1 | # Maker.js
|
2 |
|
3 | Your compass and straightedge, in JavaScript.
|
4 |
|
5 | Create line drawings using familiar constructs from geometry and drafting. Initially designated for CNC and laser cutters, Maker.js can also help you programmatically draw shapes for any purpose. It runs in both Node.js and web browsers.
|
6 |
|
7 | 2D Export formats:
|
8 | [DXF](https://maker.js.org/docs/api/modules/makerjs.exporter.html#todxf),
|
9 | [SVG](https://maker.js.org/docs/api/modules/makerjs.exporter.html#tosvg),
|
10 | [PDF](https://maker.js.org/docs/api/modules/makerjs.exporter.html#topdf),
|
11 | [Jscad CAG object](https://maker.js.org/docs/api/modules/makerjs.exporter.html#tojscadcag)
|
12 |
|
13 | 3D Export formats:
|
14 | [Jscad Script](https://maker.js.org/docs/api/modules/makerjs.exporter.html#tojscadscript),
|
15 | [Jscad CSG object](https://maker.js.org/docs/api/modules/makerjs.exporter.html#tojscadcsg),
|
16 | [STL](https://maker.js.org/docs/api/modules/makerjs.exporter.html#tojscadstl)
|
17 |
|
18 | [Demos](https://maker.js.org/demos/) - [Documentation](http://maker.js.org/docs/)
|
19 |
|
20 | 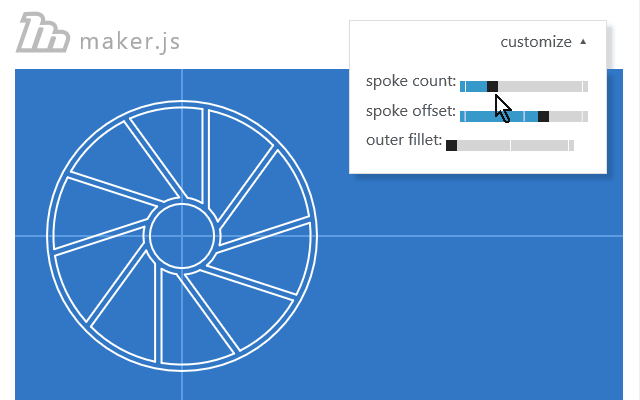
|
21 |
|
22 | ## Core concepts
|
23 |
|
24 | * [paths](https://maker.js.org/docs/basic-drawing/#Paths) - The primitive elements of a drawing are lines, arcs, and circles.
|
25 | * [models](https://maker.js.org/docs/basic-drawing/#Models) - Groups of paths to compose a shape.
|
26 | * [layers](https://maker.js.org/docs/advanced-drawing/#Layers) - Organization of models, such as by color or tool type.
|
27 | * [chains](https://maker.js.org/docs/working-with-chains/#content) - A series of lines and arcs that connect end-to-end continuously.
|
28 |
|
29 | Learn more in [the tutorial](https://maker.js.org/docs/basic-drawing/) or [API documentation](https://maker.js.org/docs/api/).
|
30 |
|
31 | ## Features
|
32 |
|
33 | * Drawings are a [simple JavaScript object](https://maker.js.org/docs/basic-drawing/#It%27s%20Just%20JSON) which can be serialized / deserialized conventionally with JSON. This also makes a drawing easy to [clone](https://maker.js.org/docs/intermediate-drawing/#Cloning).
|
34 |
|
35 | * Other people's Models can be required the Node.js way, [modified](https://maker.js.org/docs/intermediate-drawing/#Modifying%20models), and re-exported.
|
36 |
|
37 | * Models can be [scaled](https://maker.js.org/docs/intermediate-drawing/#Scaling), [distorted](https://maker.js.org/docs/intermediate-drawing/#Distorting), [measured](https://maker.js.org/docs/api/modules/makerjs.measure.html#modelextents), and [converted to different unit systems](https://maker.js.org/docs/basic-drawing/#Units).
|
38 |
|
39 | * Paths can be [distorted](https://maker.js.org/docs/api/modules/makerjs.path.html#distort).
|
40 |
|
41 | * Models can be [rotated](https://maker.js.org/docs/intermediate-drawing/#Rotating) or [mirrored](https://maker.js.org/docs/intermediate-drawing/#Mirroring).
