1 | A group of functions for working with Maps.<br>
|
2 | 📦 [Node.js](https://www.npmjs.com/package/extra-map),
|
3 | 🌐 [Web](https://www.npmjs.com/package/extra-map.web),
|
4 | 📜 [Files](https://unpkg.com/extra-map/),
|
5 | 📰 [Docs](https://nodef.github.io/extra-map/),
|
6 | 📘 [Wiki](https://github.com/nodef/extra-map/wiki/).
|
7 |
|
8 | A [Map] is a collection of key-value pairs, with unique keys. This package
|
9 | includes common set functions related to querying **about** map, **generating**
|
10 | them, **comparing** one with another, finding their **size**, **adding** and
|
11 | **removing** entries, obtaining its **properties**, getting a **part** of it,
|
12 | getting a **subset** entries in it, **finding** an entry in it, performing
|
13 | **functional** operations, **manipulating** it in various ways, **combining**
|
14 | together maps or its entries, of performing **set operations** upon it.
|
15 |
|
16 | All functions except `from*()` take set as 1st parameter. Some names
|
17 | are borrowed from Haskell, Python, Java, Processing. Methods like
|
18 | `swap()` are pure and do not modify the map itself, while methods like
|
19 | `swap$()` *do modify (update)* the map itself.
|
20 |
|
21 | This package is available in *Node.js* and *Web* formats. The web format
|
22 | is exposed as `extra_set` standalone variable and can be loaded from
|
23 | [jsDelivr CDN].
|
24 |
|
25 | > Stability: [Experimental](https://www.youtube.com/watch?v=L1j93RnIxEo).
|
26 |
|
27 | [Map]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map
|
28 | [jsDelivr CDN]: https://cdn.jsdelivr.net/npm/extra-map.web/index.js
|
29 |
|
30 | <br>
|
31 |
|
32 | ```javascript
|
33 | const map = require('extra-map');
|
34 | // import * as map from "extra-map";
|
35 | // import * as map from "https://unpkg.com/extra-map/index.mjs"; (deno)
|
36 |
|
37 | var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", 4]]);
|
38 | map.swap(x, "a", "b");
|
39 | // → Map(4) { "a" => 2, "b" => 1, "c" => 3, "d" => 4 }
|
40 |
|
41 | var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", 4]]);
|
42 | var y = new Map([["b", 20], ["c", 30], ["e", 50]]);
|
43 | map.intersection(x, y);
|
44 | // → Map(2) { "b" => 2, "c" => 3 }
|
45 |
|
46 | var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", -2]]);
|
47 | map.searchAll(x, v => Math.abs(v) === 2);
|
48 | // → [ "b", "d" ] ^ ^
|
49 |
|
50 | var x = new Map([["a", 1], ["b", 2], ["c", 3]]);
|
51 | [...map.subsets(x)];
|
52 | // → [
|
53 | // → Map(0) {},
|
54 | // → Map(1) { "a" => 1 },
|
55 | // → Map(1) { "b" => 2 },
|
56 | // → Map(2) { "a" => 1, "b" => 2 },
|
57 | // → Map(1) { "c" => 3 },
|
58 | // → Map(2) { "a" => 1, "c" => 3 },
|
59 | // → Map(2) { "b" => 2, "c" => 3 },
|
60 | // → Map(3) { "a" => 1, "b" => 2, "c" => 3 }
|
61 | // → ]
|
62 | ```
|
63 |
|
64 | <br>
|
65 | <br>
|
66 |
|
67 |
|
68 | ## Index
