1 | <img width="75px" height="75px" align="right" alt="Inquirer Logo" src="https://raw.githubusercontent.com/SBoudrias/Inquirer.js/main/assets/inquirer_readme.svg?sanitize=true" title="Inquirer.js"/>
|
2 |
|
3 | # Inquirer.js
|
4 |
|
5 | [](https://www.npmjs.com/package/inquirer)
|
6 | [](https://app.fossa.com/projects/git%2Bgithub.com%2FSBoudrias%2FInquirer.js?ref=badge_shield)
|
7 |
|
8 | A collection of common interactive command line user interfaces.
|
9 |
|
10 | > [!IMPORTANT]
|
11 | > This is the legacy version of Inquirer.js. While it still receives maintenance, it is not actively developed. For the new Inquirer, see [@inquirer/prompts](https://www.npmjs.com/package/@inquirer/prompts).
|
12 |
|
13 | ## Table of Contents
|
14 |
|
15 | 1. [Documentation](#documentation)
|
16 | 1. [Installation](#installation)
|
17 | 2. [Examples](#examples)
|
18 | 3. [Methods](#methods)
|
19 | 4. [Objects](#objects)
|
20 | 5. [Question](#question)
|
21 | 6. [Answers](#answers)
|
22 | 7. [Separator](#separator)
|
23 | 8. [Prompt Types](#prompt-types)
|
24 | 2. [User Interfaces and Layouts](#user-interfaces-and-layouts)
|
25 | 1. [Reactive Interface](#reactive-interface)
|
26 | 3. [Support](#support)
|
27 | 4. [Known issues](#issues)
|
28 | 5. [News](#news)
|
29 | 6. [Contributing](#contributing)
|
30 | 7. [License](#license)
|
31 | 8. [Plugins](#plugins)
|
32 |
|
33 | ## Goal and Philosophy
|
34 |
|
35 | **`Inquirer.js`** strives to be an easily embeddable and beautiful command line interface for [Node.js](https://nodejs.org/) (and perhaps the "CLI [Xanadu](https://en.wikipedia.org/wiki/Citizen_Kane)").
|
36 |
|
37 | **`Inquirer.js`** should ease the process of
|
38 |
|
39 | - providing _error feedback_
|
40 | - _asking questions_
|
41 | - _parsing_ input
|
42 | - _validating_ answers
|
43 | - managing _hierarchical prompts_
|
44 |
|
45 | > **Note:** **`Inquirer.js`** provides the user interface and the inquiry session flow. If you're searching for a full blown command line program utility, then check out [commander](https://github.com/visionmedia/commander.js), [vorpal](https://github.com/dthree/vorpal) or [args](https://github.com/leo/args).
|
46 |
|
47 | ## [Documentation](#documentation)
|
48 |
|
49 | <a name="documentation"></a>
|
50 |
|
51 | ### Installation
|
52 |
|
53 | <a name="installation"></a>
|
54 |
|
55 | <table>
|
56 | <tr>
|
57 | <th>npm</th>
|
58 | <th>yarn</th>
|
59 | </tr>
|
60 | <tr>
|
61 | <td>
|
62 |
|
63 | ```sh
|
64 | npm install inquirer
|
65 | ```
|
66 |
|
67 | </td>
|
68 | <td>
|
69 |
|
70 | ```sh
|
71 | yarn add inquirer
|
72 | ```
|
73 |
|
74 | </td>
|
75 | </tr>
|
76 | </table>
|
77 |
|
78 | ```javascript
|
79 | import inquirer from 'inquirer';
|
80 |
|
81 | inquirer
|
82 | .prompt([
|
83 | /* Pass your questions in here */
|
84 | ])
|
85 | .then((answers) => {
|
86 | // Use user feedback for... whatever!!
|
87 | })
|
88 | .catch((error) => {
|
89 | if (error.isTtyError) {
|
90 | // Prompt couldn't be rendered in the current environment
|
91 | } else {
|
92 | // Something else went wrong
|
93 | }
|
94 | });
|
95 | ```
|
96 |
|
97 | <a name="examples"></a>
|
98 |
|
99 | ### Examples (Run it and see it)
|
100 |
|
101 | Check out the [`packages/inquirer/examples/`](https://github.com/SBoudrias/Inquirer.js/tree/main/packages/inquirer/examples) folder for code and interface examples.
