1 | 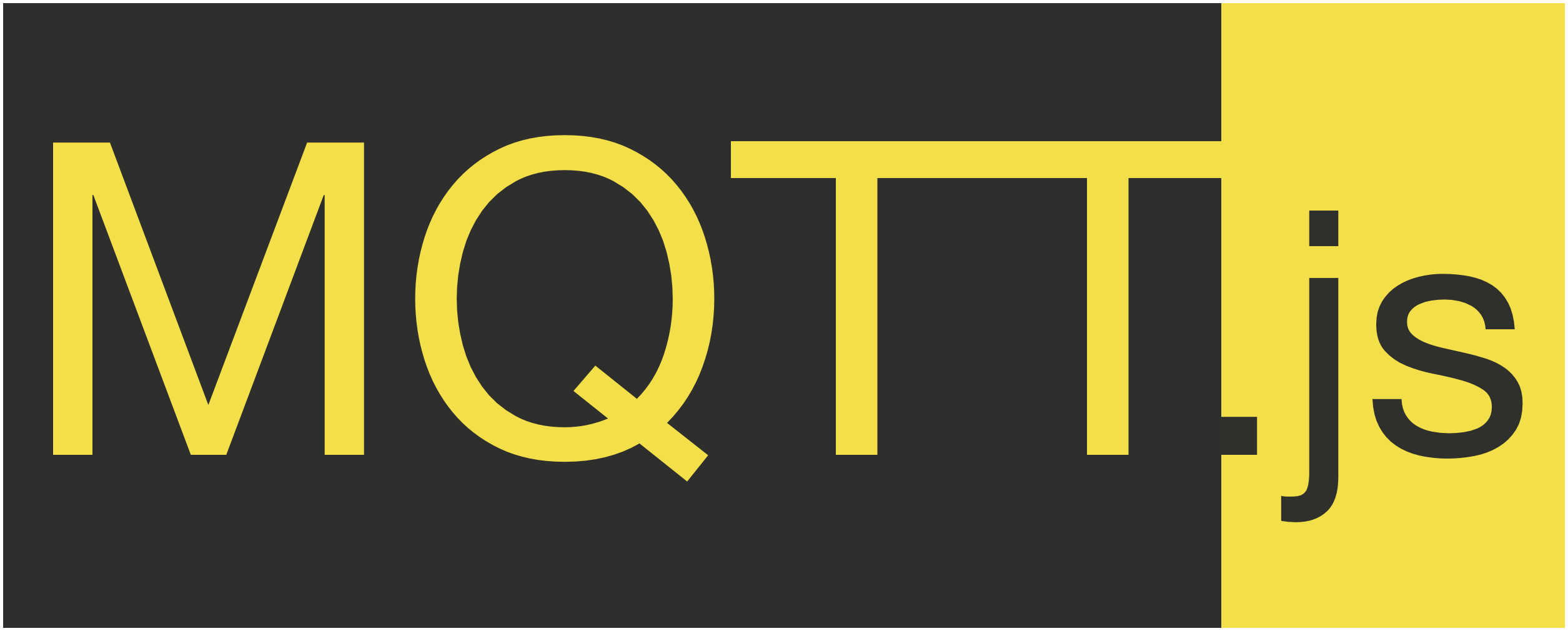
|
2 | =======
|
3 |
|
4 | [](https://travis-ci.org/mqttjs/MQTT.js)
|
5 |
|
6 | [](https://nodei.co/npm/mqtt/)
|
7 | [](https://nodei.co/npm/mqtt/)
|
8 |
|
9 | MQTT.js is a client library for the [MQTT](http://mqtt.org/) protocol, written
|
10 | in JavaScript for node.js and the browser.
|
11 |
|
12 | * [Upgrade notes](#notes)
|
13 | * [Installation](#install)
|
14 | * [Example](#example)
|
15 | * [Command Line Tools](#cli)
|
16 | * [API](#api)
|
17 | * [Browserify](#browserify)
|
18 | * [Contributing](#contributing)
|
19 | * [License](#license)
|
20 |
|
21 | MQTT.js is an OPEN Open Source Project, see the [Contributing](#contributing) section to find out what this means.
