1 | # blessed
|
2 |
|
3 | A curses-like library with a high level terminal interface API for node.js.
|
4 |
|
5 | 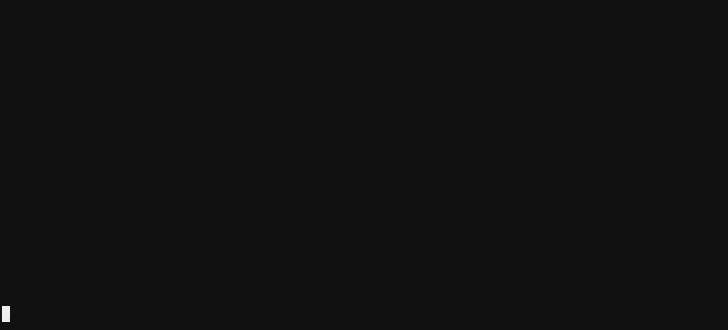
|
6 |
|
7 | Blessed is over 16,000 lines of code and terminal goodness. It's completely
|
8 | implemented in javascript, and its goal consists of two things:
|
9 |
|
10 | 1. Reimplement ncurses entirely by parsing and compiling terminfo and termcap,
|
11 | and exposing a `Program` object which can output escape sequences compatible
|
12 | with _any_ terminal.
|
13 |
|
14 | 2. Implement a widget API which is heavily optimized for terminals.
|
15 |
|
16 | The blessed renderer makes use of CSR (change-scroll-region), and BCE
|
17 | (back-color-erase). It draws the screen using the painter's algorithm and is
|
18 | sped up with smart cursor movements and a screen damage buffer. This means
|
19 | rendering of your application will be extremely efficient: blessed only draws
|
20 | the changes (damage) to the screen.
|
21 |
|
22 | Blessed is arguably as accurate as ncurses, but even more optimized in some
|
23 | ways. The widget library gives you an API which is reminiscent of the DOM.
|
24 | Anyone is able to make an awesome terminal application with blessed. There are
|
25 | terminal widget libraries for other platforms (primarily [python][urwid] and
|
26 | [perl][curses-ui]), but blessed is possibly the most DOM-like (dare I say the
|
27 | most user-friendly?).
|
28 |
|
29 | Blessed has been used to implement other popular libraries and programs.
|
30 | Examples include: the [slap text editor][slap] and [blessed-contrib][contrib].
|
31 | The blessed API itself has gone on to inspire [termui][termui] for Go.
|
32 |
|
33 | ## Important Blessed Changes (>0.0.51)
|
34 |
|
35 | - The absolute `.left` _property_ (not option) has been renamed to `.aleft`.
|
36 | The `.rleft` property has been renamed to `.left`. This should not have much
|
37 | effect on most applications. This includes all other coordinate properties.
|
38 | - `autoPadding` is now enabled by default. To revert to the original behavior,
|
39 | pass `autoPadding: false` into the screen object. That being said, it would
|
40 | be wiser to adjust your code to use `autoPadding`. non-`autoPadding` is now
|
41 | considered deprecated.
|
42 |
|
43 | ## Install
|
44 |
|
45 | ``` bash
|
46 | $ npm install blessed
|
47 | ```
|
48 |
|
49 | ## Example
|
50 |
|
51 | This will render a box with line borders containing the text `'Hello world!'`,
|
52 | perfectly centered horizontally and vertically.
|
53 |
|
54 | __NOTE__: It is recommend you use either `smartCSR` or `fastCSR` as a
|
55 | `blessed.screen` option. `autoPadding` is also recommended; it will
|
56 | automatically offset box content within borders instead of on top of them when
|
57 | coords are `0`. non-`autoPadding` _may_ be deprecated in the future. See the
|
58 | API documentation for further explanation of these options.
|
59 |
|
60 | ``` js
|
61 | var blessed = require('blessed');
|
62 |
|
63 | // Create a screen object.
|
64 | var screen = blessed.screen({
|
65 | autoPadding: true,
|
66 | smartCSR: true
|
67 | });
|
68 |
|
69 | screen.title = 'my window title';
|
70 |
|
71 | // Create a box perfectly centered horizontally and vertically.
|
72 | var box = blessed.box({
|
73 | top: 'center',
|
74 | left: 'center',
|
75 | width: '50%',
|
76 | height: '50%',
|
77 | content: 'Hello {bold}world{/bold}!',
|
78 | tags: true,
|
79 | border: {
|
80 | type: 'line'
|
81 | },
|
82 | style: {
|
83 | fg: 'white',
|
84 | bg: 'magenta',
|
85 | border: {
|
86 | fg: '#f0f0f0'
|
87 | },
|
88 | hover: {
|
89 | bg: 'green'
|
90 | }
|
91 | }
|
92 | });
|
93 |
|
94 | // Append our box to the screen.
|
95 | screen.append(box);
|
96 |
|
97 | // Add a PNG icon to the box (X11 only)
|
98 | var icon = blessed.image({
|
99 | parent: box,
|
100 | top: 0,
|
101 | left: 0,
|
102 | width: 'shrink',
|
103 | height: 'shrink',
|
104 | file: __dirname + '/my-program-icon.png',
|
105 | search: false
|
106 | });
|
107 |
|
108 | // If our box is clicked, change the content.
|
109 | box.on('click', function(data) {
|
110 | box.setContent('{center}Some different {red-fg}content{/red-fg}.{/center}');
|
111 | screen.render();
|
112 | });
|
113 |
|
114 | // If box is focused, handle `enter`/`return` and give us some more content.
|
115 | box.key('enter', function(ch, key) {
|
116 | box.setContent('{right}Even different {black-fg}content{/black-fg}.{/right}\n');
|
117 | box.setLine(1, 'bar');
|
118 | box.insertLine(1, 'foo');
|
119 | screen.render();
|
120 | });
|
121 |
|
122 | // Quit on Escape, q, or Control-C.
|
123 | screen.key(['escape', 'q', 'C-c'], function(ch, key) {
|
124 | return process.exit(0);
|
125 | });
|
126 |
|
127 | // Focus our element.
|
128 | box.focus();
|
129 |
|
130 | // Render the screen.
|
131 | screen.render();
|
132 | ```
|
133 |
|
134 |
|
135 | ## API Documentation
|
136 |
|
137 | ### Widgets
|
138 |
|
139 | Blessed comes with a number of high-level widgets so you can avoid all the
|
140 | nasty low-level terminal stuff.
|
141 |
|
142 | - [Node](#node-from-eventemitter)
|
143 | - [Screen](#screen-from-node)
|
144 | - [Element](#element-from-node)
|
145 | - [Box](#box-from-element)
|
146 | - [Text](#text-from-element)
|
147 | - [Line](#line-from-box)
|
148 | - [ScrollableBox](#scrollablebox-from-box)
|
149 | - [ScrollableText](#scrollabletext-from-scrollablebox)
|
150 | - [List](#list-from-box)
|
151 | - [Form](#form-from-box)
|
152 | - [Input](#input-from-box)
|
153 | - [Textarea](#textarea-from-input)
|
154 | - [Textbox](#textbox-from-textarea)
|
155 | - [Button](#button-from-input)
|
156 | - [ProgressBar](#progressbar-from-input)
|
157 | - [FileManager](#filemanager-from-list)
|
158 | - [Checkbox](#checkbox-from-input)
|
159 | - [RadioSet](#radioset-from-box)
|
160 | - [RadioButton](#radiobutton-from-checkbox)
|
161 | - [Prompt](#prompt-from-box)
|
162 | - [Question](#question-from-box)
|
163 | - [Message](#message-from-box)
|
164 | - [Loading](#loading-from-box)
|
165 | - [Listbar](#listbar-from-box)
|
166 | - [Log](#log-from-scrollabletext)
|
167 | - [Table](#table-from-box)
|
168 | - [ListTable](#listtable-from-list)
|
169 | - [Terminal](#terminal-from-box)
|
170 | - [Image](#image-from-box)
|
171 |
|
172 |
|
173 | #### Node (from EventEmitter)
|
174 |
|
175 | The base node which everything inherits from.
|
176 |
|
177 | ##### Options:
|
178 |
|
179 | - __screen__ - the screen to be associated with.
|
180 | - __parent__ - the desired parent.
|
181 | - __children__ - an arrray of children.
|
182 |
|
183 | ##### Properties:
|
184 |
|
185 | - inherits all from EventEmitter.
|
186 | - __type__ - type of the node (e.g. `box`).
|
187 | - __options__ - original options object.
|
188 | - __parent__ - parent node.
|
189 | - __screen__ - parent screen.
|
190 | - __children__ - array of node's children.
|
191 | - __data, _, $__ - an object for any miscellanous user data.
|
192 | - __index__ - render index (document order index) of the last render call.
|
193 |
|
194 | ##### Events:
|
195 |
|
196 | - inherits all from EventEmitter.
|
197 | - __adopt__ - received when node is added to a parent.
