1 | <p><img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/logo.png" alt="logo" width="20%" /></p>
|
2 | <p>
|
3 | <h1>Color Calendar</h1>
|
4 | </p>
|
5 | <p>
|
6 | <a href="https://www.npmjs.com/package/color-calendar">
|
7 | <img src="https://img.shields.io/npm/v/color-calendar?style=flat-square" alt="npm version" />
|
8 | </a>
|
9 | <a href="https://www.npmjs.com/package/color-calendar">
|
10 | <img src="https://img.shields.io/npm/dw/color-calendar?style=flat-square" alt="npm downloads" />
|
11 | </a>
|
12 | <img src="https://img.shields.io/bundlephobia/min/color-calendar?style=flat-square" alt="size" />
|
13 | <a href="https://www.jsdelivr.com/package/npm/color-calendar">
|
14 | <img src="https://data.jsdelivr.com/v1/package/npm/color-calendar/badge" alt="jsdelivr" />
|
15 | </a>
|
16 | <img src="https://hitcounter.pythonanywhere.com/count/tag.svg?url=https%3A%2F%2Fgithub.com%2FPawanKolhe%2Fcolor-calendar" alt="Hits">
|
17 | <img src="https://img.shields.io/npm/l/color-calendar?style=flat-square" alt="license" />
|
18 | </p>
|
19 |
|
20 | [](https://circleci.com/gh/PawanKolhe/color-calendar)
|
21 |
|
22 | <p>
|
23 | A customizable events calendar component library. Checkout <a href="https://online-tech-confs.herokuapp.com/">Demo 1</a>, <a href="https://v84yk.csb.app/">Demo 2</a> and <a href="https://codesandbox.io/s/color-calendar-bnwdu">Demo 3.</a>
|
24 | </p>
|
25 |
|
26 |
|
27 | 
|
28 | 
|
29 | 
|
30 | [](https://www.jsdelivr.com/package/npm/color-calendar)
|
31 | 
|
32 |  -->
|
33 |
|
34 | 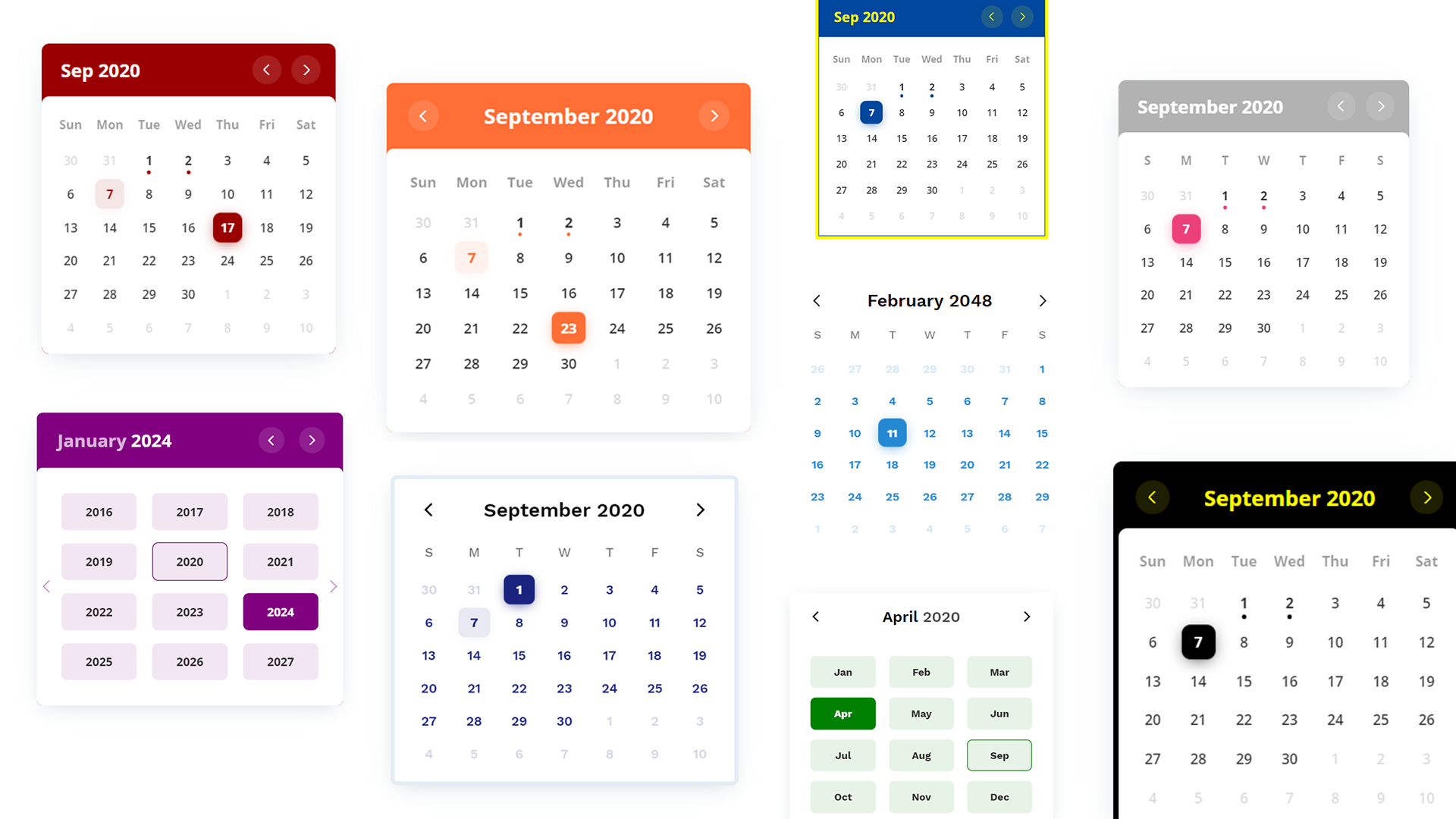
|
35 |
|
36 | - [Features](#features)
|
37 | - [Getting Started](#getting-started)
|
38 | - [CDN](#cdn)
|
39 | - [NPM](#npm)
|
40 | - [Usage](#usage)
|
41 | - [Basic](#usage-basic)
|
42 | - [React](#usage-react)
|
43 | - [Vue](#usage-vue)
|
