1 | [中文文档](https://xieguanglei.github.io/blog/post/dalaran-the-webpack-tool.html)
|
2 |
|
3 | Dalaran is a light weighted tool helping you to simplify your [Webpack](https://webpack.js.org/) config for developing. Compared with some heavy develop environment frameworks, dalaran gives you back the right to build your own progress, with [Gulp](https://gulpjs.com/) to manage tasks.
|
4 |
|
5 | > the name `Dalaran` comes from Dalaran the city, a magic city in video game World of Warcraft.
|
6 |
|
7 | Here the docs go:
|
8 |
|
9 | Get tired of doing webpack config, babel, karma and so on ? Just want to write es6/7 code without too much configuration ? This tool is what you are looking for.
|
10 |
|
11 | Basiclly the tool can do these for you:
|
12 |
|
13 | * config webpack within some default loaders, plugins; config babel within comfortable presets.
|
14 | * automatically find entries and specify dist through given rules (you are still able to customize).
|
15 | * run mocha tests in karma and chrome without much configuration.
|
16 | * provide a development enviroment using webpack-dev-middleware and express.
|
17 | * compile or pack files up when you want to publish or deploy.
|
18 |
|
19 | # Usage
|
20 |
|
21 | Basiclly, you can use the tool when you are:
|
22 |
|
23 | * Writing a front-end application.
|
24 | * Writing a front-end library.
|
25 |
|
26 | You need to dev, build, or test your code, and each of these tasks is provided within a function, so you can use the tool with gulp (or other tools).
|
27 |
|
28 | Your gulpfile may looks like:
|
29 |
|
30 | ```
|
31 | const gulp = requir('gulp');
|
32 | const dalaran = requre('dalaran');
|
33 |
|
34 | const libTasks = dalaran.libraryTasks({...options});
|
35 |
|
36 | gulp.task('dev', libTasks.dev);
|
37 | gulp.task('test', libTasks.test);
|
38 | gulp.task('prepare', function(){
|
39 | // your local tasks
|
40 | })
|
41 | ```
|
42 |
|
43 | ## Tasks for writing an library
|
44 |
|
45 | 4 tasks are provided when you are writing a library: the `dev` task, the `build` task, the `compile` task, the `test` task.
|
46 |
|
47 | You need to create these tasks by call `tasks.libraryTasks(options)`.
|
48 |
|
49 | ### options
|
50 |
|
51 | | name | description | type | default |
|
52 | | ---------------- | ----------------------------------------------------------- | ------- | ------------------ |
|
53 | | port | dev server port | Number | 3000 |
|
54 | | base | base directory of the project | Sting | process.cwd() |
|
55 | | entry | library entry source code file | String | './src/index.js' |
|
56 | | src | the source code directory | String | './src' |
|
57 | | lib | the compiled (to es5, for publishing to npm) code directory | String | './lib' |
|
58 | | demo | the demo pages directory (for development or present) | String | './demo' |
|
59 | | dist | the build file directory (for umd files) | String | './dist' |
|
60 | | umdName | the library's umd name | String | 'foo' |
|
61 | | devSuffix | the bundle file's suffix for development enviroment | String | 'bundle' |
|
62 | | buildSuffix | the bundle file's suffix for build target | String | 'min' |
|
63 | | react | whether to transform JSX | Boolean | false |
|
64 | | loaders | extra webpack loaders | Array | [] |
|
65 | | plugins | extra webpack plugins | Array | [] |
|
66 | | babelPolyfill | whether to import babelPolyfill | Boolean | false |
|
67 | | devCors | whether to enable CORS on dev server | Boolean | true |
|
68 | | watchTest | whether to use watch mode for test task | Boolean | false |
|
69 | | testEntryPattern | file path pattern for test entries | String | 'src/**/*.spec.js' |
|
70 | | lint | whether to enable lint | Boolean | false |
|
71 | | minify | whether to uglify js for build task | Boolean | true |
|
72 | | liveReload | whether to enable live reload | Boolean | fasle |
|
73 | | typescript | whether to enable typescript | Boolean | false |
|
74 |
|
75 | ### directory structure
|
76 |
|
77 | The main project's directory structure may looks like:
|
78 |
|
79 | ```
|
80 | project
|
81 | │ README.md
|
82 | │ package.json
|
83 | │ gulpfile.js
|
84 | └───demo
|
85 | │ foo.html
|
86 | │ foo.js
|
87 | │ bar.html
|
88 | │ bar.js
|
89 | └───dist
|
90 | │ foo.min.js
|
91 | └───lib
|
92 | │ │ index.js
|
93 | │ └───foo
|
94 | │ foo.js
|
95 | └───src
|
96 | │ index.js
|
97 | └───foo
|
98 | foo.js
|
99 | foo.spec.js
|
100 | ```
|
101 |
|
102 | ### dev task
|
103 |
|
104 | ```
|
105 | gulp.task('dev', libTasks.dev);
|
106 | ```
|
107 |
|
108 | You need to put demo pages in the **demo** directory, which by default is './demo'. Each two files with the same basename compose a demo page, for example, `foo.html` and `foo.js` compose the `foo` demo. The html files looks like:
|
109 |
|
110 | ```html
|
111 | <!DOCTYPE html>
|
112 | <html lang="en">
|
113 | <head>
|
114 | <meta charset="UTF-8">
|
115 | <meta name="viewport" content="width=device-width, initial-scale=1.0">
|
116 | <meta http-equiv="X-UA-Compatible" content="ie=edge">
|
117 | <title>Document</title>
|
118 | </head>
|
119 | <body>
|
120 | <script src="./foo.bundle.js"></script>
|
121 | </body>
|
122 | </html>
|
123 | ```
|
124 |
|
125 | You can modify the html file as you like but the only one you should keep is the script tag `<script src="/foo.bundle.js"></script>`, which is the bundled file of `foo.js`. The `bundle` suffix could be changed by `devSuffix` option.