|
22 |
|
23 | <a name="notes"></a>
|
24 | ## Important notes for existing users
|
25 |
|
26 | v1.0.0 improves the overall architecture of the project, which is now
|
27 | split into three components: MQTT.js keeps the Client,
|
28 | [mqtt-connection](http://npm.im/mqtt-connection) includes the barebone
|
29 | Connection code for server-side usage, and [mqtt-packet](http://npm.im/mqtt-packet)
|
30 | includes the protocol parser and generator. The new Client improves
|
31 | performance by a 30% factor, embeds Websocket support
|
32 | ([MOWS](http://npm.im/mows) is now deprecated), and it has a better
|
33 | support for QoS 1 and 2. The previous API is still supported but
|
34 | deprecated, as such, it id not documented in this README.
|
35 |
|
36 | As a __breaking change__, the `encoding` option in the old client is
|
37 | removed, and now everything is UTF-8 with the exception of the
|
38 | `password` in the CONNECT message and `payload` in the PUBLISH message,
|
39 | which are `Buffer`.
|
40 |
|
41 | Another __breaking change__ is that MQTT.js now defaults to MQTT v3.1.1,
|
42 | so to support old brokers, please read the [client options doc](#client).
|
43 |
|
44 | <a name="install"></a>
|
45 | ## Installation
|
46 |
|
47 | ```sh
|
48 | npm install mqtt --save
|
49 | ```
|
50 |
|
51 | <a name="example"></a>
|
52 | ## Example
|
53 |
|
54 | For the sake of simplicity, let's put the subscriber and the publisher in the same file:
|
55 |
|
56 | ```js
|
57 | var mqtt = require('mqtt');
|
58 | var client = mqtt.connect('mqtt://test.mosquitto.org');
|
59 |
|
60 | client.on('connect', function () {
|
61 | client.subscribe('presence');
|
62 | client.publish('presence', 'Hello mqtt');
|
63 | });
|
64 |
|
65 | client.on('message', function (topic, message) {
|
66 | // message is Buffer
|
67 | console.log(message.toString());
|
68 | client.end();
|
69 | });
|
70 | ```
|
71 |
|
72 | output:
|
73 | ```
|
74 | Hello mqtt
|
75 | ```
|
76 |
|
77 | If you want to run your own MQTT broker, you can use
|
78 | [Mosquitto](http://mosquitto.org) or
|
79 | [Mosca](http://mcollina.github.io/mosca/), and launch it.
|
80 | You can also use a test instance: test.mosquitto.org and test.mosca.io
|
81 | are both public.
|
82 |
|
83 | If you do not want to install a separate broker, you can try using the
|
84 | [server/orig](https://github.com/adamvr/MQTT.js/blob/master/examples/server/orig.js)
|
85 | example.
|
86 | It implements enough of the semantics of the MQTT protocol to
|
87 | run the example.
|
88 |
|
89 | to use MQTT.js in the browser see the [browserify](#browserify) section
|
90 |
|
91 | <a name="cli"></a>
|
92 | ## Command Line Tools
|
93 |
|
94 | MQTT.js bundles a command to interact with a broker.
|
95 | In order to have it available on your path, you should install MQTT.js
|
96 | globally:
|
97 |
|
98 | ```sh
|
99 | npm install mqtt -g
|
100 | ```
|
101 |
|
102 | Then, on one terminal
|
103 |
|
104 | ```
|
105 | mqtt sub -t 'hello' -h 'test.mosquitto.org' -v
|
106 | ```
|
107 |
|
108 | On another
|
109 |
|
110 | ```
|
111 | mqtt pub -t 'hello' -h 'test.mosquitto.org' -m 'from MQTT.js'
|
112 | ```
|
113 |
|
114 | See `mqtt help <command>` for the command help.
|
115 |
|
116 | <a name="api"></a>
|
117 | ## API
|
118 |
|
119 | * <a href="#connect"><code>mqtt.<b>connect()</b></code></a>
|
120 | * <a href="#client"><code>mqtt.<b>Client()</b></code></a>
|
121 | * <a href="#publish"><code>mqtt.Client#<b>publish()</b></code></a>
|
122 | * <a href="#subscribe"><code>mqtt.Client#<b>subscribe()</b></code></a>
|
123 | * <a href="#unsubscribe"><code>mqtt.Client#<b>unsubscribe()</b></code></a>
|
124 | * <a href="#end"><code>mqtt.Client#<b>end()</b></code></a>
|
125 | * <a href="#handleMessage"><code>mqtt.Client#<b>handleMessage()</b></code></a>
|
126 | * <a href="#store"><code>mqtt.<b>Store()</b></code></a>
|
127 | * <a href="#put"><code>mqtt.Store#<b>put()</b></code></a>
|
128 | * <a href="#del"><code>mqtt.Store#<b>del()</b></code></a>
|
129 | * <a href="#createStream"><code>mqtt.Store#<b>createStream()</b></code></a>
|
130 | * <a href="#close"><code>mqtt.Store#<b>close()</b></code></a>
|
131 |
|
132 | -------------------------------------------------------
|
133 | <a name="connect"></a>
|
134 | ### mqtt.connect([url], options)
|
135 |
|
136 | Connects to the broker specified by the given url and options and
|
137 | returns a [Client](#client).