44 | - [Options](#options)
|
45 | - [Events](#events)
|
46 | - [Methods](#methods)
|
47 | - [Types](#types)
|
48 | - [Themes](#themes)
|
49 | - [Bug Reporting](#bug)
|
50 | - [Feature Request](#feature-request)
|
51 | - [Release Notes](#release-notes)
|
52 | - [License](#license)
|
53 |
|
54 | <a id="features"></a>
|
55 |
|
56 | ## 🚀 Features
|
57 |
|
58 | - Zero dependencies
|
59 | - Add events to calendar
|
60 | - Perform some action on calendar date change
|
61 | - Month and year picker built-in
|
62 | - Themes available
|
63 | - Fully customizable using CSS variables, passing options parameters while creating calendar, or overriding CSS.
|
64 | - [Request more features](#feature-request)...
|
65 |
|
66 | <a id="getting-started"></a>
|
67 |
|
68 | ## 📦 Getting Started
|
69 |
|
70 | ### CDN
|
71 |
|
72 | #### JavaScript
|
73 |
|
74 | ```html
|
75 | <script src="https://cdn.jsdelivr.net/npm/color-calendar/dist/bundle.js">
|
76 | ```
|
77 |
|
78 | Also available on [unpkg](https://unpkg.com/browse/color-calendar/dist/).
|
79 |
|
80 | #### CSS
|
81 |
|
82 | Pick a css file according to the theme you want.
|
83 |
|
84 | ```html
|
85 | <link
|
86 | rel="stylesheet"
|
87 | href="https://cdn.jsdelivr.net/npm/color-calendar/dist/css/theme-basic.css"
|
88 | />
|
89 | <!-- or -->
|
90 | <link
|
91 | rel="stylesheet"
|
92 | href="https://cdn.jsdelivr.net/npm/color-calendar/dist/css/theme-glass.css"
|
93 | />
|
94 | ```
|
95 |
|
96 | #### Fonts
|
97 |
|
98 | Get fonts from [Google Fonts](https://fonts.google.com/)
|
99 |
|
100 | ```html
|
101 | <link
|
102 | href="https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;600;700&display=swap"
|
103 | rel="stylesheet"
|
104 | />
|
105 | ```
|
106 |
|
107 | Check what fonts the [theme](#themes) needs or pass your own fonts in [options](#options-fonts).