|
69 |
|
70 | | Property | Description |
|
71 | | ---- | ---- |
|
72 | | [is] | Check if value is a map. |
|
73 | | [keys] | List all keys. |
|
74 | | [values] | List all values. |
|
75 | | [entries] | List all key-value pairs. |
|
76 | | | |
|
77 | | [from] | Convert entries to map. |
|
78 | | [from$] | Convert entries to map. |
|
79 | | [fromLists] | Convert lists to map. |
|
80 | | [fromKeys] | Create a map from keys. |
|
81 | | [fromValues] | Create a map from values. |
|
82 | | | |
|
83 | | [compare] | Compare two maps. |
|
84 | | [isEqual] | Check if two maps are equal. |
|
85 | | | |
|
86 | | [size] | Find the size of a map. |
|
87 | | [isEmpty] | Check if a map is empty. |
|
88 | | | |
|
89 | | [get] | Get value at key. |
|
90 | | [getAll] | Get values at keys. |
|
91 | | [getPath] | Get value at path in a nested map. |
|
92 | | [hasPath] | Check if nested map has a path. |
|
93 | | [set] | Set value at key. |
|
94 | | [set$] | Set value at key. |
|
95 | | [setPath$] | Set value at path in a nested map. |
|
96 | | [swap] | Exchange two values. |
|
97 | | [swap$] | Exchange two values. |
|
98 | | [remove] | Remove value at key. |
|
99 | | [remove$] | Remove value at key. |
|
100 | | [removePath$] | Remove value at path in a nested map. |
|
101 | | | |
|
102 | | [count] | Count values which satisfy a test. |
|
103 | | [countAs] | Count occurrences of values. |
|
104 | | [min] | Find smallest value. |
|
105 | | [minEntry] | Find smallest entry. |
|
106 | | [max] | Find largest value. |
|
107 | | [maxEntry] | Find largest entry. |
|
108 | | [range] | Find smallest and largest values. |
|
109 | | [rangeEntries] | Find smallest and largest entries. |
|
110 | | | |
|
111 | | [head] | Get first entry from map (default order). |
|
112 | | [tail] | Get a map without its first entry (default order). |
|
113 | | [take] | Keep first n entries only (default order). |
|
114 | | [take$] | Keep first n entries only (default order). |
|
115 | | [drop] | Remove first n entries (default order). |
|
116 | | [drop$] | Remove first n entries (default order). |
|
117 | | | |
|
118 | | [subsets] | List all possible subsets. |
|
119 | | [randomKey] | Pick an arbitrary key. |
|
120 | | [randomEntry] | Pick an arbitrary entry. |
|
121 | | [randomSubset] | Pick an arbitrary subset. |
|
122 | | | |
|
123 | | [has] | Check if map has a key. |
|
124 | | [hasValue] | Check if map has a value. |
|
125 | | [hasEntry] | Check if map has an entry. |
|
126 | | [hasSubset] | Check if map has a subset. |
|
127 | | [find] | Find first value passing a test (default order). |
|
128 | | [findAll] | Find values passing a test. |
|
129 | | [search] | Find key of an entry passing a test. |
|
130 | | [searchAll] | Find keys of entries passing a test. |
|
131 | | [searchValue] | Find a key with given value. |
|
132 | | [searchValueAll] | Find keys with given value. |
