1 | <p align="center"><a href="mind-elixir.com" target="_blank" rel="noopener noreferrer"><img width="150" src="https://raw.githubusercontent.com/ssshooter/mind-elixir-core/master/images/logo2.png" alt="mindelixir logo2"></a></p>
|
2 |
|
3 | <p align="center">
|
4 | <a href="https://www.npmjs.com/package/mind-elixir">
|
5 | <img src="https://img.shields.io/npm/v/mind-elixir" alt="version">
|
6 | </a>
|
7 | <img src="https://img.shields.io/npm/l/mind-elixir" alt="license">
|
8 | <a href="https://app.codacy.com/gh/ssshooter/mind-elixir-core?utm_source=github.com&utm_medium=referral&utm_content=ssshooter/mind-elixir-core&utm_campaign=Badge_Grade_Settings">
|
9 | <img src="https://api.codacy.com/project/badge/Grade/09fadec5bf094886b30cea6aabf3a88b" alt="code quality">
|
10 | </a>
|
11 | <a href="https://bundlephobia.com/result?p=mind-elixir">
|
12 | <img src="https://badgen.net/bundlephobia/dependency-count/mind-elixir" alt="dependency-count">
|
13 | </a>
|
14 | <a href="https://packagephobia.com/result?p=mind-elixir">
|
15 | <img src="https://packagephobia.com/badge?p=mind-elixir" alt="dependency-count">
|
16 | </a>
|
17 | </p>
|
18 |
|
19 | Mind elixir 是一个无框架依赖的思维导图内核
|
20 |
|
21 | [English](https://github.com/ssshooter/mind-elixir-core/blob/master/readme.md)
|
22 |
|
23 | ## 立即尝试
|
24 |
|
25 | 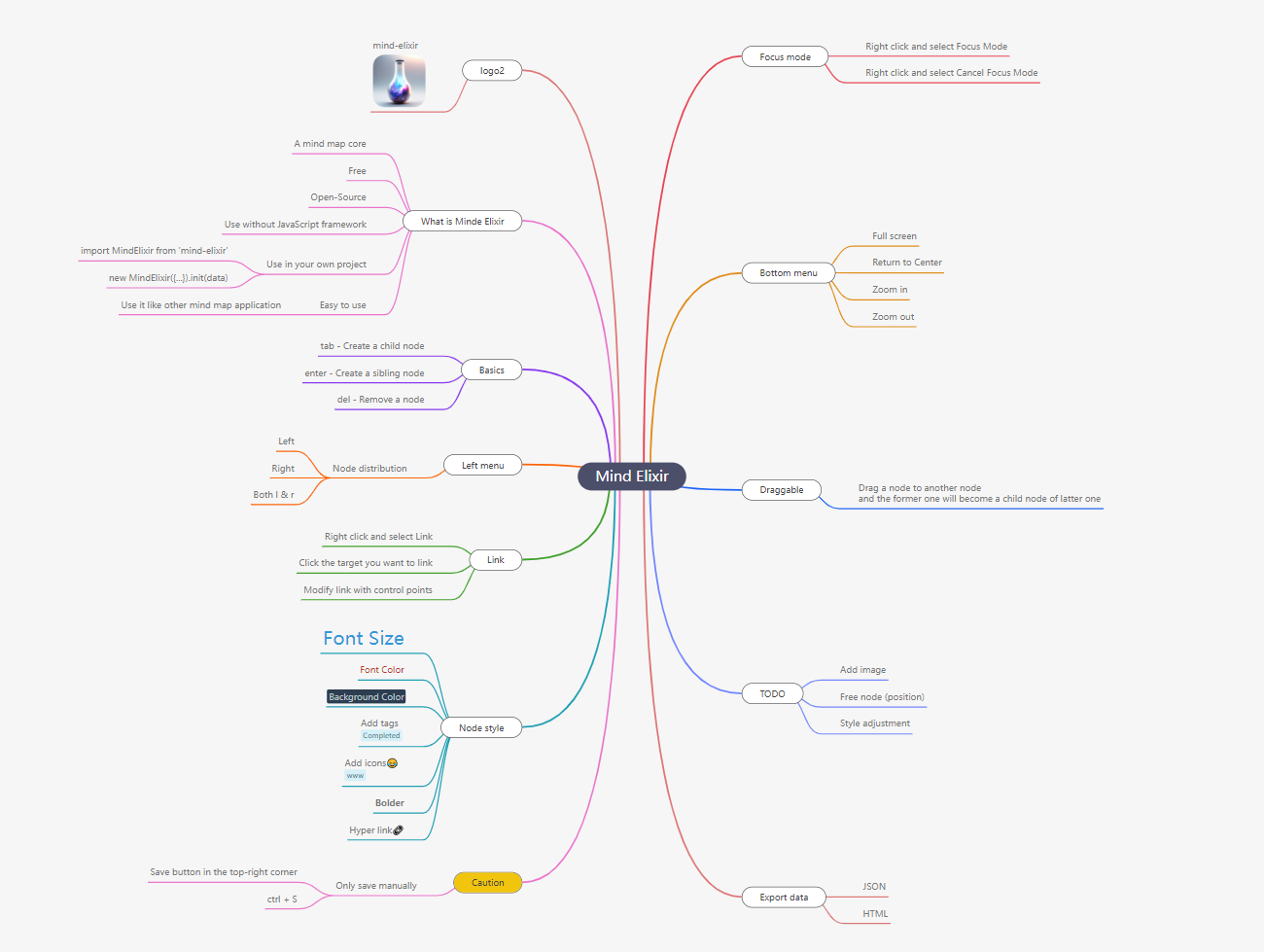
|
26 |
|
27 | https://mind-elixir.com/#/
|
28 |
|
29 | ### Playground
|
30 |
|
31 | https://codepen.io/ssshooter/pen/GVQRYK
|
32 |
|
33 | with React https://codesandbox.io/s/mind-elixir-react-9sisb
|
34 |
|
35 | with Vue https://codesandbox.io/s/mind-elixir-vue-nqjjl
|
36 |
|
37 | ## 如何使用
|
38 |
|
39 | ### 安装
|
40 |
|
41 | #### NPM
|
42 |
|
43 | ```bash
|
44 | npm i mind-elixir -S
|
45 | ```
|
46 |
|
47 | ```javascript
|
48 | import MindElixir, { E } from 'mind-elixir'
|
49 | ```
|
50 |
|
51 | #### Script 标签
|
52 |
|
53 | ```html
|
54 | <script src="https://cdn.jsdelivr.net/npm/mind-elixir/dist/mind-elixir.js"></script>
|
55 | ```
|
56 |
|
57 | ### HTML 结构
|
58 |
|
59 | ```html
|
60 | <div id="map"></div>
|
61 | <style>
|
62 | #map {
|
63 | height: 500px;
|
64 | width: 100%;
|
65 | }
|
66 | </style>
|
67 | ```
|
68 |
|
69 | ### 初始化
|
70 |
|
71 | ```javascript
|
72 | import MindElixir, { E } from 'mind-elixir'
|
73 | import { exportSvg, exportPng } from '../dist/painter'
|
74 | import example from '../dist/example1'
|
75 |
|
76 | let options = {
|
77 | el: '#map',
|
78 | direction: MindElixir.LEFT,
|
79 | // create new map data
|
80 | data: MindElixir.new('new topic') or example,
|
81 | // the data return from `.getData()`
|
82 | draggable: true, // default true
|
83 | contextMenu: true, // default true
|
84 | toolBar: true, // default true
|
85 | nodeMenu: true, // default true
|
86 | keypress: true, // default true