|
102 |
|
103 | ```shell
|
104 | yarn node packages/inquirer/examples/pizza.js
|
105 | yarn node packages/inquirer/examples/checkbox.js
|
106 | # etc...
|
107 | ```
|
108 |
|
109 | ### Methods
|
110 |
|
111 | <a name="methods"></a>
|
112 |
|
113 | > [!WARNING]
|
114 | > Those interfaces are not necessary for modern Javascript, while still maintained, they're depreciated. We highly encourage you to adopt the more ergonomic and modern API with [@inquirer/prompts](https://www.npmjs.com/package/@inquirer/prompts). Both `inquirer` and `@inquirer/prompts` are usable at the same time, so you can progressively migrate.
|
115 |
|
116 | #### `inquirer.prompt(questions, answers) -> promise`
|
117 |
|
118 | Launch the prompt interface (inquiry session)
|
119 |
|
120 | - **questions** (Array) containing [Question Object](#question) (using the [reactive interface](#reactive-interface), you can also pass a `Rx.Observable` instance)
|
121 | - **answers** (object) contains values of already answered questions. Inquirer will avoid asking answers already provided here. Defaults `{}`.
|
122 | - returns a **Promise**
|
123 |
|
124 | #### `inquirer.registerPrompt(name, prompt)`
|
125 |
|
126 | Register prompt plugins under `name`.
|
127 |
|
128 | - **name** (string) name of the this new prompt. (used for question `type`)
|
129 | - **prompt** (object) the prompt object itself (the plugin)
|
130 |
|
131 | #### `inquirer.createPromptModule() -> prompt function`
|
132 |
|
133 | Create a self contained inquirer module. If you don't want to affect other libraries that also rely on inquirer when you overwrite or add new prompt types.
|
134 |
|
135 | ```js
|
136 | const prompt = inquirer.createPromptModule();
|
137 |
|
138 | prompt(questions).then(/* ... */);
|
139 | ```
|
140 |
|
141 | ### Objects
|
142 |
|
143 | <a name="objects"></a>
|
144 |
|
145 | #### Question
|
146 |
|
147 | <a name="questions"></a>
|
148 | A question object is a `hash` containing question related values:
|
149 |
|
150 | - **type**: (String) Type of the prompt. Defaults: `input` - Possible values: `input`, `number`, `confirm`, `list`, `rawlist`, `expand`, `checkbox`, `password`, `editor`
|
151 | - **name**: (String) The name to use when storing the answer in the answers hash. If the name contains periods, it will define a path in the answers hash.
|
152 | - **message**: (String|Function) The question to print. If defined as a function, the first parameter will be the current inquirer session answers. Defaults to the value of `name` (followed by a colon).
|
153 | - **default**: (String|Number|Boolean|Array|Function) Default value(s) to use if nothing is entered, or a function that returns the default value(s). If defined as a function, the first parameter will be the current inquirer session answers.
|
154 | - **choices**: (Array|Function) Choices array or a function returning a choices array. If defined as a function, the first parameter will be the current inquirer session answers.
|
155 | Array values can be simple `numbers`, `strings`, or `objects` containing a `name` (to display in list), a `value` (to save in the answers hash), and a `short` (to display after selection) properties. The choices array can also contain [a `Separator`](#separator).
|
156 | - **validate**: (Function) Receive the user input and answers hash. Should return `true` if the value is valid, and an error message (`String`) otherwise. If `false` is returned, a default error message is provided.
|
157 | - **filter**: (Function) Receive the user input and answers hash. Returns the filtered value to be used inside the program. The value returned will be added to the _Answers_ hash.
|
158 | - **transformer**: (Function) Receive the user input, answers hash and option flags, and return a transformed value to display to the user. The transformation only impacts what is shown while editing. It does not modify the answers hash.
