1 | <p align="center"><a href="mind-elixir.com" target="_blank" rel="noopener noreferrer"><img width="150" src="https://raw.githubusercontent.com/ssshooter/mind-elixir-core/master/images/logo2.png" alt="mindelixir logo2"></a><h1 align="center">Mind Elixir</h1></p>
|
2 |
|
3 | <p align="center">
|
4 | <a href="https://www.npmjs.com/package/mind-elixir">
|
5 | <img src="https://img.shields.io/npm/v/mind-elixir" alt="version">
|
6 | </a>
|
7 | <img src="https://img.shields.io/npm/l/mind-elixir" alt="license">
|
8 | <a href="https://app.codacy.com/gh/ssshooter/mind-elixir-core?utm_source=github.com&utm_medium=referral&utm_content=ssshooter/mind-elixir-core&utm_campaign=Badge_Grade_Settings">
|
9 | <img src="https://api.codacy.com/project/badge/Grade/09fadec5bf094886b30cea6aabf3a88b" alt="code quality">
|
10 | </a>
|
11 | <a href="https://bundlephobia.com/result?p=mind-elixir">
|
12 | <img src="https://badgen.net/bundlephobia/dependency-count/mind-elixir" alt="dependency-count">
|
13 | </a>
|
14 | <a href="https://packagephobia.com/result?p=mind-elixir">
|
15 | <img src="https://packagephobia.com/badge?p=mind-elixir" alt="dependency-count">
|
16 | </a>
|
17 | </p>
|
18 |
|
19 | [中文 README](https://github.com/ssshooter/mind-elixir-core/blob/master/readme.cn.md)
|
20 |
|
21 | Mind elixir is a free open source mind map core.
|
22 |
|
23 | - Zero dependency
|
24 | - High performance
|
25 | - Lightweight
|
26 | - Framework agnostic
|
27 | - Pluginable
|
28 | - Build-in drag and drop / node edit plugin
|
29 | - Styling your node with CSS
|
30 |
|
31 | <details>
|
32 | <summary>Table of Contents</summary>
|
33 |
|
34 | - [Doc](#doc)
|
35 | - [Try now](#try-now)
|
36 | - [Playground](#playground)
|
37 | - [Vanilla JS](#vanilla-js)
|
38 | - [Use with React](#use-with-react)
|