|
42 |
|
43 | * Find [intersection points or intersection angles](https://maker.js.org/docs/intermediate-drawing/#Intersection) of paths.
|
44 |
|
45 | * [Traverse a model tree](https://maker.js.org/docs/model-trees/#content) to reason over its children.
|
46 |
|
47 | * Detect [chains](https://maker.js.org/docs/api/modules/makerjs.model.html#findchains) formed by paths connecting end to end.
|
48 |
|
49 | * Get the [points along a path](https://maker.js.org/docs/api/modules/makerjs.path.html#topoints) or along a [chain of paths](https://maker.js.org/docs/api/modules/makerjs.chain.html#topoints).
|
50 |
|
51 | * Easily add a curvature at the joint between any 2 paths, using a [traditional or a dogbone fillet](https://maker.js.org/docs/intermediate-drawing/#Fillets).
|
52 |
|
53 | * [Combine models](https://maker.js.org/docs/advanced-drawing/#Combining%20with%20Boolean%20operations) with boolean operations to get unions, intersections, or punches.
|
54 |
|
55 | * [Expand paths](https://maker.js.org/docs/advanced-drawing/#Expanding%20paths) to simulate a stroke thickness, with the option to bevel joints.
|
56 |
|
57 | * [Outline model](https://maker.js.org/docs/advanced-drawing/#Outlining%20a%20model) to create a surrounding outline, with the option to bevel joints.
|
58 |
|
59 | * Layout clones into [rows](http://maker.js.org/docs/api/modules/makerjs.layout.html#clonetorow), [columns](https://maker.js.org/docs/api/modules/makerjs.layout.html#clonetocolumn), [grids](https://maker.js.org/docs/api/modules/makerjs.layout.html#clonetogrid), [bricks](https://maker.js.org/docs/api/modules/makerjs.layout.html#clonetobrick), or [honeycombs](https://maker.js.org/docs/api/modules/makerjs.layout.html#clonetohoneycomb)
|
60 |
|
61 | #### Built-in models
|
62 |
|
63 | * [Belt](https://maker.js.org/playground/?script=Belt)
|
64 | * [Bezier Curve](https://maker.js.org/playground/?script=BezierCurve)
|
65 | * [Bolt Circle](https://maker.js.org/playground/?script=BoltCircle)
|
66 | * [Bolt Rectangle](https://maker.js.org/playground/?script=BoltRectangle)
|
67 | * [Connect the dots](https://maker.js.org/playground/?script=ConnectTheDots)
|
68 | * [Dogbone](https://maker.js.org/playground/?script=Dogbone)
|
69 | * [Dome](https://maker.js.org/playground/?script=Dome)
|
70 | * [Ellipse](https://maker.js.org/playground/?script=Ellipse)
|
71 | * [Elliptic Arc](https://maker.js.org/playground/?script=EllipticArc)
|
72 | * [Holes](https://maker.js.org/playground/?script=Holes)
|
73 | * [Oval](https://maker.js.org/playground/?script=Oval)
|
74 | * [OvalArc](https://maker.js.org/playground/?script=OvalArc)
|
75 | * [Polygon](https://maker.js.org/playground/?script=Polygon)
|
76 | * [Rectangle](https://maker.js.org/playground/?script=Rectangle)
|
77 | * [Ring](https://maker.js.org/playground/?script=Ring)
|
78 | * [RoundRectangle](https://maker.js.org/playground/?script=RoundRectangle)
|
79 | * [S curve](https://maker.js.org/playground/?script=SCurve)
|
80 | * [Slot](https://maker.js.org/playground/?script=Slot)
|
81 | * [Square](https://maker.js.org/playground/?script=Square)
|
82 | * [Star](https://maker.js.org/playground/?script=Star)
|
83 | * [Text](https://maker.js.org/playground/?script=Text)
|
84 |
|
85 | #### Import formats
|
86 |
|
87 | * [Fonts](https://maker.js.org/playground/?script=Text) (Requires [opentype.js](https://opentype.js.org/))
|
88 | * [SVG Path Data](https://maker.js.org/docs/importing/#SVG+path+data)
|
89 | * [SVG Points](https://maker.js.org/docs/importing/#SVG+points)
|
90 |
|
91 | ## Getting Started
|
92 |
|
93 | ### Try it now
|
94 |
|
95 | Visit the [Maker.js Playground](https://maker.js.org/playground/) a sample app to edit and run JavaScript from your browser.
