1 | # object-hash
|
2 |
|
3 | Generate hashes from objects and values in node and the browser. Uses node.js
|
4 | crypto module for hashing. Supports SHA1 and many others (depending on the platform)
|
5 | as well as custom streams (e.g. CRC32).
|
6 |
|
7 | [](https://www.npmjs.com/package/object-hash)
|
8 | [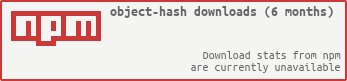](https://www.npmjs.com/package/object-hash)
|
9 |
|
10 | [](https://secure.travis-ci.org/puleos/object-hash?branch=master)
|
11 | [](https://coveralls.io/github/puleos/object-hash?branch=master)
|
12 |
|
13 | * Hash values of any type.
|
14 | * Supports a keys only option for grouping similar objects with different values.
|
15 |
|
16 | ```js
|
17 | var hash = require('object-hash');
|
18 |
|
19 | hash({foo: 'bar'}) // => '67b69634f9880a282c14a0f0cb7ba20cf5d677e9'
|
20 | hash([1, 2, 2.718, 3.14159]) // => '136b9b88375971dff9f1af09d7356e3e04281951'
|
21 | ```
|
22 |
|
23 | ## Versioning Disclaimer
|
24 |
|
25 | **IMPORTANT:** If you need lasting hash consistency, you should should lock `object-hash` at a specific version, because new versions (even patch versions) are likely to affect the result. For more info, see [this discussion](https://github.com/puleos/object-hash/issues/30).
|
26 |
|
27 | ## hash(value, options);
|
28 | Generate a hash from any object or type. Defaults to sha1 with hex encoding.
|
29 | * `algorithm` hash algo to be used: 'sha1', 'md5'. default: sha1
|
30 | * `excludeValues` {true|false} hash object keys, values ignored. default: false
|
31 | * `encoding` hash encoding, supports 'buffer', 'hex', 'binary', 'base64'. default: hex
|
32 | * `ignoreUnknown` {true|*false} ignore unknown object types. default: false
|
33 | * `replacer` optional function that replaces values before hashing. default: accept all values
|
34 | * `respectFunctionProperties` {true|false} Whether properties on functions are considered when hashing. default: true
|
35 | * `respectFunctionNames` {true|false} consider `name` property of functions for hashing. default: true
|
36 | * `respectType` {true|false} Whether special type attributes (`.prototype`, `.__proto__`, `.constructor`)
|
37 | are hashed. default: true
|
38 | * `unorderedArrays` {true|false} Sort all arrays using before hashing. Note that this affects *all* collections,
|
39 | i.e. including typed arrays, Sets, Maps, etc. default: false
|
40 | * `unorderedSets` {true|false} Sort `Set` and `Map` instances before hashing, i.e. make
|
41 | `hash(new Set([1, 2])) == hash(new Set([2, 1]))` return `true`. default: true
|
42 |
|
43 | ## hash.sha1(value);
|
44 | Hash using the sha1 algorithm.
|
45 |
|
46 | *Sugar method, equivalent to hash(value, {algorithm: 'sha1'})*
|
47 |
|
48 | ## hash.keys(value);
|
49 | Hash object keys using the sha1 algorithm, values ignored.
|
50 |
|
51 | *Sugar method, equivalent to hash(value, {excludeValues: true})*
|
52 |
|
53 | ## hash.MD5(value);
|
54 | Hash using the md5 algorithm.
|
55 |
|
56 | *Sugar method, equivalent to hash(value, {algorithm: 'md5'})*
|
57 |
|
58 | ## hash.keysMD5(value);
|
59 | Hash object keys using the md5 algorithm, values ignored.
|
60 |
|
61 | *Sugar method, equivalent to hash(value, {algorithm: 'md5', excludeValues: true})*
|
62 |
|
63 | ## hash.writeToStream(value, [options,] stream):
|
64 | Write the information that would otherwise have been hashed to a stream, e.g.:
|
65 | ```js
|
66 | hash.writeToStream({foo: 'bar', a: 42}, {respectType: false}, process.stdout)
|
67 | // => e.g. 'object:a:number:42foo:string:bar'
|
68 | ```
|
69 |
|
70 | ## Installation
|
71 |
|
72 | node:
|
73 | ```js
|
74 | npm install object-hash
|
75 | ```
|
76 |
|
77 | browser: */dist/object_hash.js*
|
78 | ```
|
79 | <script src="object_hash.js" type="text/javascript"></script>
|
80 |
|
81 | <script>
|
82 | var hash = objectHash.sha1({foo:'bar'});
|
83 |
|
84 | console.log(hash); // e003c89cdf35cdf46d8239b4692436364b7259f9
|
85 | </script>
|
86 | ```
|
87 |
|
88 | ## Example usage
|
89 | ```js
|
90 | var hash = require('object-hash');
|
91 |
|
92 | var peter = {name: 'Peter', stapler: false, friends: ['Joanna', 'Michael', 'Samir'] };
|
93 | var michael = {name: 'Michael', stapler: false, friends: ['Peter', 'Samir'] };
|
94 | var bob = {name: 'Bob', stapler: true, friends: [] };
|
95 |
|
96 | /***
|
97 | * sha1 hex encoding (default)
|
98 | */
|
99 | hash(peter);
|
100 | // 14fa461bf4b98155e82adc86532938553b4d33a9
|
101 | hash(michael);
|
102 | // 4b2b30e27699979ce46714253bc2213010db039c
|
103 | hash(bob);
|
104 | // 38d96106bc8ef3d8bd369b99bb6972702c9826d5
|
105 |
|
106 | /***
|
107 | * hash object keys, values ignored
|
108 | */
|
109 | hash(peter, { excludeValues: true });
|
110 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
111 | hash(michael, { excludeValues: true });
|
112 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
113 | hash.keys(bob);
|
114 | // 48f370a772c7496f6c9d2e6d92e920c87dd00a5c
|
115 |
|
116 | /***
|
117 | * md5 base64 encoding
|
118 | */
|
119 | hash(peter, { algorithm: 'md5', encoding: 'base64' });
|
120 | // 6rkWaaDiG3NynWw4svGH7g==
|
121 | hash(michael, { algorithm: 'md5', encoding: 'base64' });
|
122 | // djXaWpuWVJeOF8Sb6SFFNg==
|
123 | hash(bob, { algorithm: 'md5', encoding: 'base64' });
|
124 | // lFzkw/IJ8/12jZI0rQeS3w==
|
125 | ```
|
126 |
|
127 | ## Legacy Browser Support
|
128 | IE <= 8 and Opera <= 11 support dropped in version 0.3.0. If you require
|
129 | legacy browser support you must either use an ES5 shim or use version 0.2.5
|
130 | of this module.
|
131 |
|
132 | ## Development
|
133 |
|
134 | ```
|
135 | git clone https://github.com/puleos/object-hash
|
136 | ```
|
137 |
|
138 | ### gulp tasks
|
139 | * `gulp watch` (default) watch files, test and lint on change/add
|
140 | * `gulp test` unit tests
|
141 | * `gulp karma` browser unit tests
|
142 | * `gulp lint` jshint
|
143 | * `gulp dist` create browser version in /dist
|
144 |
|
145 | ## License
|
146 | MIT
|
147 |
|
\ | No newline at end of file |