1 | [//]: # "This README.md file is auto-generated, all changes to this file will be lost."
|
2 | [//]: # "To regenerate it, use `python -m synthtool`."
|
3 | <img src="https://avatars2.githubusercontent.com/u/2810941?v=3&s=96" alt="Google Cloud Platform logo" title="Google Cloud Platform" align="right" height="96" width="96"/>
|
4 |
|
5 | # [Google Auth Library: Node.js Client](https://github.com/googleapis/google-auth-library-nodejs)
|
6 |
|
7 | [](https://cloud.google.com/terms/launch-stages)
|
8 | [](https://www.npmjs.org/package/google-auth-library)
|
9 | [](https://codecov.io/gh/googleapis/google-auth-library-nodejs)
|
10 |
|
11 |
|
12 |
|
13 |
|
14 | This is Google's officially supported [node.js](http://nodejs.org/) client library for using OAuth 2.0 authorization and authentication with Google APIs.
|
15 |
|
16 |
|
17 | A comprehensive list of changes in each version may be found in
|
18 | [the CHANGELOG](https://github.com/googleapis/google-auth-library-nodejs/blob/main/CHANGELOG.md).
|
19 |
|
20 | * [Google Auth Library Node.js Client API Reference][client-docs]
|
21 | * [Google Auth Library Documentation][product-docs]
|
22 | * [github.com/googleapis/google-auth-library-nodejs](https://github.com/googleapis/google-auth-library-nodejs)
|
23 |
|
24 | Read more about the client libraries for Cloud APIs, including the older
|
25 | Google APIs Client Libraries, in [Client Libraries Explained][explained].
|
26 |
|
27 | [explained]: https://cloud.google.com/apis/docs/client-libraries-explained
|
28 |
|
29 | **Table of contents:**
|
30 |
|
31 |
|
32 | * [Quickstart](#quickstart)
|
33 |
|
34 | * [Installing the client library](#installing-the-client-library)
|
35 |
|
36 | * [Samples](#samples)
|
37 | * [Versioning](#versioning)
|
38 | * [Contributing](#contributing)
|
39 | * [License](#license)
|
40 |
|
41 | ## Quickstart
|
42 |
|
43 | ### Installing the client library
|
44 |
|
45 | ```bash
|
46 | npm install google-auth-library
|
47 | ```
|
48 |
|
49 | ## Ways to authenticate
|
50 | This library provides a variety of ways to authenticate to your Google services.
|
51 | - [Application Default Credentials](#choosing-the-correct-credential-type-automatically) - Use Application Default Credentials when you use a single identity for all users in your application. Especially useful for applications running on Google Cloud. Application Default Credentials also support workload identity federation to access Google Cloud resources from non-Google Cloud platforms.
|
52 | - [OAuth 2](#oauth2) - Use OAuth2 when you need to perform actions on behalf of the end user.
|
53 | - [JSON Web Tokens](#json-web-tokens) - Use JWT when you are using a single identity for all users. Especially useful for server->server or server->API communication.
|
54 | - [Google Compute](#compute) - Directly use a service account on Google Cloud Platform. Useful for server->server or server->API communication.
|
55 | - [Workload Identity Federation](#workload-identity-federation) - Use workload identity federation to access Google Cloud resources from Amazon Web Services (AWS), Microsoft Azure or any identity provider that supports OpenID Connect (OIDC).
|
56 | - [Impersonated Credentials Client](#impersonated-credentials-client) - access protected resources on behalf of another service account.
|
57 |
|
58 | ## Application Default Credentials
|
59 | This library provides an implementation of [Application Default Credentials](https://cloud.google.com/docs/authentication/getting-started)for Node.js. The [Application Default Credentials](https://cloud.google.com/docs/authentication/getting-started) provide a simple way to get authorization credentials for use in calling Google APIs.
|
60 |
|
61 | They are best suited for cases when the call needs to have the same identity and authorization level for the application independent of the user. This is the recommended approach to authorize calls to Cloud APIs, particularly when you're building an application that uses Google Cloud Platform.
|
62 |
|
63 | Application Default Credentials also support workload identity federation to access Google Cloud resources from non-Google Cloud platforms including Amazon Web Services (AWS), Microsoft Azure or any identity provider that supports OpenID Connect (OIDC). Workload identity federation is recommended for non-Google Cloud environments as it avoids the need to download, manage and store service account private keys locally, see: [Workload Identity Federation](#workload-identity-federation).
|
64 |
|
65 | #### Download your Service Account Credentials JSON file
|
66 |
|
67 | To use Application Default Credentials, You first need to download a set of JSON credentials for your project. Go to **APIs & Auth** > **Credentials** in the [Google Developers Console](https://console.cloud.google.com/) and select **Service account** from the **Add credentials** dropdown.
|
68 |
|
69 | > This file is your *only copy* of these credentials. It should never be
|
70 | > committed with your source code, and should be stored securely.
|
71 |
|
72 | Once downloaded, store the path to this file in the `GOOGLE_APPLICATION_CREDENTIALS` environment variable.
|
73 |
|
74 | #### Enable the API you want to use
|
75 |
|
76 | Before making your API call, you must be sure the API you're calling has been enabled. Go to **APIs & Auth** > **APIs** in the [Google Developers Console](https://console.cloud.google.com/) and enable the APIs you'd like to call. For the example below, you must enable the `DNS API`.
|
77 |
|
78 |
|
79 | #### Choosing the correct credential type automatically
|
80 |
|
81 | Rather than manually creating an OAuth2 client, JWT client, or Compute client, the auth library can create the correct credential type for you, depending upon the environment your code is running under.