39 | - [Use with Vue](#use-with-vue)
|
40 | - [Use with Vue3](#use-with-vue3)
|
41 | - [Usage](#usage)
|
42 | - [Install](#install)
|
43 | - [NPM](#npm)
|
44 | - [Script tag](#script-tag)
|
45 | - [HTML structure](#html-structure)
|
46 | - [Init](#init)
|
47 | - [Data Structure](#data-structure)
|
48 | - [Event Handling](#event-handling)
|
49 | - [Data Export And Import](#data-export-and-import)
|
50 | - [Operation Guards](#operation-guards)
|
51 | - [Theme](#theme)
|
52 | - [Not only core](#not-only-core)
|
53 |
|
54 | </details>
|
55 |
|
56 | ## Doc
|
57 |
|
58 | https://doc.mind-elixir.com/
|
59 |
|
60 | ## Try now
|
61 |
|
62 | 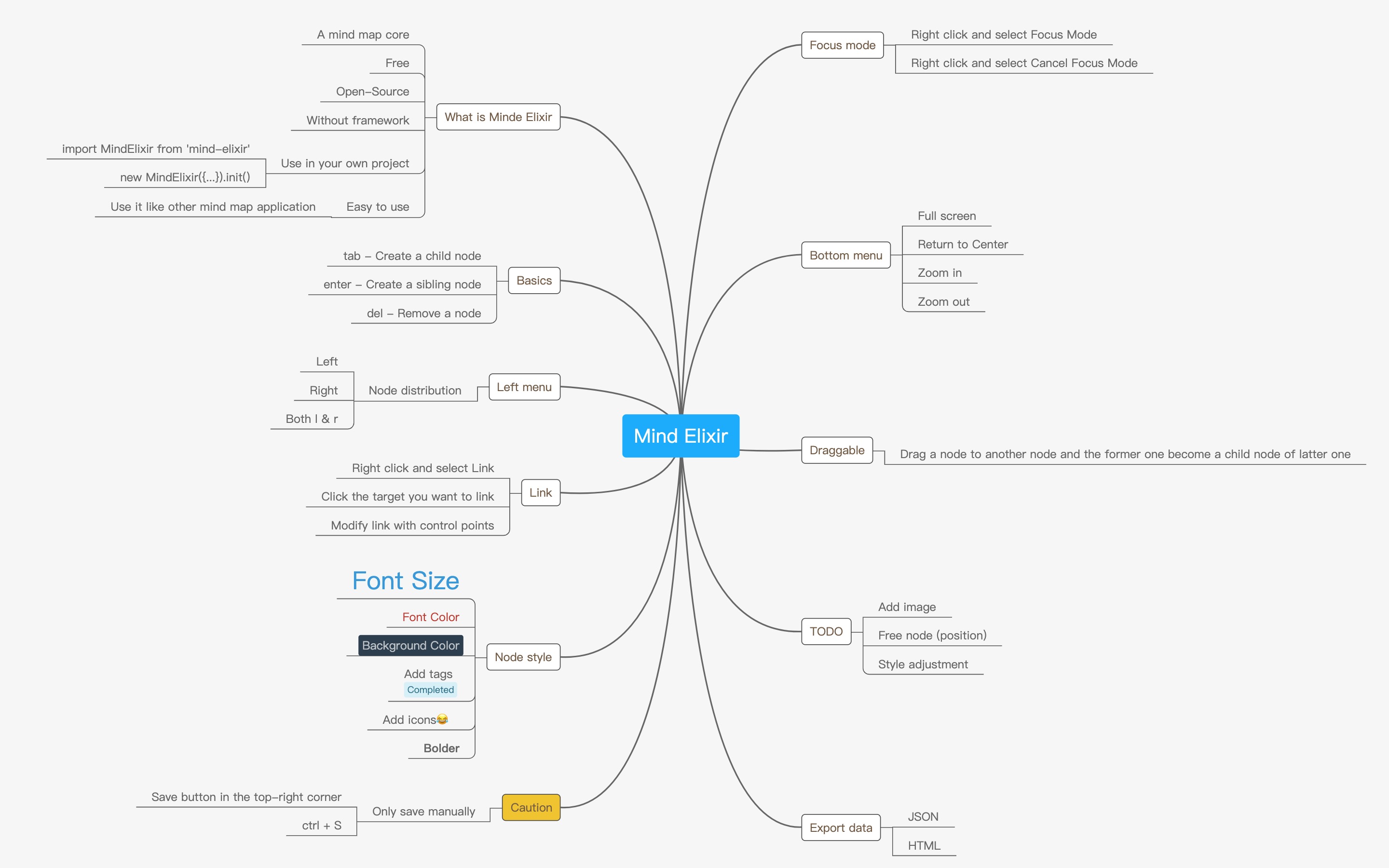
|
63 |
|
64 | https://mind-elixir.com/
|
65 |
|
66 | ### Playground
|
67 |
|
68 | #### Vanilla JS
|
69 |
|
70 | https://codepen.io/ssshooter/pen/GVQRYK
|
71 |
|
72 | #### Use with React
|
73 |
|
74 | https://codesandbox.io/s/mind-elixir-react-9sisb
|
75 |
|
76 | #### Use with Vue
|
77 |
|
78 | https://codesandbox.io/s/mind-elixir-vue-nqjjl
|
79 |
|
80 | #### Use with Vue3
|
81 |
|
82 | https://codesandbox.io/s/mind-elixir-vue3-dtcq6u
|
83 |
|
84 | ## Usage
|
85 |
|
86 | ### Install
|
87 |
|
88 | #### NPM
|
89 |
|
90 | ```bash
|
91 | npm i mind-elixir -S
|
92 | ```
|
93 |
|
94 | ```javascript
|
95 | import MindElixir, { E } from 'mind-elixir'
|
96 | ```
|
97 |
|
98 | #### Script tag
|
99 |
|
100 | ```html
|
101 | <script src="https://cdn.jsdelivr.net/npm/mind-elixir/dist/MindElixir.js"></script>
|
102 | ```
|
103 |
|
104 | ### HTML structure
|
105 |
|
106 | ```html
|
107 | <div id="map"></div>
|
108 | <style>
|
109 | #map {
|
110 | height: 500px;
|
111 | width: 100%;
|
112 | }
|
113 | </style>
|
114 | ```
|
115 |
|
116 | ### Init
|
117 |
|
118 | **Breaking Change** since 1.0.0, `data` should be passed to `init()`, not `options`.
