1 | # node-notifier [![NPM version][npm-image]][npm-url] [![Build Status][travis-image]][travis-url] [![Dependency Status][depstat-image]][depstat-url]
|
2 |
|
3 | A Node.js module for sending cross platform system notifications. Using
|
4 | Notification Center for Mac, notify-osd/libnotify-bin for Linux, Toasters for
|
5 | Windows 8/10, or taskbar Balloons for earlier Windows versions. If none of
|
6 | these requirements are met, Growl is used.
|
7 |
|
8 | 
|
9 | 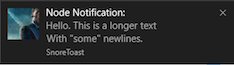
|
10 | 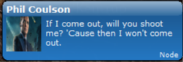
|
11 |
|
12 | ## Quick Usage
|
13 |
|
14 | Show a native notification on Mac, Windows, Linux:
|
15 |
|
16 | ```javascript
|
17 | const notifier = require('node-notifier');
|
18 | // String
|
19 | notifier.notify('Message');
|
20 |
|
21 | // Object
|
22 | notifier.notify({
|
23 | 'title': 'My notification',
|
24 | 'message': 'Hello, there!'
|
25 | });
|
26 | ```
|
27 |
|
28 | ## Requirements
|
29 | - **Mac OS X**: >= 10.8 or Growl if earlier.
|
30 | - **Linux**: `notify-osd` or `libnotify-bin` installed (Ubuntu should have this by default)
|
31 | - **Windows**: >= 8, task bar balloon if earlier or Growl if that is installed.
|
32 | - **General Fallback**: Growl
|
33 |
|
34 | Growl takes precedence over Windows balloons.
|
35 |
|
36 | See [documentation and flow chart for reporter choice](./DECISION_FLOW.md)
|
37 |
|
38 | ## Install
|
39 | ```
|
40 | $ npm install --save node-notifier
|
41 | ```
|
42 |
|
43 | ## Cross-Platform Advanced Usage
|
44 |
|
45 | Standard usage, with cross-platform fallbacks as defined in the
|
46 | [reporter flow chart](./DECISION_FLOW.md). All of the options
|
47 | below will work in a way or another on all platforms.
|
48 |
|
49 | ```javascript
|
50 | const notifier = require('node-notifier');
|
51 | const path = require('path');
|
52 |
|
53 | notifier.notify({
|
54 | title: 'My awesome title',
|
55 | message: 'Hello from node, Mr. User!',
|
56 | icon: path.join(__dirname, 'coulson.jpg'), // Absolute path (doesn't work on balloons)
|
57 | sound: true, // Only Notification Center or Windows Toasters
|
58 | wait: true // Wait with callback, until user action is taken against notification
|
59 | }, function (err, response) {
|
60 | // Response is response from notification
|
61 | });
|
62 |
|
63 | notifier.on('click', function (notifierObject, options) {
|
64 | // Triggers if `wait: true` and user clicks notification
|
65 | });
|
66 |
|
67 | notifier.on('timeout', function (notifierObject, options) {
|
68 | // Triggers if `wait: true` and notification closes
|
69 | });
|
70 | ```
|
71 |
|
72 | You can also specify what reporter you want to use if you
|
73 | want to customize it or have more specific options per system.
|
74 | See documentation for each reporter below.