|
159 | - **when**: (Function, Boolean) Receive the current user answers hash and should return `true` or `false` depending on whether or not this question should be asked. The value can also be a simple boolean.
|
160 | - **pageSize**: (Number) Change the number of lines that will be rendered when using `list`, `rawList`, `expand` or `checkbox`.
|
161 | - **prefix**: (String) Change the default _prefix_ message.
|
162 | - **suffix**: (String) Change the default _suffix_ message.
|
163 | - **askAnswered**: (Boolean) Force to prompt the question if the answer already exists.
|
164 | - **loop**: (Boolean) Enable list looping. Defaults: `true`
|
165 | - **waitUserInput**: (Boolean) Flag to enable/disable wait for user input before opening system editor - Defaults: `true`
|
166 |
|
167 | `default`, `choices`(if defined as functions), `validate`, `filter` and `when` functions can be called asynchronously. Either return a promise or use `this.async()` to get a callback you'll call with the final value.
|
168 |
|
169 | ```javascript
|
170 | {
|
171 | /* Preferred way: with promise */
|
172 | filter() {
|
173 | return new Promise(/* etc... */);
|
174 | },
|
175 |
|
176 | /* Legacy way: with this.async */
|
177 | validate: function (input) {
|
178 | // Declare function as asynchronous, and save the done callback
|
179 | const done = this.async();
|
180 |
|
181 | // Do async stuff
|
182 | setTimeout(function() {
|
183 | if (typeof input !== 'number') {
|
184 | // Pass the return value in the done callback
|
185 | done('You need to provide a number');
|
186 | } else {
|
187 | // Pass the return value in the done callback
|
188 | done(null, true);
|
189 | }
|
190 | }, 3000);
|
191 | }
|
192 | }
|
193 | ```
|
194 |
|
195 | ### Answers
|
196 |
|
197 | <a name="answers"></a>
|
198 | A key/value hash containing the client answers in each prompt.
|
199 |
|
200 | - **Key** The `name` property of the _question_ object
|
201 | - **Value** (Depends on the prompt)
|
202 | - `confirm`: (Boolean)
|
203 | - `input` : User input (filtered if `filter` is defined) (String)
|
204 | - `number`: User input (filtered if `filter` is defined) (Number)
|
205 | - `rawlist`, `list` : Selected choice value (or name if no value specified) (String)
|
206 |
|
207 | ### Separator
|
208 |
|
209 | <a name="separator"></a>
|
210 | A separator can be added to any `choices` array:
|
211 |
|
212 | ```
|
213 | // In the question object
|
214 | choices: [ "Choice A", new inquirer.Separator(), "choice B" ]
|
215 |
|
216 | // Which'll be displayed this way
|
217 | [?] What do you want to do?
|
218 | > Order a pizza
|
219 | Make a reservation
|
220 | --------
|
221 | Ask opening hours
|
222 | Talk to the receptionist
|
223 | ```
|
224 |
|
225 | The constructor takes a facultative `String` value that'll be use as the separator. If omitted, the separator will be `--------`.
|
226 |
|
227 | Separator instances have a property `type` equal to `separator`. This should allow tools façading Inquirer interface from detecting separator types in lists.
|
228 |
|
229 | <a name="prompt"></a>
|
230 |
|
231 | ### Prompt types
|
232 |
|
233 | ---
|
234 |
|
235 | > **Note:**: _allowed options written inside square brackets (`[]`) are optional. Others are required._
|
236 |
|
237 | #### List - `{type: 'list'}`
|
238 |
|
239 | Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties.
|
240 | (Note: `default` must be set to the `index` or `value` of one of the entries in `choices`)
|
241 |
|
242 | 
|
243 |
|
244 | ---
|
245 |
|
246 | #### Raw List - `{type: 'rawlist'}`
|
247 |
|
248 | Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties.
|
249 | (Note: `default` must be set to the `index` of one of the entries in `choices`)
|
250 |
|
251 | 
|
252 |
|
253 | ---
|
254 |
|
255 | #### Expand - `{type: 'expand'}`
|
256 |
|
257 | Take `type`, `name`, `message`, `choices`[, `default`] properties.
