1 | # ipfsd-ctl, the IPFS Factory
|
2 |
|
3 | [](http://protocol.ai)
|
4 | [](http://ipfs.io/)
|
5 | [](http://webchat.freenode.net/?channels=%23ipfs)
|
6 | [](https://travis-ci.com/ipfs/js-ipfsd-ctl)
|
7 | [](https://codecov.io/gh/ipfs/js-ipfs-multipart)
|
8 | [](https://david-dm.org/ipfs/js-ipfsd-ctl)
|
9 | [](https://github.com/feross/standard)
|
10 | [](https://bundlephobia.com/result?p=ipfsd-ctl)
|
11 | > Spawn IPFS daemons using JavaScript!
|
12 |
|
13 | ## Lead Maintainer
|
14 |
|
15 | [Hugo Dias](https://github.com/hugomrdias)
|
16 |
|
17 | ## Notice
|
18 | Version 1.0.0 changed a bit the api and the options methods take so please read the documentation below.
|
19 |
|
20 | ## Table of Contents
|
21 |
|
22 | - [Install](#install)
|
23 | - [Usage](#usage)
|
24 | - [API](#api)
|
25 | - [Packaging](#packaging)
|
26 | - [Contribute](#contribute)
|
27 | - [License](#license)
|
28 |
|
29 | ## Install
|
30 |
|
31 | ```sh
|
32 | npm install --save ipfsd-ctl
|
33 | ```
|
34 |
|
35 | Please ensure your project also has dependencies on `ipfs`, `ipfs-http-client` and `go-ipfs-dep`.
|
36 |
|
37 | ```sh
|
38 | npm install --save ipfs
|
39 | npm install --save ipfs-http-client
|
40 | npm install --save go-ipfs-dep
|
41 | ```
|
42 |
|
43 | If you are only going to use the `go` implementation of IPFS, you can skip installing the `js` implementation and vice versa, though both will require the `ipfs-http-client` module.
|
44 |
|
45 | If you are only using the `proc` type in-process IPFS node, you can skip installing `go-ipfs-dep` and `ipfs-http-client`.
|
46 |
|
47 | ## Usage
|
48 |
|
49 | ### Spawning a single IPFS controller: `createController`
|
50 |
|
51 | This is a shorthand for simpler use cases where factory is not needed.
|
52 |
|
53 | ```js
|
54 | // No need to create a factory when only a single controller is needed.
|
55 | // Use createController to spawn it instead.
|
56 | const Ctl = require('ipfsd-ctl')
|
57 | const ipfsd = await Ctl.createController()
|
58 | const id = await ipfsd.api.id()
|
59 |
|
60 | console.log(id)
|
61 |
|
62 | await ipfsd.stop()
|
63 | ```
|
64 |
|
65 | ### Manage multiple IPFS controllers: `createFactory`
|
66 |
|
67 | Use a factory to spawn multiple controllers based on some common template.
|
68 |
|
69 | **Spawn an IPFS daemon from Node.js**
|
70 |
|
71 | ```js
|
72 | // Create a factory to spawn two test disposable controllers, get access to an IPFS api
|
73 | // print node ids and clean all the controllers from the factory.
|
74 | const Ctl = require('ipfsd-ctl')
|
75 |
|
76 | const factory = Ctl.createFactory({ type: 'js', test: true, disposable: true })
|
77 | const ipfsd1 = await factory.spawn() // Spawns using options from `createFactory`
|
78 | const ipfsd2 = await factory.spawn({ type: 'go' }) // Spawns using options from `createFactory` but overrides `type` to spawn a `go` controller
|
79 |
|
80 | console.log(await ipfsd1.api.id())
|
81 | console.log(await ipfsd2.api.id())
|
82 |
|
83 | await factory.clean() // Clean all the controllers created by the factory calling `stop` on all of them.
|
84 | ```
|
85 |
|
86 | **Spawn an IPFS daemon from the Browser using the provided remote endpoint**
|
87 |
|
88 | ```js
|
89 | // Start a remote disposable node, and get access to the api
|
90 | // print the node id, and stop the temporary daemon
|
91 |
|
92 | const Ctl = require('ipfsd-ctl')
|
93 |
|
94 | const port = 9090
|
95 | const server = Ctl.createServer(port)
|
96 | const factory = Ctl.createFactory({ remote: true, endpoint: `http://localhost:${port}` })
|
97 |
|
98 | await server.start()
|
99 | const ipfsd = await factory.spawn()
|
100 | const id = await ipfsd.api.id()
|
101 |
|
102 | console.log(id)
|
103 |
|
104 | await ipfsd.stop()
|
105 | await server.stop()
|
106 | ```
|
107 |
|
108 | ## Disposable vs non Disposable nodes
|
109 |
|
110 | `ipfsd-ctl` can spawn `disposable` and `non-disposable` nodes.
