1 | # PostCSS Custom Properties [<img src="https://postcss.github.io/postcss/logo.svg" alt="PostCSS" width="90" height="90" align="right">][postcss]
|
2 |
|
3 | [![NPM Version][npm-img]][npm-url]
|
4 | [![CSS Standard Status][css-img]][css-url]
|
5 | [![Build Status][cli-img]][cli-url]
|
6 | [<img alt="Discord" src="https://shields.io/badge/Discord-5865F2?logo=discord&logoColor=white">][discord]
|
7 |
|
8 | [PostCSS Custom Properties] lets you use Custom Properties in CSS, following
|
9 | the [CSS Custom Properties] specification.
|
10 |
|
11 | [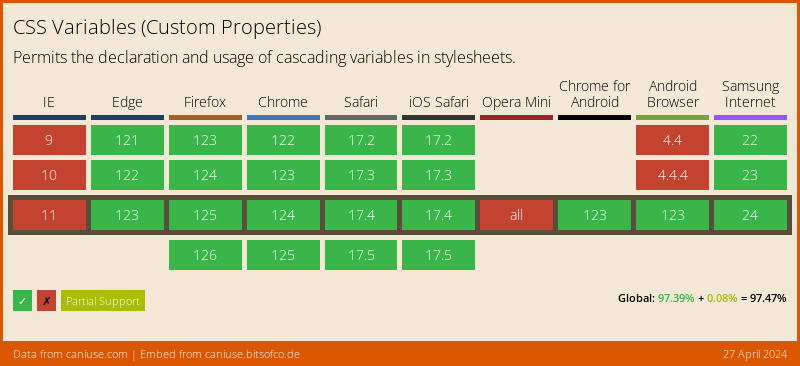](https://caniuse.com/#feat=css-variables)
|
12 |
|
13 | ```pcss
|
14 | :root {
|
15 | --color: red;
|
16 | }
|
17 |
|
18 | h1 {
|
19 | color: var(--color);
|
20 | }
|
21 |
|
22 | /* becomes */
|
23 |
|
24 | :root {
|
25 | --color: red;
|
26 | }
|
27 |
|
28 | h1 {
|
29 | color: red;
|
30 | color: var(--color);
|
31 | }
|
32 | ```
|
33 |
|
34 | **Note:** This plugin only processes variables that are defined in the `:root` selector.
|
35 |
|
36 | ## Usage
|
37 |
|
38 | Add [PostCSS Custom Properties] to your project:
|
39 |
|
40 | ```bash
|
41 | npm install postcss-custom-properties --save-dev
|
42 | ```
|
43 |
|
44 | Use [PostCSS Custom Properties] as a [PostCSS] plugin:
|
45 |
|
46 | ```js
|
47 | const postcss = require('postcss');
|
48 | const postcssCustomProperties = require('postcss-custom-properties');
|
49 |
|
50 | postcss([
|
51 | postcssCustomProperties(/* pluginOptions */)
|
52 | ]).process(YOUR_CSS /*, processOptions */);
|
53 | ```
|
54 |
|
55 | [PostCSS Custom Properties] runs in all Node environments, with special instructions for:
|
56 |
|
57 | | [Node](INSTALL.md#node) | [PostCSS CLI](INSTALL.md#postcss-cli) | [Webpack](INSTALL.md#webpack) | [Create React App](INSTALL.md#create-react-app) | [Gulp](INSTALL.md#gulp) | [Grunt](INSTALL.md#grunt) |
|
58 | | --- | --- | --- | --- | --- | --- |
|
59 |
|
60 | ## Options
|
61 |
|
62 | ### preserve
|
63 |
|
64 | The `preserve` option determines whether Custom Properties and properties using
|
65 | custom properties should be preserved in their original form. By default, both
|
66 | of these are preserved.
