1 | # 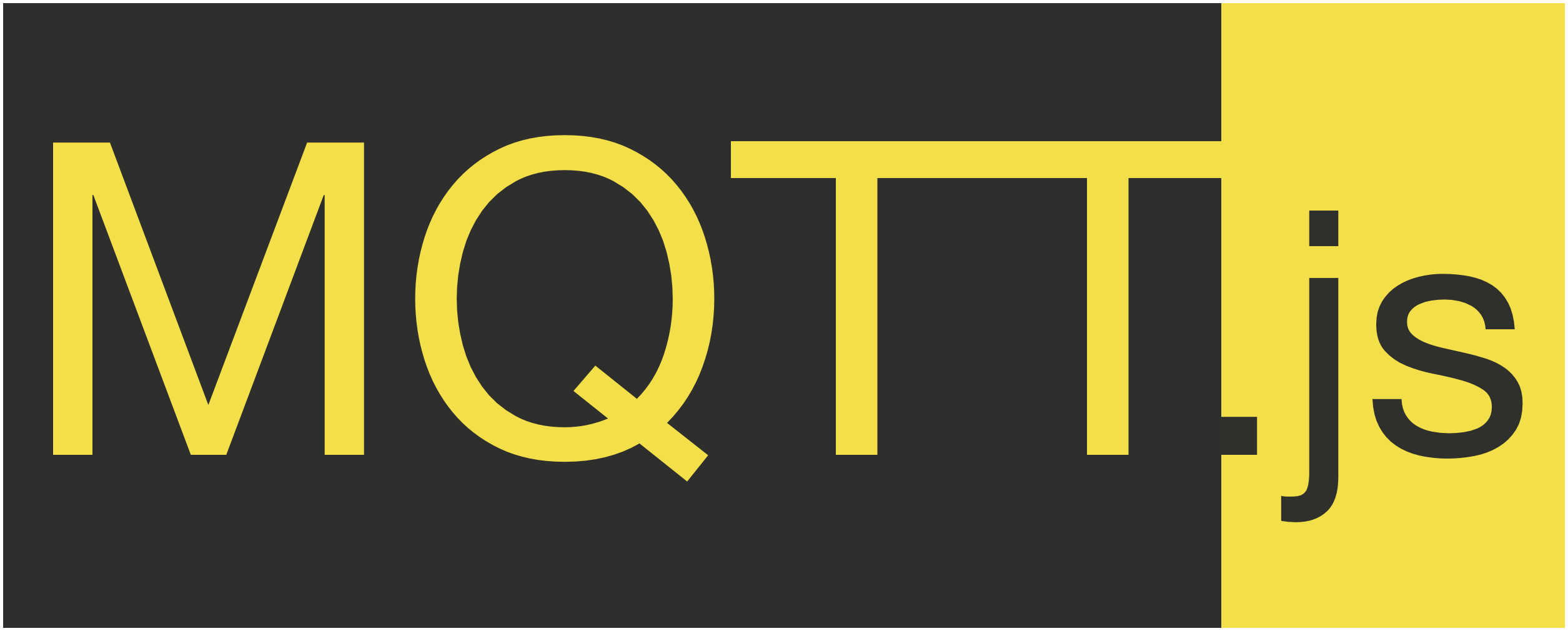
|
2 |
|
3 |  [](https://codecov.io/gh/mqttjs/MQTT.js)
|
4 |
|
5 | [](https://github.com/mqttjs/MQTT.js/graphs/commit-activity)
|
6 | [](https://github.com/mqttjs/MQTT.js/pulls)
|
7 |
|
8 | [  ](https://www.npmjs.com/package/mqtt)
|
9 |
|
10 | MQTT.js is a client library for the [MQTT](http://mqtt.org/) protocol, written
|
11 | in JavaScript for node.js and the browser.
|
12 |
|
13 | ## Table of Contents
|
14 |
|
15 | - [Upgrade notes](#notes)
|
16 | - [Installation](#install)
|
17 | - [Example](#example)
|
18 | - [React Native](#react-native)
|
19 | - [Import Styles](#example)
|
20 | - [Command Line Tools](#cli)
|
21 | - [API](#api)
|
22 | - [Browser](#browser)
|
23 | - [About QoS](#qos)
|
24 | - [TypeScript](#typescript)
|
25 | - [Weapp and Ali support](#weapp-alipay)
|
26 | - [Contributing](#contributing)
|
27 | - [Sponsor](#sponsor)
|
28 | - [License](#license)
|
29 |
|
30 | MQTT.js is an OPEN Open Source Project, see the [Contributing](#contributing) section to find out what this means.
|
31 |
|
32 | [](https://github.com/feross/standard)
|
34 |
|
35 | <a name="notes"></a>
|
36 |
|
37 | ## Important notes for existing users
|
38 |
|
39 | **v5.0.0** (07/2023)
|
40 |
|
41 | - Removes support for all end of life node versions (v12 and v14), and now supports node v18 and v20.
|
42 | - Completely rewritten in Typescript 🚀.
|
43 | - When creating `MqttClient` instance `new` is now required.
|
44 |
|
45 | **v4.0.0** (Released 04/2020) removes support for all end of life node versions, and now supports node v12 and v14. It also adds improvements to
|
46 | debug logging, along with some feature additions.
|
47 |
|
48 | As a **breaking change**, by default a error handler is built into the MQTT.js client, so if any
|
49 | errors are emitted and the user has not created an event handler on the client for errors, the client will
|
50 | not break as a result of unhandled errors. Additionally, typical TLS errors like `ECONNREFUSED`, `ECONNRESET` have been
|
51 | added to a list of TLS errors that will be emitted from the MQTT.js client, and so can be handled as connection errors.
|
52 |
|
53 | **v3.0.0** adds support for MQTT 5, support for node v10.x, and many fixes to improve reliability.
|
54 |
|
55 | **Note:** MQTT v5 support is experimental as it has not been implemented by brokers yet.
|
56 |
|
57 | **v2.0.0** removes support for node v0.8, v0.10 and v0.12, and it is 3x faster in sending
|
58 | packets. It also removes all the deprecated functionality in v1.0.0,
|
59 | mainly `mqtt.createConnection` and `mqtt.Server`. From v2.0.0,
|
60 | subscriptions are restored upon reconnection if `clean: true`.
