1 | 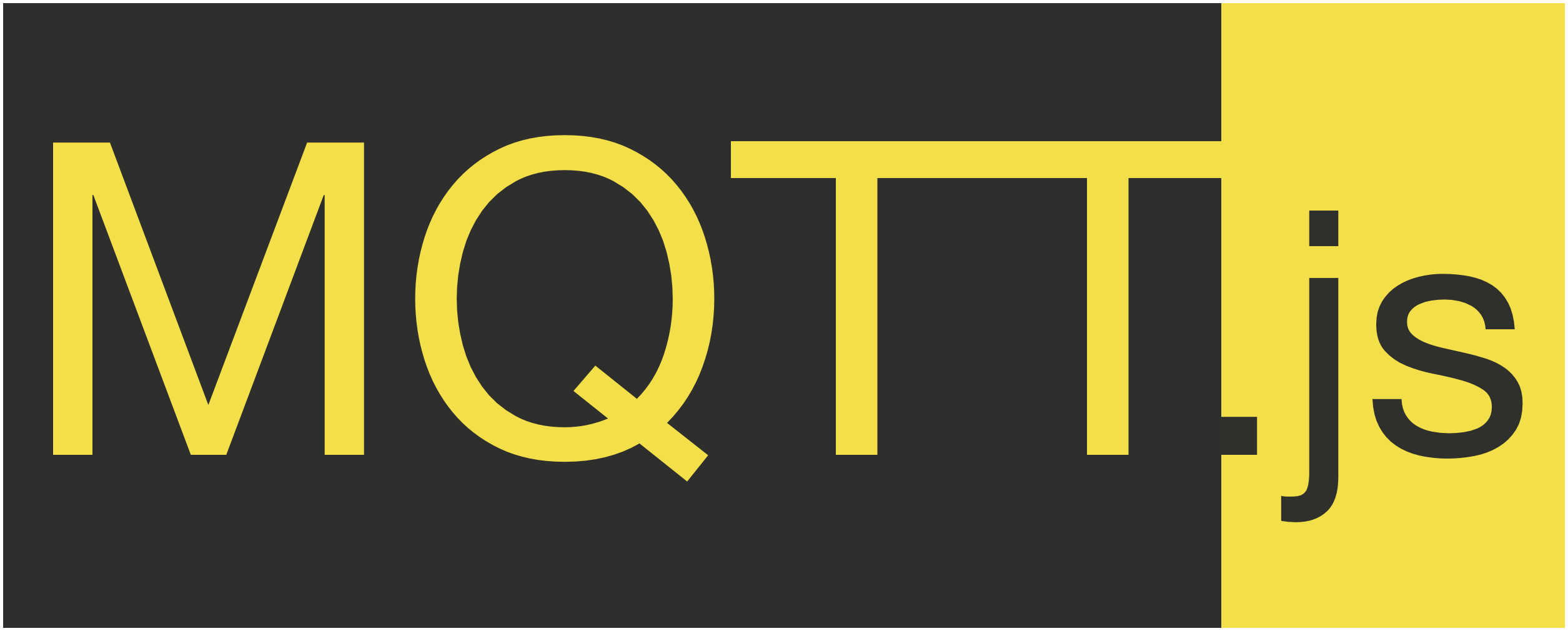
|
2 | =======
|
3 |
|
4 | [](https://travis-ci.org/mqttjs/MQTT.js)
|
5 |
|
6 | [](https://saucelabs.com/u/mqttjs)
|
7 |
|
8 | [](https://github.com/borisyankov/DefinitelyTyped/tree/master/mqtt)
|
9 |
|
10 | [](https://nodei.co/npm/mqtt/)
|
11 | [](https://nodei.co/npm/mqtt/)
|
12 |
|
13 | MQTT.js is a client library for the [MQTT](http://mqtt.org/) protocol, written
|
14 | in JavaScript for node.js and the browser.
|
15 |
|
16 | * [Upgrade notes](#notes)
|
17 | * [Installation](#install)
|
18 | * [Example](#example)
|
19 | * [Command Line Tools](#cli)
|
20 | * [API](#api)
|
21 | * [Browser](#browser)
|
22 | * [About QoS](#qos)
|
23 | * [Contributing](#contributing)
|
24 | * [License](#license)
|
25 |
|
26 | MQTT.js is an OPEN Open Source Project, see the [Contributing](#contributing) section to find out what this means.
