1 | [](https://www.npmjs.org/package/command-line-args)
|
2 | [](https://www.npmjs.org/package/command-line-args)
|
3 | [](https://travis-ci.org/75lb/command-line-args)
|
4 | [](https://coveralls.io/github/75lb/command-line-args?branch=master)
|
5 | [](https://david-dm.org/75lb/command-line-args)
|
6 | [](https://github.com/feross/standard)
|
7 | [](https://gitter.im/75lb/command-line-args?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
|
8 |
|
9 | # command-line-args
|
10 | A mature, feature-complete library to parse command-line options.
|
11 |
|
12 | *If your app requires a git-like command interface, consider using [command-line-commands](https://github.com/75lb/command-line-commands).*
|
13 |
|
14 | ## Synopsis
|
15 | You can set options using the main notation standards (getopt, getopt_long, etc.). These commands are all equivalent, setting the same values:
|
16 | ```
|
17 | $ example --verbose --timeout=1000 --src one.js --src two.js
|
18 | $ example --verbose --timeout 1000 --src one.js two.js
|
19 | $ example -vt 1000 --src one.js two.js
|
20 | $ example -vt 1000 one.js two.js
|
21 | ```
|
22 |
|
23 | To access the values, first describe the options your app accepts (see [option definitions](#optiondefinition-)).
|
24 | ```js
|
25 | const commandLineArgs = require('command-line-args')
|
26 |
|
27 | const optionDefinitions = [
|
28 | { name: 'verbose', alias: 'v', type: Boolean },
|
29 | { name: 'src', type: String, multiple: true, defaultOption: true },
|
30 | { name: 'timeout', alias: 't', type: Number }
|
31 | ]
|
32 | ```
|
33 | The [`type`](#optiontype--function) property is a setter function (the value supplied is passed through this), giving you full control over the value received.
|
34 |
|
35 | Next, parse the options using [commandLineArgs()](#commandlineargsdefinitions-argv--object-):
|
36 | ```js
|
37 | const options = commandLineArgs(optionDefinitions)
|
38 | ```
|
39 |
|
40 | `options` now looks like this:
|
41 | ```js
|
42 | {
|
43 | files: [
|
44 | 'one.js',
|
45 | 'two.js'
|
46 | ],
|
47 | verbose: true,
|
48 | timeout: 1000
|
49 | }
|
50 | ```
|
51 |
|
52 | When dealing with large amounts of options it often makes sense to [group](#optiongroup--string--arraystring) them.
|
53 |
|
54 | A usage guide can be generated using [command-line-usage](https://github.com/75lb/command-line-usage), for example:
|
55 |
|
56 | 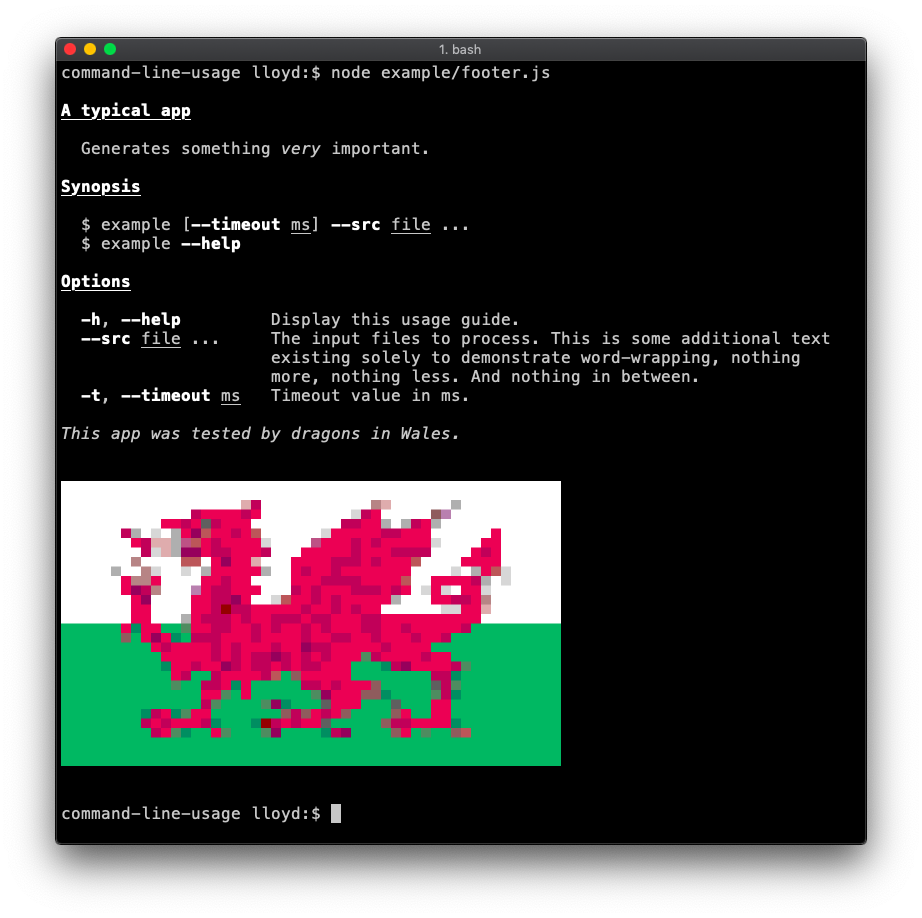
|
57 |
|
58 | ### Notation rules
|
59 |
|
60 | Notation rules for setting command-line options.
|
61 |
|
62 | * Argument order is insignificant. Whether you set `--example` at the beginning or end of the arg list makes no difference.
|
