1 | # ipfsd-ctl, the IPFS Factory
|
2 |
|
3 | [](http://protocol.ai)
|
4 | [](http://ipfs.io/)
|
5 | [](http://webchat.freenode.net/?channels=%23ipfs)
|
6 | [](https://travis-ci.com/ipfs/js-ipfsd-ctl)
|
7 | [](https://codecov.io/gh/ipfs/js-ipfs-multipart)
|
8 | [](https://david-dm.org/ipfs/js-ipfsd-ctl)
|
9 | [](https://github.com/feross/standard)
|
10 | [](https://bundlephobia.com/result?p=ipfsd-ctl)
|
11 | > Spawn IPFS daemons using JavaScript!
|
12 |
|
13 | ## Lead Maintainer
|
14 |
|
15 | [Hugo Dias](https://github.com/hugomrdias)
|
16 |
|
17 | ## Notice
|
18 | Version 1.0.0 changed a bit the api and the options methods take so please read the documentation below.
|
19 |
|
20 | ## Table of Contents
|
21 |
|
22 | - [Install](#install)
|
23 | - [Usage](#usage)
|
24 | - [API](#api)
|
25 | - [Packaging](#packaging)
|
26 | - [Contribute](#contribute)
|
27 | - [License](#license)
|
28 |
|
29 | ## Install
|
30 |
|
31 | ```sh
|
32 | npm install --save ipfsd-ctl
|
33 | ```
|
34 |
|
35 | Please ensure your project also has dependencies on `ipfs`, `ipfs-http-client` and `go-ipfs`.
|
36 |
|
37 | ```sh
|
38 | npm install --save ipfs
|
39 | npm install --save ipfs-http-client
|
40 | npm install --save go-ipfs
|
41 | ```
|
42 |
|
43 | If you are only going to use the `go` implementation of IPFS, you can skip installing the `js` implementation and vice versa, though both will require the `ipfs-http-client` module.
|
44 |
|
45 | If you are only using the `proc` type in-process IPFS node, you can skip installing `go-ipfs` and `ipfs-http-client`.
|
46 |
|
47 | > You also need to explicitly defined the options `ipfsBin`, `ipfsModule` and `ipfsHttpModule` according to your needs. Check [ControllerOptions](#controlleroptions) and [ControllerOptionsOverrides](#controlleroptionsoverrides) for more information.
|
48 |
|
49 | ## Usage
|
50 |
|
51 | ### Spawning a single IPFS controller: `createController`
|
52 |
|
53 | This is a shorthand for simpler use cases where factory is not needed.
|
54 |
|
55 | ```js
|
56 | // No need to create a factory when only a single controller is needed.
|
57 | // Use createController to spawn it instead.
|
58 | const Ctl = require('ipfsd-ctl')
|
59 | const ipfsd = await Ctl.createController({
|
60 | ipfsHttpModule: require('ipfs-http-client'),
|
61 | ipfsBin: require('go-ipfs').path()
|
62 | })
|
63 | const id = await ipfsd.api.id()
|
64 |
|
65 | console.log(id)
|
66 |
|
67 | await ipfsd.stop()
|
68 | ```
|
69 |
|
70 | ### Manage multiple IPFS controllers: `createFactory`
|
71 |
|
72 | Use a factory to spawn multiple controllers based on some common template.
|
73 |
|
74 | **Spawn an IPFS daemon from Node.js**
|
75 |
|
76 | ```js
|
77 | // Create a factory to spawn two test disposable controllers, get access to an IPFS api
|
78 | // print node ids and clean all the controllers from the factory.
|
79 | const Ctl = require('ipfsd-ctl')
|
80 |
|
81 | const factory = Ctl.createFactory(
|
82 | {
|
83 | type: 'js',
|
84 | test: true,
|
85 | disposable: true,
|
86 | ipfsHttpModule: require('ipfs-http-client'),
|
87 | ipfsModule: require('ipfs') // only if you gonna spawn 'proc' controllers
|
88 | },
|
89 | { // overrides per type
|
90 | js: {
|
91 | ipfsBin: 'path/js/ipfs/bin'
|
92 | },
|
93 | go: {
|
94 | ipfsBin: 'path/go/ipfs/bin'
|
95 | }
|
96 | }
|
97 | )
|
98 | const ipfsd1 = await factory.spawn() // Spawns using options from `createFactory`
|
99 | const ipfsd2 = await factory.spawn({ type: 'go' }) // Spawns using options from `createFactory` but overrides `type` to spawn a `go` controller
|
100 |
|
101 | console.log(await ipfsd1.api.id())
|
102 | console.log(await ipfsd2.api.id())
|
103 |
|
104 | await factory.clean() // Clean all the controllers created by the factory calling `stop` on all of them.