|
75 |
|
76 | Example:
|
77 | ```javascript
|
78 | const NotificationCenter = require('node-notifier/notifiers/notificationcenter');
|
79 | new NotificationCenter(options).notify();
|
80 |
|
81 | const NotifySend = require('node-notifier/notifiers/notifysend');
|
82 | new NotifySend(options).notify();
|
83 |
|
84 | const WindowsToaster = require('node-notifier/notifiers/toaster');
|
85 | new WindowsToaster(options).notify();
|
86 |
|
87 | const Growl = require('node-notifier/notifiers/growl');
|
88 | new Growl(options).notify();
|
89 |
|
90 | const WindowsBalloon = require('node-notifier/notifiers/balloon');
|
91 | new WindowsBalloon(options).notify();
|
92 |
|
93 | ```
|
94 |
|
95 | Or if you are using several (or you are lazy):
|
96 | (note: technically, this takes longer to require)
|
97 |
|
98 | ```javascript
|
99 | const nn = require('node-notifier');
|
100 |
|
101 | new nn.NotificationCenter(options).notify();
|
102 | new nn.NotifySend(options).notify();
|
103 | new nn.WindowsToaster(options).notify(options);
|
104 | new nn.WindowsBalloon(options).notify(options);
|
105 | new nn.Growl(options).notify(options);
|
106 | ```
|
107 |
|
108 | ## Contents
|
109 |
|
110 | * [Notification Center documentation](#usage-notificationcenter)
|
111 | * [Windows Toaster documentation](#usage-windowstoaster)
|
112 | * [Windows Balloon documentation](#usage-windowsballoon)
|
113 | * [Growl documentation](#usage-growl)
|
114 | * [Notify-send documentation](#usage-notifysend)
|
115 |
|
116 |
|
117 | ### Usage NotificationCenter
|
118 |
|
119 | Same usage and parameter setup as [terminal-notifier](https://github.com/alloy/terminal-notifier).
|
120 |
|
121 | Native Notification Center requires Mac OS X version 10.8 or higher. If you have
|
122 | an earlier version, Growl will be the fallback. If Growl isn't installed, an
|
123 | error will be returned in the callback.
|
124 |
|
125 |
|
126 | #### Example
|
127 |
|
128 | Wrapping around [terminal-notifier](https://github.com/alloy/terminal-notifier), you can
|
129 | do all terminal-notifier can do through properties to the `notify` method. E.g.
|
130 | if `terminal-notifier` says `-message`, you can do `{message: 'Foo'}`, or
|
131 | if `terminal-notifier` says `-list ALL`, you can do `{list: 'ALL'}`. Notification
|
132 | is the primary focus for this module, so listing and activating do work,
|
133 | but isn't documented.
|
134 |
|
135 | ### All notification options with their defaults:
|
136 |
|
137 | ```javascript
|
138 | const NotificationCenter = require('node-notifier').NotificationCenter;
|
139 |
|
140 | var notifier = new NotificationCenter({
|
141 | withFallback: false, // Use Growl Fallback if <= 10.8
|
142 | customPath: void 0 // Relative path if you want to use your fork of terminal-notifier
|
143 | });
|
144 |
|
145 | notifier.notify({
|
146 | 'title': void 0,
|
147 | 'subtitle': void 0,
|
148 | 'message': void 0,
|
149 | 'sound': false, // Case Sensitive string for location of sound file, or use one of OS X's native sounds (see below)
|
150 | 'icon': 'Terminal Icon', // Absolute Path to Triggering Icon
|
151 | 'contentImage': void 0, // Absolute Path to Attached Image (Content Image)
|
152 | 'open': void 0, // URL to open on Click
|
153 | 'wait': false // Wait for User Action against Notification
|
154 | }, function(error, response) {
|
155 | console.log(response);
|
156 | });
|
157 | ```
|
158 |
|
159 | **For Mac OS notifications, icon and contentImage requires OS X 10.9.**
|
160 |
|
161 | Sound can be one of these: `Basso`, `Blow`, `Bottle`, `Frog`, `Funk`, `Glass`,
|
162 | `Hero`, `Morse`, `Ping`, `Pop`, `Purr`, `Sosumi`, `Submarine`, `Tink`.
|
163 | If sound is simply `true`, `Bottle` is used.
|
164 |
|
165 | See [specific Notification Center example](./example/advanced.js).
|
166 |
|
167 | ### Usage WindowsToaster
|
168 |
|
169 | **Note:** There are some limitations for images in native Windows 8 notifications:
|
170 | The image must be a PNG image, and cannot be over 1024x1024 px, or over over 200Kb.
|
171 | You also need to specify the image by using an absolute path. These limitations are
|
172 | due to the Toast notification system. A good tip is to use something like
|
173 | `path.join` or `path.delimiter` to have cross-platform pathing.
