1 | # object-hash
|
2 |
|
3 | Generate hashes from objects and values in node and the browser. Uses node.js
|
4 | crypto module for hashing. Supports SHA1, CRC32 and many others (depending on the platform)
|
5 | as well as custom streams.
|
6 |
|
7 | [](https://www.npmjs.com/package/object-hash)
|
8 | [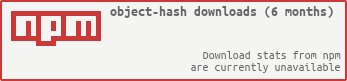](https://www.npmjs.com/package/object-hash)
|
9 |
|
10 | [](https://secure.travis-ci.org/puleos/object-hash?branch=master)
|
11 | [](https://coveralls.io/github/puleos/object-hash?branch=master)
|
12 |
|
13 | * Hash values of any type.
|
14 | * Supports a keys only option for grouping similar objects with different values.
|
15 |
|
16 | ```js
|
17 | var hash = require('object-hash');
|
18 | ```
|
19 |
|
20 | ## hash(value, options);
|
21 | Generate a hash from any object or type. Defaults to sha1 with hex encoding.
|
22 | * `algorithm` hash algo to be used: 'sha1', 'md5'. default: sha1
|
23 | * `excludeValues` {true|false} hash object keys, values ignored. default: false
|
24 | * `encoding` hash encoding, supports 'buffer', 'hex', 'binary', 'base64'. default: hex
|
25 | * `ignoreUnknown` {true|*false} ignore unknown object types. default: false
|
26 | * `replacer` optional function that replaces values before hashing. default: accept all values
|
27 | * `respectFunctionProperties` {true|false} Whether properties on functions are considered when hashing. default: true
|
28 | * `respectType` {true|false} Whether special type attributes (`.prototype`, `.__proto__`, `.constructor`)
|
29 | are hashed. default: true
|
30 | * `unorderedArrays` {true|false} Sort all arrays using before hashing. Note that this affects *all* collections,
|
31 | i.e. including typed arrays, Sets, Maps, etc. default: false
|
32 | * `unorderedSets` {true|false} Sort `Set` and `Map` instances before hashing, i.e. make
|
33 | `hash(new Set([1, 2])) == hash(new Set([2, 1]))` return `true`. default: true
|
34 |
|
35 | ## hash.sha1(value);
|
36 | Hash using the sha1 algorithm.
|
37 |
|
38 | *Sugar method, equivalent to hash(value, {algorithm: 'sha1'})*
|
39 |
|
40 | ## hash.keys(value);
|
41 | Hash object keys using the sha1 algorithm, values ignored.
|
42 |
|
43 | *Sugar method, equivalent to hash(value, {excludeValues: true})*
|
44 |
|
45 | ## hash.MD5(value);
|
46 | Hash using the md5 algorithm.
|
47 |
|
48 | *Sugar method, equivalent to hash(value, {algorithm: 'md5'})*
|
49 |
|
50 | ## hash.keysMD5(value);
|
51 | Hash object keys using the md5 algorithm, values ignored.
|
52 |
|
53 | *Sugar method, equivalent to hash(value, {algorithm: 'md5', excludeValues: true})*
|
54 |
|
55 | ## hash.writeToStream(value, [options,] stream):
|
56 | Write the information that would otherwise have been hashed to a stream, e.g.:
|
57 | ```js
|
58 | hash.writeToStream({foo: 'bar', a: 42}, {respectType: false}, process.stdout)
|
59 | // => e.g. 'object:a:number:42foo:string:bar'
|
60 | ```
|
61 |
|
62 | ## Installation
|
63 |
|
64 | node:
|
65 | ```js
|
66 | npm install object-hash
|
67 | ```
|
68 |
|
69 | browser: */dist/object_hash.js*
|
70 | ```
|
71 | <script src="object_hash.js" type="text/javascript"></script>
|
72 |
|
73 | <script>
|
74 | var hash = objectHash.sha({foo:'bar'});
|
75 |
|
76 | console.log(hash); // e003c89cdf35cdf46d8239b4692436364b7259f9
|
77 | </script>
|
78 | ```
|
79 |
|
80 | ## Example usage
|
81 | ```js
|
82 | var hash = require('object-hash');
|
83 |
|
84 | var peter = {name: 'Peter', stapler: false, friends: ['Joanna', 'Michael', 'Samir'] };
|
85 | var michael = {name: 'Michael', stapler: false, friends: ['Peter', 'Samir'] };
|
86 | var bob = {name: 'Bob', stapler: true, friends: [] };
|
87 |
|
88 | /***
|
89 | * sha1 hex encoding (default)
|
90 | */
|
91 | hash(peter);
|
92 | // 14fa461bf4b98155e82adc86532938553b4d33a9
|
93 | hash(michael);
|
94 | // 4b2b30e27699979ce46714253bc2213010db039c
|
95 | hash(bob);
|
96 | // 38d96106bc8ef3d8bd369b99bb6972702c9826d5
|
97 |
|
98 | /***
|
99 | * hash object keys, values ignored
|
100 | */
|
101 | hash(peter, { excludeValues: true });
|
102 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
103 | hash(michael, { excludeValues: true });
|
104 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
105 | hash.keys(bob);
|
106 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
107 |
|
108 | /***
|
109 | * md5 base64 encoding
|
110 | */
|
111 | hash(peter, { algorithm: 'md5', encoding: 'base64' });
|
112 | // 6rkWaaDiG3NynWw4svGH7g==
|
113 | hash(michael, { algorithm: 'md5', encoding: 'base64' });
|
114 | // djXaWpuWVJeOF8Sb6SFFNg==
|
115 | hash(bob, { algorithm: 'md5', encoding: 'base64' });
|
116 | // lFzkw/IJ8/12jZI0rQeS3w==
|
117 | ```
|
118 |
|
119 | ## Legacy Browser Support
|
120 | IE <= 8 and Opera <= 11 support dropped in version 0.3.0. If you require
|
121 | legacy browser support you must either use an ES5 shim or use version 0.2.5
|
122 | of this module.
|
123 |
|
124 | ## Development
|
125 |
|
126 | ```
|
127 | git clone https://github.com/puleos/object-hash
|
128 | ```
|
129 |
|
130 | ### gulp tasks
|
131 | * `gulp watch` (default) watch files, test and lint on change/add
|
132 | * `gulp test` unit tests
|
133 | * `gulp karma` browser unit tests
|
134 | * `gulp lint` jshint
|
135 | * `gulp dist` create browser version in /dist
|
136 |
|
137 | ## License
|
138 | MIT
|
139 |
|
\ | No newline at end of file |