1 | <p><img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/logo.png" alt="logo" width="20%" /></p>
|
2 | <p>
|
3 | <h1>Color Calendar</h1>
|
4 | </p>
|
5 | <p>
|
6 | <a href="https://www.npmjs.com/package/color-calendar">
|
7 | <img src="https://img.shields.io/npm/v/color-calendar?style=flat-square" alt="npm version" />
|
8 | </a>
|
9 | <a href="https://www.npmjs.com/package/color-calendar">
|
10 | <img src="https://img.shields.io/npm/dw/color-calendar?style=flat-square" alt="npm downloads" />
|
11 | </a>
|
12 | <img src="https://img.shields.io/bundlephobia/min/color-calendar?style=flat-square" alt="size" />
|
13 | <a href="https://www.jsdelivr.com/package/npm/color-calendar">
|
14 | <img src="https://data.jsdelivr.com/v1/package/npm/color-calendar/badge" alt="jsdelivr" />
|
15 | </a>
|
16 | <img src="https://hitcounter.pythonanywhere.com/count/tag.svg?url=https%3A%2F%2Fgithub.com%2FPawanKolhe%2Fcolor-calendar" alt="Hits">
|
17 | <img src="https://img.shields.io/npm/l/color-calendar?style=flat-square" alt="license" />
|
18 | </p>
|
19 | <p>
|
20 | A customizable events calendar component library. Checkout <a href="https://online-tech-confs.herokuapp.com/">Demo 1</a>, <a href="https://v84yk.csb.app/">Demo 2</a> and <a href="https://codesandbox.io/s/color-calendar-bnwdu">Demo 3.</a>
|
21 | </p>
|
22 |
|
23 |
|
24 | 
|
25 | 
|
26 | 
|
27 | [](https://www.jsdelivr.com/package/npm/color-calendar)
|
28 | 
|
29 |  -->
|
30 |
|
31 | 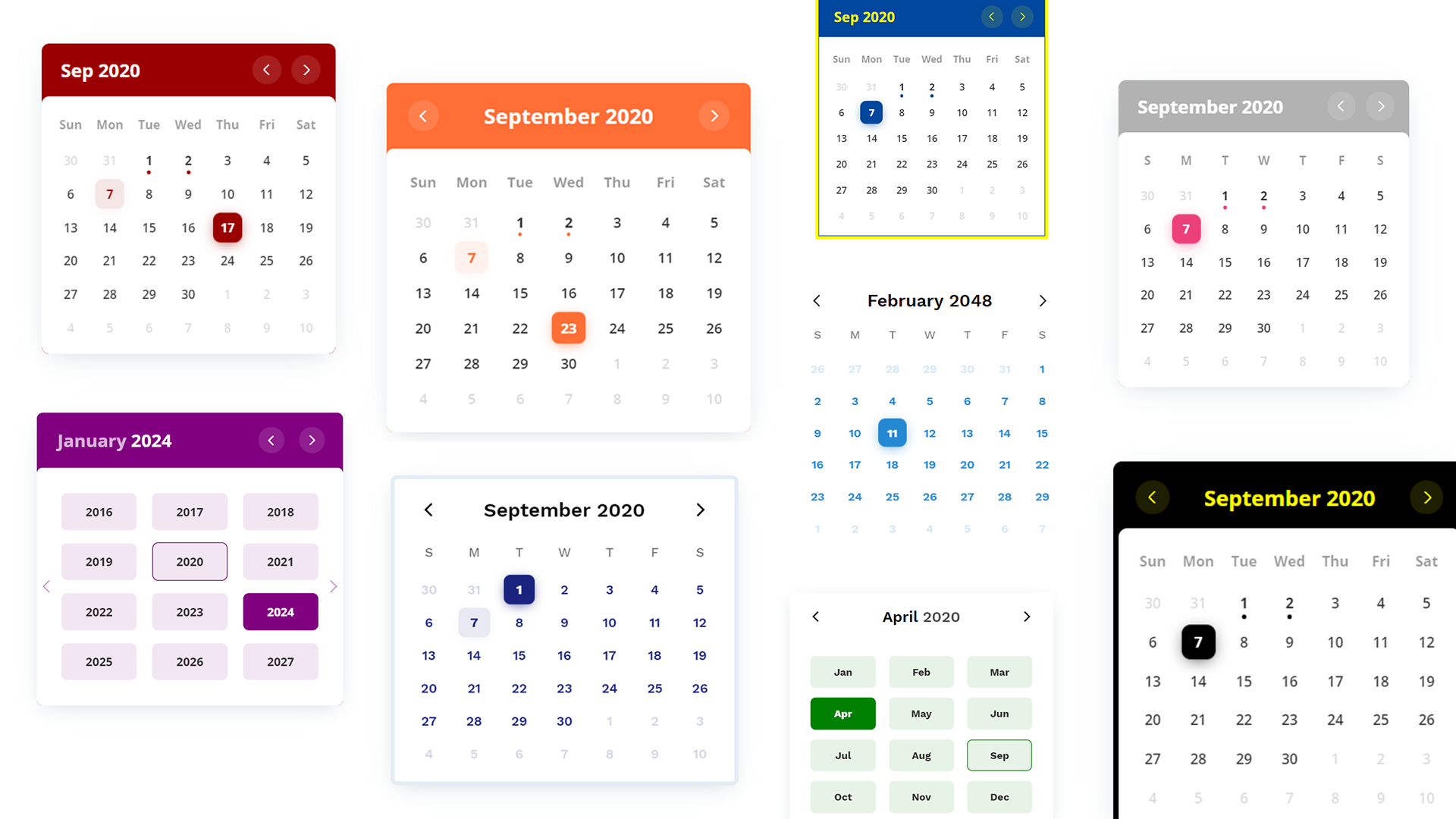
|
32 |
|
33 | * [Features](#features)
|
34 | * [Getting Started](#getting-started)
|
35 | * [CDN](#cdn)
|
36 | * [NPM](#npm)
|
37 | * [Usage](#usage)
|
38 | * [Basic](#usage-basic)
|
39 | * [React](#usage-react)
