1 | # ipfsd-ctl <!-- omit in toc -->
|
2 |
|
3 | [](http://ipfs.io)
|
4 | [](http://webchat.freenode.net/?channels=%23ipfs)
|
5 | [](https://discord.gg/ipfs)
|
6 | [](https://codecov.io/gh/ipfs/js-ipfsd-ctl)
|
7 | [](https://github.com/ipfs/js-ipfsd-ctl/actions/workflows/js-test-and-release.yml)
|
8 |
|
9 | > Spawn IPFS Daemons, JS or Go
|
10 |
|
11 | ## Table of contents <!-- omit in toc -->
|
12 |
|
13 | - [Install](#install)
|
14 | - [Notice](#notice)
|
15 | - [Usage](#usage)
|
16 | - [Spawning a single IPFS controller: `createController`](#spawning-a-single-ipfs-controller-createcontroller)
|
17 | - [Manage multiple IPFS controllers: `createFactory`](#manage-multiple-ipfs-controllers-createfactory)
|
18 | - [Disposable vs non Disposable nodes](#disposable-vs-non-disposable-nodes)
|
19 | - [API](#api)
|
20 | - [`createFactory([options], [overrides])`](#createfactoryoptions-overrides)
|
21 | - [`createController([options])`](#createcontrolleroptions)
|
22 | - [`createServer([options])`](#createserveroptions)
|
23 | - [Factory](#factory)
|
24 | - [`controllers`](#controllers)
|
25 | - [`tmpDir()`](#tmpdir)
|
26 | - [`spawn([options])`](#spawnoptions)
|
27 | - [`clean()`](#clean)
|
28 | - [Controller](#controller)
|
29 | - [`new Controller(options)`](#new-controlleroptions)
|
30 | - [`path`](#path)
|
31 | - [`exec`](#exec)
|
32 | - [`env`](#env)
|
33 | - [`initialized`](#initialized)
|
34 | - [`started`](#started)
|
35 | - [`clean`](#clean-1)
|
36 | - [`apiAddr`](#apiaddr)
|
37 | - [`gatewayAddr`](#gatewayaddr)
|
38 | - [`api`](#api-1)
|
39 | - [`init([initOptions])`](#initinitoptions)
|
40 | - [`start()`](#start)
|
41 | - [`stop()`](#stop)
|
42 | - [`cleanup()`](#cleanup)
|
43 | - [`pid()`](#pid)
|
44 | - [`version()`](#version)
|
45 | - [ControllerOptionsOverrides](#controlleroptionsoverrides)
|
46 | - [Properties](#properties)
|
47 | - [ControllerOptions](#controlleroptions)
|
48 | - [Properties](#properties-1)
|
49 | - [ipfsd-ctl environment variables](#ipfsd-ctl-environment-variables)
|
50 | - - [IPFS\_JS\_EXEC and IPFS\_GO\_EXEC](#ipfs_js_exec-and-ipfs_go_exec)
|
51 | - [Contribute](#contribute)
|
52 | - [License](#license)
|
53 | - [Contribute](#contribute-1)
|
54 |
|
55 | ## Install
|
56 |
|
57 | ```console
|
58 | $ npm i ipfsd-ctl
|
59 | ```
|
60 |
|
61 | ## Notice
|
62 |
|
63 | Version 1.0.0 changed a bit the api and the options methods take so please read the documentation below.
|
64 |
|
65 | Please ensure your project also has dependencies on `ipfs`, `ipfs-http-client` and `go-ipfs`.
|
66 |
|
67 | ```sh
|
68 | npm install --save ipfs
|
69 | npm install --save ipfs-http-client
|
70 | npm install --save go-ipfs
|
71 | ```
|
72 |
|
73 | If you are only going to use the `go` implementation of IPFS, you can skip installing the `js` implementation and vice versa, though both will require the `ipfs-http-client` module.
|
74 |
|
75 | If you are only using the `proc` type in-process IPFS node, you can skip installing `go-ipfs` and `ipfs-http-client`.
|
76 |
|
77 | > You also need to explicitly defined the options `ipfsBin`, `ipfsModule` and `ipfsHttpModule` according to your needs. Check [ControllerOptions](#controlleroptions) and [ControllerOptionsOverrides](#controlleroptionsoverrides) for more information.
