1 | <h1 align="center">Angular PDF Viewer</h1>
|
2 | <p align="center">
|
3 | <a href="https://www.npmjs.com/package/ng2-pdf-viewer">
|
4 | <img src="https://img.shields.io/npm/dm/ng2-pdf-viewer.svg?style=flat" alt="downloads">
|
5 | </a>
|
6 | <a href="https://badge.fury.io/js/ng2-pdf-viewer">
|
7 | <img src="https://badge.fury.io/js/ng2-pdf-viewer.svg" alt="npm version">
|
8 | </a>
|
9 | <a href="https://gitter.im/ngx-pdf-viewer/Lobby" title="Gitter">
|
10 | <img src="https://img.shields.io/gitter/room/nwjs/nw.js.svg" alt="Gitter"/>
|
11 | </a>
|
12 | <a href="https://www.paypal.me/vadimdez" title="Donate to this project using Paypal">
|
13 | <img src="https://img.shields.io/badge/paypal-donate-yellow.svg" alt="PayPal donate button" />
|
14 | </a>
|
15 | </p>
|
16 |
|
17 | > PDF Viewer Component for Angular 5+
|
18 |
|
19 | ### Demo page
|
20 |
|
21 | [https://vadimdez.github.io/ng2-pdf-viewer/](https://vadimdez.github.io/ng2-pdf-viewer/)
|
22 |
|
23 | #### Stackblitz Example
|
24 |
|
25 | [https://stackblitz.com/edit/ng2-pdf-viewer](https://stackblitz.com/edit/ng2-pdf-viewer)
|
26 |
|
27 | ### Blog post
|
28 |
|
29 | [https://medium.com/@vadimdez/render-pdf-in-angular-4-927e31da9c76](https://medium.com/@vadimdez/render-pdf-in-angular-4-927e31da9c76)
|
30 |
|
31 | ## Overview
|
32 |
|
33 | * [Install](README.md#install)
|
34 | * [Usage](README.md#usage)
|
35 | * [Options](README.md#options)
|
36 | * [Render local PDF file](README.md#render-local-pdf-file)
|
37 | * [Set custom path to the worker](README.md#set-custom-path-to-the-worker)
|
38 | * [Search in the PDF](README.md#search-in-the-pdf)
|
39 | * [Contribute](README.md#contribute)
|
40 |
|
41 | ## Install
|
42 |
|
43 | ### Angular >= 12
|
44 | ```
|
45 | npm install ng2-pdf-viewer
|
46 | ```
|
47 | > Partial Ivy compilated library bundles.
|
48 |
|
49 | ### Angular >= 4
|
50 | ```
|
51 | npm install ng2-pdf-viewer@^7.0.0
|
52 | ```
|
53 |
|
54 | ### Angular < 4
|
55 | ```
|
56 | npm install ng2-pdf-viewer@~3.0.8
|
57 | ```
|
58 |
|
59 | ## Usage
|
60 |
|
61 | *In case you're using ```systemjs``` see configuration [here](https://github.com/VadimDez/ng2-pdf-viewer/blob/master/SYSTEMJS.md).*
|
62 |
|
63 | Add ```PdfViewerModule``` to your module's ```imports```
|
64 |
|
65 | ```typescript
|
66 | import { NgModule } from '@angular/core';
|
67 | import { BrowserModule } from '@angular/platform-browser';
|
68 | import { AppComponent } from './app/app.component';
|
69 |
|
70 | import { PdfViewerModule } from 'ng2-pdf-viewer';
|
71 |
|
72 | @NgModule({
|
73 | imports: [BrowserModule, PdfViewerModule],
|
74 | declarations: [AppComponent],
|
75 | bootstrap: [AppComponent]
|
76 | })
|
77 |
|
78 | class AppModule {}
|
79 |
|
80 | platformBrowserDynamic().bootstrapModule(AppModule);
|
81 | ```
|
82 |
|
83 | And then use it in your component
|
84 |
|
85 | ```typescript
|
86 | import { Component } from '@angular/core';
|
87 |
|
88 | @Component({
|
89 | selector: 'example-app',
|
90 | template: `
|
91 | <pdf-viewer [src]="pdfSrc"
|
92 | [render-text]="true"
|
93 | [original-size]="false"
|
94 | style="width: 400px; height: 500px"
|
95 | ></pdf-viewer>
|
96 | `
|
97 | })
|
98 | export class AppComponent {
|
99 | pdfSrc = "https://vadimdez.github.io/ng2-pdf-viewer/assets/pdf-test.pdf";