|
27 |
|
28 | [](https://github.com/feross/standard)
|
30 |
|
31 |
|
32 | <a name="notes"></a>
|
33 | ## Important notes for existing users
|
34 |
|
35 | v2.0.0 removes support for node v0.8, v0.10 and v0.12, and it is 3x faster in sending
|
36 | packets. It also removes all the deprecated functionality in v1.0.0,
|
37 | mainly `mqtt.createConnection` and `mqtt.Server`. From v2.0.0,
|
38 | subscriptions are restored upon reconnection if `clean: true`.
|
39 | v1.x.x is now in *LTS*, and it will keep being supported as long as
|
40 | there are v0.8, v0.10 and v0.12 users.
|
41 |
|
42 | v1.0.0 improves the overall architecture of the project, which is now
|
43 | split into three components: MQTT.js keeps the Client,
|
44 | [mqtt-connection](http://npm.im/mqtt-connection) includes the barebone
|
45 | Connection code for server-side usage, and [mqtt-packet](http://npm.im/mqtt-packet)
|
46 | includes the protocol parser and generator. The new Client improves
|
47 | performance by a 30% factor, embeds Websocket support
|
48 | ([MOWS](http://npm.im/mows) is now deprecated), and it has a better
|
49 | support for QoS 1 and 2. The previous API is still supported but
|
50 | deprecated, as such, it id not documented in this README.
|
51 |
|
52 | As a __breaking change__, the `encoding` option in the old client is
|
53 | removed, and now everything is UTF-8 with the exception of the
|
54 | `password` in the CONNECT message and `payload` in the PUBLISH message,
|
55 | which are `Buffer`.
|
56 |
|
57 | Another __breaking change__ is that MQTT.js now defaults to MQTT v3.1.1,
|
58 | so to support old brokers, please read the [client options doc](#client).
|
59 |
|
60 | <a name="install"></a>
|
61 | ## Installation
|
62 |
|
63 | ```sh
|
64 | npm install mqtt --save
|
65 | ```
|
66 |
|
67 | <a name="example"></a>
|
68 | ## Example
|
69 |
|
70 | For the sake of simplicity, let's put the subscriber and the publisher in the same file:
|
71 |
|
72 | ```js
|
73 | var mqtt = require('mqtt')
|
74 | var client = mqtt.connect('mqtt://test.mosquitto.org')
|
75 |
|
76 | client.on('connect', function () {
|
77 | client.subscribe('presence')
|
78 | client.publish('presence', 'Hello mqtt')
|
79 | })
|
80 |
|
81 | client.on('message', function (topic, message) {
|
82 | // message is Buffer
|
83 | console.log(message.toString())
|
84 | client.end()
|
85 | })
|
86 | ```
|
87 |
|
88 | output:
|
89 | ```
|
90 | Hello mqtt
|
91 | ```
|
92 |
|
93 | If you want to run your own MQTT broker, you can use
|
94 | [Mosquitto](http://mosquitto.org) or
|
95 | [Mosca](http://mcollina.github.io/mosca/), and launch it.
|
96 | You can also use a test instance: test.mosquitto.org and test.mosca.io
|
97 | are both public.
|
98 |
|
99 | If you do not want to install a separate broker, you can try using the
|
100 | [server/orig](https://github.com/adamvr/MQTT.js/blob/master/examples/server/orig.js)
|
101 | example.
|
102 | It implements enough of the semantics of the MQTT protocol to
|
103 | run the example.
|
104 |
|
105 | to use MQTT.js in the browser see the [browserify](#browserify) section
|
106 |
|
107 | <a name="cli"></a>
|
108 | ## Command Line Tools
|
109 |
|
110 | MQTT.js bundles a command to interact with a broker.
|
111 | In order to have it available on your path, you should install MQTT.js
|
112 | globally:
|
113 |
|
114 | ```sh
|
115 | npm install mqtt -g
|
116 | ```
|
117 |
|
118 | Then, on one terminal
|
119 |
|
120 | ```
|
121 | mqtt sub -t 'hello' -h 'test.mosquitto.org' -v
|
122 | ```
|
123 |
|
124 | On another
|
125 |
|
126 | ```
|
127 | mqtt pub -t 'hello' -h 'test.mosquitto.org' -m 'from MQTT.js'
|
128 | ```
|
129 |
|
130 | See `mqtt help <command>` for the command help.