|
111 |
|
112 | - `disposable`- Disposable nodes are useful for tests or other temporary use cases, by default they create a temporary repo and automatically initialise and start the node, plus they cleanup everything when stopped.
|
113 | - `non-disposable` - Non disposable nodes will by default attach to any nodes running on the default or the supplied repo. Requires the user to initialize and start the node, as well as stop and cleanup afterwards.
|
114 |
|
115 | ## API
|
116 |
|
117 | ### `createFactory([options], [overrides])`
|
118 | Creates a factory that can spawn multiple controllers and pre-define options for them.
|
119 |
|
120 | - `options` **[ControllerOptions](#controlleroptions)** Controllers options.
|
121 | - `overrides` **[ControllerOptionsOverrides](#controlleroptionsoverrides)** Pre-defined options overrides per controller type.
|
122 |
|
123 | Returns a **[Factory](#factory)**
|
124 |
|
125 | ### `createController([options])`
|
126 | Creates a controller.
|
127 |
|
128 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
129 |
|
130 | Returns **Promise<[Controller](#controller)>**
|
131 |
|
132 | ### `createServer([options])`
|
133 | Create an Endpoint Server. This server is used by a client node to control a remote node. Example: Spawning a go-ipfs node from a browser.
|
134 |
|
135 | - `options` **[Object]** Factory options. Defaults to: `{ port: 43134 }`
|
136 | - `port` **number** Port to start the server on.
|
137 |
|
138 | Returns a **Server**
|
139 |
|
140 | ### Factory
|
141 |
|
142 | #### `controllers`
|
143 | **Controller[]** List of all the controllers spawned.
|
144 |
|
145 | #### `tmpDir()`
|
146 | Create a temporary repo to create controllers manually.
|
147 |
|
148 | Returns **Promise<String>** - Path to the repo.
|
149 |
|
150 | #### `spawn([options])`
|
151 | Creates a controller for a IPFS node.
|
152 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
153 |
|
154 | Returns **Promise<[Controller](#controller)>**
|
155 |
|
156 | #### `clean()`
|
157 | Cleans all controllers spawned.
|
158 |
|
159 | Returns **Promise<[Factory](#factory)>**
|
160 |
|
161 | ### Controller
|
162 | Class controller for a IPFS node.
|
163 |
|
164 | #### `new Controller(options)`
|
165 |
|
166 | - `options` **[ControllerOptions](#controlleroptions)**
|
167 |
|
168 | #### `path`
|
169 | **String** Repo path.
|
170 |
|
171 | #### `exec`
|
172 | **String** Executable path.
|
173 |
|
174 | #### `env`
|
175 | **Object** ENV object.
|
176 |
|
177 | #### `initalized`
|
178 | **Boolean** Flag with the current init state.
|
179 |
|
180 | #### `started`
|
181 | **Boolean** Flag with the current start state.
|
182 |
|
183 | #### `clean`
|
184 | **Boolean** Flag with the current clean state.
|
185 |
|
186 | #### `apiAddr`
|
187 | **Multiaddr** API address
|
188 |
|
189 | #### `gatewayAddr`
|
190 | **Multiaddr** Gateway address
|
191 |
|
192 | #### `api`
|
193 | **Object** IPFS core interface
|
194 |
|
195 | #### `init([initOptions])`
|
196 | Initialises controlled node
|
197 |
|
198 | - `initOptions` **[Object]** IPFS init options https://github.com/ipfs/js-ipfs/blob/master/README.md#optionsinit
|
199 |
|
200 | Returns **Promise<[Controller](#controller)>**
|
201 |
|
202 | #### `start()`
|
203 | Starts controlled node.
|
204 |
|
205 | Returns **Promise<IPFS>**
|
206 |
|
207 | #### `stop()`
|
208 | Stops controlled node.
|
209 |
|
210 | Returns **Promise<[Controller](#controller)>**
|
211 |
|
212 | #### `cleanup()`
|
213 | Cleans controlled node, a disposable controller calls this automatically.
|
214 |
|
215 | Returns **Promise<[Controller](#controller)>**
|
216 |
|
217 |
|
218 | #### `pid()`
|
219 | Get the pid of the controlled node process if aplicable.