|
108 |
|
109 | ### NPM
|
110 |
|
111 | > _You might need to use a module bundler such as webpack, rollup, parcel, etc._
|
112 |
|
113 | #### Installation
|
114 |
|
115 | ```bash
|
116 | npm i color-calendar
|
117 | ```
|
118 |
|
119 | #### Import
|
120 |
|
121 | ```javascript
|
122 | import Calendar from "color-calendar";
|
123 | ```
|
124 |
|
125 | #### CSS
|
126 |
|
127 | ```javascript
|
128 | import "color-calendar/dist/css/theme-<THEME-NAME>.css";
|
129 | ```
|
130 |
|
131 | Then add fonts.
|
132 |
|
133 | <a id="usage"></a>
|
134 |
|
135 | ## 🔨 Usage
|
136 |
|
137 | <a id="usage-basic"></a>
|
138 |
|
139 | ### Basic
|
140 |
|
141 | #### JavaScript
|
142 |
|
143 | ```javascript
|
144 | new Calendar();
|
145 | ```
|
146 |
|
147 | _Or_ you can pass in **options**.
|
148 |
|
149 | ```javascript
|
150 | new Calendar({
|
151 | id: "#color-calendar",
|
152 | calendarSize: "small",
|
153 | });
|
154 | ```
|
155 |
|
156 | #### HTML
|
157 |
|
158 | ```html
|
159 | <div id="color-calendar"></div>
|
160 | ```
|
161 |
|
162 | [Example](https://codesandbox.io/s/color-calendar-bnwdu)
|
163 |
|
164 | <a id="usage-react"></a>
|
165 |
|
166 | ### React
|
167 |
|
168 | [Example](https://codesandbox.io/s/color-calendar-react-y0cyf?file=/src/CalendarComponent.jsx)
|
169 |
|
170 | <a id="usage-vue"></a>
|
171 |
|
172 | ### Vue
|
173 |
|
174 | [Example](https://codesandbox.io/s/color-calendar-vue-byo6e?file=/src/components/ColorCalendar.vue)
|
175 |
|
176 | <a id="options"></a>
|
177 |
|
178 | ## ⚙️ Options
|
179 |
|
180 | ### `id`
|
181 |
|
182 | Type: `String`
|
183 | Default: `#color-calendar`
|
184 |
|
185 | Selector referencing HTMLElement where the calendar instance will bind to.
|
186 |
|
187 | ### `calendarSize`
|
188 |
|
189 | Type: `String`
|
190 | Default: `large`
|
191 | Options: `small` | `large`
|
192 |
|
193 | Size of calendar UI.
|
194 |
|
195 | ### `layoutModifiers`
|
196 |
|
197 | Type: [`LayoutModifier`](#type-layout-modifier)[]
|
198 | Default: `[]`
|
199 | Example: `['month-left-align']`
|
200 |
|
201 | Modifiers to alter the layout of the calendar.
|
202 |
|
203 | ### `eventsData`
|
204 |
|
205 | Type: [`EventData`](#type-event-data)[]
|
206 | Default: `null`
|
207 |
|
208 | ```javascript
|
209 | [
|
210 | {
|
211 | start: '2020-08-17T06:00:00',
|
212 | end: '2020-08-18T20:30:00',
|
213 | name: 'Blockchain 101'
|
214 | ...
|
215 | },
|
216 | ...
|
217 | ]
|
218 | ```
|
219 |
|
220 | Array of objects containing events information.
|
221 |
|
222 | > Note: Properties `start` and `end` are _required_ for each event in the [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) date and time format. You can _optionally_ choose to add other properties.
|
223 |
|
224 | ### `theme`
|
225 |
|
226 | Type: `String`
|
227 | Default: `basic`
|
228 | Options: `basic` | `glass`
|
229 |
|
230 | Choose from available themes.
|
231 |
|
232 | ### `primaryColor`
|
233 |
|
234 | Type: `String`
|
235 | Default: _based on theme_
|
236 | Example: `#1a237e`
|
237 |
|
238 | Main color accent of calendar. _Pick a color in rgb, hex or hsl format._
|
239 |
|
240 | ### `headerColor`
|
241 |
|
242 | Type: `String`
|
243 | Default: _based on theme_
|
244 | Example: `rgb(0, 102, 0)`
|
245 |
|
246 | Color of header text.