|
133 | | | |
|
134 | | [forEach] | Call a function for each value. |
|
135 | | [some] | Check if any value satisfies a test. |
|
136 | | [every] | Check if all values satisfy a test. |
|
137 | | [map] | Transform values of a map. |
|
138 | | [map$] | Transform values of a map. |
|
139 | | [reduce] | Reduce values of set to a single value. |
|
140 | | [filter] | Keep entries which pass a test. |
|
141 | | [filter$] | Keep entries which pass a test. |
|
142 | | [filterAt] | Keep values at given keys. |
|
143 | | [filterAt$] | Keep values at given keys. |
|
144 | | [reject] | Discard entries which pass a test. |
|
145 | | [reject$] | Discard entries which pass a test. |
|
146 | | [rejectAt] | Discard values at given keys. |
|
147 | | [rejectAt$] | Discard values at given keys. |
|
148 | | [flat] | Flatten nested map to given depth. |
|
149 | | [flatMap] | Flatten nested map, based on map function. |
|
150 | | [zip] | Combine matching entries from maps. |
|
151 | | | |
|
152 | | [partition] | Segregate entries by test result. |
|
153 | | [partitionAs] | Segregate entries by similarity. |
|
154 | | [chunk] | Break map into chunks of given size. |
|
155 | | | |
|
156 | | [concat] | Append entries from maps, preferring last. |
|
157 | | [concat$] | Append entries from maps, preferring last. |
|
158 | | [join] | Join entries together into a string. |
|
159 | | | |
|
160 | | [isDisjoint] | Check if maps have no common keys. |
|
161 | | [unionKeys] | Obtain keys present in any map. |
|
162 | | [union] | Obtain entries present in any map. |
|
163 | | [union$] | Obtain entries present in any map. |
|
164 | | [intersectionKeys] | Obtain keys present in all maps. |
|
165 | | [intersection] | Obtain entries present in both maps. |
|
166 | | [intersection$] | Obtain entries present in both maps. |
|
167 | | [difference] | Obtain entries not present in another map. |
|
168 | | [difference$] | Obtain entries not present in another map. |
|
169 | | [symmetricDifference] | Obtain entries not present in both maps. |
|
170 | | [symmetricDifference$] | Obtain entries not present in both maps. |
|
171 | | [cartesianProduct] | List cartesian product of maps. |
|
172 |
|
173 | <br>
|
174 | <br>
|
175 |
|
176 |
|
177 | [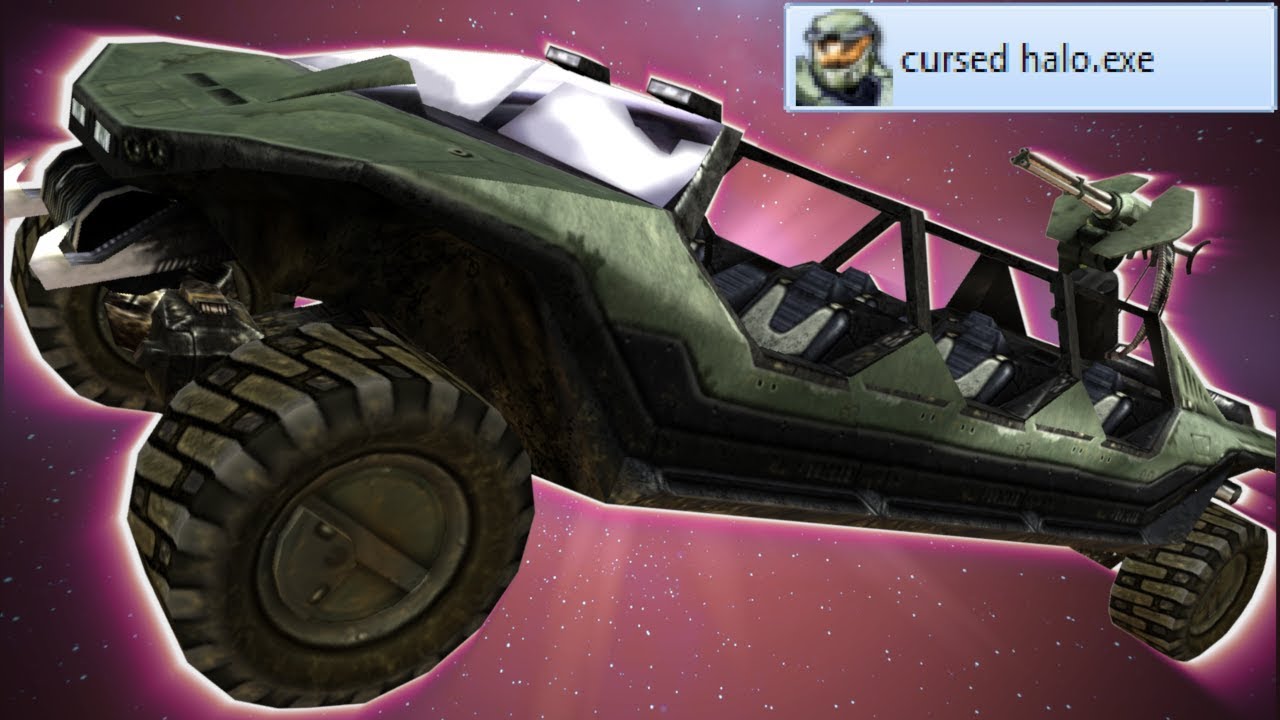](https://www.youtube.com/watch?v=dMxIjGjMJz0)
|
178 | [](https://nodef.github.io)
|
179 | [](https://zenodo.org/badge/latestdoi/133659342)
|
180 | [](https://coveralls.io/github/nodef/extra-map?branch=master)
|
181 | [](https://codeclimate.com/github/nodef/extra-map/test_coverage)
|
182 | [](https://codeclimate.com/github/nodef/extra-map/maintainability)
|
183 |
|
184 |
|
185 | [is]: https://github.com/nodef/extra-map/wiki/is
|
186 | [keys]: https://github.com/nodef/extra-map/wiki/keys
|
187 | [values]: https://github.com/nodef/extra-map/wiki/values
|
