1 | [](https://nodei.co/npm/mongodb/) [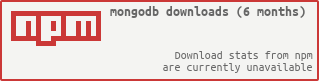](https://nodei.co/npm/mongodb/)
|
2 |
|
3 | [](http://travis-ci.org/mongodb/node-mongodb-native)
|
4 | [](https://coveralls.io/github/mongodb/node-mongodb-native?branch=2.1)
|
5 | [](https://gitter.im/mongodb/node-mongodb-native?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
|
6 |
|
7 | # Description
|
8 |
|
9 | The official [MongoDB](https://www.mongodb.com/) driver for Node.js. Provides a high-level API on top of [mongodb-core](https://www.npmjs.com/package/mongodb-core) that is meant for end users.
|
10 |
|
11 | **NOTE: v3.x was recently released with breaking API changes. You can find a list of changes [here](CHANGES_3.0.0.md).**
|
12 |
|
13 | ## MongoDB Node.JS Driver
|
14 |
|
15 | | what | where |
|
16 | |---------------|------------------------------------------------|
|
17 | | documentation | http://mongodb.github.io/node-mongodb-native |
|
18 | | api-doc | http://mongodb.github.io/node-mongodb-native/3.1/api |
|
19 | | source | https://github.com/mongodb/node-mongodb-native |
|
20 | | mongodb | http://www.mongodb.org |
|
21 |
|
22 | ### Bugs / Feature Requests
|
23 |
|
24 | Think you’ve found a bug? Want to see a new feature in `node-mongodb-native`? Please open a
|
25 | case in our issue management tool, JIRA:
|
26 |
|
27 | - Create an account and login [jira.mongodb.org](https://jira.mongodb.org).
|
28 | - Navigate to the NODE project [jira.mongodb.org/browse/NODE](https://jira.mongodb.org/browse/NODE).
|
29 | - Click **Create Issue** - Please provide as much information as possible about the issue type and how to reproduce it.
|
30 |
|
31 | Bug reports in JIRA for all driver projects (i.e. NODE, PYTHON, CSHARP, JAVA) and the
|
32 | Core Server (i.e. SERVER) project are **public**.
|
33 |
|
34 | ### Support / Feedback
|
35 |
|
36 | For issues with, questions about, or feedback for the Node.js driver, please look into our [support channels](https://docs.mongodb.com/manual/support). Please do not email any of the driver developers directly with issues or questions - you're more likely to get an answer on the [MongoDB Community Forums](https://community.mongodb.com/tags/c/drivers-odms-connectors/7/node-js-driver).
|
37 |
|
38 | ### Change Log
|
39 |
|
40 | Change history can be found in [`HISTORY.md`](HISTORY.md).
|
41 |
|
42 | ### Compatibility
|
43 |
|
44 | For version compatibility matrices, please refer to the following links:
|
45 |
|
46 | * [MongoDB](https://docs.mongodb.com/ecosystem/drivers/driver-compatibility-reference/#reference-compatibility-mongodb-node)
|
47 | * [NodeJS](https://docs.mongodb.com/ecosystem/drivers/driver-compatibility-reference/#reference-compatibility-language-node)
|
48 |
|
49 | # Installation
|
50 |
|
51 | The recommended way to get started using the Node.js 3.0 driver is by using the `npm` (Node Package Manager) to install the dependency in your project.
|
52 |
|
53 | ## MongoDB Driver
|
54 |
|
55 | Given that you have created your own project using `npm init` we install the MongoDB driver and its dependencies by executing the following `npm` command.
|
56 |
|
57 | ```bash
|
58 | npm install mongodb --save
|
59 | ```
|
60 |
|
61 | This will download the MongoDB driver and add a dependency entry in your `package.json` file.
|
62 |
|
63 | You can also use the [Yarn](https://yarnpkg.com/en) package manager.
