1 | # ipfsd-ctl, the IPFS Factory
|
2 |
|
3 | [](http://protocol.ai)
|
4 | [](http://ipfs.io/)
|
5 | [](http://webchat.freenode.net/?channels=%23ipfs)
|
6 | [](https://github.com/ipfs/js-ipfsd-ctl/actions/workflows/js-test-and-release.yml)
|
7 | [](https://codecov.io/gh/ipfs/js-ipfs-multipart)
|
8 | [](https://github.com/feross/standard)
|
9 | [](https://bundlephobia.com/result?p=ipfsd-ctl)
|
10 | > Spawn IPFS daemons using JavaScript!
|
11 |
|
12 | ## Lead Maintainer
|
13 |
|
14 | [Hugo Dias](https://github.com/hugomrdias)
|
15 |
|
16 | ## Notice
|
17 | Version 1.0.0 changed a bit the api and the options methods take so please read the documentation below.
|
18 |
|
19 | ## Table of Contents
|
20 |
|
21 | - [Install](#install)
|
22 | - [Usage](#usage)
|
23 | - [API](#api)
|
24 | - [Packaging](#packaging)
|
25 | - [Contribute](#contribute)
|
26 | - [License](#license)
|
27 |
|
28 | ## Install
|
29 |
|
30 | ```sh
|
31 | npm install --save ipfsd-ctl
|
32 | ```
|
33 |
|
34 | Please ensure your project also has dependencies on `ipfs`, `ipfs-http-client` and `go-ipfs`.
|
35 |
|
36 | ```sh
|
37 | npm install --save ipfs
|
38 | npm install --save ipfs-http-client
|
39 | npm install --save go-ipfs
|
40 | ```
|
41 |
|
42 | If you are only going to use the `go` implementation of IPFS, you can skip installing the `js` implementation and vice versa, though both will require the `ipfs-http-client` module.
|
43 |
|
44 | If you are only using the `proc` type in-process IPFS node, you can skip installing `go-ipfs` and `ipfs-http-client`.
|
45 |
|
46 | > You also need to explicitly defined the options `ipfsBin`, `ipfsModule` and `ipfsHttpModule` according to your needs. Check [ControllerOptions](#controlleroptions) and [ControllerOptionsOverrides](#controlleroptionsoverrides) for more information.
|
47 |
|
48 | ## Usage
|
49 |
|
50 | ### Spawning a single IPFS controller: `createController`
|
51 |
|
52 | This is a shorthand for simpler use cases where factory is not needed.
|
53 |
|
54 | ```js
|
55 | // No need to create a factory when only a single controller is needed.
|
56 | // Use createController to spawn it instead.
|
57 | const Ctl = require('ipfsd-ctl')
|
58 | const ipfsd = await Ctl.createController({
|
59 | ipfsHttpModule,
|
60 | ipfsBin: goIpfsModule.path()
|
61 | })
|
62 | const id = await ipfsd.api.id()
|
63 |
|
64 | console.log(id)
|
65 |
|
66 | await ipfsd.stop()
|
67 | ```
|
68 |
|
69 | ### Manage multiple IPFS controllers: `createFactory`
|
70 |
|
71 | Use a factory to spawn multiple controllers based on some common template.
|
72 |
|
73 | **Spawn an IPFS daemon from Node.js**
|
74 |
|
75 | ```js
|
76 | // Create a factory to spawn two test disposable controllers, get access to an IPFS api
|
77 | // print node ids and clean all the controllers from the factory.
|
78 | const Ctl = require('ipfsd-ctl')
|
79 |
|
80 | const factory = Ctl.createFactory(
|
81 | {
|
82 | type: 'js',
|
83 | test: true,
|
84 | disposable: true,
|
85 | ipfsHttpModule,
|
86 | ipfsModule: (await import('ipfs')) // only if you gonna spawn 'proc' controllers
|
87 | },
|
88 | { // overrides per type
|
89 | js: {
|
90 | ipfsBin: ipfsModule.path()
|
91 | },
|
92 | go: {
|
93 | ipfsBin: goIpfsModule.path()
|
94 | }
|
95 | }
|
96 | )
|
97 | const ipfsd1 = await factory.spawn() // Spawns using options from `createFactory`
|
98 | const ipfsd2 = await factory.spawn({ type: 'go' }) // Spawns using options from `createFactory` but overrides `type` to spawn a `go` controller
|
99 |
|
100 | console.log(await ipfsd1.api.id())
|
101 | console.log(await ipfsd2.api.id())
|
102 |
|
103 | await factory.clean() // Clean all the controllers created by the factory calling `stop` on all of them.
