1 | ##### Basic Usage
|
2 | 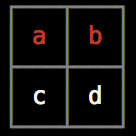
|
3 | ```javascript
|
4 | // By default, headers will be red, and borders will be grey
|
5 | // Those defaults are overwritten in a lot of these examples and within the tests.
|
6 | // It makes unit tests easier and visually cleaner, and does not require a screenshot image.
|
7 | var table = new Table({head:['a','b']});
|
8 |
|
9 | table.push(['c','d']);
|
10 |
|
11 | ```
|
12 |
|
13 |
|
14 | ##### Basic Usage - No color styles
|
15 | ┌──────┬─────────────────────┬─────────────────────────┬─────────────────┐
|
16 | │ Rel │ Change │ By │ When │
|
17 | ├──────┼─────────────────────┼─────────────────────────┼─────────────────┤
|
18 | │ v0.1 │ Testing something … │ rauchg@gmail.com │ 7 minutes ago │
|
19 | ├──────┼─────────────────────┼─────────────────────────┼─────────────────┤
|
20 | │ v0.1 │ Testing something … │ rauchg@gmail.com │ 8 minutes ago │
|
21 | └──────┴─────────────────────┴─────────────────────────┴─────────────────┘
|
22 | ```javascript
|
23 | var table = new Table({
|
24 | head: ['Rel', 'Change', 'By', 'When']
|
25 | , style: {
|
26 | 'padding-left': 1
|
27 | , 'padding-right': 1
|
28 | , head: [] //overriding header style to not use colors
|
29 | , border: [] //overriding border style to not use colors
|
30 | }
|
31 | , colWidths: [6, 21, 25, 17]
|
32 | });
|
33 |
|
34 | table.push(
|
35 | ['v0.1', 'Testing something cool', 'rauchg@gmail.com', '7 minutes ago']
|
36 | , ['v0.1', 'Testing something cool', 'rauchg@gmail.com', '8 minutes ago']
|
37 | );
|
38 |
|
39 | ```
|
40 |
|
41 |
|
42 | ##### Create vertical tables by adding objects a that specify key-value pairs
|
43 | ┌────┬──────────────────────┐
|
44 | │v0.1│Testing something cool│
|
45 | ├────┼──────────────────────┤
|
46 | │v0.1│Testing something cool│
|
47 | └────┴──────────────────────┘
|
48 | ```javascript
|
49 | var table = new Table({ style: {'padding-left':0, 'padding-right':0, head:[], border:[]} });
|
50 |
|
51 | table.push(
|
52 | {'v0.1': 'Testing something cool'}
|
53 | , {'v0.1': 'Testing something cool'}
|
54 | );
|
55 |
|
56 | ```
|
57 |
|
58 |
|
59 | ##### Cross tables are similar to vertical tables, but include an empty string for the first header
|
60 | ┌────────┬────────┬──────────────────────┐
|
61 | │ │Header 1│Header 2 │
|
62 | ├────────┼────────┼──────────────────────┤
|
63 | │Header 3│v0.1 │Testing something cool│
|
64 | ├────────┼────────┼──────────────────────┤
|
65 | │Header 4│v0.1 │Testing something cool│
|
66 | └────────┴────────┴──────────────────────┘
|
67 | ```javascript
|
68 | var table = new Table({ head: ["", "Header 1", "Header 2"], style: {'padding-left':0, 'padding-right':0, head:[], border:[]} }); // clear styles to prevent color output
|
69 |
|
70 | table.push(
|
71 | {"Header 3": ['v0.1', 'Testing something cool'] }
|
72 | , {"Header 4": ['v0.1', 'Testing something cool'] }
|
73 | );
|
74 |
|
75 | ```
|
76 |
|
77 |
|
78 | ##### Stylize the table with custom border characters
|
79 | ╔══════╤═════╤══════╗
|
80 | ║ foo │ bar │ baz ║
|
81 | ╟──────┼─────┼──────╢
|
82 | ║ frob │ bar │ quuz ║
|
83 | ╚══════╧═════╧══════╝
|
84 | ```javascript
|
85 | var table = new Table({
|
86 | chars: {
|
87 | 'top': '═'
|
88 | , 'top-mid': '╤'
|
89 | , 'top-left': '╔'
|
90 | , 'top-right': '╗'
|
91 | , 'bottom': '═'
|
92 | , 'bottom-mid': '╧'
|
93 | , 'bottom-left': '╚'
|
94 | , 'bottom-right': '╝'
|
95 | , 'left': '║'
|
96 | , 'left-mid': '╟'
|
97 | , 'right': '║'
|
98 | , 'right-mid': '╢'
|
99 | },
|
100 | style: {
|
101 | head: []
|
102 | , border: []
|
103 | }
|
104 | });
|
105 |
|
106 | table.push(
|
107 | ['foo', 'bar', 'baz']
|
108 | , ['frob', 'bar', 'quuz']
|
109 | );
|
110 |
|
111 | ```
|
112 |
|