|
64 |
|
65 | ## Troubleshooting
|
66 |
|
67 | The MongoDB driver depends on several other packages. These are:
|
68 |
|
69 | * [mongodb-core](https://github.com/mongodb-js/mongodb-core)
|
70 | * [bson](https://github.com/mongodb/js-bson)
|
71 | * [kerberos](https://github.com/mongodb-js/kerberos)
|
72 | * [node-gyp](https://github.com/nodejs/node-gyp)
|
73 |
|
74 | The `kerberos` package is a C++ extension that requires a build environment to be installed on your system. You must be able to build Node.js itself in order to compile and install the `kerberos` module. Furthermore, the `kerberos` module requires the MIT Kerberos package to correctly compile on UNIX operating systems. Consult your UNIX operation system package manager for what libraries to install.
|
75 |
|
76 | **Windows already contains the SSPI API used for Kerberos authentication. However, you will need to install a full compiler tool chain using Visual Studio C++ to correctly install the Kerberos extension.**
|
77 |
|
78 | ### Diagnosing on UNIX
|
79 |
|
80 | If you don’t have the build-essentials, this module won’t build. In the case of Linux, you will need gcc, g++, Node.js with all the headers and Python. The easiest way to figure out what’s missing is by trying to build the Kerberos project. You can do this by performing the following steps.
|
81 |
|
82 | ```bash
|
83 | git clone https://github.com/mongodb-js/kerberos
|
84 | cd kerberos
|
85 | npm install
|
86 | ```
|
87 |
|
88 | If all the steps complete, you have the right toolchain installed. If you get the error "node-gyp not found," you need to install `node-gyp` globally:
|
89 |
|
90 | ```bash
|
91 | npm install -g node-gyp
|
92 | ```
|
93 |
|
94 | If it correctly compiles and runs the tests you are golden. We can now try to install the `mongod` driver by performing the following command.
|
95 |
|
96 | ```bash
|
97 | cd yourproject
|
98 | npm install mongodb --save
|
99 | ```
|
100 |
|
101 | If it still fails the next step is to examine the npm log. Rerun the command but in this case in verbose mode.
|
102 |
|
103 | ```bash
|
104 | npm --loglevel verbose install mongodb
|
105 | ```
|
106 |
|
107 | This will print out all the steps npm is performing while trying to install the module.
|
108 |
|
109 | ### Diagnosing on Windows
|
110 |
|
111 | A compiler tool chain known to work for compiling `kerberos` on Windows is the following.
|
112 |
|
113 | * Visual Studio C++ 2010 (do not use higher versions)
|
114 | * Windows 7 64bit SDK
|
115 | * Python 2.7 or higher
|
116 |
|
117 | Open the Visual Studio command prompt. Ensure `node.exe` is in your path and install `node-gyp`.
|
118 |
|
119 | ```bash
|
120 | npm install -g node-gyp
|
121 | ```
|
122 |
|
123 | Next, you will have to build the project manually to test it. Clone the repo, install dependencies and rebuild:
|
124 |
|
125 | ```bash
|
126 | git clone https://github.com/christkv/kerberos.git
|
127 | cd kerberos
|
128 | npm install
|
129 | node-gyp rebuild
|
130 | ```
|
131 |
|
132 | This should rebuild the driver successfully if you have everything set up correctly.
|
133 |
|
134 | ### Other possible issues
|
135 |
|
136 | Your Python installation might be hosed making gyp break. Test your deployment environment first by trying to build Node.js itself on the server in question, as this should unearth any issues with broken packages (and there are a lot of broken packages out there).
|
137 |
|
138 | Another tip is to ensure your user has write permission to wherever the Node.js modules are being installed.
|
139 |
|
140 | ## Quick Start
|
141 |
|
142 | This guide will show you how to set up a simple application using Node.js and MongoDB. Its scope is only how to set up the driver and perform the simple CRUD operations. For more in-depth coverage, see the [tutorials](docs/reference/content/tutorials/main.md).
|
143 |
|
144 | ### Create the `package.json` file
|
145 |
|
146 | First, create a directory where your application will live.