63 | * Options with a [type](#optiontype--function) of `Boolean` do not need to supply a value. Setting `--flag` or `-f` will set that option's value to `true`. This is the only [type](#optiontype--function) with special behaviour.
|
64 | * Three ways to set an option value
|
65 | * `--option value`
|
66 | * `--option=value`
|
67 | * `-o value`
|
68 | * Two ways to a set list of values (on options with [multiple](#optionmultiple--boolean) set)
|
69 | * `--list one two three`
|
70 | * `--list one --list two --list three`
|
71 | * Short options ([alias](#optionalias--string)) can be set in groups. The following are equivalent:
|
72 | * `-a -b -c`
|
73 | * `-abc`
|
74 |
|
75 | ### Ambiguous values
|
76 |
|
77 | Imagine we are using "grep-tool" to search for the string `'-f'`:
|
78 |
|
79 | ```
|
80 | $ grep-tool --search -f
|
81 | ```
|
82 |
|
83 | We have an issue here: command-line-args will assume we are setting two options (`--search` and `-f`). In actuality, we are passing one option (`--search`) and one value (`-f`). In cases like this, avoid ambiguity by using `--option=value` notation:
|
84 |
|
85 | ```
|
86 | $ grep-tool --search=-f
|
87 | ```
|
88 |
|
89 | ### Partial parsing
|
90 |
|
91 | By default, if the user sets an option without a valid [definition](#exp_module_definition--OptionDefinition) an `UNKNOWN_OPTION` exception is thrown. However, in some cases you may only be interested in a subset of the options wishing to pass the remainder to another library. See [here](https://github.com/75lb/command-line-args/blob/master/example/mocha.js) for a example showing where this might be necessary.
|
92 |
|
93 | To enable partial parsing, set `partial: true` in the method options:
|
94 |
|
95 | ```js
|
96 | const optionDefinitions = [
|
97 | { name: 'value', type: Number }
|
98 | ]
|
99 | const options = commandLineArgs(optionDefinitions, { partial: true })
|
100 | ```
|
101 |
|
102 | Now, should any unknown args be passed at the command line:
|
103 |
|
104 | ```
|
105 | $ example --milk --value 2 --bread cheese
|
106 | ```
|
107 |
|
108 | They will be returned in the `_unknown` property of the `commandLineArgs` output with no exceptions thrown:
|
109 |
|
110 | ```js
|
111 | {
|
112 | value: 2,
|
113 | _unknown: [ '--milk', '--bread', 'cheese']
|
114 | }
|
115 | ```
|
116 |
|
117 |
|
118 | ## Install
|
119 |
|
120 | ```sh
|
121 | $ npm install command-line-args --save
|
122 | ```
|
123 |
|
124 | # API Reference
|
125 | <a name="exp_module_command-line-args--commandLineArgs"></a>
|
126 |
|
127 | ### commandLineArgs(optionDefinitions, [options]) ⇒ <code>object</code> ⏏
|
128 | Returns an object containing all options set on the command line. By default it parses the global [`process.argv`](https://nodejs.org/api/process.html#process_process_argv) array.