|
82 |
|
83 | For example, a JWT auth client will be created when your code is running on your local developer machine, and a Compute client will be created when the same code is running on Google Cloud Platform. If you need a specific set of scopes, you can pass those in the form of a string or an array to the `GoogleAuth` constructor.
|
84 |
|
85 | The code below shows how to retrieve a default credential type, depending upon the runtime environment.
|
86 |
|
87 | ```js
|
88 | const {GoogleAuth} = require('google-auth-library');
|
89 |
|
90 | /**
|
91 | * Instead of specifying the type of client you'd like to use (JWT, OAuth2, etc)
|
92 | * this library will automatically choose the right client based on the environment.
|
93 | */
|
94 | async function main() {
|
95 | const auth = new GoogleAuth({
|
96 | scopes: 'https://www.googleapis.com/auth/cloud-platform'
|
97 | });
|
98 | const client = await auth.getClient();
|
99 | const projectId = await auth.getProjectId();
|
100 | const url = `https://dns.googleapis.com/dns/v1/projects/${projectId}`;
|
101 | const res = await client.request({ url });
|
102 | console.log(res.data);
|
103 | }
|
104 |
|
105 | main().catch(console.error);
|
106 | ```
|
107 |
|
108 | ## OAuth2
|
109 |
|
110 | This library comes with an [OAuth2](https://developers.google.com/identity/protocols/OAuth2) client that allows you to retrieve an access token and refreshes the token and retry the request seamlessly if you also provide an `expiry_date` and the token is expired. The basics of Google's OAuth2 implementation is explained on [Google Authorization and Authentication documentation](https://developers.google.com/accounts/docs/OAuth2Login).
|
111 |
|
112 | In the following examples, you may need a `CLIENT_ID`, `CLIENT_SECRET` and `REDIRECT_URL`. You can find these pieces of information by going to the [Developer Console](https://console.cloud.google.com/), clicking your project > APIs & auth > credentials.
|
113 |
|
114 | For more information about OAuth2 and how it works, [see here](https://developers.google.com/identity/protocols/OAuth2).
|
115 |
|
116 | #### A complete OAuth2 example
|
117 |
|
118 | Let's take a look at a complete example.
|
119 |
|
120 | ``` js
|
121 | const {OAuth2Client} = require('google-auth-library');
|
122 | const http = require('http');
|
123 | const url = require('url');
|
124 | const open = require('open');
|
125 | const destroyer = require('server-destroy');
|
126 |
|
127 | // Download your OAuth2 configuration from the Google
|
128 | const keys = require('./oauth2.keys.json');
|
129 |
|
130 | /**
|
131 | * Start by acquiring a pre-authenticated oAuth2 client.
|
132 | */
|
133 | async function main() {
|
134 | const oAuth2Client = await getAuthenticatedClient();
|
135 | // Make a simple request to the People API using our pre-authenticated client. The `request()` method
|
136 | // takes an GaxiosOptions object. Visit https://github.com/JustinBeckwith/gaxios.
|
137 | const url = 'https://people.googleapis.com/v1/people/me?personFields=names';
|
138 | const res = await oAuth2Client.request({url});
|
139 | console.log(res.data);
|
140 |
|
141 | // After acquiring an access_token, you may want to check on the audience, expiration,
|
142 | // or original scopes requested. You can do that with the `getTokenInfo` method.
|
143 | const tokenInfo = await oAuth2Client.getTokenInfo(
|
144 | oAuth2Client.credentials.access_token
|
145 | );
|
146 | console.log(tokenInfo);
|
147 | }
|
148 |
|
149 | /**
|
150 | * Create a new OAuth2Client, and go through the OAuth2 content
|
151 | * workflow. Return the full client to the callback.
|
152 | */
|
153 | function getAuthenticatedClient() {
|
154 | return new Promise((resolve, reject) => {
|
155 | // create an oAuth client to authorize the API call. Secrets are kept in a `keys.json` file,
|
156 | // which should be downloaded from the Google Developers Console.
|
157 | const oAuth2Client = new OAuth2Client(
|
158 | keys.web.client_id,
|
159 | keys.web.client_secret,
|
160 | keys.web.redirect_uris[0]
|
161 | );
|
162 |
|
163 | // Generate the url that will be used for the consent dialog.
|
164 | const authorizeUrl = oAuth2Client.generateAuthUrl({
|
165 | access_type: 'offline',
|
166 | scope: 'https://www.googleapis.com/auth/userinfo.profile',
|
167 | });
|
168 |
|
169 | // Open an http server to accept the oauth callback. In this simple example, the
|
170 | // only request to our webserver is to /oauth2callback?code=<code>
|
171 | const server = http
|
172 | .createServer(async (req, res) => {
|
173 | try {
|
174 | if (req.url.indexOf('/oauth2callback') > -1) {
|
175 | // acquire the code from the querystring, and close the web server.
|
176 | const qs = new url.URL(req.url, 'http://localhost:3000')
|
177 | .searchParams;
|
178 | const code = qs.get('code');
|
179 | console.log(`Code is ${code}`);
|
180 | res.end('Authentication successful! Please return to the console.');
|
181 | server.destroy();
|
182 |
|
183 | // Now that we have the code, use that to acquire tokens.
|
184 | const r = await oAuth2Client.getToken(code);
|
185 | // Make sure to set the credentials on the OAuth2 client.