|
147 |
|
148 | ```bash
|
149 | mkdir myproject
|
150 | cd myproject
|
151 | ```
|
152 |
|
153 | Enter the following command and answer the questions to create the initial structure for your new project:
|
154 |
|
155 | ```bash
|
156 | npm init
|
157 | ```
|
158 |
|
159 | Next, install the driver dependency.
|
160 |
|
161 | ```bash
|
162 | npm install mongodb --save
|
163 | ```
|
164 |
|
165 | You should see **NPM** download a lot of files. Once it's done you'll find all the downloaded packages under the **node_modules** directory.
|
166 |
|
167 | ### Start a MongoDB Server
|
168 |
|
169 | For complete MongoDB installation instructions, see [the manual](https://docs.mongodb.org/manual/installation/).
|
170 |
|
171 | 1. Download the right MongoDB version from [MongoDB](https://www.mongodb.org/downloads)
|
172 | 2. Create a database directory (in this case under **/data**).
|
173 | 3. Install and start a ``mongod`` process.
|
174 |
|
175 | ```bash
|
176 | mongod --dbpath=/data
|
177 | ```
|
178 |
|
179 | You should see the **mongod** process start up and print some status information.
|
180 |
|
181 | ### Connect to MongoDB
|
182 |
|
183 | Create a new **app.js** file and add the following code to try out some basic CRUD
|
184 | operations using the MongoDB driver.
|
185 |
|
186 | Add code to connect to the server and the database **myproject**:
|
187 |
|
188 | ```js
|
189 | const MongoClient = require('mongodb').MongoClient;
|
190 | const assert = require('assert');
|
191 |
|
192 | // Connection URL
|
193 | const url = 'mongodb://localhost:27017';
|
194 |
|
195 | // Database Name
|
196 | const dbName = 'myproject';
|
197 |
|
198 | // Use connect method to connect to the server
|
199 | MongoClient.connect(url, function(err, client) {
|
200 | assert.equal(null, err);
|
201 | console.log("Connected successfully to server");
|
202 |
|
203 | const db = client.db(dbName);
|
204 |
|
205 | client.close();
|
206 | });
|
207 | ```
|
208 |
|
209 | Run your app from the command line with:
|
210 |
|
211 | ```bash
|
212 | node app.js
|
213 | ```
|
214 |
|
215 | The application should print **Connected successfully to server** to the console.
|
216 |
|
217 | ### Insert a Document
|
218 |
|
219 | Add to **app.js** the following function which uses the **insertMany**
|
220 | method to add three documents to the **documents** collection.
|
221 |
|
222 | ```js
|
223 | const insertDocuments = function(db, callback) {
|
224 | // Get the documents collection
|
225 | const collection = db.collection('documents');
|
226 | // Insert some documents
|
227 | collection.insertMany([
|
228 | {a : 1}, {a : 2}, {a : 3}
|
229 | ], function(err, result) {
|
230 | assert.equal(err, null);
|
231 | assert.equal(3, result.result.n);
|
232 | assert.equal(3, result.ops.length);
|
233 | console.log("Inserted 3 documents into the collection");
|
234 | callback(result);
|
235 | });
|
236 | }
|
237 | ```
|
238 |
|
239 | The **insert** command returns an object with the following fields:
|
240 |
|
241 | * **result** Contains the result document from MongoDB
|
242 | * **ops** Contains the documents inserted with added **_id** fields
|
243 | * **connection** Contains the connection used to perform the insert
|
244 |
|
245 | Add the following code to call the **insertDocuments** function:
|
246 |
|
247 | ```js
|
248 | const MongoClient = require('mongodb').MongoClient;
|
249 | const assert = require('assert');
|
250 |
|
251 | // Connection URL
|
252 | const url = 'mongodb://localhost:27017';
|
253 |
|
254 | // Database Name
|
255 | const dbName = 'myproject';
|
256 |
|
257 | // Use connect method to connect to the server
|
258 | MongoClient.connect(url, function(err, client) {
|
259 | assert.equal(null, err);
|
260 | console.log("Connected successfully to server");
|
261 |
|
262 | const db = client.db(dbName);
|
263 |
|
264 | insertDocuments(db, function() {
|
265 | client.close();
|
266 | });
|
267 | });
|
268 | ```
|
269 |
|
270 | Run the updated **app.js** file:
|
271 |
|
272 | ```bash
|
273 | node app.js
|
274 | ```
|
275 |
|
276 | The operation returns the following output:
|
277 |
|
278 | ```bash
|
279 | Connected successfully to server
|
280 | Inserted 3 documents into the collection
|
281 | ```
|
282 |
|
283 | ### Find All Documents
|
284 |
|
285 | Add a query that returns all the documents.
