1 | # Mocha-Each - Parameterized Test for Mocha
|
2 |
|
3 | [][npm-version]
|
4 | [][travis-ci]
|
5 | [][coveralls]
|
6 | [][david]
|
7 | [][david-dev]
|
8 |
|
9 | [npm-version]: https://www.npmjs.org/package/mocha-each
|
10 | [travis-ci]: https://travis-ci.org/ryym/mocha-each
|
11 | [coveralls]: https://coveralls.io/github/ryym/mocha-each?branch=master
|
12 | [david]: https://david-dm.org/ryym/mocha-each
|
13 | [david-dev]: https://david-dm.org/ryym/mocha-each#info=devDependencies
|
14 |
|
15 | This module provides a way to write simple parameterized tests in [Mocha].
|
16 |
|
17 | [Mocha]: https://mochajs.org/
|
18 |
|
19 | ## Installation
|
20 |
|
21 | ```
|
22 | npm install --save-dev mocha-each
|
23 | ```
|
24 |
|
25 | ## Usage
|
26 |
|
27 | ### Basic
|
28 |
|
29 | The function mocha-each provides takes parameters as an array and returns a
|
30 | parameterized test function. You can define test cases for each parameter by the function.
|
31 | All the test cases you defined are executed even if one or more cases fail during the test.
|
32 |
|
33 | ```javascript
|
34 | // test.js
|
35 | const assert = require('assert');
|
36 | const forEach = require('mocha-each');
|
37 |
|
38 | function add(a, b) {
|
39 | return parseInt(a) + parseInt(b);
|
40 | }
|
41 |
|
42 | describe('add()', () => {
|
43 | forEach([
|
44 | [1, 1, 2],
|
45 | [2, -2, 0],
|
46 | [140, 48, 188]
|
47 | ])
|
48 | .it('adds %d and %d then returns %d', (left, right, expected) => {
|
49 | assert.equal(add(left, right), expected);
|
50 | });
|
51 |
|
52 | context('with invalid arguments', () => {
|
53 | forEach([
|
54 | [1, 'foo'],
|
55 | [null, 10],
|
56 | [{}, []]
|
57 | ])
|
58 | .it('adds %j and %j then returns NaN', (left, right) => {
|
59 | const value = add(left, right);
|
60 | assert(isNaN(value));
|
61 | });
|
62 | });
|
63 | });
|
64 | ```
|
65 |
|
66 | Result:
|
67 |
|
68 | 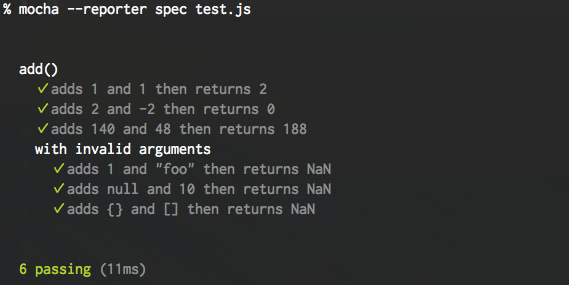
|
69 |
|
70 | ### Asynchronous code
|
71 |
|
72 | When testing asynchronous code, you need to add a callback as a last argument of
|
73 | the test function and call it. The callback is same as the one which Mocha's `it` gives.
|
74 | See [Mocha - asynchronous code] for the detail.
|
75 |
|
76 | [Mocha - asynchronous code]: https://mochajs.org/#asynchronous-code
|
77 |
|
78 | ```javascript
|
79 | forEach([
|
80 | [0, 1],
|
81 | [2, 3]
|
82 | ])
|
83 | .it('does async operation', (arg, expected, done) => {
|
84 | fetchData(arg)
|
85 | .then(actual => assert.equal(actual, expected))
|
86 | .then(done);
|
87 | });
|
88 | ```
|
89 |
|
90 | ### Exclusive or inclusive tests
|
91 |
|
92 | You can call [.only()] and [.skip()] in the same way as Mocha's `it`.
|
93 |
|
94 | ```javascript
|
95 | // Run only these parameterized tests.
