1 | ##### use colSpan to span columns - (colSpan above normal cell)
|
2 | ┌───────────────┐
|
3 | │ greetings │
|
4 | ├───────────────┤
|
5 | │ greetings │
|
6 | ├───────┬───────┤
|
7 | │ hello │ howdy │
|
8 | └───────┴───────┘
|
9 | ```javascript
|
10 | var table = new Table({style:{head:[],border:[]}});
|
11 |
|
12 | table.push(
|
13 | [{colSpan:2,content:'greetings'}],
|
14 | [{colSpan:2,content:'greetings'}],
|
15 | ['hello','howdy']
|
16 | );
|
17 |
|
18 | ```
|
19 |
|
20 |
|
21 | ##### use colSpan to span columns - (colSpan below normal cell)
|
22 | ┌───────┬───────┐
|
23 | │ hello │ howdy │
|
24 | ├───────┴───────┤
|
25 | │ greetings │
|
26 | ├───────────────┤
|
27 | │ greetings │
|
28 | └───────────────┘
|
29 | ```javascript
|
30 | var table = new Table({style:{head:[],border:[]}});
|
31 |
|
32 | table.push(
|
33 | ['hello','howdy'],
|
34 | [{colSpan:2,content:'greetings'}],
|
35 | [{colSpan:2,content:'greetings'}]
|
36 | );
|
37 |
|
38 | ```
|
39 |
|
40 |
|
41 | ##### use rowSpan to span rows - (rowSpan on the left side)
|
42 | ┌───────────┬───────────┬───────┐
|
43 | │ greetings │ │ hello │
|
44 | │ │ greetings ├───────┤
|
45 | │ │ │ howdy │
|
46 | └───────────┴───────────┴───────┘
|
47 | ```javascript
|
48 | var table = new Table({style:{head:[],border:[]}});
|
49 |
|
50 | table.push(
|
51 | [{rowSpan:2,content:'greetings'},{rowSpan:2,content:'greetings',vAlign:'center'},'hello'],
|
52 | ['howdy']
|
53 | );
|
54 |
|
55 | ```
|
56 |
|
57 |
|
58 | ##### use rowSpan to span rows - (rowSpan on the right side)
|
59 | ┌───────┬───────────┬───────────┐
|
60 | │ hello │ greetings │ │
|
61 | ├───────┤ │ │
|
62 | │ howdy │ │ greetings │
|
63 | └───────┴───────────┴───────────┘
|
64 | ```javascript
|
65 | var table = new Table({style:{head:[],border:[]}});
|
66 |
|
67 | table.push(
|
68 | ['hello',{rowSpan:2,content:'greetings'},{rowSpan:2,content:'greetings',vAlign:'bottom'}],
|
69 | ['howdy']
|
70 | );
|
71 |
|
72 | ```
|
73 |
|
74 |
|
75 | ##### mix rowSpan and colSpan together for complex table layouts
|
76 | ┌───────┬─────┬────┐
|
77 | │ hello │ sup │ hi │
|
78 | ├───────┤ │ │
|
79 | │ howdy │ │ │
|
80 | ├───┬───┼──┬──┤ │
|
81 | │ o │ k │ │ │ │
|
82 | └───┴───┴──┴──┴────┘
|
83 | ```javascript
|
84 | var table = new Table({style:{head:[],border:[]}});
|
85 |
|
86 | table.push(
|
87 | [{content:'hello',colSpan:2},{rowSpan:2, colSpan:2,content:'sup'},{rowSpan:3,content:'hi'}],
|
88 | [{content:'howdy',colSpan:2}],
|
89 | ['o','k','','']
|
90 | );
|
91 |
|
92 | ```
|
93 |
|
94 |
|
95 | ##### multi-line content will flow across rows in rowSpan cells
|
96 | ┌───────┬───────────┬───────────┐
|
97 | │ hello │ greetings │ greetings │
|
98 | ├───────┤ friends │ friends │
|
99 | │ howdy │ │ │
|
100 | └───────┴───────────┴───────────┘
|
101 | ```javascript
|
102 | var table = new Table({style:{head:[],border:[]}});
|
103 |
|
104 | table.push(
|
105 | ['hello',{rowSpan:2,content:'greetings\nfriends'},{rowSpan:2,content:'greetings\nfriends'}],
|
106 | ['howdy']
|
107 | );
|
108 |
|
109 | ```
|
110 |
|
111 |
|
112 | ##### multi-line content will flow across rows in rowSpan cells - (complex layout)
|
113 | ┌───────┬─────┬────┐
|
114 | │ hello │ sup │ hi │
|
115 | ├───────┤ man │ yo │
|
116 | │ howdy │ hey │ │
|
117 | ├───┬───┼──┬──┤ │
|
118 | │ o │ k │ │ │ │
|
119 | └───┴───┴──┴──┴────┘
|
120 | ```javascript
|
121 | var table = new Table({style:{head:[],border:[]}});