|
258 | Note: `default` must be the `index` of the desired default selection of the array. If `default` key not provided, then `help` will be used as default choice
|
259 |
|
260 | Note that the `choices` object will take an extra parameter called `key` for the `expand` prompt. This parameter must be a single (lowercased) character. The `h` option is added by the prompt and shouldn't be defined by the user.
|
261 |
|
262 | See `examples/expand.js` for a running example.
|
263 |
|
264 | 
|
265 | 
|
266 |
|
267 | ---
|
268 |
|
269 | #### Checkbox - `{type: 'checkbox'}`
|
270 |
|
271 | Take `type`, `name`, `message`, `choices`[, `filter`, `validate`, `default`, `loop`] properties. `default` is expected to be an Array of the checked choices value.
|
272 |
|
273 | Choices marked as `{checked: true}` will be checked by default.
|
274 |
|
275 | Choices whose property `disabled` is truthy will be unselectable. If `disabled` is a string, then the string will be outputted next to the disabled choice, otherwise it'll default to `"Disabled"`. The `disabled` property can also be a synchronous function receiving the current answers as argument and returning a boolean or a string.
|
276 |
|
277 | 
|
278 |
|
279 | ---
|
280 |
|
281 | #### Confirm - `{type: 'confirm'}`
|
282 |
|
283 | Take `type`, `name`, `message`, [`default`, `transformer`] properties. `default` is expected to be a boolean if used.
|
284 |
|
285 | 
|
286 |
|
287 | ---
|
288 |
|
289 | #### Input - `{type: 'input'}`
|
290 |
|
291 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
|
292 |
|
293 | 
|
294 |
|
295 | ---
|
296 |
|
297 | #### Input - `{type: 'number'}`
|
298 |
|
299 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
|
300 |
|
301 | ---
|
302 |
|
303 | #### Password - `{type: 'password'}`
|
304 |
|
305 | Take `type`, `name`, `message`, `mask`,[, `default`, `filter`, `validate`] properties.
|
306 |
|
307 | 
|
308 |
|
309 | ---
|
310 |
|
311 | Note that `mask` is required to hide the actual user input.
|
312 |
|
313 | #### Editor - `{type: 'editor'}`
|
314 |
|
315 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `postfix`, `waitUserInput`] properties
|
316 |
|
317 | Launches an instance of the users preferred editor on a temporary file. Once the user exits their editor, the contents of the temporary file are read in as the result. The editor to use is determined by reading the $VISUAL or $EDITOR environment variables. If neither of those are present, notepad (on Windows) or vim (Linux or Mac) is used.
|
318 |
|
319 | The `postfix` property is useful if you want to provide an extension.
|
320 |
|
321 | <a name="layouts"></a>
|
322 |
|
323 | ### Use in Non-Interactive Environments
|
324 |
|
325 | `prompt()` requires that it is run in an interactive environment. (I.e. [One where `process.stdin.isTTY` is `true`](https://nodejs.org/docs/latest-v12.x/api/process.html#process_a_note_on_process_i_o)). If `prompt()` is invoked outside of such an environment, then `prompt()` will return a rejected promise with an error. For convenience, the error will have a `isTtyError` property to programmatically indicate the cause.
|
326 |
|
327 | <a name="reactive"></a>
|
328 |
|
329 | ## Reactive interface
|
330 |
|
331 | Internally, Inquirer uses the [JS reactive extension](https://github.com/ReactiveX/rxjs) to handle events and async flows.