|
174 |
|
175 | **Windows 10 Note:** You might have to activate banner notification for the toast to show.
|
176 |
|
177 | From [mikaelbr/gulp-notify#90 (comment)](https://github.com/mikaelbr/gulp-notify/issues/90#issuecomment-129333034)
|
178 | > You can make it work by going to System > Notifications & Actions. The 'toast' app needs to have Banners enabled. (You can activate banners by clicking on the 'toast' app and setting the 'Show notification banners' to On)
|
179 |
|
180 | [toaster](https://github.com/nels-o/toaster) is used to get native Windows Toasts!
|
181 |
|
182 | ```javascript
|
183 | const WindowsToaster = require('node-notifier').WindowsToaster;
|
184 |
|
185 | var notifier = new WindowsToaster({
|
186 | withFallback: false, // Fallback to Growl or Balloons?
|
187 | customPath: void 0 // Relative path if you want to use your fork of toast.exe
|
188 | });
|
189 |
|
190 | notifier.notify({
|
191 | title: void 0,
|
192 | message: void 0,
|
193 | icon: void 0, // Absolute path to Icon
|
194 | sound: false, // true | false.
|
195 | wait: false, // Wait for User Action against Notification
|
196 | }, function(error, response) {
|
197 | console.log(response);
|
198 | });
|
199 | ```
|
200 |
|
201 | ### Usage Growl
|
202 |
|
203 | ```javascript
|
204 | const Growl = require('node-notifier').Growl;
|
205 |
|
206 | var notifier = new Growl({
|
207 | name: 'Growl Name Used', // Defaults as 'Node'
|
208 | host: 'localhost',
|
209 | port: 23053
|
210 | });
|
211 |
|
212 | notifier.notify({
|
213 | title: 'Foo',
|
214 | message: 'Hello World',
|
215 | icon: fs.readFileSync(__dirname + "/coulson.jpg"),
|
216 | wait: false, // Wait for User Action against Notification
|
217 |
|
218 | // and other growl options like sticky etc.
|
219 | sticky: false,
|
220 | label: void 0,
|
221 | priority: void 0
|
222 | });
|
223 | ```
|
224 |
|
225 | See more information about using
|
226 | [growly](https://github.com/theabraham/growly/).
|
227 |
|
228 | ### Usage WindowsBalloon
|
229 |
|
230 | For earlier Windows versions, the taskbar balloons are used (unless
|
231 | fallback is activated and Growl is running). For balloons, a great
|
232 | project called [notifu](http://www.paralint.com/projects/notifu/) is used.
|
233 |
|
234 | ```javascript
|
235 | const WindowsBalloon = require('node-notifier').WindowsBalloon;
|
236 |
|
237 | var notifier = new WindowsBalloon({
|
238 | withFallback: false, // Try Windows Toast and Growl first?
|
239 | customPath: void 0 // Relative path if you want to use your fork of notifu
|
240 | });
|
241 |
|
242 | notifier.notify({
|
243 | title: void 0,
|
244 | message: void 0,
|
245 | sound: false, // true | false.
|
246 | time: 5000, // How long to show balloon in ms
|
247 | wait: false, // Wait for User Action against Notification
|
248 | }, function(error, response) {
|
249 | console.log(response);
|
250 | });
|
251 | ```
|
252 |
|
253 | See full usage on the [project homepage:
|
254 | notifu](http://www.paralint.com/projects/notifu/).
|
255 |
|
256 | ### Usage NotifySend
|
257 |
|
258 | Note: notify-send doesn't support the wait flag.
|
259 |
|
260 | ```javascript
|
261 | const NotifySend = require('node-notifier').NotifySend;
|
262 |
|
263 | var notifier = new NotifySend();
|
264 |
|
265 | notifier.notify({
|
266 | title: 'Foo',
|
267 | message: 'Hello World',
|
268 | icon: __dirname + "/coulson.jpg",
|
269 |
|
270 | // .. and other notify-send flags:
|
271 | urgency: void 0,
|
272 | time: void 0,
|
273 | category: void 0,
|
274 | hint: void 0,
|
275 | });
|
276 | ```
|
277 |
|
278 | See flags and options [on the man pages](http://manpages.ubuntu.com/manpages/gutsy/man1/notify-send.1.html)
|
279 |
|
280 | ## CLI
|
281 |
|
282 | You can also use node-notifier as a CLI (as of `v4.2.0`).