|
286 |
|
287 | ```js
|
288 | const findDocuments = function(db, callback) {
|
289 | // Get the documents collection
|
290 | const collection = db.collection('documents');
|
291 | // Find some documents
|
292 | collection.find({}).toArray(function(err, docs) {
|
293 | assert.equal(err, null);
|
294 | console.log("Found the following records");
|
295 | console.log(docs)
|
296 | callback(docs);
|
297 | });
|
298 | }
|
299 | ```
|
300 |
|
301 | This query returns all the documents in the **documents** collection. Add the **findDocument** method to the **MongoClient.connect** callback:
|
302 |
|
303 | ```js
|
304 | const MongoClient = require('mongodb').MongoClient;
|
305 | const assert = require('assert');
|
306 |
|
307 | // Connection URL
|
308 | const url = 'mongodb://localhost:27017';
|
309 |
|
310 | // Database Name
|
311 | const dbName = 'myproject';
|
312 |
|
313 | // Use connect method to connect to the server
|
314 | MongoClient.connect(url, function(err, client) {
|
315 | assert.equal(null, err);
|
316 | console.log("Connected correctly to server");
|
317 |
|
318 | const db = client.db(dbName);
|
319 |
|
320 | insertDocuments(db, function() {
|
321 | findDocuments(db, function() {
|
322 | client.close();
|
323 | });
|
324 | });
|
325 | });
|
326 | ```
|
327 |
|
328 | ### Find Documents with a Query Filter
|
329 |
|
330 | Add a query filter to find only documents which meet the query criteria.
|
331 |
|
332 | ```js
|
333 | const findDocuments = function(db, callback) {
|
334 | // Get the documents collection
|
335 | const collection = db.collection('documents');
|
336 | // Find some documents
|
337 | collection.find({'a': 3}).toArray(function(err, docs) {
|
338 | assert.equal(err, null);
|
339 | console.log("Found the following records");
|
340 | console.log(docs);
|
341 | callback(docs);
|
342 | });
|
343 | }
|
344 | ```
|
345 |
|
346 | Only the documents which match ``'a' : 3`` should be returned.
|
347 |
|
348 | ### Update a document
|
349 |
|
350 | The following operation updates a document in the **documents** collection.
|
351 |
|
352 | ```js
|
353 | const updateDocument = function(db, callback) {
|
354 | // Get the documents collection
|
355 | const collection = db.collection('documents');
|
356 | // Update document where a is 2, set b equal to 1
|
357 | collection.updateOne({ a : 2 }
|
358 | , { $set: { b : 1 } }, function(err, result) {
|
359 | assert.equal(err, null);
|
360 | assert.equal(1, result.result.n);
|
361 | console.log("Updated the document with the field a equal to 2");
|
362 | callback(result);
|
363 | });
|
364 | }
|
365 | ```
|
366 |
|
367 | The method updates the first document where the field **a** is equal to **2** by adding a new field **b** to the document set to **1**. Next, update the callback function from **MongoClient.connect** to include the update method.
|
368 |
|
369 | ```js
|
370 | const MongoClient = require('mongodb').MongoClient;
|
371 | const assert = require('assert');
|
372 |
|
373 | // Connection URL
|
374 | const url = 'mongodb://localhost:27017';
|
375 |
|
376 | // Database Name
|
377 | const dbName = 'myproject';
|
378 |
|
379 | // Use connect method to connect to the server
|
380 | MongoClient.connect(url, function(err, client) {
|
381 | assert.equal(null, err);
|
382 | console.log("Connected successfully to server");
|
383 |
|
384 | const db = client.db(dbName);
|
385 |
|
386 | insertDocuments(db, function() {
|
387 | updateDocument(db, function() {
|
388 | client.close();
|
389 | });
|
390 | });
|
391 | });
|
392 | ```
|
393 |
|
394 | ### Remove a document
|
395 |
|
396 | Remove the document where the field **a** is equal to **3**.
