1 | [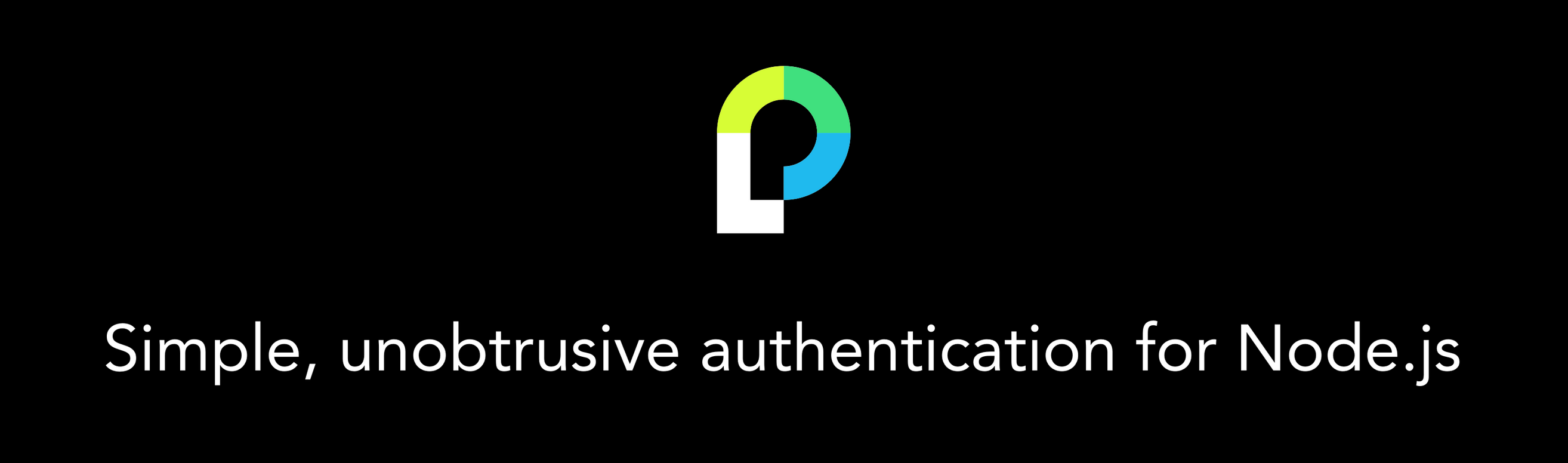](http://passportjs.org)
|
2 |
|
3 | # Passport
|
4 |
|
5 | Passport is [Express](http://expressjs.com/)-compatible authentication
|
6 | middleware for [Node.js](http://nodejs.org/).
|
7 |
|
8 | Passport's sole purpose is to authenticate requests, which it does through an
|
9 | extensible set of plugins known as _strategies_. Passport does not mount
|
10 | routes or assume any particular database schema, which maximizes flexibility and
|
11 | allows application-level decisions to be made by the developer. The API is
|
12 | simple: you provide Passport a request to authenticate, and Passport provides
|
13 | hooks for controlling what occurs when authentication succeeds or fails.
|
14 |
|
15 | ---
|
16 |
|
17 | <p align="center">
|
18 | <sup>Sponsors</sup>
|
19 | <br>
|
20 | <a href="https://workos.com/?utm_campaign=github_repo&utm_medium=referral&utm_content=passport_js&utm_source=github"><img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/workos.png"></a><br/>
|
21 | <a href="https://workos.com/?utm_campaign=github_repo&utm_medium=referral&utm_content=passport_js&utm_source=github"><b>Your app, enterprise-ready.</b><br/>Start selling to enterprise customers with just a few lines of code. Add Single Sign-On (and more) in minutes instead of months.</a>
|
22 | <br>
|
23 | <br>
|
24 | <a href="https://www.descope.com/?utm_source=PassportJS&utm_medium=referral&utm_campaign=oss-sponsorship">
|
25 | <picture>
|
26 | <source media="(prefers-color-scheme: dark)" srcset="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope-dark.svg">
|
27 | <source media="(prefers-color-scheme: light)" srcset="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope.svg">
|
28 | <img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope.svg" width="275">
|
29 | </picture>
|
30 | </a><br/>
|
31 | <a href="https://www.descope.com/?utm_source=PassportJS&utm_medium=referral&utm_campaign=oss-sponsorship"><b>Drag and drop your auth</b><br/>Add authentication and user management to your consumer and business apps with a few lines of code.</a>
|
32 | <br>
|
33 | <br>
|
34 | <a href="https://fusionauth.io/?utm_source=passportjs&utm_medium=referral&utm_campaign=sponsorship"><img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/fusionauth.png" width="275"></a><br/>
|
35 | <a href="https://fusionauth.io/?utm_source=passportjs&utm_medium=referral&utm_campaign=sponsorship"><b>Auth. Built for Devs, by Devs</b><br/>Add login, registration, SSO, MFA, and a bazillion other features to your app in minutes. Integrates with any codebase and installs on any server, anywhere in the world.</a>
|
36 | </p>
|
37 |
|
38 | ---
|
39 |
|
40 | Status:
|
41 | [](https://travis-ci.org/jaredhanson/passport)
|
42 | [](https://coveralls.io/r/jaredhanson/passport)
|
43 | [](https://david-dm.org/jaredhanson/passport)
|
44 |
|
45 |
|
46 | ## Install
|
47 |
|
48 | ```
|
49 | $ npm install passport
|
50 | ```
|
51 |
|
52 | ## Usage
|
53 |
|
54 | #### Strategies
|
55 |
|
56 | Passport uses the concept of strategies to authenticate requests. Strategies
|
57 | can range from verifying username and password credentials, delegated
|
