1 | var Util = require('./util');
|
2 |
|
3 | // BEGIN(BROWSER)
|
4 | /*
|
5 | ### Mock.Random
|
6 |
|
7 | Mock.Random 是一个工具类,用于生成各种随机数据。Mock.Random 的方法在数据模板中称为“占位符”,引用格式为 `@占位符(参数 [, 参数])` 。例如:
|
8 |
|
9 | var Random = Mock.Random;
|
10 | Random.email()
|
11 | // => "n.clark@miller.io"
|
12 | Mock.mock('@EMAIL')
|
13 | // => "y.lee@lewis.org"
|
14 | Mock.mock( { email: '@EMAIL' } )
|
15 | // => { email: "v.lewis@hall.gov" }
|
16 |
|
17 | 可以在上面的例子中看到,直接调用 'Random.email()' 时方法名 `email()` 是小写的,而数据模板中的 `@EMAIL` 却是大写。这并对数据模板中的占位符做了特殊处理,也非强制的编写方式,事实上在数据模板中使用小写的 `@email` 也可以达到同样的效果。不过,这是建议的编码风格,以便在阅读时从视觉上提高占位符的识别率,快速识别占位符和普通字符。
|
18 |
|
19 | 在浏览器中,为了减少需要拼写的字符,Mock.js 把 Mock.Random 暴露给了 window 对象,使之称为全局变量,从而可以直接访问 Random。因此上面例子中的 `var Random = Mock.Random;` 可以省略。在后面的例子中,也将做同样的处理。
|
20 |
|
21 | > 在 Node.js 中,仍然需要通过 `Mock.Random` 访问。
|
22 |
|
23 | Mock.Random 中的方法与数据模板的 `@占位符` 一一对应,在需要时可以为 Mock.Random 扩展方法,然后在数据模板中通过 `@扩展方法` 引用。例如:
|
24 |
|
25 | Random.extend({
|
26 | xzs: [], // 十二星座?程序员日历?
|
27 | xz: function(date){
|
28 | return ''
|
29 | }
|
30 | })
|
31 | Random.xz()
|
32 | // => ""
|
33 | Mock.mock('@XZ')
|
34 | // => ""
|
35 | Mock.mock({ xz: '@XZ'})
|
36 | // => ""
|
37 |
|
38 | Mock.js 的 [在线编辑器](http://mockjs.com/mock.html) 演示了完整的语法规则和占位符。
|
39 |
|
40 | 下面是 Mock.Random 内置支持的方法说明。
|
41 |
|
42 | (功能,方法签名,参数说明,示例)
|
43 | */
|
44 | var Random = (function() {
|
45 | var Random = {
|
46 | extend: Util.extend
|
47 | }
|
48 | /*
|
49 | #### Basics
|
50 | */
|
51 | Random.extend({
|
52 | /*
|
53 | ##### Random.boolean(min, max, cur)
|
54 |
|
55 | 返回一个随机的布尔值。
|
56 |
|
57 | * Random.boolean()
|
58 | * Random.boolean(min, max, cur)
|
59 | * Random.bool()
|
60 | * Random.bool(min, max, cur)
|
61 |
|
62 | `Random.bool(min, max, cur)` 是 `Random.boolean(min, max, cur)` 的简写。在数据模板中,即可以使用(推荐) `BOOLEAN(min, max, cur)`,也可以使用 `BOOL(min, max, cur)`。
|
63 |
|
64 | 参数的含义和默认值如下所示:
|
65 |
|
66 | * 参数 min:可选。指示参数 cur 出现的概率。概率计算公式为 `min / (min + max)`。该参数的默认值为 1,即有 50% 的概率返回参数 cur。
|
67 | * 参数 max:可选。指示参数 cur 的相反值(!cur)出现的概率。概率计算公式为 `max / (min + max)`。该参数的默认值为 1,即有 50% 的概率返回参数 cur。
|
68 | * 参数 cur:可选。可选值为布尔值 true 或 false。如果未传入任何参数,则返回 true 和 false 的概率各为 50%。该参数没有默认值,在该方法的内部,依据原生方法 Math.random() 返回的(浮点)数来计算和返回布尔值,例如在最简单的情况下,返回值是表达式 `Math.random() >= 0.5` 的执行结果。
|
69 |
|
70 | 使用示例如下所示:
|
71 |
|
72 | var Random = Mock.Random;
|
73 | Random.boolean()
|
74 | // => true
|
75 | Random.boolean(1, 9, true)
|
76 | // => false
|
77 | Random.bool()
|
78 | // => false
|
79 | Random.bool(1, 9, false)
|
80 | // => true
|
81 |
|
82 | > 事实上,原生方法 Math.random() 返回的随机(浮点)数的分布并不均匀,是货真价实的伪随机数,将来会替换为基于 ?? 来生成随机数。?? 对 Math.random() 的实现机制进行了分析和统计,并提供了随机数的参考实现,可以访问[这里](http://??)。
|
83 | TODO 统计
|
84 |
|
85 | */
|
86 | boolean: function(min, max, cur) {
|
87 | if (cur !== undefined) {
|
88 | min = typeof min !== 'undefined' && !isNaN(min) ? parseInt(min, 10) : 1
|
89 | max = typeof max !== 'undefined' && !isNaN(max) ? parseInt(max, 10) : 1
|
90 | return Math.random() > 1.0 / (min + max) * min ? !cur : cur
|
91 | }
|
92 |
|
93 | return Math.random() >= 0.5
|
94 | },
|
95 | bool: function(min, max, cur) {
|
96 | return this.boolean(min, max, cur)
|
97 | },
|
98 | /*
|
99 | ##### Random.natural(min, max)
|
100 |
|
101 | 返回一个随机的自然数(大于等于 0 的整数)。
|
102 |
|
103 | * Random.natural()
|
104 | * Random.natural(min)
|
105 | * Random.natural(min, max)
|
106 |
|
107 | 参数的含义和默认值如下所示:
|
108 | * 参数 min:可选。指示随机自然数的最小值。默认值为 0。
|
109 | * 参数 max:可选。指示随机自然数的最小值。默认值为 9007199254740992。
|
110 |
|
111 | 使用示例如下所示:
|
112 |
|
113 | var Random = Mock.Random;
|
114 | Random.natural()
|
115 | // => 1002794054057984
|
116 | Random.natural(10000)
|
117 | // => 71529071126209
|
118 | Random.natural(60, 100)
|
119 | // => 77
|
120 | */
|
121 | natural: function(min, max) {
|
122 | min = typeof min !== 'undefined' ? parseInt(min, 10) : 0
|
123 | max = typeof max !== 'undefined' ? parseInt(max, 10) : 9007199254740992 // 2^53
|
124 | return Math.round(Math.random() * (max - min)) + min
|
125 | },
|
126 | /*
|
127 | ##### Random.integer(min, max)
|
128 |
|
129 | 返回一个随机的整数。
|
130 |
|
131 | * Random.integer()
|
132 | * Random.integer(min)
|
133 | * Random.integer(min, max)
|
134 |
|
135 | 参数含义和默认值如下所示:
|
136 | * 参数 min:可选。指示随机整数的最小值。默认值为 -9007199254740992。
|
137 | * 参数 max:可选。指示随机整数的最大值。默认值为 9007199254740992。
|
138 |
|
139 | 使用示例如下所示:
|
140 | Random.integer()
|
141 | // => -3815311811805184
|
142 | Random.integer(10000)
|
143 | // => 4303764511003750
|
144 | Random.integer(60,100)
|
145 | // => 96
|
146 | */
|
147 | integer: function(min, max) {
|
148 | min = typeof min !== 'undefined' ? parseInt(min, 10) : -9007199254740992
|
149 | max = typeof max !== 'undefined' ? parseInt(max, 10) : 9007199254740992 // 2^53
|
150 | return Math.round(Math.random() * (max - min)) + min
|
151 | },
|
152 | int: function(min, max) {
|
153 | return this.integer(min, max)
|
154 | },
|
155 | /*
|
156 | ##### Random.float(min, max, dmin, dmax)
|
157 | 返回一个随机的浮点数。
|
158 |
|
159 | * Random.float()
|
160 | * Random.float(min)
|
161 | * Random.float(min, max)
|
162 | * Random.float(min, max, dmin)
|
163 | * Random.float(min, max, dmin, dmax)
|
164 |
|
165 | 参数的含义和默认值如下所示:
|
166 | * min:可选。整数部分的最小值。默认值为 -9007199254740992。
|