|
61 | v1.x.x is now in _LTS_, and it will keep being supported as long as
|
62 | there are v0.8, v0.10 and v0.12 users.
|
63 |
|
64 | As a **breaking change**, the `encoding` option in the old client is
|
65 | removed, and now everything is UTF-8 with the exception of the
|
66 | `password` in the CONNECT message and `payload` in the PUBLISH message,
|
67 | which are `Buffer`.
|
68 |
|
69 | Another **breaking change** is that MQTT.js now defaults to MQTT v3.1.1,
|
70 | so to support old brokers, please read the [client options doc](#client).
|
71 |
|
72 | **v1.0.0** improves the overall architecture of the project, which is now
|
73 | split into three components: MQTT.js keeps the Client,
|
74 | [mqtt-connection](http://npm.im/mqtt-connection) includes the barebone
|
75 | Connection code for server-side usage, and [mqtt-packet](http://npm.im/mqtt-packet)
|
76 | includes the protocol parser and generator. The new Client improves
|
77 | performance by a 30% factor, embeds Websocket support
|
78 | ([MOWS](http://npm.im/mows) is now deprecated), and it has a better
|
79 | support for QoS 1 and 2. The previous API is still supported but
|
80 | deprecated, as such, it is not documented in this README.
|
81 |
|
82 | <a name="install"></a>
|
83 |
|
84 | ## Installation
|
85 |
|
86 | ```sh
|
87 | npm install mqtt --save
|
88 | ```
|
89 |
|
90 | <a name="example"></a>
|
91 |
|
92 | ## Example
|
93 |
|
94 | For the sake of simplicity, let's put the subscriber and the publisher in the same file:
|
95 |
|
96 | ```js
|
97 | const mqtt = require("mqtt");
|
98 | const client = mqtt.connect("mqtt://test.mosquitto.org");
|
99 |
|
100 | client.on("connect", () => {
|
101 | client.subscribe("presence", (err) => {
|
102 | if (!err) {
|
103 | client.publish("presence", "Hello mqtt");
|
104 | }
|
105 | });
|
106 | });
|
107 |
|
108 | client.on("message", (topic, message) => {
|
109 | // message is Buffer
|
110 | console.log(message.toString());
|
111 | client.end();
|
112 | });
|
113 | ```
|
114 |
|
115 | output:
|
116 |
|
117 | ```sh
|
118 | Hello mqtt
|
119 | ```
|
120 |
|
121 | <a name="example-react-native"></a>
|
122 |
|
123 | ### React Native
|
124 |
|
125 | MQTT.js can be used in React Native applications. To use it, see the [React Native example](https://github.com/MaximoLiberata/react-native-mqtt.js-example)
|
126 |
|
127 | If you want to run your own MQTT broker, you can use
|
128 | [Mosquitto](http://mosquitto.org) or
|
129 | [Aedes-cli](https://github.com/moscajs/aedes-cli), and launch it.
|
130 |
|
131 | You can also use a test instance: test.mosquitto.org.
|
132 |
|
133 | If you do not want to install a separate broker, you can try using the
|
134 | [Aedes](https://github.com/moscajs/aedes).
|
135 |
|
136 | <a name="import_styles"></a>
|
137 |
|
138 | ## Import styles
|
139 |
|
140 | ### CommonJS (Require)
|
141 |
|
142 | ```js
|
143 | const mqtt = require("mqtt") // require mqtt
|
144 | const client = mqtt.connect("mqtt://test.mosquitto.org") // create a client
|
145 | ```
|
146 |
|
147 | ### ES6 Modules (Import)
|
148 |
|
149 | #### Default import
|
150 |
|
151 | ```js
|
152 | import mqtt from "mqtt"; // import namespace "mqtt"
|
153 | let client = mqtt.connect("mqtt://test.mosquitto.org"); // create a client
|
154 | ```
|
155 |
|
156 | #### Importing individual components
|
157 |
|
158 | ```js
|
159 | import { connect } from "mqtt"; // import connect from mqtt
|
160 | let client = connect("mqtt://test.mosquitto.org"); // create a client
|
161 | ```
|
162 |
|
163 | <a name="cli"></a>
|
164 |
|
165 | ## Command Line Tools
|
166 |
|
167 | MQTT.js bundles a command to interact with a broker.
|
168 | In order to have it available on your path, you should install MQTT.js
|
169 | globally:
|
170 |
|
171 | ```sh
|
172 | npm install mqtt -g
|
173 | ```
|
174 |
|
175 | Then, on one terminal
|
176 |
|
177 | ```sh
|
178 | mqtt sub -t 'hello' -h 'test.mosquitto.org' -v
|
179 | ```
|
180 |
|
181 | On another
|
182 |
|
183 | ```sh
|
184 | mqtt pub -t 'hello' -h 'test.mosquitto.org' -m 'from MQTT.js'
|
185 | ```
|
186 |
|
187 | See `mqtt help <command>` for the command help.
|
188 |
|
189 | <a name="debug"></a>
|
190 |
|
191 | ## Debug Logs
|
192 |
|
193 | MQTT.js uses the [debug](https://www.npmjs.com/package/debug#cmd) package for debugging purposes. To enable debug logs, add the following environment variable on runtime :
|
194 |
|
195 | ```ps
|
196 | # (example using PowerShell, the VS Code default)
|
197 | $env:DEBUG='mqttjs*'
|
198 | ```
|
199 |
|
200 | <a name="reconnecting"></a>
|
201 |
|
202 | ## About Reconnection
|
203 |
|
204 | An important part of any websocket connection is what to do when a connection
|
205 | drops off and the client needs to reconnect. MQTT has built-in reconnection
|
206 | support that can be configured to behave in ways that suit the application.
|
207 |
|
208 | #### Refresh Authentication Options / Signed Urls with `transformWsUrl` (Websocket Only)
|
209 |
|
210 | When an mqtt connection drops and needs to reconnect, it's common to require
|
211 | that any authentication associated with the connection is kept current with
|
212 | the underlying auth mechanism. For instance some applications may pass an auth
|
213 | token with connection options on the initial connection, while other cloud
|
214 | services may require a url be signed with each connection.