|
138 |
|
139 | The URL can be on the following protocols: 'mqtt', 'mqtts', 'tcp',
|
140 | 'tls', 'ws', 'wss'. The URL can also be an object as returned by
|
141 | [`URL.parse()`](http://nodejs.org/api/url.html#url_url_parse_urlstr_parsequerystring_slashesdenotehost),
|
142 | in that case the two objects are merged, i.e. you can pass a single
|
143 | object with both the URL and the connect options.
|
144 |
|
145 | You can also specify a `servers` options with content: `[{ host:
|
146 | 'localhost', port: 1883 }, ... ]`, in that case that array is iterated
|
147 | at every connect.
|
148 |
|
149 | For all MQTT-related options, see the [Client](#client)
|
150 | constructor.
|
151 |
|
152 | -------------------------------------------------------
|
153 | <a name="client"></a>
|
154 | ### mqtt.Client(streamBuilder, options)
|
155 |
|
156 | The `Client` class wraps a client connection to an
|
157 | MQTT broker over an arbitrary transport method (TCP, TLS,
|
158 | WebSocket, ecc).
|
159 |
|
160 | `Client` automatically handles the following:
|
161 |
|
162 | * Regular server pings
|
163 | * QoS flow
|
164 | * Automatic reconnections
|
165 | * Start publishing before being connected
|
166 |
|
167 | The arguments are:
|
168 |
|
169 | * `streamBuilder` is a function that returns a subclass of the `Stream` class that supports
|
170 | the `connect` event. Typically a `net.Socket`.
|
171 | * `options` is the client connection options (see: the [connect packet](https://github.com/mcollina/mqtt-packet#connect)). Defaults:
|
172 | * `keepalive`: `10` seconds, set to `0` to disable
|
173 | * `clientId`: `'mqttjs'_ + crypto.randomBytes(16).toString('hex')`
|
174 | * `protocolId`: `'MQTT'`
|
175 | * `protocolVersion`: `4`
|
176 | * `clean`: `true`, set to false to receive QoS 1 and 2 messages while
|
177 | offline
|
178 | * `reconnectPeriod`: `1000` milliseconds, interval between two
|
179 | reconnections
|
180 | * `connectTimeout`: `30 * 1000` milliseconds, time to wait before a
|
181 | CONNACK is received
|
182 | * `incomingStore`: a [Store](#store) for the incoming packets
|
183 | * `outgoingStore`: a [Store](#store) for the outgoing packets
|
184 | * `will`: a message that will sent by the broker automatically when
|
185 | the client disconnect badly. The format is:
|
186 | * `topic`: the topic to publish
|
187 | * `payload`: the message to publish
|
188 | * `qos`: the QoS
|
189 | * `retain`: the retain flag
|
190 |
|
191 | In case mqtts (mqtt over tls) is required, the `options` object is
|
192 | passed through to
|
193 | [`tls.connect()`](http://nodejs.org/api/tls.html#tls_tls_connect_options_callback).
|
194 | If you are using a **self-signed certificate**, pass the `rejectUnauthorized: false` option.
|
195 | Beware that you are exposing yourself to man in the middle attacks, so it is a configuration
|
196 | that is not recommended for production environments.
|
197 |
|
198 | If you are connecting to a broker that supports only MQTT 3.1 (not
|
199 | 3.1.1 compliant), you should pass these additional options:
|
200 |
|
201 | ```js
|
202 | {
|
203 | protocolId: 'MQIsdp',
|
204 | protocolVersion: 3
|
205 | }
|
206 | ```
|
207 |
|
208 | This is confirmed on RabbitMQ 3.2.4, and on Mosquitto < 1.3. Mosquitto
|
209 | version 1.3 and 1.4 works fine without those.