|
122 |
|
123 | table.push(
|
124 | [{content:'hello',colSpan:2},{rowSpan:2, colSpan:2,content:'sup\nman\nhey'},{rowSpan:3,content:'hi\nyo'}],
|
125 | [{content:'howdy',colSpan:2}],
|
126 | ['o','k','','']
|
127 | );
|
128 |
|
129 | ```
|
130 |
|
131 |
|
132 | ##### rowSpan cells can have a staggered layout
|
133 | ┌───┬───┐
|
134 | │ a │ b │
|
135 | │ ├───┤
|
136 | │ │ c │
|
137 | ├───┤ │
|
138 | │ d │ │
|
139 | └───┴───┘
|
140 | ```javascript
|
141 | var table = new Table({style:{head:[],border:[]}});
|
142 |
|
143 | table.push(
|
144 | [{content:'a',rowSpan:2},'b'],
|
145 | [{content:'c',rowSpan:2}],
|
146 | ['d']
|
147 | );
|
148 |
|
149 | ```
|
150 |
|
151 |
|
152 | ##### the layout manager automatically create empty cells to fill in the table
|
153 | ┌───┬───┬──┐
|
154 | │ a │ b │ │
|
155 | │ ├───┤ │
|
156 | │ │ │ │
|
157 | │ ├───┴──┤
|
158 | │ │ c │
|
159 | ├───┤ │
|
160 | │ │ │
|
161 | └───┴──────┘
|
162 | ```javascript
|
163 | var table = new Table({style:{head:[],border:[]}});
|
164 |
|
165 | //notice we only create 3 cells here, but the table ends up having 6.
|
166 | table.push(
|
167 | [{content:'a',rowSpan:3,colSpan:2},'b'],
|
168 | [],
|
169 | [{content:'c',rowSpan:2,colSpan:2}],
|
170 | []
|
171 | );
|
172 | ```
|
173 |
|
174 |
|
175 | ##### use table `rowHeights` option to fix row height. The truncation symbol will be shown on the last line.
|
176 | ┌───────┐
|
177 | │ hello │
|
178 | │ hi… │
|
179 | └───────┘
|
180 | ```javascript
|
181 | var table = new Table({rowHeights:[2],style:{head:[],border:[]}});
|
182 |
|
183 | table.push(['hello\nhi\nsup']);
|
184 |
|
185 | ```
|
186 |
|
187 |
|
188 | ##### if `colWidths` is not specified, the layout manager will automatically widen rows to fit the content
|
189 | ┌─────────────┐
|
190 | │ hello there │
|
191 | ├──────┬──────┤
|
192 | │ hi │ hi │
|
193 | └──────┴──────┘
|
194 | ```javascript
|
195 | var table = new Table({style:{head:[],border:[]}});
|
196 |
|
197 | table.push(
|
198 | [{colSpan:2,content:'hello there'}],
|
199 | ['hi', 'hi']
|
200 | );
|
201 |
|
202 | ```
|
203 |
|
204 |
|
205 | ##### you can specify a column width for only the first row, other rows will be automatically widened to fit content
|
206 | ┌─────────────┐
|
207 | │ hello there │
|
208 | ├────┬────────┤
|
209 | │ hi │ hi │
|
210 | └────┴────────┘
|
211 | ```javascript
|
212 | var table = new Table({colWidths:[4],style:{head:[],border:[]}});
|
213 |
|
214 | table.push(
|
215 | [{colSpan:2,content:'hello there'}],
|
216 | ['hi',{hAlign:'center',content:'hi'}]
|
217 | );
|
218 |
|
219 | ```
|
220 |
|
221 |
|
222 | ##### a column with a null column width will be automatically widened to fit content
|
223 | ┌─────────────┐
|
224 | │ hello there │
|
225 | ├────────┬────┤
|
226 | │ hi │ hi │
|
227 | └────────┴────┘
|
228 | ```javascript
|
229 | var table = new Table({colWidths:[null, 4],style:{head:[],border:[]}});
|
230 |
|
231 | table.push(
|
232 | [{colSpan:2,content:'hello there'}],
|
233 | [{hAlign:'right',content:'hi'}, 'hi']
|
234 | );
|
235 |
|
236 | ```
|
237 |
|
238 |
|
239 | ##### feel free to use colors in your content strings, column widths will be calculated correctly
|
240 | 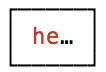
|
241 | ```javascript
|
242 | var table = new Table({colWidths:[5],style:{head:[],border:[]}});
|
243 |
|
244 | table.push([colors.red('hello')]);
|
245 |
|
246 | ```
|
247 |
|