1 | # httpntlm
|
2 |
|
3 | __httpntlm__ is a Node.js library to do HTTP NTLM authentication
|
4 |
|
5 | It's a port from the Python libary [python-ntml](https://code.google.com/p/python-ntlm/) with added NTLMv2 support.
|
6 |
|
7 | ## Donate
|
8 |
|
9 | Help keep my open source project alive! Your donation, no matter how small, makes a real difference.
|
10 |
|
11 | Thank you for your support!
|
12 |
|
13 |
|
14 | [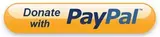](https://www.paypal.com/donate/?hosted_button_id=2CKNJLZJBW8ZC) [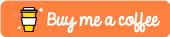](https://www.buymeacoffee.com/samdecrock)
|
15 |
|
16 |
|
17 |
|
18 | ## Install
|
19 |
|
20 | You can install __httpntlm__ using the Node Package Manager (npm):
|
21 |
|
22 | npm install httpntlm
|
23 |
|
24 | ## How to use
|
25 |
|
26 | ```js
|
27 | var httpntlm = require('httpntlm');
|
28 |
|
29 | httpntlm.get({
|
30 | url: "https://someurl.com",
|
31 | username: 'm$',
|
32 | password: 'stinks',
|
33 | workstation: 'choose.something',
|
34 | domain: ''
|
35 | }, function (err, res){
|
36 | if(err) return console.log(err);
|
37 |
|
38 | console.log(res.headers);
|
39 | console.log(res.body);
|
40 | });
|
41 | ```
|
42 |
|
43 | It supports __http__ and __https__.
|
44 |
|
45 | ## pre-encrypt the password
|
46 | ```js
|
47 |
|
48 | var httpntlm = require('httpntlm');
|
49 | var ntlm = httpntlm.ntlm;
|
50 | var lm = ntlm.create_LM_hashed_password('Azx123456');
|
51 | var nt = ntlm.create_NT_hashed_password('Azx123456');
|
52 | console.log(lm);
|
53 | console.log(Array.prototype.slice.call(lm, 0));
|
54 | lm = Buffer.from([ 183, 180, 19, 95, 163, 5, 118, 130, 30, 146, 159, 252, 1, 57, 81, 39 ]);
|
55 | console.log(lm);
|
56 |
|
57 | console.log(nt);
|
58 | console.log(Array.prototype.slice.call(nt, 0));
|
59 | nt = Buffer.from([150, 27, 7, 219, 220, 207, 134, 159, 42, 60, 153, 28, 131, 148, 14, 1]);
|
60 | console.log(nt);
|
61 |
|
62 |
|
63 | httpntlm.get({
|
64 | url: "https://someurl.com",
|
65 | username: 'm$',
|
66 | lm_password: lm,
|
67 | nt_password: nt,
|
68 | workstation: 'choose.something',
|
69 | domain: ''
|
70 | }, function (err, res){
|
71 | if(err) return console.log(err);
|
72 |
|
73 | console.log(res.headers);
|
74 | console.log(res.body);
|
75 | });
|
76 |
|
77 | /* you can save the array into your code and use it when you need it
|
78 |
|
79 | <Buffer b7 b4 13 5f a3 05 76 82 1e 92 9f fc 01 39 51 27>// before convert to array
|
80 | [ 183, 180, 19, 95, 163, 5, 118, 130, 30, 146, 159, 252, 1, 57, 81, 39 ]// convert to array
|
81 | <Buffer b7 b4 13 5f a3 05 76 82 1e 92 9f fc 01 39 51 27>//convert back to buffer
|
82 |
|
83 | <Buffer 96 1b 07 db dc cf 86 9f 2a 3c 99 1c 83 94 0e 01>
|
84 | [ 150, 27, 7, 219, 220, 207, 134, 159, 42, 60, 153, 28, 131, 148, 14, 1 ]
|
85 | <Buffer 96 1b 07 db dc cf 86 9f 2a 3c 99 1c 83 94 0e 01>
|
86 | */
|
87 |
|
88 | ```
|
89 |
|
90 |
|
91 | ## Options
|
92 |
|
93 | - `url:` _{String}_ URL to connect. (Required)
|
94 | - `username:` _{String}_ Username. (Required)
|
95 | - `password:` _{String}_ Password. (Required)
|
96 | - `workstation:` _{String}_ Name of workstation or `''`.
|
97 | - `domain:` _{String}_ Name of domain or `''`.
|
98 |
|
99 | if you already got the encrypted password,you should use this two param to replace the 'password' param.
|
100 |
|
101 | - `lm_password` _{Buffer}_ encrypted lm password.(Required)
|
102 | - `nt_password` _{Buffer}_ encrypted nt password. (Required)
|
103 |
|
104 | You can also pass along all other options of [httpreq](https://github.com/SamDecrock/node-httpreq), including custom headers, cookies, body data, ... and use POST, PUT or DELETE instead of GET.