|
332 |
|
333 | This mean you can take advantage of this feature to provide more advanced flows. For example, you can dynamically add questions to be asked:
|
334 |
|
335 | ```js
|
336 | const prompts = new Rx.Subject();
|
337 | inquirer.prompt(prompts);
|
338 |
|
339 | // At some point in the future, push new questions
|
340 | prompts.next({
|
341 | /* question... */
|
342 | });
|
343 | prompts.next({
|
344 | /* question... */
|
345 | });
|
346 |
|
347 | // When you're done
|
348 | prompts.complete();
|
349 | ```
|
350 |
|
351 | And using the return value `process` property, you can access more fine grained callbacks:
|
352 |
|
353 | ```js
|
354 | inquirer.prompt(prompts).ui.process.subscribe(onEachAnswer, onError, onComplete);
|
355 | ```
|
356 |
|
357 | ## Support (OS Terminals)
|
358 |
|
359 | <a name="support"></a>
|
360 |
|
361 | You should expect mostly good support for the CLI below. This does not mean we won't
|
362 | look at issues found on other command line - feel free to report any!
|
363 |
|
364 | - **Mac OS**:
|
365 | - Terminal.app
|
366 | - iTerm
|
367 | - **Windows ([Known issues](#issues))**:
|
368 | - [Windows Terminal](https://github.com/microsoft/terminal)
|
369 | - [ConEmu](https://conemu.github.io/)
|
370 | - cmd.exe
|
371 | - Powershell
|
372 | - Cygwin
|
373 | - **Linux (Ubuntu, openSUSE, Arch Linux, etc)**:
|
374 | - gnome-terminal (Terminal GNOME)
|
375 | - konsole
|
376 |
|
377 | ## Known issues
|
378 |
|
379 | <a name="issues"></a>
|
380 |
|
381 | - **nodemon** - Makes the arrow keys print gibrish on list prompts.
|
382 | Workaround: Add `{ stdin : false }` in the configuration file or pass `--no-stdin` in the CLI.
|
383 | Please refer to [this issue](https://github.com/SBoudrias/Inquirer.js/issues/844#issuecomment-736675867)
|
384 |
|
385 | - **grunt-exec** - Calling a node script that uses Inquirer from grunt-exec can cause the program to crash. To fix this, add to your grunt-exec config `stdio: 'inherit'`.
|
386 | Please refer to [this issue](https://github.com/jharding/grunt-exec/issues/85)
|
387 |
|
388 | - **Windows network streams** - Running Inquirer together with network streams in Windows platform inside some terminals can result in process hang.
|
389 | Workaround: run inside another terminal.
|
390 | Please refer to [this issue](https://github.com/nodejs/node/issues/21771)
|
391 |
|
392 | ## News on the march (Release notes)
|
393 |
|
394 | <a name="news"></a>
|
395 |
|
396 | Please refer to the [GitHub releases section for the changelog](https://github.com/SBoudrias/Inquirer.js/releases)
|
397 |
|
398 | ## Contributing
|
399 |
|
400 | <a name="contributing"></a>
|
401 |
|
402 | **Unit test**
|
403 | Please add a unit test for every new feature or bug fix. `yarn test` to run the test suite.
|
404 |
|
405 | **Documentation**
|
406 | Add documentation for every API change. Feel free to send typo fixes and better docs!
|
407 |
|
408 | We're looking to offer good support for multiple prompts and environments. If you want to
|
409 | help, we'd like to keep a list of testers for each terminal/OS so we can contact you and
|
410 | get feedback before release. Let us know if you want to be added to the list (just tweet
|
411 | to [@vaxilart](https://twitter.com/Vaxilart)) or just add your name to [the wiki](https://github.com/SBoudrias/Inquirer.js/wiki/Testers)
|
412 |
|
413 | ## License
|
414 |
|
415 | <a name="license"></a>
|
416 |
|
417 | Copyright (c) 2023 Simon Boudrias (twitter: [@vaxilart](https://twitter.com/Vaxilart))<br/>
|
418 | Licensed under the MIT license.
|
419 |
|
420 | ## Plugins
|
421 |
|
422 | <a name="plugins"></a>
|
423 |
|
424 | You can build custom prompts, or use open sourced ones. See [`@inquirer/core` documentation for building custom prompts](https://github.com/SBoudrias/Inquirer.js/tree/main/packages/core).