58 | authentication using [OAuth](http://oauth.net/) (for example, via [Facebook](http://www.facebook.com/)
|
59 | or [Twitter](http://twitter.com/)), or federated authentication using [OpenID](http://openid.net/).
|
60 |
|
61 | Before authenticating requests, the strategy (or strategies) used by an
|
62 | application must be configured.
|
63 |
|
64 | ```javascript
|
65 | passport.use(new LocalStrategy(
|
66 | function(username, password, done) {
|
67 | User.findOne({ username: username }, function (err, user) {
|
68 | if (err) { return done(err); }
|
69 | if (!user) { return done(null, false); }
|
70 | if (!user.verifyPassword(password)) { return done(null, false); }
|
71 | return done(null, user);
|
72 | });
|
73 | }
|
74 | ));
|
75 | ```
|
76 |
|
77 | There are 480+ strategies. Find the ones you want at: [passportjs.org](http://passportjs.org)
|
78 |
|
79 | #### Sessions
|
80 |
|
81 | Passport will maintain persistent login sessions. In order for persistent
|
82 | sessions to work, the authenticated user must be serialized to the session, and
|
83 | deserialized when subsequent requests are made.
|
84 |
|
85 | Passport does not impose any restrictions on how your user records are stored.
|
86 | Instead, you provide functions to Passport which implements the necessary
|
87 | serialization and deserialization logic. In a typical application, this will be
|
88 | as simple as serializing the user ID, and finding the user by ID when
|
89 | deserializing.
|
90 |
|
91 | ```javascript
|
92 | passport.serializeUser(function(user, done) {
|
93 | done(null, user.id);
|
94 | });
|
95 |
|
96 | passport.deserializeUser(function(id, done) {
|
97 | User.findById(id, function (err, user) {
|
98 | done(err, user);
|
99 | });
|
100 | });
|
101 | ```
|
102 |
|
103 | #### Middleware
|
104 |
|
105 | To use Passport in an [Express](http://expressjs.com/) or
|
106 | [Connect](http://senchalabs.github.com/connect/)-based application, configure it
|
107 | with the required `passport.initialize()` middleware. If your application uses
|
108 | persistent login sessions (recommended, but not required), `passport.session()`
|
109 | middleware must also be used.
|
110 |
|
111 | ```javascript
|
112 | var app = express();
|
113 | app.use(require('serve-static')(__dirname + '/../../public'));
|
114 | app.use(require('cookie-parser')());
|
115 | app.use(require('body-parser').urlencoded({ extended: true }));
|
116 | app.use(require('express-session')({ secret: 'keyboard cat', resave: true, saveUninitialized: true }));
|
117 | app.use(passport.initialize());
|
118 | app.use(passport.session());
|
119 | ```
|
120 |
|
121 | #### Authenticate Requests
|
122 |
|
123 | Passport provides an `authenticate()` function, which is used as route
|
124 | middleware to authenticate requests.
|
125 |
|
126 | ```javascript
|
127 | app.post('/login',
|
128 | passport.authenticate('local', { failureRedirect: '/login' }),
|
129 | function(req, res) {
|
130 | res.redirect('/');
|
131 | });
|
132 | ```
|
133 |
|
134 | ## Strategies
|
135 |
|
136 | Passport has a comprehensive set of **over 480** authentication strategies
|
137 | covering social networking, enterprise integration, API services, and more.