|
215 |
|
216 | By the time the reconnect happens in the application lifecycle, the original
|
217 | auth data may have expired.
|
218 |
|
219 | To address this we can use a hook called `transformWsUrl` to manipulate
|
220 | either of the connection url or the client options at the time of a reconnect.
|
221 |
|
222 | Example (update clientId & username on each reconnect):
|
223 |
|
224 | ```js
|
225 | const transformWsUrl = (url, options, client) => {
|
226 | client.options.username = `token=${this.get_current_auth_token()}`;
|
227 | client.options.clientId = `${this.get_updated_clientId()}`;
|
228 |
|
229 | return `${this.get_signed_cloud_url(url)}`;
|
230 | }
|
231 |
|
232 | const connection = await mqtt.connectAsync(<wss url>, {
|
233 | ...,
|
234 | transformWsUrl: transformUrl,
|
235 | });
|
236 |
|
237 | ```
|
238 |
|
239 | Now every time a new WebSocket connection is opened (hopefully not too often),
|
240 | we will get a fresh signed url or fresh auth token data.
|
241 |
|
242 | Note: Currently this hook does _not_ support promises, meaning that in order to
|
243 | use the latest auth token, you must have some outside mechanism running that
|
244 | handles application-level authentication refreshing so that the websocket
|
245 | connection can simply grab the latest valid token or signed url.
|
246 |
|
247 | #### Customize Websockets with `createWebsocket` (Websocket Only)
|
248 |
|
249 | When you need to add a custom websocket subprotocol or header to open a connection
|
250 | through a proxy with custom authentication this callback allows you to create your own
|
251 | instance of a websocket which will be used in the mqtt client.
|
252 |
|
253 | ```js
|
254 | const createWebsocket = (url, websocketSubProtocols, options) => {
|
255 | const subProtocols = [
|
256 | websocketSubProtocols[0],
|
257 | 'myCustomSubprotocolOrOAuthToken',
|
258 | ]
|
259 | return new WebSocket(url, subProtocols)
|
260 | }
|
261 |
|
262 | const client = await mqtt.connectAsync(<wss url>, {
|
263 | ...,
|
264 | createWebsocket: createWebsocket,
|
265 | });
|
266 | ```
|
267 |
|
268 | #### Enabling Reconnection with `reconnectPeriod` option
|
269 |
|
270 | To ensure that the mqtt client automatically tries to reconnect when the
|
271 | connection is dropped, you must set the client option `reconnectPeriod` to a
|
272 | value greater than 0. A value of 0 will disable reconnection and then terminate
|
273 | the final connection when it drops.
|
274 |
|
275 | The default value is 1000 ms which means it will try to reconnect 1 second
|
276 | after losing the connection.
|
277 |
|
278 | <a name="topicalias"></a>
|
279 |
|
280 | ## About Topic Alias Management
|
281 |
|
282 | ### Enabling automatic Topic Alias using
|
283 |
|
284 | If the client sets the option `autoUseTopicAlias:true` then MQTT.js uses existing topic alias automatically.
|
285 |
|
286 | example scenario:
|
287 |
|
288 | ```bash
|
289 | 1. PUBLISH topic:'t1', ta:1 (register)
|
290 | 2. PUBLISH topic:'t1' -> topic:'', ta:1 (auto use existing map entry)
|
291 | 3. PUBLISH topic:'t2', ta:1 (register overwrite)
|
292 | 4. PUBLISH topic:'t2' -> topic:'', ta:1 (auto use existing map entry based on the receent map)
|
293 | 5. PUBLISH topic:'t1' (t1 is no longer mapped to ta:1)
|
294 | ```
|
295 |
|
296 | User doesn't need to manage which topic is mapped to which topic alias.
|
297 | If the user want to register topic alias, then publish topic with topic alias.
|
298 | If the user want to use topic alias, then publish topic without topic alias. If there is a mapped topic alias then added it as a property and update the topic to empty string.
|
299 |
|
300 | ### Enabling automatic Topic Alias assign
|
301 |
|
302 | If the client sets the option `autoAssignTopicAlias:true` then MQTT.js uses existing topic alias automatically.
|
303 | If no topic alias exists, then assign a new vacant topic alias automatically. If topic alias is fully used, then LRU(Least Recently Used) topic-alias entry is overwritten.
|
304 |
|
305 | example scenario:
|
306 |
|
307 | ```bash
|
308 | The broker returns CONNACK (TopicAliasMaximum:3)
|
309 | 1. PUBLISH topic:'t1' -> 't1', ta:1 (auto assign t1:1 and register)
|
310 | 2. PUBLISH topic:'t1' -> '' , ta:1 (auto use existing map entry)
|
311 | 3. PUBLISH topic:'t2' -> 't2', ta:2 (auto assign t1:2 and register. 2 was vacant)
|
312 | 4. PUBLISH topic:'t3' -> 't3', ta:3 (auto assign t1:3 and register. 3 was vacant)
|
313 | 5. PUBLISH topic:'t4' -> 't4', ta:1 (LRU entry is overwritten)
|
314 | ```
|
315 |
|
316 | Also user can manually register topic-alias pair using PUBLISH topic:'some', ta:X. It works well with automatic topic alias assign.
|
317 |
|
318 | <a name="api"></a>
|
319 |
|
320 | ## API
|
321 |
|
322 | - [`mqtt.connect()`](#connect)
|
323 | - [`mqtt.connectAsync()`](#connect-async)
|
324 | - [`mqtt.Client()`](#client)
|
325 | - [`mqtt.Client#connect()`](#client-connect)
|
326 | - [`mqtt.Client#publish()`](#publish)
|
327 | - [`mqtt.Client#publishAsync()`](#publish-async)
|
328 | - [`mqtt.Client#subscribe()`](#subscribe)
|
329 | - [`mqtt.Client#subscribeAsync()`](#subscribe-async)
|
330 | - [`mqtt.Client#unsubscribe()`](#unsubscribe)
|
331 | - [`mqtt.Client#unsubscribeAsync()`](#unsubscribe-async)
|
332 | - [`mqtt.Client#end()`](#end)
|
333 | - [`mqtt.Client#endAsync()`](#end-async)
|
334 | - [`mqtt.Client#removeOutgoingMessage()`](#removeOutgoingMessage)
|
335 | - [`mqtt.Client#reconnect()`](#reconnect)
|
336 | - [`mqtt.Client#handleMessage()`](#handleMessage)
|
337 | - [`mqtt.Client#connected`](#connected)
|
338 | - [`mqtt.Client#reconnecting`](#reconnecting)
|
339 | - [`mqtt.Client#getLastMessageId()`](#getLastMessageId)
|
340 | - [`mqtt.Store()`](#store)
|
341 | - [`mqtt.Store#put()`](#put)
|
342 | - [`mqtt.Store#del()`](#del)
|
343 | - [`mqtt.Store#createStream()`](#createStream)
|
344 | - [`mqtt.Store#close()`](#close)
|
345 |
|
346 | ---
|
347 |
|
348 | <a name="connect"></a>
|
349 |
|
350 | ### mqtt.connect([url], options)
|
351 |
|
352 | Connects to the broker specified by the given url and options and
|
353 | returns a [Client](#client).
