1 | <p align="center">
|
2 | <a href="mind-elixir.com" target="_blank" rel="noopener noreferrer">
|
3 | <img width="150" src="https://raw.githubusercontent.com/ssshooter/mind-elixir-core/master/images/logo2.png" alt="mindelixir logo2">
|
4 | </a>
|
5 | <h1 align="center">Mind Elixir</h1>
|
6 | </p>
|
7 |
|
8 | <p align="center">
|
9 | <a href="https://www.npmjs.com/package/mind-elixir">
|
10 | <img src="https://img.shields.io/npm/v/mind-elixir" alt="version">
|
11 | </a>
|
12 | <a href="https://github.com/ssshooter/mind-elixir-core/blob/master/LICENSE">
|
13 | <img src="https://img.shields.io/npm/l/mind-elixir" alt="license">
|
14 | </a>
|
15 | <a href="https://app.codacy.com/gh/ssshooter/mind-elixir-core?utm_source=github.com&utm_medium=referral&utm_content=ssshooter/mind-elixir-core&utm_campaign=Badge_Grade_Settings">
|
16 | <img src="https://api.codacy.com/project/badge/Grade/09fadec5bf094886b30cea6aabf3a88b" alt="code quality">
|
17 | </a>
|
18 | <a href="https://bundlephobia.com/result?p=mind-elixir">
|
19 | <img src="https://badgen.net/bundlephobia/dependency-count/mind-elixir" alt="dependency-count">
|
20 | </a>
|
21 | <a href="https://packagephobia.com/result?p=mind-elixir">
|
22 | <img src="https://packagephobia.com/badge?p=mind-elixir" alt="package size">
|
23 | </a>
|
24 | </p>
|
25 |
|
26 | [中文 README](https://github.com/ssshooter/mind-elixir-core/blob/master/readme.cn.md)
|
27 |
|
28 | Mind elixir is a free open source mind map core.
|
29 |
|
30 | - Lightweight
|
31 | - High performance
|
32 | - Framework agnostic
|
33 | - Pluginable
|
34 | - Build-in drag and drop / node edit plugin
|
35 | - Summarize nodes
|
36 | - Undo / Redo
|
37 | - Styling your node with CSS
|
38 |
|
39 | <details>
|