|
198 | - __remove__ - received when node is removed from it's current parent.
|
199 | - __reparent__ - received when node gains a new parent.
|
200 | - __attach__ - received when node is attached to the screen directly or
|
201 | somewhere in its ancestry.
|
202 | - __detach__ - received when node is detached from the screen directly or
|
203 | somewhere in its ancestry.
|
204 |
|
205 | ##### Methods:
|
206 |
|
207 | - inherits all from EventEmitter.
|
208 | - __prepend(node)__ - prepend a node to this node's children.
|
209 | - __append(node)__ - append a node to this node's children.
|
210 | - __remove(node)__ - remove child node from node.
|
211 | - __insert(node, i)__ - insert a node to this node's children at index `i`.
|
212 | - __insertBefore(node, refNode)__ - insert a node to this node's children
|
213 | before the reference node.
|
214 | - __insertAfter(node, refNode)__ - insert a node from node after the reference
|
215 | node.
|
216 | - __detach()__ - remove node from its parent.
|
217 | - __emitDescendants(type, args..., [iterator])__ - emit event for element, and
|
218 | recursively emit same event for all descendants.
|
219 | - __get(name, [default])__ - get user property with a potential default value.
|
220 | - __set(name, value)__ - set user property to value.
|
221 |
|
222 |
|
223 | #### Screen (from Node)
|
224 |
|
225 | The screen on which every other node renders.
|
226 |
|
227 | ##### Options:
|
228 |
|
229 | - __program__ - the blessed `Program` to be associated with. will be
|
230 | automatically instantiated if none is provided.
|
231 | - __smartCSR__ - attempt to perform CSR optimization on all possible elements
|
232 | (not just full-width ones, elements with uniform cells to their sides).
|
233 | this is known to cause flickering with elements that are not full-width,
|
234 | however, it is more optimal for terminal rendering.
|
235 | - __fastCSR__ - do CSR on any element within 20 cols of the screen edge on
|
236 | either side. faster than `smartCSR`, but may cause flickering depending on
|
237 | what is on each side of the element.
|
238 | - __useBCE__ - attempt to perform `back_color_erase` optimizations for terminals
|
239 | that support it. it will also work with terminals that don't support it, but
|
240 | only on lines with the default background color. as it stands with the current
|
241 | implementation, it's uncertain how much terminal performance this adds at the
|
242 | cost of overhead within node.
|
243 | - __resizeTimeout__ - amount of time (in ms) to redraw the screen after the
|
244 | terminal is resized (default: 300).
|
245 | - __tabSize__ - the width of tabs within an element's content.
|
246 | - __autoPadding__ - automatically position child elements with border and
|
247 | padding in mind (__NOTE__: this is a recommended option. it may become
|
248 | default in the future).
|
249 | - __artificialCursor__ - have blessed draw a custom cursor and hide the
|
250 | terminal cursor (__experimental__).
|
251 | - __cursorShape__ - shape of the artificial cursor. can be: block, underline,
|
252 | or line.
|
253 | - __cursorBlink__ - whether the artificial cursor blinks.
|
254 | - __cursorColor__ - color of the artificial color. accepts any valid color
|
255 | value (`null` is default).
|
256 | - __log__ - create a log file. see `log` method.
|
257 | - __dump__ - dump all output and input to desired file. can be used together
|
258 | with `log` option if set as a boolean.
|
259 | - __debug__ - debug mode. enables usage of the `debug` method. also creates a
|
260 | debug console which will display when pressing F12. it will display all log
|
261 | and debug messages.
|
262 | - __ignoreLocked__ - Array of keys in their full format (e.g. `C-c`) to ignore
|
263 | when keys are locked. Useful for creating a key that will _always_ exit no
|
264 | matter whether the keys are locked.
|
265 | - __dockBorders__ - automatically "dock" borders with other elements instead of
|
266 | overlapping, depending on position (__experimental__). for example:
|
267 | These border-overlapped elements:
|
268 | ```
|
269 | ┌─────────┌─────────┐
|
270 | │ box1 │ box2 │
|
271 | └─────────└─────────┘
|
272 | ```
|
273 | Become:
|
274 | ```
|
275 | ┌─────────┬─────────┐
|
276 | │ box1 │ box2 │
|
277 | └─────────┴─────────┘
|
278 | ```
|
279 | - __ignoreDockContrast__ - normally, dockable borders will not dock if the
|
280 | colors or attributes are different. this option will allow them to dock
|
281 | regardless. it may produce some odd looking multi-colored borders though.
|
282 | - __fullUnicode__ - allow for rendering of East Asian double-width characters,
|
283 | utf-16 surrogate pairs, and unicode combining characters. this allows you to
|
284 | display text above the basic multilingual plane. this is behind an option
|
285 | because it may affect performance slightly negatively. without this option
|
286 | enabled, all double-width, surrogate pair, and combining characters will be
|
287 | replaced by `'??'`, `'?'`, `''` respectively. (NOTE: iTerm2 cannot display
|
288 | combining characters properly. blessed simply removes them from an element's
|
289 | content if iTerm2 is detected).
|
290 |
|
291 | ##### Properties:
|
292 |
|
293 | - inherits all from Node.
|
294 | - __program__ - the blessed Program object.
|
295 | - __tput__ - the blessed Tput object (only available if you passed `tput: true`
|
296 | to the Program constructor.)
|
297 | - __focused__ - top of the focus history stack.
|
298 | - __width__ - width of the screen (same as `program.cols`).
|
299 | - __height__ - height of the screen (same as `program.rows`).
|
300 | - __cols__ - same as `screen.width`.
|
301 | - __rows__ - same as `screen.height`.
|
302 | - __left__ - relative left offset, always zero.
|
303 | - __right__ - relative right offset, always zero.
|
304 | - __top__ - relative top offset, always zero.
|
305 | - __bottom__ - relative bottom offset, always zero.
|
306 | - __aleft__ - absolute left offset, always zero.
|
307 | - __aright__ - absolute right offset, always zero.
|
308 | - __atop__ - absolute top offset, always zero.
|
309 | - __abottom__ - absolute bottom offset, always zero.
|
310 | - __grabKeys__ - whether the focused element grabs all keypresses.
|
311 | - __lockKeys__ - prevent keypresses from being received by any element.
|
312 | - __hover__ - the currently hovered element. only set if mouse events are bound.
|
313 | - __title__ - set or get window title.
|
314 |
|
315 | ##### Events:
|
316 |
|
317 | - inherits all from Node.
|
318 | - __resize__ - received on screen resize.
|
319 | - __mouse__ - received on mouse events.
|
320 | - __keypress__ - received on key events.
|
321 | - __element [name]__ - global events received for all elements.
|
322 | - __key [name]__ - received on key event for [name].
|
323 | - __focus, blur__ - received when the terminal window focuses/blurs. requires a
|
324 | terminal supporting the focus protocol and focus needs to be passed to
|
325 | program.enableMouse().
|
326 | - __prerender__ - received before render.
|
327 | - __render__ - received on render.
|
328 |
|
329 | ##### Methods:
|
330 |
|
331 | - inherits all from Node.
|
332 | - __log(msg, ...)__ - write string to the log file if one was created.
|
333 | - __debug(msg, ...)__ - same as the log method, but only gets called if the
|
334 | `debug` option was set.
|
335 | - __alloc()__ - allocate a new pending screen buffer and a new output screen
|
336 | buffer.
|
337 | - __draw(start, end)__ - draw the screen based on the contents of the screen
|
338 | buffer.
|
339 | - __render()__ - render all child elements, writing all data to the screen
|
340 | buffer and drawing the screen.
|
341 | - __clearRegion(x1, x2, y1, y2)__ - clear any region on the screen.
|
342 | - __fillRegion(attr, ch, x1, x2, y1, y2)__ - fill any region with a character
|
343 | of a certain attribute.
|
344 | - __focusOffset(offset)__ - focus element by offset of focusable elements.
|
345 | - __focusPrevious()__ - focus previous element in the index.
|
346 | - __focusNext()__ - focus next element in the index.
|
347 | - __focusPush(element)__ - push element on the focus stack (equivalent to
|
348 | `screen.focused = el`).
|
349 | - __focusPop()__ - pop element off the focus stack.
|
350 | - __saveFocus()__ - save the focused element.
|
351 | - __restoreFocus()__ - restore the saved focused element.
|
352 | - __rewindFocus()__ - "rewind" focus to the last visible and attached element.
|
353 | - __key(name, listener)__ - bind a keypress listener for a specific key.
|
354 | - __onceKey(name, listener)__ - bind a keypress listener for a specific key
|
355 | once.
|
356 | - __unkey(name, listener)__ - remove a keypress listener for a specific key.
|
357 | - __spawn(file, args, options)__ - spawn a process in the foreground, return to
|
358 | blessed app after exit.
|
359 | - __exec(file, args, options, callback)__ - spawn a process in the foreground,
|
360 | return to blessed app after exit. executes callback on error or exit.
|
361 | - __readEditor([options], callback)__ - read data from text editor.
