1 | # esri-loader
|
2 |
|
3 | [](https://travis-ci.org/Esri/esri-loader/builds/) [](https://github.com/Esri/esri-loader/releases) [](https://www.npmjs.com/package/esri-loader) [](https://github.com/Esri/esri-loader/blob/master/LICENSE) [](https://github.com/Esri/esri-loader/stargazers)
|
4 |
|
5 | A tiny library to help load modules from either the [4.x](https://developers.arcgis.com/javascript/) or [3.x](https://developers.arcgis.com/javascript/3/) versions of the [ArcGIS API for JavaScript](https://developers.arcgis.com/javascript/) in non-Dojo applications.
|
6 |
|
7 | 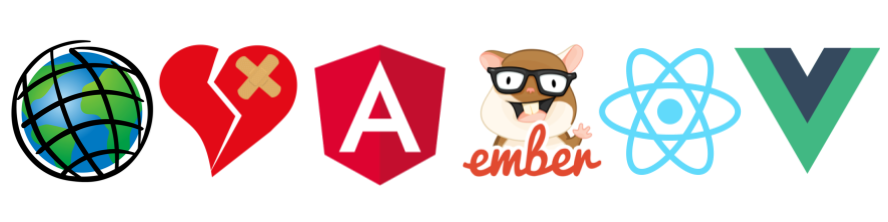
|
8 |
|
9 | See below for more information on [why this library is needed](#why-is-this-needed) and how it can help improve application load performance and allow [using the ArcGIS API in isomorphic/universal applications](#isomorphicuniversal-applications).
|
10 |
|
11 | **NOTE**: If you want to use the ArcGIS API in an [Ember](#ember) or [AngularJS](https://angularjs.org/) (1.x) application, you should use one of these libraries instead:
|
12 |
|
13 | - [ember-esri-loader](https://github.com/Esri/ember-esri-loader) - An Ember addon that wraps this library
|
14 | - [angular-esri-map](https://github.com/Esri/angular-esri-map), which is actually where the code in this library was originally extracted from
|
15 |
|
16 | Otherwise you'll want to follow the [Install](#install) and [Usage](#usage) instructions below to use this library directly in your application.
|
17 |
|
18 | See the [Examples](#examples) section below for links to applications that use this library.
|
19 |
|
20 | ## Table of Contents
|
21 |
|
22 | - [esri-loader](#esri-loader)
|
23 | - [Table of contents](#table-of-contents)
|
24 | - [Install](#install)
|
25 | - [Usage](#usage)
|
26 | - [Loading Modules from the ArcGIS API for JavaScript](#loading-modules-from-the-arcgis-api-for-javascript)
|
27 | - [Lazy Loading the ArcGIS API for JavaScript](#lazy-loading-the-arcgis-api-for-javascript)
|
28 | - [Loading Styles](#loading-styles)
|
29 | - [Why is this needed?](#why-is-this-needed)
|
30 | - [Examples](#examples)
|
31 | - [Advanced Usage](#advanced-usage)
|
32 | - [ArcGIS Types](#arcgis-types)
|
33 | - [Using Classes Synchronously](#using-classes-synchronously)
|
34 | - [Configuring Dojo](#configuring-dojo)
|
35 | - [Overriding ArcGIS Styles](#overriding-arcgis-styles)
|
36 | - [Pre-loading the ArcGIS API for JavaScript](#pre-loading-the-arcgis-api-for-javascript)
|
37 | - [Isomorphic/universal applications](#isomorphicuniversal-applications)
|
38 | - [Using your own script tag](#using-your-own-script-tag)
|
39 | - [Using the esriLoader Global](#using-the-esriloader-global)
|
40 | - [Updating from previous versions](#updating-from-previous-versions)
|
41 | - [From < v1.5](#from--v15)
|
42 | - [From angular-esri-loader](#from-angular-esri-loader)
|
43 | - [Dependencies](#dependencies)
|
44 | - [Browsers](#browsers)
|
45 | - [Promises](#promises)
|
46 | - [Issues](#issues)
|
47 | - [Contributing](#contributing)
|
48 | - [Licensing](#licensing)
|
49 |
|
50 | ## Install
|
51 |
|
52 | ```bash
|
53 | npm install --save esri-loader
|
54 | ```
|
55 |
|
56 | or
|
57 |
|
58 | ```bash
|
59 | yarn add esri-loader
|
60 | ```
|
61 |
|
62 | ## Usage
|
63 |
|
64 | The code snippets below show how to load the ArcGIS API and its modules and then use them to create a map. Where you would place similar code in your application will depend on which application framework you are using. See below for [example applications](#examples).
