1 | # chalk-pipe
|
2 |
|
3 | [](https://github.com/LitoMore/chalk-pipe/actions)
|
4 | [](https://www.npmjs.com/package/chalk-pipe)
|
5 | [](https://github.com/LitoMore/chalk-pipe/blob/main/LICENSE)
|
6 | [](https://github.com/xojs/xo)
|
7 |
|
8 | Create chalk style schemes with simpler style strings
|
9 |
|
10 | 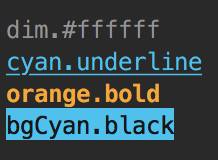
|
11 |
|
12 | ## Install
|
13 |
|
14 | ```sh
|
15 | npm install chalk-pipe
|
16 | ```
|
17 |
|
18 | ## Usage
|
19 |
|
20 | ```js
|
21 | import chalkPipe from 'chalk-pipe';
|
22 |
|
23 | console.log(chalkPipe('blue.bold')('Hello world!'));
|
24 | ```
|
25 |
|
26 | Use dot `.` to separeate multiple styles:
|
27 |
|
28 | ```js
|
29 | const link = chalkPipe('blue.underline');
|
30 | const error = chalkPipe('bgRed.#cccccc');
|
31 | const warning = chalkPipe('orange.bold');
|
32 |
|
33 | console.log(link('Link!'));
|
34 | console.log(error('Error!'));
|
35 | console.log(warning('Warning!'));
|
36 | ```
|
37 |
|
38 | `chalkPipe` is also `chalk`:
|
39 |
|
40 | ```js
|
41 | const blue = chalkPipe('blue');
|
42 | const link = blue.underline;
|
43 |
|
44 | console.log(link('Link!'));
|
45 | ```
|
46 |
|
47 | ### Use custom chalk
|
48 |
|
49 | ```js
|
50 | import chalkPipe, {chalk, Chalk} from 'chalk-pipe';
|
51 |
|
52 | const customChalk = new Chalk({level: 1});
|
53 |
|
54 | console.log(chalkPipe('underline', chalk.blue)('Link!'));
|
55 | console.log(chalkPipe('underline', customChalk.blue)('Link!'));
|
56 | ```
|
57 |
|
58 | ## Built-in Chalk
|
59 |
|
60 | All Chalk exported functions, variables, and declarations are exposed for convenience.
|
61 |
|
62 | This can be useful if you want to use `chalk` directly.
|
63 |
|
64 | ```js
|
65 | import {chalk, Chalk} from 'chalk-pipe';
|
66 |
|
67 | const customChalk = new Chalk({level: 0});
|
68 |
|
69 | console.log(chalk.blue('Hello'))
|
70 | console.log(customChalk.green('World'));
|
71 | ```
|
72 |
|
73 | ## API
|
74 |
|
75 | ### chalkPipe(styles)(text)
|
76 |
|
77 | Example:
|
78 |
|
79 | ```js
|
80 | chalkPipe('blue.underline')('Link!');
|
81 | ```
|
82 |
|
83 | ### chalkPipe(styles, chalk)(text)
|
84 |
|
85 | Example:
|
86 |
|
87 | ```js
|
88 | import {Chalk} from 'chalk-pipe';
|
89 |
|
90 | const chalk = new Chalk({level: 1});
|
91 |
|
92 | chalkPipe('underline', chalk.blue)('Link!');
|
93 | ```
|
94 |
|
95 | ### keywordNames
|
96 |
|
97 | All supported keyword names are exposed as array of strings for convenience.
|
98 |
|
99 | ```js
|
100 | import {keywordNames} from 'chalk-pipe';
|
101 |
|
102 | console.log(keywordNames.includes('pink'));
|
103 | //=> true
|
104 | ```
|
105 |
|
106 | ## Supported styles
|
107 |
|
108 | - [Modifiers](https://github.com/chalk/chalk#modifiers)
|
109 | - [Colors](https://github.com/chalk/chalk#colors)
|
110 | - [Background colors](https://github.com/chalk/chalk#background-colors)
|
111 | - [Hex triplet](https://en.wikipedia.org/wiki/Web_colors#Hex_triplet)
|
112 | - [CSS keywords](https://www.w3.org/wiki/CSS/Properties/color/keywords)
|
113 |
|
114 | ## Related
|
115 |
|
116 | - [chalk-pipe-cli](https://github.com/LitoMore/chalk-pipe-cli) - CLI for this module
|
117 | - [ink-color-pipe](https://github.com/LitoMore/ink-color-pipe) - Ink component for this module
|
118 | - [inquirer-chalk-pipe](https://github.com/LitoMore/inquirer-chalk-pipe) - A inquirer plugin for input chalk-pipe style strings
|
119 | - [chalk](https://github.com/chalk/chalk) - Output colored text to terminal
|
120 |
|
121 | ## License
|
122 |
|
123 | MIT © [LitoMore](https://github.com/LitoMore)
|