src/client/app/home/home.component.html` and add
|
382 |
|
383 | ```html
|
384 | <div [froalaEditor]>Hello, Froala!</div>
|
385 | ```
|
386 |
|
387 | #### Run webpack app
|
388 |
|
389 | ```bash
|
390 | npm run start
|
391 | ```
|
392 |
|
393 |
|
394 |
|
395 | ### Use with `system.js` and `JIT`
|
396 |
|
397 | #### Create Angular app
|
398 |
|
399 | *Note*: you can skip this part if you already have application generated.
|
400 |
|
401 | ```bash
|
402 | git clone https://github.com/angular/quickstart.git angular-quickstart
|
403 | cd angular-quickstart
|
404 | npm install
|
405 | ```
|
406 |
|
407 | #### Add angular-froala-wysiwyg
|
408 |
|
409 | - install `angular-froala-wysiwyg`
|
410 |
|
411 | ```bash
|
412 | npm install angular-froala-wysiwyg --save
|
413 | ```
|
414 |
|
415 | - open `src/index.html` and add
|
416 |
|
417 | ```html
|
418 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_editor.pkgd.min.css">
|
419 | <link rel="stylesheet" href="node_modules/froala-editor/css/froala_style.min.css">
|
420 | ```
|
421 |
|
422 | - open `src/app/app.module.ts` and add
|
423 |
|
424 | ```typescript
|
425 | // Import all Froala Editor plugins.
|
426 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
427 |
|
428 | // Import a single Froala Editor plugin.
|
429 | // import 'froala-editor/js/plugins/align.min.js';
|
430 |
|
431 | // Import a Froala Editor language file.
|
432 | // import 'froala-editor/js/languages/de.js';
|
433 |
|
434 | // Import Angular2 plugin.
|
435 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
436 | ...
|
437 |
|
438 | @NgModule({
|
439 | ...
|
440 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
441 | ...
|
442 | })
|
443 | ```
|
444 |
|
445 | - open `src/app/app.component.ts` file and add to the template
|
446 |
|
447 | ```html
|
448 | <div [froalaEditor]>Hello, Froala!</div>
|
449 | ```
|
450 |
|
451 | - open `src/systemjs.config.js` file and add to map
|
452 |
|
453 | ```javascript
|
454 | map: {
|
455 | ...
|
456 | 'angular-froala-wysiwyg': 'npm:angular-froala-wysiwyg/bundles/angular-froala-wysiwyg.umd.js',
|
457 | ...
|
458 | }
|
459 | ```
|
460 |
|
461 | -
|
462 |
|
463 | #### Run app
|
464 |
|
465 | ```bash
|
466 | npm run start
|
467 | ```
|
468 |
|
469 |
|
470 |
|
471 | ### Use with `aot`
|
472 |
|
473 | #### Create Angular app
|
474 |
|
475 | 1. ng new froala-aot
|
476 |
|
477 | 2. npm install font-awesome
|
478 |
|
479 | 3. npm install froala-editor
|
480 |
|
481 | - Go to `angular.json` and change `architect.build.outputPath` to `src/dist` and add following code to `architect.build.options.assets`
|
482 | ```javascript
|
483 | {
|
484 | "glob": "**/*",
|
485 | "input": "./node_modules/froala-editor",
|
486 | "output": "assets/froala-editor/"
|
487 | },
|
488 | {
|
489 | "glob": "**/*",
|
490 | "input": "./node_modules/font-awesome",
|
491 | "output": "assets/font-awesome/"
|
492 | },
|
493 | {
|
494 | "glob": "**/*",
|
495 | "input": "./node_modules/jquery",
|
496 | "output": "assets/jquery/"
|
497 | }
|
498 | ```
|
499 | - Go to `package.json` and update `scripts.build` to `ng build --aot` and `scripts.start` to `ng serve --aot`
|
500 |
|
501 | #### Add angular-froala-wysiwyg
|
502 |
|
503 | - install `angular-froala-wysiwyg`
|
504 |
|
505 | ```bash
|
506 | npm install angular-froala-wysiwyg --save
|
507 | ```
|
508 |
|
509 | - open `src/index.html` and add
|
510 |
|
511 | ```html
|
512 | <link rel="stylesheet" href="assets/font-awesome/css/font-awesome.min.css">
|
513 | <link rel="stylesheet" href="assets/froala-editor/css/froala_editor.pkgd.min.css">
|
514 | ```
|
515 |
|
516 | - open `src/app/app.module.ts` and add
|
517 |
|
518 | ```typescript
|
519 | // Import all Froala Editor plugins.
|
520 | // import 'froala-editor/js/plugins.pkgd.min.js';
|
521 |
|
522 | // Import a single Froala Editor plugin.
|
523 | // import 'froala-editor/js/plugins/align.min.js';
|
524 |
|
525 | // Import a Froala Editor language file.
|
526 | // import 'froala-editor/js/languages/de.js';
|
527 |
|
528 | // Import Angular2 plugin.