|
65 |
|
66 | ### Loading Modules from the ArcGIS API for JavaScript
|
67 |
|
68 | #### From the Latest Version
|
69 |
|
70 | Here's an example of how you could load and use the latest 4.x `WebMap` and `MapView` classes in a component to create a map (based on [this sample](https://developers.arcgis.com/javascript/latest/sample-code/sandbox/index.html?sample=webmap-basic)):
|
71 |
|
72 | ```js
|
73 | import { loadModules } from 'esri-loader';
|
74 |
|
75 | // first, we use Dojo's loader to require the map class
|
76 | loadModules(['esri/views/MapView', 'esri/WebMap'])
|
77 | .then(([MapView, WebMap]) => {
|
78 | // then we load a web map from an id
|
79 | var webmap = new WebMap({
|
80 | portalItem: { // autocasts as new PortalItem()
|
81 | id: 'f2e9b762544945f390ca4ac3671cfa72'
|
82 | }
|
83 | });
|
84 | // and we show that map in a container w/ id #viewDiv
|
85 | var view = new MapView({
|
86 | map: webmap,
|
87 | container: 'viewDiv'
|
88 | });
|
89 | })
|
90 | .catch(err => {
|
91 | // handle any errors
|
92 | console.error(err);
|
93 | });
|
94 | ```
|
95 |
|
96 | #### From a Specific Version
|
97 |
|
98 | If you don't want to use the latest version of the ArcGIS API hosted on Esri's CDN, you'll need to pass options with the URL to whichever version you want to use. For example, the snippet below uses v3.x of the ArcGIS API to create a map.
|
99 |
|
100 | ```js
|
101 | import { loadModules } from 'esri-loader';
|
102 |
|
103 | // if the API hasn't already been loaded (i.e. the first time this is run)
|
104 | // loadModules() will call loadScript() and pass these options, which,
|
105 | // in this case are only needed b/c we're using v3.x instead of the latest 4.x
|
106 | const options = { version: '3.30' };
|
107 |
|
108 | loadModules(['esri/map'], options)
|
109 | .then(([Map]) => {
|
110 | // create map with the given options at a DOM node w/ id 'mapNode'
|
111 | let map = new Map('mapNode', {
|
112 | center: [-118, 34.5],
|
113 | zoom: 8,
|
114 | basemap: 'dark-gray'
|
115 | });
|
116 | })
|
117 | .catch(err => {
|
118 | // handle any script or module loading errors
|
119 | console.error(err);
|
120 | });
|
121 | ```
|
122 |
|
123 | You can load the ["next" version of the ArcGIS API](https://github.com/Esri/feedback-js-api-next#esri-loader) by passing `next` as the version.
|
124 |
|
125 | ### From a Specific URL
|
126 |
|
127 | You can also load the modules from a specific URL, for example from a version of the SDK that you host on your own server. In this case, instead of passing the `version` option, you would pass the URL as a string like:
|
128 |
|
129 | ```js
|
130 | const options = { url: `http://server/path/to/esri` };
|
131 | ```
|
132 |
|
133 | ### Lazy Loading the ArcGIS API for JavaScript
|
134 |
|
135 | Lazy loading the ArcGIS API can dramatically improve the initial load performance of your application, especially if your users may never end up visiting any routes that need to show a map or 3D scene. That is why it is the default behavior of esri-loader. In the above snippets, the first time `loadModules()` is called, it will attempt lazy load the ArcGIS API by calling `loadScript()` for you. Subsequent calls to `loadModules()` will not attempt to load the script once `loadScript()` has been called.
|
136 |
|
137 | See below for information on how you can [pre-load the ArcGIS API](#pre-loading-the-arcgis-api-for-javascript) after initial render but before a user visits a route that needs it.
|
138 |
|
139 | ### Loading Styles
|
140 |
|
141 | Before you can use the ArcGIS API in your app, you'll need to load the styles that correspond to the version you are using. Just like the ArcGIS API modules, you'll probably want to [lazy load](#lazy-loading-the-arcgis-api-for-javascript) the styles only once they are needed by the application.
|
142 |
|
143 | #### When you load the script
|
144 |
|
145 | The easiest way to do that is to pass the `css` option to `loadModules()` or `loadScript()`:
|
146 |
|
147 | ```js
|
148 | import { loadModules } from 'esri-loader';
|
149 |
|
150 | // before loading the modules for the first time,
|
151 | // also lazy load the CSS for the version of
|
152 | // the script that you're loading from the CDN
|
153 | const options = { css: true };
|
154 |
|
155 | loadModules(['esri/views/MapView', 'esri/WebMap'], options)
|
156 | .then(([MapView, WebMap]) => {
|
157 | // the styles, script, and modules have all been loaded (in that order)
|
158 | });
|
159 | ```
|
160 |
|
161 | Passing `css: true` does not work when loading the script using the `url` option. In that case you'll need to pass the URL to the styles like: `css: 'http://server/path/to/esri/css/main.css'`.