|
96 | forEach([
|
97 | 0, 1, 2, 3
|
98 | ])
|
99 | .it.only('works fine', number => {
|
100 | assert(number);
|
101 | });
|
102 |
|
103 | // Ignore these parameterized tests.
|
104 | forEach([
|
105 | 'foo', 'bar', 'baz'
|
106 | ])
|
107 | .it.skip('also works fine', word => {
|
108 | assert(word);
|
109 | });
|
110 | ```
|
111 |
|
112 | Note:
|
113 | When you use the `.only()`, mocha-each creates a nameless test suite by [describe()]
|
114 | to define exclusive parameterized tests because we can't call `.only()` multiple times
|
115 | (see [Mocha docs][.only()]).
|
116 |
|
117 | [.only()]: http://mochajs.org/#exclusive-tests
|
118 | [.skip()]: http://mochajs.org/#inclusive-tests
|
119 | [describe()]: https://mochajs.org/#interfaces
|
120 |
|
121 | ## API
|
122 |
|
123 | ### forEach(parameters).it(title, testCase)
|
124 |
|
125 | #### parameters: `Array`
|
126 |
|
127 | An array of parameters. Each parameter will be applied to `testCase` as its arguments.
|
128 |
|
129 | #### title: `String` or `Function`
|
130 |
|
131 | A title of the test case. You can define each title as a string or function.
|
132 |
|
133 | ##### String title
|
134 |
|
135 | When it is a string, you can use placeholders which will be replaced by the parameters.
|
136 | mocha-each uses [sprintf-js] to replace placeholders.
|
137 |
|
138 | [sprintf-js]: https://github.com/alexei/sprintf.js
|
139 |
|
140 | example:
|
141 |
|
142 | ```javascript
|
143 | forEach([
|
144 | ['Good morning', 9],
|
145 | ['Hello', 12],
|
146 | ['Godd evening', 21]
|
147 | ])
|
148 | .it('greets "%s" at %d:00', (expected, time) => {
|
149 | const greet = greeter.at(time).greet();
|
150 | assert.equal(greet, expected);
|
151 | });
|
152 | // =>
|
153 | // greets "Good morning" at 9:00
|
154 | // greets "Hello" at 12:00
|
155 | // greets "Good evening" at 21:00
|
156 | ```
|
157 |
|
158 | ##### Function title
|
159 |
|
160 | When it is a function, it takes each parameter and index as its arguments like:
|
161 |
|
162 | ```
|
163 | title(p[0], p[1], .., p[n - 1], index);
|
164 | ```
|
165 |
|
166 | #### testCase: `Function`
|
167 |
|
168 | A test function which will be called for each parameter. The context of the function
|
169 | is same as Mocha's `it` so that you can use configuration methods like [timeout].
|
170 |
|
171 | [timeout]: https://mochajs.org/#timeouts
|
172 |
|
173 | ```javascript
|
174 | forEach([
|
175 | //...
|
176 | ])
|
177 | .it('is a slow test', function(p0, p1, p2 /*, done */) {
|
178 | this.timeout(3000); // Configure timeout.
|
179 | use(p0, p1, p2);
|
180 | //...
|
181 | });
|
182 | ```
|
183 |
|
184 | ## Supported interfaces
|
185 |
|
186 | Though Mocha provides [several interfaces], currently only the BDD interface is supported.
|
187 |
|
188 | [several interfaces]: https://mochajs.org/#interfaces
|
189 |
|
190 | ## Tips
|
191 |
|
192 | You can define the name of mocha-each function as you like when requiring it.
|
193 | Here's a example which uses more BDD-like name.
|
194 |
|
195 | ```javascript
|
196 | const withThese = require('mocha-each');
|
197 |
|
198 | describe('findByName()', () => {
|
199 | withThese([
|
200 | [1, 'foo'],
|
201 | [2, 'bar'],
|
202 | [3, 'baz']
|
203 | ])
|
204 | .it('should find data by name', (id, name) => {
|
205 | const data = findByName(name);
|
206 | assert.equal(data.id, id);
|
207 | });
|
208 | });
|
209 | ```
|
210 |
|
211 | ## License
|
212 |
|
213 | MIT
|