|
247 |
|
248 | ### `headerBackgroundColor`
|
249 |
|
250 | Type: `String`
|
251 | Default: _based on theme_
|
252 | Example: `black`
|
253 |
|
254 | Background color of header. _Only works on some themes._
|
255 |
|
256 | ### `weekdaysColor`
|
257 |
|
258 | Type: `String`
|
259 | Default: _based on theme_
|
260 |
|
261 | Color of weekdays text. _Only works on some themes._
|
262 |
|
263 | ### `weekdayDisplayType`
|
264 |
|
265 | Type: `String`
|
266 | Default: `short`
|
267 | Options: [WeekdayDisplayType](#type-weekday-display-type) (`short` | `long-lower` | `long-upper`)
|
268 |
|
269 | Select how weekdays will be displayed. E.g. M, Mon, or MON.
|
270 |
|
271 | ### `monthDisplayType`
|
272 |
|
273 | Type: `String`
|
274 | Default: `long`
|
275 | Options: [MonthDisplayType](#type-month-display-type) (`short` | `long`)
|
276 |
|
277 | Select how month will be displayed in header. E.g. Feb, February.
|
278 |
|
279 | ### `startWeekday`
|
280 |
|
281 | Type: `Number`
|
282 | Default: `0`
|
283 | Options: `0` | `1` | `2` | `3` | `4` | `5` | `6`
|
284 |
|
285 | Starting weekday. Mapping: 0 (Sun), 1 (Mon), 2 (Tues), 3 (Wed), 4 (Thurs), 5 (Fri), 6 (Sat)
|
286 |
|
287 | <a id="options-fonts"></a>
|
288 |
|
289 | ### `fontFamilyHeader`
|
290 |
|
291 | Type: `String`
|
292 | Default: _based on theme_
|
293 | Example value: `'Open Sans', sans-serif`
|
294 |
|
295 | Font of calendar header text.
|
296 |
|
297 | ### `fontFamilyWeekdays`
|
298 |
|
299 | Type: `String`
|
300 | Default: _based on theme_
|
301 |
|
302 | Font of calendar weekdays text.
|
303 |
|
304 | ### `fontFamilyBody`
|
305 |
|
306 | Type: `String`
|
307 | Default: _based on theme_
|
308 |
|
309 | Font of calendar days as well as month and year picker text.
|
310 |
|
311 | ### `dropShadow`
|
312 |
|
313 | Type: `String`
|
314 | Default: _based on theme_
|
315 |
|
316 | Set CSS of calendar drop shadow.
|
317 |
|
318 | ### `border`
|
319 |
|
320 | Type: `String`
|
321 | Default: _based on theme_
|
322 | Example value: `5px solid black`
|
323 |
|
324 | Set CSS style of border.
|
325 |
|
326 | ### `borderRadius`
|
327 |
|
328 | Type: `String`
|
329 | Default: `0.5rem`
|
330 |
|
331 | Set CSS border radius of calendar.
|
332 |
|
333 | ### `disableMonthYearPickers`
|
334 |
|
335 | Type: `Boolean`
|
336 | Default: `false`
|
337 |
|
338 | If month and year picker should be disabled.
|
339 |
|
340 | ### `disableDayClick`
|
341 |
|
342 | Type: `Boolean`
|
343 | Default: `false`
|
344 |
|
345 | If day click should be disabled.
|
346 |
|
347 | ### `disableMonthArrowClick`
|
348 |
|
349 | Type: `Boolean`
|
350 | Default: `false`
|
351 |
|
352 | If month arrows should be disabled.
|
353 |
|
354 | ### `customMonthValues`
|
355 |
|
356 | Type: `String[]`
|
357 | Default: `undefined`
|
358 |
|
359 | Set custom display values for Month.
|
360 |
|
361 | ### `customWeekdayValues`
|
362 |
|
363 | Type: `String[]`
|
364 | Default: `undefined`
|
365 |
|
366 | Set custom display values for Weekdays.
|
367 |
|
368 | ```javascript
|
369 | ["Lun", "Mar", "Mer", "Jeu", "Ven", "Sam", "Dim"];
|
370 | ```
|
371 |
|
372 | <a id="events"></a>
|
373 |
|
374 | ## 🖱 Events
|
375 |
|
376 | ### `dateChanged`
|
377 |
|
378 | Type: `Function`
|
379 | Props:
|
380 |
|
381 | - `currentDate`
|
382 | - Type: `Date`
|
383 | - Currently selected date
|
384 | - `filteredDateEvents`
|
385 | - Type: [`EventData`](#type-event-data)[]
|
386 | - All events on that particular date
|
387 |
|
388 | ```typescript
|
389 | const options = {
|
390 | ...