|
126 |
|
127 | The js file looks like:
|
128 |
|
129 | ```
|
130 | import MyLib from '../src/index';
|
131 | // demo code
|
132 | ```
|
133 |
|
134 | Run `gulp dev`, it will open your browser with `http://127.0.0.1:3000` (by default) and show the list of demos as following:
|
135 |
|
136 | 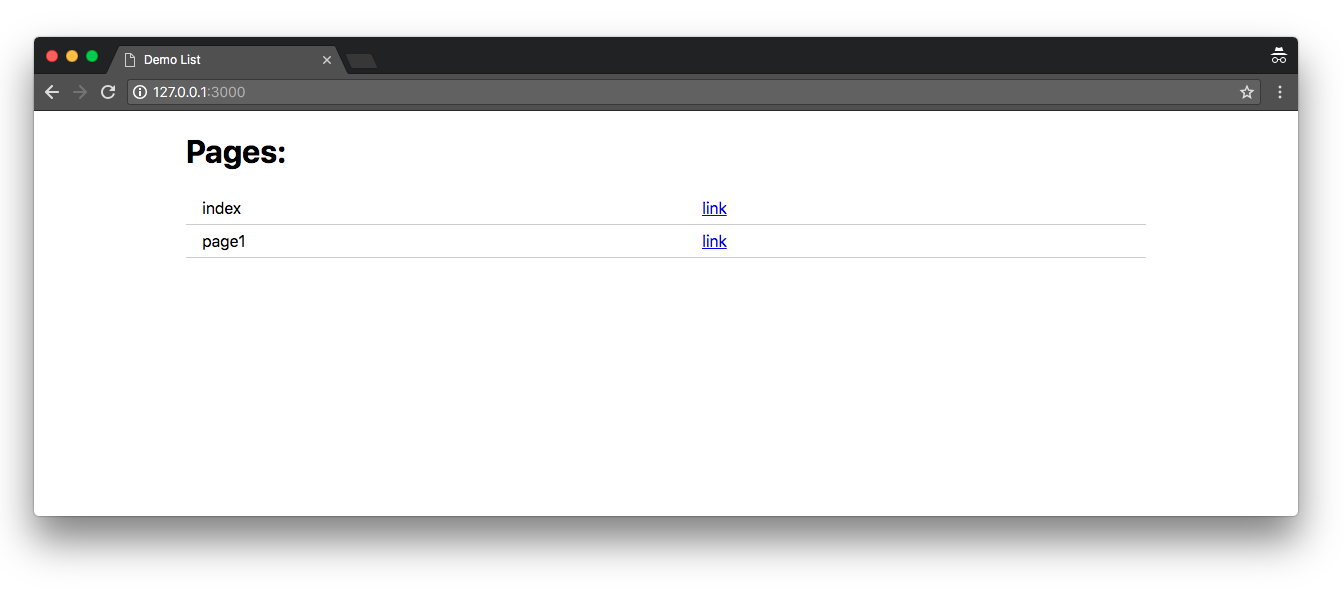
|
137 |
|
138 | Click `link` to enter link pages and do developing.
|
139 |
|
140 | Notice that if you set `lint` to true, a default config file will be used, unless you put a .eslintrc or .tslintrc in your project's root directory.
|
141 |
|
142 | ### test task
|
143 |
|
144 | By configuring the `testEntryPattern` option, you can run tests in Karma and Chrome. A test file (`foo.spec.js` eg.) looks like:
|
145 |
|
146 | ```javascript
|
147 | import expect from 'expect';
|
148 | import MyLib from '../src/index';
|
149 |
|
150 | describe('mylib', function () {
|
151 |
|
152 | it('mylib should be ok', function(){
|
153 | expect(!!MyLib).toBeTruthy();
|
154 | });
|
155 |
|
156 | });
|
157 | ```
|
158 |
|
159 | Run `gulp test` and the test result will be ouputed to console.