|
162 |
|
163 | When passing the `css` option to `loadModules()` it actually passes it to `loadScript()`, So you can also use the same values (either `true` or stylesheet URL) when you call `loadScript()` directly.
|
164 |
|
165 | #### Using loadCss()
|
166 |
|
167 | Alternatively, you can use the provided `loadCss()` function to load the ArcGIS styles at any point in your application's life cycle. For example:
|
168 |
|
169 | ```js
|
170 | import { loadCss } from 'esri-loader';
|
171 |
|
172 | // by default loadCss() loads styles for the latest 4.x version
|
173 | loadCss();
|
174 |
|
175 | // or for a specific CDN version
|
176 | loadCss('3.30');
|
177 |
|
178 | // or a from specific URL, like a locally hosted version
|
179 | loadCss('http://server/path/to/esri/css/main.css');
|
180 | ```
|
181 |
|
182 | See below for information on how to [override ArcGIS styles](#overriding-arcgis-styles) that you've lazy loaded with `loadModules()` or `loadCss()`.
|
183 |
|
184 | #### Using traditional means
|
185 |
|
186 | Of course, you don't need to use esri-loader to load the styles. See the [ArcGIS API for JavaScript documentation](https://developers.arcgis.com/javascript/latest/guide/get-api/index.html) for more information on how to load the ArcGIS styles by more traditional means such as adding `<link>` tags to your HTML, or `@import` statements to your CSS.
|
187 |
|
188 | ## Why is this needed?
|
189 |
|
190 | Unfortunately, you can't simply `npm install` the ArcGIS API and then `import` 'esri' modules in a non-Dojo application. The only reliable way to load ArcGIS API for JavaScript modules is using Dojo's AMD loader. However, when using the ArcGIS API in an application built with another framework, you typically want to use the tooling and conventions of that framework rather than the Dojo build system. This library lets you do that by providing an ES module that you can `import` and use to dynamically inject an ArcGIS API script tag in the page and then use its Dojo loader to load only the ArcGIS API modules as needed.
|
191 |
|
192 | There have been a few different solutions to this problem over the years, but only the [@arcgis/webpack-plugin](https://github.com/Esri/arcgis-webpack-plugin) and esri-loader allow you to improve your application's initial load performance, especially on mobile, by [lazy loading the ArcGIS API and modules](#lazy-loading-the-arcgis-api-for-javascript) only when they're needed (i.e. to render a map or 3D scene).
|
193 |
|
194 | [This blog post](https://community.esri.com/people/TWayson-esristaff/blog/2018/05/10/arcgiswebpack-plugin-vs-esri-loader) explains why you would choose one or the other of those solutions, but here's the TLDR:
|
195 | - Are you using ArcGIS API >= v4.7?
|
196 | - Are you using [webpack](https://webpack.github.io/)?
|
197 | - Are you (willing and) able to configure webpack?
|
198 |
|
199 | If you answered "Yes" to all those questions you can use [@arcgis/webpack-plugin](https://github.com/Esri/arcgis-webpack-plugin) in your application and get the benefits of being able to use `import` statements and types for 'esri' modules.
|
200 |
|
201 | Otherwise, you should use esri-loader, which allows you to use the ArcGIS API:
|
202 | - both 4.x _and_ 3.x
|
203 | - with _any_ module loader: (i.e. [rollup.js](https://rollupjs.org/), [Parcel](https://parceljs.org) etc.)
|
204 | - with [whatever cli or boilerplate your team prefers](#examples)
|
205 | - in [isomorphic/universal (server-rendered) applications](#isomorphicuniversal-applications)
|
206 | - without having to load the ArcGIS API just to run your unit tests
|
207 |
|
208 | ## Examples
|
209 |
|
210 | Here are some applications and framework-specific wrapper libraries that use this library. We don't guarantee that these examples are current, so check the version of esri-loader their commit history before using them as a reference. They are presented by framework in alphabetical order - not picking any favorites here :stuck_out_tongue_winking_eye::
|
211 |
|
212 | ### [Angular](https://angular.io/)
|
213 |
|
214 | #### Reusable libraries for Angular
|
215 |
|
216 | - [angular-esri-components](https://github.com/TheKeithStewart/angular-esri-components) - A set of Angular components to work with ArcGIS API for JavaScript v4.3
|
217 |
|
218 | #### Example Angular applications
|
219 |
|
220 | - [angular-cli-esri-map](https://github.com/Esri/angular-cli-esri-map) - Example of how to build a simple mapping component using Angular CLI.