167 | * max:可选。整数部分的最大值。默认值为 9007199254740992。
|
168 | * dmin:可选。小数部分位数的最小值。默认值为 0。
|
169 | * dmin:可选。小数部分位数的最大值。默认值为 17。
|
170 |
|
171 | 使用示例如下所示:
|
172 | Random.float()
|
173 | // => -1766114241544192.8
|
174 | Random.float(0)
|
175 | // => 556530504040448.25
|
176 | Random.float(60, 100)
|
177 | // => 82.56779679549358
|
178 | Random.float(60, 100, 3)
|
179 | // => 61.718533677927894
|
180 | Random.float(60, 100, 3, 5)
|
181 | // => 70.6849
|
182 | */
|
183 | float: function(min, max, dmin, dmax) {
|
184 | dmin = dmin === undefined ? 0 : dmin
|
185 | dmin = Math.max(Math.min(dmin, 17), 0)
|
186 | dmax = dmax === undefined ? 17 : dmax
|
187 | dmax = Math.max(Math.min(dmax, 17), 0)
|
188 | var ret = this.integer(min, max) + '.';
|
189 | for (var i = 0, dcount = this.natural(dmin, dmax); i < dcount; i++) {
|
190 | ret += this.character('number')
|
191 | }
|
192 | return parseFloat(ret, 10)
|
193 | },
|
194 | /*
|
195 | ##### Random.character(pool)
|
196 |
|
197 | 返回一个随机字符。
|
198 |
|
199 | * Random.character()
|
200 | * Random.character('lower/upper/number/symbol')
|
201 | * Random.character(pool)
|
202 |
|
203 | 参数的含义和默认值如下所示:
|
204 | * 参数 pool:可选。表示字符池,将从中选择一个字符返回。
|
205 | * 如果传入 'lower'、'upper'、'number'、'symbol',表示从内置的字符池从选取:
|
206 |
|
207 | {
|
208 | lower: "abcdefghijklmnopqrstuvwxyz",
|
209 | upper: "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
|
210 | number: "0123456789",
|
211 | symbol: "!@#$%^&*()[]"
|
212 | }
|
213 |
|
214 | * 如果未传入该参数,则从 `'lower' + 'upper' + 'number' + 'symbol'` 中随机选取一个字符返回。
|
215 |
|
216 | 使用示例如下所示:
|
217 |
|
218 | Random.character()
|
219 | // => "P"
|
220 | Random.character('lower')
|
221 | // => "y"
|
222 | Random.character('upper')
|
223 | // => "X"
|
224 | Random.character('number')
|
225 | // => "1"
|
226 | Random.character('symbol')
|
227 | // => "&"
|
228 | Random.character('aeiou')
|
229 | // => "u"
|
230 | */
|
231 | character: function(pool) {
|
232 | var pools = {
|
233 | lower: "abcdefghijklmnopqrstuvwxyz",
|
234 | upper: "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
|
235 | number: "0123456789",
|
236 | symbol: "!@#$%^&*()[]"
|
237 | }
|
238 | pools.alpha = pools.lower + pools.upper
|
239 | pools['undefined'] = pools.lower + pools.upper + pools.number + pools.symbol
|
240 |
|
241 | pool = pools[('' + pool).toLowerCase()] || pool
|
242 | return pool.charAt(Random.natural(0, pool.length - 1))
|
243 | },
|
244 | char: function(pool) {
|
245 | return this.character(pool)
|
246 | },
|
247 | /*
|
248 | ##### Random.string(pool, min, max)
|
249 |
|
250 | 返回一个随机字符串。
|
251 |
|
252 | * Random.string()
|
253 | * Random.string( length )
|
254 | * Random.string( pool, length )
|
255 | * Random.string( min, max )
|
256 | * Random.string( pool, min, max )
|
257 |
|
258 | 参数的含义和默认如下所示:
|
259 | * 参数 pool:可选。表示字符池,将从中选择一个字符返回。
|
260 | * 如果传入 'lower'、'upper'、'number'、'symbol',表示从内置的字符池从选取:
|
261 |
|
262 | {
|
263 | lower: "abcdefghijklmnopqrstuvwxyz",
|
264 | upper: "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
|
265 | number: "0123456789",
|
266 | symbol: "!@#$%^&*()[]"
|
267 | }
|
268 |
|
269 | * 如果未传入该参数,则从 `'lower' + 'upper' + 'number' + 'symbol'` 中随机选取一个字符返回。
|
270 | * 参数 min:可选。随机字符串的最小长度。默认值为 3。
|
271 | * 参数 max:可选。随机字符串的最大长度。默认值为 7。
|
272 |
|
273 | 使用示例如下所示:
|
274 |
|
275 | Random.string()
|
276 | // => "pJjDUe"
|
277 | Random.string( 5 )
|
278 | // => "GaadY"
|
279 | Random.string( 'lower', 5 )
|
280 | // => "jseqj"
|
281 | Random.string( 7, 10 )
|
282 | // => "UuGQgSYk"
|
283 | Random.string( 'aeiou', 1, 3 )
|
284 | // => "ea"
|
285 | */
|
286 | string: function(pool, min, max) {
|
287 | var length;
|
288 |
|
289 | // string( pool, min, max )
|
290 | if (arguments.length === 3) {
|
291 | length = Random.natural(min, max)
|
292 | }
|
293 | if (arguments.length === 2) {
|
294 | // string( pool, length )
|
295 | if (typeof arguments[0] === 'string') {
|
296 | length = min
|
297 | } else {
|
298 | // string( min, max )
|
299 | length = Random.natural(pool, min)
|
300 | pool = undefined
|
301 | }
|
302 | }
|
303 | // string( length )
|
304 | if (arguments.length === 1) {
|
305 | length = pool
|
306 | pool = undefined
|
307 | }
|
308 | if (arguments.length === 0) {
|
309 | length = Random.natural(3, 7)
|
310 | }
|
311 |
|
312 | var text = ''
|
313 | for (var i = 0; i < length; i++) {
|
314 | text += Random.character(pool)
|
315 | }
|
316 | return text
|
317 | },
|
318 | str: function(pool, min, max) {
|
319 | return this.string(pool, min, max)
|
320 | },
|
321 | /*
|
322 | ##### Random.range(start, stop, step)
|
323 |
|
324 | 返回一个整型数组。
|
325 |
|
326 | * Random.range(stop)
|
327 | * Random.range(start, stop)
|
328 | * Random.range(start, stop, step)
|
329 |
|
330 | 参数的含义和默认值如下所示:
|
331 | * 参数 start:必选。数组中整数的起始值。
|
332 | * 参数 stop:可选。数组中整数的结束值(不包含在返回值中)。
|
333 | * 参数 step:可选。数组中整数之间的步长。默认值为 1。
|
334 |
|
335 | 使用示例如下所示:
|
336 |
|
337 | Random.range(10)
|
338 | // => [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
|
339 | Random.range(3, 7)
|
340 | // => [3, 4, 5, 6]
|
341 | Random.range(1, 10, 2)
|
342 | // => [1, 3, 5, 7, 9]
|
343 | Random.range(1, 10, 3)
|
344 | // => [1, 4, 7]
|
345 |
|
346 | Generate an integer Array containing an arithmetic progression.