40 | <summary>Table of Contents</summary>
|
41 |
|
42 | - [Try now](#try-now)
|
43 | - [Playground](#playground)
|
44 | - [Usage](#usage)
|
45 | - [Install](#install)
|
46 | - [NPM](#npm)
|
47 | - [Script tag](#script-tag)
|
48 | - [Init](#init)
|
49 | - [Data Structure](#data-structure)
|
50 | - [Event Handling](#event-handling)
|
51 | - [Data Export And Import](#data-export-and-import)
|
52 | - [Operation Guards](#operation-guards)
|
53 | - [Methods](#methods)
|
54 | - [Theme](#theme)
|
55 | - [Shortcuts](#shortcuts)
|
56 | - [Not only core](#not-only-core)
|
57 | - [Development](#development)
|
58 | - [Thanks](#thanks)
|
59 |
|
60 | </details>
|
61 |
|
62 | ## Try now
|
63 |
|
64 | 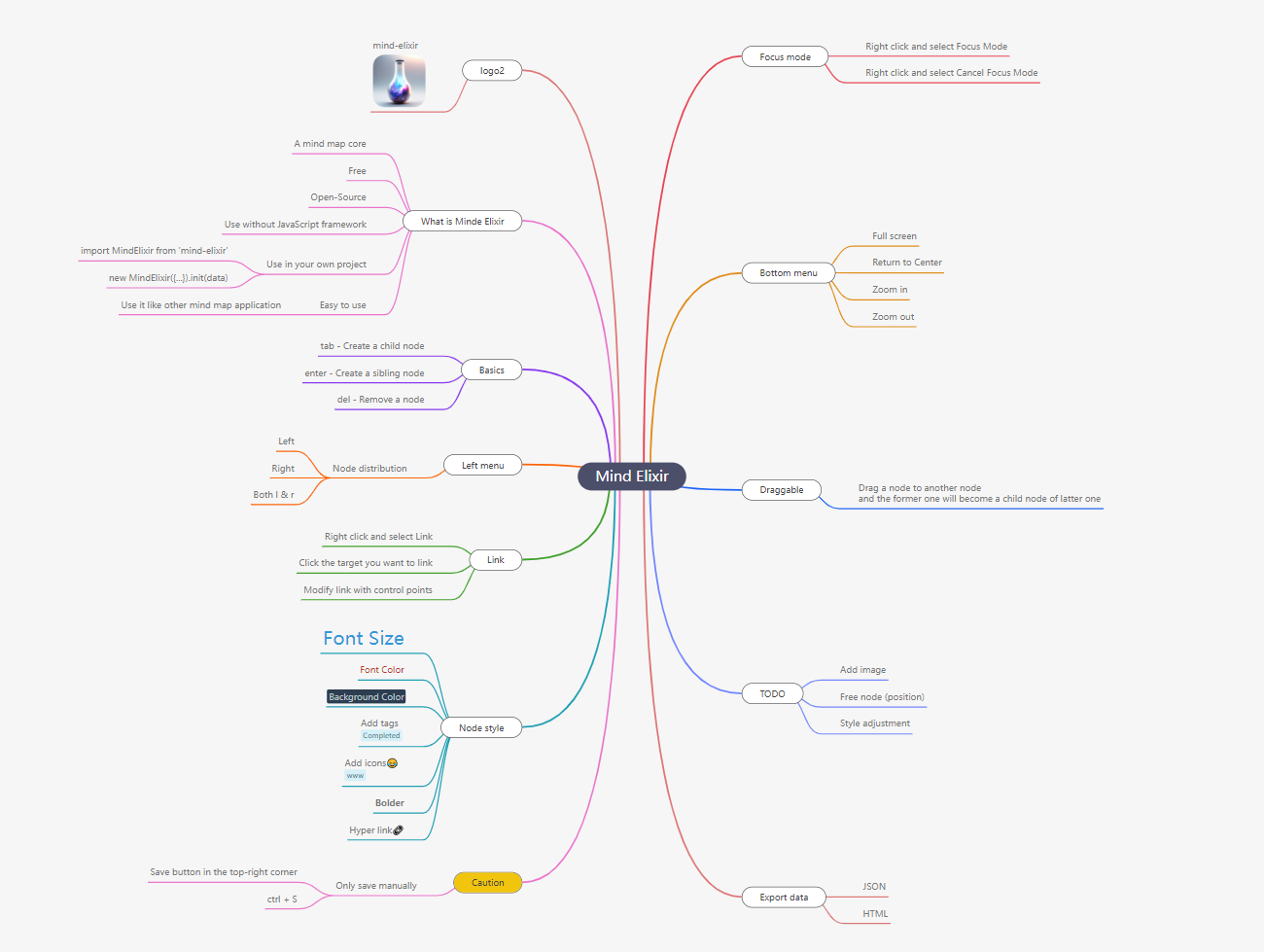
|
65 |
|
66 | https://mind-elixir.com/
|
67 |
|
68 | ### Playground
|
69 |
|
70 | - Vanilla JS - https://codepen.io/ssshooter/pen/OJrJowN
|
71 | - React - https://codesandbox.io/s/mind-elixir-3-x-react-18-x-vy9fcq
|
72 | - Vue3 - https://codesandbox.io/s/mind-elixir-3-x-vue3-lth484
|
73 | - Vue2 - https://codesandbox.io/s/mind-elixir-3-x-vue-2-x-5kdfjp
|
74 |
|
75 | ## Usage
|
76 |
|
77 | ### Install
|
78 |
|
79 | #### NPM
|
80 |
|
81 | ```bash
|
82 | npm i mind-elixir -S
|
83 | ```
|
84 |
|
85 | ```javascript
|
86 | import MindElixir from 'mind-elixir'
|
87 | ```
|
88 |
|
89 | #### Script tag
|
90 |
|
91 | ```html
|
92 | <script src="https://cdn.jsdelivr.net/npm/mind-elixir/dist/MindElixir.js"></script>
|
93 | ```
|
94 |
|
95 | ### Init
|
96 |
|
97 | ```html
|
98 | <div id="map"></div>
|
99 | <style>
|
100 | #map {
|
101 | height: 500px;
|
102 | width: 100%;
|
103 | }
|
104 | </style>
|
105 | ```
|
106 |
|
107 | **Breaking Change** since 1.0.0, `data` should be passed to `init()`, not `options`.