|
186 | oAuth2Client.setCredentials(r.tokens);
|
187 | console.info('Tokens acquired.');
|
188 | resolve(oAuth2Client);
|
189 | }
|
190 | } catch (e) {
|
191 | reject(e);
|
192 | }
|
193 | })
|
194 | .listen(3000, () => {
|
195 | // open the browser to the authorize url to start the workflow
|
196 | open(authorizeUrl, {wait: false}).then(cp => cp.unref());
|
197 | });
|
198 | destroyer(server);
|
199 | });
|
200 | }
|
201 |
|
202 | main().catch(console.error);
|
203 | ```
|
204 |
|
205 | #### Handling token events
|
206 |
|
207 | This library will automatically obtain an `access_token`, and automatically refresh the `access_token` if a `refresh_token` is present. The `refresh_token` is only returned on the [first authorization](https://github.com/googleapis/google-api-nodejs-client/issues/750#issuecomment-304521450), so if you want to make sure you store it safely. An easy way to make sure you always store the most recent tokens is to use the `tokens` event:
|
208 |
|
209 | ```js
|
210 | const client = await auth.getClient();
|
211 |
|
212 | client.on('tokens', (tokens) => {
|
213 | if (tokens.refresh_token) {
|
214 | // store the refresh_token in my database!
|
215 | console.log(tokens.refresh_token);
|
216 | }
|
217 | console.log(tokens.access_token);
|
218 | });
|
219 |
|
220 | const url = `https://dns.googleapis.com/dns/v1/projects/${projectId}`;
|
221 | const res = await client.request({ url });
|
222 | // The `tokens` event would now be raised if this was the first request
|
223 | ```
|
224 |
|
225 | #### Retrieve access token
|
226 | With the code returned, you can ask for an access token as shown below:
|
227 |
|
228 | ``` js
|
229 | const tokens = await oauth2Client.getToken(code);
|
230 | // Now tokens contains an access_token and an optional refresh_token. Save them.
|
231 | oauth2Client.setCredentials(tokens);
|
232 | ```
|
233 |
|
234 | #### Obtaining a new Refresh Token
|
235 | If you need to obtain a new `refresh_token`, ensure the call to `generateAuthUrl` sets the `access_type` to `offline`. The refresh token will only be returned for the first authorization by the user. To force consent, set the `prompt` property to `consent`:
|
236 |
|
237 | ```js
|
238 | // Generate the url that will be used for the consent dialog.
|
239 | const authorizeUrl = oAuth2Client.generateAuthUrl({
|
240 | // To get a refresh token, you MUST set access_type to `offline`.
|
241 | access_type: 'offline',
|
242 | // set the appropriate scopes
|
243 | scope: 'https://www.googleapis.com/auth/userinfo.profile',
|
244 | // A refresh token is only returned the first time the user
|
245 | // consents to providing access. For illustration purposes,
|
246 | // setting the prompt to 'consent' will force this consent
|
247 | // every time, forcing a refresh_token to be returned.
|
248 | prompt: 'consent'
|
249 | });
|
250 | ```
|
251 |
|
252 | #### Checking `access_token` information
|
253 | After obtaining and storing an `access_token`, at a later time you may want to go check the expiration date,
|
254 | original scopes, or audience for the token. To get the token info, you can use the `getTokenInfo` method:
|
255 |
|
256 | ```js
|
257 | // after acquiring an oAuth2Client...
|
258 | const tokenInfo = await oAuth2Client.getTokenInfo('my-access-token');
|
259 |
|
260 | // take a look at the scopes originally provisioned for the access token
|
261 | console.log(tokenInfo.scopes);
|
262 | ```
|
263 |
|
264 | This method will throw if the token is invalid.
|
265 |
|
266 | #### OAuth2 with Installed Apps (Electron)
|
267 | If you're authenticating with OAuth2 from an installed application (like Electron), you may not want to embed your `client_secret` inside of the application sources. To work around this restriction, you can choose the `iOS` application type when creating your OAuth2 credentials in the [Google Developers console](https://console.cloud.google.com/):
|
268 |
|
269 | 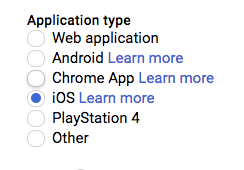
|
270 |
|
271 | If using the `iOS` type, when creating the OAuth2 client you won't need to pass a `client_secret` into the constructor:
|
272 | ```js
|
273 | const oAuth2Client = new OAuth2Client({
|
274 | clientId: <your_client_id>,
|
275 | redirectUri: <your_redirect_uri>
|
276 | });
|
277 | ```
|
278 |
|
279 | ## JSON Web Tokens
|
280 | The Google Developers Console provides a `.json` file that you can use to configure a JWT auth client and authenticate your requests, for example when using a service account.
|
281 |
|
282 | ``` js
|
283 | const {JWT} = require('google-auth-library');
|
284 | const keys = require('./jwt.keys.json');
|
285 |
|
286 | async function main() {
|
287 | const client = new JWT({
|
288 | email: keys.client_email,
|
289 | key: keys.private_key,
|
290 | scopes: ['https://www.googleapis.com/auth/cloud-platform'],
|
291 | });
|
292 | const url = `https://dns.googleapis.com/dns/v1/projects/${keys.project_id}`;
|
293 | const res = await client.request({url});
|
294 | console.log(res.data);
|
295 | }
|
296 |
|
297 | main().catch(console.error);
|
298 | ```
|
299 |
|
300 | The parameters for the JWT auth client including how to use it with a `.pem` file are explained in [samples/jwt.js](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/jwt.js).
|
301 |
|
302 | #### Loading credentials from environment variables
|
303 | Instead of loading credentials from a key file, you can also provide them using an environment variable and the `GoogleAuth.fromJSON()` method. This is particularly convenient for systems that deploy directly from source control (Heroku, App Engine, etc).