|
40 | * [Vue](#usage-vue)
|
41 | * [Options](#options)
|
42 | * [Events](#events)
|
43 | * [Methods](#methods)
|
44 | * [Types](#types)
|
45 | * [Themes](#themes)
|
46 | * [Bug Reporting](#bug)
|
47 | * [Feature Request](#feature-request)
|
48 | * [Release Notes](#release-notes)
|
49 | * [License](#license)
|
50 |
|
51 | <a id="features"></a>
|
52 | ## 🚀 Features
|
53 | - Zero dependencies
|
54 | - Add events to calendar
|
55 | - Perform some action on calendar date change
|
56 | - Month and year picker built-in
|
57 | - Themes available
|
58 | - Fully customizable using CSS variables, passing options parameters while creating calendar, or overriding CSS.
|
59 | - [Request more features](#feature-request)...
|
60 |
|
61 | <a id="getting-started"></a>
|
62 | ## 📦 Getting Started
|
63 |
|
64 | ### CDN
|
65 | #### JavaScript
|
66 | ```html
|
67 | <script src="https://cdn.jsdelivr.net/npm/color-calendar/dist/bundle.js">
|
68 | ```
|
69 | Also available on [unpkg](https://unpkg.com/browse/color-calendar/dist/).
|
70 |
|
71 | #### CSS
|
72 | Pick a css file according to the theme you want.
|
73 | ```html
|
74 | <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/color-calendar/dist/css/theme-basic.css">
|
75 | <!-- or -->
|
76 | <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/color-calendar/dist/css/theme-glass.css">
|
77 | ```
|
78 |
|
79 | #### Fonts
|
80 | Get fonts from [Google Fonts](https://fonts.google.com/)
|
81 | ```html
|
82 | <link href="https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;600;700&display=swap" rel="stylesheet">
|
83 | ```
|
84 | Check what fonts the [theme](#themes) needs or pass your own fonts in [options](#options-fonts).