|
283 |
|
284 | ```shell
|
285 | $ notify -h
|
286 |
|
287 | # notify
|
288 | ## Options
|
289 | * --help (alias -h)
|
290 | * --title (alias -t)
|
291 | * --subtitle (alias -st)
|
292 | * --message (alias -m)
|
293 | * --icon (alias -i)
|
294 | * --sound (alias -s)
|
295 | * --open (alias -o)
|
296 |
|
297 | ## Example
|
298 |
|
299 | $ notify -t "Hello" -m "My Message" -s --open http://github.com
|
300 | $ notify -t "Agent Coulson" --icon https://raw.githubusercontent.com/mikaelbr/node-notifier/master/example/coulson.jpg -m "Well, that's new. "
|
301 | $ notify -m "My Message" -s Glass
|
302 | $ echo "My Message" | notify -t "Hello"
|
303 | ```
|
304 |
|
305 | You can also pass message in as `stdin`:
|
306 |
|
307 | ```js
|
308 | ➜ echo "Message" | notify
|
309 |
|
310 | # Works with existing arguments
|
311 | ➜ echo "Message" | notify -t "My Title"
|
312 | ➜ echo "Some message" | notify -t "My Title" -s
|
313 | ```
|
314 |
|
315 | ## Thanks to OSS
|
316 |
|
317 | `node-notifier` is made possible through Open Source Software. A very special thanks to all the modules `node-notifier` uses.
|
318 | * [terminal-notifier](https://github.com/alloy/terminal-notifier)
|
319 | * [toaster](https://github.com/nels-o/toaster)
|
320 | * [notifu](http://www.paralint.com/projects/notifu/)
|
321 | * [growly](https://github.com/theabraham/growly/)
|
322 |
|
323 | [![NPM downloads][npm-downloads]][npm-url]
|
324 |
|
325 | ## Common Issues
|
326 |
|
327 | ### Use inside tmux session
|
328 |
|
329 | When using node-notifier within a tmux session, it can cause a hang in the system. This can be solved by following the steps described in this comment: https://github.com/julienXX/terminal-notifier/issues/115#issuecomment-104214742
|
330 |
|
331 | See more info here: https://github.com/mikaelbr/node-notifier/issues/61#issuecomment-163560801
|
332 |
|
333 |
|
334 | ### Within Electron Packaging
|
335 |
|
336 | If packaging your Electron app as an `asar`, you will find node-notifier will fail to load. Due to the way asar works, you cannot execute a binary from within an asar. As a simple solution, when packaging the app into an asar please make sure you `--unpack` the vendor folder of node-notifier, so the module still has access to the notification binaries. To do this, you can do so by using the following command:
|
337 |
|
338 | ```bash
|
339 | asar pack . app.asar --unpack "./node_modules/node-notifier/vendor/**"
|
340 | ```
|
341 |
|
342 |
|
343 | ## License
|
344 |
|
345 | [MIT License](http://en.wikipedia.org/wiki/MIT_License)
|
346 |
|
347 | [npm-url]: https://npmjs.org/package/node-notifier
|
348 | [npm-image]: http://img.shields.io/npm/v/node-notifier.svg?style=flat
|
349 | [npm-downloads]: http://img.shields.io/npm/dm/node-notifier.svg?style=flat
|
350 |
|
351 | [travis-url]: http://travis-ci.org/mikaelbr/node-notifier
|
352 | [travis-image]: http://img.shields.io/travis/mikaelbr/node-notifier.svg?style=flat
|
353 |
|
354 | [depstat-url]: https://gemnasium.com/mikaelbr/node-notifier
|
355 | [depstat-image]: http://img.shields.io/gemnasium/mikaelbr/node-notifier.svg?style=flat
|