1 | 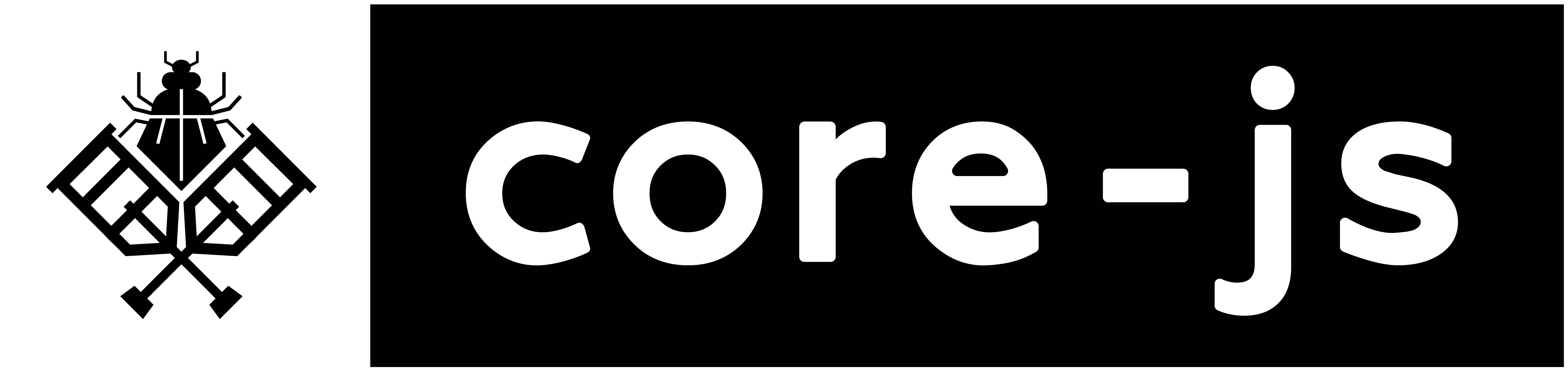
|
2 |
|
3 | <div align="center">
|
4 |
|
5 | [](https://opencollective.com/core-js) [](https://github.com/zloirock/core-js/blob/master/CONTRIBUTING.md) [](https://www.npmjs.com/package/core-js) [](https://npm-stat.com/charts.html?package=core-js&package=core-js-pure&package=core-js-compat&from=2014-11-18)
|
6 |
|
7 | </div>
|
8 |
|
9 | **I highly recommend reading this: [So, what's next?](https://github.com/zloirock/core-js/blob/master/docs/2023-02-14-so-whats-next.md)**
|
10 | ---
|
11 |
|
12 | > Modular standard library for JavaScript. Includes polyfills for [ECMAScript up to 2023](https://github.com/zloirock/core-js#ecmascript): [promises](https://github.com/zloirock/core-js#ecmascript-promise), [symbols](https://github.com/zloirock/core-js#ecmascript-symbol), [collections](https://github.com/zloirock/core-js#ecmascript-collections), iterators, [typed arrays](https://github.com/zloirock/core-js#ecmascript-typed-arrays), many other features, [ECMAScript proposals](https://github.com/zloirock/core-js#ecmascript-proposals), [some cross-platform WHATWG / W3C features and proposals](#web-standards) like [`URL`](https://github.com/zloirock/core-js#url-and-urlsearchparams). You can load only required features or use it without global namespace pollution.
|
13 |
|
14 | ## Raising funds
|
15 |
|
16 | `core-js` isn't backed by a company, so the future of this project depends on you. Become a sponsor or a backer if you are interested in `core-js`: [**Open Collective**](https://opencollective.com/core-js), [**Patreon**](https://patreon.com/zloirock), [**Boosty**](https://boosty.to/zloirock), **Bitcoin ( bc1qlea7544qtsmj2rayg0lthvza9fau63ux0fstcz )**, [**Alipay**](https://user-images.githubusercontent.com/2213682/219464783-c17ad329-17ce-4795-82a7-f609493345ed.png).