|
85 |
|
86 | ### NPM
|
87 | > _You might need to use a module bundler such as webpack, rollup, parcel, etc._
|
88 | #### Installation
|
89 | ```bash
|
90 | npm i color-calendar
|
91 | ```
|
92 | #### Import
|
93 | ```javascript
|
94 | import Calendar from 'color-calendar';
|
95 | ```
|
96 |
|
97 | #### CSS
|
98 | ```javascript
|
99 | import 'color-calendar/dist/css/theme-<THEME-NAME>.css';
|
100 | ```
|
101 |
|
102 | Then add fonts.
|
103 |
|
104 | <a id="usage"></a>
|
105 | ## 🔨 Usage
|
106 | <a id="usage-basic"></a>
|
107 | ### Basic
|
108 | #### JavaScript
|
109 | ```javascript
|
110 | new Calendar()
|
111 | ```
|
112 | _Or_ you can pass in **options**.
|
113 | ```javascript
|
114 | new Calendar({
|
115 | id: '#color-calendar',
|
116 | calendarSize: 'small'
|
117 | })
|
118 | ```
|
119 |
|
120 | #### HTML
|
121 | ```html
|
122 | <div id="color-calendar"></div>
|
123 | ```
|
124 | [Example](https://codesandbox.io/s/color-calendar-bnwdu)
|
125 |
|
126 | <a id="usage-react"></a>
|
127 | ### React
|
128 | [Example](https://codesandbox.io/s/color-calendar-react-y0cyf?file=/src/CalendarComponent.jsx)
|
129 |
|
130 | <a id="usage-vue"></a>
|
131 | ### Vue
|
132 | [Example](https://codesandbox.io/s/color-calendar-vue-byo6e?file=/src/components/ColorCalendar.vue)
|
133 |
|
134 | <a id="options"></a>
|
135 | ## ⚙️ Options
|
136 |
|
137 | ### `id`
|
138 | Type: `String`
|
139 | Default: `#color-calendar`
|
140 |
|
141 | Selector referencing HTMLElement where the calendar instance will bind to.
|
142 |
|
143 | ### `calendarSize`
|
144 | Type: `String`
|
145 | Default: `large`
|
146 | Options: `small` | `large`
|
147 |
|
148 | Size of calendar UI.
|
149 |
|
150 | ### `layoutModifiers`
|
151 | Type: [`LayoutModifier`](#type-layout-modifier)[]
|
152 | Default: `[]`
|
153 | Example: `['month-left-align']`
|
154 |
|
155 | Modifiers to alter the layout of the calendar.
|
156 |
|
157 | ### `eventsData`
|
158 | Type: [`EventData`](#type-event-data)[]
|
159 | Default: `null`
|
160 |
|
161 | ```javascript
|
162 | [
|
163 | {
|
164 | start: '2020-08-17T06:00:00',
|
165 | end: '2020-08-18T20:30:00',
|
166 | name: 'Blockchain 101'
|
167 | ...
|
168 | },
|
169 | ...
|
170 | ]
|
171 | ```
|
172 |
|
173 | Array of objects containing events information.
|
174 | > Note: Properties `start` and `end` are *required* for each event in the [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) date and time format. You can _optionally_ choose to add other properties.
|
175 |
|
176 | ### `theme`
|
177 | Type: `String`
|
178 | Default: `basic`
|
179 | Options: `basic` | `glass`
|
180 |
|
181 | Choose from available themes.
|
182 |
|
183 | ### `primaryColor`
|
184 | Type: `String`
|
185 | Default: *based on theme*
|
186 | Example: `#1a237e`
|
187 |
|
188 | Main color accent of calendar. *Pick a color in rgb, hex or hsl format.*
|
189 |
|
190 | ### `headerColor`
|
191 | Type: `String`
|
192 | Default: *based on theme*
|
193 | Example: `rgb(0, 102, 0)`
|
194 |
|
195 | Color of header text.