|
221 |
|
222 | ### [CanJS](https://canjs.com/)
|
223 |
|
224 | - [can-arcgis](https://github.com/roemhildtg/can-arcgis) - CanJS configurable mapping app (inspired by [cmv-app](https://github.com/cmv/cmv-app)) and components built for the ArcGIS JS API 4.x, bundled with [StealJS](https://stealjs.com/)
|
225 |
|
226 | ### [Choo](https://choo.io/)
|
227 |
|
228 | - [esri-choo-example](https://github.com/jwasilgeo/esri-choo-example) - An example Choo application that shows how to use esri-loader to create a custom map view.
|
229 |
|
230 | ### [Dojo 2+](https://dojo.io)
|
231 |
|
232 | - [dojo-esri-loader](https://github.com/odoe/dojo-esri-loader) - Dojo 5 app with esri-loader ([blog post](https://odoe.net/blog/dojo-framework-with-arcgis-api-for-javascript/))
|
233 |
|
234 | - [esri-dojo](https://github.com/jamesmilneruk/esri-dojo) - An example of how to use Esri Loader with Dojo 2+. This example is a simple map that allows you to place markers on it.
|
235 |
|
236 | ### [Electron](https://electron.atom.io/)
|
237 |
|
238 | - [ng-cli-electron-esri](https://github.com/TheKeithStewart/ng-cli-electron-esri) - This project is meant to demonstrate how to run a mapping application using the ArcGIS API for JavaScript inside of Electron
|
239 |
|
240 | ### [Ember](https://www.emberjs.com/)
|
241 |
|
242 | See the [examples over at ember-esri-loader](https://github.com/Esri/ember-esri-loader/#examples)
|
243 |
|
244 | ### [Glimmer.js](https://glimmerjs.com/)
|
245 |
|
246 | - [esri-glimmer-example](https://github.com/tomwayson/esri-glimmer-example) - An example of how to use the ArcGIS API for JavaScript in a https://glimmerjs.com/ application
|
247 |
|
248 | ### [Hyperapp](https://hyperapp.js.org/)
|
249 |
|
250 | - [esri-hyperapp-example](https://github.com/jwasilgeo/esri-hyperapp-example) - An example Hyperapp application that shows how to use esri-loader to create a custom map view and component.
|
251 |
|
252 | ### [Ionic](https://ionicframework.com/)
|
253 |
|
254 | - [ionic2-esri-map](https://github.com/andygup/ionic2-esri-map) - Prototype app demonstrating how to use Ionic 3+ with the ArcGIS API for JavaScript
|
255 |
|
256 | ### [Preact](https://github.com/developit/preact)
|
257 |
|
258 | - [esri-preact-pwa](https://github.com/tomwayson/esri-preact-pwa) - An example progressive web app (PWA) using the ArcGIS API for JavaScript built with Preact
|
259 |
|
260 | ### [React](https://facebook.github.io/react/)
|
261 |
|
262 | #### Reusable libraries for React
|
263 |
|
264 | - [react-arcgis](https://github.com/Esri/react-arcgis) - React component kit for Esri ArcGIS JS API
|
265 | - [esri-loader-react](https://github.com/davetimmins/esri-loader-react) - A React component wrapper around esri-loader ([blog post](https://davetimmins.github.io/2017/07/19/esri-loader-react/))
|
266 | - [arcgis-react-redux-legend](https://github.com/davetimmins/arcgis-react-redux-legend) - Legend control for ArcGIS JS v4 using React and Redux
|
267 |
|
268 | #### Example React applications
|
269 | - [create-arcgis-app](https://github.com/tomwayson/create-arcgis-app/) - An example of how to use the ArcGIS platform in an application created with Create React App and React Router.
|
270 | - [next-arcgis-app](https://github.com/tomwayson/next-arcgis-app/) - An example of how to use the ArcGIS platform in an application built with Next.js
|
271 | - [esri-loader-react-starter-kit](https://github.com/tomwayson/esri-loader-react-starter-kit) - A fork of the [react-starter-kit](https://github.com/kriasoft/react-starter-kit) showing how to use esri-loader in an isomorphic/universal React application
|
272 | - [create-react-app-esri-loader](https://github.com/davetimmins/create-react-app-esri-loader/) - An example create-react-app application that uses [esri-loader-react](https://github.com/davetimmins/esri-loader-react) to load the ArcGIS API
|
273 | - [React-Typescript-App-with-ArcGIS-JSAPI](https://github.com/guzhongren/React-Typescript-App-with-ArcGIS-JSAPI) - An example create-react-app application that uses [esri-loader](https://github.com/Esri/esri-loader), [esri-loader-react](https://github.com/davetimmins/esri-loader-react), [Typescript](https://www.typescriptlang.org/), [Webpack3](https://webpack.js.org/) to create MapView
|
274 |
|
275 | ### [Riot](https://riot.js.org/)
|
276 |
|
277 | - [esri-riot-example](https://github.com/jwasilgeo/esri-riot-example) - An example Riot application that shows how to use esri-loader to create a custom `<esri-map-view>` component.