|
347 | http://underscorejs.org/#range
|
348 | */
|
349 | range: function(start, stop, step) {
|
350 | if (arguments.length <= 1) {
|
351 | stop = start || 0;
|
352 | start = 0;
|
353 | }
|
354 | step = arguments[2] || 1;
|
355 |
|
356 | start = +start, stop = +stop, step = +step
|
357 |
|
358 | var len = Math.max(Math.ceil((stop - start) / step), 0);
|
359 | var idx = 0;
|
360 | var range = new Array(len);
|
361 |
|
362 | while (idx < len) {
|
363 | range[idx++] = start;
|
364 | start += step;
|
365 | }
|
366 |
|
367 | return range;
|
368 | }
|
369 | })
|
370 | /*
|
371 | #### Date
|
372 | */
|
373 | Random.extend({
|
374 | patternLetters: {
|
375 | yyyy: 'getFullYear',
|
376 | yy: function(date) {
|
377 | return ('' + date.getFullYear()).slice(2)
|
378 | },
|
379 | y: 'yy',
|
380 |
|
381 | MM: function(date) {
|
382 | var m = date.getMonth() + 1
|
383 | return m < 10 ? '0' + m : m
|
384 | },
|
385 | M: function(date) {
|
386 | return date.getMonth() + 1
|
387 | },
|
388 |
|
389 | dd: function(date) {
|
390 | var d = date.getDate()
|
391 | return d < 10 ? '0' + d : d
|
392 | },
|
393 | d: 'getDate',
|
394 |
|
395 | HH: function(date) {
|
396 | var h = date.getHours()
|
397 | return h < 10 ? '0' + h : h
|
398 | },
|
399 | H: 'getHours',
|
400 | hh: function(date) {
|
401 | var h = date.getHours() % 12
|
402 | return h < 10 ? '0' + h : h
|
403 | },
|
404 | h: function(date) {
|
405 | return date.getHours() % 12
|
406 | },
|
407 |
|
408 | mm: function(date) {
|
409 | var m = date.getMinutes()
|
410 | return m < 10 ? '0' + m : m
|
411 | },
|
412 | m: 'getMinutes',
|
413 |
|
414 | ss: function(date) {
|
415 | var s = date.getSeconds()
|
416 | return s < 10 ? '0' + s : s
|
417 | },
|
418 | s: 'getSeconds',
|
419 |
|
420 | SS: function(date) {
|
421 | var ms = date.getMilliseconds()
|
422 | return ms < 10 && '00' + ms || ms < 100 && '0' + ms || ms
|
423 | },
|
424 | S: 'getMilliseconds',
|
425 |
|
426 | A: function(date) {
|
427 | return date.getHours() < 12 ? 'AM' : 'PM'
|
428 | },
|
429 | a: function(date) {
|
430 | return date.getHours() < 12 ? 'am' : 'pm'
|
431 | },
|
432 | T: 'getTime'
|
433 | }
|
434 | })
|
435 | Random.extend({
|
436 | rformat: new RegExp((function() {
|
437 | var re = []
|
438 | for (var i in Random.patternLetters) re.push(i)
|
439 | return '(' + re.join('|') + ')'
|
440 | })(), 'g'),
|
441 | /*
|
442 | ##### Random.format(date, format)
|
443 |
|
444 | 格式化日期。
|
445 | */
|
446 | format: function(date, format) {
|
447 | var patternLetters = Random.patternLetters,
|
448 | rformat = Random.rformat
|
449 | return format.replace(rformat, function($0, flag) {
|
450 | return typeof patternLetters[flag] === 'function' ?
|
451 | patternLetters[flag](date) :
|
452 | patternLetters[flag] in patternLetters ?
|
453 | arguments.callee($0, patternLetters[flag]) :
|
454 | date[patternLetters[flag]]()
|
455 | })
|
456 | },
|
457 | /*
|
458 | ##### Random.format(date, format)
|
459 |
|
460 | 生成一个随机的 Date 对象。
|
461 | */
|
462 | randomDate: function(min, max) { // min, max
|
463 | min = min === undefined ? new Date(0) : min
|
464 | max = max === undefined ? new Date() : max
|
465 | return new Date(Math.random() * (max.getTime() - min.getTime()))
|
466 | },
|
467 | /*
|
468 | ##### Random.date(format)
|
469 |
|
470 | 返回一个随机的日期字符串。
|
471 |
|
472 | * Random.date()
|
473 | * Random.date(format)
|
474 |
|
475 | 参数的含义和默认值如下所示:
|
476 |
|
477 | * 参数 format:可选。指示生成的日期字符串的格式。默认值为 `yyyy-MM-dd`。可选的占位符参考自 [Ext.Date](http://docs.sencha.com/ext-js/4-1/#!/api/Ext.Date),如下所示:
|
478 |
|
479 | Format Description Example
|
480 | ------- ----------------------------------------------------------- -------
|
481 | yyyy A full numeric representation of a year, 4 digits 1999 or 2003
|
482 | yy A two digit representation of a year 99 or 03
|
483 | y A two digit representation of a year 99 or 03
|
484 | MM Numeric representation of a month, with leading zeros 01 to 12
|
485 | M Numeric representation of a month, without leading zeros 1 to 12
|
486 | dd Day of the month, 2 digits with leading zeros 01 to 31
|
487 | d Day of the month without leading zeros 1 to 31
|
488 | HH 24-hour format of an hour with leading zeros 00 to 23
|
489 | H 24-hour format of an hour without leading zeros 0 to 23
|
490 | hh 12-hour format of an hour without leading zeros 1 to 12
|
491 | h 12-hour format of an hour with leading zeros 01 to 12
|
492 | mm Minutes, with leading zeros 00 to 59
|
493 | m Minutes, without leading zeros 0 to 59
|
494 | ss Seconds, with leading zeros 00 to 59
|
495 | s Seconds, without leading zeros 0 to 59
|
496 | SS Milliseconds, with leading zeros 000 to 999
|
497 | S Milliseconds, without leading zeros 0 to 999
|
498 | A Uppercase Ante meridiem and Post meridiem AM or PM
|
499 | a Lowercase Ante meridiem and Post meridiem am or pm
|
500 |
|
501 | 使用示例如下所示:
|
502 |
|
503 | Random.date()
|
504 | // => "2002-10-23"
|
505 | Random.date('yyyy-MM-dd')
|
506 | // => "1983-01-29"
|
507 | Random.date('yy-MM-dd')
|
508 | // => "79-02-14"
|
509 | Random.date('y-MM-dd')
|
510 | // => "81-05-17"
|
511 | Random.date('y-M-d')
|
512 | // => "84-6-5"
|
513 |
|
514 | */
|
515 | date: function(format) {
|
516 | format = format || 'yyyy-MM-dd'
|
517 | return this.format(this.randomDate(), format)
|
518 | },
|
519 | /*
|
520 | ##### Random.time(format)
|
521 |
|
522 | 返回一个随机的时间字符串。
|
523 |
|
524 | * Random.time()
|
525 | * Random.time(format)
|
526 |
|
527 | 参数的含义和默认值如下所示:
|
528 |
|
529 | * 参数 format:可选。指示生成的时间字符串的格式。默认值为 `HH:mm:ss`。可选的占位符参考自 [Ext.Date](http://docs.sencha.com/ext-js/4-1/#!/api/Ext.Date),请参见 [Random.date(format)](#Random.date(format))。
|
530 |
|
531 | 使用示例如下所示:
|
532 |
|
533 | Random.time()
|
534 | // => "00:14:47"
|
535 | Random.time('A HH:mm:ss')
|
536 | // => "PM 20:47:37"
|
537 | Random.time('a HH:mm:ss')
|
538 | // => "pm 17:40:00"
|
539 | Random.time('HH:mm:ss')
|
540 | // => "03:57:53"
|
541 | Random.time('H:m:s')
|
542 | // => "3:5:13"
|
543 | */
|
544 | time: function(format) {
|
545 | format = format || 'HH:mm:ss'
|
546 | return this.format(this.randomDate(), format)
|
547 | },
|
548 | /*
|
549 | ##### Random.datetime(format)
|
550 |
|
551 | 返回一个随机的日期和时间字符串。
|
552 |
|
553 | * Random.datetime()
|
554 | * Random.datetime(format)
|
555 |
|
556 | 参数的含义和默认值如下所示:
|
557 |
|
558 | * 参数 format:可选。指示生成的日期和时间字符串的格式。默认值为 `yyyy-MM-dd HH:mm:ss`。可选的占位符参考自 [Ext.Date](http://docs.sencha.com/ext-js/4-1/#!/api/Ext.Date),请参见 [Random.date(format)](#Random.date(format))。
|
559 |
|
560 | 使用示例如下所示:
|
561 |
|
562 | Random.datetime()
|
563 | // => "1977-11-17 03:50:15"
|
564 | Random.datetime('yyyy-MM-dd A HH:mm:ss')
|