|
104 | ```
|
105 |
|
106 | **Spawn an IPFS daemon from the Browser using the provided remote endpoint**
|
107 |
|
108 | ```js
|
109 | // Start a remote disposable node, and get access to the api
|
110 | // print the node id, and stop the temporary daemon
|
111 |
|
112 | const Ctl = require('ipfsd-ctl')
|
113 |
|
114 | const port = 9090
|
115 | const server = Ctl.createServer(port, {
|
116 | ipfsModule,
|
117 | ipfsHttpModule
|
118 | },
|
119 | {
|
120 | js: {
|
121 | ipfsBin: ipfsModule.path()
|
122 | },
|
123 | go: {
|
124 | ipfsBin: goIpfsModule.path()
|
125 | },
|
126 | })
|
127 | const factory = Ctl.createFactory({
|
128 | ipfsHttpModule,
|
129 | remote: true,
|
130 | endpoint: `http://localhost:${port}` // or you can set process.env.IPFSD_CTL_SERVER to http://localhost:9090
|
131 | })
|
132 |
|
133 | await server.start()
|
134 | const ipfsd = await factory.spawn()
|
135 | const id = await ipfsd.api.id()
|
136 |
|
137 | console.log(id)
|
138 |
|
139 | await ipfsd.stop()
|
140 | await server.stop()
|
141 | ```
|
142 |
|
143 | ## Disposable vs non Disposable nodes
|
144 |
|
145 | `ipfsd-ctl` can spawn `disposable` and `non-disposable` nodes.
|
146 |
|
147 | - `disposable`- Disposable nodes are useful for tests or other temporary use cases, by default they create a temporary repo and automatically initialise and start the node, plus they cleanup everything when stopped.
|
148 | - `non-disposable` - Non disposable nodes will by default attach to any nodes running on the default or the supplied repo. Requires the user to initialize and start the node, as well as stop and cleanup afterwards.
|
149 |
|
150 | ## API
|
151 |
|
152 | ### `createFactory([options], [overrides])`
|
153 | Creates a factory that can spawn multiple controllers and pre-define options for them.
|
154 |
|
155 | - `options` **[ControllerOptions](#controlleroptions)** Controllers options.
|
156 | - `overrides` **[ControllerOptionsOverrides](#controlleroptionsoverrides)** Pre-defined options overrides per controller type.
|
157 |
|
158 | Returns a **[Factory](#factory)**
|
159 |
|
160 | ### `createController([options])`
|
161 | Creates a controller.
|
162 |
|
163 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
164 |
|
165 | Returns **Promise<[Controller](#controller)>**
|
166 |
|
167 | ### `createServer([options])`
|
168 | Create an Endpoint Server. This server is used by a client node to control a remote node. Example: Spawning a go-ipfs node from a browser.
|
169 |
|
170 | - `options` **[Object]** Factory options. Defaults to: `{ port: 43134 }`
|
171 | - `port` **number** Port to start the server on.
|
172 |
|
173 | Returns a **Server**
|
174 |
|
175 | ### Factory
|
176 |
|
177 | #### `controllers`
|
178 | **Controller[]** List of all the controllers spawned.
|
179 |
|
180 | #### `tmpDir()`
|
181 | Create a temporary repo to create controllers manually.
|
182 |
|
183 | Returns **Promise<String>** - Path to the repo.
|
184 |
|
185 | #### `spawn([options])`
|
186 | Creates a controller for a IPFS node.
|
187 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
188 |
|
189 | Returns **Promise<[Controller](#controller)>**
|
190 |
|
191 | #### `clean()`
|
192 | Cleans all controllers spawned.
|
193 |
|
194 | Returns **Promise<[Factory](#factory)>**
|
195 |
|
196 | ### Controller
|
197 | Class controller for a IPFS node.
|
198 |
|
199 | #### `new Controller(options)`
|
200 |
|
201 | - `options` **[ControllerOptions](#controlleroptions)**
|
202 |
|
203 | #### `path`
|
204 | **String** Repo path.
|
205 |
|
206 | #### `exec`
|
207 | **String** Executable path.
|
208 |
|
209 | #### `env`
|
210 | **Object** ENV object.
|
211 |
|
212 | #### `initialized`
|
213 | **Boolean** Flag with the current init state.
|
214 |
|
215 | #### `started`
|
216 | **Boolean** Flag with the current start state.
|
217 |
|
218 | #### `clean`
|
219 | **Boolean** Flag with the current clean state.