|
278 |
|
279 | ### [Stencil](https://stenciljs.com/)
|
280 |
|
281 | - [esri-stencil-example](https://github.com/Dzeneralen/esri-stencil-example) - An example Stencil application that shows how to use esri-loader to create a custom map view component and implement some basic routing controlling the map state
|
282 |
|
283 | ### [Vue.js](https://vuejs.org/)
|
284 |
|
285 | - [CreateMap](https://github.com/oppoudel/CreateMap) - Create Map: City of Baltimore - https://gis.baltimorecity.gov/createmap/#/
|
286 | - [City of Baltimore: Map Gallery](https://github.com/oppoudel/MapGallery_Vue) - Map Gallery built with Vue.js that uses this library to load the ArcGIS API
|
287 | - [vue-jsapi4](https://github.com/odoe/vue-jsapi4) - An example of how to use the [ArcGIS API for Javascript](https://developers.arcgis.com/javascript/) in a [NUXT](https://nuxtjs.org/) application ([blog post](https://odoe.net/blog/arcgis-api-4-for-js-with-vue-cli-and-nuxt/), [video](https://youtu.be/hqJzzgM8seo))
|
288 | - [esri-vue-cli-example](https://github.com/tomwayson/esri-vue-cli-example) - An example of how to use the [ArcGIS API for JavaScript 3.x](https://developers.arcgis.com/javascript/3/) in a [vue-cli](https://github.com/vuejs/vue-cli) application
|
289 |
|
290 | ## Advanced Usage
|
291 |
|
292 | ### [FAQS](https://github.com/Esri/esri-loader/issues?utf8=%E2%9C%93&q=label%3AFAQ+sort%3Aupdated-desc)
|
293 |
|
294 | ### ArcGIS Types
|
295 |
|
296 | This library doesn't make any assumptions about which version of the ArcGIS API you are using, so you will have to install the appropriate types. Furthermore, because you don't `import` esri modules directly with esri-loader, you'll have to follow the instructions below to use the types in your application.
|
297 |
|
298 | #### 4.x Types
|
299 |
|
300 | Follow [these instructions](https://github.com/Esri/jsapi-resources/tree/master/4.x/typescript) to install the 4.x types.
|
301 |
|
302 | After installing the 4.x types, you can use the `__esri` namespace for the types as seen in [this example](https://github.com/kgs916/angular2-esri4-components/blob/68861b286fd3a4814c495c2bd723e336e917ced2/src/lib/esri4-map/esri4-map.component.ts#L20-L26).
|
303 |
|
304 | #### 3.x Types
|
305 |
|
306 | You can use [these instructions](https://github.com/Esri/jsapi-resources/tree/master/3.x/typescript) to install the 3.x types.
|
307 |
|
308 | The `__esri` namespace is not defined for 3.x types, but you can `import * as esri from 'esri';` to use the types [as shown here](https://github.com/Esri/angular-cli-esri-map/issues/17#issue-360490589).
|
309 |
|
310 | #### TypeScript import()
|
311 |
|
312 | TypeScript 2.9 added a way to `import()` types which allows types to be imported without importing the module. For more information on import types see [this post](https://davidea.st/articles/typescript-2-9-import-types). You can use this as an alternative to the 4.x `_esri` namespace or `import * as esri from 'esri'` for 3.x.
|
313 |
|
314 | After you've installed the [4.x](#4x-types) or [3.x](#4x-types) types as described above, you can then use TypeScript's `import()` like:
|
315 |
|
316 | ```ts
|
317 | // define a type that is an array of the 4.x types you are using
|
318 | // and indicate that loadModules() will resolve with that type
|
319 | type MapModules = [typeof import("esri/WebMap"), typeof import("esri/views/MapView")];
|
320 | const [WebMap, MapView] = await (loadModules(["esri/WebMap", "esri/views/MapView"]) as Promise<MapModules>);
|
321 | // the returned objects now have type
|
322 | const webmap = new WebMap({portalItem: {id: this.webmapid}});
|
323 | ```
|
324 |
|
325 | A more complete 4.x sample can be [seen here](https://codesandbox.io/s/xv8mw2890w?fontsize=14&module=%2Fsrc%2Fmapping.ts).
|
326 |
|
327 | This also works with the 3.x types:
|
328 |
|
329 | ```ts
|
330 | // define a type that is an array of the 3.x types you are using
|
331 | // and indicate that loadModules() will resolve with that type
|
332 | type MapModules = [typeof import("esri/map"), typeof import("esri/geometry/Extent")];
|
333 | const [Map, Extent] = await (loadModules(["esri/map", "esri/geometry/Extent"], options) as Promise<MapModules>);
|
334 | // the returned objects now have type
|
335 | let map = new Map("viewDiv"...