|
119 |
|
120 | ```javascript
|
121 | import MindElixir, { E } from 'mind-elixir'
|
122 | import example from 'mind-elixir/dist/example1'
|
123 |
|
124 | let options = {
|
125 | el: '#map', // or HTMLDivElement
|
126 | direction: MindElixir.LEFT,
|
127 | draggable: true, // default true
|
128 | contextMenu: true, // default true
|
129 | toolBar: true, // default true
|
130 | nodeMenu: true, // default true
|
131 | keypress: true, // default true
|
132 | locale: 'en', // [zh_CN,zh_TW,en,ja,pt,ru] waiting for PRs
|
133 | overflowHidden: false, // default false
|
134 | mainLinkStyle: 2, // [1,2] default 1
|
135 | mainNodeVerticalGap: 15, // default 25
|
136 | mainNodeHorizontalGap: 15, // default 65
|
137 | contextMenuOption: {
|
138 | focus: true,
|
139 | link: true,
|
140 | extend: [
|
141 | {
|
142 | name: 'Node edit',
|
143 | onclick: () => {
|
144 | alert('extend menu')
|
145 | },
|
146 | },
|
147 | ],
|
148 | },
|
149 | allowUndo: false,
|
150 | before: {
|
151 | insertSibling(el, obj) {
|
152 | return true
|
153 | },
|
154 | async addChild(el, obj) {
|
155 | await sleep()
|
156 | return true
|
157 | },
|
158 | },
|
159 | }
|
160 |
|
161 | let mind = new MindElixir(options)
|
162 |
|
163 | mind.install(plugin) // install your plugin
|
164 |
|
165 | // create new map data
|
166 | const data = MindElixir.new('new topic')
|
167 | // or `example`
|
168 | // or the data return from `.getData()`
|
169 | mind.init(data)
|
170 |
|
171 | // get a node
|
172 | E('node-id')
|
173 | ```
|
174 |
|
175 | ### Data Structure
|
176 |
|
177 | ```javascript
|
178 | // whole node data structure up to now
|
179 | const nodeData = {
|
180 | topic: 'node topic',
|
181 | id: 'bd1c24420cd2c2f5',
|
182 | style: { fontSize: '32', color: '#3298db', background: '#ecf0f1' },
|
183 | parent: null,
|
184 | tags: ['Tag'],
|
185 | icons: ['😀'],
|
186 | hyperLink: 'https://github.com/ssshooter/mind-elixir-core',
|
187 | image: {
|
188 | url: 'https://raw.githubusercontent.com/ssshooter/mind-elixir-core/master/images/logo2.png', // required
|
189 | // you need to query the height and width of the image and calculate the appropriate value to display the image
|
190 | height: 90, // required
|
191 | width: 90, // required
|
192 | },
|
193 | children: [
|
194 | {
|
195 | topic: 'child',
|
196 | id: 'xxxx',
|
197 | // ...
|
198 | },
|
199 | ],
|
200 | }
|
201 | ```
|
202 |
|
203 | ### Event Handling
|
204 |
|
205 | ```javascript
|
206 | mind.bus.addListener('operation', operation => {
|
207 | console.log(operation)
|
208 | // return {
|
209 | // name: action name,
|
210 | // obj: target object
|
211 | // }
|
212 |
|
213 | // name: [insertSibling|addChild|removeNode|beginEdit|finishEdit]
|
214 | // obj: target
|
215 |
|
216 | // name: moveNode
|
217 | // obj: {from:target1,to:target2}
|
218 | })
|
219 |
|
220 | mind.bus.addListener('selectNode', node => {
|
221 | console.log(node)
|
222 | })
|
223 |
|
224 | mind.bus.addListener('expandNode', node => {
|
225 | console.log('expandNode: ', node)
|
226 | })
|
227 | ```
|
228 |
|
229 | ### Data Export And Import
|
230 |
|
231 | ```javascript
|
232 | // data export
|
233 | const data = mind.getData() // javascript object, see src/example.js
|
234 | mind.getDataString() // stringify object
|
235 | mind.getDataMd() // markdown
|
236 |
|
237 | // data import
|
238 | // initiate
|
239 | let mind = new MindElixir(options)
|
240 | mind.init(data)
|
241 | // data update
|
242 | mind.refresh(data)
|
243 | ```
|
244 |
|
245 | ### Operation Guards
|
246 |
|
247 | ```javascript
|
248 | let mind = new MindElixir({
|
249 | // ...
|
250 | before: {
|
251 | insertSibling(el, obj) {
|
252 | console.log(el, obj)
|
253 | if (this.currentNode.nodeObj.parent.root) {
|
254 | return false
|
255 | }
|
256 | return true
|
257 | },
|
258 | async addChild(el, obj) {
|
259 | await sleep()
|
260 | if (this.currentNode.nodeObj.parent.root) {
|
261 | return false
|
262 | }
|
263 | return true
|
264 | },
|
265 | },
|
266 | })
|
267 | ```
|
268 |
|
269 | ## Theme
|
270 |
|
271 | ```javascript
|
272 | const options = {
|
273 | // ...
|
274 | theme: {
|
275 | name: 'Dark',
|
276 | // main lines color palette
|
277 | palette: ['#848FA0', '#748BE9', '#D2F9FE', '#4145A5', '#789AFA', '#706CF4', '#EF987F', '#775DD5', '#FCEECF', '#DA7FBC'],
|
278 | // overwrite css variables
|
279 | cssVar: {
|
280 | '--main-color': '#ffffff',
|
281 | '--main-bgcolor': '#4c4f69',
|
282 | '--color': '#cccccc',
|
283 | '--bgcolor': '#252526',
|
284 | },
|
285 | // all variables see /src/index.less
|
286 | },
|
287 | // ...
|
288 | }
|
289 | ```
|
290 |
|
291 | ## Not only core
|
292 |
|
293 | - [@mind-elixir/export-xmind](https://github.com/ssshooter/export-xmind)
|
294 | - [@mind-elixir/export-html](https://github.com/ssshooter/export-html)
|
295 | - [@mind-elixir/export-image](https://github.com/ssshooter/export-image) (WIP🚧)
|
296 | - [mind-elixir-react](https://github.com/ssshooter/mind-elixir-react)
|