|
129 |
|
130 | By default, an exception is thrown if the user sets an unknown option (one without a valid [definition](#exp_module_definition--OptionDefinition)). To enable __partial parsing__, invoke `commandLineArgs` with the `partial` option - all unknown arguments will be returned in the `_unknown` property.
|
131 |
|
132 | **Kind**: Exported function
|
133 | **Throws**:
|
134 |
|
135 | - `UNKNOWN_OPTION` if `options.partial` is false and the user set an undefined option
|
136 | - `NAME_MISSING` if an option definition is missing the required `name` property
|
137 | - `INVALID_TYPE` if an option definition has a `type` value that's not a function
|
138 | - `INVALID_ALIAS` if an alias is numeric, a hyphen or a length other than 1
|
139 | - `DUPLICATE_NAME` if an option definition name was used more than once
|
140 | - `DUPLICATE_ALIAS` if an option definition alias was used more than once
|
141 | - `DUPLICATE_DEFAULT_OPTION` if more than one option definition has `defaultOption: true`
|
142 |
|
143 |
|
144 | | Param | Type | Description |
|
145 | | --- | --- | --- |
|
146 | | optionDefinitions | <code>[Array.<definition>](#module_definition)</code> | An array of [OptionDefinition](#exp_module_definition--OptionDefinition) objects |
|
147 | | [options] | <code>object</code> | Options. |
|
148 | | [options.argv] | <code>Array.<string></code> | An array of strings, which if passed will be parsed instead of `process.argv`. |
|
149 | | [options.partial] | <code>boolean</code> | If `true`, an array of unknown arguments is returned in the `_unknown` property of the output. |
|
150 |
|
151 | <a name="exp_module_definition--OptionDefinition"></a>
|
152 |
|
153 | ## OptionDefinition ⏏
|
154 | Describes a command-line option. Additionally, you can add `description` and `typeLabel` properties and make use of [command-line-usage](https://github.com/75lb/command-line-usage).
|
155 |
|
156 | **Kind**: Exported class
|
157 | * [OptionDefinition](#exp_module_definition--OptionDefinition) ⏏
|
158 | * [.name](#module_definition--OptionDefinition.OptionDefinition+name) : <code>string</code>
|
159 | * [.type](#module_definition--OptionDefinition.OptionDefinition+type) : <code>function</code>
|
160 | * [.alias](#module_definition--OptionDefinition.OptionDefinition+alias) : <code>string</code>
|
161 | * [.multiple](#module_definition--OptionDefinition.OptionDefinition+multiple) : <code>boolean</code>
|
162 | * [.defaultOption](#module_definition--OptionDefinition.OptionDefinition+defaultOption) : <code>boolean</code>
|
163 | * [.defaultValue](#module_definition--OptionDefinition.OptionDefinition+defaultValue) : <code>\*</code>
|
164 | * [.group](#module_definition--OptionDefinition.OptionDefinition+group) : <code>string</code> \| <code>Array.<string></code>
|
165 |
|
166 | <a name="module_definition--OptionDefinition.OptionDefinition+name"></a>
|
167 |
|
168 | ### option.name : <code>string</code>
|
169 | The only required definition property is `name`, so the simplest working example is
|
170 | ```js
|
171 | [
|
172 | { name: "file" },
|
173 | { name: "verbose" },
|
174 | { name: "depth"}
|
175 | ]
|
176 | ```
|
177 |
|
178 | In this case, the value of each option will be either a Boolean or string.