|
362 | - __setEffects(el, fel, over, out, effects, temp)__ - set effects based on
|
363 | two events and attributes.
|
364 | - __insertLine(n, y, top, bottom)__ - insert a line into the screen (using csr:
|
365 | this bypasses the output buffer).
|
366 | - __deleteLine(n, y, top, bottom)__ - delete a line from the screen (using csr:
|
367 | this bypasses the output buffer).
|
368 | - __insertBottom(top, bottom)__ - insert a line at the bottom of the screen.
|
369 | - __insertTop(top, bottom)__ - insert a line at the top of the screen.
|
370 | - __deleteBottom(top, bottom)__ - delete a line at the bottom of the screen.
|
371 | - __deleteTop(top, bottom)__ - delete a line at the top of the screen.
|
372 | - __enableMouse([el])__ - enable mouse events for the screen and optionally an element (automatically called when a form of on('mouse') is bound).
|
373 | - __enableKeys([el])__ - enable keypress events for the screen and optionally an element (automatically called when a form of on('keypress') is bound).
|
374 | - __enableInput([el])__ - enable key and mouse events. calls bot enableMouse and enableKeys.
|
375 | - __copyToClipboard(text)__ - attempt to copy text to clipboard using iTerm2's
|
376 | proprietary sequence. returns true if successful.
|
377 | - __cursorShape(shape, blink)__ - attempt to change cursor shape. will not work
|
378 | in all terminals (see artificial cursors for a solution to this). returns
|
379 | true if successful.
|
380 | - __cursorColor(color)__ - attempt to change cursor color. returns true if
|
381 | successful.
|
382 | - __cursorReset()__ - attempt to reset cursor. returns true if successful.
|
383 | - __screenshot([xi, xl, yi, yl])__ - take an SGR screenshot of the screen
|
384 | within the region. returns a string containing only characters and SGR codes.
|
385 | can be displayed by simply echoing it in a terminal.
|
386 |
|
387 |
|
388 | #### Element (from Node)
|
389 |
|
390 | The base element.
|
391 |
|
392 | ##### Options:
|
393 |
|
394 | - __fg, bg, bold, underline__ - attributes.
|
395 | - __style__ - may contain attributes in the format of:
|
396 | ``` js
|
397 | {
|
398 | fg: 'blue',
|
399 | bg: 'black',
|
400 | border: {
|
401 | fg: 'blue'
|
402 | },
|
403 | scrollbar: {
|
404 | bg: 'blue'
|
405 | },
|
406 | focus: {
|
407 | bg: 'red'
|
408 | },
|
409 | hover: {
|
410 | bg: 'red'
|
411 | }
|
412 | }
|
413 | ```
|
414 | - __border__ - border object, see below.
|
415 | - __content__ - element's text content.
|
416 | - __clickable__ - element is clickable.
|
417 | - __input__ - element is focusable and can receive key input.
|
418 | - __focused__ - element is focused.
|
419 | - __hidden__ - whether the element is hidden.
|
420 | - __label__ - a simple text label for the element.
|
421 | - __hoverText__ - a floating text label for the element which appears on mouseover.
|
422 | - __align__ - text alignment: `left`, `center`, or `right`.
|
423 | - __valign__ - vertical text alignment: `top`, `middle`, or `bottom`.
|
424 | - __shrink__ - shrink/flex/grow to content and child elements. width/height
|
425 | during render.
|
426 | - __padding__ - amount of padding on the inside of the element. can be a number
|
427 | or an object containing the properties: `left`, `right`, `top`, and `bottom`.
|
428 | - __width, height__ - width/height of the element, can be a number, percentage
|
429 | (`0-100%`), or keyword (`half` or `shrink`). percentages can also have
|
430 | offsets (`50%+1`, `50%-1`).
|
431 | - __left, right, top, bottom__ - offsets of the element __relative to its
|
432 | parent__. can be a number, percentage (`0-100%`), or keyword (`center`).
|
433 | `right` and `bottom` do not accept keywords. percentages can also have
|
434 | offsets (`50%+1`, `50%-1`).
|
435 | - __position__ - can contain the above options.
|
436 | - __scrollable__ - whether the element is scrollable or not.
|
437 | - __ch__ - background character (default is whitespace ` `).
|
438 | - __draggable__ - allow the element to be dragged with the mouse.
|
439 | - __shadow__ - draw a translucent offset shadow behind the element.
|
440 |
|
441 | ##### Properties:
|
442 |
|
443 | - inherits all from Node.
|
444 | - __name__ - name of the element. useful for form submission.
|
445 | - __border__ - border object.
|
446 | - __type__ - type of border (`line` or `bg`). `bg` by default.
|
447 | - __ch__ - character to use if `bg` type, default is space.
|
448 | - __bg, fg__ - border foreground and background, must be numbers (-1 for
|
449 | default).
|
450 | - __bold, underline__ - border attributes.
|
451 | - __style__ - contains attributes (e.g. `fg/bg/underline`). see above.
|
452 | - __position__ - raw width, height, and offsets.
|
453 | - __content__ - raw text content.
|
454 | - __hidden__ - whether the element is hidden or not.
|
455 | - __visible__ - whether the element is visible or not.
|
456 | - __detached__ - whether the element is attached to a screen in its ancestry
|
457 | somewhere.
|
458 | - __fg, bg__ - foreground and background, must be numbers (-1 for default).
|
459 | - __bold, underline__ - attributes.
|
460 | - __width__ - calculated width.
|
461 | - __height__ - calculated height.
|
462 | - __left__ - calculated relative left offset.
|
463 | - __right__ - calculated relative right offset.
|
464 | - __top__ - calculated relative top offset.
|
465 | - __bottom__ - calculated relative bottom offset.
|
466 | - __aleft__ - calculated absolute left offset.
|
467 | - __aright__ - calculated absolute right offset.
|
468 | - __atop__ - calculated absolute top offset.
|
469 | - __abottom__ - calculated absolute bottom offset.
|
470 | - __draggable__ - whether the element is draggable. set to true to allow
|
471 | dragging.
|
472 |
|
473 | ##### Events:
|
474 |
|
475 | - inherits all from Node.
|
476 | - __blur, focus__ - received when an element is focused or unfocused.
|
477 | - __mouse__ - received on mouse events for this element.
|
478 | - __mousedown, mouseup__ - mouse button was pressed or released.
|
479 | - __wheeldown, wheelup__ - wheel was scrolled down or up.
|
480 | - __mouseover, mouseout__ - element was hovered or unhovered.
|
481 | - __mousemove__ - mouse was moved somewhere on this element.
|
482 | - __click__ - element was clicked (slightly smarter than mouseup).
|
483 | - __keypress__ - received on key events for this element.
|
484 | - __move__ - received when the element is moved.
|
485 | - __resize__ - received when the element is resized.
|
486 | - __key [name]__ - received on key event for [name].
|
487 | - __prerender__ - received before a call to render.
|
488 | - __render__ - received after a call to render.
|
489 |
|
490 | ##### Methods:
|
491 |
|
492 | - inherits all from Node.
|
493 | - note: if the `scrollable` option is enabled, Element inherits all methods
|
494 | from ScrollableBox.
|
495 | - __render()__ - write content and children to the screen buffer.
|
496 | - __hide()__ - hide element.
|
497 | - __show()__ - show element.
|
498 | - __toggle()__ - toggle hidden/shown.
|
499 | - __focus()__ - focus element.
|
500 | - __key(name, listener)__ - bind a keypress listener for a specific key.
|
501 | - __onceKey(name, listener)__ - bind a keypress listener for a specific key
|
502 | once.
|
503 | - __unkey(name, listener)__ - remove a keypress listener for a specific key.
|
504 | - __onScreenEvent(type, handler)__ - same as`el.on('screen', ...)` except this
|
505 | will automatically keep track of which listeners are bound to the screen
|
506 | object. for use with `removeScreenEvent()`, `free()`, and `destroy()`.
|
507 | - __removeScreenEvent(type, handler)__ - same as`el.removeListener('screen',
|
508 | ...)` except this will automatically keep track of which listeners are bound
|
509 | to the screen object. for use with `onScreenEvent()`, `free()`, and
|
510 | `destroy()`.
|
511 | - __free()__ - free up the element. automatically unbind all events that may
|
512 | have been bound to the screen object. this prevents memory leaks. for use
|
513 | with `onScreenEvent()`, `removeScreenEvent()`, and `destroy()`.
|
514 | - __destroy()__ - same as the `detach()` method, except this will automatically
|
515 | call `free()` and unbind any screen events to prevent memory leaks. for use
|
516 | with `onScreenEvent()`, `removeScreenEvent()`, and `free()`.
|
517 | - __setIndex(z)__ - set the z-index of the element (changes rendering order).
|
518 | - __setFront()__ - put the element in front of its siblings.
|
519 | - __setBack()__ - put the element in back of its siblings.
|
520 | - __setLabel(text/options)__ - set the label text for the top-left corner.