|
210 |
|
211 | #### Event `'connect'`
|
212 |
|
213 | `function() {}`
|
214 |
|
215 | Emitted on successful (re)connection (i.e. connack rc=0).
|
216 |
|
217 | #### Event `'reconnect'`
|
218 |
|
219 | `function() {}`
|
220 |
|
221 | Emitted when a reconnect starts.
|
222 |
|
223 | #### Event `'close'`
|
224 |
|
225 | `function() {}`
|
226 |
|
227 | Emitted after a disconnection.
|
228 |
|
229 | #### Event `'offline'`
|
230 |
|
231 | `function() {}`
|
232 |
|
233 | Emitted when the client goes offline.
|
234 |
|
235 | #### Event `'error'`
|
236 |
|
237 | `function(error) {}`
|
238 |
|
239 | Emitted when the client cannot connect (i.e. connack rc != 0) or when a
|
240 | parsing error occurs.
|
241 |
|
242 | ### Event `'message'`
|
243 |
|
244 | `function(topic, message, packet) {}`
|
245 |
|
246 | Emitted when the client receives a publish packet
|
247 | * `topic` topic of the received packet
|
248 | * `message` payload of the received packet
|
249 | * `packet` received packet, as defined in
|
250 | [mqtt-packet](https://github.com/mcollina/mqtt-packet#publish)
|
251 |
|
252 | -------------------------------------------------------
|
253 | <a name="publish"></a>
|
254 | ### mqtt.Client#publish(topic, message, [options], [callback])
|
255 |
|
256 | Publish a message to a topic
|
257 |
|
258 | * `topic` is the topic to publish to, `String`
|
259 | * `message` is the message to publish, `Buffer` or `String`
|
260 | * `options` is the options to publish with, including:
|
261 | * `qos` QoS level, `Number`, default `0`
|
262 | * `retain` retain flag, `Boolean`, default `false`
|
263 | * `callback` callback fired when the QoS handling completes,
|
264 | or at the next tick if QoS 0.
|
265 |
|
266 | -------------------------------------------------------
|
267 | <a name="subscribe"></a>
|
268 | ### mqtt.Client#subscribe(topic/topic array/topic object, [options], [callback])
|
269 |
|
270 | Subscribe to a topic or topics
|
271 |
|
272 | * `topic` is a `String` topic to subscribe to or an `Array` of
|
273 | topics to subscribe to. It can also be an object, it has as object
|
274 | keys the topic name and as value the QoS, like `{'test1': 0, 'test2': 1}`.
|
275 | * `options` is the options to subscribe with, including:
|
276 | * `qos` qos subscription level, default 0
|
277 | * `callback` - `function(err, granted)`
|
278 | callback fired on suback where:
|
279 | * `err` a subscription error
|
280 | * `granted` is an array of `{topic, qos}` where:
|
281 | * `topic` is a subscribed to topic
|
282 | * `qos` is the granted qos level on it
|
283 |
|
284 | -------------------------------------------------------
|
285 | <a name="unsubscribe"></a>
|
286 | ### mqtt.Client#unsubscribe(topic/topic array, [options], [callback])
|
287 |
|
288 | Unsubscribe from a topic or topics
|
289 |
|
290 | * `topic` is a `String` topic or an array of topics to unsubscribe from
|
291 | * `callback` fired on unsuback
|
292 |
|
293 | -------------------------------------------------------
|
294 | <a name="end"></a>
|
295 | ### mqtt.Client#end([force], [cb])
|
296 |
|
297 | Close the client, accepts the following options:
|
298 |
|
299 | * `force`: passing it to true will close the client right away, without
|
300 | waiting for the in-flight messages to be acked. This parameter is
|
301 | optional.
|
302 | * `cb`: will be called when the client is closed. This parameter is
|
303 | optional.
|
304 |
|
305 | -------------------------------------------------------
|
306 | <a name="handleMessage"></a>
|
307 | ### mqtt.Client#handleMessage(packet, callback)
|
308 |
|
309 | Handle messages with backpressure support, one at a time.
|
310 | Override at will, but __always call `callback`__, or the client
|
311 | will hang.
|
312 |
|
313 | -------------------------------------------------------
|
314 | <a name="store"></a>
|
315 | ### mqtt.Store()
|
316 |
|
317 | In-memory implementation of the message store.