565 | // => "1976-04-24 AM 03:48:25"
|
566 | Random.datetime('yy-MM-dd a HH:mm:ss')
|
567 | // => "73-01-18 pm 22:12:32"
|
568 | Random.datetime('y-MM-dd HH:mm:ss')
|
569 | // => "79-06-24 04:45:16"
|
570 | Random.datetime('y-M-d H:m:s')
|
571 | // => "02-4-23 2:49:40"
|
572 | */
|
573 | datetime: function(format) {
|
574 | format = format || 'yyyy-MM-dd HH:mm:ss'
|
575 | return this.format(this.randomDate(), format)
|
576 | },
|
577 | /*
|
578 | Ranndom.now(unit, format)
|
579 | Ranndom.now()
|
580 | Ranndom.now(unit)
|
581 | Ranndom.now(format)
|
582 |
|
583 | 参考自 http://momentjs.cn/docs/#/manipulating/start-of/
|
584 | */
|
585 | now: function(unit, format) {
|
586 | if (arguments.length === 1) {
|
587 | if (!/year|month|week|day|hour|minute|second|week/.test(unit)) {
|
588 | format = unit
|
589 | unit = ''
|
590 | }
|
591 | }
|
592 | unit = (unit || '').toLowerCase()
|
593 | format = format || 'yyyy-MM-dd HH:mm:ss'
|
594 |
|
595 | var date = new Date()
|
596 | /* jshint -W086 */
|
597 | switch (unit) {
|
598 | case 'year':
|
599 | date.setMonth(0)
|
600 | case 'month':
|
601 | date.setDate(1)
|
602 | case 'week':
|
603 | case 'day':
|
604 | date.setHours(0)
|
605 | case 'hour':
|
606 | date.setMinutes(0)
|
607 | case 'minute':
|
608 | date.setSeconds(0)
|
609 | case 'second':
|
610 | date.setMilliseconds(0)
|
611 | }
|
612 | switch (unit) {
|
613 | case 'week':
|
614 | date.setDate(date.getDate() - date.getDay())
|
615 | }
|
616 |
|
617 | return this.format(date, format)
|
618 | }
|
619 | })
|
620 | /*
|
621 | #### Image
|
622 | */
|
623 | Random.extend({
|
624 | ad_size: [
|
625 | '300x250', '250x250', '240x400', '336x280', '180x150',
|
626 | '720x300', '468x60', '234x60', '88x31', '120x90',
|
627 | '120x60', '120x240', '125x125', '728x90', '160x600',
|
628 | '120x600', '300x600'
|
629 | ],
|
630 | screen_size: [
|
631 | '320x200', '320x240', '640x480', '800x480', '800x480',
|
632 | '1024x600', '1024x768', '1280x800', '1440x900', '1920x1200',
|
633 | '2560x1600'
|
634 | ],
|
635 | video_size: ['720x480', '768x576', '1280x720', '1920x1080'],
|
636 | /*
|
637 | ##### Random.img(size, background, foreground, format, text)
|
638 |
|
639 | * Random.img()
|
640 | * Random.img(size)
|
641 | * Random.img(size, background)
|
642 | * Random.img(size, background, text)
|
643 | * Random.img(size, background, foreground, text)
|
644 | * Random.img(size, background, foreground, format, text)
|
645 |
|
646 | 生成一个随机的图片地址。
|
647 |
|
648 | **参数的含义和默认值**如下所示:
|
649 |
|
650 | * 参数 size:可选。指示图片的宽高,格式为 `'宽x高'`。默认从下面的数组中随机读取一个:
|
651 |
|
652 | [
|
653 | '300x250', '250x250', '240x400', '336x280',
|
654 | '180x150', '720x300', '468x60', '234x60',
|
655 | '88x31', '120x90', '120x60', '120x240',
|
656 | '125x125', '728x90', '160x600', '120x600',
|
657 | '300x600'
|
658 | ]
|
659 |
|
660 | * 参数 background:可选。指示图片的背景色。默认值为 '#000000'。
|
661 | * 参数 foreground:可选。指示图片的前景色(文件)。默认值为 '#FFFFFF'。
|
662 | * 参数 format:可选。指示图片的格式。默认值为 'png',可选值包括:'png'、'gif'、'jpg'。
|
663 | * 参数 text:可选。指示图片上文字。默认为 ''。
|
664 |
|
665 | **使用示例**如下所示:
|
666 |
|
667 | Random.img()
|
668 | // => "http://dummyimage.com/125x125"
|
669 | Random.img('200x100')
|
670 | // => "http://dummyimage.com/200x100"
|
671 | Random.img('200x100', '#fb0a2a')
|
672 | // => "http://dummyimage.com/200x100/fb0a2a"
|
673 | Random.img('200x100', '#02adea', 'hello')
|
674 | // => "http://dummyimage.com/200x100/02adea&text=hello"
|
675 | Random.img('200x100', '#00405d', '#FFF', 'mock')
|
676 | // => "http://dummyimage.com/200x100/00405d/FFF&text=mock"
|
677 | Random.img('200x100', '#ffcc33', '#FFF', 'png', 'js')
|
678 | // => "http://dummyimage.com/200x100/ffcc33/FFF.png&text=js"
|
679 |
|
680 | 生成的路径所对应的图片如下所示:
|
681 |
|
682 | 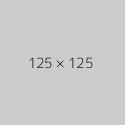
|
683 | 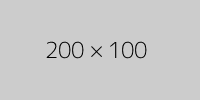
|
684 | 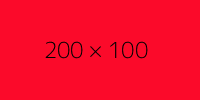
|
685 | 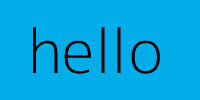
|
686 | 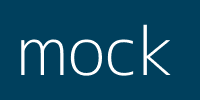
|
687 | 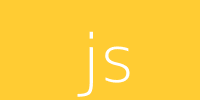
|
688 | */
|
689 | image: function(size, background, foreground, format, text) {
|
690 | if (arguments.length === 4) {
|
691 | text = format
|
692 | format = undefined
|
693 | }
|
694 | if (arguments.length === 3) {
|
695 | text = foreground
|
696 | foreground = undefined
|
697 | }
|
698 | if (!size) size = this.pick(this.ad_size)
|
699 |
|
700 | if (background && ~background.indexOf('#')) background = background.slice(1)
|
701 | if (foreground && ~foreground.indexOf('#')) foreground = foreground.slice(1)
|
702 |
|
703 | // http://dummyimage.com/600x400/cc00cc/470047.png&text=hello
|
704 | return 'http://dummyimage.com/' + size +
|
705 | (background ? '/' + background : '') +
|
706 | (foreground ? '/' + foreground : '') +
|
707 | (format ? '.' + format : '') +
|
708 | (text ? '&text=' + text : '')
|
709 | },
|
710 | img: function() {
|
711 | return this.image.apply(this, arguments)
|
712 | }
|
713 | })
|
714 | Random.extend({
|
715 | /*
|
716 | BrandColors
|
717 | http://brandcolors.net/
|
718 | A collection of major brand color codes curated by Galen Gidman.
|
719 | 大牌公司的颜色集合
|
720 |
|
721 | // 获取品牌和颜色
|
722 | $('h2').each(function(index, item){
|
723 | item = $(item)
|
724 | console.log('\'' + item.text() + '\'', ':', '\'' + item.next().text() + '\'', ',')
|
725 | })
|
726 | */
|
727 | brandColors: {
|
728 | '4ormat': '#fb0a2a',
|
729 | '500px': '#02adea',
|
730 | 'About.me (blue)': '#00405d',
|
731 | 'About.me (yellow)': '#ffcc33',
|
732 | 'Addvocate': '#ff6138',
|
733 | 'Adobe': '#ff0000',
|
734 | 'Aim': '#fcd20b',
|
735 | 'Amazon': '#e47911',
|
736 | 'Android': '#a4c639',
|
737 | 'Angie\'s List': '#7fbb00',
|
738 | 'AOL': '#0060a3',
|
739 | 'Atlassian': '#003366',
|
740 | 'Behance': '#053eff',
|
741 | 'Big Cartel': '#97b538',
|
742 | 'bitly': '#ee6123',
|
743 | 'Blogger': '#fc4f08',
|
744 | 'Boeing': '#0039a6',
|
745 | 'Booking.com': '#003580',
|
746 | 'Carbonmade': '#613854',
|
747 | 'Cheddar': '#ff7243',
|
748 | 'Code School': '#3d4944',
|
749 | 'Delicious': '#205cc0',
|
750 | 'Dell': '#3287c1',
|
751 | 'Designmoo': '#e54a4f',
|
752 | 'Deviantart': '#4e6252',
|
753 | 'Designer News': '#2d72da',
|
754 | 'Devour': '#fd0001',
|
755 | 'DEWALT': '#febd17',
|
756 | 'Disqus (blue)': '#59a3fc',
|
757 | 'Disqus (orange)': '#db7132',
|
758 | 'Dribbble': '#ea4c89',
|
759 | 'Dropbox': '#3d9ae8',
|
760 | 'Drupal': '#0c76ab',
|
761 | 'Dunked': '#2a323a',
|
762 | 'eBay': '#89c507',
|
763 | 'Ember': '#f05e1b',
|
764 | 'Engadget': '#00bdf6',
|
765 | 'Envato': '#528036',
|
766 | 'Etsy': '#eb6d20',
|
767 | 'Evernote': '#5ba525',
|
768 | 'Fab.com': '#dd0017',
|
769 | 'Facebook': '#3b5998',