|
521 | example options: `{text:'foo',side:'left'}`
|
522 | - __removeLabel()__ - remove the label completely.
|
523 | - __setHover(text/options)__ - set a hover text box to follow the cursor.
|
524 | similar to the "title" DOM attribute in the browser.
|
525 | example options: `{text:'foo'}`
|
526 | - __removeHover()__ - remove the hover label completely.
|
527 | - __enableMouse()__ - enable mouse events for the element (automatically called when a form of on('mouse') is bound).
|
528 | - __enableKeys()__ - enable keypress events for the element (automatically called when a form of on('keypress') is bound).
|
529 | - __enableInput()__ - enable key and mouse events. calls bot enableMouse and enableKeys.
|
530 | - __enableDrag()__ - enable dragging of the element.
|
531 | - __disableDrag()__ - disable dragging of the element.
|
532 | - __screenshot([xi, xl, yi, yl])__ - take an SGR screenshot of the element
|
533 | within the region. returns a string containing only characters and SGR codes.
|
534 | can be displayed by simply echoing it in a terminal.
|
535 |
|
536 | ###### Content Methods
|
537 |
|
538 | Methods for dealing with text content, line by line. Useful for writing a
|
539 | text editor, irc client, etc.
|
540 |
|
541 | Note: all of these methods deal with pre-aligned, pre-wrapped text. If you use
|
542 | deleteTop() on a box with a wrapped line at the top, it may remove 3-4 "real"
|
543 | lines (rows) depending on how long the original line was.
|
544 |
|
545 | The `lines` parameter can be a string or an array of strings. The `line`
|
546 | parameter must be a string.
|
547 |
|
548 | - __setContent(text)__ - set the content. note: when text is input, it will be
|
549 | stripped of all non-SGR escape codes, tabs will be replaced with 8 spaces,
|
550 | and tags will be replaced with SGR codes (if enabled).
|
551 | - __getContent()__ - return content, slightly different from `el.content`.
|
552 | assume the above formatting.
|
553 | - __setText(text)__ - similar to `setContent`, but ignore tags and remove escape
|
554 | codes.
|
555 | - __getText()__ - similar to `getContent`, but return content with tags and
|
556 | escape codes removed.
|
557 | - __insertLine(i, lines)__ - insert a line into the box's content.
|
558 | - __deleteLine(i)__ - delete a line from the box's content.
|
559 | - __getLine(i)__ - get a line from the box's content.
|
560 | - __getBaseLine(i)__ - get a line from the box's content from the visible top.
|
561 | - __setLine(i, line)__ - set a line in the box's content.
|
562 | - __setBaseLine(i, line)__ - set a line in the box's content from the visible
|
563 | top.
|
564 | - __clearLine(i)__ - clear a line from the box's content.
|
565 | - __clearBaseLine(i)__ - clear a line from the box's content from the visible
|
566 | top.
|
567 | - __insertTop(lines)__ - insert a line at the top of the box.
|
568 | - __insertBottom(lines)__ - insert a line at the bottom of the box.
|
569 | - __deleteTop()__ - delete a line at the top of the box.
|
570 | - __deleteBottom()__ - delete a line at the bottom of the box.
|
571 | - __unshiftLine(lines)__ - unshift a line onto the top of the content.
|
572 | - __shiftLine(i)__ - shift a line off the top of the content.
|
573 | - __pushLine(lines)__ - push a line onto the bottom of the content.
|
574 | - __popLine(i)__ - pop a line off the bottom of the content.
|
575 | - __getLines()__ - an array containing the content lines.
|
576 | - __getScreenLines()__ - an array containing the lines as they are displayed on
|
577 | the screen.
|
578 | - __strWidth(text)__ - get a string's displayed width, taking into account
|
579 | double-width, surrogate pairs, combining characters, tags, and SGR escape
|
580 | codes.
|
581 |
|
582 |
|
583 | #### Box (from Element)
|
584 |
|
585 | A box element which draws a simple box containing `content` or other elements.
|
586 |
|
587 | ##### Options:
|
588 |
|
589 | - inherits all from Element.
|
590 |
|
591 | ##### Properties:
|
592 |
|
593 | - inherits all from Element.
|
594 |
|
595 | ##### Events:
|
596 |
|
597 | - inherits all from Element.
|
598 |
|
599 | ##### Methods:
|
600 |
|
601 | - inherits all from Element.
|
602 |
|
603 |
|
604 | #### Text (from Element)
|
605 |
|
606 | An element similar to Box, but geared towards rendering simple text elements.
|
607 |
|
608 | ##### Options:
|
609 |
|
610 | - inherits all from Element.
|
611 | - __fill__ - fill the entire line with chosen bg until parent bg ends, even if
|
612 | there is not enough text to fill the entire width. __(deprecated)__
|
613 | - __align__ - text alignment: `left`, `center`, or `right`.
|
614 |
|
615 | Inherits all options, properties, events, and methods from Element.
|
616 |
|
617 |
|
618 | #### Line (from Box)
|
619 |
|
620 | A simple line which can be `line` or `bg` styled.
|
621 |
|
622 | ##### Options:
|
623 |
|
624 | - inherits all from Box.
|
625 | - __orientation__ - can be `vertical` or `horizontal`.
|
626 | - __type, bg, fg, ch__ - treated the same as a border object.
|
627 | (attributes can be contained in `style`).
|
628 |
|
629 | Inherits all options, properties, events, and methods from Box.
|
630 |
|
631 |
|
632 | #### ScrollableBox (from Box)
|
633 |
|
634 | __DEPRECATED__ - Use Box with the `scrollable` option instead.
|
635 |
|
636 | A box with scrollable content.
|
637 |
|
638 | ##### Options:
|
639 |
|
640 | - inherits all from Box.
|
641 | - __baseLimit__ - a limit to the childBase. default is `Infinity`.
|
642 | - __alwaysScroll__ - a option which causes the ignoring of `childOffset`. this
|
643 | in turn causes the childBase to change every time the element is scrolled.
|
644 | - __scrollbar__ - object enabling a scrollbar.
|
645 | - __scrollbar.style__ - style of the scrollbar.
|
646 | - __scrollbar.track__ - style of the scrollbar track if present (takes regular
|
647 | style options).
|
648 |
|
649 | ##### Properties:
|
650 |
|
651 | - inherits all from Box.
|
652 | - __childBase__ - the offset of the top of the scroll content.
|
653 | - __childOffset__ - the offset of the chosen item/line.
|
654 |
|
655 | ##### Events:
|
656 |
|
657 | - inherits all from Box.
|
658 | - __scroll__ - received when the element is scrolled.
|
659 |
|
660 | ##### Methods:
|
661 |
|
662 | - __scroll(offset)__ - scroll the content by a relative offset.
|
663 | - __scrollTo(index)__ - scroll the content to an absolute index.
|
664 | - __setScroll(index)__ - same as `scrollTo`.
|
665 | - __setScrollPerc(perc)__ - set the current scroll index in percentage (0-100).
|
666 | - __getScroll()__ - get the current scroll index in lines.
|
667 | - __getScrollHeight()__ - get the actual height of the scrolling area.
|
668 | - __getScrollPerc()__ - get the current scroll index in percentage.
|
669 | - __resetScroll()__ - reset the scroll index to its initial state.
|
670 |
|
671 |
|
672 | #### ScrollableText (from ScrollableBox)
|
673 |
|
674 | __DEPRECATED__ - Use Box with the `scrollable` and `alwaysScroll` options
|
675 | instead.
|
676 |
|
677 | A scrollable text box which can display and scroll text, as well as handle
|
678 | pre-existing newlines and escape codes.
|
679 |
|
680 | ##### Options:
|
681 |
|
682 | - inherits all from ScrollableBox.
|
683 | - __mouse__ - whether to enable automatic mouse support for this element.
|
684 | - __keys__ - use predefined keys for navigating the text.
|
685 | - __vi__ - use vi keys with the `keys` option.
|
686 |
|
687 | ##### Properties:
|
688 |
|
689 | - inherits all from ScrollableBox.
|
690 |
|
691 | ##### Events:
|
692 |
|
693 | - inherits all from ScrollableBox.
|
694 |
|
695 | ##### Methods:
|
696 |
|
697 | - inherits all from ScrollableBox.
|
698 |
|
699 |
|
700 | #### List (from Box)
|
701 |
|
702 | A scrollable list which can display selectable items.
|
703 |
|
704 | ##### Options:
|
705 |
|
706 | - inherits all from Box.
|
707 | - __style.selected__ - style for a selected item.
|
708 | - __style.item__ - style for an unselected item.
|
709 | - __mouse__ - whether to automatically enable mouse support for this list
|
710 | (allows clicking items).
|
711 | - __keys__ - use predefined keys for navigating the list.
|
712 | - __vi__ - use vi keys with the `keys` option.
|
713 | - __items__ - an array of strings which become the list's items.
