1 | A [BigInt] can represent whole numbers larger than 2โตยณ - 1 [(1)].<br>
|
2 | ๐ฆ [Node.js](https://www.npmjs.com/package/extra-bigint),
|
3 | ๐ [Web](https://www.npmjs.com/package/extra-bigint.web),
|
4 | ๐ [Files](https://unpkg.com/extra-bigint/),
|
5 | ๐ฐ [Docs](https://nodef.github.io/extra-bigint/),
|
6 | ๐ [Wiki](https://github.com/nodef/extra-bigint/wiki/).
|
7 |
|
8 | *ES2020* introduced **BigInt** as a built-in object. **BigInt** enables us to
|
9 | represent integers with arbitrary precision, allowing us to perform mathematical
|
10 | operations on large integers [(2)]. This package includes common bigint
|
11 | functions related to querying **about** numbers, **comparing** numbers,
|
12 | performing **rounded division**, performing **modulo** operations, **controlling**
|
13 | **range** of numbers, performing **arithmetic** operations, obtaining **divisors**
|
14 | of a number (and related operations), getting the number of possible
|
15 | **arrangements** of a set of objects, performing **geometry**-related
|
16 | calculations, performing basic **statistical** analysis, and finding various
|
17 | **statistical means**.
|
18 |
|
19 | This package is available in *Node.js* and *Web* formats. To use it on the web,
|
20 | simply use the `extra_bigint` global variable after loading with a `<script>`
|
21 | tag from the [jsDelivr CDN].
|
22 |
|
23 | > Stability: [Experimental](https://www.youtube.com/watch?v=L1j93RnIxEo).
|
24 |
|
25 | [BigInt]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt
|
26 | [(1)]: https://www.geeksforgeeks.org/bigint-in-javascript/
|
27 | [(2)]: https://www.smashingmagazine.com/2019/07/essential-guide-javascript-newest-data-type-bigint/
|
28 | [jsDelivr CDN]: https://cdn.jsdelivr.net/npm/extra-bigint.web/index.js
|
29 |
|
30 | <br>
|
31 |
|
32 | ```javascript
|
33 | const xbigint = require('extra-bigint');
|
34 | // import * as xbigint from "extra-bigint";
|
35 | // import * as xbigint from "https://unpkg.com/extra-bigint/index.mjs"; (deno)
|
36 |
|
37 | xbigint.isPrime(113n);
|
38 | // โ true
|
39 |
|
40 | xbigint.floorDiv(7n, 3n);
|
41 | // โ 2n
|
42 |
|
43 | xbigint.sqrt(81n);
|
44 | // โ 9n
|
45 |
|
46 | xbigint.lcm(2n, 3n, 4n);
|
47 | // โ 12n
|
48 |
|
49 | xbigint.log2(8n);
|
50 | // โ 3n
|
51 |
|
52 | xbigint.sum(1n, 2n, 3n, 4n);
|
53 | // โ 10n
|
54 |
|
55 | xbigint.mean(1n, 7n, 8n);
|
56 | // โ 5n
|
57 | ```
|
58 |
|
59 | <br>
|
60 | <br>
|
61 |
|
62 |
|
63 | ## Index
|
64 |
|
65 | | Property | Description |
|