|
96 |
|
97 | Each of the [demos](https://maker.js.org/demos/#content) will also open in the playground so that you can explore and modify their code.
|
98 |
|
99 | ### To use in a web browser
|
100 |
|
101 | Download the browser-based version of Maker.js, then upload it to your website:
|
102 | [https://maker.js.org/target/js/browser.maker.js](https://maker.js.org/target/js/browser.maker.js)
|
103 |
|
104 | Add a script tag in your HTML:
|
105 | ```html
|
106 | <script src="https://maker.js.org/target/js/browser.maker.js" type="text/javascript"></script>
|
107 | ```
|
108 |
|
109 | *Note: You may also need [additional libraries](https://maker.js.org/docs/getting-started/#For+the+browser)*
|
110 |
|
111 | In your JavaScript, use the require function to get a reference:
|
112 |
|
113 | ```javascript
|
114 | var makerjs = require('makerjs');
|
115 | ```
|
116 |
|
117 | ### To use via CDN
|
118 |
|
119 | Add a script tag to your HTML:
|
120 | ```
|
121 | <script src="https://cdn.jsdelivr.net/npm/makerjs@0/target/js/browser.maker.js"></script>
|
122 | ```
|
123 | To work with Bezier Curves, you will also need a copy of [Bezier.js by Pomax](http://pomax.github.io/bezierjs/)
|
124 | ```
|
125 | <script src="https://cdn.jsdelivr.net/npm/bezier-js@2/bezier.js"></script>
|
126 | ```
|
127 | To work with fonts, you will need both Bezier.js(above) and a copy of [Opentype.js by Frederik De Bleser](https://github.com/nodebox/opentype.js)
|
128 | ```
|
129 | <script src="https://cdn.jsdelivr.net/npm/opentype.js@0/dist/opentype.js"></script>
|
130 | ```
|
131 |
|
132 | In your JavaScript, use the `require` function to get a reference:
|
133 | ```
|
134 | var makerjs = require('makerjs');
|
135 | ```
|
136 |
|
137 | ### To use in Node.js
|
138 |
|
139 | To depend on Maker.js, run this from the command line:
|
140 | ```bash
|
141 | npm install makerjs --save
|
142 | ```
|
143 |
|
144 | In your JavaScript, use the `require` function to get a reference:
|
145 |
|
146 | ```javascript
|
147 | var makerjs = require('makerjs');
|
148 | ```
|
149 |
|
150 | ## Contributing
|
151 | There are many ways to contribute to Maker.js:
|
152 | * [★ Star Maker.js on GitHub](https://github.com/Microsoft/maker.js)
|
153 | * Submit bugs and feature requests [on GitHub](https://github.com/Microsoft/maker.js/issues).
|
154 | * Create a demo for the [gallery](http://maker.js.org/demos/#content).
|
155 | * Create lessons or videos for the [documentation](http://maker.js.org/docs/#content).
|
156 | * Enhance the [website](https://github.com/Microsoft/maker.js/tree/gh-pages).
|
157 | * Add features to the [Playground app](https://maker.js.org/playground/).
|
158 | * Create a new Maker.js app, and we will link to it here.
|
159 | * Find some TODO's in the [core source code](https://github.com/Microsoft/maker.js/tree/master).
|
160 | * Create unit tests for the core.
|
161 |
|
162 | Some of these may require a [contributor agreement](https://github.com/Microsoft/maker.js/blob/master/CONTRIBUTING.md).
|
163 |
|
164 | ### Credits
|
165 | Maker.js depends on:
|
166 | * [clone](https://github.com/pvorb/node-clone) by Paul Vorbach
|
167 | * [bezier-js](https://github.com/Pomax/bezierjs) by Pomax
|
168 | * [graham_scan](https://github.com/brian3kb/graham_scan_js) by Brian Barnett
|
169 | * [kdbush](https://github.com/mourner/kdbush) by Vladimir Agafonkin
|
170 | ---
|
171 |
|
172 | Maker.js is a Microsoft Garage project. The Microsoft Garage turns fresh ideas into real projects. Learn more at [http://microsoft.com/garage](http://microsoft.com/garage).
|