|
714 | - __search__ - a function that is called when `vi` mode is enabled and the key
|
715 | `/` is pressed. This function accepts a callback function which should be
|
716 | called with the search string. The search string is then used to jump to an
|
717 | item that is found in `items`.
|
718 | - __interactive__ - whether the list is interactive and can have items selected
|
719 | (default: true).
|
720 | - __invertSelected__ - whether to automatically override tags and invert fg of
|
721 | item when selected (default: `true`).
|
722 |
|
723 | ##### Properties:
|
724 |
|
725 | - inherits all from Box.
|
726 |
|
727 | ##### Events:
|
728 |
|
729 | - inherits all from Box.
|
730 | - __select__ - received when an item is selected.
|
731 | - __cancel__ - list was canceled (when `esc` is pressed with the `keys` option).
|
732 | - __action__ - either a select or a cancel event was received.
|
733 |
|
734 | ##### Methods:
|
735 |
|
736 | - inherits all from Box.
|
737 | - __add/addItem(text)__ - add an item based on a string.
|
738 | - __getItemIndex(child)__ - returns the item index from the list. child can be
|
739 | an element, index, or string.
|
740 | - __getItem(child)__ - returns the item element. child can be an element,
|
741 | index, or string.
|
742 | - __removeItem(child)__ - removes an item from the list. child can be an
|
743 | element, index, or string.
|
744 | - __clearItems()__ - clears all items from the list.
|
745 | - __setItems(items)__ - sets the list items to multiple strings.
|
746 | - __select(index)__ - select an index of an item.
|
747 | - __move(offset)__ - select item based on current offset.
|
748 | - __up(amount)__ - select item above selected.
|
749 | - __down(amount)__ - select item below selected.
|
750 | - __pick(callback)__ - show/focus list and pick an item. the callback is
|
751 | executed with the result.
|
752 | - __fuzzyFind([string/regex/callback])__ - find an item based on its text
|
753 | content.
|
754 |
|
755 |
|
756 | #### Form (from Box)
|
757 |
|
758 | A form which can contain form elements.
|
759 |
|
760 | ##### Options:
|
761 |
|
762 | - inherits all from Box.
|
763 | - __keys__ - allow default keys (tab, vi keys, enter).
|
764 | - __vi__ - allow vi keys.
|
765 |
|
766 | ##### Properties:
|
767 |
|
768 | - inherits all from Box.
|
769 | - __submission__ - last submitted data.
|
770 |
|
771 | ##### Events:
|
772 |
|
773 | - inherits all from Box.
|
774 | - __submit__ - form is submitted. receives a data object.
|
775 | - __cancel__ - form is discarded.
|
776 | - __reset__ - form is cleared.
|
777 |
|
778 | ##### Methods:
|
779 |
|
780 | - inherits all from Box.
|
781 | - __focusNext()__ - focus next form element.
|
782 | - __focusPrevious()__ - focus previous form element.
|
783 | - __submit()__ - submit the form.
|
784 | - __cancel()__ - discard the form.
|
785 | - __reset()__ - clear the form.
|
786 |
|
787 |
|
788 | #### Input (from Box)
|
789 |
|
790 | A form input.
|
791 |
|
792 |
|
793 | #### Textarea (from Input)
|
794 |
|
795 | A box which allows multiline text input.
|
796 |
|
797 | ##### Options:
|
798 |
|
799 | - inherits all from Input.
|
800 | - __keys__ - use pre-defined keys (`i` or `enter` for insert, `e` for editor,
|
801 | `C-e` for editor while inserting).
|
802 | - __mouse__ - use pre-defined mouse events (right-click for editor).
|
803 | - __inputOnFocus__ - call `readInput()` when the element is focused.
|
804 | automatically unfocus.
|
805 |
|
806 | ##### Properties:
|
807 |
|
808 | - inherits all from Input.
|
809 | - __value__ - the input text. __read-only__.
|
810 |
|
811 | ##### Events:
|
812 |
|
813 | - inherits all from Input.
|
814 | - __submit__ - value is submitted (enter).
|
815 | - __cancel__ - value is discared (escape).
|
816 | - __action__ - either submit or cancel.
|
817 |
|
818 | ##### Methods:
|
819 |
|
820 | - inherits all from Input.
|
821 | - __submit__ - submit the textarea (emits `submit`).
|
822 | - __cancel__ - cancel the textarea (emits `cancel`).
|
823 | - __readInput(callback)__ - grab key events and start reading text from the
|
824 | keyboard. takes a callback which receives the final value.
|
825 | - __readEditor(callback)__ - open text editor in `$EDITOR`, read the output from
|
826 | the resulting file. takes a callback which receives the final value.
|
827 | - __getValue()__ - the same as `this.value`, for now.
|
828 | - __clearValue()__ - clear input.
|
829 | - __setValue(text)__ - set value.
|
830 |
|
831 |
|
832 | #### Textbox (from Textarea)
|
833 |
|
834 | A box which allows text input.
|
835 |
|
836 | ##### Options:
|
837 |
|
838 | - inherits all from Textarea.
|
839 | - __secret__ - completely hide text.
|
840 | - __censor__ - replace text with asterisks (`*`).
|
841 |
|
842 | ##### Properties:
|
843 |
|
844 | - inherits all from Textarea.
|
845 | - __secret__ - completely hide text.
|
846 | - __censor__ - replace text with asterisks (`*`).
|
847 |
|
848 | ##### Events:
|
849 |
|
850 | - inherits all from Textarea.
|
851 |
|
852 | ##### Methods:
|
853 |
|
854 | - inherits all from Textarea.
|
855 |
|
856 |
|
857 | #### Button (from Input)
|
858 |
|
859 | A button which can be focused and allows key and mouse input.
|
860 |
|
861 | ##### Options:
|
862 |
|
863 | - inherits all from Input.
|
864 |
|
865 | ##### Properties:
|
866 |
|
867 | - inherits all from Input.
|
868 |
|
869 | ##### Events:
|
870 |
|
871 | - inherits all from Input.
|
872 | - __press__ - received when the button is clicked/pressed.
|
873 |
|
874 | ##### Methods:
|
875 |
|
876 | - inherits all from Input.
|
877 | - __press()__ - press button. emits `press`.
|
878 |
|
879 |
|
880 | #### ProgressBar (from Input)
|
881 |
|
882 | A progress bar allowing various styles. This can also be used as a form input.
|
883 |
|
884 | ##### Options:
|
885 |
|
886 | - inherits all from Input.
|
887 | - __orientation__ - can be `horizontal` or `vertical`.
|
888 | - __style.bar__ - style of the bar contents itself.
|
889 | - __pch__ - the character to fill the bar with (default is space).
|
890 | - __filled__ - the amount filled (0 - 100).
|
891 | - __value__ - same as `filled`.
|
892 | - __keys__ - enable key support.
|
893 | - __mouse__ - enable mouse support.
|
894 |
|
895 | ##### Properties:
|
896 |
|
897 | - inherits all from Input.
|
898 |
|
899 | ##### Events:
|
900 |
|
901 | - inherits all from Input.
|
902 | - __reset__ - bar was reset.
|
903 | - __complete__ - bar has completely filled.
|
904 |
|
905 | ##### Methods:
|
906 |
|
907 | - inherits all from Input.
|
908 | - __progress(amount)__ - progress the bar by a fill amount.
|
909 | - __setProgress(amount)__ - set progress to specific amount.
|
910 | - __reset()__ - reset the bar.
|
911 |
|
912 |
|
913 | #### FileManager (from List)
|
914 |
|
915 | A very simple file manager for selecting files.
|
916 |
|
917 | ##### Options:
|
918 |
|
919 | - inherits all from List.
|
920 | - __cwd__ - current working directory.
|
921 |
|
922 | ##### Properties:
|
923 |
|
924 | - inherits all from List.
|
925 | - __cwd__ - current working directory.
|
926 |
|
927 | ##### Events:
|
928 |
|
929 | - inherits all from List.
|
930 | - __cd__ - directory was selected and navigated to.
|
931 | - __file__ - file was selected.
|
932 |
|
933 | ##### Methods:
|
934 |
|
935 | - inherits all from List.
|
936 | - __refresh([cwd], [callback])__ - refresh the file list (perform a `readdir` on `cwd`
|
937 | and update the list items).
|
938 | - __pick([cwd], callback)__ - pick a single file and return the path in the callback.
|
939 | - __reset([cwd], [callback])__ - reset back to original cwd.
|
940 |
|
941 |
|
942 | #### Checkbox (from Input)
|
943 |
|
944 | A checkbox which can be used in a form element.
|
945 |
|
946 | ##### Options:
|
947 |
|
948 | - inherits all from Input.
|
949 | - __checked__ - whether the element is checked or not.
|
950 | - __mouse__ - enable mouse support.
|
951 |
|
952 | ##### Properties:
|
953 |
|
954 | - inherits all from Input.
|
955 | - __text__ - the text next to the checkbox (do not use setContent, use
|
956 | `check.text = ''`).