|
770 | 'Firefox': '#e66000',
|
771 | 'Flickr (blue)': '#0063dc',
|
772 | 'Flickr (pink)': '#ff0084',
|
773 | 'Forrst': '#5b9a68',
|
774 | 'Foursquare': '#25a0ca',
|
775 | 'Garmin': '#007cc3',
|
776 | 'GetGlue': '#2d75a2',
|
777 | 'Gimmebar': '#f70078',
|
778 | 'GitHub': '#171515',
|
779 | 'Google Blue': '#0140ca',
|
780 | 'Google Green': '#16a61e',
|
781 | 'Google Red': '#dd1812',
|
782 | 'Google Yellow': '#fcca03',
|
783 | 'Google+': '#dd4b39',
|
784 | 'Grooveshark': '#f77f00',
|
785 | 'Groupon': '#82b548',
|
786 | 'Hacker News': '#ff6600',
|
787 | 'HelloWallet': '#0085ca',
|
788 | 'Heroku (light)': '#c7c5e6',
|
789 | 'Heroku (dark)': '#6567a5',
|
790 | 'HootSuite': '#003366',
|
791 | 'Houzz': '#73ba37',
|
792 | 'HTML5': '#ec6231',
|
793 | 'IKEA': '#ffcc33',
|
794 | 'IMDb': '#f3ce13',
|
795 | 'Instagram': '#3f729b',
|
796 | 'Intel': '#0071c5',
|
797 | 'Intuit': '#365ebf',
|
798 | 'Kickstarter': '#76cc1e',
|
799 | 'kippt': '#e03500',
|
800 | 'Kodery': '#00af81',
|
801 | 'LastFM': '#c3000d',
|
802 | 'LinkedIn': '#0e76a8',
|
803 | 'Livestream': '#cf0005',
|
804 | 'Lumo': '#576396',
|
805 | 'Mixpanel': '#a086d3',
|
806 | 'Meetup': '#e51937',
|
807 | 'Nokia': '#183693',
|
808 | 'NVIDIA': '#76b900',
|
809 | 'Opera': '#cc0f16',
|
810 | 'Path': '#e41f11',
|
811 | 'PayPal (dark)': '#1e477a',
|
812 | 'PayPal (light)': '#3b7bbf',
|
813 | 'Pinboard': '#0000e6',
|
814 | 'Pinterest': '#c8232c',
|
815 | 'PlayStation': '#665cbe',
|
816 | 'Pocket': '#ee4056',
|
817 | 'Prezi': '#318bff',
|
818 | 'Pusha': '#0f71b4',
|
819 | 'Quora': '#a82400',
|
820 | 'QUOTE.fm': '#66ceff',
|
821 | 'Rdio': '#008fd5',
|
822 | 'Readability': '#9c0000',
|
823 | 'Red Hat': '#cc0000',
|
824 | 'Resource': '#7eb400',
|
825 | 'Rockpack': '#0ba6ab',
|
826 | 'Roon': '#62b0d9',
|
827 | 'RSS': '#ee802f',
|
828 | 'Salesforce': '#1798c1',
|
829 | 'Samsung': '#0c4da2',
|
830 | 'Shopify': '#96bf48',
|
831 | 'Skype': '#00aff0',
|
832 | 'Snagajob': '#f47a20',
|
833 | 'Softonic': '#008ace',
|
834 | 'SoundCloud': '#ff7700',
|
835 | 'Space Box': '#f86960',
|
836 | 'Spotify': '#81b71a',
|
837 | 'Sprint': '#fee100',
|
838 | 'Squarespace': '#121212',
|
839 | 'StackOverflow': '#ef8236',
|
840 | 'Staples': '#cc0000',
|
841 | 'Status Chart': '#d7584f',
|
842 | 'Stripe': '#008cdd',
|
843 | 'StudyBlue': '#00afe1',
|
844 | 'StumbleUpon': '#f74425',
|
845 | 'T-Mobile': '#ea0a8e',
|
846 | 'Technorati': '#40a800',
|
847 | 'The Next Web': '#ef4423',
|
848 | 'Treehouse': '#5cb868',
|
849 | 'Trulia': '#5eab1f',
|
850 | 'Tumblr': '#34526f',
|
851 | 'Twitch.tv': '#6441a5',
|
852 | 'Twitter': '#00acee',
|
853 | 'TYPO3': '#ff8700',
|
854 | 'Ubuntu': '#dd4814',
|
855 | 'Ustream': '#3388ff',
|
856 | 'Verizon': '#ef1d1d',
|
857 | 'Vimeo': '#86c9ef',
|
858 | 'Vine': '#00a478',
|
859 | 'Virb': '#06afd8',
|
860 | 'Virgin Media': '#cc0000',
|
861 | 'Wooga': '#5b009c',
|
862 | 'WordPress (blue)': '#21759b',
|
863 | 'WordPress (orange)': '#d54e21',
|
864 | 'WordPress (grey)': '#464646',
|
865 | 'Wunderlist': '#2b88d9',
|
866 | 'XBOX': '#9bc848',
|
867 | 'XING': '#126567',
|
868 | 'Yahoo!': '#720e9e',
|
869 | 'Yandex': '#ffcc00',
|
870 | 'Yelp': '#c41200',
|
871 | 'YouTube': '#c4302b',
|
872 | 'Zalongo': '#5498dc',
|
873 | 'Zendesk': '#78a300',
|
874 | 'Zerply': '#9dcc7a',
|
875 | 'Zootool': '#5e8b1d'
|
876 | },
|
877 | brands: function() {
|
878 | var brands = [];
|
879 | for (var b in this.brandColors) {
|
880 | brands.push(b)
|
881 | }
|
882 | return brands
|
883 | },
|
884 | /*
|
885 | https://github.com/imsky/holder
|
886 | Holder renders image placeholders entirely on the client side.
|
887 |
|
888 | dataImageHolder: function(size) {
|
889 | return 'holder.js/' + size
|
890 | },
|
891 | */
|
892 | dataImage: function(size, text) {
|
893 | var canvas = (typeof document !== 'undefined') && document.createElement('canvas'),
|
894 | ctx = canvas && canvas.getContext && canvas.getContext("2d");
|
895 | if (!canvas || !ctx) return ''
|
896 |
|
897 | if (!size) size = this.pick(this.ad_size)
|
898 | text = text !== undefined ? text : size
|
899 |
|
900 | size = size.split('x')
|
901 |
|
902 | var width = parseInt(size[0], 10),
|
903 | height = parseInt(size[1], 10),
|
904 | background = this.brandColors[this.pick(this.brands())],
|
905 | foreground = '#FFF',
|
906 | text_height = 14,
|
907 | font = 'sans-serif';
|
908 |
|
909 | canvas.width = width
|
910 | canvas.height = height
|
911 | ctx.textAlign = "center"
|
912 | ctx.textBaseline = "middle"
|
913 | ctx.fillStyle = background
|
914 | ctx.fillRect(0, 0, width, height)
|
915 | ctx.fillStyle = foreground
|
916 | ctx.font = "bold " + text_height + "px " + font
|
917 | ctx.fillText(text, (width / 2), (height / 2), width)
|
918 | return canvas.toDataURL("image/png")
|
919 | }
|
920 | })
|
921 | /*
|
922 | #### Color
|
923 | */
|
924 | Random.extend({
|
925 | /*
|
926 | ##### Random.color()
|
927 |
|
928 | 随机生成一个颜色,格式为 '#RRGGBB'。
|
929 |
|
930 | * Random.color()
|
931 |
|
932 | 使用示例如下所示:
|
933 |
|
934 | Random.color()
|
935 | // => "#3538b2"
|
936 | */
|
937 | color: function() {
|
938 | var colour = Math.floor(Math.random() * (16 * 16 * 16 * 16 * 16 * 16 - 1)).toString(16)
|
939 | colour = "#" + ("000000" + colour).slice(-6)
|
940 | return colour
|
941 | }
|
942 | })
|
943 | /*
|
944 | #### Helpers
|
945 | */
|
946 | Random.extend({
|
947 | /*
|
948 | ##### Random.capitalize(word)
|
949 |
|
950 | 把字符串的第一个字母转换为大写。
|
951 |
|
952 | * Random.capitalize(word)
|
953 |
|
954 | 使用示例如下所示:
|
955 |
|
956 | Random.capitalize('hello')
|
957 | // => "Hello"
|
958 | */
|
959 | capitalize: function(word) {
|
960 | return (word + '').charAt(0).toUpperCase() + (word + '').substr(1)
|
961 | },
|
962 | /*
|
963 | ##### Random.upper(str)
|
964 |
|
965 | 把字符串转换为大写。
|
966 |
|
967 | * Random.upper(str)
|
968 |
|
969 | 使用示例如下所示:
|
970 |
|
971 | Random.upper('hello')
|
972 | // => "HELLO"
|
973 | */
|
974 | upper: function(str) {
|
975 | return (str + '').toUpperCase()
|
976 | },
|
977 | /*
|
978 | ##### Random.lower(str)
|
979 |
|
980 | 把字符串转换为小写。
|
981 |
|
982 | 使用示例如下所示:
|
983 |
|
984 | Random.lower('HELLO')
|
985 | // => "hello"
|
986 | */
|
987 | lower: function(str) {
|
988 | return (str + '').toLowerCase()
|
989 | },
|
990 | /*
|
991 | ##### Random.pick(arr)
|
992 |
|
993 | 从数组中随机选取一个元素,并返回。
|
994 |
|
995 | * Random.pick(arr)
|
996 |
|
997 | 使用示例如下所示:
|
998 |
|
999 | Random.pick(['a', 'e', 'i', 'o', 'u'])
|
1000 | // => "o"
|
1001 | */
|
1002 | pick: function(arr) {
|
1003 | arr = arr || []
|
1004 | return arr[this.natural(0, arr.length - 1)]
|
1005 | },
|
1006 | /*
|
1007 | Given an array, scramble the order and return it.