|
318 |
|
319 | Another implementaion is
|
320 | [mqtt-level-store](http://npm.im/mqtt-level-store) which uses
|
321 | [Level-browserify](http://npm.im/level-browserify) to store the inflight
|
322 | data, making it usable both in Node and the Browser.
|
323 |
|
324 | -------------------------------------------------------
|
325 | <a name="put"></a>
|
326 | ### mqtt.Store#put(packet, callback)
|
327 |
|
328 | Adds a packet to the store, a packet is
|
329 | anything that has a `messageId` property.
|
330 | The callback is called when the packet has been stored.
|
331 |
|
332 | -------------------------------------------------------
|
333 | <a name="createStream"></a>
|
334 | ### mqtt.Store#createStream()
|
335 |
|
336 | Creates a stream with all the packets in the store.
|
337 |
|
338 | -------------------------------------------------------
|
339 | <a name="del"></a>
|
340 | ### mqtt.Store#del(packet, cb)
|
341 |
|
342 | Removes a packet from the store, a packet is
|
343 | anything that has a `messageId` property.
|
344 | The callback is called when the packet has been removed.
|
345 |
|
346 | -------------------------------------------------------
|
347 | <a name="close"></a>
|
348 | ### mqtt.Store#close(cb)
|
349 |
|
350 | Closes the Store.
|
351 |
|
352 | <a name="browserify"></a>
|
353 | ## Browserify
|
354 |
|
355 | In order to use MQTT.js as a browserify module you can either require it in your browserify bundles or build it as a stand alone module. The exported module is AMD/CommonJs compatible and it will add an object in the global space.
|
356 |
|
357 | ```javascript
|
358 | npm install -g browserify // install browserify
|
359 | cd node_modules/mqtt
|
360 | npm install . // install dev dependencies
|
361 | browserify mqtt.js -s mqtt > browserMqtt.js // require mqtt in your client-side app
|
362 | ```
|
363 |
|
364 | you can then use mqtt.js in the browser with the same api than node's one.
|
365 |
|
366 | ```html
|
367 | <html>
|
368 | <head>
|
369 | <title>test Ws mqtt.js</title>
|
370 | </head>
|
371 | <body>
|
372 | <script src="./browserMqtt.js"></script>
|
373 | <script>
|
374 | var client = mqtt.connect(); // you add a ws:// url here
|
375 | client.subscribe("mqtt/demo");
|
376 |
|
377 | client.on("message", function(topic, payload) {
|
378 | alert([topic, payload].join(": "));
|
379 | client.end();
|
380 | });
|
381 |
|
382 | client.publish("mqtt/demo", "hello world!");
|
383 | </script>
|
384 | </body>
|
385 | </html>
|
386 | ```
|
387 |
|
388 | Your broker should accept websocket connection (see [MQTT over Websockets](https://github.com/mcollina/mosca/wiki/MQTT-over-Websockets) to setup [Mosca](http://mcollina.github.io/mosca/)).
|
389 |
|
390 | <a name="contributing"></a>
|
391 | ## Contributing
|
392 |
|
393 | MQTT.js is an **OPEN Open Source Project**. This means that:
|
394 |
|
395 | > Individuals making significant and valuable contributions are given commit-access to the project to contribute as they see fit. This project is more like an open wiki than a standard guarded open source project.
|
396 |
|
397 | See the [CONTRIBUTING.md](https://github.com/mqttjs/MQTT.js/blob/master/CONTRIBUTING.md) file for more details.
|
398 |
|
399 | ### Contributors
|
400 |
|
401 | MQTT.js is only possible due to the excellent work of the following contributors:
|
402 |
|
403 | <table><tbody>
|
404 | <tr><th align="left">Adam Rudd</th><td><a href="https://github.com/adamvr">GitHub/adamvr</a></td><td><a href="http://twitter.com/adam_vr">Twitter/@adam_vr</a></td></tr>
|
405 | <tr><th align="left">Matteo Collina</th><td><a href="https://github.com/mcollina">GitHub/mcollina</a></td><td><a href="http://twitter.com/matteocollina">Twitter/@matteocollina</a></td></tr>
|
406 | </tbody></table>
|
407 |
|
408 | <a name="license"></a>
|
409 | ## License
|
410 |
|
411 | MIT
|