|
957 | - __checked__ - whether the element is checked or not.
|
958 | - __value__ - same as `checked`.
|
959 |
|
960 | ##### Events:
|
961 |
|
962 | - inherits all from Input.
|
963 | - __check__ - received when element is checked.
|
964 | - __uncheck__ received when element is unchecked.
|
965 |
|
966 | ##### Methods:
|
967 |
|
968 | - inherits all from Input.
|
969 | - __check()__ - check the element.
|
970 | - __uncheck()__ - uncheck the element.
|
971 | - __toggle()__ - toggle checked state.
|
972 |
|
973 |
|
974 | #### RadioSet (from Box)
|
975 |
|
976 | An element wrapping RadioButtons. RadioButtons within this element will be
|
977 | mutually exclusive with each other.
|
978 |
|
979 | ##### Options:
|
980 |
|
981 | - inherits all from Box.
|
982 |
|
983 | ##### Properties:
|
984 |
|
985 | - inherits all from Box.
|
986 |
|
987 | ##### Events:
|
988 |
|
989 | - inherits all from Box.
|
990 |
|
991 | ##### Methods:
|
992 |
|
993 | - inherits all from Box.
|
994 |
|
995 |
|
996 | #### RadioButton (from Checkbox)
|
997 |
|
998 | A radio button which can be used in a form element.
|
999 |
|
1000 | ##### Options:
|
1001 |
|
1002 | - inherits all from Checkbox.
|
1003 |
|
1004 | ##### Properties:
|
1005 |
|
1006 | - inherits all from Checkbox.
|
1007 |
|
1008 | ##### Events:
|
1009 |
|
1010 | - inherits all from Checkbox.
|
1011 |
|
1012 | ##### Methods:
|
1013 |
|
1014 | - inherits all from Checkbox.
|
1015 |
|
1016 |
|
1017 | #### Prompt (from Box)
|
1018 |
|
1019 | A prompt box containing a text input, okay, and cancel buttons (automatically
|
1020 | hidden).
|
1021 |
|
1022 | ##### Options:
|
1023 |
|
1024 | - inherits all from Box.
|
1025 |
|
1026 | ##### Properties:
|
1027 |
|
1028 | - inherits all from Box.
|
1029 |
|
1030 | ##### Events:
|
1031 |
|
1032 | - inherits all from Box.
|
1033 |
|
1034 | ##### Methods:
|
1035 |
|
1036 | - inherits all from Box.
|
1037 | - __input/setInput/readInput(text, value, callback)__ - show the prompt and
|
1038 | wait for the result of the textbox. set text and initial value.
|
1039 |
|
1040 |
|
1041 | #### Question (from Box)
|
1042 |
|
1043 | A question box containing okay and cancel buttons (automatically hidden).
|
1044 |
|
1045 | ##### Options:
|
1046 |
|
1047 | - inherits all from Box.
|
1048 |
|
1049 | ##### Properties:
|
1050 |
|
1051 | - inherits all from Box.
|
1052 |
|
1053 | ##### Events:
|
1054 |
|
1055 | - inherits all from Box.
|
1056 |
|
1057 | ##### Methods:
|
1058 |
|
1059 | - inherits all from Box.
|
1060 | - __ask(question, callback)__ - ask a `question`. `callback` will yield the
|
1061 | result.
|
1062 |
|
1063 |
|
1064 | #### Message (from Box)
|
1065 |
|
1066 | A box containing a message to be displayed (automatically hidden).
|
1067 |
|
1068 | ##### Options:
|
1069 |
|
1070 | - inherits all from Box.
|
1071 |
|
1072 | ##### Properties:
|
1073 |
|
1074 | - inherits all from Box.
|
1075 |
|
1076 | ##### Events:
|
1077 |
|
1078 | - inherits all from Box.
|
1079 |
|
1080 | ##### Methods:
|
1081 |
|
1082 | - inherits all from Box.
|
1083 | - __log/display(text, [time], callback)__ - display a message for a time
|
1084 | (default is 3 seconds). set time to 0 for a perpetual message that is
|
1085 | dismissed on keypress.
|
1086 | - __error(text, [time], callback)__ - display an error in the same way.
|
1087 |
|
1088 |
|
1089 | #### Loading (from Box)
|
1090 |
|
1091 | A box with a spinning line to denote loading (automatically hidden).
|
1092 |
|
1093 | ##### Options:
|
1094 |
|
1095 | - inherits all from Box.
|
1096 |
|
1097 | ##### Properties:
|
1098 |
|
1099 | - inherits all from Box.
|
1100 |
|
1101 | ##### Events:
|
1102 |
|
1103 | - inherits all from Box.
|
1104 |
|
1105 | ##### Methods:
|
1106 |
|
1107 | - inherits all from Box.
|
1108 | - __load(text)__ - display the loading box with a message. will lock keys until
|
1109 | `stop` is called.
|
1110 | - __stop()__ - hide loading box. unlock keys.
|
1111 |
|
1112 |
|
1113 | #### Listbar (from Box)
|
1114 |
|
1115 | A horizontal list. Useful for a main menu bar.
|
1116 |
|
1117 | ##### Options:
|
1118 |
|
1119 | - inherits all from Box.
|
1120 | - __style.selected__ - style for a selected item.
|
1121 | - __style.item__ - style for an unselected item.
|
1122 | - __commands/items__ - set buttons using an object with keys as titles of
|
1123 | buttons, containing of objects containing keys of `keys` and `callback`.
|
1124 | - __autoCommandKeys__ - automatically bind list buttons to keys 0-9.
|
1125 |
|
1126 | ##### Properties:
|
1127 |
|
1128 | - inherits all from Box.
|
1129 |
|
1130 | ##### Events:
|
1131 |
|
1132 | - inherits all from Box.
|
1133 |
|
1134 | ##### Methods:
|
1135 |
|
1136 | - inherits all from Box.
|
1137 | - __setItems(commands)__ - set commands (see `commands` option above).
|
1138 | - __add/addItem/appendItem(item, callback)__ - append an item to the bar.
|
1139 | - __select(offset)__ - select an item on the bar.
|
1140 | - __removeItem(child)__ - remove item from the bar.
|
1141 | - __move(offset)__ - move relatively across the bar.
|
1142 | - __moveLeft(offset)__ - move left relatively across the bar.
|
1143 | - __moveRight(offset)__ - move right relatively across the bar.
|
1144 | - __selectTab(index)__ - select button and execute its callback.
|
1145 |
|
1146 |
|
1147 | #### Log (from ScrollableText)
|
1148 |
|
1149 | A log permanently scrolled to the bottom.
|
1150 |
|
1151 | ##### Options:
|
1152 |
|
1153 | - inherits all from ScrollableText.
|
1154 | - __scrollback__ - amount of scrollback allowed. default: Infinity.
|
1155 | - __scrollOnInput__ - scroll to bottom on input even if the user has scrolled
|
1156 | up. default: false.
|
1157 |
|
1158 | ##### Properties:
|
1159 |
|
1160 | - inherits all from ScrollableText.
|
1161 | - __scrollback__ - amount of scrollback allowed. default: Infinity.
|
1162 | - __scrollOnInput__ - scroll to bottom on input even if the user has scrolled
|
1163 | up. default: false.
|
1164 |
|
1165 | ##### Events:
|
1166 |
|
1167 | - inherits all from ScrollableText.
|
1168 | - __log__ - emitted on a log line. passes in line.
|
1169 |
|
1170 | ##### Methods:
|
1171 |
|
1172 | - inherits all from ScrollableText.
|
1173 | - __log/add(text)__ - add a log line.
|
1174 |
|
1175 |
|
1176 | #### Table (from Box)
|
1177 |
|
1178 | A stylized table of text elements.
|
1179 |
|
1180 | ##### Options:
|
1181 |
|
1182 | - inherits all from Box.
|
1183 | - __rows/data__ - array of array of strings representing rows.
|
1184 | - __pad__ - spaces to attempt to pad on the sides of each cell. `2` by default:
|
1185 | one space on each side (only useful if the width is shrunken).
|
1186 | - __noCellBorders__ - do not draw inner cells.
|
1187 | - __fillCellBorders__ - fill cell borders with the adjacent background color.
|
1188 | - __style.header__ - header style.
|
1189 | - __style.cell__ - cell style.
|
1190 |
|
1191 | ##### Properties:
|
1192 |
|
1193 | - inherits all from Box.
|
1194 |
|
1195 | ##### Events:
|
1196 |
|
1197 | - inherits all from Box.
|
1198 |
|
1199 | ##### Methods:
|
1200 |
|
1201 | - inherits all from Box.
|
1202 | - __setRows/setData(rows)__ - set rows in table. array of arrays of strings.
|
1203 | ``` js
|
1204 | table.setData([
|
1205 | [ 'Animals', 'Foods' ],
|
1206 | [ 'Elephant', 'Apple' ],
|
1207 | [ 'Bird', 'Orange' ]
|
1208 | ]);
|
1209 | ```
|
1210 |
|
1211 |
|
1212 | #### ListTable (from List)
|
1213 |
|
1214 | A stylized table of text elements with a list.