66 | | ---- | ---- |
|
67 | | [is] | Check if value is a bigint. |
|
68 | | | |
|
69 | | [compare] | Compare two bigints. |
|
70 | | | |
|
71 | | [abs] | Get the absolute of a bigint. |
|
72 | | [sign] | Get the sign of a bigint. |
|
73 | | | |
|
74 | | [floorDiv] | Perform floor-divison of two bigints (\\). |
|
75 | | [ceilDiv] | Perform ceiling-divison of two bigints. |
|
76 | | [roundDiv] | Perform rounded-divison of two bigints. |
|
77 | | | |
|
78 | | [rem] | Find the remainder of x/y with sign of x (truncated division). |
|
79 | | [mod] | Find the remainder of x/y with sign of y (floored division). |
|
80 | | [modp] | Find the remainder of x/y with +ve sign (euclidean division). |
|
81 | | | |
|
82 | | [constrain] | Constrain a bigint within a minimum and a maximum value. |
|
83 | | [remap] | Re-map a bigint from one range to another. |
|
84 | | [lerp] | Linearly interpolate a bigint between two bigints. |
|
85 | | | |
|
86 | | [isPow2] | Check if bigint is a power-of-2. |
|
87 | | [isPow10] | Check if bigint is a power-of-10. |
|
88 | | [prevPow2] | Find largest power-of-2 less than or equal to given bigint. |
|
89 | | [prevPow10] | Find largest power-of-10 less than or equal to given bigint. |
|
90 | | [nextPow2] | Find smallest power-of-2 greater than or equal to given bigint. |
|
91 | | [nextPow10] | Find smallest power-of-10 greater than or equal to given bigint. |
|
92 | | [log2] | Find the base-2 logarithm of a bigint. |
|
93 | | [log10] | Find the base-10 logarithm of a bigint. |
|
94 | | | |
|
95 | | [sqrt] | Find the square root of a bigint. |
|
96 | | [cbrt] | Find the cube root of a bigint. |
|
97 | | [root] | Find the nth root of a bigint. |
|
98 | | | |
|
99 | | [properDivisors] | List all divisors of a bigint, except itself. |
|
100 | | [aliquotSum] | Sum all proper divisors of a bigint. |
|
101 | | [minPrimeFactor] | Find the least prime number which divides a bigint. |
|
102 | | [maxPrimeFactor] | Find the greatest prime number which divides a bigint. |
|
103 | | [primeFactors] | Find the prime factors of a bigint. |
|
104 | | [primeExponentials] | Find the prime factors and respective exponents of a bigint. |
|
105 | | [isPrime] | Check if bigint is prime. |
|
106 | | [gcd] | Find the greatest common divisor of bigints. |
|
107 | | [lcm] | Find the least common multiple of bigints. |
|
108 | | | |
|
109 | | [factorial] | Find the factorial of a bigint. |
|
110 | | [binomial] | Find the number of ways to choose k elements from a set of n elements. |
|
