1 | # httpntlm
|
2 |
|
3 | __httpntlm__ is a Node.js library to do HTTP NTLM authentication
|
4 |
|
5 | It's a port from the Python libary [python-ntml](https://code.google.com/p/python-ntlm/) with added NTLMv2 support.
|
6 |
|
7 | ## Donate
|
8 |
|
9 | Help keep my open source project alive! Your donation, no matter how small, makes a real difference.
|
10 |
|
11 | Thank you for your support!
|
12 |
|
13 |
|
14 | [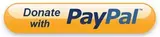](https://www.paypal.com/donate/?hosted_button_id=2CKNJLZJBW8ZC) [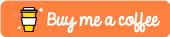](https://www.buymeacoffee.com/samdecrock)
|
15 |
|
16 |
|
17 |
|
18 | ## Install
|
19 |
|
20 | You can install __httpntlm__ using the Node Package Manager (npm):
|
21 |
|
22 | npm install httpntlm
|
23 |
|
24 | ## How to use
|
25 |
|
26 | ```js
|
27 | var httpntlm = require('httpntlm');
|
28 |
|
29 | httpntlm.get({
|
30 | url: "https://someurl.com",
|
31 | username: 'm$',
|
32 | password: 'stinks',
|
33 | workstation: 'choose.something',
|
34 | domain: ''
|
35 | }, function (err, res){
|
36 | if(err) return console.log(err);
|
37 |
|
38 | console.log(res.headers);
|
39 | console.log(res.body);
|
40 | });
|
41 | ```
|
42 |
|
43 | It supports __http__ and __https__.
|
44 |
|
45 | ## pre-encrypt the password
|
46 | ```js
|
47 |
|
48 | var httpntlm = require('httpntlm');
|
49 | var ntlm = httpntlm.ntlm;
|
50 | var lm = ntlm.create_LM_hashed_password('Azx123456');
|
51 | var nt = ntlm.create_NT_hashed_password('Azx123456');
|
52 | console.log(lm);
|
53 | console.log(Array.prototype.slice.call(lm, 0));
|
54 | lm = Buffer.from([ 183, 180, 19, 95, 163, 5, 118, 130, 30, 146, 159, 252, 1, 57, 81, 39 ]);
|
55 | console.log(lm);
|
56 |
|
57 | console.log(nt);
|
58 | console.log(Array.prototype.slice.call(nt, 0));
|
59 | nt = Buffer.from([150, 27, 7, 219, 220, 207, 134, 159, 42, 60, 153, 28, 131, 148, 14, 1]);
|
60 | console.log(nt);
|
61 |
|
62 |
|
63 | httpntlm.get({
|
64 | url: "https://someurl.com",
|
65 | username: 'm$',
|
66 | lm_password: lm,
|
67 | nt_password: nt,
|
68 | workstation: 'choose.something',
|
69 | domain: ''
|
70 | }, function (err, res){
|
71 | if(err) return console.log(err);
|
72 |
|
73 | console.log(res.headers);
|
74 | console.log(res.body);
|
75 | });
|
76 |
|
77 | /* you can save the array into your code and use it when you need it
|
78 |
|
79 | <Buffer b7 b4 13 5f a3 05 76 82 1e 92 9f fc 01 39 51 27>// before convert to array
|
80 | [ 183, 180, 19, 95, 163, 5, 118, 130, 30, 146, 159, 252, 1, 57, 81, 39 ]// convert to array
|
81 | <Buffer b7 b4 13 5f a3 05 76 82 1e 92 9f fc 01 39 51 27>//convert back to buffer
|
82 |
|
83 | <Buffer 96 1b 07 db dc cf 86 9f 2a 3c 99 1c 83 94 0e 01>
|
84 | [ 150, 27, 7, 219, 220, 207, 134, 159, 42, 60, 153, 28, 131, 148, 14, 1 ]
|
85 | <Buffer 96 1b 07 db dc cf 86 9f 2a 3c 99 1c 83 94 0e 01>
|
86 | */
|
87 |
|
88 | ```
|
89 |
|
90 |
|
91 | ## Options
|
92 |
|
93 | - `url:` _{String}_ URL to connect. (Required)
|
94 | - `username:` _{String}_ Username (optional, default: '')
|
95 | - `password:` _{String}_ Password (optional, default: '')
|
96 | - `workstation:` _{String}_ Name of workstation (optional, default: '')
|
97 | - `domain:` _{String}_ Name of domain (optional, default: '')
|
98 | - `agent:` _{Agent}_ In case you want to reuse the keepaliveAgent over different calls (optional)
|
99 |
|
100 | if you already got the encrypted password,you should use this two param to replace the 'password' param.
|
101 |
|
102 | - `lm_password` _{Buffer}_ encrypted lm password.(Required)
|
103 | - `nt_password` _{Buffer}_ encrypted nt password. (Required)
|
104 |
|
105 | You can also pass along all other options of [httpreq](https://github.com/SamDecrock/node-httpreq), including custom headers, cookies, body data, ... and use POST, PUT or DELETE instead of GET.