|
160 |
|
161 | ### build task
|
162 |
|
163 | ```javascript
|
164 | gulp.task('build', libTasks.build);
|
165 | ```
|
166 |
|
167 | Run `gulp build` will pack your `entry` (by default is './src/index.js') to a umd style file and put it in `dist` directory. You need to provide a `umdName`, whick the file's name will be `${umdName.toLowercase()}.${buildSuffix}.js`. If you load the umd script directly into html, then `window.${umdName}` is available.
|
168 |
|
169 | ### compile task
|
170 |
|
171 | ```javascript
|
172 | gulp.task('build', libTasks.build);
|
173 | ```
|
174 |
|
175 | If your source code contains only `js` file (which means you don't need extra webpack loaders to transform `.less`, `.txt`, `.jpg`), you can `compile` the source file from es6 / jsx to es5 and publish to npm for further use. At the time, you can also avoid pack dependencies to umd file. The compiled files will be put in `lib` directory(you can configure it by the `lib` option).
|
176 |
|
177 | Run `gulp compile`, and compile task will be done.
|
178 |
|
179 | ## Tasks for writing an appliction
|
180 |
|
181 | 3 tasks will be provided for developing an application: the `dev` task, the `build` task, the `test` task.
|
182 |
|
183 | You need to create these tasks by call `tasks.applicationTasks(options)`.
|
184 |
|
185 | ### options
|
186 |
|
187 | | name | description | type | default |
|
188 | | ---------------- | ----------------------------------------------------- | ------- | ------------------ |
|
189 | | port | dev server port | Number | 3000 |
|
190 | | base | base directory of the project | Sting | process.cwd() |
|
191 | | demo | the demo pages directory (for development or present) | String | './demo' |
|
192 | | dist | the build file directory (for umd files) | String | './dist' |
|
193 | | devSuffix | the bundle file's suffix for development enviroment | String | 'bundle' |
|
194 | | buildSuffix | the bundle file's suffix for build target | String | 'bundle' |
|
195 | | react | whether to transform JSX | Boolean | false |
|
196 | | loaders | extra webpack loaders | Array | [] |
|
197 | | plugins | extra webpack plugins | Array | [] |
|
198 | | babelPolyfill | whether to import babelPolyfill | Boolean | false |
|
199 | | devCors | whether to enable CORS on dev server | Boolean | true |
|
200 | | watchTest | whether to use watch mode for test task | Boolean | false |
|
201 | | testEntryPattern | file path pattern for test entries | String | 'src/**/*.spec.js' |
|
202 | | publicPath | deploy publicPath | String | './' |
|
203 | | lint | whether to enable lint | Boolean | false |
|
204 | | minify | whether to uglify js for build task | Boolean | true |
|
205 | | liveReload | whether to enable live reload | Boolean | fasle |
|
206 | | typescript | whether to enable typescript | Boolean | false |
|
207 |
|
208 | Compared with libaray task options, there are several differences:
|
209 |
|
210 | 1. You don't need to provide entry option.
|
211 | 2. You don't need to provide umdName option.
|
212 | 3. You don't need to provide lib option.
|
213 | 4. Default option of buildSuffix is `bundle` but not `min`.
|
214 |
|
215 | ### directory structure
|
216 |
|
217 | The main project's directory structure may looks like:
|
218 |
|
219 | ```bash
|
220 | project
|
221 | │ README.md
|
222 | │ package.json
|
223 | │ gulpfile.js
|
224 | └───demo
|
225 | │ foo.html
|
226 | │ foo.js
|
227 | │ bar.html
|
228 | │ bar.js
|
229 | └───dist
|
230 | │ foo.html
|
231 | │ foo.bundle.js
|
232 | │ bar.html
|
233 | │ bar.bundle.js
|
234 | └───src
|
235 | │ index.js
|
236 | └───foo
|
237 | foo.js
|
238 | foo.spec.js
|
239 | ```
|
240 |
|
241 | Compared with library tasks, there are 2 main differences.
|
242 |
|
243 | 1. lib directory is not necessary anymore.
|
244 | 2. dist directory is a map to demo directory (for library tasks there's only 1 file `${umdName}.${buildSuffix}.js`).
|
245 |
|
246 | ### dev task
|
247 |
|
248 | Dev task is exactly the same with library tasks.
|
249 |
|
250 | ### test task
|
251 |
|
252 | Test task is exactly the same with library tasks.
|
253 |
|
254 | ### build task
|
255 |
|
256 | Unlike library tasks, the build task for application will pack up every page (the demo) into dist directory. It will also copy html files from demo directory to dist directory. You can deploy the js files on server, and load the js on your own page. You can also deploy the whole dist directory to some static server (gh-pages, eg), it works too.
|
257 |
|
258 | > Notice that there's no `compile` task for application.
|
259 |
|
260 | ## If you are still confused
|
261 |
|
262 | You may check the code in `packages` directory for more reference. Each of the project is an example how to use the tool. |
\ | No newline at end of file |