|
78 |
|
79 | ## Usage
|
80 |
|
81 | ### Spawning a single IPFS controller: `createController`
|
82 |
|
83 | This is a shorthand for simpler use cases where factory is not needed.
|
84 |
|
85 | ```js
|
86 | // No need to create a factory when only a single controller is needed.
|
87 | // Use createController to spawn it instead.
|
88 | const Ctl = require('ipfsd-ctl')
|
89 | const ipfsd = await Ctl.createController({
|
90 | ipfsHttpModule,
|
91 | ipfsBin: goIpfsModule.path()
|
92 | })
|
93 | const id = await ipfsd.api.id()
|
94 |
|
95 | console.log(id)
|
96 |
|
97 | await ipfsd.stop()
|
98 | ```
|
99 |
|
100 | ### Manage multiple IPFS controllers: `createFactory`
|
101 |
|
102 | Use a factory to spawn multiple controllers based on some common template.
|
103 |
|
104 | **Spawn an IPFS daemon from Node.js**
|
105 |
|
106 | ```js
|
107 | // Create a factory to spawn two test disposable controllers, get access to an IPFS api
|
108 | // print node ids and clean all the controllers from the factory.
|
109 | const Ctl = require('ipfsd-ctl')
|
110 |
|
111 | const factory = Ctl.createFactory(
|
112 | {
|
113 | type: 'js',
|
114 | test: true,
|
115 | disposable: true,
|
116 | ipfsHttpModule,
|
117 | ipfsModule: (await import('ipfs')) // only if you gonna spawn 'proc' controllers
|
118 | },
|
119 | { // overrides per type
|
120 | js: {
|
121 | ipfsBin: ipfsModule.path()
|
122 | },
|
123 | go: {
|
124 | ipfsBin: goIpfsModule.path()
|
125 | }
|
126 | }
|
127 | )
|
128 | const ipfsd1 = await factory.spawn() // Spawns using options from `createFactory`
|
129 | const ipfsd2 = await factory.spawn({ type: 'go' }) // Spawns using options from `createFactory` but overrides `type` to spawn a `go` controller
|
130 |
|
131 | console.log(await ipfsd1.api.id())
|
132 | console.log(await ipfsd2.api.id())
|
133 |
|
134 | await factory.clean() // Clean all the controllers created by the factory calling `stop` on all of them.
|
135 | ```
|
136 |
|
137 | **Spawn an IPFS daemon from the Browser using the provided remote endpoint**
|
138 |
|
139 | ```js
|
140 | // Start a remote disposable node, and get access to the api
|
141 | // print the node id, and stop the temporary daemon
|
142 |
|
143 | const Ctl = require('ipfsd-ctl')
|
144 |
|
145 | const port = 9090
|
146 | const server = Ctl.createServer(port, {
|
147 | ipfsModule,
|
148 | ipfsHttpModule
|
149 | },
|
150 | {
|
151 | js: {
|
152 | ipfsBin: ipfsModule.path()
|
153 | },
|
154 | go: {
|
155 | ipfsBin: goIpfsModule.path()
|
156 | },
|
157 | })
|
158 | const factory = Ctl.createFactory({
|
159 | ipfsHttpModule,
|
160 | remote: true,
|
161 | endpoint: `http://localhost:${port}` // or you can set process.env.IPFSD_CTL_SERVER to http://localhost:9090
|
162 | })
|
163 |
|
164 | await server.start()
|
165 | const ipfsd = await factory.spawn()
|
166 | const id = await ipfsd.api.id()
|
167 |
|
168 | console.log(id)
|
169 |
|
170 | await ipfsd.stop()
|
171 | await server.stop()
|
172 | ```
|
173 |
|
174 | ## Disposable vs non Disposable nodes
|
175 |
|
176 | `ipfsd-ctl` can spawn `disposable` and `non-disposable` nodes.
|
177 |
|
178 | - `disposable`- Disposable nodes are useful for tests or other temporary use cases, by default they create a temporary repo and automatically initialise and start the node, plus they cleanup everything when stopped.
|
179 | - `non-disposable` - Non disposable nodes will by default attach to any nodes running on the default or the supplied repo. Requires the user to initialize and start the node, as well as stop and cleanup afterwards.