|
106 |
|
107 | ## NTLMv2
|
108 |
|
109 | When NTLMv2 extended security and target information can be negotiated with the server, this library assumes
|
110 | the server supports NTLMv2 and creates responses according to the NTLMv2 specification (the actually supported
|
111 | NTLM version cannot be negotiated).
|
112 | Otherwise, NTLMv1 or NTLMv1 with NTLMv2 extended security will be used.
|
113 |
|
114 | ## Advanced
|
115 |
|
116 | If you want to use the NTLM-functions yourself, you can access the ntlm-library like this (https example):
|
117 |
|
118 | ```js
|
119 | var ntlm = require('httpntlm').ntlm;
|
120 | var async = require('async');
|
121 | var httpreq = require('httpreq');
|
122 | var HttpsAgent = require('agentkeepalive').HttpsAgent;
|
123 | var keepaliveAgent = new HttpsAgent();
|
124 |
|
125 | var options = {
|
126 | url: "https://someurl.com",
|
127 | username: 'm$',
|
128 | password: 'stinks',
|
129 | workstation: 'choose.something',
|
130 | domain: ''
|
131 | };
|
132 |
|
133 | async.waterfall([
|
134 | function (callback){
|
135 | var type1msg = ntlm.createType1Message(options);
|
136 |
|
137 | httpreq.get(options.url, {
|
138 | headers:{
|
139 | 'Connection' : 'keep-alive',
|
140 | 'Authorization': type1msg
|
141 | },
|
142 | agent: keepaliveAgent
|
143 | }, callback);
|
144 | },
|
145 |
|
146 | function (res, callback){
|
147 | if(!res.headers['www-authenticate'])
|
148 | return callback(new Error('www-authenticate not found on response of second request'));
|
149 |
|
150 | var type2msg = ntlm.parseType2Message(res.headers['www-authenticate']);
|
151 | var type3msg = ntlm.createType3Message(type2msg, options);
|
152 |
|
153 | setImmediate(function() {
|
154 | httpreq.get(options.url, {
|
155 | headers:{
|
156 | 'Connection' : 'Close',
|
157 | 'Authorization': type3msg
|
158 | },
|
159 | allowRedirects: false,
|
160 | agent: keepaliveAgent
|
161 | }, callback);
|
162 | });
|
163 | }
|
164 | ], function (err, res) {
|
165 | if(err) return console.log(err);
|
166 |
|
167 | console.log(res.headers);
|
168 | console.log(res.body);
|
169 | });
|
170 | ```
|
171 |
|
172 | ## Download binary files
|
173 |
|
174 | ```javascript
|
175 | httpntlm.get({
|
176 | url: "https://someurl.com/file.xls",
|
177 | username: 'm$',
|
178 | password: 'stinks',
|
179 | workstation: 'choose.something',
|
180 | domain: '',
|
181 | binary: true
|
182 | }, function (err, response) {
|
183 | if(err) return console.log(err);
|
184 | fs.writeFile("file.xls", response.body, function (err) {
|
185 | if(err) return console.log("error writing file");
|
186 | console.log("file.xls saved!");
|
187 | });
|
188 | });
|
189 | ```
|
190 |
|
191 | ## More information
|
192 |
|
193 | * [python-ntlm](https://code.google.com/p/python-ntlm/)
|
194 | * [NTLM Authentication Scheme for HTTP](https://web.archive.org/web/20200724074947/https://www.innovation.ch/personal/ronald/ntlm.html)
|
195 | * [LM hash on Wikipedia](http://en.wikipedia.org/wiki/LM_hash)
|
196 |
|
197 | ## Contributing
|
198 |
|
199 | Running tests in an open source package is crucial for ensuring the quality and reliability of the codebase. When you submit code changes, it's essential to ensure that these changes don't break existing functionality or introduce new bugs.
|
200 |
|
201 | To run the unit tests, simply run
|
202 |
|
203 | node ./tests/unittests.js
|
204 |
|
205 | All tests should return `true`
|
206 |
|
207 | To run the integration tests, first start the NTLM server with
|
208 |
|
209 | node ./tests/integrationtests-server.js
|
210 |
|
211 | Next, run the integration tests with:
|
212 |
|
213 | node ./tests/integrationtests.js
|
214 |
|
215 |
|
216 | ## License (MIT)
|
217 |
|
218 | Copyright (c) Sam Decrock <https://github.com/SamDecrock/>
|
219 |
|
220 | Permission is hereby granted, free of charge, to any person obtaining a copy
|
221 | of this software and associated documentation files (the "Software"), to deal
|
222 | in the Software without restriction, including without limitation the rights
|
223 | to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
224 | copies of the Software, and to permit persons to whom the Software is
|
225 | furnished to do so, subject to the following conditions:
|
226 |
|
227 | The above copyright notice and this permission notice shall be included in
|
228 | all copies or substantial portions of the Software.
|
229 |
|
230 | THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
231 | IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
232 | FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
233 | AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
234 | LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
235 | OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
236 | THE SOFTWARE.
|