|
425 |
|
426 | You can either call the custom prompts directly (preferred), or you can register them (depreciated):
|
427 |
|
428 | ```js
|
429 | import customPrompt from '$$$/custom-prompt';
|
430 |
|
431 | // 1. Preferred solution with new plugins
|
432 | const answer = await customPrompt({ ...config });
|
433 |
|
434 | // 2. Depreciated interface (or for old plugins)
|
435 | inquirer.registerPrompt('custom', customPrompt);
|
436 | const answers = await inquirer.prompt([
|
437 | {
|
438 | type: 'custom',
|
439 | ...config,
|
440 | },
|
441 | ]);
|
442 | ```
|
443 |
|
444 | When using Typescript and `registerPrompt`, you'll also need to define your prompt signature. Since Typescript is static, we cannot infer available plugins from function calls.
|
445 |
|
446 | ```ts
|
447 | import customPrompt from '$$$/custom-prompt';
|
448 |
|
449 | declare module 'inquirer' {
|
450 | interface QuestionMap {
|
451 | // 1. Easiest option
|
452 | custom: Parameters<typeof customPrompt>[0];
|
453 |
|
454 | // 2. Or manually define the prompt config
|
455 | custom_alt: { message: string; option: number[] };
|
456 | }
|
457 | }
|
458 | ```
|
459 |
|
460 | ### Prompts
|
461 |
|
462 | [**autocomplete**](https://github.com/mokkabonna/inquirer-autocomplete-prompt)<br>
|
463 | Presents a list of options as the user types, compatible with other packages such as fuzzy (for search)<br>
|
464 | <br>
|
465 | 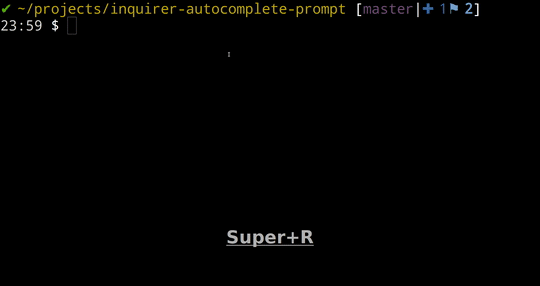
|
466 |
|
467 | [**checkbox-plus**](https://github.com/faressoft/inquirer-checkbox-plus-prompt)<br>
|
468 | Checkbox list with autocomplete and other additions<br>
|
469 | <br>
|
470 | 
|
471 |
|
472 | [**inquirer-date-prompt**](https://github.com/haversnail/inquirer-date-prompt)<br>