|
354 |
|
355 | The URL can be on the following protocols: 'mqtt', 'mqtts', 'tcp',
|
356 | 'tls', 'ws', 'wss', 'wxs', 'alis'. If you are trying to connect to a unix socket just append the `+unix` suffix to the protocol (ex: `mqtt+unix`). This will set the `unixSocket` property automatically.
|
357 |
|
358 | The URL can also be an object as returned by
|
359 | [`URL.parse()`](http://nodejs.org/api/url.html#url_url_parse_urlstr_parsequerystring_slashesdenotehost),
|
360 | in that case the two objects are merged, i.e. you can pass a single
|
361 | object with both the URL and the connect options.
|
362 |
|
363 | You can also specify a `servers` options with content: `[{ host:
|
364 | 'localhost', port: 1883 }, ... ]`, in that case that array is iterated
|
365 | at every connect.
|
366 |
|
367 | For all MQTT-related options, see the [Client](#client)
|
368 | constructor.
|
369 |
|
370 | <a name="connect-async"></a>
|
371 |
|
372 | ### connectAsync([url], options)
|
373 |
|
374 | Asynchronous wrapper around the [`connect`](#connect) function.
|
375 |
|
376 | Returns a `Promise` that resolves to a `mqtt.Client` instance when the client
|
377 | fires a `'connect'` or `'end'` event, or rejects with an error if the `'error'`
|
378 | is fired.
|
379 |
|
380 | Note that the `manualConnect` option will cause the promise returned by this
|
381 | function to never resolve or reject as the underlying client never fires any
|
382 | events.
|
383 |
|
384 | ---
|
385 |
|
386 | <a name="client"></a>
|
387 |
|
388 | ### mqtt.Client(streamBuilder, options)
|
389 |
|
390 | The `Client` class wraps a client connection to an
|
391 | MQTT broker over an arbitrary transport method (TCP, TLS,
|
392 | WebSocket, ecc).
|
393 | `Client` is an [EventEmitter](https://nodejs.dev/en/learn/the-nodejs-event-emitter/) that has it's own [events](#events)
|
394 |
|
395 | `Client` automatically handles the following:
|
396 |
|
397 | - Regular server pings
|
398 | - QoS flow
|
399 | - Automatic reconnections
|
400 | - Start publishing before being connected
|
401 |
|
402 | The arguments are:
|
403 |
|
404 | - `streamBuilder` is a function that returns a subclass of the `Stream` class that supports
|
405 | the `connect` event. Typically a `net.Socket`.
|
406 | - `options` is the client connection options (see: the [connect packet](https://github.com/mcollina/mqtt-packet#connect)). Defaults:
|
407 | - `wsOptions`: is the WebSocket connection options. Default is `{}`.
|
408 | It's specific for WebSockets. For possible options have a look at: <https://github.com/websockets/ws/blob/master/doc/ws.md>.
|
409 | - `keepalive`: `60` seconds, set to `0` to disable
|
410 | - `reschedulePings`: reschedule ping messages after sending packets (default `true`)
|
411 | - `clientId`: `'mqttjs_' + Math.random().toString(16).substr(2, 8)`
|
412 | - `protocolId`: `'MQTT'`
|
413 | - `protocolVersion`: `4`
|
414 | - `clean`: `true`, set to false to receive QoS 1 and 2 messages while
|
415 | offline
|
416 | - `reconnectPeriod`: `1000` milliseconds, interval between two
|
417 | reconnections. Disable auto reconnect by setting to `0`.
|
418 | - `connectTimeout`: `30 * 1000` milliseconds, time to wait before a
|
419 | CONNACK is received
|
420 | - `username`: the username required by your broker, if any
|
421 | - `password`: the password required by your broker, if any
|
422 | - `incomingStore`: a [Store](#store) for the incoming packets
|
423 | - `outgoingStore`: a [Store](#store) for the outgoing packets
|
424 | - `queueQoSZero`: if connection is broken, queue outgoing QoS zero messages (default `true`)
|
425 | - `customHandleAcks`: MQTT 5 feature of custom handling puback and pubrec packets. Its callback:
|
426 |
|
427 | ```js
|
428 | customHandleAcks: function(topic, message, packet, done) {/*some logic with calling done(error, reasonCode)*/}
|
429 | ```
|
430 |
|
431 | - `autoUseTopicAlias`: enabling automatic Topic Alias using functionality
|
432 | - `autoAssignTopicAlias`: enabling automatic Topic Alias assign functionality
|
433 | - `properties`: properties MQTT 5.0.