|
131 |
|
132 | <a name="api"></a>
|
133 | ## API
|
134 |
|
135 | * <a href="#connect"><code>mqtt.<b>connect()</b></code></a>
|
136 | * <a href="#client"><code>mqtt.<b>Client()</b></code></a>
|
137 | * <a href="#publish"><code>mqtt.Client#<b>publish()</b></code></a>
|
138 | * <a href="#subscribe"><code>mqtt.Client#<b>subscribe()</b></code></a>
|
139 | * <a href="#unsubscribe"><code>mqtt.Client#<b>unsubscribe()</b></code></a>
|
140 | * <a href="#end"><code>mqtt.Client#<b>end()</b></code></a>
|
141 | * <a href="#handleMessage"><code>mqtt.Client#<b>handleMessage()</b></code></a>
|
142 | * <a href="#connected"><code>mqtt.Client#<b>connected</b></code></a>
|
143 | * <a href="#reconnecting"><code>mqtt.Client#<b>reconnecting</b></code></a>
|
144 | * <a href="#store"><code>mqtt.<b>Store()</b></code></a>
|
145 | * <a href="#put"><code>mqtt.Store#<b>put()</b></code></a>
|
146 | * <a href="#del"><code>mqtt.Store#<b>del()</b></code></a>
|
147 | * <a href="#createStream"><code>mqtt.Store#<b>createStream()</b></code></a>
|
148 | * <a href="#close"><code>mqtt.Store#<b>close()</b></code></a>
|
149 |
|
150 | -------------------------------------------------------
|
151 | <a name="connect"></a>
|
152 | ### mqtt.connect([url], options)
|
153 |
|
154 | Connects to the broker specified by the given url and options and
|
155 | returns a [Client](#client).
|
156 |
|
157 | The URL can be on the following protocols: 'mqtt', 'mqtts', 'tcp',
|
158 | 'tls', 'ws', 'wss'. The URL can also be an object as returned by
|
159 | [`URL.parse()`](http://nodejs.org/api/url.html#url_url_parse_urlstr_parsequerystring_slashesdenotehost),
|
160 | in that case the two objects are merged, i.e. you can pass a single
|
161 | object with both the URL and the connect options.
|
162 |
|
163 | You can also specify a `servers` options with content: `[{ host:
|
164 | 'localhost', port: 1883 }, ... ]`, in that case that array is iterated
|
165 | at every connect.
|
166 |
|
167 | For all MQTT-related options, see the [Client](#client)
|
168 | constructor.
|
169 |
|
170 | -------------------------------------------------------
|
171 | <a name="client"></a>
|
172 | ### mqtt.Client(streamBuilder, options)
|
173 |
|
174 | The `Client` class wraps a client connection to an
|
175 | MQTT broker over an arbitrary transport method (TCP, TLS,
|
176 | WebSocket, ecc).
|
177 |
|
178 | `Client` automatically handles the following:
|
179 |
|
180 | * Regular server pings
|
181 | * QoS flow
|
182 | * Automatic reconnections
|
183 | * Start publishing before being connected
|
184 |
|
185 | The arguments are:
|
186 |
|
187 | * `streamBuilder` is a function that returns a subclass of the `Stream` class that supports
|
188 | the `connect` event. Typically a `net.Socket`.
|
189 | * `options` is the client connection options (see: the [connect packet](https://github.com/mcollina/mqtt-packet#connect)). Defaults:
|
190 | * `keepalive`: `10` seconds, set to `0` to disable
|
191 | * `reschedulePings`: reschedule ping messages after sending packets (default `true`)
|
192 | * `clientId`: `'mqttjs_' + Math.random().toString(16).substr(2, 8)`
|
193 | * `protocolId`: `'MQTT'`
|
194 | * `protocolVersion`: `4`
|
195 | * `clean`: `true`, set to false to receive QoS 1 and 2 messages while
|
196 | offline
|
197 | * `reconnectPeriod`: `1000` milliseconds, interval between two
|
198 | reconnections
|
199 | * `connectTimeout`: `30 * 1000` milliseconds, time to wait before a
|
200 | CONNACK is received
|
201 | * `username`: the username required by your broker, if any
|
202 | * `password`: the password required by your broker, if any
|
203 | * `incomingStore`: a [Store](#store) for the incoming packets
|
204 | * `outgoingStore`: a [Store](#store) for the outgoing packets
|
205 | * `queueQoSZero`: if connection is broken, queue outgoing QoS zero messages (default `true`)
|
206 | * `will`: a message that will sent by the broker automatically when
|
207 | the client disconnect badly. The format is:
|
208 | * `topic`: the topic to publish
|
209 | * `payload`: the message to publish
|
210 | * `qos`: the QoS
|
211 | * `retain`: the retain flag
|
212 |
|
213 | In case mqtts (mqtt over tls) is required, the `options` object is
|
214 | passed through to
|
215 | [`tls.connect()`](http://nodejs.org/api/tls.html#tls_tls_connect_options_callback).