|
304 |
|
305 | Start by exporting your credentials:
|
306 |
|
307 | ```
|
308 | $ export CREDS='{
|
309 | "type": "service_account",
|
310 | "project_id": "your-project-id",
|
311 | "private_key_id": "your-private-key-id",
|
312 | "private_key": "your-private-key",
|
313 | "client_email": "your-client-email",
|
314 | "client_id": "your-client-id",
|
315 | "auth_uri": "https://accounts.google.com/o/oauth2/auth",
|
316 | "token_uri": "https://accounts.google.com/o/oauth2/token",
|
317 | "auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
|
318 | "client_x509_cert_url": "your-cert-url"
|
319 | }'
|
320 | ```
|
321 | Now you can create a new client from the credentials:
|
322 |
|
323 | ```js
|
324 | const {auth} = require('google-auth-library');
|
325 |
|
326 | // load the environment variable with our keys
|
327 | const keysEnvVar = process.env['CREDS'];
|
328 | if (!keysEnvVar) {
|
329 | throw new Error('The $CREDS environment variable was not found!');
|
330 | }
|
331 | const keys = JSON.parse(keysEnvVar);
|
332 |
|
333 | async function main() {
|
334 | // load the JWT or UserRefreshClient from the keys
|
335 | const client = auth.fromJSON(keys);
|
336 | client.scopes = ['https://www.googleapis.com/auth/cloud-platform'];
|
337 | const url = `https://dns.googleapis.com/dns/v1/projects/${keys.project_id}`;
|
338 | const res = await client.request({url});
|
339 | console.log(res.data);
|
340 | }
|
341 |
|
342 | main().catch(console.error);
|
343 | ```
|
344 |
|
345 | #### Using a Proxy
|
346 | You can set the `HTTPS_PROXY` or `https_proxy` environment variables to proxy HTTPS requests. When `HTTPS_PROXY` or `https_proxy` are set, they will be used to proxy SSL requests that do not have an explicit proxy configuration option present.
|
347 |
|
348 | ## Compute
|
349 | If your application is running on Google Cloud Platform, you can authenticate using the default service account or by specifying a specific service account.
|
350 |
|
351 | **Note**: In most cases, you will want to use [Application Default Credentials](#choosing-the-correct-credential-type-automatically). Direct use of the `Compute` class is for very specific scenarios.
|
352 |
|
353 | ``` js
|
354 | const {auth, Compute} = require('google-auth-library');
|
355 |
|
356 | async function main() {
|
357 | const client = new Compute({
|
358 | // Specifying the service account email is optional.
|
359 | serviceAccountEmail: 'my-service-account@example.com'
|
360 | });
|
361 | const projectId = await auth.getProjectId();
|
362 | const url = `https://dns.googleapis.com/dns/v1/projects/${projectId}`;
|
363 | const res = await client.request({url});
|
364 | console.log(res.data);
|
365 | }
|
366 |
|
367 | main().catch(console.error);
|
368 | ```
|
369 |
|
370 | ## Workload Identity Federation
|
371 |
|
372 | Using workload identity federation, your application can access Google Cloud resources from Amazon Web Services (AWS), Microsoft Azure or any identity provider that supports OpenID Connect (OIDC).
|
373 |
|
374 | Traditionally, applications running outside Google Cloud have used service account keys to access Google Cloud resources. Using identity federation, you can allow your workload to impersonate a service account.
|
375 | This lets you access Google Cloud resources directly, eliminating the maintenance and security burden associated with service account keys.
|
376 |
|
377 | ### Accessing resources from AWS
|
378 |
|
379 | In order to access Google Cloud resources from Amazon Web Services (AWS), the following requirements are needed:
|
380 | - A workload identity pool needs to be created.
|
381 | - AWS needs to be added as an identity provider in the workload identity pool (The Google [organization policy](https://cloud.google.com/iam/docs/manage-workload-identity-pools-providers#restrict) needs to allow federation from AWS).
|
382 | - Permission to impersonate a service account needs to be granted to the external identity.
|
383 |
|
384 | Follow the detailed [instructions](https://cloud.google.com/iam/docs/access-resources-aws) on how to configure workload identity federation from AWS.
|
385 |
|
386 | After configuring the AWS provider to impersonate a service account, a credential configuration file needs to be generated.
|
387 | Unlike service account credential files, the generated credential configuration file will only contain non-sensitive metadata to instruct the library on how to retrieve external subject tokens and exchange them for service account access tokens.
|
388 | The configuration file can be generated by using the [gcloud CLI](https://cloud.google.com/sdk/).
|
389 |
|
390 | To generate the AWS workload identity configuration, run the following command:
|
391 |
|
392 | ```bash
|
393 | # Generate an AWS configuration file.
|
394 | gcloud iam workload-identity-pools create-cred-config \
|
395 | projects/$PROJECT_NUMBER/locations/global/workloadIdentityPools/$POOL_ID/providers/$AWS_PROVIDER_ID \
|
396 | --service-account $SERVICE_ACCOUNT_EMAIL \
|
397 | --aws \
|
398 | --output-file /path/to/generated/config.json
|
399 | ```
|
400 |
|
401 | Where the following variables need to be substituted:
|
402 | - `$PROJECT_NUMBER`: The Google Cloud project number.
|
403 | - `$POOL_ID`: The workload identity pool ID.
|
404 | - `$AWS_PROVIDER_ID`: The AWS provider ID.
|
405 | - `$SERVICE_ACCOUNT_EMAIL`: The email of the service account to impersonate.
|
406 |
|
407 | This will generate the configuration file in the specified output file.
|
408 |
|
409 | You can now [start using the Auth library](#using-external-identities) to call Google Cloud resources from AWS.