|
138 |
|
139 | ## Search all strategies
|
140 |
|
141 | There is a **Strategy Search** at [passportjs.org](http://passportjs.org)
|
142 |
|
143 | The following table lists commonly used strategies:
|
144 |
|
145 | |Strategy | Protocol |Developer |
|
146 | |---------------------------------------------------------------|--------------------------|------------------------------------------------|
|
147 | |[Local](https://github.com/jaredhanson/passport-local) | HTML form |[Jared Hanson](https://github.com/jaredhanson) |
|
148 | |[OpenID](https://github.com/jaredhanson/passport-openid) | OpenID |[Jared Hanson](https://github.com/jaredhanson) |
|
149 | |[BrowserID](https://github.com/jaredhanson/passport-browserid) | BrowserID |[Jared Hanson](https://github.com/jaredhanson) |
|
150 | |[Facebook](https://github.com/jaredhanson/passport-facebook) | OAuth 2.0 |[Jared Hanson](https://github.com/jaredhanson) |
|
151 | |[Google](https://github.com/jaredhanson/passport-google) | OpenID |[Jared Hanson](https://github.com/jaredhanson) |
|
152 | |[Google](https://github.com/jaredhanson/passport-google-oauth) | OAuth / OAuth 2.0 |[Jared Hanson](https://github.com/jaredhanson) |
|
153 | |[Twitter](https://github.com/jaredhanson/passport-twitter) | OAuth |[Jared Hanson](https://github.com/jaredhanson) |
|
154 | |[Azure Active Directory](https://github.com/AzureAD/passport-azure-ad) | OAuth 2.0 / OpenID / SAML |[Azure](https://github.com/azuread) |
|
155 |
|
156 | ## Examples
|
157 |
|
158 | - For a complete, working example, refer to the [example](https://github.com/passport/express-4.x-local-example)
|
159 | that uses [passport-local](https://github.com/jaredhanson/passport-local).
|
160 | - **Local Strategy**: Refer to the following tutorials for setting up user authentication via LocalStrategy (`passport-local`):
|
161 | - Mongo
|
162 | - Express v3x - [Tutorial](http://mherman.org/blog/2016/09/25/node-passport-and-postgres/#.V-govpMrJE5) / [working example](https://github.com/mjhea0/passport-local-knex)
|
163 | - Express v4x - [Tutorial](http://mherman.org/blog/2015/01/31/local-authentication-with-passport-and-express-4/) / [working example](https://github.com/mjhea0/passport-local-express4)
|
164 | - Postgres
|
165 | - [Tutorial](http://mherman.org/blog/2015/01/31/local-authentication-with-passport-and-express-4/) / [working example](https://github.com/mjhea0/passport-local-express4)
|
166 | - **Social Authentication**: Refer to the following tutorials for setting up various social authentication strategies:
|
167 | - Express v3x - [Tutorial](http://mherman.org/blog/2013/11/10/social-authentication-with-passport-dot-js/) / [working example](https://github.com/mjhea0/passport-examples)
|
168 | - Express v4x - [Tutorial](http://mherman.org/blog/2015/09/26/social-authentication-in-node-dot-js-with-passport) / [working example](https://github.com/mjhea0/passport-social-auth)
|
169 |
|
170 | ## Related Modules
|
171 |
|
172 | - [Locomotive](https://github.com/jaredhanson/locomotive) — Powerful MVC web framework
|
173 | - [OAuthorize](https://github.com/jaredhanson/oauthorize) — OAuth service provider toolkit
|
174 | - [OAuth2orize](https://github.com/jaredhanson/oauth2orize) — OAuth 2.0 authorization server toolkit
|
175 | - [connect-ensure-login](https://github.com/jaredhanson/connect-ensure-login) — middleware to ensure login sessions
|
176 |
|
177 | The [modules](https://github.com/jaredhanson/passport/wiki/Modules) page on the
|
178 | [wiki](https://github.com/jaredhanson/passport/wiki) lists other useful modules
|
179 | that build upon or integrate with Passport.
|
180 |
|
181 | ## License
|
182 |
|
183 | [The MIT License](http://opensource.org/licenses/MIT)
|
184 |
|
185 | Copyright (c) 2011-2021 Jared Hanson <[https://www.jaredhanson.me/](https://www.jaredhanson.me/)>
|