|
336 | ```
|
337 | A more complete 3.x sample can be [seen here](https://codesandbox.io/s/rj6jloy4nm?fontsize=14&module=%2Fsrc%2Fmapping.ts).
|
338 |
|
339 | #### Types in Angular CLI Applications
|
340 |
|
341 | For Angular CLI applications, you will also need to add "arcgis-js-api" to `compilerOptions.types` in src/tsconfig.app.json and src/tsconfig.spec.json [as shown here](https://gist.github.com/tomwayson/e6260adfd56c2529313936528b8adacd#adding-the-arcgis-api-for-javascript-types).
|
342 |
|
343 | ### Using Classes Synchronously
|
344 |
|
345 | Let's say you need to create a map in one component, and then later in another component add a graphic to that map. Unlike creating a map, creating a graphic and adding it to a map is ordinarily a synchronous operation, so it can be inconvenient to have to wait for `loadModules()` just to load the `Graphic` class. One way to handle this is have the function that creates the map _also_ load the `Graphic` class before its needed. You can then hold onto that class for later use to be exposed by a function like `addGraphicToMap(view, graphicJson)`:
|
346 |
|
347 | ```javascript
|
348 | // utils/map.js
|
349 | import { loadModules } from 'esri-loader';
|
350 |
|
351 | // NOTE: module, not global scope
|
352 | let _Graphic;
|
353 |
|
354 | // this will be called by the map component
|
355 | export function loadMap(element, mapOptions) {
|
356 | // NOTE:
|
357 | return loadModules(['esri/Map', 'esri/views/MapView', 'esri/Graphic'], {
|
358 | css: true
|
359 | }).then(([Map, MapView, Graphic]) => {
|
360 | // hold onto the graphic class for later use
|
361 | _Graphic = Graphic;
|
362 | // create the Map
|
363 | const map = new Map(mapOptions);
|
364 | // return a view showing the map at the element
|
365 | return new MapView({
|
366 | map,
|
367 | container: element
|
368 | });
|
369 | });
|
370 | }
|
371 |
|
372 | // this will be called by the component that needs to add the graphic to the map
|
373 | export function addGraphicToMap(view, graphicJson) {
|
374 | // make sure that the graphic class has already been loaded
|
375 | if (!_Graphic) {
|
376 | throw new Error('You must load a map before creating new graphics');
|
377 | }
|
378 | view.graphics.add(new _Graphic(graphicJson));
|
379 | }
|
380 | ```
|
381 |
|
382 | You can [see this pattern in use in a real-world application](https://github.com/tomwayson/create-arcgis-app/blob/master/src/utils/map.js).
|
383 |
|
384 | See [#124 (comment)](https://github.com/Esri/esri-loader/issues/124#issuecomment-408482410) and [#71 (comment)](https://github.com/Esri/esri-loader/issues/71#issuecomment-381356848) for more background on this pattern.
|
385 |
|
386 | ### Configuring Dojo
|
387 |
|
388 | You can pass a [`dojoConfig`](https://dojotoolkit.org/documentation/tutorials/1.10/dojo_config/) option to `loadModules()` or `loadScript()` to configure Dojo before the script tag is loaded. This is useful if you want to use esri-loader to load Dojo packages that are not included in the ArcGIS API for JavaScript such as [FlareClusterLayer](https://github.com/nickcam/FlareClusterLayer).