|
196 |
|
197 | ### `headerBackgroundColor`
|
198 | Type: `String`
|
199 | Default: *based on theme*
|
200 | Example: `black`
|
201 |
|
202 | Background color of header. *Only works on some themes.*
|
203 |
|
204 | ### `weekdaysColor`
|
205 | Type: `String`
|
206 | Default: *based on theme*
|
207 |
|
208 | Color of weekdays text. *Only works on some themes.*
|
209 |
|
210 | ### `weekdayDisplayType`
|
211 | Type: `String`
|
212 | Default: `short`
|
213 | Options: [WeekdayDisplayType](#type-weekday-display-type) (`short` | `long-lower` | `long-upper`)
|
214 |
|
215 | Select how weekdays will be displayed. E.g. M, Mon, or MON.
|
216 |
|
217 | ### `monthDisplayType`
|
218 | Type: `String`
|
219 | Default: `long`
|
220 | Options: [MonthDisplayType](#type-month-display-type) (`short` | `long`)
|
221 |
|
222 | Select how month will be displayed in header. E.g. Feb, February.
|
223 |
|
224 | ### `startWeekday`
|
225 | Type: `Number`
|
226 | Default: `0`
|
227 | Options: `0` | `1` | `2` | `3` | `4` | `5` | `6`
|
228 |
|
229 | Starting weekday. Mapping: 0 (Sun), 1 (Mon), 2 (Tues), 3 (Wed), 4 (Thurs), 5 (Fri), 6 (Sat)
|
230 |
|
231 | <a id="options-fonts"></a>
|
232 | ### `fontFamilyHeader`
|
233 | Type: `String`
|
234 | Default: *based on theme*
|
235 | Example value: `'Open Sans', sans-serif`
|
236 |
|
237 | Font of calendar header text.
|
238 |
|
239 | ### `fontFamilyWeekdays`
|
240 | Type: `String`
|
241 | Default: *based on theme*
|
242 |
|
243 | Font of calendar weekdays text.
|
244 |
|
245 | ### `fontFamilyBody`
|
246 | Type: `String`
|
247 | Default: *based on theme*
|
248 |
|
249 | Font of calendar days as well as month and year picker text.
|
250 |
|
251 | ### `dropShadow`
|
252 | Type: `String`
|
253 | Default: *based on theme*
|
254 |
|
255 | Set CSS of calendar drop shadow.
|
256 |
|
257 | ### `border`
|
258 | Type: `String`
|
259 | Default: *based on theme*
|
260 | Example value: `5px solid black`
|
261 |
|
262 | Set CSS style of border.
|
263 |
|
264 | ### `borderRadius`
|
265 | Type: `String`
|
266 | Default: `0.5rem`
|
267 |
|
268 | Set CSS border radius of calendar.
|
269 |
|
270 | ### `disableMonthYearPickers`
|
271 | Type: `Boolean`
|
272 | Default: `false`
|
273 |
|
274 | If month and year picker should be disabled.
|
275 |
|
276 | ### `disableDayClick`
|
277 | Type: `Boolean`
|
278 | Default: `false`
|
279 |
|
280 | If day click should be disabled.
|
281 |
|
282 | ### `disableMonthArrowClick`
|
283 | Type: `Boolean`
|
284 | Default: `false`
|
285 |
|
286 | If month arrows should be disabled.
|
287 |
|
288 | <a id="events"></a>
|
289 | ## 🖱 Events
|
290 |
|
291 | ### `dateChanged`
|
292 | Type: `Function`
|
293 | Props:
|
294 | - `currentDate`
|
295 | - Type: `Date`
|
296 | - Currently selected date
|
297 | - `filteredDateEvents`
|
298 | - Type: [`EventData`](#type-event-data)[]
|
299 | - All events on that particular date
|
300 | ```typescript
|
301 | const options = {
|
302 | ...