|
473 | Customizable date/time selector with localization support<br>
|
474 | <br>
|
475 | 
|
476 |
|
477 | [**datetime**](https://github.com/DerekTBrown/inquirer-datepicker-prompt)<br>
|
478 | Customizable date/time selector using both number pad and arrow keys<br>
|
479 | <br>
|
480 | 
|
481 |
|
482 | [**inquirer-select-line**](https://github.com/adam-golab/inquirer-select-line)<br>
|
483 | Prompt for selecting index in array where add new element<br>
|
484 | <br>
|
485 | 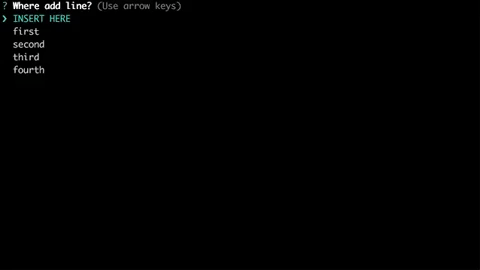
|
486 |
|
487 | [**command**](https://github.com/sullof/inquirer-command-prompt)<br>
|
488 | Simple prompt with command history and dynamic autocomplete<br>
|
489 |
|
490 | [**inquirer-fuzzy-path**](https://github.com/adelsz/inquirer-fuzzy-path)<br>
|
491 | Prompt for fuzzy file/directory selection.<br>
|
492 | <br>
|
493 | 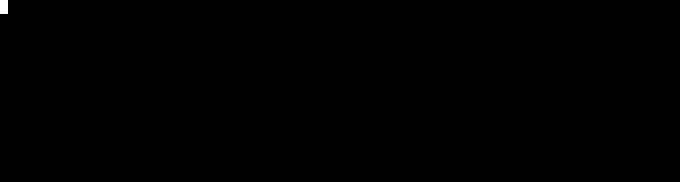
|
494 |
|
495 | [**inquirer-emoji**](https://github.com/tannerntannern/inquirer-emoji)<br>
|
496 | Prompt for inputting emojis.<br>
|
497 | <br>
|
498 | 
|
499 |
|
500 | [**inquirer-chalk-pipe**](https://github.com/LitoMore/inquirer-chalk-pipe)<br>
|
501 | Prompt for input chalk-pipe style strings<br>
|
502 | <br>
|
503 | 
|
504 |
|
505 | [**inquirer-search-checkbox**](https://github.com/clinyong/inquirer-search-checkbox)<br>
|
506 | Searchable Inquirer checkbox<br>
|
507 | 
|
508 |
|
509 | [**inquirer-search-list**](https://github.com/robin-rpr/inquirer-search-list)<br>
|
510 | Searchable Inquirer list<br>
|
511 | <br>
|
512 | 
|
513 |
|
514 | [**inquirer-prompt-suggest**](https://github.com/olistic/inquirer-prompt-suggest)<br>
|
515 | Inquirer prompt for your less creative users.<br>