|
389 |
|
390 | ```js
|
391 | import { loadModules } from 'esri-loader';
|
392 |
|
393 | // in this case options are only needed so we can configure Dojo before loading the API
|
394 | const options = {
|
395 | // tell Dojo where to load other packages
|
396 | dojoConfig: {
|
397 | async: true,
|
398 | packages: [
|
399 | {
|
400 | location: '/path/to/fcl',
|
401 | name: 'fcl'
|
402 | }
|
403 | ]
|
404 | }
|
405 | };
|
406 |
|
407 | loadModules(['esri/map', 'fcl/FlareClusterLayer_v3'], options)
|
408 | .then(([Map, FlareClusterLayer]) => {
|
409 | // you can now create a new FlareClusterLayer and add it to a new Map
|
410 | })
|
411 | .catch(err => {
|
412 | // handle any errors
|
413 | console.error(err);
|
414 | });
|
415 | ```
|
416 |
|
417 | ### Overriding ArcGIS Styles
|
418 |
|
419 | If you want to override ArcGIS styles that you have [lazy loaded using `loadModules()` or `loadCss()`](#loading-styles), you may need to insert the ArcGIS styles into the document _above_ your custom styles in order to ensure the [rules of CSS precedence](https://css-tricks.com/precedence-css-order-css-matters/) are applied correctly. For this reason, `loadCss()` accepts a [selector](https://developer.mozilla.org/en-US/docs/Web/API/Document_object_model/Locating_DOM_elements_using_selectors#Selectors) (string) as optional second argument that it uses to query the DOM node (i.e. `<link>` or `<script>`) that contains your custom styles and then insert the ArcGIS styles above that node. You can also pass that selector as the `insertCssBefore` option to `loadModules()`:
|
420 |
|
421 | ```js
|
422 | import { loadModules } from 'esri-loader';
|
423 |
|
424 | // lazy load the CSS before loading the modules
|
425 | const options = {
|
426 | css: true,
|
427 | // insert the stylesheet link above the first <style> tag on the page
|
428 | insertCssBefore: 'style'
|
429 | };
|
430 |
|
431 | // before loading the modules, this will call:
|
432 | // loadCss('https://js.arcgis.com/4.12/themes/light/main.css', 'style')
|
433 | loadModules(['esri/views/MapView', 'esri/WebMap'], options);
|
434 | ```
|
435 |
|
436 | Alternatively you could insert it before the first `<link>` tag w/ `insertCssBefore: 'link[rel="stylesheet"]'`, etc.
|
437 |
|
438 | ### Pre-loading the ArcGIS API for JavaScript
|
439 |
|
440 | If you have good reason to believe that the user is going to transition to a map route, you may want to start pre-loading the ArcGIS API as soon as possible w/o blocking rendering, for example:
|
441 |
|
442 | ```js
|
443 | import { loadScript, loadModules } from 'esri-loader';
|
444 |
|
445 | // preload the ArcGIS API
|
446 | // NOTE: in this case, we're not passing any options to loadScript()
|
447 | // so it will default to loading the latest 4.x version of the API from the CDN
|
448 | this.loadScriptPromise = loadScript();
|
449 |
|
450 | // later, for example once a component has been rendered,
|
451 | // you can wait for the above promise to resolve (if it hasn't already)
|
452 | this.loadScriptPromise
|
453 | .then(() => {
|
454 | // you can now load the map modules and create the map
|
455 | // by using loadModules()
|
456 | })
|
457 | .catch(err => {
|
458 | // handle any script loading errors
|
459 | console.error(err);
|
460 | });
|
461 | ```
|
462 |
|
463 | ### Isomorphic/universal applications
|
464 |
|
465 | This library also allows you to use the ArcGIS API in [isomorphic or universal](https://medium.com/airbnb-engineering/isomorphic-javascript-the-future-of-web-apps-10882b7a2ebc#.4nyzv6jea) applications that are rendered on the server. There's really no difference in how you invoke the functions exposed by this library, however you should avoid trying to call them from any code that runs on the server. The easiest way to do this is to use them in component lifecyle hooks that are only invoked in a browser, for example, React's [`componentDidMount`](https://reactjs.org/docs/react-component.html#componentdidmount) or Vue's [mounted](https://vuejs.org/v2/api/#mounted). See [tomwayson/esri-loader-react-starter-kit](https://github.com/tomwayson/esri-loader-react-starter-kit/) for [an example of a component that lazy loads the ArcGIS API and renders a map only once a specific route is loaded in a browser](https://github.com/tomwayson/esri-loader-react-starter-kit/commit/a513b7fe207a809105fcb621a26a687cc47918b4).
|
466 |
|
467 | Alternatively, you could use checks like the following to ensure these functions aren't invoked on the server:
|
468 |
|
469 | ```js
|
470 | import { loadScript } from 'esri-loader';
|
471 |
|
472 | if (typeof window !== 'undefined') {
|
473 | // this is running in a browser,
|
474 | // pre-load the ArcGIS API for later use in components
|
475 | this.loadScriptPromise = loadScript();
|
476 | }
|
477 | ```
|
478 |
|
479 | ### Using your own script tag
|
480 |
|
481 | It is possible to use this library only to load modules (i.e. not to lazy load or pre-load the ArcGIS API). In this case you will need to add a `data-esri-loader` attribute to the script tag you use to load the ArcGIS API for JavaScript. Example:
|
482 |
|
483 | ```html
|
484 | <!-- index.html -->
|
485 | <script src="https://js.arcgis.com/4.11/" data-esri-loader="loaded"></script>
|
486 | ```
|
487 |
|
488 | ### Using the esriLoader Global
|
489 |
|
490 | Typically you would [install the esri-loader package](#install) and then `import` the functions you need as shown in all the above examples. However, esri-loader is also distributed as an ES5 [UMD](http://jargon.js.org/_glossary/UMD.md) bundle on [UNPKG](https://unpkg.com/) so that you can load it via a script tag and use the above functions from the `esriLoader` global.