|
108 |
|
109 | ```javascript
|
110 | import MindElixir from 'mind-elixir'
|
111 | import example from 'mind-elixir/dist/example1'
|
112 |
|
113 | let options = {
|
114 | el: '#map', // or HTMLDivElement
|
115 | direction: MindElixir.LEFT,
|
116 | draggable: true, // default true
|
117 | contextMenu: true, // default true
|
118 | toolBar: true, // default true
|
119 | nodeMenu: true, // default true
|
120 | keypress: true, // default true
|
121 | locale: 'en', // [zh_CN,zh_TW,en,ja,pt,ru] waiting for PRs
|
122 | overflowHidden: false, // default false
|
123 | mainLinkStyle: 2, // [1,2] default 1
|
124 | contextMenuOption: {
|
125 | focus: true,
|
126 | link: true,
|
127 | extend: [
|
128 | {
|
129 | name: 'Node edit',
|
130 | onclick: () => {
|
131 | alert('extend menu')
|
132 | },
|
133 | },
|
134 | ],
|
135 | },
|
136 | before: {
|
137 | insertSibling(el, obj) {
|
138 | return true
|
139 | },
|
140 | async addChild(el, obj) {
|
141 | await sleep()
|
142 | return true
|
143 | },
|
144 | },
|
145 | }
|
146 |
|
147 | let mind = new MindElixir(options)
|
148 |
|
149 | mind.install(plugin) // install your plugin
|
150 |
|
151 | // create new map data
|
152 | const data = MindElixir.new('new topic')
|
153 | // or `example`
|
154 | // or the data return from `.getData()`
|
155 | mind.init(data)
|
156 |
|
157 | // get a node
|
158 | MindElixir.E('node-id')
|
159 | ```
|
160 |
|
161 | ### Data Structure
|
162 |
|
163 | ```javascript
|
164 | // whole node data structure up to now
|
165 | const nodeData = {
|
166 | topic: 'node topic',
|
167 | id: 'bd1c24420cd2c2f5',
|
168 | style: { fontSize: '32', color: '#3298db', background: '#ecf0f1' },
|
169 | expanded: true,
|
170 | parent: null,
|
171 | tags: ['Tag'],
|
172 | icons: ['😀'],
|
173 | hyperLink: 'https://github.com/ssshooter/mind-elixir-core',
|
174 | image: {
|
175 | url: 'https://raw.githubusercontent.com/ssshooter/mind-elixir-core/master/images/logo2.png', // required
|
176 | // you need to query the height and width of the image and calculate the appropriate value to display the image
|
177 | height: 90, // required
|
178 | width: 90, // required
|
179 | },
|
180 | children: [
|
181 | {
|
182 | topic: 'child',
|
183 | id: 'xxxx',
|
184 | // ...
|
185 | },
|
186 | ],
|
187 | }
|
188 | ```
|
189 |
|
190 | ### Event Handling
|
191 |
|
192 | ```javascript
|
193 | mind.bus.addListener('operation', operation => {
|
194 | console.log(operation)
|
195 | // return {
|
196 | // name: action name,
|
197 | // obj: target object
|
198 | // }
|
199 |
|
200 | // name: [insertSibling|addChild|removeNode|beginEdit|finishEdit]
|
201 | // obj: target
|
202 |
|
203 | // name: moveNode
|
204 | // obj: {from:target1,to:target2}
|
205 | })
|
206 |
|
207 | mind.bus.addListener('selectNode', node => {
|
208 | console.log(node)
|
209 | })
|
210 |
|
211 | mind.bus.addListener('expandNode', node => {
|
212 | console.log('expandNode: ', node)
|
213 | })
|
214 | ```
|
215 |
|
216 | ### Data Export And Import
|
217 |
|
218 | ```javascript
|
219 | // data export
|
220 | const data = mind.getData() // javascript object, see src/example.js
|
221 | mind.getDataString() // stringify object
|
222 | mind.getDataMd() // markdown
|
223 |
|
224 | // data import
|
225 | // initiate
|
226 | let mind = new MindElixir(options)
|
227 | mind.init(data)
|
228 | // data update
|
229 | mind.refresh(data)
|
230 | ```
|
231 |
|
232 | ### Operation Guards
|
233 |
|
234 | ```javascript
|
235 | let mind = new MindElixir({
|
236 | // ...