|
1215 |
|
1216 | ##### Options:
|
1217 |
|
1218 | - inherits all from List.
|
1219 | - __rows/data__ - array of array of strings representing rows.
|
1220 | - __pad__ - spaces to attempt to pad on the sides of each cell. `2` by default:
|
1221 | one space on each side (only useful if the width is shrunken).
|
1222 | - __noCellBorders__ - do not draw inner cells.
|
1223 | - __style.header__ - header style.
|
1224 | - __style.cell__ - cell style.
|
1225 |
|
1226 | ##### Properties:
|
1227 |
|
1228 | - inherits all from List.
|
1229 |
|
1230 | ##### Events:
|
1231 |
|
1232 | - inherits all from List.
|
1233 |
|
1234 | ##### Methods:
|
1235 |
|
1236 | - inherits all from List.
|
1237 | - __setRows/setData(rows)__ - set rows in table. array of arrays of strings.
|
1238 | ``` js
|
1239 | table.setData([
|
1240 | [ 'Animals', 'Foods' ],
|
1241 | [ 'Elephant', 'Apple' ],
|
1242 | [ 'Bird', 'Orange' ]
|
1243 | ]);
|
1244 | ```
|
1245 |
|
1246 |
|
1247 | #### Terminal (from Box)
|
1248 |
|
1249 | A box which spins up a pseudo terminal and renders the output. Useful for
|
1250 | writing a terminal multiplexer, or something similar to an mc-like file
|
1251 | manager. Requires term.js and pty.js to be installed. See
|
1252 | `example/multiplex.js` for an example terminal multiplexer.
|
1253 |
|
1254 | ##### Options:
|
1255 |
|
1256 | - inherits all from Box.
|
1257 | - __handler__ - handler for input data.
|
1258 | - __shell__ - name of shell. `$SHELL` by default.
|
1259 | - __args__ - args for shell.
|
1260 | - __cursor__ - can be `line`, `underline`, and `block`.
|
1261 | - Other options similar to term.js'.
|
1262 |
|
1263 | ##### Properties:
|
1264 |
|
1265 | - inherits all from Box.
|
1266 | - __term__ - reference to the headless term.js terminal.
|
1267 | - __pty__ - reference to the pty.js pseudo terminal.
|
1268 |
|
1269 | ##### Events:
|
1270 |
|
1271 | - inherits all from Box.
|
1272 | - __title__ - window title from terminal.
|
1273 | - Other events similar to ScrollableBox.
|
1274 |
|
1275 | ##### Methods:
|
1276 |
|
1277 | - inherits all from Box.
|
1278 | - __write(data)__ - write data to the terminal.
|
1279 | - __screenshot([xi, xl, yi, xl])__ - nearly identical to `element.screenshot`,
|
1280 | however, the specified region includes the terminal's _entire_ scrollback,
|
1281 | rather than just what is visible on the screen.
|
1282 | - Other methods similar to ScrollableBox.
|
1283 |
|
1284 |
|
1285 | #### Image (from Box)
|
1286 |
|
1287 | Display an image in the terminal (jpeg, png, gif) using w3mimgdisplay. Requires
|
1288 | w3m to be installed. X11 required: works in xterm, urxvt, and possibly other
|
1289 | terminals.
|
1290 |
|
1291 | ##### Options:
|
1292 |
|
1293 | - inherits all from Box.
|
1294 | - __file__ - path to image.
|
1295 | - __w3m__ - path to w3mimgdisplay. if a proper w3mimgdisplay path is not given,
|
1296 | blessed will search the entire disk for the binary.
|
1297 |
|
1298 | ##### Properties:
|
1299 |
|
1300 | - inherits all from Box.
|
1301 |
|
1302 | ##### Events:
|
1303 |
|
1304 | - inherits all from Box.
|
1305 |
|
1306 | ##### Methods:
|
1307 |
|
1308 | - inherits all from Box.
|
1309 | - __setImage(img, callback)__ - set the image in the box to a new path.
|
1310 | - __clearImage(callback)__ - clear the current image.
|
1311 | - __imageSize(img, callback)__ - get the size of an image file in pixels.
|
1312 | - __termSize(callback)__ - get the size of the terminal in pixels.
|
1313 | - __getPixelRatio(callback)__ - get the pixel to cell ratio for the terminal.
|
1314 |
|
1315 |
|
1316 | ### Artificial Cursors
|
1317 |
|
1318 | Terminal cursors can be tricky. They all have different custom escape codes to
|
1319 | alter. As an _experimental_ alternative, blessed can draw a cursor for you,
|
1320 | allowing you to have a custom cursor that you control.
|
1321 |
|
1322 | ``` js
|
1323 | var screen = blessed.screen({
|
1324 | cursor: {
|
1325 | artificial: true,
|
1326 | shape: 'line',
|
1327 | blink: true,
|
1328 | color: null // null for default
|
1329 | }
|
1330 | });
|
1331 | ```
|
1332 |
|
1333 | That's it. It's controlled the same way as the regular cursor.
|
1334 |
|
1335 | To create a custom cursor:
|
1336 |
|
1337 | ``` js
|
1338 | var screen = blessed.screen({
|
1339 | cursor: {
|
1340 | artificial: true,
|
1341 | shape: {
|
1342 | bg: 'red',
|
1343 | fg: 'white',
|
1344 | bold: true,
|
1345 | ch: '#'
|
1346 | },
|
1347 | blink: true
|
1348 | }
|
1349 | });
|
1350 | ```
|
1351 |
|
1352 |
|
1353 | ### Positioning
|
1354 |
|
1355 | Offsets may be a number, a percentage (e.g. `50%`), or a keyword (e.g.
|
1356 | `center`).
|
1357 |
|
1358 | Dimensions may be a number, or a percentage (e.g. `50%`).
|
1359 |
|
1360 | Positions are treated almost _exactly_ the same as they are in CSS/CSSOM when
|
1361 | an element has the `position: absolute` CSS property.
|
1362 |
|
1363 | When an element is created, it can be given coordinates in its constructor:
|
1364 |
|
1365 | ``` js
|
1366 | var box = blessed.box({
|
1367 | left: 'center',
|
1368 | top: 'center',
|
1369 | bg: 'yellow',
|
1370 | width: '50%',
|
1371 | height: '50%'
|
1372 | });
|
1373 | ```
|
1374 |
|
1375 | This tells blessed to create a box, perfectly centered __relative to its
|
1376 | parent__, 50% as wide and 50% as tall as its parent.
|
1377 |
|
1378 | Percentages can also have offsets applied to them:
|
1379 |
|
1380 | ``` js
|
1381 | ...
|
1382 | height: '50%-1',
|
1383 | left: '45%+1',
|
1384 | ...
|
1385 | ```
|
1386 |
|
1387 | To access the calculated offsets, relative to the parent:
|
1388 |
|
1389 | ``` js
|
1390 | console.log(box.left);
|
1391 | console.log(box.top);
|
1392 | ```
|
1393 |
|
1394 | To access the calculated offsets, absolute (relative to the screen):
|
1395 |
|
1396 | ``` js
|
1397 | console.log(box.aleft);
|
1398 | console.log(box.atop);
|
1399 | ```
|
1400 |
|
1401 | #### Overlapping offsets and dimensions greater than parents'
|
1402 |
|
1403 | This still needs to be tested a bit, but it should work.
|
1404 |
|
1405 |
|
1406 | ### Content
|
1407 |
|
1408 | Every element can have text content via `setContent`. If `tags: true` was
|
1409 | passed to the element's constructor, the content can contain tags. For example:
|
1410 |
|
1411 | ```
|
1412 | box.setContent('hello {red-fg}{green-bg}{bold}world{/bold}{/green-bg}{/red-fg}');
|
1413 | ```
|
1414 |
|
1415 | To make this more concise `{/}` cancels all character attributes.
|
1416 |
|
1417 | ```
|
1418 | box.setContent('hello {red-fg}{green-bg}{bold}world{/}');
|
1419 | ```
|
1420 |
|
1421 | Newlines and alignment are also possible in content.
|
1422 |
|
1423 | ``` js
|
1424 | box.setContent('hello\n'
|
1425 | + '{right}world{/right}\n'
|
1426 | + '{center}foo{/center}');
|
1427 | ```
|
1428 |
|
1429 | This will produce a box that looks like:
|
1430 |
|
1431 | ```
|
1432 | | hello |
|
1433 | | world |
|
1434 | | foo |
|
1435 | ```
|
1436 |
|
1437 | Content can also handle SGR escape codes. This means if you got output from a
|
1438 | program, say `git log` for example, you can feed it directly to an element's
|
1439 | content and the colors will be parsed appropriately.
|
1440 |
|
1441 | This means that while `{red-fg}foo{/red-fg}` produces `^[[31mfoo^[[39m`, you
|
1442 | could just feed `^[[31mfoo^[[39m` directly to the content.