|
180 |
|
181 | ## API
|
182 |
|
183 | ### `createFactory([options], [overrides])`
|
184 |
|
185 | Creates a factory that can spawn multiple controllers and pre-define options for them.
|
186 |
|
187 | - `options` **[ControllerOptions](#controlleroptions)** Controllers options.
|
188 | - `overrides` **[ControllerOptionsOverrides](#controlleroptionsoverrides)** Pre-defined options overrides per controller type.
|
189 |
|
190 | Returns a **[Factory](#factory)**
|
191 |
|
192 | ### `createController([options])`
|
193 |
|
194 | Creates a controller.
|
195 |
|
196 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
197 |
|
198 | Returns **Promise<[Controller](#controller)>**
|
199 |
|
200 | ### `createServer([options])`
|
201 |
|
202 | Create an Endpoint Server. This server is used by a client node to control a remote node. Example: Spawning a go-ipfs node from a browser.
|
203 |
|
204 | - `options` **\[Object]** Factory options. Defaults to: `{ port: 43134 }`
|
205 | - `port` **number** Port to start the server on.
|
206 |
|
207 | Returns a **Server**
|
208 |
|
209 | ### Factory
|
210 |
|
211 | #### `controllers`
|
212 |
|
213 | **Controller\[]** List of all the controllers spawned.
|
214 |
|
215 | #### `tmpDir()`
|
216 |
|
217 | Create a temporary repo to create controllers manually.
|
218 |
|
219 | Returns **Promise\<String>** - Path to the repo.
|
220 |
|
221 | #### `spawn([options])`
|
222 |
|
223 | Creates a controller for a IPFS node.
|
224 |
|
225 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
226 |
|
227 | Returns **Promise<[Controller](#controller)>**
|
228 |
|
229 | #### `clean()`
|
230 |
|
231 | Cleans all controllers spawned.
|
232 |
|
233 | Returns **Promise<[Factory](#factory)>**
|
234 |
|
235 | ### Controller
|
236 |
|
237 | Class controller for a IPFS node.
|
238 |
|
239 | #### `new Controller(options)`
|
240 |
|
241 | - `options` **[ControllerOptions](#controlleroptions)**
|
242 |
|
243 | #### `path`
|
244 |
|
245 | **String** Repo path.
|
246 |
|
247 | #### `exec`
|
248 |
|
249 | **String** Executable path.
|
250 |
|
251 | #### `env`
|
252 |
|
253 | **Object** ENV object.
|
254 |
|
255 | #### `initialized`
|
256 |
|
257 | **Boolean** Flag with the current init state.
|
258 |
|
259 | #### `started`
|
260 |
|
261 | **Boolean** Flag with the current start state.
|
262 |
|
263 | #### `clean`
|
264 |
|
265 | **Boolean** Flag with the current clean state.
|
266 |
|
267 | #### `apiAddr`
|
268 |
|
269 | **Multiaddr** API address
|
270 |
|
271 | #### `gatewayAddr`
|
272 |
|
273 | **Multiaddr** Gateway address
|
274 |
|
275 | #### `api`
|
276 |
|
277 | **Object** IPFS core interface
|
278 |
|
279 | #### `init([initOptions])`
|
280 |
|
281 | Initialises controlled node
|
282 |
|
283 | - `initOptions` **\[Object]** IPFS init options <https://github.com/ipfs/js-ipfs/blob/master/README.md#optionsinit>
|
284 |
|
285 | Returns **Promise<[Controller](#controller)>**
|
286 |
|
287 | #### `start()`
|
288 |
|
289 | Starts controlled node.
|
290 |
|
291 | Returns **Promise\<IPFS>**
|
292 |
|
293 | #### `stop()`
|
294 |
|
295 | Stops controlled node.
|
296 |
|
297 | Returns **Promise<[Controller](#controller)>**
|
298 |
|
299 | #### `cleanup()`
|
300 |
|
301 | Cleans controlled node, a disposable controller calls this automatically.
|
302 |
|
303 | Returns **Promise<[Controller](#controller)>**
|
304 |
|
305 | #### `pid()`
|
306 |
|
307 | Get the pid of the controlled node process if aplicable.