|
1008 | ##### Random.shuffle(arr)
|
1009 |
|
1010 | 打乱数组中元素的顺序,并返回。
|
1011 |
|
1012 | * Random.shuffle(arr)
|
1013 |
|
1014 | 使用示例如下所示:
|
1015 |
|
1016 | Random.shuffle(['a', 'e', 'i', 'o', 'u'])
|
1017 | // => ["o", "u", "e", "i", "a"]
|
1018 | */
|
1019 | shuffle: function(arr) {
|
1020 | arr = arr || []
|
1021 | var old = arr.slice(0),
|
1022 | result = [],
|
1023 | index = 0,
|
1024 | length = old.length;
|
1025 | for (var i = 0; i < length; i++) {
|
1026 | index = this.natural(0, old.length - 1)
|
1027 | result.push(old[index])
|
1028 | old.splice(index, 1)
|
1029 | }
|
1030 | return result
|
1031 | }
|
1032 | })
|
1033 | /*
|
1034 | #### Text
|
1035 | */
|
1036 | Random.extend({
|
1037 | /*
|
1038 | ##### Random.paragraph(len)
|
1039 |
|
1040 | 随机生成一段文本。
|
1041 |
|
1042 | * Random.paragraph()
|
1043 | * Random.paragraph(len)
|
1044 | * Random.paragraph(min, max)
|
1045 |
|
1046 | 参数的含义和默认值如下所示:
|
1047 |
|
1048 | * 参数 len:可选。指示文本中句子的个数。默认值为 3 到 7 之间的随机数。
|
1049 | * 参数 min:可选。指示文本中句子的最小个数。默认值为 3。
|
1050 | * 参数 max:可选。指示文本中句子的最大个数。默认值为 7。
|
1051 |
|
1052 | 使用示例如下所示:
|
1053 |
|
1054 | Random.paragraph()
|
1055 | // => "Yohbjjz psxwibxd jijiccj kvemj eidnus disnrst rcconm bcjrof tpzhdo ncxc yjws jnmdmty. Dkmiwza ibudbufrnh ndmcpz tomdyh oqoonsn jhoy rueieihtt vsrjpudcm sotfqsfyv mjeat shnqmslfo oirnzu cru qmpt ggvgxwv jbu kjde. Kzegfq kigj dtzdd ngtytgm comwwoox fgtee ywdrnbam utu nyvlyiv tubouw lezpkmyq fkoa jlygdgf pgv gyerges wbykcxhwe bcpmt beqtkq. Mfxcqyh vhvpovktvl hrmsgfxnt jmnhyndk qohnlmgc sicmlnsq nwku dxtbmwrta omikpmajv qda qrn cwoyfaykxa xqnbv bwbnyov hbrskzt. Pdfqwzpb hypvtknt bovxx noramu xhzam kfb ympmebhqxw gbtaszonqo zmsdgcku mjkjc widrymjzj nytudruhfr uudsitbst cgmwewxpi bye. Eyseox wyef ikdnws weoyof dqecfwokkv svyjdyulk glusauosnu achmrakky kdcfp kujrqcq xojqbxrp mpfv vmw tahxtnw fhe lcitj."
|
1056 | Random.paragraph(2)
|
1057 | // => "Dlpec hnwvovvnq slfehkf zimy qpxqgy vwrbi mok wozddpol umkek nffjcmk gnqhhvm ztqkvjm kvukg dqubvqn xqbmoda. Vdkceijr fhhyemx hgkruvxuvr kuez wmkfv lusfksuj oewvvf cyw tfpo jswpseupm ypybap kwbofwg uuwn rvoxti ydpeeerf."
|
1058 | Random.paragraph(1, 3)
|
1059 | // => "Qdgfqm puhxle twi lbeqjqfi bcxeeecu pqeqr srsx tjlnew oqtqx zhxhkvq pnjns eblxhzzta hifj csvndh ylechtyu."
|
1060 | */
|
1061 | paragraph: function(min, max) {
|
1062 | var len;
|
1063 | if (arguments.length === 0) len = Random.natural(3, 7)
|
1064 | if (arguments.length === 1) len = max = min
|
1065 | if (arguments.length === 2) {
|
1066 | min = parseInt(min, 10)
|
1067 | max = parseInt(max, 10)
|
1068 | len = Random.natural(min, max)
|
1069 | }
|
1070 |
|
1071 | var arr = []
|
1072 | for (var i = 0; i < len; i++) {
|
1073 | arr.push(Random.sentence())
|
1074 | }
|
1075 | return arr.join(' ')
|
1076 | },
|
1077 | /*
|
1078 | ##### Random.sentence(len)
|
1079 |
|
1080 | 随机生成一个句子,第一个的单词的首字母大写。
|
1081 |
|
1082 | * Random.sentence()
|
1083 | * Random.sentence(len)
|
1084 | * Random.sentence(min, max)
|
1085 |
|
1086 | 参数的含义和默认值如下所示:
|
1087 |
|
1088 | * 参数 len:可选。指示句子中单词的个数。默认值为 12 到 18 之间的随机数。
|
1089 | * 参数 min:可选。指示句子中单词的最小个数。默认值为 12。
|
1090 | * 参数 max:可选。指示句子中单词的最大个数。默认值为 18。
|
1091 |
|
1092 | 使用示例如下所示:
|
1093 |
|
1094 | Random.sentence()
|
1095 | // => "Jovasojt qopupwh plciewh dryir zsqsvlkga yeam."
|
1096 | Random.sentence(5)
|
1097 | // => "Fwlymyyw htccsrgdk rgemfpyt cffydvvpc ycgvno."
|
1098 | Random.sentence(3, 5)
|
1099 | // => "Mgl qhrprwkhb etvwfbixm jbqmg."
|
1100 | */
|
1101 | sentence: function(min, max) {
|
1102 | var len;
|
1103 | if (arguments.length === 0) len = Random.natural(12, 18)
|
1104 | if (arguments.length === 1) len = max = min
|
1105 | if (arguments.length === 2) {
|
1106 | min = parseInt(min, 10)
|
1107 | max = parseInt(max, 10)
|
1108 | len = Random.natural(min, max)
|
1109 | }
|
1110 |
|
1111 | var arr = []
|
1112 | for (var i = 0; i < len; i++) {
|
1113 | arr.push(Random.word())
|
1114 | }
|
1115 | return Random.capitalize(arr.join(' ')) + '.'