|
179 |
|
180 | | # | Command line args | .parse() output |
|
181 | | --- | -------------------- | ------------ |
|
182 | | 1 | `--file` | `{ file: true }` |
|
183 | | 2 | `--file lib.js --verbose` | `{ file: "lib.js", verbose: true }` |
|
184 | | 3 | `--verbose very` | `{ verbose: "very" }` |
|
185 | | 4 | `--depth 2` | `{ depth: "2" }` |
|
186 |
|
187 | Unicode option names and aliases are valid, for example:
|
188 | ```js
|
189 | [
|
190 | { name: 'один' },
|
191 | { name: '两' },
|
192 | { name: 'три', alias: 'т' }
|
193 | ]
|
194 | ```
|
195 |
|
196 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
197 | <a name="module_definition--OptionDefinition.OptionDefinition+type"></a>
|
198 |
|
199 | ### option.type : <code>function</code>
|
200 | The `type` value is a setter function (you receive the output from this), enabling you to be specific about the type and value received.
|
201 |
|
202 | You can use a class, if you like:
|
203 |
|
204 | ```js
|
205 | const fs = require('fs')
|
206 |
|
207 | function FileDetails(filename){
|
208 | if (!(this instanceof FileDetails)) return new FileDetails(filename)
|
209 | this.filename = filename
|
210 | this.exists = fs.existsSync(filename)
|
211 | }
|
212 |
|
213 | const cli = commandLineArgs([
|
214 | { name: 'file', type: FileDetails },
|
215 | { name: 'depth', type: Number }
|
216 | ])
|
217 | ```
|
218 |
|
219 | | # | Command line args| .parse() output |
|
220 | | --- | ----------------- | ------------ |
|
221 | | 1 | `--file asdf.txt` | `{ file: { filename: 'asdf.txt', exists: false } }` |
|
222 |
|
223 | The `--depth` option expects a `Number`. If no value was set, you will receive `null`.
|
224 |
|
225 | | # | Command line args | .parse() output |
|
226 | | --- | ----------------- | ------------ |
|
227 | | 2 | `--depth` | `{ depth: null }` |
|
228 | | 3 | `--depth 2` | `{ depth: 2 }` |
|
229 |
|
230 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
231 | **Default**: <code>String</code>
|
232 | <a name="module_definition--OptionDefinition.OptionDefinition+alias"></a>
|
233 |
|
234 | ### option.alias : <code>string</code>
|
235 | getopt-style short option names. Can be any single character (unicode included) except a digit or hypen.
|
236 |
|
237 | ```js
|
238 | [
|
239 | { name: "hot", alias: "h", type: Boolean },
|
240 | { name: "discount", alias: "d", type: Boolean },
|
241 | { name: "courses", alias: "c" , type: Number }
|
242 | ]
|
243 | ```
|
244 |
|
245 | | # | Command line | .parse() output |
|
246 | | --- | ------------ | ------------ |
|
247 | | 1 | `-hcd` | `{ hot: true, courses: null, discount: true }` |
|
248 | | 2 | `-hdc 3` | `{ hot: true, discount: true, courses: 3 }` |
|
249 |
|
250 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
251 | <a name="module_definition--OptionDefinition.OptionDefinition+multiple"></a>
|
252 |
|
253 | ### option.multiple : <code>boolean</code>
|
254 | Set this flag if the option takes a list of values. You will receive an array of values, each passed through the `type` function (if specified).
|
255 |
|
256 | ```js
|
257 | [
|
258 | { name: "files", type: String, multiple: true }
|
259 | ]
|
260 | ```
|
261 |
|
262 | | # | Command line | .parse() output |
|
263 | | --- | ------------ | ------------ |
|
264 | | 1 | `--files one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` |
|
265 | | 2 | `--files one.js --files two.js` | `{ files: [ 'one.js', 'two.js' ] }` |
|
266 | | 3 | `--files *` | `{ files: [ 'one.js', 'two.js' ] }` |
|
267 |
|
268 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
269 | <a name="module_definition--OptionDefinition.OptionDefinition+defaultOption"></a>
|
270 |
|
271 | ### option.defaultOption : <code>boolean</code>
|
272 | Any unclaimed command-line args will be set on this option. This flag is typically set on the most commonly-used option to make for more concise usage (i.e. `$ myapp *.js` instead of `$ myapp --files *.js`).