|
410 |
|
411 | ### Access resources from Microsoft Azure
|
412 |
|
413 | In order to access Google Cloud resources from Microsoft Azure, the following requirements are needed:
|
414 | - A workload identity pool needs to be created.
|
415 | - Azure needs to be added as an identity provider in the workload identity pool (The Google [organization policy](https://cloud.google.com/iam/docs/manage-workload-identity-pools-providers#restrict) needs to allow federation from Azure).
|
416 | - The Azure tenant needs to be configured for identity federation.
|
417 | - Permission to impersonate a service account needs to be granted to the external identity.
|
418 |
|
419 | Follow the detailed [instructions](https://cloud.google.com/iam/docs/access-resources-azure) on how to configure workload identity federation from Microsoft Azure.
|
420 |
|
421 | After configuring the Azure provider to impersonate a service account, a credential configuration file needs to be generated.
|
422 | Unlike service account credential files, the generated credential configuration file will only contain non-sensitive metadata to instruct the library on how to retrieve external subject tokens and exchange them for service account access tokens.
|
423 | The configuration file can be generated by using the [gcloud CLI](https://cloud.google.com/sdk/).
|
424 |
|
425 | To generate the Azure workload identity configuration, run the following command:
|
426 |
|
427 | ```bash
|
428 | # Generate an Azure configuration file.
|
429 | gcloud iam workload-identity-pools create-cred-config \
|
430 | projects/$PROJECT_NUMBER/locations/global/workloadIdentityPools/$POOL_ID/providers/$AZURE_PROVIDER_ID \
|
431 | --service-account $SERVICE_ACCOUNT_EMAIL \
|
432 | --azure \
|
433 | --output-file /path/to/generated/config.json
|
434 | ```
|
435 |
|
436 | Where the following variables need to be substituted:
|
437 | - `$PROJECT_NUMBER`: The Google Cloud project number.
|
438 | - `$POOL_ID`: The workload identity pool ID.
|
439 | - `$AZURE_PROVIDER_ID`: The Azure provider ID.
|
440 | - `$SERVICE_ACCOUNT_EMAIL`: The email of the service account to impersonate.
|
441 |
|
442 | This will generate the configuration file in the specified output file.
|
443 |
|
444 | You can now [start using the Auth library](#using-external-identities) to call Google Cloud resources from Azure.
|
445 |
|
446 | ### Accessing resources from an OIDC identity provider
|
447 |
|
448 | In order to access Google Cloud resources from an identity provider that supports [OpenID Connect (OIDC)](https://openid.net/connect/), the following requirements are needed:
|
449 | - A workload identity pool needs to be created.
|
450 | - An OIDC identity provider needs to be added in the workload identity pool (The Google [organization policy](https://cloud.google.com/iam/docs/manage-workload-identity-pools-providers#restrict) needs to allow federation from the identity provider).
|
451 | - Permission to impersonate a service account needs to be granted to the external identity.
|
452 |
|
453 | Follow the detailed [instructions](https://cloud.google.com/iam/docs/access-resources-oidc) on how to configure workload identity federation from an OIDC identity provider.
|
454 |
|
455 | After configuring the OIDC provider to impersonate a service account, a credential configuration file needs to be generated.
|
456 | Unlike service account credential files, the generated credential configuration file will only contain non-sensitive metadata to instruct the library on how to retrieve external subject tokens and exchange them for service account access tokens.
|
457 | The configuration file can be generated by using the [gcloud CLI](https://cloud.google.com/sdk/).
|
458 |
|
459 | For OIDC providers, the Auth library can retrieve OIDC tokens either from a local file location (file-sourced credentials) or from a local server (URL-sourced credentials).
|
460 |
|
461 | **File-sourced credentials**
|
462 | For file-sourced credentials, a background process needs to be continuously refreshing the file location with a new OIDC token prior to expiration.
|
463 | For tokens with one hour lifetimes, the token needs to be updated in the file every hour. The token can be stored directly as plain text or in JSON format.
|
464 |
|
465 | To generate a file-sourced OIDC configuration, run the following command:
|
466 |
|
467 | ```bash
|
468 | # Generate an OIDC configuration file for file-sourced credentials.
|
469 | gcloud iam workload-identity-pools create-cred-config \
|
470 | projects/$PROJECT_NUMBER/locations/global/workloadIdentityPools/$POOL_ID/providers/$OIDC_PROVIDER_ID \
|
471 | --service-account $SERVICE_ACCOUNT_EMAIL \
|
472 | --credential-source-file $PATH_TO_OIDC_ID_TOKEN \
|
473 | # Optional arguments for file types. Default is "text":
|
474 | # --credential-source-type "json" \
|
475 | # Optional argument for the field that contains the OIDC credential.
|
476 | # This is required for json.
|
477 | # --credential-source-field-name "id_token" \
|
478 | --output-file /path/to/generated/config.json
|
479 | ```
|
480 |
|
481 | Where the following variables need to be substituted:
|
482 | - `$PROJECT_NUMBER`: The Google Cloud project number.
|
483 | - `$POOL_ID`: The workload identity pool ID.
|
484 | - `$OIDC_PROVIDER_ID`: The OIDC provider ID.
|
485 | - `$SERVICE_ACCOUNT_EMAIL`: The email of the service account to impersonate.
|
486 | - `$PATH_TO_OIDC_ID_TOKEN`: The file path where the OIDC token will be retrieved from.
|
487 |
|
488 | This will generate the configuration file in the specified output file.
|
489 |
|
490 | **URL-sourced credentials**
|
491 | For URL-sourced credentials, a local server needs to host a GET endpoint to return the OIDC token. The response can be in plain text or JSON.