111 | | [multinomial] | Find the number of ways to put n objects in m bins (n=sum(kแตข)). |
|
112 | | | |
|
113 | | [hypot] | Find the length of hypotenuse. |
|
114 | | | |
|
115 | | [sum] | Find the sum of bigints (ฮฃ). |
|
116 | | [product] | Find the product of bigints (โ). |
|
117 | | [median] | Find the value separating the higher and lower halves of bigints. |
|
118 | | [modes] | Find the values that appear most often. |
|
119 | | [min] | Find the smallest bigint. |
|
120 | | [max] | Find the largest bigint. |
|
121 | | [range] | Find the minimum and maximum bigint. |
|
122 | | [variance] | Find the mean of squared deviation of bigints from its mean. |
|
123 | | | |
|
124 | | [arithmeticMean] | Find the arithmetic mean of bigints (ยต). |
|
125 | | [geometricMean] | Find the geometric mean of bigints. |
|
126 | | [harmonicMean] | Find the harmonic mean of bigints. |
|
127 | | [quadriaticMean] | Find the quadriatic mean of bigints. |
|
128 | | [cubicMean] | Find the cubic mean of bigints. |
|
129 |
|
130 | <br>
|
131 | <br>
|
132 |
|
133 |
|
134 | [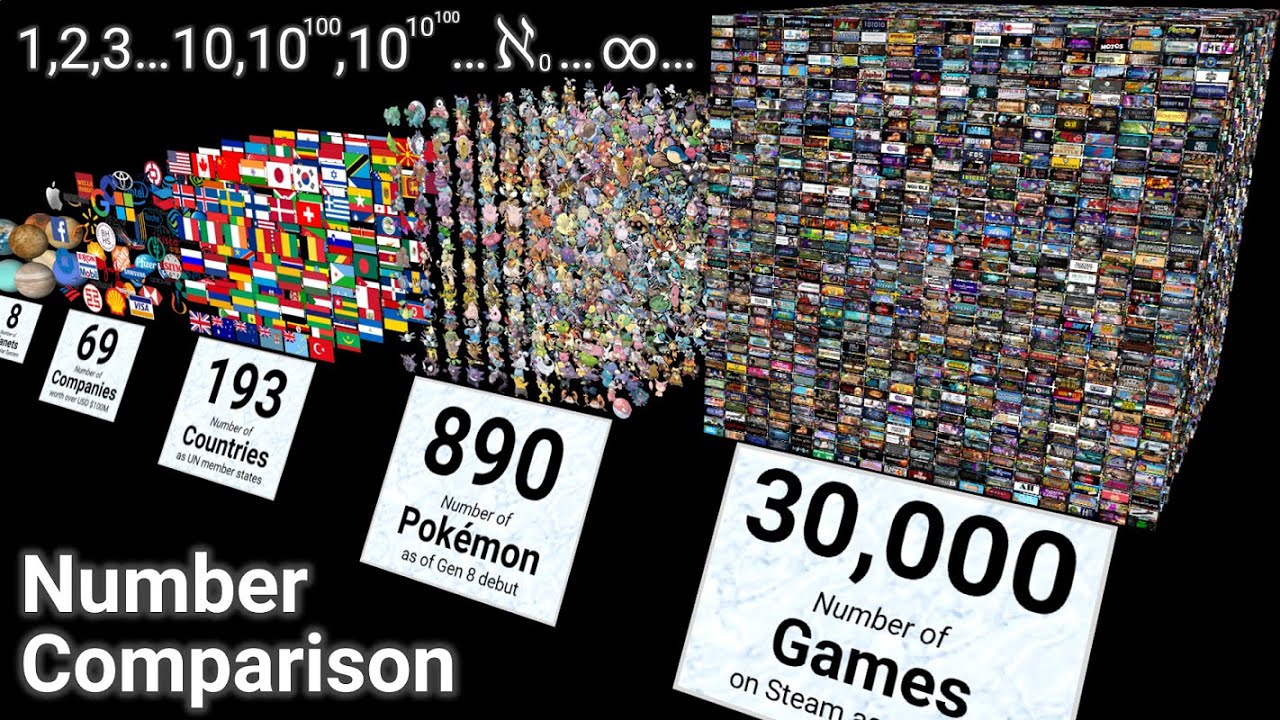](https://www.youtube.com/watch?v=RJS3Z2DYEO4)<br>
|
135 | [](https://nodef.github.io)
|
136 | [](https://zenodo.org/badge/latestdoi/274701321)
|
137 | [](https://coveralls.io/github/nodef/extra-bigint?branch=master)
|
138 | [](https://codeclimate.com/github/nodef/extra-bigint/test_coverage)
|
139 | [](https://codeclimate.com/github/nodef/extra-bigint/maintainability)
|
140 |
|
141 |
|
142 | [is]: https://github.com/nodef/extra-bigint/wiki/is
|
143 | [compare]: https://github.com/nodef/extra-bigint/wiki/compare
|
144 | [abs]: https://github.com/nodef/extra-bigint/wiki/abs
|
145 | [sign]: https://github.com/nodef/extra-bigint/wiki/sign
|
146 | [floorDiv]: https://github.com/nodef/extra-bigint/wiki/floorDiv
|
147 | [ceilDiv]: https://github.com/nodef/extra-bigint/wiki/ceilDiv
|
148 | [roundDiv]: https://github.com/nodef/extra-bigint/wiki/roundDiv
|
149 | [rem]: https://github.com/nodef/extra-bigint/wiki/rem
|
150 | [mod]: https://github.com/nodef/extra-bigint/wiki/mod
|
151 | [modp]: https://github.com/nodef/extra-bigint/wiki/modp
|
152 | [constrain]: https://github.com/nodef/extra-bigint/wiki/constrain
|
153 | [remap]: https://github.com/nodef/extra-bigint/wiki/remap
|
154 | [lerp]: https://github.com/nodef/extra-bigint/wiki/lerp
|
155 | [isPow2]: https://github.com/nodef/extra-bigint/wiki/isPow2
|
156 | [isPow10]: https://github.com/nodef/extra-bigint/wiki/isPow10
|