|
308 |
|
309 | Returns **Promise\<number>**
|
310 |
|
311 | #### `version()`
|
312 |
|
313 | Get the version of the controlled node.
|
314 |
|
315 | Returns **Promise\<string>**
|
316 |
|
317 | ### ControllerOptionsOverrides
|
318 |
|
319 | Type: \[Object]
|
320 |
|
321 | #### Properties
|
322 |
|
323 | - `js` **\[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **JS** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
324 | - `go` **\[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Go** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
325 | - `proc` **\[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Proc** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
326 |
|
327 | ### ControllerOptions
|
328 |
|
329 | Type: \[Object]
|
330 |
|
331 | #### Properties
|
332 |
|
333 | - `test` **\[boolean]** Flag to activate custom config for tests.
|
334 | - `remote` **\[boolean]** Use remote endpoint to spawn the nodes. Defaults to `true` when not in node.
|
335 | - `endpoint` **\[string]** Endpoint URL to manage remote Controllers. (Defaults: '<http://localhost:43134>').
|
336 | - `disposable` **\[boolean]** A new repo is created and initialized for each invocation, as well as cleaned up automatically once the process exits.
|
337 | - `type` **\[string]** The daemon type, see below the options:
|
338 | - go - spawn go-ipfs daemon
|
339 | - js - spawn js-ipfs daemon
|
340 | - proc - spawn in-process js-ipfs node
|
341 | - `env` **\[Object]** Additional environment variables, passed to executing shell. Only applies for Daemon controllers.
|
342 | - `args` **\[Array]** Custom cli args.
|
343 | - `ipfsHttpModule` **\[Object]** Reference to a IPFS HTTP Client object.
|
344 | - `ipfsModule` **\[Object]** Reference to a IPFS API object.
|
345 | - `ipfsBin` **\[string]** Path to a IPFS exectutable.
|
346 | - `ipfsOptions` **\[IpfsOptions]** Options for the IPFS instance same as <https://github.com/ipfs/js-ipfs#ipfs-constructor>. `proc` nodes receive these options as is, daemon nodes translate the options as far as possible to cli arguments.
|
347 | - `forceKill` **\[boolean]** - Whether to use SIGKILL to quit a daemon that does not stop after `.stop()` is called. (default `true`)
|
348 | - `forceKillTimeout` **\[Number]** - How long to wait before force killing a daemon in ms. (default `5000`)
|
349 |
|
350 | ## ipfsd-ctl environment variables
|
351 |
|
352 | In additional to the API described in previous sections, `ipfsd-ctl` also supports several environment variables. This are often very useful when running in different environments, such as CI or when doing integration/interop testing.
|
353 |
|
354 | *Environment variables precedence order is as follows. Top to bottom, top entry has highest precedence:*
|
355 |
|
356 | - command line options/method arguments
|
357 | - env variables
|
358 | - default values
|
359 |
|
360 | Meaning that, environment variables override defaults in the configuration file but are superseded by options to `df.spawn({...})`
|
361 |
|
362 | #### IPFS\_JS\_EXEC and IPFS\_GO\_EXEC
|
363 |
|
364 | An alternative way of specifying the executable path for the `js-ipfs` or `go-ipfs` executable, respectively.
|
365 |
|
366 | ## Contribute
|
367 |
|
368 | Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfsd-ctl/issues)!
|
369 |
|
370 | This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md).
|
371 |
|
372 | [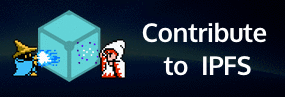](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md)
|
373 |
|
374 | ## License
|
375 |
|
376 | Licensed under either of
|
377 |
|
378 | - Apache 2.0, ([LICENSE-APACHE](LICENSE-APACHE) / <http://www.apache.org/licenses/LICENSE-2.0>)
|
379 | - MIT ([LICENSE-MIT](LICENSE-MIT) / <http://opensource.org/licenses/MIT>)
|
380 |
|
381 | ## Contribute
|
382 |
|
383 | Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfs-unixfs-importer/issues)!
|
384 |
|
385 | This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md).
|
386 |
|
387 | [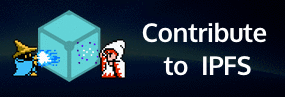](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md)
|