|
105 | ```
|
106 |
|
107 | **Spawn an IPFS daemon from the Browser using the provided remote endpoint**
|
108 |
|
109 | ```js
|
110 | // Start a remote disposable node, and get access to the api
|
111 | // print the node id, and stop the temporary daemon
|
112 |
|
113 | const Ctl = require('ipfsd-ctl')
|
114 |
|
115 | const port = 9090
|
116 | const server = Ctl.createServer(port, {
|
117 | ipfsModule: require('ipfs'),
|
118 | ipfsHttpModule: require('ipfs-http-client')
|
119 | },
|
120 | {
|
121 | js: {
|
122 | ipfsBin: 'path/js/ipfs/bin'
|
123 | },
|
124 | go: {
|
125 | ipfsBin: 'path/go/ipfs/bin'
|
126 | },
|
127 | })
|
128 | const factory = Ctl.createFactory({
|
129 | ipfsHttpModule: require('ipfs-http-client'),
|
130 | remote: true,
|
131 | endpoint: `http://localhost:${port}` // or you can set process.env.IPFSD_CTL_SERVER to http://localhost:9090
|
132 | })
|
133 |
|
134 | await server.start()
|
135 | const ipfsd = await factory.spawn()
|
136 | const id = await ipfsd.api.id()
|
137 |
|
138 | console.log(id)
|
139 |
|
140 | await ipfsd.stop()
|
141 | await server.stop()
|
142 | ```
|
143 |
|
144 | ## Disposable vs non Disposable nodes
|
145 |
|
146 | `ipfsd-ctl` can spawn `disposable` and `non-disposable` nodes.
|
147 |
|
148 | - `disposable`- Disposable nodes are useful for tests or other temporary use cases, by default they create a temporary repo and automatically initialise and start the node, plus they cleanup everything when stopped.
|
149 | - `non-disposable` - Non disposable nodes will by default attach to any nodes running on the default or the supplied repo. Requires the user to initialize and start the node, as well as stop and cleanup afterwards.
|
150 |
|
151 | ## API
|
152 |
|
153 | ### `createFactory([options], [overrides])`
|
154 | Creates a factory that can spawn multiple controllers and pre-define options for them.
|
155 |
|
156 | - `options` **[ControllerOptions](#controlleroptions)** Controllers options.
|
157 | - `overrides` **[ControllerOptionsOverrides](#controlleroptionsoverrides)** Pre-defined options overrides per controller type.
|
158 |
|
159 | Returns a **[Factory](#factory)**
|
160 |
|
161 | ### `createController([options])`
|
162 | Creates a controller.
|
163 |
|
164 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
165 |
|
166 | Returns **Promise<[Controller](#controller)>**
|
167 |
|
168 | ### `createServer([options])`
|
169 | Create an Endpoint Server. This server is used by a client node to control a remote node. Example: Spawning a go-ipfs node from a browser.
|
170 |
|
171 | - `options` **[Object]** Factory options. Defaults to: `{ port: 43134 }`
|
172 | - `port` **number** Port to start the server on.
|
173 |
|
174 | Returns a **Server**
|
175 |
|
176 | ### Factory
|
177 |
|
178 | #### `controllers`
|
179 | **Controller[]** List of all the controllers spawned.
|
180 |
|
181 | #### `tmpDir()`
|
182 | Create a temporary repo to create controllers manually.
|
183 |
|
184 | Returns **Promise<String>** - Path to the repo.
|
185 |
|
186 | #### `spawn([options])`
|
187 | Creates a controller for a IPFS node.
|
188 | - `options` **[ControllerOptions](#controlleroptions)** Factory options.
|
189 |
|
190 | Returns **Promise<[Controller](#controller)>**
|
191 |
|
192 | #### `clean()`
|
193 | Cleans all controllers spawned.
|
194 |
|
195 | Returns **Promise<[Factory](#factory)>**
|
196 |
|
197 | ### Controller
|
198 | Class controller for a IPFS node.
|
199 |
|
200 | #### `new Controller(options)`
|
201 |
|
202 | - `options` **[ControllerOptions](#controlleroptions)**
|
203 |
|
204 | #### `path`
|
205 | **String** Repo path.
|
206 |
|
207 | #### `exec`
|
208 | **String** Executable path.
|
209 |
|
210 | #### `env`
|
211 | **Object** ENV object.
|
212 |
|
213 | #### `initialized`
|
214 | **Boolean** Flag with the current init state.
|
215 |
|
216 | #### `started`
|
217 | **Boolean** Flag with the current start state.
|
218 |
|
219 | #### `clean`
|
220 | **Boolean** Flag with the current clean state.