157 | [prevPow2]: https://github.com/nodef/extra-bigint/wiki/prevPow2
|
158 | [prevPow10]: https://github.com/nodef/extra-bigint/wiki/prevPow10
|
159 | [nextPow2]: https://github.com/nodef/extra-bigint/wiki/nextPow2
|
160 | [nextPow10]: https://github.com/nodef/extra-bigint/wiki/nextPow10
|
161 | [log2]: https://github.com/nodef/extra-bigint/wiki/log2
|
162 | [log10]: https://github.com/nodef/extra-bigint/wiki/log10
|
163 | [sqrt]: https://github.com/nodef/extra-bigint/wiki/sqrt
|
164 | [cbrt]: https://github.com/nodef/extra-bigint/wiki/cbrt
|
165 | [root]: https://github.com/nodef/extra-bigint/wiki/root
|
166 | [properDivisors]: https://github.com/nodef/extra-bigint/wiki/properDivisors
|
167 | [aliquotSum]: https://github.com/nodef/extra-bigint/wiki/aliquotSum
|
168 | [minPrimeFactor]: https://github.com/nodef/extra-bigint/wiki/minPrimeFactor
|
169 | [maxPrimeFactor]: https://github.com/nodef/extra-bigint/wiki/maxPrimeFactor
|
170 | [primeFactors]: https://github.com/nodef/extra-bigint/wiki/primeFactors
|
171 | [primeExponentials]: https://github.com/nodef/extra-bigint/wiki/primeExponentials
|
172 | [isPrime]: https://github.com/nodef/extra-bigint/wiki/isPrime
|
173 | [gcd]: https://github.com/nodef/extra-bigint/wiki/gcd
|
174 | [lcm]: https://github.com/nodef/extra-bigint/wiki/lcm
|
175 | [factorial]: https://github.com/nodef/extra-bigint/wiki/factorial
|
176 | [binomial]: https://github.com/nodef/extra-bigint/wiki/binomial
|
177 | [multinomial]: https://github.com/nodef/extra-bigint/wiki/multinomial
|
178 | [hypot]: https://github.com/nodef/extra-bigint/wiki/hypot
|
179 | [sum]: https://github.com/nodef/extra-bigint/wiki/sum
|
180 | [product]: https://github.com/nodef/extra-bigint/wiki/product
|
181 | [median]: https://github.com/nodef/extra-bigint/wiki/median
|
182 | [modes]: https://github.com/nodef/extra-bigint/wiki/modes
|
183 | [min]: https://github.com/nodef/extra-bigint/wiki/min
|
184 | [max]: https://github.com/nodef/extra-bigint/wiki/max
|
185 | [range]: https://github.com/nodef/extra-bigint/wiki/range
|
186 | [variance]: https://github.com/nodef/extra-bigint/wiki/variance
|
187 | [arithmeticMean]: https://github.com/nodef/extra-bigint/wiki/arithmeticMean
|
188 | [geometricMean]: https://github.com/nodef/extra-bigint/wiki/geometricMean
|
189 | [harmonicMean]: https://github.com/nodef/extra-bigint/wiki/harmonicMean
|
190 | [quadriaticMean]: https://github.com/nodef/extra-bigint/wiki/quadriaticMean
|
191 | [cubicMean]: https://github.com/nodef/extra-bigint/wiki/cubicMean
|