|
434 | `object` that supports the following properties:
|
435 | - `sessionExpiryInterval`: representing the Session Expiry Interval in seconds `number`,
|
436 | - `receiveMaximum`: representing the Receive Maximum value `number`,
|
437 | - `maximumPacketSize`: representing the Maximum Packet Size the Client is willing to accept `number`,
|
438 | - `topicAliasMaximum`: representing the Topic Alias Maximum value indicates the highest value that the Client will accept as a Topic Alias sent by the Server `number`,
|
439 | - `requestResponseInformation`: The Client uses this value to request the Server to return Response Information in the CONNACK `boolean`,
|
440 | - `requestProblemInformation`: The Client uses this value to indicate whether the Reason String or User Properties are sent in the case of failures `boolean`,
|
441 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`,
|
442 | - `authenticationMethod`: the name of the authentication method used for extended authentication `string`,
|
443 | - `authenticationData`: Binary Data containing authentication data `binary`
|
444 | - `authPacket`: settings for auth packet `object`
|
445 | - `will`: a message that will sent by the broker automatically when
|
446 | the client disconnect badly. The format is:
|
447 | - `topic`: the topic to publish
|
448 | - `payload`: the message to publish
|
449 | - `qos`: the QoS
|
450 | - `retain`: the retain flag
|
451 | - `properties`: properties of will by MQTT 5.0:
|
452 | - `willDelayInterval`: representing the Will Delay Interval in seconds `number`,
|
453 | - `payloadFormatIndicator`: Will Message is UTF-8 Encoded Character Data or not `boolean`,
|
454 | - `messageExpiryInterval`: value is the lifetime of the Will Message in seconds and is sent as the Publication Expiry Interval when the Server publishes the Will Message `number`,
|
455 | - `contentType`: describing the content of the Will Message `string`,
|
456 | - `responseTopic`: String which is used as the Topic Name for a response message `string`,
|
457 | - `correlationData`: The Correlation Data is used by the sender of the Request Message to identify which request the Response Message is for when it is received `binary`,
|
458 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`
|
459 | - `transformWsUrl` : optional `(url, options, client) => url` function
|
460 | For ws/wss protocols only. Can be used to implement signing
|
461 | urls which upon reconnect can have become expired.
|
462 | - `createWebsocket` : optional `url, websocketSubProtocols, options) => Websocket` function
|
463 | For ws/wss protocols only. Can be used to implement a custom websocket subprotocol or implementation.
|
464 | - `resubscribe` : if connection is broken and reconnects,
|
465 | subscribed topics are automatically subscribed again (default `true`)
|
466 | - `messageIdProvider`: custom messageId provider. when `new UniqueMessageIdProvider()` is set, then non conflict messageId is provided.
|
467 | - `log`: custom log function. Default uses [debug](https://www.npmjs.com/package/debug) package.
|
468 | - `manualConnect`: prevents the constructor to call `connect`. In this case after the `mqtt.connect` is called you should call `client.connect` manually.
|
469 | - `timerVariant`: defaults to `auto`, which tries to determine which timer is most appropriate for you environment, if you're having detection issues, you can set it to `worker` or `native`. If none suits you, you can pass a timer object with set and clear properties:
|
470 | ```js
|
471 | timerVariant: {
|
472 | set: (func, timer) => setInterval(func, timer),
|
473 | clear: (id) => clearInterval(id)
|
474 | }
|
475 | ```
|
476 |
|
477 | - `unixSocket`: if you want to connect to a unix socket, set this to true
|
478 |
|
479 | In case mqtts (mqtt over tls) is required, the `options` object is passed through to [`tls.connect()`](http://nodejs.org/api/tls.html#tls_tls_connect_options_callback). If using a **self-signed certificate**, set `rejectUnauthorized: false`. However, be cautious as this exposes you to potential man in the middle attacks and isn't recommended for production.
|
480 |
|
481 | For those supporting multiple TLS protocols on a single port, like MQTTS and MQTT over WSS, utilize the `ALPNProtocols` option. This lets you define the Application Layer Protocol Negotiation (ALPN) protocol. You can set `ALPNProtocols` as a string array, Buffer, or Uint8Array based on your setup.
|
482 |
|
483 | If you are connecting to a broker that supports only MQTT 3.1 (not
|
484 | 3.1.1 compliant), you should pass these additional options:
|
485 |
|
486 | ```js
|
487 | {
|
488 | protocolId: 'MQIsdp',
|
489 | protocolVersion: 3
|
490 | }
|
491 | ```
|
492 |
|
493 | This is confirmed on RabbitMQ 3.2.4, and on Mosquitto < 1.3. Mosquitto
|
494 | version 1.3 and 1.4 works fine without those.
|
495 |
|
496 | <a name="events"></a>
|
497 |
|
498 | #### Event `'connect'`
|
499 |
|
500 | `function (connack) {}`
|
501 |
|
502 | Emitted on successful (re)connection (i.e. connack rc=0).
|
503 |
|
504 | - `connack` received connack packet. When `clean` connection option is `false` and server has a previous session
|
505 | for `clientId` connection option, then `connack.sessionPresent` flag is `true`. When that is the case,
|
506 | you may rely on stored session and prefer not to send subscribe commands for the client.
|
507 |
|
508 | #### Event `'reconnect'`
|
509 |
|
510 | `function () {}`
|
511 |
|
512 | Emitted when a reconnect starts.
|
513 |
|
514 | #### Event `'close'`
|
515 |
|
516 | `function () {}`
|
517 |
|
518 | Emitted after a disconnection.
|
519 |
|
520 | #### Event `'disconnect'`
|
521 |
|
522 | `function (packet) {}`
|
523 |
|
524 | Emitted after receiving disconnect packet from broker. MQTT 5.0 feature.
|
525 |
|
526 | #### Event `'offline'`
|
527 |
|
528 | `function () {}`
|
529 |
|
530 | Emitted when the client goes offline.
|
531 |
|
532 | #### Event `'error'`
|
533 |
|
534 | `function (error) {}`
|
535 |
|
536 | Emitted when the client cannot connect (i.e. connack rc != 0) or when a
|
537 | parsing error occurs.
|
538 |
|
539 | The following TLS errors will be emitted as an `error` event:
|
540 |
|
541 | - `ECONNREFUSED`
|
542 | - `ECONNRESET`
|
543 | - `EADDRINUSE`
|
544 | - `ENOTFOUND`
|
545 |
|
546 | #### Event `'end'`
|
547 |
|
548 | `function () {}`
|
549 |
|
550 | Emitted when [`mqtt.Client#end()`](#end) is called.
|
551 | If a callback was passed to `mqtt.Client#end()`, this event is emitted once the
|
552 | callback returns.