|
1443 |
|
1444 |
|
1445 | ### Event Bubbling
|
1446 |
|
1447 | Events can bubble in blessed. For example:
|
1448 |
|
1449 | Receiving all click events for `box`:
|
1450 |
|
1451 | ``` js
|
1452 | box.on('click', function(mouse) {
|
1453 | box.setContent('You clicked ' + mouse.x + ', ' + mouse.y + '.');
|
1454 | screen.render();
|
1455 | });
|
1456 | ```
|
1457 |
|
1458 | Receiving all click events for `box`, as well as all of its children:
|
1459 |
|
1460 | ``` js
|
1461 | box.on('element click', function(el, mouse) {
|
1462 | box.setContent('You clicked '
|
1463 | + el.type + ' at ' + mouse.x + ', ' + mouse.y + '.');
|
1464 | screen.render();
|
1465 | if (el === box) {
|
1466 | return false; // Cancel propagation.
|
1467 | }
|
1468 | });
|
1469 | ```
|
1470 |
|
1471 | `el` gets passed in as the first argument. It refers to the target element the
|
1472 | event occurred on. Returning `false` will cancel propagation up the tree.
|
1473 |
|
1474 |
|
1475 | ### Rendering
|
1476 |
|
1477 | To actually render the screen buffer, you must call `render`.
|
1478 |
|
1479 | ``` js
|
1480 | box.setContent('Hello {#0fe1ab-fg}world{/}.');
|
1481 | screen.render();
|
1482 | ```
|
1483 |
|
1484 | Elements are rendered with the lower elements in the children array being
|
1485 | painted first. In terms of the painter's algorithm, the lowest indicies in the
|
1486 | array are the furthest away, just like in the DOM.
|
1487 |
|
1488 |
|
1489 | ### Windows compatibility
|
1490 |
|
1491 | Currently there is no `mouse` or `resize` event support on Windows.
|
1492 |
|
1493 | Windows users will need to explicitly set `term` when creating a screen like so
|
1494 | (__NOTE__: This is no longer necessary as of the latest versions of blessed.
|
1495 | This is now handled automatically):
|
1496 |
|
1497 | ``` js
|
1498 | var screen = blessed.screen({ term: 'windows-ansi' });
|
1499 | ```
|
1500 |
|
1501 |
|
1502 | ### Testing
|
1503 |
|
1504 | Most tests contained in the `test/` directory are interactive. It's up to the
|
1505 | programmer to determine whether the test is properly displayed. In the future
|
1506 | it might be better to do something similar to vttest.
|
1507 |
|
1508 |
|
1509 | ## Lower-Level Usage
|
1510 |
|
1511 | This will actually parse the xterm terminfo and compile every
|
1512 | string capability to a javascript function:
|
1513 |
|
1514 | ``` js
|
1515 | var blessed = require('blessed');
|
1516 |
|
1517 | var tput = blessed.tput({
|
1518 | terminal: 'xterm-256color',
|
1519 | extended: true
|
1520 | });
|
1521 |
|
1522 | process.stdout.write(tput.setaf(4) + 'Hello' + tput.sgr0() + '\n');
|
1523 | ```
|
1524 |
|
1525 | To play around with it on the command line, it works just like tput:
|
1526 |
|
1527 | ``` bash
|
1528 | $ tput.js setaf 2
|
1529 | $ tput.js sgr0
|
1530 | $ echo "$(tput.js setaf 2)Hello World$(tput.js sgr0)"
|
1531 | ```
|
1532 |
|
1533 | The main functionality is exposed in the main `blessed` module:
|
1534 |
|
1535 | ``` js
|
1536 | var blessed = require('blessed')
|
1537 | , program = blessed.program();
|
1538 |
|
1539 | program.key('q', function(ch, key) {
|
1540 | program.clear();
|
1541 | program.disableMouse();
|
1542 | program.showCursor();
|
1543 | program.normalBuffer();
|
1544 | process.exit(0);
|
1545 | });
|
1546 |
|
1547 | program.on('mouse', function(data) {
|
1548 | if (data.action === 'mousemove') {
|
1549 | program.move(data.x, data.y);
|
1550 | program.bg('red');
|
1551 | program.write('x');
|
1552 | program.bg('!red');
|
1553 | }
|
1554 | });
|
1555 |
|
1556 | program.alternateBuffer();
|
1557 | program.enableMouse();
|
1558 | program.hideCursor();
|
1559 | program.clear();
|
1560 |
|
1561 | program.move(1, 1);
|
1562 | program.bg('black');
|
1563 | program.write('Hello world', 'blue fg');
|
1564 | program.setx((program.cols / 2 | 0) - 4);
|
1565 | program.down(5);
|
1566 | program.write('Hi again!');
|
1567 | program.bg('!black');
|
1568 | program.feed();
|
1569 | ```
|
1570 |
|
1571 |
|
1572 | ## FAQ
|
1573 |
|
1574 | 1. Why doesn't the Linux console render lines correctly on Ubuntu?
|
1575 | - You need to install the `ncurses-base` package __and__ the `ncurses-term`
|
1576 | package. (#98)
|
1577 | 2. Why do vertical lines look chopped up in iTerm2?
|
1578 | - All ACS vertical lines look this way in iTerm2.
|
1579 | 3. Why can't I use my mouse in Terminal.app?
|
1580 | - Terminal.app does not support mouse events.
|
1581 | 4. Why doesn't the Image element appear in my terminal?
|
1582 | - The Image element uses w3m to display images. This generally only works on
|
1583 | X11+xterm/urxvt, but it _may_ work on other unix terminals.
|
1584 | 5. Why can't my mouse clicks register beyond 255-287 cells?
|
1585 | - Older versions of VTE do not support any modern mouse protocol. On top of
|
1586 | that, the old x10 protocol it does implement is bugged. Through several
|
1587 | workarounds we've managed to get the cell limit from 127 to 255/287. If
|
1588 | you're not happy with this, you may want to look into using xterm or
|
1589 | urxvt, or a terminal which uses a modern VTE, like gnome-terminal.
|
1590 | 6. Is blessed efficient?
|
1591 | - Yes. Blessed implements CSR and uses the painter's algorithm to render the
|
1592 | screen. It maintains two screen buffers so it only needs to render what
|
1593 | has changed on the terminal screen.
|
1594 | 7. Will blessed work with all terminals?
|
1595 | - Yes. blessed has a terminfo/termcap parser and compiler that was written
|
1596 | from scratch. It should work with every terminal as long as a terminfo
|
1597 | file is provided. If you notice any compatibility issues in your termial,
|
1598 | do not hesitate to post an issue.
|
1599 | 8. What is "curses" and "ncurses"?
|
1600 | - ["curses"][curses] was an old library written in the early days of unix
|
1601 | which allowed a programmer to not have to worry about terminal
|
1602 | compatibility. ["ncurses"][ncurses] is a free reimplementation of curses.
|
1603 | It improved upon it quite a bit and is now the standard library for
|
1604 | implementing terminal programs. Blessed uses neither of these, and instead
|
1605 | handles terminal compatibility itself.
|
1606 | 9. What is the difference between blessed and blessed-contrib?
|
1607 | - blessed is a major piece of code which reimplements curses from the ground
|
1608 | up. A UI API is then layered on top of this. blessed-contrib is a popular
|
1609 | library built on top of blessed which makes clever use of modules to
|
1610 | implement useful widgets like graphs, ascii art, and so on.
|
1611 | 10. Are there blessed-like solutions for non-javascript platforms?
|
1612 | - Yes. There are some fantastic solutions out there.
|
1613 | - Perl: [Curses::UI][curses-ui]
|
1614 | - Python: [Urwid][urwid]
|
1615 | - Go: [termui][termui] & [termbox-go][termbox]
|
1616 |
|
1617 |
|
1618 | ## Contribution and License Agreement
|
1619 |
|
1620 | If you contribute code to this project, you are implicitly allowing your code
|
1621 | to be distributed under the MIT license. You are also implicitly verifying that
|
1622 | all code is your original work. `</legalese>`
|
1623 |
|
1624 |
|
1625 | ## License
|
1626 |
|
1627 | Copyright (c) 2013-2015, Christopher Jeffrey. (MIT License)
|
1628 |
|
1629 | See LICENSE for more info.
|
1630 |
|
1631 | [slap]: https://github.com/slap-editor/slap
|
1632 | [contrib]: https://github.com/yaronn/blessed-contrib
|
1633 | [termui]: https://github.com/gizak/termui
|
1634 | [curses]: https://en.wikipedia.org/wiki/Curses_(programming_library)
|
1635 | [ncurses]: https://en.wikipedia.org/wiki/Ncurses
|
1636 | [urwid]: http://urwid.org/reference/index.html
|
1637 | [curses-ui]: http://search.cpan.org/~mdxi/Curses-UI-0.9609/lib/Curses/UI.pm
|
1638 | [termbox]: https://github.com/nsf/termbox-go
|