|
100 | }
|
101 | ```
|
102 |
|
103 | ## Options
|
104 |
|
105 | * [[src]](#src)
|
106 | * [[(page)]](#page)
|
107 | * [[stick-to-page]](#stick-to-page)
|
108 | * [[external-link-target]](#external-link-target)
|
109 | * [[render-text]](#render-text)
|
110 | * [[render-text-mode]](#render-text-mode)
|
111 | * [[rotation]](#rotation)
|
112 | * [[zoom]](#zoom)
|
113 | * [[zoom-scale]](#zoom-scale)
|
114 | * [[original-size]](#original-size)
|
115 | * [[fit-to-page]](#fit-to-page)
|
116 | * [[show-all]](#show-all)
|
117 | * [[autoresize]](#autoresize)
|
118 | * [[c-maps-url]](#c-maps-url)
|
119 | * [[show-borders]](#show-borders)
|
120 | * [(after-load-complete)](#after-load-complete)
|
121 | * [(page-rendered)](#page-rendered)
|
122 | * [(text-layer-rendered)](#text-layer-rendered)
|
123 | * [(error)](#error)
|
124 | * [(on-progress)](#on-progress)
|
125 |
|
126 | #### [src]
|
127 |
|
128 | | Property | Type | Required |
|
129 | | --- | ---- | --- |
|
130 | | [src] | *string, object, UInt8Array* | Required |
|
131 |
|
132 | Pass pdf location
|
133 |
|
134 | ```
|
135 | [src]="'https://vadimdez.github.io/ng2-pdf-viewer/assets/pdf-test.pdf'"
|
136 | ```
|
137 |
|
138 | For more control you can pass options object to ```[src]```. [See other attributes for the object here](https://github.com/mozilla/pdf.js/blob/master/src/display/api.js#L130-L222).
|
139 |
|
140 | Options object for loading protected PDF would be:
|
141 |
|
142 | ```js
|
143 | {
|
144 | url: 'https://vadimdez.github.io/ng2-pdf-viewer/assets/pdf-test.pdf',
|
145 | withCredentials: true
|
146 | }
|
147 | ```
|
148 |
|
149 | #### [page]
|
150 |
|
151 |
|
152 | | Property | Type | Required |
|
153 | | --- | ---- | --- |
|
154 | | [page] or [(page)] | *number* | *Required* with [show-all]="false" or *Optional* with [show-all]="true" |
|
155 |
|
156 | Page number
|
157 |
|
158 | ```
|
159 | [page]="1"
|
160 | ```
|
161 | supports two way data binding as well
|
162 | ```
|
163 | [(page)]="pageVariable"
|
164 | ```
|
165 |
|
166 | If you want that the `two way data binding` actually updates your `page` variable on page change/scroll - you have to be sure that you define the height of the container, for example:
|
167 | ```css
|
168 | pdf-viewer {
|
169 | height: 100vh;
|
170 | }
|
171 | ```
|
172 |
|
173 | #### [stick-to-page]
|
174 |
|
175 | | Property | Type | Required |
|
176 | | --- | ---- | --- |
|
177 | | [stick-to-page] | *boolean* | *Optional* |
|
178 |
|
179 | Sticks view to the page. Works in combination with `[show-all]="true"` and `page`.
|
180 |
|
181 | ```
|
182 | [stick-to-page]="true"
|
183 | ```
|
184 |
|
185 | #### [render-text]
|
186 |
|
187 | | Property | Type | Required |
|
188 | | --- | ---- | --- |
|
189 | | [render-text] | *boolean* | *Optional* |
|
190 |
|
191 | Enable text rendering, allows to select text
|
192 | ```
|
193 | [render-text]="true"
|
194 | ```
|
195 |
|
196 | #### [render-text-mode]
|
197 |
|
198 | | Property | Type | Required |
|
199 | | --- | ---- | --- |
|
200 | | [render-text-mode] | *RenderTextMode* | *Optional* |
|
201 |
|
202 | Used in combination with `[render-text]="true"`
|
203 |
|
204 | Controls if the text layer is enabled, and the selection mode that is used.