|
273 |
|
274 | ```js
|
275 | [
|
276 | { name: "files", type: String, multiple: true, defaultOption: true }
|
277 | ]
|
278 | ```
|
279 |
|
280 | | # | Command line | .parse() output |
|
281 | | --- | ------------ | ------------ |
|
282 | | 1 | `--files one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` |
|
283 | | 2 | `one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` |
|
284 | | 3 | `*` | `{ files: [ 'one.js', 'two.js' ] }` |
|
285 |
|
286 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
287 | <a name="module_definition--OptionDefinition.OptionDefinition+defaultValue"></a>
|
288 |
|
289 | ### option.defaultValue : <code>\*</code>
|
290 | An initial value for the option.
|
291 |
|
292 | ```js
|
293 | [
|
294 | { name: "files", type: String, multiple: true, defaultValue: [ "one.js" ] },
|
295 | { name: "max", type: Number, defaultValue: 3 }
|
296 | ]
|
297 | ```
|
298 |
|
299 | | # | Command line | .parse() output |
|
300 | | --- | ------------ | ------------ |
|
301 | | 1 | | `{ files: [ 'one.js' ], max: 3 }` |
|
302 | | 2 | `--files two.js` | `{ files: [ 'two.js' ], max: 3 }` |
|
303 | | 3 | `--max 4` | `{ files: [ 'one.js' ], max: 4 }` |
|
304 |
|
305 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
306 | <a name="module_definition--OptionDefinition.OptionDefinition+group"></a>
|
307 |
|
308 | ### option.group : <code>string</code> \| <code>Array.<string></code>
|
309 | When your app has a large amount of options it makes sense to organise them in groups.
|
310 |
|
311 | There are two automatic groups: `_all` (contains all options) and `_none` (contains options without a `group` specified in their definition).
|
312 |
|
313 | ```js
|
314 | [
|
315 | { name: "verbose", group: "standard" },
|
316 | { name: "help", group: [ "standard", "main" ] },
|
317 | { name: "compress", group: [ "server", "main" ] },
|
318 | { name: "static", group: "server" },
|
319 | { name: "debug" }
|
320 | ]
|
321 | ```
|
322 |
|
323 | <table>
|
324 | <tr>
|
325 | <th>#</th><th>Command Line</th><th>.parse() output</th>
|
326 | </tr>
|
327 | <tr>
|
328 | <td>1</td><td><code>--verbose</code></td><td><pre><code>
|
329 | {
|
330 | _all: { verbose: true },
|
331 | standard: { verbose: true }
|
332 | }
|
333 | </code></pre></td>
|
334 | </tr>
|
335 | <tr>
|
336 | <td>2</td><td><code>--debug</code></td><td><pre><code>
|
337 | {
|
338 | _all: { debug: true },
|
339 | _none: { debug: true }
|
340 | }
|
341 | </code></pre></td>
|
342 | </tr>
|
343 | <tr>
|
344 | <td>3</td><td><code>--verbose --debug --compress</code></td><td><pre><code>
|
345 | {
|
346 | _all: {
|
347 | verbose: true,
|
348 | debug: true,
|
349 | compress: true
|
350 | },
|
351 | standard: { verbose: true },
|
352 | server: { compress: true },
|
353 | main: { compress: true },
|
354 | _none: { debug: true }
|
355 | }
|
356 | </code></pre></td>
|
357 | </tr>
|
358 | <tr>
|
359 | <td>4</td><td><code>--compress</code></td><td><pre><code>
|
360 | {
|
361 | _all: { compress: true },
|
362 | server: { compress: true },
|
363 | main: { compress: true }
|
364 | }
|
365 | </code></pre></td>
|
366 | </tr>
|
367 | </table>
|
368 |
|
369 | **Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code>
|
370 |
|
371 |
|
372 | * * *
|
373 |
|
374 | © 2014-17 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/75lb/jsdoc-to-markdown).
|