|
17 |
|
18 | ---
|
19 |
|
20 | <a href="https://opencollective.com/core-js/sponsor/0/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/0/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/1/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/1/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/2/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/2/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/3/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/3/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/4/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/4/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/5/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/5/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/6/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/6/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/7/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/7/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/8/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/8/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/9/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/9/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/10/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/10/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/11/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/11/avatar.svg"></a>
|
21 |
|
22 | ---
|
23 |
|
24 | <a href="https://opencollective.com/core-js#backers" target="_blank"><img src="https://opencollective.com/core-js/backers.svg?width=890"></a>
|
25 |
|
26 | ---
|
27 |
|
28 | [*Example of usage*](https://tinyurl.com/2mknex43):
|
29 | ```js
|
30 | import 'core-js/actual';
|
31 |
|
32 | Promise.resolve(42).then(it => console.log(it)); // => 42
|
33 |
|
34 | Array.from(new Set([1, 2, 3]).union(new Set([3, 4, 5]))); // => [1, 2, 3, 4, 5]
|
35 |
|
36 | [1, 2].flatMap(it => [it, it]); // => [1, 1, 2, 2]
|
37 |
|
38 | (function * (i) { while (true) yield i++; })(1)
|
39 | .drop(1).take(5)
|
40 | .filter(it => it % 2)
|
41 | .map(it => it ** 2)
|
42 | .toArray(); // => [9, 25]
|
43 |
|
44 | structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
|
45 | ```
|
46 |
|
47 | *You can load only required features*:
|
48 | ```js
|
49 | import 'core-js/actual/promise';
|
50 | import 'core-js/actual/set';
|
51 | import 'core-js/actual/iterator';
|
52 | import 'core-js/actual/array/from';
|
53 | import 'core-js/actual/array/flat-map';
|
54 | import 'core-js/actual/structured-clone';
|
55 |
|
56 | Promise.resolve(42).then(it => console.log(it)); // => 42
|
57 |
|
58 | Array.from(new Set([1, 2, 3]).union(new Set([3, 4, 5]))); // => [1, 2, 3, 4, 5]
|
59 |
|
60 | [1, 2].flatMap(it => [it, it]); // => [1, 1, 2, 2]
|
61 |
|
62 | (function * (i) { while (true) yield i++; })(1)
|
63 | .drop(1).take(5)
|
64 | .filter(it => it % 2)
|
65 | .map(it => it ** 2)
|
66 | .toArray(); // => [9, 25]
|
67 |
|
68 | structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
|
69 | ```
|
70 |
|
71 | *Or use it without global namespace pollution*:
|
72 | ```js
|
73 | import Promise from 'core-js-pure/actual/promise';
|
74 | import Set from 'core-js-pure/actual/set';
|
75 | import Iterator from 'core-js-pure/actual/iterator';
|
76 | import from from 'core-js-pure/actual/array/from';
|
77 | import flatMap from 'core-js-pure/actual/array/flat-map';
|
78 | import structuredClone from 'core-js-pure/actual/structured-clone';
|
79 |
|
80 | Promise.resolve(42).then(it => console.log(it)); // => 42
|
81 |
|
82 | from(new Set([1, 2, 3]).union(new Set([3, 4, 5]))); // => [1, 2, 3, 4, 5]
|
83 |
|
84 | flatMap([1, 2], it => [it, it]); // => [1, 1, 2, 2]
|
85 |
|
86 | Iterator.from(function * (i) { while (true) yield i++; }(1))
|
87 | .drop(1).take(5)
|
88 | .filter(it => it % 2)
|
89 | .map(it => it ** 2)
|
90 | .toArray(); // => [9, 25]
|
91 |
|
92 | structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
|
93 | ```
|
94 |
|
95 | **It's a global version (first 2 examples), for more info see [`core-js` documentation](https://github.com/zloirock/core-js/blob/master/README.md).**
|