|
205 |
|
206 | `0 = RenderTextMode.DISABLED` - disable the text selection layer
|
207 |
|
208 | `1 = RenderTextMode.ENABLED` - enables the text selection layer
|
209 |
|
210 | `2 = RenderTextMode.ENHANCED` - enables enhanced text selection
|
211 |
|
212 | ```
|
213 | [render-text-mode]="1"
|
214 | ```
|
215 |
|
216 | #### [external-link-target]
|
217 |
|
218 | | Property | Type | Required |
|
219 | | --- | ---- | --- |
|
220 | | [external-link-target] | *string* | *Optional* |
|
221 |
|
222 | Used in combination with `[render-text]="true"`
|
223 |
|
224 | Link target
|
225 | * `blank`
|
226 | * `none`
|
227 | * `self`
|
228 | * `parent`
|
229 | * `top`
|
230 | ```
|
231 | [external-link-target]="'blank'"
|
232 | ```
|
233 |
|
234 | #### [rotation]
|
235 |
|
236 | | Property | Type | Required |
|
237 | | --- | ---- | --- |
|
238 | | [rotation] | *number* | *Optional* |
|
239 |
|
240 | Rotate PDF
|
241 |
|
242 | *Allowed step is 90 degree, ex. 0, 90, 180*
|
243 | ```
|
244 | [rotation]="90"
|
245 | ```
|
246 |
|
247 | #### [zoom]
|
248 |
|
249 | | Property | Type | Required |
|
250 | | --- | ---- | --- |
|
251 | | [zoom] | *number* | *Optional* |
|
252 |
|
253 | Zoom pdf
|
254 | ```
|
255 | [zoom]="0.5"
|
256 | ```
|
257 |
|
258 | #### [zoom-scale]
|
259 |
|
260 | | Property | Type | Required |
|
261 | | --- | ---- | --- |
|
262 | | [zoom-scale] | *'page-width'\|'page-fit'\|'page-height'* | *Optional* |
|
263 |
|
264 | Defines how the Zoom scale is computed when `[original-size]="false"`, by default set to 'page-width'.
|
265 |
|
266 | - *'page-width'* with zoom of 1 will display a page width that take all the possible horizontal space in the container
|
267 |
|
268 | - *'page-height'* with zoom of 1 will display a page height that take all the possible vertical space in the container
|
269 |
|
270 | - *'page-fit'* with zoom of 1 will display a page that will be scaled to either width or height to fit completely in the container
|
271 |
|
272 | ```
|
273 | [zoom-scale]="'page-width'"
|
274 | ```
|
275 |
|
276 | #### [original-size]
|
277 |
|
278 | | Property | Type | Required |
|
279 | | --- | ---- | --- |
|
280 | | [original-size] | *boolean* | *Optional* |
|
281 |
|
282 | * if set to *true* - size will be as same as original document
|
283 | * if set to *false* - size will be as same as container block
|
284 |
|
285 | ```
|
286 | [original-size]="true"
|
287 | ```
|
288 |
|
289 | #### [fit-to-page]
|
290 |
|
291 | | Property | Type | Required |
|
292 | | --- | ---- | --- |
|
293 | | [fit-to-page] | *boolean* | *Optional* |
|
294 |
|
295 | Works in combination with `[original-size]="true"`. You can show your document in original size, and make sure that it's not bigger then container block.
|
296 |
|
297 | ```
|
298 | [fit-to-page]="false"
|
299 | ```
|
300 |
|
301 | #### [show-all]
|
302 |
|
303 | | Property | Type | Required |
|
304 | | --- | ---- | --- |
|
305 | | [show-all] | *boolean* | *Optional* |
|
306 |
|
307 | Show single or all pages altogether
|
308 |
|
309 | ```
|
310 | [show-all]="true"
|
311 | ```
|
312 |
|
313 | #### [autoresize]
|
314 |
|
315 | | Property | Type | Required |
|
316 | | --- | ---- | --- |
|
317 | | [autoresize] | *boolean* | *Optional* |
|
318 |
|
319 | Turn on or off auto resize.