188 | [entries]: https://github.com/nodef/extra-map/wiki/entries
|
189 | [from]: https://github.com/nodef/extra-map/wiki/from
|
190 | [from$]: https://github.com/nodef/extra-map/wiki/from$
|
191 | [fromLists]: https://github.com/nodef/extra-map/wiki/fromLists
|
192 | [fromKeys]: https://github.com/nodef/extra-map/wiki/fromKeys
|
193 | [fromValues]: https://github.com/nodef/extra-map/wiki/fromValues
|
194 | [compare]: https://github.com/nodef/extra-map/wiki/compare
|
195 | [isEqual]: https://github.com/nodef/extra-map/wiki/isEqual
|
196 | [size]: https://github.com/nodef/extra-map/wiki/size
|
197 | [isEmpty]: https://github.com/nodef/extra-map/wiki/isEmpty
|
198 | [get]: https://github.com/nodef/extra-map/wiki/get
|
199 | [getAll]: https://github.com/nodef/extra-map/wiki/getAll
|
200 | [getPath]: https://github.com/nodef/extra-map/wiki/getPath
|
201 | [hasPath]: https://github.com/nodef/extra-map/wiki/hasPath
|
202 | [set]: https://github.com/nodef/extra-map/wiki/set
|
203 | [set$]: https://github.com/nodef/extra-map/wiki/set$
|
204 | [setPath$]: https://github.com/nodef/extra-map/wiki/setPath$
|
205 | [swap]: https://github.com/nodef/extra-map/wiki/swap
|
206 | [swap$]: https://github.com/nodef/extra-map/wiki/swap$
|
207 | [remove]: https://github.com/nodef/extra-map/wiki/remove
|
208 | [remove$]: https://github.com/nodef/extra-map/wiki/remove$
|
209 | [removePath$]: https://github.com/nodef/extra-map/wiki/removePath$
|
210 | [count]: https://github.com/nodef/extra-map/wiki/count
|
211 | [countAs]: https://github.com/nodef/extra-map/wiki/countAs
|
212 | [min]: https://github.com/nodef/extra-map/wiki/min
|
213 | [minEntry]: https://github.com/nodef/extra-map/wiki/minEntry
|
214 | [max]: https://github.com/nodef/extra-map/wiki/max
|
215 | [maxEntry]: https://github.com/nodef/extra-map/wiki/maxEntry
|
216 | [range]: https://github.com/nodef/extra-map/wiki/range
|
217 | [rangeEntries]: https://github.com/nodef/extra-map/wiki/rangeEntries
|
218 | [head]: https://github.com/nodef/extra-map/wiki/head
|
219 | [tail]: https://github.com/nodef/extra-map/wiki/tail
|
220 | [take]: https://github.com/nodef/extra-map/wiki/take
|
221 | [take$]: https://github.com/nodef/extra-map/wiki/take$
|
222 | [drop]: https://github.com/nodef/extra-map/wiki/drop
|
223 | [drop$]: https://github.com/nodef/extra-map/wiki/drop$
|
224 | [subsets]: https://github.com/nodef/extra-map/wiki/subsets
|
225 | [randomKey]: https://github.com/nodef/extra-map/wiki/randomKey
|
226 | [randomEntry]: https://github.com/nodef/extra-map/wiki/randomEntry
|
227 | [randomSubset]: https://github.com/nodef/extra-map/wiki/randomSubset
|
228 | [has]: https://github.com/nodef/extra-map/wiki/has
|
229 | [hasValue]: https://github.com/nodef/extra-map/wiki/hasValue
|
230 | [hasEntry]: https://github.com/nodef/extra-map/wiki/hasEntry
|
231 | [hasSubset]: https://github.com/nodef/extra-map/wiki/hasSubset