|
303 | dateChanged: (currentDate?: Date, filteredDateEvents?: EventData[]) => {
|
304 | // do something
|
305 | };
|
306 | ...
|
307 | }
|
308 | ```
|
309 |
|
310 | Emitted when the selected date is changed.
|
311 |
|
312 | ### `monthChanged`
|
313 | Type: `Function`
|
314 | Props:
|
315 | - `currentDate`
|
316 | - Type: `Date`
|
317 | - Currently selected date
|
318 | - `filteredMonthEvents`
|
319 | - Type: [`EventData`](#type-event-data)[]
|
320 | - All events on that particular month
|
321 |
|
322 | Emitted when the current month is changed.
|
323 |
|
324 | <a id="methods"></a>
|
325 | ## 🔧 Methods
|
326 | ### `reset()`
|
327 |
|
328 | Reset calendar to today's date.
|
329 | ```javascript
|
330 | // Example
|
331 | let myCal = new Calendar();
|
332 | myCal.reset();
|
333 | ```
|
334 |
|
335 | ### `setDate()`
|
336 | Props:
|
337 | | Props | Type | Required | Description |
|
338 | |-------|------|----------|--------------------|
|
339 | | date | Date | required | New date to be set |
|
340 |
|
341 | Set new selected date.
|
342 |
|
343 | ```javascript
|
344 | // Example
|
345 | myCal.setDate(new Date());
|
346 | ```
|
347 |
|
348 | ### `getSelectedDate()`
|
349 | Return:
|
350 | - Type: `Date`
|
351 | - Description: `Currently selected date`
|
352 |
|
353 | Get currently selected date.
|
354 |
|
355 | ### `getEventsData()`
|
356 | Return:
|
357 | - Type: [EventData](#type-event-data)[]
|
358 | - Description: `All events`
|
359 |
|
360 | Get events array.
|
361 |
|
362 | ### `setEventsData()`
|
363 | Props:
|
364 | | Props | Type | Required | Description |
|
365 | |--------|------------|----------|------------------|
|
366 | | events | [EventData](#type-event-data)[] | required | Events to be set |
|
367 |
|
368 | Return:
|
369 | - Type: `Number`
|
370 | - Description: `Number of events set`
|
371 |
|
372 | Set a new events array.
|
373 |
|
374 | ### `addEventsData()`
|
375 | Props:
|
376 | | Props | Type | Required | Description |
|
377 | |--------|------------|----------|--------------------|
|
378 | | events | [EventData](#type-event-data)[] | required | Events to be added |
|
379 |
|
380 | Return:
|
381 | - Type: `Number`
|
382 | - Description: `Number of events added`
|
383 |
|
384 | Add events of the events array.
|
385 |
|
386 | ### `setWeekdayDisplayType()`
|
387 | Props:
|
388 | | Props | Type | Required | Description |
|
389 | |--------|------------|----------|--------------------|
|
390 | | weekdayDisplayType | [WeekdayDisplayType](#type-weekday-display-type) | required | New weekday type |
|
391 |
|
392 | Set weekdays display type.
|
393 |
|
394 | ```javascript
|
395 | // Example
|
396 | myCal.setWeekdayDisplayType("short");
|
397 | ```
|
398 |
|
399 | ### `setMonthDisplayType()`
|
400 | Props:
|
401 | | Props | Type | Required | Description |
|
402 | |--------|------------|----------|--------------------|
|
403 | | monthDisplayType | [MonthDisplayType](#type-month-display-type) | required | New month display type |
|
404 |
|
405 | Set month display type.
|
406 |
|
407 | <a id="types"></a>
|
408 | ## 💎 Types
|
409 | <a id="type-event-data"></a>
|
410 | ### `EventData`
|
411 | ```javascript
|
412 | {
|
413 | start: string, // ISO 8601 date and time format
|
414 | end: string, // ISO 8601 date and time format
|
415 | [key: string]: any,
|
416 | }
|
417 | ```
|
418 |
|
419 | <a id="type-weekday-display-type"></a>
|
420 | ### `WeekdayDisplayType`
|
421 | `"short"` | `"long-lower"` | `"long-upper"`
|
422 |
|
423 | ```markdown
|
424 | // "short"
|
425 | M T W ...
