1 | # ArtGen
|
2 |
|
3 | [](https://travis-ci.com/kevinbai0/ArtGen) [](http://img.badgesize.io/https://unpkg.com/artgenjs?compression=gzip) [](https://packagephobia.now.sh/result?p=artgenjs)
|
4 |
|
5 | ArtGen is a library that's meant to provide an easy way for both developers and artists create visual generative art on the web by taking a declarative and partly functional approach that abstracts away the messy of the code.
|
6 |
|
7 | The goal of ArtGen is to provide a lightweight and fast library. No dependencies were used to keep the final build as small as possible.
|
8 |
|
9 | 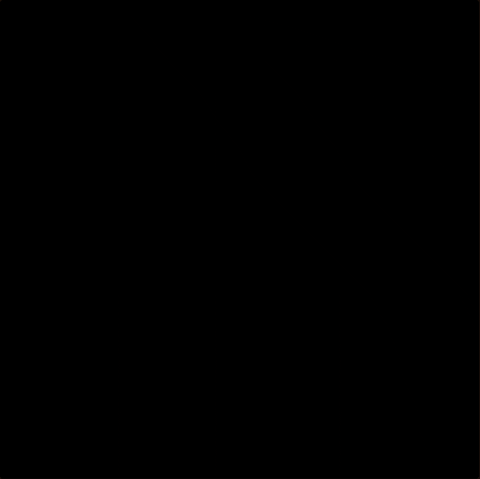
|
10 |
|
11 | Note: this project is currently still in development so the current release may be broken.
|
12 |
|
13 | ## What it looks like (in code)
|
14 |
|
15 | ```javascript
|
16 |
|
17 | const example = ({unwrap, rgba}) => {
|
18 | // define constants
|
19 | const constants = [
|
20 | 2.553308700841444,
|
21 | 1.3112688558630707,
|
22 | 1.781073930670376,
|
23 | 1.2974055728293994
|
24 | ]
|
25 | /*const constants = [
|
26 | unwrap([1,3]),
|
27 | unwrap([1,3]),
|
28 | unwrap([1,3]),
|
29 | unwrap([1,3])
|
30 | ]*/
|
31 | console.log(constants)
|
32 |
|
33 | // define helper functions
|
34 | const calcX = (x, y, c) =>
|
35 | Math.sin(2 * Math.sin(x) * Math.cos(c[0] * x - y * c[2])) +
|
36 | c[2] * Math.sin(1 * Math.cos(c[0] * x * c[3]))
|
37 | const calcY = (x, y, c) =>
|
38 | Math.sin(Math.PI * Math.cos(c[1] * x)) +
|
39 | c[3] * Math.sin((1 / Math.E) * c[1] * y)
|
40 |
|
41 | // init x,y values
|
42 | let x = 0.1, y = -0.1
|
43 |
|
44 | // Run every frame for animation
|
45 | const draw = value =>
|
46 | [...Array(1000)].map((_, i) => {
|
47 | x = calcX(x, y, constants)
|
48 | y = calcY(x, y, constants)
|
49 | const fill = rgba(
|
50 | Math.max(200 - x / 2, 0), // red
|
51 | (i / 1000) * 250, // green
|
52 | y * 200, // blue
|
53 | 0.1 // alpha
|
54 | )
|
55 |
|
56 | return ArtGen.utils.GenPoint(x * 150, y * 150, {
|
57 | fill,
|
58 | zIndex: i,
|
59 | radius: 1
|
60 | })
|
61 | })
|
62 |
|
63 | return {
|
64 | draw,
|
65 | iterate: value => value + 1,
|
66 | endIf: duration => duration >= 10000
|
67 | }
|
68 | }
|
69 | ```
|
70 |
|
71 | ## Installation
|
72 |
|
73 | If you’re using npm:
|
74 |
|
75 | ```bash
|
76 | npm install artgenjs
|
77 | ```
|
78 |
|
79 | or include it as a `script` in your HTML file:
|
80 |
|
81 | ```javascript
|
82 | <script src="https://unpkg.com/artgenjs"></script>
|
83 | ```
|