|
232 | [find]: https://github.com/nodef/extra-map/wiki/find
|
233 | [findAll]: https://github.com/nodef/extra-map/wiki/findAll
|
234 | [search]: https://github.com/nodef/extra-map/wiki/search
|
235 | [searchAll]: https://github.com/nodef/extra-map/wiki/searchAll
|
236 | [searchValue]: https://github.com/nodef/extra-map/wiki/searchValue
|
237 | [searchValueAll]: https://github.com/nodef/extra-map/wiki/searchValueAll
|
238 | [forEach]: https://github.com/nodef/extra-map/wiki/forEach
|
239 | [some]: https://github.com/nodef/extra-map/wiki/some
|
240 | [every]: https://github.com/nodef/extra-map/wiki/every
|
241 | [map]: https://github.com/nodef/extra-map/wiki/map
|
242 | [map$]: https://github.com/nodef/extra-map/wiki/map$
|
243 | [reduce]: https://github.com/nodef/extra-map/wiki/reduce
|
244 | [filter]: https://github.com/nodef/extra-map/wiki/filter
|
245 | [filter$]: https://github.com/nodef/extra-map/wiki/filter$
|
246 | [filterAt]: https://github.com/nodef/extra-map/wiki/filterAt
|
247 | [filterAt$]: https://github.com/nodef/extra-map/wiki/filterAt$
|
248 | [reject]: https://github.com/nodef/extra-map/wiki/reject
|
249 | [reject$]: https://github.com/nodef/extra-map/wiki/reject$
|
250 | [rejectAt]: https://github.com/nodef/extra-map/wiki/rejectAt
|
251 | [rejectAt$]: https://github.com/nodef/extra-map/wiki/rejectAt$
|
252 | [flat]: https://github.com/nodef/extra-map/wiki/flat
|
253 | [flatMap]: https://github.com/nodef/extra-map/wiki/flatMap
|
254 | [zip]: https://github.com/nodef/extra-map/wiki/zip
|
255 | [partition]: https://github.com/nodef/extra-map/wiki/partition
|
256 | [partitionAs]: https://github.com/nodef/extra-map/wiki/partitionAs
|
257 | [chunk]: https://github.com/nodef/extra-map/wiki/chunk
|
258 | [concat]: https://github.com/nodef/extra-map/wiki/concat
|
259 | [concat$]: https://github.com/nodef/extra-map/wiki/concat$
|
260 | [join]: https://github.com/nodef/extra-map/wiki/join
|
261 | [isDisjoint]: https://github.com/nodef/extra-map/wiki/isDisjoint
|
262 | [unionKeys]: https://github.com/nodef/extra-map/wiki/unionKeys
|
263 | [union]: https://github.com/nodef/extra-map/wiki/union
|
264 | [union$]: https://github.com/nodef/extra-map/wiki/union$
|
265 | [intersectionKeys]: https://github.com/nodef/extra-map/wiki/intersectionKeys
|
266 | [intersection]: https://github.com/nodef/extra-map/wiki/intersection
|
267 | [intersection$]: https://github.com/nodef/extra-map/wiki/intersection$
|
268 | [difference]: https://github.com/nodef/extra-map/wiki/difference
|
269 | [difference$]: https://github.com/nodef/extra-map/wiki/difference$
|
270 | [symmetricDifference]: https://github.com/nodef/extra-map/wiki/symmetricDifference
|
271 | [symmetricDifference$]: https://github.com/nodef/extra-map/wiki/symmetricDifference$
|
272 | [cartesianProduct]: https://github.com/nodef/extra-map/wiki/cartesianProduct
|