|
529 | import { FroalaEditorModule, FroalaViewModule } from 'angular-froala-wysiwyg';
|
530 | ...
|
531 |
|
532 | @NgModule({
|
533 | ...
|
534 | imports: [FroalaEditorModule.forRoot(), FroalaViewModule.forRoot(), ... ],
|
535 | ...
|
536 | })
|
537 | ```
|
538 |
|
539 | - open `src/app/app.component.ts` file and add to the template
|
540 |
|
541 | ```html
|
542 | <div [froalaEditor]>Hello, Froala!</div>
|
543 | ```
|
544 |
|
545 | #### Run app
|
546 |
|
547 | ```bash
|
548 | npm run build
|
549 | npm run start
|
550 | ```
|
551 |
|
552 |
|
553 | ## Usage
|
554 |
|
555 | ### Options
|
556 |
|
557 | You can pass editor options as Input (optional).
|
558 |
|
559 | `[froalaEditor]='options'`
|
560 |
|
561 | You can pass any existing Froala option. Consult the [Froala documentation](https://www.froala.com/wysiwyg-editor/docs/options) to view the list of all the available options:
|
562 |
|
563 | ```typescript
|
564 | public options: Object = {
|
565 | placeholderText: 'Edit Your Content Here!',
|
566 | charCounterCount: false
|
567 | }
|
568 | ```
|
569 |
|
570 | Aditional option is used:
|
571 | * **immediateAngularModelUpdate**: (default: false) This option synchronizes the angular model as soon as a key is released in the editor. Note that it may affect performances.
|
572 |
|
573 |
|
574 |
|
575 | ### Events and Methods
|
576 |
|
577 | Events can be passed in with the options, with a key events and object where the key is the event name and the value is the callback function.
|
578 |
|
579 | ```typescript
|
580 | public options: Object = {
|
581 | placeholder: "Edit Me",
|
582 | events : {
|
583 | 'focus' : function(e, editor) {
|
584 | console.log(editor.selection.get());
|
585 | }
|
586 | }
|
587 | }
|
588 | ```
|
589 |
|
590 | Using the editor instance from the arguments of the callback you can call editor methods as described in the [method docs](http://froala.com/wysiwyg-editor/docs/methods).
|
591 |
|
592 | Froala events are described in the [events docs](https://froala.com/wysiwyg-editor/docs/events).
|
593 |
|
594 |
|
595 |
|
596 | ### Model
|
597 |
|
598 | The WYSIWYG HTML editor content model.
|
599 |
|
600 | `[(froalaModel)]="editorContent"`
|
601 |
|
602 | Pass initial content:
|
603 |
|
604 | ```typescript
|
605 | public editorContent: string = 'My Document\'s Title'
|
606 | ```
|
607 |
|
608 | Use the content in other places:
|
609 |
|
610 | ```html
|
611 | <input [ngModel]="editorContent"/>
|
612 | <input [(ngModel)]="editorContent"/> <!-- For two way binding -->
|
613 | ```
|
614 |
|
615 | Other two way binding example:
|
616 |
|
617 | ```html
|
618 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
619 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
620 | ```
|
621 |
|
622 | Use it with reactive forms:
|
623 |
|
624 | ```html
|
625 | <form [formGroup]="form" (ngSubmit)="onSubmit()">
|
626 | <textarea [froalaEditor] formControlName="formModel"></textarea>
|
627 | <button type="submit">Submit</button>
|
628 | </form>
|
629 | ```
|
630 |
|
631 | If you want to use two-way binding to display the form model in other places you must include `[(froalaModel)]`:
|
632 |
|
633 | ```html
|
634 | <form [formGroup]="form" (ngSubmit)="onSubmit()">
|
635 | <textarea [froalaEditor] formControlName="formModel" [(froalaModel)]="form.formModel"></textarea>
|
636 | <div [froalaView]="form.formModel"></div>
|
637 | <button type="submit">Submit</button>
|
638 | </form>
|
639 | ```
|
640 |
|
641 | If you want to wrap froalaEditor directive into a component that supports reactive forms please see [froala.component.ts](https://github.com/froala/angular-froala-wysiwyg/blob/master/demo/src/app/froala.component.ts) from demo.
|
642 |
|
643 | ### Extend functionality
|
644 |
|
645 | You can extend the functionality by adding a custom button like bellow:
|
646 |
|
647 | ```typescript
|
648 | // Import Froala Editor.
|
649 | import FroalaEditor from 'froala-editor';
|
650 |
|
651 | // We will make usage of the Init hook and make the implementation there.