|
426 |
|
427 | // "long-lower"
|
428 | Mon Tue Wed ...
|
429 |
|
430 | // "long-upper"
|
431 | MON TUE WED ...
|
432 | ```
|
433 |
|
434 | <a id="type-month-display-type"></a>
|
435 | ### `MonthDisplayType`
|
436 | `"short"` | `"long"`
|
437 |
|
438 | ```markdown
|
439 | // "short"
|
440 | Jan Feb Mar ...
|
441 |
|
442 | // "long"
|
443 | January February March ...
|
444 | ```
|
445 |
|
446 | <a id="type-layout-modifier"></a>
|
447 | ### `LayoutModifier`
|
448 | `"month-align-left"`
|
449 |
|
450 | <a id="themes"></a>
|
451 | ## 🎨 Themes
|
452 | Currently 2 themes available. More on the way.
|
453 |
|
454 | ### `basic`
|
455 | <img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/theme-basic.PNG" alt="Basic Theme" width="400" />
|
456 |
|
457 | #### CSS Custom Properties
|
458 | `--cal-color-primary`: #000000;
|
459 | `--cal-font-family-header`: "Work Sans", sans-serif;
|
460 | `--cal-font-family-weekdays`: "Work Sans", sans-serif;
|
461 | `--cal-font-family-body`: "Work Sans", sans-serif;
|
462 | `--cal-drop-shadow`: 0 7px 30px -10px rgba(150, 170, 180, 0.5);
|
463 | `--cal-border`: none;
|
464 | `--cal-border-radius`: 0.5rem;
|
465 | `--cal-header-color`: black;
|
466 | `--cal-weekdays-color`: black;
|
467 |
|
468 | ### `glass`
|
469 | <img src="https://raw.githubusercontent.com/PawanKolhe/color-calendar/master/screenshots/theme-glass.PNG" alt="Glass Theme" width="400" />
|
470 |
|
471 | #### CSS Custom Properties
|
472 | `--cal-color-primary`: #EC407A;
|
473 | `--cal-font-family-header`: 'Open Sans', sans-serif;
|
474 | `--cal-font-family-weekdays`: 'Open Sans', sans-serif;
|
475 | `--cal-font-family-body`: 'Open Sans', sans-serif;
|
476 | `--cal-drop-shadow`: 0 7px 30px -10px rgba(150, 170, 180, 0.5);
|
477 | `--cal-border`: none;
|
478 | `--cal-border-radius`: 0.5rem;
|
479 | `--cal-header-color`: white;
|
480 | `--cal-header-background-color`: rgba(0, 0, 0, 0.3);
|
481 |
|
482 | <a id="bug"></a>
|
483 | ## 🐛 Bug Reporting
|
484 | Feel free to [open an issue](https://github.com/PawanKolhe/color-calendar/issues) on GitHub if you find any bug.
|
485 |
|
486 | <a id="feature-request"></a>
|
487 | ## ⭐ Feature Request
|
488 | - Feel free to [Open an issue](https://github.com/PawanKolhe/color-calendar/issues) on GitHub to request any additional features you might need for your use case.
|
489 | - Connect with me on [LinkedIn](https://www.linkedin.com/in/kolhepawan/). I'd love ❤️️ to hear where you are using this library.
|
490 |
|
491 | <a id="release-notes"></a>
|
492 | ## 📋 Release Notes
|
493 | Check [here](https://github.com/PawanKolhe/color-calendar/releases) for release notes.
|
494 |
|
495 | <a id="license"></a>
|
496 | ## 📜 License
|
497 | This software is open source, licensed under the [MIT License](https://github.com/PawanKolhe/color-calendar/blob/master/LICENSE).
|