|
516 | <br>
|
517 | 
|
518 |
|
519 | [**inquirer-s3**](https://github.com/HQarroum/inquirer-s3)<br>
|
520 | An S3 object selector for Inquirer.<br>
|
521 | <br>
|
522 | 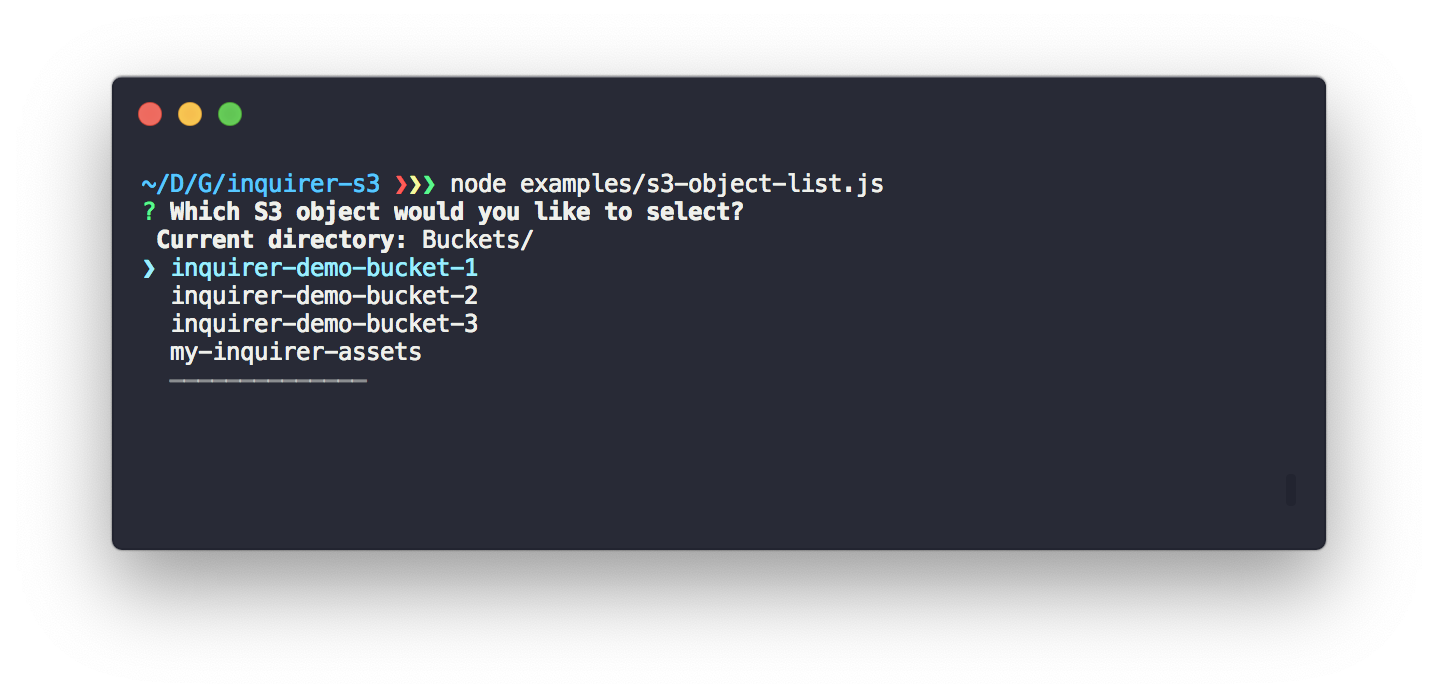
|
523 |
|
524 | [**inquirer-autosubmit-prompt**](https://github.com/yaodingyd/inquirer-autosubmit-prompt)<br>
|
525 | Auto submit based on your current input, saving one extra enter<br>
|
526 |
|
527 | [**inquirer-file-tree-selection-prompt**](https://github.com/anc95/inquirer-file-tree-selection)<br>
|
528 | Inquirer prompt for to select a file or directory in file tree<br>
|
529 | <br>
|
530 | 
|
531 |
|
532 | [**inquirer-tree-prompt**](https://github.com/insightfuls/inquirer-tree-prompt)<br>
|
533 | Inquirer prompt to select from a tree<br>
|
534 | <br>
|
535 | 
|
536 |
|
537 | [**inquirer-table-prompt**](https://github.com/eduardoboucas/inquirer-table-prompt)<br>
|
538 | A table-like prompt for Inquirer.<br>
|
539 | <br>
|
540 | 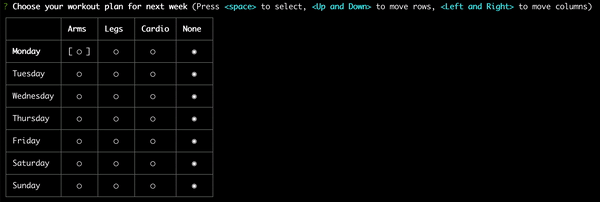
|
541 |
|
542 | [**inquirer-table-input**](https://github.com/edelciomolina/inquirer-table-input)<br>
|
543 | A table editing prompt for Inquirer.<br>
|
544 | <br>
|
545 | 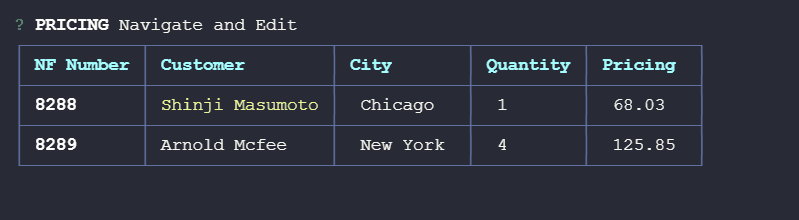
|
546 |
|
547 | [**inquirer-interrupted-prompt**](https://github.com/lnquy065/inquirer-interrupted-prompt)<br>
|
548 | Turning any existing inquirer and its plugin prompts into prompts that can be interrupted with a custom key.<br>
|
549 | <br>
|
550 | 
|
551 |
|
552 | [**inquirer-press-to-continue**](https://github.com/leonzalion/inquirer-press-to-continue)<br>
|
553 | A "press any key to continue" prompt for Inquirer.js<br>
|
554 | <br>
|
555 | 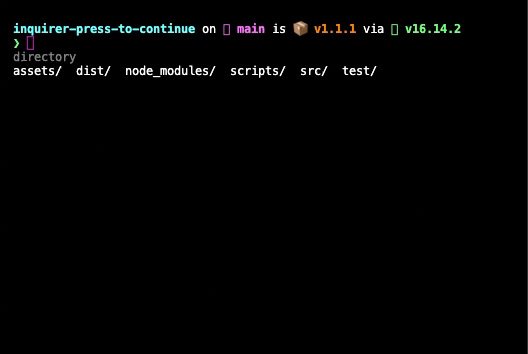
|