|
1116 | },
|
1117 | /*
|
1118 | ##### Random.word(len)
|
1119 |
|
1120 | 随机生成一个单词。
|
1121 |
|
1122 | * Random.word()
|
1123 | * Random.word(len)
|
1124 | * Random.word(min, max)
|
1125 |
|
1126 | 参数的含义和默认值如下所示:
|
1127 |
|
1128 | * 参数 len:可选。指示单词中字符的个数。默认值为 3 到 10 之间的随机数。
|
1129 | * 参数 min:可选。指示单词中字符的最小个数。默认值为 3。
|
1130 | * 参数 max:可选。指示单词中字符的最大个数。默认值为 10。
|
1131 |
|
1132 | 使用示例如下所示:
|
1133 |
|
1134 | Random.word()
|
1135 | // => "fxpocl"
|
1136 | Random.word(5)
|
1137 | // => "xfqjb"
|
1138 | Random.word(3, 5)
|
1139 | // => "kemh"
|
1140 |
|
1141 | > 目前,单字中字符是随机的小写字母,未来会根据词法生成“可读”的单词。
|
1142 | */
|
1143 | word: function(min, max) {
|
1144 | var len;
|
1145 | if (arguments.length === 0) len = Random.natural(3, 10)
|
1146 | if (arguments.length === 1) len = max = min
|
1147 | if (arguments.length === 2) {
|
1148 | min = parseInt(min, 10)
|
1149 | max = parseInt(max, 10)
|
1150 | len = Random.natural(min, max)
|
1151 | }
|
1152 |
|
1153 | var result = '';
|
1154 | for (var i = 0; i < len; i++) {
|
1155 | result += Random.character('lower')
|
1156 | }
|
1157 |
|
1158 | return result
|
1159 | },
|
1160 | /*
|
1161 | ##### Random.title(len)
|
1162 |
|
1163 | 随机生成一句标题,其中每个单词的首字母大写。
|
1164 |
|
1165 | * Random.title()
|
1166 | * Random.title(len)
|
1167 | * Random.title(min, max)
|
1168 |
|
1169 | 参数的含义和默认值如下所示:
|
1170 |
|
1171 | * 参数 len:可选。指示单词中字符的个数。默认值为 3 到 7 之间的随机数。
|
1172 | * 参数 min:可选。指示单词中字符的最小个数。默认值为 3。
|
1173 | * 参数 max:可选。指示单词中字符的最大个数。默认值为 7。
|
1174 |
|
1175 | 使用示例如下所示:
|
1176 |
|
1177 | Random.title()
|
1178 | // => "Rduqzr Muwlmmlg Siekwvo Ktn Nkl Orn"
|
1179 | Random.title(5)
|
1180 | // => "Ahknzf Btpehy Xmpc Gonehbnsm Mecfec"
|
1181 | Random.title(3, 5)
|
1182 | // => "Hvjexiondr Pyickubll Owlorjvzys Xfnfwbfk"
|
1183 | */
|
1184 | title: function(min, max) {
|
1185 | var len,
|
1186 | result = [];
|
1187 |
|
1188 | if (arguments.length === 0) len = Random.natural(3, 7)
|
1189 | if (arguments.length === 1) len = max = min
|
1190 | if (arguments.length === 2) {
|
1191 | min = parseInt(min, 10)
|
1192 | max = parseInt(max, 10)
|
1193 | len = Random.natural(min, max)
|
1194 | }
|
1195 |
|
1196 | for (var i = 0; i < len; i++) {
|
1197 | result.push(this.capitalize(this.word()))
|
1198 | }
|
1199 | return result.join(' ')
|
1200 | }
|
1201 | })
|
1202 | /*
|
1203 | #### Name
|
1204 | */
|
1205 | Random.extend({
|
1206 | /*
|
1207 | ##### Random.first()
|
1208 |
|
1209 | 随机生成一个常见的英文名。
|
1210 |
|
1211 | * Random.first()
|
1212 |
|
1213 | 使用示例如下所示:
|
1214 |
|
1215 | Random.first()
|
1216 | // => "Nancy"
|
1217 | */
|
1218 | first: function() {
|
1219 | var names = [
|
1220 | // male
|
1221 | "James", "John", "Robert", "Michael", "William",
|
1222 | "David", "Richard", "Charles", "Joseph", "Thomas",
|
1223 | "Christopher", "Daniel", "Paul", "Mark", "Donald",
|
1224 | "George", "Kenneth", "Steven", "Edward", "Brian",
|
1225 | "Ronald", "Anthony", "Kevin", "Jason", "Matthew",
|
1226 | "Gary", "Timothy", "Jose", "Larry", "Jeffrey",
|
1227 | "Frank", "Scott", "Eric"
|
1228 | ].concat([
|
1229 | // female
|
1230 | "Mary", "Patricia", "Linda", "Barbara", "Elizabeth",
|
1231 | "Jennifer", "Maria", "Susan", "Margaret", "Dorothy",
|
1232 | "Lisa", "Nancy", "Karen", "Betty", "Helen",
|
1233 | "Sandra", "Donna", "Carol", "Ruth", "Sharon",
|
1234 | "Michelle", "Laura", "Sarah", "Kimberly", "Deborah",
|
1235 | "Jessica", "Shirley", "Cynthia", "Angela", "Melissa",
|
1236 | "Brenda", "Amy", "Anna"
|
1237 | ])
|
1238 | return this.pick(names)
|
1239 | return this.capitalize(this.word())
|
1240 | },
|
1241 | /*
|
1242 | ##### Random.last()
|
1243 |
|
1244 | 随机生成一个常见的英文姓。
|
1245 |
|
1246 | * Random.last()
|
1247 |
|
1248 | 使用示例如下所示:
|
1249 |
|
1250 | Random.last()
|
1251 | // => "Martinez"
|
1252 | */
|
1253 | last: function() {
|
1254 | var names = [
|
1255 | "Smith", "Johnson", "Williams", "Brown", "Jones",
|
1256 | "Miller", "Davis", "Garcia", "Rodriguez", "Wilson",
|
1257 | "Martinez", "Anderson", "Taylor", "Thomas", "Hernandez",
|
1258 | "Moore", "Martin", "Jackson", "Thompson", "White",
|
1259 | "Lopez", "Lee", "Gonzalez", "Harris", "Clark",
|
1260 | "Lewis", "Robinson", "Walker", "Perez", "Hall",
|
1261 | "Young", "Allen"
|
1262 | ]
|
1263 | return this.pick(names)
|
1264 | return this.capitalize(this.word())
|
1265 | },
|
1266 | /*
|
1267 | ##### Random.name(middle)
|
1268 |
|
1269 | 随机生成一个常见的英文姓名。
|
1270 |
|
1271 | * Random.name()
|
1272 | * Random.name(middle)
|
1273 |
|
1274 | 参数的含义和默认值如下所示:
|
1275 |
|
1276 | * 参数 middle:可选。布尔值。指示是否生成中间名。
|
1277 |
|
1278 | 使用示例如下所示:
|
1279 |
|
1280 | Random.name()
|
1281 | // => "Larry Wilson"
|
1282 | Random.name(true)
|
1283 | // => "Helen Carol Martinez"
|
1284 | */
|
1285 | name: function(middle) {
|
1286 | return this.first() + ' ' + (middle ? this.first() + ' ' : '') + this.last()
|
1287 | }
|
1288 | })
|
1289 | /*
|
1290 | #### Web
|
1291 | */
|
1292 | Random.extend({
|
1293 | /*
|
1294 | ##### Random.url()
|
1295 |
|
1296 | 随机生成一个 URL。
|
1297 |
|
1298 | * Random.url()
|
1299 |
|
1300 | 使用示例如下所示:
|
1301 |
|
1302 | Random.url()
|
1303 | // => "http://vrcq.edu/ekqtyfi"
|
1304 | */
|
1305 | url: function() {
|
1306 | return 'http://' + this.domain() + '/' + this.word()
|
1307 | },
|
1308 | /*
|
1309 | ##### Random.domain()
|
1310 |
|
1311 | 随机生成一个域名。
|
1312 |
|
1313 | * Random.domain()
|
1314 |
|
1315 | 使用示例如下所示:
|
1316 |
|
1317 | Random.domain()
|
1318 | // => "kozfnb.org"
|
1319 | */
|
1320 | domain: function(tld) {
|
1321 | return this.word() + '.' + (tld || this.tld())
|
1322 | },
|
1323 | /*
|
1324 | ##### Random.email()
|
1325 |
|
1326 | 随机生成一个邮件地址。
|
1327 |
|
1328 | * Random.email()
|
1329 |
|
1330 | 使用示例如下所示:
|
1331 |
|
1332 | Random.email()
|
1333 | // => "x.davis@jackson.edu"
|
1334 | */
|
1335 | email: function(domain) {
|
1336 | return this.character('lower') + '.' + this.last().toLowerCase() + '@' + this.last().toLowerCase() + '.' + this.tld()
|
1337 | return this.word() + '@' + (domain || this.domain())
|
1338 | },
|
1339 | /*
|
1340 | ##### Random.ip()
|
1341 |
|
1342 | 随机生成一个 IP 地址。
|
1343 |
|
1344 | * Random.ip()
|
1345 |
|
1346 | 使用示例如下所示:
|
1347 |
|
1348 | Random.ip()
|
1349 | // => "34.206.109.169"
|