|
391 | dateChanged: (currentDate?: Date, filteredDateEvents?: EventData[]) => {
|
392 | // do something
|
393 | };
|
394 | ...
|
395 | }
|
396 | ```
|
397 |
|
398 | Emitted when the selected date is changed.
|
399 |
|
400 | ### `selectedDateClicked`
|
401 |
|
402 | Type: `Function`
|
403 | Props:
|
404 |
|
405 | - `currentDate`
|
406 | - Type: `Date`
|
407 | - Currently selected date
|
408 | - `filteredMonthEvents`
|
409 | - Type: [`EventData`](#type-event-data)[]
|
410 | - All events on that particular month
|
411 |
|
412 | Emitted when the selected date is clicked.
|
413 |
|
414 | ### `monthChanged`
|
415 |
|
416 | Type: `Function`
|
417 | Props:
|
418 |
|
419 | - `currentDate`
|
420 | - Type: `Date`
|
421 | - Currently selected date
|
422 | - `filteredMonthEvents`
|
423 | - Type: [`EventData`](#type-event-data)[]
|
424 | - All events on that particular month
|
425 |
|
426 | Emitted when the current month is changed.
|
427 |
|
428 | <a id="methods"></a>
|
429 |
|
430 | ## 🔧 Methods
|
431 |
|
432 | ### `reset()`
|
433 |
|
434 | Reset calendar to today's date.
|
435 |
|
436 | ```javascript
|
437 | // Example
|
438 | let myCal = new Calendar();
|
439 | myCal.reset();
|
440 | ```
|
441 |
|
442 | ### `setDate()`
|
443 |
|
444 | Props:
|
445 | | Props | Type | Required | Description |
|
446 | |-------|------|----------|--------------------|
|
447 | | date | Date | required | New date to be set |
|
448 |
|
449 | Set new selected date.
|
450 |
|
451 | ```javascript
|
452 | // Example
|
453 | myCal.setDate(new Date());
|
454 | ```
|
455 |
|
456 | ### `getSelectedDate()`
|
457 |
|
458 | Return:
|
459 |
|
460 | - Type: `Date`
|
461 | - Description: `Currently selected date`
|
462 |
|
463 | Get currently selected date.
|
464 |
|
465 | ### `getEventsData()`
|
466 |
|
467 | Return:
|
468 |
|
469 | - Type: [EventData](#type-event-data)[]
|
470 | - Description: `All events`
|
471 |
|
472 | Get events array.
|
473 |
|
474 | ### `setEventsData()`
|
475 |
|
476 | Props:
|
477 | | Props | Type | Required | Description |
|
478 | |--------|------------|----------|------------------|
|
479 | | events | [EventData](#type-event-data)[] | required | Events to be set |
|
480 |
|
481 | Return:
|
482 |
|
483 | - Type: `Number`
|
484 | - Description: `Number of events set`
|
485 |
|
486 | Set a new events array.
|
487 |
|
488 | ### `addEventsData()`
|
489 |
|
490 | Props:
|
491 | | Props | Type | Required | Description |
|
492 | |--------|------------|----------|--------------------|
|
493 | | events | [EventData](#type-event-data)[] | required | Events to be added |
|
494 |
|
495 | Return:
|
496 |
|
497 | - Type: `Number`
|
498 | - Description: `Number of events added`
|
499 |
|
500 | Add events of the events array.
|
501 |
|
502 | ### `setWeekdayDisplayType()`
|
503 |
|
504 | Props:
|
505 | | Props | Type | Required | Description |
|
506 | |--------|------------|----------|--------------------|
|
507 | | weekdayDisplayType | [WeekdayDisplayType](#type-weekday-display-type) | required | New weekday type |
|
508 |
|
509 | Set weekdays display type.
|
510 |
|
511 | ```javascript
|
512 | // Example
|
513 | myCal.setWeekdayDisplayType("short");
|
514 | ```
|
515 |
|
516 | ### `setMonthDisplayType()`
|
517 |
|
518 | Props:
|
519 | | Props | Type | Required | Description |
|
520 | |--------|------------|----------|--------------------|
|
521 | | monthDisplayType | [MonthDisplayType](#type-month-display-type) | required | New month display type |
|
522 |
|
523 | Set month display type.