|
397 |
|
398 | ```js
|
399 | const removeDocument = function(db, callback) {
|
400 | // Get the documents collection
|
401 | const collection = db.collection('documents');
|
402 | // Delete document where a is 3
|
403 | collection.deleteOne({ a : 3 }, function(err, result) {
|
404 | assert.equal(err, null);
|
405 | assert.equal(1, result.result.n);
|
406 | console.log("Removed the document with the field a equal to 3");
|
407 | callback(result);
|
408 | });
|
409 | }
|
410 | ```
|
411 |
|
412 | Add the new method to the **MongoClient.connect** callback function.
|
413 |
|
414 | ```js
|
415 | const MongoClient = require('mongodb').MongoClient;
|
416 | const assert = require('assert');
|
417 |
|
418 | // Connection URL
|
419 | const url = 'mongodb://localhost:27017';
|
420 |
|
421 | // Database Name
|
422 | const dbName = 'myproject';
|
423 |
|
424 | // Use connect method to connect to the server
|
425 | MongoClient.connect(url, function(err, client) {
|
426 | assert.equal(null, err);
|
427 | console.log("Connected successfully to server");
|
428 |
|
429 | const db = client.db(dbName);
|
430 |
|
431 | insertDocuments(db, function() {
|
432 | updateDocument(db, function() {
|
433 | removeDocument(db, function() {
|
434 | client.close();
|
435 | });
|
436 | });
|
437 | });
|
438 | });
|
439 | ```
|
440 |
|
441 | ### Index a Collection
|
442 |
|
443 | [Indexes](https://docs.mongodb.org/manual/indexes/) can improve your application's
|
444 | performance. The following function creates an index on the **a** field in the
|
445 | **documents** collection.
|
446 |
|
447 | ```js
|
448 | const indexCollection = function(db, callback) {
|
449 | db.collection('documents').createIndex(
|
450 | { "a": 1 },
|
451 | null,
|
452 | function(err, results) {
|
453 | console.log(results);
|
454 | callback();
|
455 | }
|
456 | );
|
457 | };
|
458 | ```
|
459 |
|
460 | Add the ``indexCollection`` method to your app:
|
461 |
|
462 | ```js
|
463 | const MongoClient = require('mongodb').MongoClient;
|
464 | const assert = require('assert');
|
465 |
|
466 | // Connection URL
|
467 | const url = 'mongodb://localhost:27017';
|
468 |
|
469 | const dbName = 'myproject';
|
470 |
|
471 | // Use connect method to connect to the server
|
472 | MongoClient.connect(url, function(err, client) {
|
473 | assert.equal(null, err);
|
474 | console.log("Connected successfully to server");
|
475 |
|
476 | const db = client.db(dbName);
|
477 |
|
478 | insertDocuments(db, function() {
|
479 | indexCollection(db, function() {
|
480 | client.close();
|
481 | });
|
482 | });
|
483 | });
|
484 | ```
|
485 |
|
486 | For more detailed information, see the [tutorials](docs/reference/content/tutorials/main.md).
|
487 |
|
488 | ## Next Steps
|
489 |
|
490 | * [MongoDB Documentation](http://mongodb.org)
|
491 | * [Read about Schemas](http://learnmongodbthehardway.com)
|
492 | * [Star us on GitHub](https://github.com/mongodb/node-mongodb-native)
|
493 |
|
494 | ## License
|
495 |
|
496 | [Apache 2.0](LICENSE.md)
|
497 |
|
498 | © 2009-2012 Christian Amor Kvalheim
|
499 | © 2012-present MongoDB [Contributors](CONTRIBUTORS.md)
|