|
652 | import { Component, OnInit } from '@angular/core';
|
653 |
|
654 | @Component({
|
655 | selector: 'app-demo',
|
656 | template: `<div class="sample">
|
657 | <h2>Sample 11: Add Custom Button</h2>
|
658 | <div [froalaEditor]="options" [(froalaModel)]="content" ></div>
|
659 | </div>`,
|
660 |
|
661 |
|
662 | export class AppComponent implements OnInit{
|
663 |
|
664 | ngOnInit () {
|
665 | FroalaEditor.DefineIcon('alert', {NAME: 'info'});
|
666 | FroalaEditor.RegisterCommand('alert', {
|
667 | title: 'Hello',
|
668 | focus: false,
|
669 | undo: false,
|
670 | refreshAfterCallback: false,
|
671 |
|
672 | callback: () => {
|
673 | alert('Hello!', this);
|
674 | }
|
675 | });
|
676 | }
|
677 |
|
678 | public options: Object = {
|
679 | charCounterCount: true,
|
680 | toolbarButtons: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
681 | toolbarButtonsXS: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
682 | toolbarButtonsSM: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
683 | toolbarButtonsMD: ['bold', 'italic', 'underline', 'paragraphFormat','alert'],
|
684 | };
|
685 | }
|
686 | ```
|
687 |
|
688 |
|
689 | ### Special tags
|
690 |
|
691 | You can also use the editor on **img**, **button**, **input** and **a** tags:
|
692 |
|
693 | ```html
|
694 | <img [froalaEditor] [(froalaModel)]="imgObj"/>
|
695 | ```
|
696 |
|
697 | The model must be an object containing the attributes for your special tags. Example:
|
698 |
|
699 | ```typescript
|
700 | public imgObj: Object = {
|
701 | src: 'path/to/image.jpg'
|
702 | };
|
703 | ```
|
704 |
|
705 | The froalaModel will change as the attributes change during usage.
|
706 |
|
707 | * froalaModel can contain a special attribute named **innerHTML** which inserts innerHTML in the element: If you are using 'button' tag, you can specify the button text like this:
|
708 |
|
709 | ```typescript
|
710 | public buttonModel: Object = {
|
711 | innerHTML: 'Click Me'
|
712 | };
|
713 | ```
|
714 | As the button text is modified by the editor, the **innerHTML** attribute from buttonModel model will be modified too.
|
715 |
|
716 |
|
717 |
|
718 | ### Specific option for special tags
|
719 |
|
720 | * **angularIgnoreAttrs**: (default: null) This option is an array of attributes that you want to ignore when the editor updates the froalaModel:
|
721 |
|
722 | ```typescript
|
723 | public inputOptions: Object = {
|
724 | angularIgnoreAttrs: ['class', 'id']
|
725 | };
|
726 | ```
|
727 |
|
728 |
|
729 |
|
730 | ## Manual Initialization
|
731 |
|
732 | Gets the functionality to operate on the editor: create, destroy and get editor instance. Use it if you want to manually initialize the editor.
|
733 |
|
734 | `(froalaInit)="initialize($event)"`
|
735 |
|
736 | Where `initialize` is the name of a function in your component which will receive an object with different methods to control the editor initialization process.
|
737 |
|
738 | ```typescript
|
739 | public initialize(initControls) {
|
740 | this.initControls = initControls;
|
741 | this.deleteAll = function() {
|
742 | this.initControls.getEditor()('html.set', '');
|
743 | };
|
744 | }
|
745 | ```
|
746 |
|
747 | The object received by the function will contain the following methods:
|
748 |
|
749 | - **initialize**: Call this method to initialize the Froala Editor
|
750 | - **destroy**: Call this method to destroy the Froala Editor
|
751 | - **getEditor**: Call this method to retrieve the editor that was created. This method will return *null* if the editor was not yet created
|
752 |
|
753 |
|
754 |
|
755 |
|
756 | ## Displaying HTML
|
757 |
|
758 | To display content created with the froala editor use the froalaView directive.
|
759 |
|
760 | `[froalaView]="editorContent"`
|
761 |
|
762 | ```html
|
763 | <div [froalaEditor] [(froalaModel)]="editorContent"></div>
|
764 | <div [froalaView]="editorContent"></div>
|
765 | ```
|
766 |
|
767 |
|
768 |
|
769 | ## License
|
770 |
|
771 | The `angular-froala-wyswiyg` project is under MIT license. However, in order to use Froala WYSIWYG HTML Editor plugin you should purchase a license for it.
|
772 |
|
773 | Froala Editor has [3 different licenses](http://froala.com/wysiwyg-editor/pricing) for commercial use.
|
774 | For details please see [License Agreement](http://froala.com/wysiwyg-editor/terms).
|
775 |
|
776 |
|
777 |
|
778 | ## Development environment setup
|
779 |
|
780 | If you want to contribute to angular-froala-wyswiyg, you will first need to install the required tools to get the project going.
|
781 |
|
782 | #### Prerequisites
|
783 |
|
784 | * [Node Package Manager](https://npmjs.org/) (NPM)
|
785 | * [Git](http://git-scm.com/)
|
786 |
|
787 | #### Install dependencies
|
788 |
|
789 | $ npm install
|
790 |
|
791 | #### Build
|
792 |
|
793 | $ npm run demo.build
|
794 |
|
795 | #### Run Demo
|
796 |
|
797 | $ npm run start
|