|
220 |
|
221 | Returns **Promise<number>**
|
222 |
|
223 |
|
224 | #### `version()`
|
225 | Get the version of the controlled node.
|
226 |
|
227 | Returns **Promise<string>**
|
228 |
|
229 |
|
230 | ### ControllerOptionsOverrides
|
231 |
|
232 | Type: [Object]
|
233 |
|
234 | #### Properties
|
235 | - `js` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **JS** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
236 | - `go` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Go** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
237 | - `proc` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Proc** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
238 |
|
239 |
|
240 | ### ControllerOptions
|
241 |
|
242 | Type: [Object]
|
243 |
|
244 | #### Properties
|
245 | - `test` **[boolean]** Flag to activate custom config for tests.
|
246 | - `remote` **[boolean]** Use remote endpoint to spawn the nodes. Defaults to `true` when not in node.
|
247 | - `endpoint` **[string]** Endpoint URL to manage remote Controllers. (Defaults: 'http://localhost:43134').
|
248 | - `disposable` **[boolean]** A new repo is created and initialized for each invocation, as well as cleaned up automatically once the process exits.
|
249 | - `type` **[string]** The daemon type, see below the options:
|
250 | - go - spawn go-ipfs daemon
|
251 | - js - spawn js-ipfs daemon
|
252 | - proc - spawn in-process js-ipfs node
|
253 | - `env` **[Object]** Additional environment variables, passed to executing shell. Only applies for Daemon controllers.
|
254 | - `args` **[Array]** Custom cli args.
|
255 | - `ipfsHttpModule` **[Object]** Define the `ipfs-http-client` package to be used by ctl. Both `ref` and `path` should be specified to make sure all node types (daemon, remote daemon, browser in process node, etc) use the correct version.
|
256 | - `ipfsHttpModule.ref` **[Object]** Reference to a IPFS HTTP Client object. (defaults to the local require(`ipfs-http-client`))
|
257 | - `ipfsHttpModule.path` **[string]** Path to a IPFS HTTP Client to be required. (defaults to the local require.resolve('ipfs-http-client'))
|
258 | - `ipfsModule` **[Object]** Define the `ipfs` package to be used by ctl. Both `ref` and `path` should be specified to make sure all node types (daemon, remote daemon, browser in process node, etc) use the correct version.
|
259 | - `ipfsModule.ref` **[Object]** Reference to a IPFS API object. (defaults to the local require(`ipfs`))
|
260 | - `ipfsModule.path` **[string]** Path to a IPFS API implementation to be required. (defaults to the local require.resolve('ipfs'))
|
261 | - `ipfsBin` **[string]** Path to a IPFS exectutable . (defaults to the local 'js-ipfs/src/bin/cli.js')
|
262 | - `ipfsOptions` **[IpfsOptions]** Options for the IPFS instance same as https://github.com/ipfs/js-ipfs#ipfs-constructor. `proc` nodes receive these options as is, daemon nodes translate the options as far as possible to cli arguments.
|
263 | - `forceKill` **[boolean]** - Whether to use SIGKILL to quit a daemon that does not stop after `.stop()` is called. (default `true`)
|
264 | - `forceKillTimeout` **[Number]** - How long to wait before force killing a daemon in ms. (default `5000`)
|
265 |
|
266 |
|
267 | ## ipfsd-ctl environment variables
|
268 |
|
269 | In additional to the API described in previous sections, `ipfsd-ctl` also supports several environment variables. This are often very useful when running in different environments, such as CI or when doing integration/interop testing.
|
270 |
|
271 | _Environment variables precedence order is as follows. Top to bottom, top entry has highest precedence:_
|
272 |
|
273 | - command line options/method arguments
|
274 | - env variables
|
275 | - default values
|
276 |
|
277 | Meaning that, environment variables override defaults in the configuration file but are superseded by options to `df.spawn({...})`
|
278 |
|
279 | #### IPFS_JS_EXEC and IPFS_GO_EXEC
|
280 |
|
281 | An alternative way of specifying the executable path for the `js-ipfs` or `go-ipfs` executable, respectively.
|
282 |
|
283 |
|
284 | ## Contribute
|
285 |
|
286 | Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfsd-ctl/issues)!
|
287 |
|
288 | This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md).
|
289 |
|
290 | [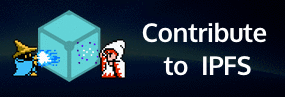](https://github.com/ipfs/community/blob/master/contributing.md)
|
291 |
|
292 | ## License
|
293 |
|
294 | [MIT](LICENSE)
|