|
221 |
|
222 | #### `apiAddr`
|
223 | **Multiaddr** API address
|
224 |
|
225 | #### `gatewayAddr`
|
226 | **Multiaddr** Gateway address
|
227 |
|
228 | #### `api`
|
229 | **Object** IPFS core interface
|
230 |
|
231 | #### `init([initOptions])`
|
232 | Initialises controlled node
|
233 |
|
234 | - `initOptions` **[Object]** IPFS init options https://github.com/ipfs/js-ipfs/blob/master/README.md#optionsinit
|
235 |
|
236 | Returns **Promise<[Controller](#controller)>**
|
237 |
|
238 | #### `start()`
|
239 | Starts controlled node.
|
240 |
|
241 | Returns **Promise<IPFS>**
|
242 |
|
243 | #### `stop()`
|
244 | Stops controlled node.
|
245 |
|
246 | Returns **Promise<[Controller](#controller)>**
|
247 |
|
248 | #### `cleanup()`
|
249 | Cleans controlled node, a disposable controller calls this automatically.
|
250 |
|
251 | Returns **Promise<[Controller](#controller)>**
|
252 |
|
253 |
|
254 | #### `pid()`
|
255 | Get the pid of the controlled node process if aplicable.
|
256 |
|
257 | Returns **Promise<number>**
|
258 |
|
259 |
|
260 | #### `version()`
|
261 | Get the version of the controlled node.
|
262 |
|
263 | Returns **Promise<string>**
|
264 |
|
265 |
|
266 | ### ControllerOptionsOverrides
|
267 |
|
268 | Type: [Object]
|
269 |
|
270 | #### Properties
|
271 | - `js` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **JS** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
272 | - `go` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Go** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
273 | - `proc` **[[ControllerOptions](#controlleroptions)]** Pre-defined defaults options for **Proc** controllers these are deep merged with options passed to `Factory.spawn(options)`.
|
274 |
|
275 |
|
276 | ### ControllerOptions
|
277 |
|
278 | Type: [Object]
|
279 |
|
280 | #### Properties
|
281 | - `test` **[boolean]** Flag to activate custom config for tests.
|
282 | - `remote` **[boolean]** Use remote endpoint to spawn the nodes. Defaults to `true` when not in node.
|
283 | - `endpoint` **[string]** Endpoint URL to manage remote Controllers. (Defaults: 'http://localhost:43134').
|
284 | - `disposable` **[boolean]** A new repo is created and initialized for each invocation, as well as cleaned up automatically once the process exits.
|
285 | - `type` **[string]** The daemon type, see below the options:
|
286 | - go - spawn go-ipfs daemon
|
287 | - js - spawn js-ipfs daemon
|
288 | - proc - spawn in-process js-ipfs node
|
289 | - `env` **[Object]** Additional environment variables, passed to executing shell. Only applies for Daemon controllers.
|
290 | - `args` **[Array]** Custom cli args.
|
291 | - `ipfsHttpModule` **[Object]** Reference to a IPFS HTTP Client object.
|
292 | - `ipfsModule` **[Object]** Reference to a IPFS API object.
|
293 | - `ipfsBin` **[string]** Path to a IPFS exectutable.
|
294 | - `ipfsOptions` **[IpfsOptions]** Options for the IPFS instance same as https://github.com/ipfs/js-ipfs#ipfs-constructor. `proc` nodes receive these options as is, daemon nodes translate the options as far as possible to cli arguments.
|
295 | - `forceKill` **[boolean]** - Whether to use SIGKILL to quit a daemon that does not stop after `.stop()` is called. (default `true`)
|
296 | - `forceKillTimeout` **[Number]** - How long to wait before force killing a daemon in ms. (default `5000`)
|
297 |
|
298 |
|
299 | ## ipfsd-ctl environment variables
|
300 |
|
301 | In additional to the API described in previous sections, `ipfsd-ctl` also supports several environment variables. This are often very useful when running in different environments, such as CI or when doing integration/interop testing.
|
302 |
|
303 | _Environment variables precedence order is as follows. Top to bottom, top entry has highest precedence:_
|
304 |
|
305 | - command line options/method arguments
|
306 | - env variables
|
307 | - default values
|
308 |
|
309 | Meaning that, environment variables override defaults in the configuration file but are superseded by options to `df.spawn({...})`
|
310 |
|
311 | #### IPFS_JS_EXEC and IPFS_GO_EXEC
|
312 |
|
313 | An alternative way of specifying the executable path for the `js-ipfs` or `go-ipfs` executable, respectively.
|
314 |
|
315 |
|
316 | ## Contribute
|
317 |
|
318 | Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfsd-ctl/issues)!
|
319 |
|
320 | This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md).
|
321 |
|
322 | [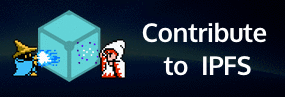](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md)
|
323 |
|
324 | ## License
|
325 |
|
326 | [MIT](LICENSE)
|