|
553 |
|
554 | #### Event `'message'`
|
555 |
|
556 | `function (topic, message, packet) {}`
|
557 |
|
558 | Emitted when the client receives a publish packet
|
559 |
|
560 | - `topic` topic of the received packet
|
561 | - `message` payload of the received packet
|
562 | - `packet` received packet, as defined in
|
563 | [mqtt-packet](https://github.com/mcollina/mqtt-packet#publish)
|
564 |
|
565 | #### Event `'packetsend'`
|
566 |
|
567 | `function (packet) {}`
|
568 |
|
569 | Emitted when the client sends any packet. This includes .published() packets
|
570 | as well as packets used by MQTT for managing subscriptions and connections
|
571 |
|
572 | - `packet` received packet, as defined in
|
573 | [mqtt-packet](https://github.com/mcollina/mqtt-packet)
|
574 |
|
575 | #### Event `'packetreceive'`
|
576 |
|
577 | `function (packet) {}`
|
578 |
|
579 | Emitted when the client receives any packet. This includes packets from
|
580 | subscribed topics as well as packets used by MQTT for managing subscriptions
|
581 | and connections
|
582 |
|
583 | - `packet` received packet, as defined in
|
584 | [mqtt-packet](https://github.com/mcollina/mqtt-packet)
|
585 |
|
586 | ---
|
587 |
|
588 | <a name="client-connect"></a>
|
589 |
|
590 | ### mqtt.Client#connect()
|
591 |
|
592 | By default client connects when constructor is called. To prevent this you can set `manualConnect` option to `true` and call `client.connect()` manually.
|
593 |
|
594 | <a name="publish"></a>
|
595 |
|
596 | ### mqtt.Client#publish(topic, message, [options], [callback])
|
597 |
|
598 | Publish a message to a topic
|
599 |
|
600 | - `topic` is the topic to publish to, `String`
|
601 | - `message` is the message to publish, `Buffer` or `String`
|
602 | - `options` is the options to publish with, including:
|
603 | - `qos` QoS level, `Number`, default `0`
|
604 | - `retain` retain flag, `Boolean`, default `false`
|
605 | - `dup` mark as duplicate flag, `Boolean`, default `false`
|
606 | - `properties`: MQTT 5.0 properties `object`
|
607 | - `payloadFormatIndicator`: Payload is UTF-8 Encoded Character Data or not `boolean`,
|
608 | - `messageExpiryInterval`: the lifetime of the Application Message in seconds `number`,
|
609 | - `topicAlias`: value that is used to identify the Topic instead of using the Topic Name `number`,
|
610 | - `responseTopic`: String which is used as the Topic Name for a response message `string`,
|
611 | - `correlationData`: used by the sender of the Request Message to identify which request the Response Message is for when it is received `binary`,
|
612 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`,
|
613 | - `subscriptionIdentifier`: representing the identifier of the subscription `number`,
|
614 | - `contentType`: String describing the content of the Application Message `string`
|
615 | - `cbStorePut` - `function ()`, fired when message is put into `outgoingStore` if QoS is `1` or `2`.
|
616 | - `callback` - `function (err)`, fired when the QoS handling completes,
|
617 | or at the next tick if QoS 0. An error occurs if client is disconnecting.
|
618 |
|
619 | <a name="publish-async"></a>
|
620 |
|
621 | ### mqtt.Client#publishAsync(topic, message, [options])
|
622 |
|
623 | Async [`publish`](#publish). Returns a `Promise<void>`.
|
624 |
|
625 | ---
|
626 |
|
627 | <a name="subscribe"></a>
|
628 |
|
629 | ### mqtt.Client#subscribe(topic/topic array/topic object, [options], [callback])
|
630 |
|
631 | Subscribe to a topic or topics
|
632 |
|
633 | - `topic` is a `String` topic to subscribe to or an `Array` of
|
634 | topics to subscribe to. It can also be an object, it has as object
|
635 | keys the topic name and as value the QoS, like `{'test1': {qos: 0}, 'test2': {qos: 1}}`.
|
636 | MQTT `topic` wildcard characters are supported (`+` - for single level and `#` - for multi level)
|
637 | - `options` is the options to subscribe with, including:
|
638 | - `qos` QoS subscription level, default 0
|
639 | - `nl` No Local MQTT 5.0 flag (If the value is true, Application Messages MUST NOT be forwarded to a connection with a ClientID equal to the ClientID of the publishing connection)
|
640 | - `rap` Retain as Published MQTT 5.0 flag (If true, Application Messages forwarded using this subscription keep the RETAIN flag they were published with. If false, Application Messages forwarded using this subscription have the RETAIN flag set to 0.)
|
641 | - `rh` Retain Handling MQTT 5.0 (This option specifies whether retained messages are sent when the subscription is established.)
|
642 | - `properties`: `object`
|
643 | - `subscriptionIdentifier`: representing the identifier of the subscription `number`,
|
644 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`
|
645 | - `callback` - `function (err, granted)`
|
646 | callback fired on suback where:
|
647 | - `err` a subscription error or an error that occurs when client is disconnecting
|
648 | - `granted` is an array of `{topic, qos}` where:
|
649 | - `topic` is a subscribed to topic
|
650 | - `qos` is the granted QoS level on it
|
651 |
|
652 | <a name="subscribe-async"></a>
|
653 |
|
654 | ### mqtt.Client#subscribeAsync(topic/topic array/topic object, [options])
|
655 |
|
656 | Async [`subscribe`](#subscribe). Returns a `Promise<granted[]>`.
|
657 |
|
658 | ---
|
659 |
|
660 | <a name="unsubscribe"></a>
|
661 |
|
662 | ### mqtt.Client#unsubscribe(topic/topic array, [options], [callback])
|
663 |
|
664 | Unsubscribe from a topic or topics
|
665 |
|
666 | - `topic` is a `String` topic or an array of topics to unsubscribe from
|
667 | - `options`: options of unsubscribe.
|
668 | - `properties`: `object`
|
669 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`
|
670 | - `callback` - `function (err)`, fired on unsuback. An error occurs if client is disconnecting.
|
671 |
|
672 | <a name="unsubscribe-async"></a>
|
673 |
|
674 | ### mqtt.Client#unsubscribeAsync(topic/topic array, [options])
|
675 |
|
676 | Async [`unsubscribe`](#unsubscribe). Returns a `Promise<void>`.