|
87 | locale: 'en', // [zh_CN,zh_TW,en,ja,pt] waiting for PRs
|
88 | overflowHidden: false, // default false
|
89 | mainLinkStyle: 2, // [1,2] default 1
|
90 | contextMenuOption: {
|
91 | focus: true,
|
92 | link: true,
|
93 | extend: [
|
94 | {
|
95 | name: 'Node edit',
|
96 | onclick: () => {
|
97 | alert('extend menu')
|
98 | },
|
99 | },
|
100 | ],
|
101 | },
|
102 | allowUndo: false,
|
103 | before: {
|
104 | insertSibling(el, obj) {
|
105 | return true
|
106 | },
|
107 | async addChild(el, obj) {
|
108 | await sleep()
|
109 | return true
|
110 | },
|
111 | },
|
112 | }
|
113 |
|
114 | let mind = new MindElixir(options)
|
115 | mind.init()
|
116 |
|
117 | // get a node
|
118 | E('node-id')
|
119 |
|
120 | ```
|
121 |
|
122 | ### 数据结构
|
123 |
|
124 | ```javascript
|
125 | // whole node data structure up to now
|
126 | {
|
127 | topic: 'node topic',
|
128 | id: 'bd1c24420cd2c2f5',
|
129 | style: { fontSize: '32', color: '#3298db', background: '#ecf0f1' },
|
130 | parent: null,
|
131 | tags: ['Tag'],
|
132 | icons: ['😀'],
|
133 | hyperLink: 'https://github.com/ssshooter/mind-elixir-core',
|
134 | }
|
135 | ```
|
136 |
|
137 | ### 事件处理
|
138 |
|
139 | ```javascript
|
140 | mind.bus.addListener('operation', operation => {
|
141 | console.log(operation)
|
142 | // return {
|
143 | // name: action name,
|
144 | // obj: target object
|
145 | // }
|
146 |
|
147 | // name: [insertSibling|addChild|removeNode|beginEdit|finishEdit]
|
148 | // obj: target
|
149 |
|
150 | // name: moveNode
|
151 | // obj: {from:target1,to:target2}
|
152 | })
|
153 |
|
154 | mind.bus.addListener('selectNode', node => {
|
155 | console.log(node)
|
156 | })
|
157 |
|
158 | mind.bus.addListener('expandNode', node => {
|
159 | console.log('expandNode: ', node)
|
160 | })
|
161 | ```
|
162 |
|
163 | ### 数据导出
|
164 |
|
165 | ```javascript
|
166 | mind.getData() // javascript object, see src/example.js
|
167 | mind.getDataString() // stringify object
|
168 | mind.getDataMd() // markdown
|
169 | ```
|
170 |
|
171 | ### 输出图片
|
172 |
|
173 | **WIP**
|
174 |
|
175 | ```javascript
|
176 | import painter from 'mind-elixir/dist/painter'
|
177 | painter.exportSvg()
|
178 | painter.exportPng()
|
179 | ```
|
180 |
|
181 | ### 操作拦截
|
182 |
|
183 | ```javascript
|
184 | let mind = new MindElixir({
|
185 | ...
|
186 | before: {
|
187 | insertSibling(el, obj) {
|
188 | console.log(el, obj)
|
189 | if (this.currentNode.nodeObj.parent.root) {
|
190 | return false
|
191 | }
|
192 | return true
|
193 | },
|
194 | async addChild(el, obj) {
|
195 | await sleep()
|
196 | if (this.currentNode.nodeObj.parent.root) {
|
197 | return false
|
198 | }
|
199 | return true
|
200 | },
|
201 | },
|
202 | })
|
203 | ```
|
204 |
|
205 | ## 文档
|
206 |
|
207 | https://doc.mind-elixir.com/
|