|
492 | Additional required request headers can also be specified.
|
493 |
|
494 | To generate a URL-sourced OIDC workload identity configuration, run the following command:
|
495 |
|
496 | ```bash
|
497 | # Generate an OIDC configuration file for URL-sourced credentials.
|
498 | gcloud iam workload-identity-pools create-cred-config \
|
499 | projects/$PROJECT_NUMBER/locations/global/workloadIdentityPools/$POOL_ID/providers/$OIDC_PROVIDER_ID \
|
500 | --service-account $SERVICE_ACCOUNT_EMAIL \
|
501 | --credential-source-url $URL_TO_GET_OIDC_TOKEN \
|
502 | --credential-source-headers $HEADER_KEY=$HEADER_VALUE \
|
503 | # Optional arguments for file types. Default is "text":
|
504 | # --credential-source-type "json" \
|
505 | # Optional argument for the field that contains the OIDC credential.
|
506 | # This is required for json.
|
507 | # --credential-source-field-name "id_token" \
|
508 | --output-file /path/to/generated/config.json
|
509 | ```
|
510 |
|
511 | Where the following variables need to be substituted:
|
512 | - `$PROJECT_NUMBER`: The Google Cloud project number.
|
513 | - `$POOL_ID`: The workload identity pool ID.
|
514 | - `$OIDC_PROVIDER_ID`: The OIDC provider ID.
|
515 | - `$SERVICE_ACCOUNT_EMAIL`: The email of the service account to impersonate.
|
516 | - `$URL_TO_GET_OIDC_TOKEN`: The URL of the local server endpoint to call to retrieve the OIDC token.
|
517 | - `$HEADER_KEY` and `$HEADER_VALUE`: The additional header key/value pairs to pass along the GET request to `$URL_TO_GET_OIDC_TOKEN`, e.g. `Metadata-Flavor=Google`.
|
518 |
|
519 | You can now [start using the Auth library](#using-external-identities) to call Google Cloud resources from an OIDC provider.
|
520 |
|
521 | ### Using External Identities
|
522 |
|
523 | External identities (AWS, Azure and OIDC-based providers) can be used with `Application Default Credentials`.
|
524 | In order to use external identities with Application Default Credentials, you need to generate the JSON credentials configuration file for your external identity as described above.
|
525 | Once generated, store the path to this file in the `GOOGLE_APPLICATION_CREDENTIALS` environment variable.
|
526 |
|
527 | ```bash
|
528 | export GOOGLE_APPLICATION_CREDENTIALS=/path/to/config.json
|
529 | ```
|
530 |
|
531 | The library can now automatically choose the right type of client and initialize credentials from the context provided in the configuration file.
|
532 |
|
533 | ```js
|
534 | async function main() {
|
535 | const auth = new GoogleAuth({
|
536 | scopes: 'https://www.googleapis.com/auth/cloud-platform'
|
537 | });
|
538 | const client = await auth.getClient();
|
539 | const projectId = await auth.getProjectId();
|
540 | // List all buckets in a project.
|
541 | const url = `https://storage.googleapis.com/storage/v1/b?project=${projectId}`;
|
542 | const res = await client.request({ url });
|
543 | console.log(res.data);
|
544 | }
|
545 | ```
|
546 |
|
547 | When using external identities with Application Default Credentials in Node.js, the `roles/browser` role needs to be granted to the service account.
|
548 | The `Cloud Resource Manager API` should also be enabled on the project.
|
549 | This is needed since the library will try to auto-discover the project ID from the current environment using the impersonated credential.
|
550 | To avoid this requirement, the project ID can be explicitly specified on initialization.
|
551 |
|
552 | ```js
|
553 | const auth = new GoogleAuth({
|
554 | scopes: 'https://www.googleapis.com/auth/cloud-platform',
|
555 | // Pass the project ID explicitly to avoid the need to grant `roles/browser` to the service account
|
556 | // or enable Cloud Resource Manager API on the project.
|
557 | projectId: 'CLOUD_RESOURCE_PROJECT_ID',
|
558 | });
|
559 | ```
|
560 |
|
561 | You can also explicitly initialize external account clients using the generated configuration file.
|
562 |
|
563 | ```js
|
564 | const {ExternalAccountClient} = require('google-auth-library');
|
565 | const jsonConfig = require('/path/to/config.json');
|
566 |
|
567 | async function main() {
|
568 | const client = ExternalAccountClient.fromJSON(jsonConfig);
|
569 | client.scopes = ['https://www.googleapis.com/auth/cloud-platform'];
|
570 | // List all buckets in a project.
|
571 | const url = `https://storage.googleapis.com/storage/v1/b?project=${projectId}`;
|
572 | const res = await client.request({url});
|
573 | console.log(res.data);
|
574 | }
|
575 | ```
|
576 |
|
577 | ## Working with ID Tokens
|
578 | ### Fetching ID Tokens
|
579 | If your application is running on Cloud Run or Cloud Functions, or using Cloud Identity-Aware
|
580 | Proxy (IAP), you will need to fetch an ID token to access your application. For
|
581 | this, use the method `getIdTokenClient` on the `GoogleAuth` client.
|
582 |
|
583 | For invoking Cloud Run services, your service account will need the
|
584 | [`Cloud Run Invoker`](https://cloud.google.com/run/docs/authenticating/service-to-service)
|
585 | IAM permission.
|
586 |
|
587 | For invoking Cloud Functions, your service account will need the
|
588 | [`Function Invoker`](https://cloud.google.com/functions/docs/securing/authenticating#function-to-function)
|
589 | IAM permission.