|
216 | If you are using a **self-signed certificate**, pass the `rejectUnauthorized: false` option.
|
217 | Beware that you are exposing yourself to man in the middle attacks, so it is a configuration
|
218 | that is not recommended for production environments.
|
219 |
|
220 | If you are connecting to a broker that supports only MQTT 3.1 (not
|
221 | 3.1.1 compliant), you should pass these additional options:
|
222 |
|
223 | ```js
|
224 | {
|
225 | protocolId: 'MQIsdp',
|
226 | protocolVersion: 3
|
227 | }
|
228 | ```
|
229 |
|
230 | This is confirmed on RabbitMQ 3.2.4, and on Mosquitto < 1.3. Mosquitto
|
231 | version 1.3 and 1.4 works fine without those.
|
232 |
|
233 | #### Event `'connect'`
|
234 |
|
235 | `function (connack) {}`
|
236 |
|
237 | Emitted on successful (re)connection (i.e. connack rc=0).
|
238 | * `connack` received connack packet. When `clean` connection option is `false` and server has a previous session
|
239 | for `clientId` connection option, then `connack.sessionPresent` flag is `true`. When that is the case,
|
240 | you may rely on stored session and prefer not to send subscribe commands for the client.
|
241 |
|
242 | #### Event `'reconnect'`
|
243 |
|
244 | `function () {}`
|
245 |
|
246 | Emitted when a reconnect starts.
|
247 |
|
248 | #### Event `'close'`
|
249 |
|
250 | `function () {}`
|
251 |
|
252 | Emitted after a disconnection.
|
253 |
|
254 | #### Event `'offline'`
|
255 |
|
256 | `function () {}`
|
257 |
|
258 | Emitted when the client goes offline.
|
259 |
|
260 | #### Event `'error'`
|
261 |
|
262 | `function (error) {}`
|
263 |
|
264 | Emitted when the client cannot connect (i.e. connack rc != 0) or when a
|
265 | parsing error occurs.
|
266 |
|
267 | ### Event `'message'`
|
268 |
|
269 | `function (topic, message, packet) {}`
|
270 |
|
271 | Emitted when the client receives a publish packet
|
272 | * `topic` topic of the received packet
|
273 | * `message` payload of the received packet
|
274 | * `packet` received packet, as defined in
|
275 | [mqtt-packet](https://github.com/mcollina/mqtt-packet#publish)
|
276 |
|
277 | ### Event `'packetsend'`
|
278 |
|
279 | `function (packet) {}`
|
280 |
|
281 | Emitted when the client sends any packet. This includes .published() packets
|
282 | as well as packets used by MQTT for managing subscriptions and connections
|
283 | * `packet` received packet, as defined in
|
284 | [mqtt-packet](https://github.com/mcollina/mqtt-packet)
|
285 |
|
286 | ### Event `'packetreceive'`
|
287 |
|
288 | `function (packet) {}`
|
289 |
|
290 | Emitted when the client receives any packet. This includes packets from
|
291 | subscribed topics as well as packets used by MQTT for managing subscriptions
|
292 | and connections
|
293 | * `packet` received packet, as defined in
|
294 | [mqtt-packet](https://github.com/mcollina/mqtt-packet)
|
295 |
|
296 | -------------------------------------------------------
|
297 | <a name="publish"></a>
|
298 | ### mqtt.Client#publish(topic, message, [options], [callback])
|
299 |
|
300 | Publish a message to a topic
|
301 |
|
302 | * `topic` is the topic to publish to, `String`
|
303 | * `message` is the message to publish, `Buffer` or `String`
|
304 | * `options` is the options to publish with, including:
|
305 | * `qos` QoS level, `Number`, default `0`
|
306 | * `retain` retain flag, `Boolean`, default `false`
|
307 | * `callback` - `function (err)`, fired when the QoS handling completes,
|
308 | or at the next tick if QoS 0. An error occurs if client is disconnecting.