|
220 |
|
221 | #### `apiAddr`
|
222 | **Multiaddr** API address
|
223 |
|
224 | #### `gatewayAddr`
|
225 | **Multiaddr** Gateway address
|
226 |
|
227 | #### `api`
|
228 | **Object** IPFS core interface
|
229 |
|
230 | #### `init([initOptions])`
|
231 | Initialises controlled node
|
232 |
|
233 | - `initOptions` **[Object]** IPFS init options https://github.com/ipfs/js-ipfs/blob/master/README.md#optionsinit
|
234 |
|
235 | Returns **Promise<[Controller](#controller)>**
|
236 |
|
237 | #### `start()`
|
238 | Starts controlled node.
|
239 |
|
240 | Returns **Promise<IPFS>**
|
241 |
|
242 | #### `stop()`
|
243 | Stops controlled node.
|
244 |
|
245 | Returns **Promise<[Controller](#controller)>**
|
246 |
|
247 | #### `cleanup()`
|
248 | Cleans controlled node, a disposable controller calls this automatically.
|
249 |
|
250 | Returns **Promise<[Controller](#controller)>**
|
251 |
|
252 |
|
253 | #### `pid()`
|
254 | Get the pid of the controlled node process if aplicable.
|
255 |
|
256 | Returns **Promise<number>**
|
257 |
|
258 |
|
259 | #### `version()`
|
260 | Get the version of the controlled node.
|
261 |
|
262 | Returns **Promise<string>**
|
263 |
|
264 |
|
265 | ### ControllerOptionsOverrides
|
266 |
|
267 | Type: [Object]
|
268 |
|
269 | #### Properties
|
270 | - `js` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **JS** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
271 | - `go` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Go** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
272 | - `proc` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Proc** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
273 |
|
274 |
|
275 | ### ControllerOptions
|
276 |
|
277 | Type: [Object]
|
278 |
|
279 | #### Properties
|
280 | - `test` **[boolean]** Flag to activate custom config for tests.
|
281 | - `remote` **[boolean]** Use remote endpoint to spawn the nodes. Defaults to `true` when not in node.
|
282 | - `endpoint` **[string]** Endpoint URL to manage remote Controllers. (Defaults: 'http://localhost:43134').
|
283 | - `disposable` **[boolean]** A new repo is created and initialized for each invocation, as well as cleaned up automatically once the process exits.
|
284 | - `type` **[string]** The daemon type, see below the options:
|
285 | - go - spawn go-ipfs daemon
|
286 | - js - spawn js-ipfs daemon
|
287 | - proc - spawn in-process js-ipfs node
|
288 | - `env` **[Object]** Additional environment variables, passed to executing shell. Only applies for Daemon controllers.
|
289 | - `args` **[Array]** Custom cli args.
|
290 | - `ipfsHttpModule` **[Object]** Reference to a IPFS HTTP Client object.
|
291 | - `ipfsModule` **[Object]** Reference to a IPFS API object.
|
292 | - `ipfsBin` **[string]** Path to a IPFS exectutable.
|
293 | - `ipfsOptions` **[IpfsOptions]** Options for the IPFS instance same as https://github.com/ipfs/js-ipfs#ipfs-constructor. `proc` nodes receive these options as is, daemon nodes translate the options as far as possible to cli arguments.
|
294 | - `forceKill` **[boolean]** - Whether to use SIGKILL to quit a daemon that does not stop after `.stop()` is called. (default `true`)
|
295 | - `forceKillTimeout` **[Number]** - How long to wait before force killing a daemon in ms. (default `5000`)
|
296 |
|
297 |
|
298 | ## ipfsd-ctl environment variables
|
299 |
|
300 | In additional to the API described in previous sections, `ipfsd-ctl` also supports several environment variables. This are often very useful when running in different environments, such as CI or when doing integration/interop testing.
|
301 |
|
302 | _Environment variables precedence order is as follows. Top to bottom, top entry has highest precedence:_
|
303 |
|
304 | - command line options/method arguments
|
305 | - env variables
|
306 | - default values
|
307 |
|
308 | Meaning that, environment variables override defaults in the configuration file but are superseded by options to `df.spawn({...})`
|
309 |
|
310 | #### IPFS_JS_EXEC and IPFS_GO_EXEC
|
311 |
|
312 | An alternative way of specifying the executable path for the `js-ipfs` or `go-ipfs` executable, respectively.
|
313 |
|
314 |
|
315 | ## Contribute
|
316 |
|
317 | Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfsd-ctl/issues)!
|
318 |
|
319 | This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md).
|
320 |
|
321 | [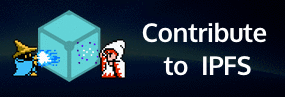](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md)
|
322 |
|
323 | ## License
|
324 |
|
325 | [MIT](LICENSE)
|