|
320 |
|
321 | **!Important** To make `[autoresize]` work - make sure that `[original-size]="false"` and `pdf-viewer` tag has `max-width` or `display` are set.
|
322 |
|
323 | ```
|
324 | [autoresize]="true"
|
325 | ```
|
326 |
|
327 | #### [c-maps-url]
|
328 |
|
329 | | Property | Type | Required |
|
330 | | --- | ---- | --- |
|
331 | | [c-maps-url] | *string* | Optional |
|
332 |
|
333 | Url for non-latin characters source maps.
|
334 | ```
|
335 | [c-maps-url]="'assets/cmaps/'"
|
336 | ```
|
337 |
|
338 | Default url is: [https://unpkg.com/pdfjs-dist@2.0.550/cmaps/](https://unpkg.com/pdfjs-dist@2.0.550/cmaps/)
|
339 |
|
340 | To serve cmaps on your own you need to copy ```node_modules/pdfjs-dist/cmaps``` to ```assets/cmaps```.
|
341 |
|
342 | ### [show-borders]
|
343 |
|
344 | | Property | Type | Required |
|
345 | | --- | ---- | --- |
|
346 | | [show-borders] | *boolean* | Optional |
|
347 |
|
348 | Show page borders
|
349 | ```
|
350 | [show-borders]="true"
|
351 | ```
|
352 |
|
353 | #### (after-load-complete)
|
354 |
|
355 | | Property | Type | Required |
|
356 | | --- | ---- | --- |
|
357 | | (after-load-complete) | *callback* | *Optional* |
|
358 |
|
359 | Get PDF information with callback
|
360 |
|
361 | First define callback function "callBackFn" in your controller,
|
362 | ```typescript
|
363 | callBackFn(pdf: PDFDocumentProxy) {
|
364 | // do anything with "pdf"
|
365 | }
|
366 | ```
|
367 |
|
368 | And then use it in your template:
|
369 | ```
|
370 | (after-load-complete)="callBackFn($event)"
|
371 | ```
|
372 |
|
373 | #### (page-rendered)
|
374 |
|
375 | | Property | Type | Required |
|
376 | | --- | ---- | --- |
|
377 | | (page-rendered) | *callback* | *Optional* |
|
378 |
|
379 | Get event when a page is rendered. Called for every page rendered.
|
380 |
|
381 | Define callback in your component:
|
382 |
|
383 | ```typescript
|
384 | pageRendered(e: CustomEvent) {
|
385 | console.log('(page-rendered)', e);
|
386 | }
|
387 | ```
|
388 |
|
389 | And then bind it to `<pdf-viewer>`:
|
390 |
|
391 | ```angular2html
|
392 | (page-rendered)="pageRendered($event)"
|
393 | ```
|
394 |
|
395 | #### (pages-initialized)
|
396 |
|
397 | | Property | Type | Required |
|
398 | | --- | ---- | --- |
|
399 | | (pages-initialized) | *callback* | *Optional* |
|
400 |
|
401 | Get event when the pages are initialized.
|
402 |
|
403 | Define callback in your component:
|
404 |
|
405 | ```typescript
|
406 | pageInitialized(e: CustomEvent) {
|
407 | console.log('(pages-initialized)', e);
|
408 | }
|
409 | ```
|
410 |
|
411 | And then bind it to `<pdf-viewer>`:
|
412 |
|
413 | ```angular2html
|
414 | (pages-initialized)="pageInitialized($event)"
|
415 | ```
|
416 |
|
417 | #### (text-layer-rendered)
|
418 |
|
419 | | Property | Type | Required |
|
420 | | --- | ---- | --- |
|
421 | | (text-layer-rendered) | *callback* | *Optional* |
|
422 |
|
423 | Get event when a text layer is rendered.