|
309 |
|
310 | -------------------------------------------------------
|
311 | <a name="subscribe"></a>
|
312 | ### mqtt.Client#subscribe(topic/topic array/topic object, [options], [callback])
|
313 |
|
314 | Subscribe to a topic or topics
|
315 |
|
316 | * `topic` is a `String` topic to subscribe to or an `Array` of
|
317 | topics to subscribe to. It can also be an object, it has as object
|
318 | keys the topic name and as value the QoS, like `{'test1': 0, 'test2': 1}`.
|
319 | * `options` is the options to subscribe with, including:
|
320 | * `qos` qos subscription level, default 0
|
321 | * `callback` - `function (err, granted)`
|
322 | callback fired on suback where:
|
323 | * `err` a subscription error or an error that occurs when client is disconnecting
|
324 | * `granted` is an array of `{topic, qos}` where:
|
325 | * `topic` is a subscribed to topic
|
326 | * `qos` is the granted qos level on it
|
327 |
|
328 | -------------------------------------------------------
|
329 | <a name="unsubscribe"></a>
|
330 | ### mqtt.Client#unsubscribe(topic/topic array, [callback])
|
331 |
|
332 | Unsubscribe from a topic or topics
|
333 |
|
334 | * `topic` is a `String` topic or an array of topics to unsubscribe from
|
335 | * `callback` - `function (err)`, fired on unsuback. An error occurs if client is disconnecting.
|
336 |
|
337 | -------------------------------------------------------
|
338 | <a name="end"></a>
|
339 | ### mqtt.Client#end([force], [cb])
|
340 |
|
341 | Close the client, accepts the following options:
|
342 |
|
343 | * `force`: passing it to true will close the client right away, without
|
344 | waiting for the in-flight messages to be acked. This parameter is
|
345 | optional.
|
346 | * `cb`: will be called when the client is closed. This parameter is
|
347 | optional.
|
348 |
|
349 | -------------------------------------------------------
|
350 | <a name="handleMessage"></a>
|
351 | ### mqtt.Client#handleMessage(packet, callback)
|
352 |
|
353 | Handle messages with backpressure support, one at a time.
|
354 | Override at will, but __always call `callback`__, or the client
|
355 | will hang.
|
356 |
|
357 | -------------------------------------------------------
|
358 | <a name="connected"></a>
|
359 | ### mqtt.Client#connected
|
360 |
|
361 | Boolean : set to `true` if the client is connected. `false` otherwise.
|
362 |
|
363 | -------------------------------------------------------
|
364 | <a name="reconnecting"></a>
|
365 | ### mqtt.Client#reconnecting
|
366 |
|
367 | Boolean : set to `true` if the client is trying to reconnect to the server. `false` otherwise.
|
368 |
|
369 | -------------------------------------------------------
|
370 | <a name="store"></a>
|
371 | ### mqtt.Store()
|
372 |
|
373 | In-memory implementation of the message store.
|
374 |
|
375 | Another implementaion is
|
376 | [mqtt-level-store](http://npm.im/mqtt-level-store) which uses
|
377 | [Level-browserify](http://npm.im/level-browserify) to store the inflight
|
378 | data, making it usable both in Node and the Browser.
|
379 |
|
380 | -------------------------------------------------------
|
381 | <a name="put"></a>
|
382 | ### mqtt.Store#put(packet, callback)
|
383 |
|
384 | Adds a packet to the store, a packet is
|
385 | anything that has a `messageId` property.
|
386 | The callback is called when the packet has been stored.
|
387 |
|
388 | -------------------------------------------------------
|
389 | <a name="createStream"></a>
|
390 | ### mqtt.Store#createStream()
|
391 |
|
392 | Creates a stream with all the packets in the store.
|
393 |
|
394 | -------------------------------------------------------
|
395 | <a name="del"></a>
|
396 | ### mqtt.Store#del(packet, cb)
|
397 |
|
398 | Removes a packet from the store, a packet is
|
399 | anything that has a `messageId` property.
|
400 | The callback is called when the packet has been removed.
|
401 |
|
402 | -------------------------------------------------------
|
403 | <a name="close"></a>
|
404 | ### mqtt.Store#close(cb)
|
405 |
|
406 | Closes the Store.
|
407 |
|
408 | <a name="browser"></a>
|
409 | ## Browser
|
410 |
|
411 | <a name="cdn"></a>
|
412 | ### Via CDN
|
413 |
|
414 | The MQTT.js bundle is available through http://unpkg.com, specifically
|
415 | at https://unpkg.com/mqtt/dist/mqtt.min.js.
|
416 | See http://unpkg.com for the full documentation on version ranges.