|
590 |
|
591 | ``` js
|
592 | // Make a request to a protected Cloud Run service.
|
593 | const {GoogleAuth} = require('google-auth-library');
|
594 |
|
595 | async function main() {
|
596 | const url = 'https://cloud-run-1234-uc.a.run.app';
|
597 | const auth = new GoogleAuth();
|
598 | const client = await auth.getIdTokenClient(url);
|
599 | const res = await client.request({url});
|
600 | console.log(res.data);
|
601 | }
|
602 |
|
603 | main().catch(console.error);
|
604 | ```
|
605 |
|
606 | A complete example can be found in [`samples/idtokens-serverless.js`](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/idtokens-serverless.js).
|
607 |
|
608 | For invoking Cloud Identity-Aware Proxy, you will need to pass the Client ID
|
609 | used when you set up your protected resource as the target audience.
|
610 |
|
611 | ``` js
|
612 | // Make a request to a protected Cloud Identity-Aware Proxy (IAP) resource
|
613 | const {GoogleAuth} = require('google-auth-library');
|
614 |
|
615 | async function main()
|
616 | const targetAudience = 'iap-client-id';
|
617 | const url = 'https://iap-url.com';
|
618 | const auth = new GoogleAuth();
|
619 | const client = await auth.getIdTokenClient(targetAudience);
|
620 | const res = await client.request({url});
|
621 | console.log(res.data);
|
622 | }
|
623 |
|
624 | main().catch(console.error);
|
625 | ```
|
626 |
|
627 | A complete example can be found in [`samples/idtokens-iap.js`](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/idtokens-iap.js).
|
628 |
|
629 | ### Verifying ID Tokens
|
630 |
|
631 | If you've [secured your IAP app with signed headers](https://cloud.google.com/iap/docs/signed-headers-howto),
|
632 | you can use this library to verify the IAP header:
|
633 |
|
634 | ```js
|
635 | const {OAuth2Client} = require('google-auth-library');
|
636 | // Expected audience for App Engine.
|
637 | const expectedAudience = `/projects/your-project-number/apps/your-project-id`;
|
638 | // IAP issuer
|
639 | const issuers = ['https://cloud.google.com/iap'];
|
640 | // Verify the token. OAuth2Client throws an Error if verification fails
|
641 | const oAuth2Client = new OAuth2Client();
|
642 | const response = await oAuth2Client.getIapCerts();
|
643 | const ticket = await oAuth2Client.verifySignedJwtWithCertsAsync(
|
644 | idToken,
|
645 | response.pubkeys,
|
646 | expectedAudience,
|
647 | issuers
|
648 | );
|
649 |
|
650 | // Print out the info contained in the IAP ID token
|
651 | console.log(ticket)
|
652 | ```
|
653 |
|
654 | A complete example can be found in [`samples/verifyIdToken-iap.js`](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/verifyIdToken-iap.js).
|
655 |
|
656 | ## Impersonated Credentials Client
|
657 |
|
658 | Google Cloud Impersonated credentials used for [Creating short-lived service account credentials](https://cloud.google.com/iam/docs/creating-short-lived-service-account-credentials).
|
659 |
|
660 | Provides authentication for applications where local credentials impersonates a remote service account using [IAM Credentials API](https://cloud.google.com/iam/docs/reference/credentials/rest).
|
661 |
|
662 | An Impersonated Credentials Client is instantiated with a `sourceClient`. This
|
663 | client should use credentials that have the "Service Account Token Creator" role (`roles/iam.serviceAccountTokenCreator`),
|
664 | and should authenticate with the `https://www.googleapis.com/auth/cloud-platform`, or `https://www.googleapis.com/auth/iam` scopes.
|
665 |
|
666 | `sourceClient` is used by the Impersonated
|
667 | Credentials Client to impersonate a target service account with a specified
|
668 | set of scopes.
|
669 |
|
670 | ### Sample Usage
|
671 |
|
672 | ```javascript
|
673 | const { GoogleAuth, Impersonated } = require('google-auth-library');
|
674 | const { SecretManagerServiceClient } = require('@google-cloud/secret-manager');
|
675 |
|
676 | async function main() {
|
677 |
|
678 | // Acquire source credentials:
|
679 | const auth = new GoogleAuth();
|
680 | const client = await auth.getClient();
|
681 |
|
682 | // Impersonate new credentials:
|
683 | let targetClient = new Impersonated({
|
684 | sourceClient: client,
|
685 | targetPrincipal: 'impersonated-account@projectID.iam.gserviceaccount.com',
|
686 | lifetime: 30,
|
687 | delegates: [],
|
688 | targetScopes: ['https://www.googleapis.com/auth/cloud-platform']
|
689 | });
|
690 |
|
691 | // Get impersonated credentials:
|
692 | const authHeaders = await targetClient.getRequestHeaders();
|
693 | // Do something with `authHeaders.Authorization`.
|
694 |
|
695 | // Use impersonated credentials:
|
696 | const url = 'https://www.googleapis.com/storage/v1/b?project=anotherProjectID'
|
697 | const resp = await targetClient.request({ url });
|
698 | for (const bucket of resp.data.items) {
|
699 | console.log(bucket.name);
|
700 | }
|
701 |
|
702 | // Use impersonated credentials with google-cloud client library
|
703 | // Note: this works only with certain cloud client libraries utilizing gRPC
|
704 | // e.g., SecretManager, KMS, AIPlatform
|
705 | // will not currently work with libraries using REST, e.g., Storage, Compute
|
706 | const smClient = new SecretManagerServiceClient({
|
707 | projectId: anotherProjectID,
|
708 | auth: {
|
709 | getClient: () => targetClient,
|
710 | },
|
711 | });
|
712 | const secretName = 'projects/anotherProjectNumber/secrets/someProjectName/versions/1';
|
713 | const [accessResponse] = await smClient.accessSecretVersion({
|
714 | name: secretName,
|
715 | });
|
716 |
|
717 | const responsePayload = accessResponse.payload.data.toString('utf8');
|
718 | // Do something with the secret contained in `responsePayload`.