|
424 |
|
425 | Define callback in your component:
|
426 |
|
427 | ```typescript
|
428 | textLayerRendered(e: CustomEvent) {
|
429 | console.log('(text-layer-rendered)', e);
|
430 | }
|
431 | ```
|
432 |
|
433 | And then bind it to `<pdf-viewer>`:
|
434 |
|
435 | ```angular2html
|
436 | (text-layer-rendered)="textLayerRendered($event)"
|
437 | ```
|
438 |
|
439 | #### (error)
|
440 |
|
441 | | Property | Type | Required |
|
442 | | --- | ---- | --- |
|
443 | | (error) | *callback* | *Optional* |
|
444 |
|
445 | Error handling callback
|
446 |
|
447 | Define callback in your component's class
|
448 |
|
449 | ```typescript
|
450 | onError(error: any) {
|
451 | // do anything
|
452 | }
|
453 | ```
|
454 |
|
455 | Then add it to `pdf-component` in component's template
|
456 |
|
457 | ```html
|
458 | (error)="onError($event)"
|
459 | ```
|
460 |
|
461 | #### (on-progress)
|
462 |
|
463 | | Property | Type | Required |
|
464 | | --- | ---- | --- |
|
465 | | (on-progress) | *callback* | *Optional* |
|
466 |
|
467 | Loading progress callback - provides progress information `total` and `loaded` bytes. Is called several times during pdf loading phase.
|
468 |
|
469 | Define callback in your component's class
|
470 |
|
471 | ```typescript
|
472 | onProgress(progressData: PDFProgressData) {
|
473 | // do anything with progress data. For example progress indicator
|
474 | }
|
475 | ```
|
476 |
|
477 | Then add it to `pdf-component` in component's template
|
478 |
|
479 | ```html
|
480 | (on-progress)="onProgress($event)"
|
481 | ```
|
482 |
|
483 | ## Render local PDF file
|
484 |
|
485 | In your `html` template add `input`:
|
486 |
|
487 | ```html
|
488 | <input (change)="onFileSelected()" type="file" id="file">
|
489 | ```
|
490 |
|
491 | and then add `onFileSelected` method to your component:
|
492 |
|
493 | ```typescript
|
494 | onFileSelected() {
|
495 | let $img: any = document.querySelector('#file');
|
496 |
|
497 | if (typeof (FileReader) !== 'undefined') {
|
498 | let reader = new FileReader();
|
499 |
|
500 | reader.onload = (e: any) => {
|
501 | this.pdfSrc = e.target.result;
|
502 | };
|
503 |
|
504 | reader.readAsArrayBuffer($img.files[0]);
|
505 | }
|
506 | }
|
507 | ```
|
508 |
|
509 |
|
510 | ## Set custom path to the worker
|
511 |
|
512 | By default the `worker` is loaded from `cdnjs.cloudflare.com`.
|
513 |
|
514 | In your code update `path` to the worker to be for example `/pdf.worker.js`
|
515 | ```typescript
|
516 | (window as any).pdfWorkerSrc = '/pdf.worker.js';
|
517 | ```
|
518 | *This should be set before `pdf-viewer` component is rendered.*
|
519 |
|
520 |
|
521 | ## Search in the PDF
|
522 |
|
523 | Use `eventBus` for the search functionality.
|
524 |
|
525 | In your component's ts file:
|
526 |
|
527 | * Add reference to `pdf-viewer` component,
|
528 | * then when needed execute `search()` linke this:
|
529 |
|
530 | ```typescript
|
531 | @ViewChild(PdfViewerComponent) private pdfComponent: PdfViewerComponent;
|
532 |
|
533 | search(stringToSearch: string) {
|
534 | this.pdfComponent.eventBus.dispatch('find', {
|
535 | query: stringToSearch, type: 'again', caseSensitive: false, findPrevious: undefined, highlightAll: true, phraseSearch: true
|
536 | });
|
537 | }
|
538 | ```
|
539 |
|
540 | ## Contribute
|
541 | [See CONTRIBUTING.md](CONTRIBUTING.md)
|
542 |
|
543 | ## Donation
|
544 | If this project help you reduce time to develop, you can give me a cup of tea :)
|
545 |
|
546 | [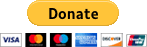](https://www.paypal.me/vadimdez)
|
547 |
|
548 | ## License
|
549 |
|
550 | [MIT](https://tldrlegal.com/license/mit-license) © [Vadym Yatsyuk](https://github.com/vadimdez)
|
551 |
|