|
105 |
|
106 | ## NTLMv2
|
107 |
|
108 | When NTLMv2 extended security and target information can be negotiated with the server, this library assumes
|
109 | the server supports NTLMv2 and creates responses according to the NTLMv2 specification (the actually supported
|
110 | NTLM version cannot be negotiated).
|
111 | Otherwise, NTLMv1 or NTLMv1 with NTLMv2 extended security will be used.
|
112 |
|
113 | ## Advanced
|
114 |
|
115 | If you want to use the NTLM-functions yourself, you can access the ntlm-library like this (https example):
|
116 |
|
117 | ```js
|
118 | var ntlm = require('httpntlm').ntlm;
|
119 | var async = require('async');
|
120 | var httpreq = require('httpreq');
|
121 | var HttpsAgent = require('agentkeepalive').HttpsAgent;
|
122 | var keepaliveAgent = new HttpsAgent();
|
123 |
|
124 | var options = {
|
125 | url: "https://someurl.com",
|
126 | username: 'm$',
|
127 | password: 'stinks',
|
128 | workstation: 'choose.something',
|
129 | domain: ''
|
130 | };
|
131 |
|
132 | async.waterfall([
|
133 | function (callback){
|
134 | var type1msg = ntlm.createType1Message(options);
|
135 |
|
136 | httpreq.get(options.url, {
|
137 | headers:{
|
138 | 'Connection' : 'keep-alive',
|
139 | 'Authorization': type1msg
|
140 | },
|
141 | agent: keepaliveAgent
|
142 | }, callback);
|
143 | },
|
144 |
|
145 | function (res, callback){
|
146 | if(!res.headers['www-authenticate'])
|
147 | return callback(new Error('www-authenticate not found on response of second request'));
|
148 |
|
149 | var type2msg = ntlm.parseType2Message(res.headers['www-authenticate']);
|
150 | var type3msg = ntlm.createType3Message(type2msg, options);
|
151 |
|
152 | setImmediate(function() {
|
153 | httpreq.get(options.url, {
|
154 | headers:{
|
155 | 'Connection' : 'Close',
|
156 | 'Authorization': type3msg
|
157 | },
|
158 | allowRedirects: false,
|
159 | agent: keepaliveAgent
|
160 | }, callback);
|
161 | });
|
162 | }
|
163 | ], function (err, res) {
|
164 | if(err) return console.log(err);
|
165 |
|
166 | console.log(res.headers);
|
167 | console.log(res.body);
|
168 | });
|
169 | ```
|
170 |
|
171 | ## Download binary files
|
172 |
|
173 | ```javascript
|
174 | httpntlm.get({
|
175 | url: "https://someurl.com/file.xls",
|
176 | username: 'm$',
|
177 | password: 'stinks',
|
178 | workstation: 'choose.something',
|
179 | domain: '',
|
180 | binary: true
|
181 | }, function (err, response) {
|
182 | if(err) return console.log(err);
|
183 | fs.writeFile("file.xls", response.body, function (err) {
|
184 | if(err) return console.log("error writing file");
|
185 | console.log("file.xls saved!");
|
186 | });
|
187 | });
|
188 | ```
|
189 |
|
190 | ## More information
|
191 |
|
192 | * [python-ntlm](https://code.google.com/p/python-ntlm/)
|
193 | * [NTLM Authentication Scheme for HTTP](http://www.innovation.ch/personal/ronald/ntlm.html)
|
194 | * [LM hash on Wikipedia](http://en.wikipedia.org/wiki/LM_hash)
|
195 |
|
196 | ## Contributing
|
197 |
|
198 | Running tests in an open source package is crucial for ensuring the quality and reliability of the codebase. When you submit code changes, it's essential to ensure that these changes don't break existing functionality or introduce new bugs.
|
199 |
|
200 | To run the tests, simply run
|
201 |
|
202 | node test.js
|
203 |
|
204 | All tests should return `true`
|
205 |
|
206 |
|
207 | ## License (MIT)
|
208 |
|
209 | Copyright (c) Sam Decrock <https://github.com/SamDecrock/>
|
210 |
|
211 | Permission is hereby granted, free of charge, to any person obtaining a copy
|
212 | of this software and associated documentation files (the "Software"), to deal
|
213 | in the Software without restriction, including without limitation the rights
|
214 | to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
215 | copies of the Software, and to permit persons to whom the Software is
|
216 | furnished to do so, subject to the following conditions:
|
217 |
|
218 | The above copyright notice and this permission notice shall be included in
|
219 | all copies or substantial portions of the Software.
|
220 |
|
221 | THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
222 | IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
223 | FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
224 | AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
225 | LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
226 | OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
227 | THE SOFTWARE.
|