1350 | */
|
1351 | ip: function() {
|
1352 | return this.natural(0, 255) + '.' +
|
1353 | this.natural(0, 255) + '.' +
|
1354 | this.natural(0, 255) + '.' +
|
1355 | this.natural(0, 255)
|
1356 | },
|
1357 | tlds: ['com', 'org', 'edu', 'gov', 'co.uk', 'net', 'io'],
|
1358 | /*
|
1359 | ##### Random.tld()
|
1360 |
|
1361 | 随机生成一个顶级域名。
|
1362 |
|
1363 | * Random.tld()
|
1364 |
|
1365 | 使用示例如下所示:
|
1366 |
|
1367 | Random.tld()
|
1368 | // => "io"
|
1369 | */
|
1370 | tld: function() { // Top Level Domain
|
1371 | return this.pick(this.tlds)
|
1372 | }
|
1373 | })
|
1374 | /*
|
1375 | #### Address
|
1376 | TODO
|
1377 | */
|
1378 | Random.extend({
|
1379 | areas: ['东北', '华北', '华东', '华中', '华南', '西南', '西北'],
|
1380 | /*
|
1381 | ##### Random.area()
|
1382 |
|
1383 | 随机生成一个大区。
|
1384 |
|
1385 | * Random.area()
|
1386 |
|
1387 | 使用示例如下所示:
|
1388 |
|
1389 | Random.area()
|
1390 | // => "华北"
|
1391 | */
|
1392 | area: function() {
|
1393 | return this.pick(this.areas)
|
1394 | },
|
1395 |
|
1396 | /*
|
1397 | > 23 个省:
|
1398 | '河北省', '山西省', '辽宁省', '吉林省', '黑龙江省', '江苏省', '浙江省', '安徽省', '福建省', '江西省', '山东省', '河南省', '湖北省', '湖南省', '广东省', '海南省', '四川省', '贵州省', '云南省', '陕西省', '甘肃省', '青海省', '台湾省',
|
1399 | > 4 个直辖市:
|
1400 | '北京市', '天津市', '上海市', '重庆市',
|
1401 | > 5 个自治区:
|
1402 | '广西壮族自治区', '内蒙古自治区', '西藏自治区', '宁夏回族自治区', '新疆维吾尔自治区',
|
1403 | > 2 个特别行政区:
|
1404 | '香港特别行政区', '澳门特别行政区'
|
1405 | */
|
1406 | regions: [
|
1407 | '110000 北京市', '120000 天津市', '130000 河北省', '140000 山西省', '150000 内蒙古自治区',
|
1408 | '210000 辽宁省', '220000 吉林省', '230000 黑龙江省',
|
1409 | '310000 上海市', '320000 江苏省', '330000 浙江省', '340000 安徽省', '350000 福建省', '360000 江西省', '370000 山东省',
|
1410 | '410000 河南省', '420000 湖北省', '430000 湖南省', '440000 广东省', '450000 广西壮族自治区', '460000 海南省',
|
1411 | '500000 重庆市', '510000 四川省', '520000 贵州省', '530000 云南省', '540000 西藏自治区',
|
1412 | '610000 陕西省', '620000 甘肃省', '630000 青海省', '640000 宁夏回族自治区', '650000 新疆维吾尔自治区', '650000 新疆维吾尔自治区',
|
1413 | '710000 台湾省',
|
1414 | '810000 香港特别行政区', '820000 澳门特别行政区'
|
1415 | ],
|
1416 | /*
|
1417 | ##### Random.region()
|
1418 |
|
1419 | 随机生成一个省(或直辖市、自治区、特别行政区)。
|
1420 |
|
1421 | * Random.region()
|
1422 |
|
1423 | 使用示例如下所示:
|
1424 |
|
1425 | Random.region()
|
1426 | // => "辽宁省"
|
1427 | */
|
1428 | region: function() {
|
1429 | return this.pick(this.regions).split(' ')[1]
|
1430 | },
|
1431 |
|
1432 |
|
1433 | address: function() {
|
1434 |
|
1435 | },
|
1436 | city: function() {
|
1437 |
|
1438 | },
|
1439 | phone: function() {
|
1440 |
|
1441 | },
|
1442 | areacode: function() {
|
1443 |
|
1444 | },
|
1445 | street: function() {
|
1446 |
|
1447 | },
|
1448 | street_suffixes: function() {
|
1449 |
|
1450 | },
|
1451 | street_suffix: function() {
|
1452 |
|
1453 | },
|
1454 | states: function() {
|
1455 |
|
1456 | },
|
1457 | state: function() {
|
1458 |
|
1459 | },
|
1460 | zip: function(len) {
|
1461 | var zip = ''
|
1462 | for (var i = 0; i < (len || 6); i++) zip += this.natural(0, 9)
|
1463 | return zip
|
1464 | }
|
1465 | })
|
1466 | // TODO ...
|
1467 | Random.extend({
|
1468 | todo: function() {
|
1469 | return 'todo'
|
1470 | }
|
1471 | })
|
1472 |
|
1473 | /*
|
1474 | #### Miscellaneous
|
1475 | */
|
1476 | Random.extend({
|
1477 | // Dice
|
1478 | d4: function() {
|
1479 | return this.natural(1, 4)
|
1480 | },
|
1481 | d6: function() {
|
1482 | return this.natural(1, 6)
|
1483 | },
|
1484 | d8: function() {
|
1485 | return this.natural(1, 8)
|
1486 | },
|
1487 | d12: function() {
|
1488 | return this.natural(1, 12)
|
1489 | },
|
1490 | d20: function() {
|
1491 | return this.natural(1, 20)
|
1492 | },
|
1493 | d100: function() {
|
1494 | return this.natural(1, 100)
|
1495 | },
|
1496 | /*
|
1497 | http://www.broofa.com/2008/09/javascript-uuid-function/
|
1498 | http://www.ietf.org/rfc/rfc4122.txt
|
1499 | http://chancejs.com/#guid
|
1500 |
|
1501 | ##### Random.guid()
|
1502 |
|
1503 | 随机生成一个 GUID。
|
1504 |
|
1505 | * Random.guid()
|
1506 |
|
1507 | 使用示例如下所示:
|
1508 |
|
1509 | Random.guid()
|
1510 | // => "662C63B4-FD43-66F4-3328-C54E3FF0D56E"
|
1511 | */
|
1512 | guid: function() {
|
1513 |
|
1514 | var pool = "ABCDEF1234567890",
|
1515 | guid = this.string(pool, 8) + '-' +
|
1516 | this.string(pool, 4) + '-' +
|
1517 | this.string(pool, 4) + '-' +
|
1518 | this.string(pool, 4) + '-' +
|
1519 | this.string(pool, 12);
|
1520 | return guid
|
1521 | },
|
1522 | /*
|
1523 | [身份证](http://baike.baidu.com/view/1697.htm#4)
|
1524 | 地址码 6 + 出生日期码 8 + 顺序码 3 + 校验码 1
|
1525 | [《中华人民共和国行政区划代码》国家标准(GB/T2260)](http://zhidao.baidu.com/question/1954561.html)
|
1526 |
|
1527 | ##### Random.id()
|
1528 |
|
1529 | 随机生成一个 18 位身份证。
|
1530 |
|
1531 | * Random.id()
|
1532 |
|
1533 | 使用示例如下所示:
|
1534 |
|
1535 | Random.id()
|
1536 | // => "420000200710091854"
|
1537 | */
|
1538 | id: function() {
|
1539 | var id,
|
1540 | sum = 0,
|
1541 | rank = [
|
1542 | "7", "9", "10", "5", "8", "4", "2", "1", "6", "3", "7", "9", "10", "5", "8", "4", "2"
|
1543 | ],
|
1544 | last = [
|
1545 | "1", "0", "X", "9", "8", "7", "6", "5", "4", "3", "2"
|
1546 | ];
|
1547 |
|
1548 | id = this.pick(this.regions).split(' ')[0] +
|
1549 | this.date('yyyyMMdd') +
|
1550 | this.string('number', 3)
|
1551 |
|
1552 | for (var i = 0; i < id.length; i++) {
|
1553 | sum += id[i] * rank[i];
|
1554 | }
|
1555 | id += last[sum % 11];
|
1556 |
|
1557 | return id
|
1558 | },
|
1559 |
|
1560 | /*
|
1561 | 自增主键
|
1562 | auto increment primary key
|
1563 |
|
1564 | ##### Random.increment()
|
1565 |
|
1566 | 生成一个全局的自增整数。
|
1567 |
|
1568 | * Random.increment(step)
|
1569 |
|
1570 | 参数的含义和默认值如下所示:
|
1571 | * 参数 step:可选。整数自增的步长。默认值为 1。
|
1572 |
|
1573 | 使用示例如下所示:
|
1574 |
|
1575 | Random.increment()
|
1576 | // => 1
|
1577 | Random.increment(100)
|
1578 | // => 101
|
1579 | Random.increment(1000)
|
1580 | // => 1101
|
1581 | */
|
1582 | autoIncrementInteger: 0,
|
1583 | increment: function(step) {
|
1584 | return this.autoIncrementInteger += (+step || 1)
|
1585 | },
|
1586 | inc: function(step) {
|
1587 | return this.increment(step)
|
1588 | }
|
1589 | })
|
1590 |
|
1591 | return Random
|
1592 | })()
|
1593 | // END(BROWSER)
|
1594 |
|
1595 | module.exports = Random |
\ | No newline at end of file |