|
491 |
|
492 | ```html
|
493 | <script src="https://unpkg.com/esri-loader"></script>
|
494 | <script>
|
495 | esriLoader.loadModules(['esri/views/MapView', 'esri/WebMap'])
|
496 | .then(([MapView, WebMap]) => {
|
497 | // use MapView and WebMap classes as shown above
|
498 | });
|
499 | </script>
|
500 | ```
|
501 |
|
502 | ## Updating from previous versions
|
503 |
|
504 | ### From < v1.5
|
505 |
|
506 | If you have an application using a version that is less than v1.5, [this commit](https://github.com/odoe/vue-jsapi4/pull/1/commits/4cb6413c0ea31fdd09e94f3a0ce0d1669a9fd5ad) shows the kinds of changes you'll need to make. In most cases, you should be able to replace a series of calls to `isLoaded()`, `bootstrap()`, and `dojoRequire()` with a single call to `loadModules()`.
|
507 |
|
508 | ### From angular-esri-loader
|
509 |
|
510 | The angular-esri-loader wrapper library is no longer needed and has been deprecated in favor of using esri-loader directly. See [this issue](https://github.com/Esri/esri-loader/issues/75) for suggestions on how to replace angular-esri-loader with the latest version of esri-loader.
|
511 |
|
512 | ## Dependencies
|
513 |
|
514 | ### Browsers
|
515 |
|
516 | This library doesn't have any external dependencies, but the functions it exposes to load the ArcGIS API and its modules expect to be run in a browser. This library officially supports [the same browsers that are supported by the latest version of the ArcGIS API for JavaScript](https://developers.arcgis.com/javascript/latest/guide/system-requirements/index.html#supported-browsers). Since this library also works with [v3.x of the ArcGIS API](https://developers.arcgis.com/javascript/3/), the community [has made some effort](https://github.com/Esri/esri-loader/pull/67) to get it to work with [some of the older browsers supported by 3.x](https://developers.arcgis.com/javascript/3/jshelp/supported_browsers.html) like IE < 11.
|
517 |
|
518 | You cannot run the ArcGIS API for JavaScript in [Node.js](https://nodejs.org/), but you _can_ use this library in [isomorphic/universal applications](#isomorphicuniversal-applications) as well as [Electron](#electron). If you need to execute requests to ArcGIS REST services from something like a Node.js CLI application, see [arcgis-rest-js](https://github.com/Esri/arcgis-rest-js).
|
519 |
|
520 | ### Promises
|
521 |
|
522 | Since v1.5 asynchronous functions like `loadModules()` and `loadScript()` return [`Promise`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise)s, so if your application has to support [browsers that don't support Promise (i.e. IE)](https://caniuse.com/#search=promise) you have a few options.
|
523 |
|
524 | If there's already a Promise implementation loaded on the page you can configure esri-loader to use that implementation. For example, in [ember-esri-loader](https://github.com/Esri/ember-esri-loader), we configure esri-loader to use the RSVP Promise implementation included with Ember.js.
|
525 |
|
526 | ```js
|
527 | import { utils } from 'esri-loader';
|
528 |
|
529 | init () {
|
530 | this._super(...arguments);
|
531 | // have esriLoader use Ember's RSVP promise
|
532 | utils.Promise = Ember.RSVP.Promise;
|
533 | },
|
534 | ```
|
535 |
|
536 | Otherwise, you should consider using a [Promise polyfill](https://www.google.com/search?q=promise+polyfill), ideally [only when needed](https://philipwalton.com/articles/loading-polyfills-only-when-needed/).
|
537 |
|
538 | ## Issues
|
539 |
|
540 | Find a bug or want to request a new feature? Please let us know by [submitting an issue](https://github.com/Esri/esri-loader/issues/).
|
541 |
|
542 | ## Contributing
|
543 |
|
544 | Esri welcomes contributions from anyone and everyone. Please see our [guidelines for contributing](https://github.com/esri/contributing).
|
545 |
|
546 | ## Licensing
|
547 |
|
548 | Copyright © 2016-2019 Esri
|
549 |
|
550 | Licensed under the Apache License, Version 2.0 (the "License");
|
551 | you may not use this file except in compliance with the License.
|
552 | You may obtain a copy of the License at
|
553 |
|
554 | https://www.apache.org/licenses/LICENSE-2.0
|
555 |
|
556 | Unless required by applicable law or agreed to in writing, software
|
557 | distributed under the License is distributed on an "AS IS" BASIS,
|
558 | WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
559 | See the License for the specific language governing permissions and
|
560 | limitations under the License.
|
561 |
|
562 | A copy of the license is available in the repository's [LICENSE]( ./LICENSE) file.
|
563 |
|
\ | No newline at end of file |