|
677 |
|
678 | ---
|
679 |
|
680 | <a name="end"></a>
|
681 |
|
682 | ### mqtt.Client#end([force], [options], [callback])
|
683 |
|
684 | Close the client, accepts the following options:
|
685 |
|
686 | - `force`: passing it to true will close the client right away, without
|
687 | waiting for the in-flight messages to be acked. This parameter is
|
688 | optional.
|
689 | - `options`: options of disconnect.
|
690 | - `reasonCode`: Disconnect Reason Code `number`
|
691 | - `properties`: `object`
|
692 | - `sessionExpiryInterval`: representing the Session Expiry Interval in seconds `number`,
|
693 | - `reasonString`: representing the reason for the disconnect `string`,
|
694 | - `userProperties`: The User Property is allowed to appear multiple times to represent multiple name, value pairs `object`,
|
695 | - `serverReference`: String which can be used by the Client to identify another Server to use `string`
|
696 | - `callback`: will be called when the client is closed. This parameter is
|
697 | optional.
|
698 |
|
699 | <a name="end-async"></a>
|
700 |
|
701 | ### mqtt.Client#endAsync([force], [options])
|
702 |
|
703 | Async [`end`](#end). Returns a `Promise<void>`.
|
704 |
|
705 | ---
|
706 |
|
707 | <a name="removeOutgoingMessage"></a>
|
708 |
|
709 | ### mqtt.Client#removeOutgoingMessage(mId)
|
710 |
|
711 | Remove a message from the outgoingStore.
|
712 | The outgoing callback will be called with Error('Message removed') if the message is removed.
|
713 |
|
714 | After this function is called, the messageId is released and becomes reusable.
|
715 |
|
716 | - `mId`: The messageId of the message in the outgoingStore.
|
717 |
|
718 | ---
|
719 |
|
720 | <a name="reconnect"></a>
|
721 |
|
722 | ### mqtt.Client#reconnect()
|
723 |
|
724 | Connect again using the same options as connect()
|
725 |
|
726 | ---
|
727 |
|
728 | <a name="handleMessage"></a>
|
729 |
|
730 | ### mqtt.Client#handleMessage(packet, callback)
|
731 |
|
732 | Handle messages with backpressure support, one at a time.
|
733 | Override at will, but **always call `callback`**, or the client
|
734 | will hang.
|
735 |
|
736 | ---
|
737 |
|
738 | <a name="connected"></a>
|
739 |
|
740 | ### mqtt.Client#connected
|
741 |
|
742 | Boolean : set to `true` if the client is connected. `false` otherwise.
|
743 |
|
744 | ---
|
745 |
|
746 | <a name="getLastMessageId"></a>
|
747 |
|
748 | ### mqtt.Client#getLastMessageId()
|
749 |
|
750 | Number : get last message id. This is for sent messages only.
|
751 |
|
752 | ---
|
753 |
|
754 | <a name="reconnecting"></a>
|
755 |
|
756 | ### mqtt.Client#reconnecting
|
757 |
|
758 | Boolean : set to `true` if the client is trying to reconnect to the server. `false` otherwise.
|
759 |
|
760 | ---
|
761 |
|
762 | <a name="store"></a>
|
763 |
|
764 | ### mqtt.Store(options)
|
765 |
|
766 | In-memory implementation of the message store.
|
767 |
|
768 | - `options` is the store options:
|
769 | - `clean`: `true`, clean inflight messages when close is called (default `true`)
|
770 |
|
771 | Other implementations of `mqtt.Store`:
|
772 |
|
773 | - [mqtt-jsonl-store](https://github.com/robertsLando/mqtt-jsonl-store) which uses
|
774 | [jsonl-db](https://github.com/AlCalzone/jsonl-db) to store inflight data, it works only on Node.
|
775 | - [mqtt-level-store](http://npm.im/mqtt-level-store) which uses
|
776 | [Level-browserify](http://npm.im/level-browserify) to store the inflight
|
777 | data, making it usable both in Node and the Browser.
|
778 | - [mqtt-nedb-store](https://github.com/behrad/mqtt-nedb-store) which
|
779 | uses [nedb](https://www.npmjs.com/package/nedb) to store the inflight
|
780 | data.
|
781 | - [mqtt-localforage-store](http://npm.im/mqtt-localforage-store) which uses
|
782 | [localForage](http://npm.im/localforage) to store the inflight
|
783 | data, making it usable in the Browser without browserify.
|
784 |
|
785 | ---
|
786 |
|
787 | <a name="put"></a>
|
788 |
|
789 | ### mqtt.Store#put(packet, callback)
|
790 |
|
791 | Adds a packet to the store, a packet is
|
792 | anything that has a `messageId` property.
|
793 | The callback is called when the packet has been stored.
|
794 |
|
795 | ---
|
796 |
|
797 | <a name="createStream"></a>
|
798 |
|
799 | ### mqtt.Store#createStream()
|
800 |
|
801 | Creates a stream with all the packets in the store.
|
802 |
|
803 | ---
|
804 |
|
805 | <a name="del"></a>
|
806 |
|
807 | ### mqtt.Store#del(packet, cb)
|
808 |
|
809 | Removes a packet from the store, a packet is
|
810 | anything that has a `messageId` property.
|
811 | The callback is called when the packet has been removed.
|
812 |
|
813 | ---
|
814 |
|
815 | <a name="close"></a>
|
816 |
|
817 | ### mqtt.Store#close(cb)
|
818 |
|
819 | Closes the Store.
|
820 |
|
821 | <a name="browser"></a>
|
822 | <a name="webpack"></a>
|
823 | <a name="vite"></a>
|
824 |
|
825 | ## Browser
|
826 |
|
827 | > [!IMPORTANT]
|
828 | > The only protocol supported in browsers is MQTT over WebSockets, so you must use `ws://` or `wss://` protocols.
|
829 |
|
830 | While the [ws](https://www.npmjs.com/package/ws) module is used in NodeJS, [WebSocket](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket) is used in browsers. This is totally transparent to users except for the following:
|
831 |
|
832 | - The `wsOption` is not supported in browsers.
|
833 | - Browsers doesn't allow to catch many WebSocket errors for [security reasons](https://stackoverflow.com/a/31003057) as:
|
834 |
|
835 | > Access to this information could allow a malicious Web page to gain information about your network, so they require browsers report all connection-time errors in an indistinguishable way.