|
417 |
|
418 | <a name="browserify"></a>
|
419 | ### Browserify
|
420 |
|
421 | In order to use MQTT.js as a browserify module you can either require it in your browserify bundles or build it as a stand alone module. The exported module is AMD/CommonJs compatible and it will add an object in the global space.
|
422 |
|
423 | ```javascript
|
424 | npm install -g browserify // install browserify
|
425 | cd node_modules/mqtt
|
426 | npm install . // install dev dependencies
|
427 | browserify mqtt.js -s mqtt > browserMqtt.js // require mqtt in your client-side app
|
428 | ```
|
429 |
|
430 | <a name="webpack"></a>
|
431 | ### Webpack
|
432 |
|
433 | Just like browserify, export MQTT.js as library. The exported module would be `var mqtt = xxx` and it will add an object in the global space. You could also export module in other [formats (AMD/CommonJS/others)](http://webpack.github.io/docs/configuration.html#output-librarytarget) by setting **output.libraryTarget** in webpack configuration.
|
434 |
|
435 | ```javascript
|
436 | npm install -g webpack // install webpack
|
437 |
|
438 | cd node_modules/mqtt
|
439 | npm install . // install dev dependencies
|
440 | webpack mqtt.js ./browserMqtt.js --output-library mqtt
|
441 | ```
|
442 |
|
443 | you can then use mqtt.js in the browser with the same api than node's one.
|
444 |
|
445 | ```html
|
446 | <html>
|
447 | <head>
|
448 | <title>test Ws mqtt.js</title>
|
449 | </head>
|
450 | <body>
|
451 | <script src="./browserMqtt.js"></script>
|
452 | <script>
|
453 | var client = mqtt.connect() // you add a ws:// url here
|
454 | client.subscribe("mqtt/demo")
|
455 |
|
456 | client.on("message", function (topic, payload) {
|
457 | alert([topic, payload].join(": "))
|
458 | client.end()
|
459 | })
|
460 |
|
461 | client.publish("mqtt/demo", "hello world!")
|
462 | </script>
|
463 | </body>
|
464 | </html>
|
465 | ```
|
466 |
|
467 | Your broker should accept websocket connection (see [MQTT over Websockets](https://github.com/mcollina/mosca/wiki/MQTT-over-Websockets) to setup [Mosca](http://mcollina.github.io/mosca/)).
|
468 |
|
469 | <a name="qos"></a>
|
470 | ## About QoS
|
471 |
|
472 | Here is how QoS works:
|
473 |
|
474 | * QoS 0 : received **at most once** : The packet is sent, and that's it. There is no validation about whether it has been received.
|
475 | * QoS 1 : received **at least once** : The packet is sent and stored as long as the client has not received a confirmation from the server. MQTT ensures that it *will* be received, but there can be duplicates.
|
476 | * QoS 2 : received **exactly once** : Same as QoS 1 but there is no duplicates.
|
477 |
|
478 | About data consumption, obviously, QoS 2 > QoS 1 > QoS 0, if that's a concern to you.
|
479 |
|
480 | <a name="contributing"></a>
|
481 | ## Contributing
|
482 |
|
483 | MQTT.js is an **OPEN Open Source Project**. This means that:
|
484 |
|
485 | > Individuals making significant and valuable contributions are given commit-access to the project to contribute as they see fit. This project is more like an open wiki than a standard guarded open source project.
|
486 |
|
487 | See the [CONTRIBUTING.md](https://github.com/mqttjs/MQTT.js/blob/master/CONTRIBUTING.md) file for more details.
|
488 |
|
489 | ### Contributors
|
490 |
|
491 | MQTT.js is only possible due to the excellent work of the following contributors:
|
492 |
|
493 | <table><tbody>
|
494 | <tr><th align="left">Adam Rudd</th><td><a href="https://github.com/adamvr">GitHub/adamvr</a></td><td><a href="http://twitter.com/adam_vr">Twitter/@adam_vr</a></td></tr>
|
495 | <tr><th align="left">Matteo Collina</th><td><a href="https://github.com/mcollina">GitHub/mcollina</a></td><td><a href="http://twitter.com/matteocollina">Twitter/@matteocollina</a></td></tr>
|
496 | <tr><th align="left">Maxime Agor</th><td><a href="https://github.com/4rzael">GitHub/4rzael</a></td><td><a href="http://twitter.com/4rzael">Twitter/@4rzael</a></td></tr>
|
497 | </tbody></table>
|
498 |
|
499 | <a name="license"></a>
|
500 | ## License
|
501 |
|
502 | MIT
|