|
719 | };
|
720 |
|
721 | main();
|
722 | ```
|
723 |
|
724 |
|
725 | ## Samples
|
726 |
|
727 | Samples are in the [`samples/`](https://github.com/googleapis/google-auth-library-nodejs/tree/main/samples) directory. Each sample's `README.md` has instructions for running its sample.
|
728 |
|
729 | | Sample | Source Code | Try it |
|
730 | | --------------------------- | --------------------------------- | ------ |
|
731 | | Adc | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/adc.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/adc.js,samples/README.md) |
|
732 | | Compute | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/compute.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/compute.js,samples/README.md) |
|
733 | | Credentials | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/credentials.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/credentials.js,samples/README.md) |
|
734 | | Headers | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/headers.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/headers.js,samples/README.md) |
|
735 | | ID Tokens for Identity-Aware Proxy (IAP) | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/idtokens-iap.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/idtokens-iap.js,samples/README.md) |
|
736 | | ID Tokens for Serverless | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/idtokens-serverless.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/idtokens-serverless.js,samples/README.md) |
|
737 | | Jwt | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/jwt.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/jwt.js,samples/README.md) |
|
738 | | Keepalive | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/keepalive.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/keepalive.js,samples/README.md) |
|
739 | | Keyfile | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/keyfile.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/keyfile.js,samples/README.md) |
|
740 | | Oauth2-code Verifier | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/oauth2-codeVerifier.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/oauth2-codeVerifier.js,samples/README.md) |
|
741 | | Oauth2 | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/oauth2.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/oauth2.js,samples/README.md) |
|
742 | | Sign Blob | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/signBlob.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/signBlob.js,samples/README.md) |
|
743 | | Verifying ID Tokens from Identity-Aware Proxy (IAP) | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/verifyIdToken-iap.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/verifyIdToken-iap.js,samples/README.md) |
|
744 | | Verify Id Token | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/main/samples/verifyIdToken.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/verifyIdToken.js,samples/README.md) |
|
745 |
|
746 |
|
747 |
|
748 | The [Google Auth Library Node.js Client API Reference][client-docs] documentation
|
749 | also contains samples.
|
750 |
|
751 | ## Supported Node.js Versions
|
752 |
|
753 | Our client libraries follow the [Node.js release schedule](https://nodejs.org/en/about/releases/).
|
754 | Libraries are compatible with all current _active_ and _maintenance_ versions of
|
755 | Node.js.
|
756 |
|
757 | Client libraries targeting some end-of-life versions of Node.js are available, and
|
758 | can be installed via npm [dist-tags](https://docs.npmjs.com/cli/dist-tag).
|
759 | The dist-tags follow the naming convention `legacy-(version)`.
|
760 |
|
761 | _Legacy Node.js versions are supported as a best effort:_
|
762 |
|
763 | * Legacy versions will not be tested in continuous integration.
|
764 | * Some security patches may not be able to be backported.
|
765 | * Dependencies will not be kept up-to-date, and features will not be backported.
|
766 |
|
767 | #### Legacy tags available
|
768 |
|
769 | * `legacy-8`: install client libraries from this dist-tag for versions
|
770 | compatible with Node.js 8.
|
771 |
|
772 | ## Versioning
|
773 |
|
774 | This library follows [Semantic Versioning](http://semver.org/).
|
775 |
|
776 |
|
777 | This library is considered to be **General Availability (GA)**. This means it
|
778 | is stable; the code surface will not change in backwards-incompatible ways
|
779 | unless absolutely necessary (e.g. because of critical security issues) or with
|
780 | an extensive deprecation period. Issues and requests against **GA** libraries
|
781 | are addressed with the highest priority.
|
782 |
|
783 |
|
784 |
|
785 |
|
786 |
|
787 | More Information: [Google Cloud Platform Launch Stages][launch_stages]
|
788 |
|
789 | [launch_stages]: https://cloud.google.com/terms/launch-stages
|
790 |
|
791 | ## Contributing
|
792 |
|
793 | Contributions welcome! See the [Contributing Guide](https://github.com/googleapis/google-auth-library-nodejs/blob/main/CONTRIBUTING.md).
|
794 |
|
795 | Please note that this `README.md`, the `samples/README.md`,
|
796 | and a variety of configuration files in this repository (including `.nycrc` and `tsconfig.json`)
|
797 | are generated from a central template. To edit one of these files, make an edit
|
798 | to its templates in
|
799 | [directory](https://github.com/googleapis/synthtool).
|
800 |
|
801 | ## License
|
802 |
|
803 | Apache Version 2.0
|
804 |
|
805 | See [LICENSE](https://github.com/googleapis/google-auth-library-nodejs/blob/main/LICENSE)
|
806 |
|
807 | [client-docs]: https://cloud.google.com/nodejs/docs/reference/google-auth-library/latest
|
808 | [product-docs]: https://cloud.google.com/docs/authentication/
|
809 | [shell_img]: https://gstatic.com/cloudssh/images/open-btn.png
|
810 | [projects]: https://console.cloud.google.com/project
|
811 | [billing]: https://support.google.com/cloud/answer/6293499#enable-billing
|
812 |
|
813 | [auth]: https://cloud.google.com/docs/authentication/getting-started
|