|
836 |
|
837 | So listening for `client.on('error')` may not catch all the errors you would get in NodeJS env.
|
838 |
|
839 | ### Bundle
|
840 |
|
841 | MQTT.js is bundled using [esbuild](https://esbuild.github.io/). It is tested working with all bundlers like Webpack, Vite and React.
|
842 |
|
843 | You can find all mqtt bundles versions in `dist` folder:
|
844 |
|
845 | - `mqtt.js` - iife format, not minified
|
846 | - `mqtt.min.js` - iife format, minified
|
847 | - `mqtt.esm.js` - esm format minified
|
848 |
|
849 | Starting from MQTT.js > 5.2.0 you can import mqtt in your code like this:
|
850 |
|
851 | ```js
|
852 | import mqtt from 'mqtt'
|
853 | ```
|
854 |
|
855 | This will be automatically handled by your bundler.
|
856 |
|
857 | Otherwise you can choose to use a specific bundle like:
|
858 |
|
859 | ```js
|
860 | import * as mqtt from 'mqtt/dist/mqtt'
|
861 | import * as mqtt from 'mqtt/dist/mqtt.min'
|
862 | import mqtt from 'mqtt/dist/mqtt.esm'
|
863 | ```
|
864 |
|
865 | <a name="cdn"></a>
|
866 |
|
867 | ### Via CDN
|
868 |
|
869 | The MQTT.js bundle is available through <http://unpkg.com>, specifically
|
870 | at <https://unpkg.com/mqtt/dist/mqtt.min.js>.
|
871 | See <http://unpkg.com> for the full documentation on version ranges.
|
872 |
|
873 | <a name="qos"></a>
|
874 |
|
875 | ## About QoS
|
876 |
|
877 | Here is how QoS works:
|
878 |
|
879 | - QoS 0 : received **at most once** : The packet is sent, and that's it. There is no validation about whether it has been received.
|
880 | - QoS 1 : received **at least once** : The packet is sent and stored as long as the client has not received a confirmation from the server. MQTT ensures that it _will_ be received, but there can be duplicates.
|
881 | - QoS 2 : received **exactly once** : Same as QoS 1 but there is no duplicates.
|
882 |
|
883 | About data consumption, obviously, QoS 2 > QoS 1 > QoS 0, if that's a concern to you.
|
884 |
|
885 | <a name="typescript"></a>
|
886 |
|
887 | ## Usage with TypeScript
|
888 |
|
889 | Starting from v5 this project is written in TypeScript and the type definitions are included in the package.
|
890 |
|
891 | Example:
|
892 |
|
893 | ```ts
|
894 | import { connect } from "mqtt"
|
895 | const client = connect('mqtt://test.mosquitto.org')
|
896 | ```
|
897 |
|
898 | <a name="weapp-alipay"></a>
|
899 |
|
900 | ## WeChat and Ali Mini Program support
|
901 |
|
902 | ### WeChat Mini Program
|
903 |
|
904 | Supports [WeChat Mini Program](https://mp.weixin.qq.com/). Use the `wxs` protocol. See [the WeChat docs](https://mp.weixin.qq.com/debug/wxadoc/dev/api/network-socket.html).
|
905 |
|
906 | ```js
|
907 | import 'abortcontroller-polyfill/dist/abortcontroller-polyfill-only' // import before mqtt.
|
908 | const mqtt = require("mqtt");
|
909 | const client = mqtt.connect("wxs://test.mosquitto.org", {
|
910 | timerVariant: 'native' // more info ref issue: #1797
|
911 | });
|
912 | ```
|
913 |
|
914 | ### Ali Mini Program
|
915 |
|
916 | Supports [Ali Mini Program](https://open.alipay.com/channel/miniIndex.htm). Use the `alis` protocol. See [the Alipay docs](https://docs.alipay.com/mini/developer/getting-started).
|
917 | <a name="example"></a>
|
918 |
|
919 | ```js
|
920 | const mqtt = require("mqtt");
|
921 | const client = mqtt.connect("alis://test.mosquitto.org");
|
922 | ```
|
923 |
|
924 | <a name="contributing"></a>
|
925 |
|
926 | ## Contributing
|
927 |
|
928 | MQTT.js is an **OPEN Open Source Project**. This means that:
|
929 |
|
930 | > Individuals making significant and valuable contributions are given commit-access to the project to contribute as they see fit. This project is more like an open wiki than a standard guarded open source project.
|
931 |
|
932 | See the [CONTRIBUTING.md](https://github.com/mqttjs/MQTT.js/blob/master/CONTRIBUTING.md) file for more details.
|
933 |
|
934 | ### Contributors
|
935 |
|
936 | MQTT.js is only possible due to the excellent work of the following contributors:
|
937 |
|
938 | | Name | GitHub | Twitter |
|
939 | | ------------------ | -------------------------------------------------- | ---------------------------------------------------------- |
|
940 | | Adam Rudd | [GitHub/adamvr](https://github.com/adamvr) | [Twitter/@adam_vr](http://twitter.com/adam_vr) |
|
941 | | Matteo Collina | [GitHub/mcollina](https://github.com/mcollina) | [Twitter/@matteocollina](http://twitter.com/matteocollina) |
|
942 | | Maxime Agor | [GitHub/4rzael](https://github.com/4rzael) | [Twitter/@4rzael](http://twitter.com/4rzael) |
|
943 | | Siarhei Buntsevich | [GitHub/scarry1992](https://github.com/scarry1992) | |
|
944 | | Daniel Lando | [GitHub/robertsLando](https://github.com/robertsLando) | |
|
945 |
|
946 | <a name="sponsor"></a>
|
947 |
|
948 | ## Sponsor
|
949 |
|
950 | If you would like to support MQTT.js, please consider sponsoring the author and active maintainers:
|
951 |
|
952 | - [Matteo Collina](https://github.com/sponsors/mcollina): author of MQTT.js
|
953 | - [Daniel Lando](https://github.com/sponsors/robertsLando): active maintainer
|
954 |
|
955 | <a name="license"></a>
|
956 |
|
957 | ## License
|
958 |
|
959 | MIT
|