|
524 |
|
525 | <a id="types"></a>
|
526 |
|
527 | ## 💎 Types
|
528 |
|
529 | <a id="type-event-data"></a>
|
530 |
|
531 | ### `EventData`
|
532 |
|
533 | ```javascript
|
534 | {
|
535 | start: string, // ISO 8601 date and time format
|
536 | end: string, // ISO 8601 date and time format
|
537 | [key: string]: any,
|
538 | }
|
539 | ```
|
540 |
|
541 | <a id="type-weekday-display-type"></a>
|
542 |
|
543 | ### `WeekdayDisplayType`
|
544 |
|
545 | `"short"` | `"long-lower"` | `"long-upper"`
|
546 |
|
547 | ```markdown
|
548 | // "short"
|
549 | M T W ...
|
550 |
|
551 | // "long-lower"
|
552 | Mon Tue Wed ...
|
553 |
|
554 | // "long-upper"
|
555 | MON TUE WED ...
|
556 | ```
|
557 |
|
558 | <a id="type-month-display-type"></a>
|
559 |
|
560 | ### `MonthDisplayType`
|
561 |
|
562 | `"short"` | `"long"`
|
563 |
|
564 | ```markdown
|
565 | // "short"
|
566 | Jan Feb Mar ...
|
567 |
|
568 | // "long"
|
569 | January February March ...
|
570 | ```
|
571 |
|
572 | <a id="type-layout-modifier"></a>
|
573 |
|
574 | ### `LayoutModifier`
|
575 |
|
576 | `"month-align-left"`
|
577 |
|
578 | <a id="themes"></a>
|
579 |
|
580 | ## 🎨 Themes
|
581 |
|
582 | Currently 2 themes available. More on the way.
|
583 |
|
584 | ### `basic`
|
585 |
|
586 | <img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/theme-basic.PNG" alt="Basic Theme" width="400" />
|
587 |
|
588 | #### CSS Custom Properties
|
589 |
|
590 | `--cal-color-primary`: #000000;
|
591 | `--cal-font-family-header`: "Work Sans", sans-serif;
|
592 | `--cal-font-family-weekdays`: "Work Sans", sans-serif;
|
593 | `--cal-font-family-body`: "Work Sans", sans-serif;
|
594 | `--cal-drop-shadow`: 0 7px 30px -10px rgba(150, 170, 180, 0.5);
|
595 | `--cal-border`: none;
|
596 | `--cal-border-radius`: 0.5rem;
|
597 | `--cal-header-color`: black;
|
598 | `--cal-weekdays-color`: black;
|
599 |
|
600 | ### `glass`
|
601 |
|
602 | <img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/theme-glass.PNG" alt="Glass Theme" width="400" />
|
603 |
|
604 | #### CSS Custom Properties
|
605 |
|
606 | `--cal-color-primary`: #EC407A;
|
607 | `--cal-font-family-header`: 'Open Sans', sans-serif;
|
608 | `--cal-font-family-weekdays`: 'Open Sans', sans-serif;
|
609 | `--cal-font-family-body`: 'Open Sans', sans-serif;
|
610 | `--cal-drop-shadow`: 0 7px 30px -10px rgba(150, 170, 180, 0.5);
|
611 | `--cal-border`: none;
|
612 | `--cal-border-radius`: 0.5rem;
|
613 | `--cal-header-color`: white;
|
614 | `--cal-header-background-color`: rgba(0, 0, 0, 0.3);
|
615 |
|
616 | <a id="bug"></a>
|
617 |
|
618 | ## 🐛 Bug Reporting
|
619 |
|
620 | Feel free to [open an issue](https://github.com/PawanKolhe/color-calendar/issues) on GitHub if you find any bug.
|
621 |
|
622 | <a id="feature-request"></a>
|
623 |
|
624 | ## ⭐ Feature Request
|
625 |
|
626 | - Feel free to [Open an issue](https://github.com/PawanKolhe/color-calendar/issues) on GitHub to request any additional features you might need for your use case.
|
627 | - Connect with me on [LinkedIn](https://www.linkedin.com/in/kolhepawan/). I'd love ❤️️ to hear where you are using this library.
|
628 |
|
629 | <a id="release-notes"></a>
|
630 |
|
631 | ## 📋 Release Notes
|
632 |
|
633 | Check [here](https://github.com/PawanKolhe/color-calendar/releases) for release notes.
|
634 |
|
635 | <a id="license"></a>
|
636 |
|
637 | ## 📜 License
|
638 |
|
639 | This software is open source, licensed under the [MIT License](https://github.com/PawanKolhe/color-calendar/blob/master/LICENSE).
|