|
237 | before: {
|
238 | insertSibling(el, obj) {
|
239 | console.log(el, obj)
|
240 | if (this.currentNode.nodeObj.parent.root) {
|
241 | return false
|
242 | }
|
243 | return true
|
244 | },
|
245 | async addChild(el, obj) {
|
246 | await sleep()
|
247 | if (this.currentNode.nodeObj.parent.root) {
|
248 | return false
|
249 | }
|
250 | return true
|
251 | },
|
252 | },
|
253 | })
|
254 | ```
|
255 |
|
256 | ## Methods
|
257 |
|
258 | https://github.com/ssshooter/mind-elixir-core/blob/master/api/mind-elixir.api.md
|
259 |
|
260 | ## Theme
|
261 |
|
262 | ```javascript
|
263 | const options = {
|
264 | // ...
|
265 | theme: {
|
266 | name: 'Dark',
|
267 | // main lines color palette
|
268 | palette: ['#848FA0', '#748BE9', '#D2F9FE', '#4145A5', '#789AFA', '#706CF4', '#EF987F', '#775DD5', '#FCEECF', '#DA7FBC'],
|
269 | // overwrite css variables
|
270 | cssVar: {
|
271 | '--main-color': '#ffffff',
|
272 | '--main-bgcolor': '#4c4f69',
|
273 | '--color': '#cccccc',
|
274 | '--bgcolor': '#252526',
|
275 | '--panel-color': '255, 255, 255',
|
276 | '--panel-bgcolor': '45, 55, 72',
|
277 | },
|
278 | // all variables see /src/index.less
|
279 | },
|
280 | // ...
|
281 | }
|
282 |
|
283 | // ...
|
284 |
|
285 | mind.changeTheme({
|
286 | name: 'Latte',
|
287 | palette: ['#dd7878', '#ea76cb', '#8839ef', '#e64553', '#fe640b', '#df8e1d', '#40a02b', '#209fb5', '#1e66f5', '#7287fd'],
|
288 | cssVar: {
|
289 | '--main-color': '#444446',
|
290 | '--main-bgcolor': '#ffffff',
|
291 | '--color': '#777777',
|
292 | '--bgcolor': '#f6f6f6',
|
293 | },
|
294 | })
|
295 | ```
|
296 |
|
297 | Be aware that Mind Elixir will not observe the change of `prefers-color-scheme`. Please change the theme **manually** when the scheme changes.
|
298 |
|
299 | ## Shortcuts
|
300 |
|
301 | | Shortcut | Function |
|
302 | | ------------------ | -------------------------------- |
|
303 | | Enter | Insert Sibling Node |
|
304 | | Tab | Insert Child Node |
|
305 | | F1 | Center the Map |
|
306 | | F2 | Begin Editing the Current Node |
|
307 | | Up Arrow | Select the Previous Sibling Node |
|
308 | | Down Arrow | Select the Next Sibling Node |
|
309 | | Left/Right Arrow | Select Parent or First Child |
|
310 | | PageUp | Move Up Node |
|
311 | | PageDown | Move Down Node |
|
312 | | Ctrl + Up Arrow | Change Layout Pattern to Side |
|
313 | | Ctrl + Left Arrow | Change Layout Pattern to Left |
|
314 | | Ctrl + Right Arrow | Change Layout Pattern to Right |
|
315 | | Ctrl + C | Copy the Current Node |
|
316 | | Ctrl + V | Paste the Copied Node |
|
317 | | Ctrl + "+" | Zoom In Mind Map |
|
318 | | Ctrl + "-" | Zoom Out Mind Map |
|
319 | | Ctrl + 0 | Reset Zoom Level |
|
320 |
|
321 | ## Not only core
|
322 |
|
323 | - [@mind-elixir/node-menu](https://github.com/ssshooter/node-menu)
|
324 | - [@mind-elixir/node-menu-neo](https://github.com/ssshooter/node-menu-neo)
|
325 | - [@mind-elixir/export-xmind](https://github.com/ssshooter/export-xmind)
|
326 | - [@mind-elixir/export-html](https://github.com/ssshooter/export-html)
|
327 | - [@mind-elixir/export-image](https://github.com/ssshooter/export-image) (WIP🚧)
|
328 | - [mind-elixir-react](https://github.com/ssshooter/mind-elixir-react)
|
329 |
|
330 | ## Development
|
331 |
|
332 | ```
|
333 | pnpm i
|
334 | npm start
|
335 | ```
|
336 |
|
337 | Test generated files with `dev.dist.ts`:
|
338 |
|
339 | ```
|
340 | npm run build
|
341 | npm link
|
342 | npm link mind-elixir
|
343 | ```
|
344 |
|
345 | ## Thanks
|
346 |
|
347 | - [@viselect/vanilla](https://github.com/simonwep/selection/tree/master/packages/vanilla)
|