1 | [](https://travis-ci.org/samccone/monocle)
|
2 |
|
3 | # Monocle -- a tool for watching things
|
4 |
|
5 | [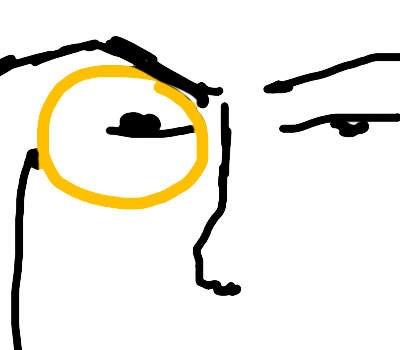](https://raw.github.com/samccone/monocle/master/logo.png)
|
6 |
|
7 | Have you ever wanted to watch a folder and all of its files/nested folders for changes. well now you can!
|
8 |
|
9 | ## Installation
|
10 |
|
11 | ```
|
12 | npm install monocle
|
13 | ```
|
14 |
|
15 | ## Usage
|
16 |
|
17 | ### Watch a directory:
|
18 |
|
19 | ```js
|
20 | var monocle = require('monocle')()
|
21 | monocle.watchDirectory({
|
22 | root: <root directory>,
|
23 | fileFilter: <optional>,
|
24 | directoryFilter: <optional>,
|
25 | listener: fn(fs.stat+ object), //triggered on file change / addition
|
26 | complete: <fn> //file watching all set up
|
27 | });
|
28 | ```
|
29 |
|
30 | The listener will recive an object with the following
|
31 |
|
32 | ```js
|
33 | name: <filename>,
|
34 | path: <filepath-relative>,
|
35 | fullPath: <filepath-absolute>,
|
36 | parentDir: <parentDir-relative>,
|
37 | fullParentDir: <parentDir-absolute>,
|
38 | stat: <see fs.stats>
|
39 | ```
|
40 |
|
41 | [fs.stats](http://nodejs.org/api/fs.html#fs_class_fs_stats)
|
42 |
|
43 | When a new file is added to the directoy it triggers a file change and thus will be passed to your specified listener.
|
44 |
|
45 | The two filters are passed through to `readdirp`. More documentation can be found [here](https://github.com/thlorenz/readdirp#filters)
|
46 |
|
47 | ### Watch a list of files:
|
48 |
|
49 | ```js
|
50 | Monocle.watchFiles({
|
51 | files: [], //path of file(s)
|
52 | listener: <fn(fs.stat+ object)>, //triggered on file / addition
|
53 | complete: <fn> //file watching all set up
|
54 | });
|
55 | ```
|
56 |
|
57 | ## Why not just use fs.watch ?
|
58 |
|
59 | - file watching is really bad cross platforms in node
|
60 | - you need to be smart when using fs.watch as compared to fs.watchFile
|
61 | - Monocle takes care of this logic for you!
|
62 | - windows systems use fs.watch
|
63 | - osx and linux uses fs.watchFile
|
64 |
|
65 |
|
66 | ## License
|
67 |
|
68 | BSD
|