|
67 |
|
68 | ```js
|
69 | postcssCustomProperties({
|
70 | preserve: false
|
71 | });
|
72 | ```
|
73 |
|
74 | ```pcss
|
75 | :root {
|
76 | --color: red;
|
77 | }
|
78 |
|
79 | h1 {
|
80 | color: var(--color);
|
81 | }
|
82 |
|
83 | /* becomes */
|
84 |
|
85 | h1 {
|
86 | color: red;
|
87 | }
|
88 | ```
|
89 |
|
90 | ### importFrom
|
91 |
|
92 | The `importFrom` option specifies sources where Custom Properties can be imported
|
93 | from, which might be CSS, JS, and JSON files, functions, and directly passed
|
94 | objects.
|
95 |
|
96 | ```js
|
97 | postcssCustomProperties({
|
98 | importFrom: 'path/to/file.css' // => :root { --color: red }
|
99 | });
|
100 | ```
|
101 |
|
102 | ```pcss
|
103 | h1 {
|
104 | color: var(--color);
|
105 | }
|
106 |
|
107 | /* becomes */
|
108 |
|
109 | h1 {
|
110 | color: red;
|
111 | }
|
112 | ```
|
113 |
|
114 | Multiple sources can be passed into this option, and they will be parsed in the
|
115 | order they are received. JavaScript files, JSON files, functions, and objects
|
116 | will need to namespace Custom Properties using the `customProperties` or
|
117 | `custom-properties` key.
|
118 |
|
119 | ```js
|
120 | postcssCustomProperties({
|
121 | importFrom: [
|
122 | 'path/to/file.css', // :root { --color: red; }
|
123 | 'and/then/this.js', // module.exports = { customProperties: { '--color': 'red' } }
|
124 | 'and/then/that.json', // { "custom-properties": { "--color": "red" } }
|
125 | {
|
126 | customProperties: { '--color': 'red' }
|
127 | },
|
128 | () => {
|
129 | const customProperties = { '--color': 'red' };
|
130 |
|
131 | return { customProperties };
|
132 | }
|
133 | ]
|
134 | });
|
135 | ```
|
136 |
|
137 | See example imports written in [CSS](test/import-properties.css),
|
138 | [JS](test/import-properties.js), and [JSON](test/import-properties.json).
|
139 |
|
140 | ### overrideImportFromWithRoot
|
141 |
|
142 | The `overrideImportFromWithRoot` option determines if properties added via `importFrom` are overridden by properties that exist in `:root`.
|
143 | Defaults to `false`.
|
144 |
|
145 | ```js
|
146 | postcssCustomProperties({
|
147 | overrideImportFromWithRoot: true
|
148 | });
|
149 | ```
|
150 |
|
151 | ### exportTo
|
152 |
|
153 | The `exportTo` option specifies destinations where Custom Properties can be exported
|
154 | to, which might be CSS, JS, and JSON files, functions, and directly passed
|
155 | objects.
|
156 |
|
157 | ```js
|
158 | postcssCustomProperties({
|
159 | exportTo: 'path/to/file.css' // :root { --color: red; }
|
160 | });
|
161 | ```
|
162 |
|
163 | Multiple destinations can be passed into this option, and they will be parsed
|
164 | in the order they are received. JavaScript files, JSON files, and objects will
|
165 | need to namespace Custom Properties using the `customProperties` or
|
166 | `custom-properties` key.
|
167 |
|
168 | ```js
|
169 | const cachedObject = { customProperties: {} };
|
170 |
|
171 | postcssCustomProperties({
|
172 | exportTo: [
|
173 | 'path/to/file.css', // :root { --color: red; }
|
174 | 'and/then/this.js', // module.exports = { customProperties: { '--color': 'red' } }
|
175 | 'and/then/this.mjs', // export const customProperties = { '--color': 'red' } }
|
176 | 'and/then/that.json', // { "custom-properties": { "--color": "red" } }
|
177 | 'and/then/that.scss', // $color: red;
|
178 | cachedObject,
|
179 | customProperties => {
|
180 | customProperties // { '--color': 'red' }
|
181 | }
|
182 | ]
|
183 | });
|
184 | ```
|
185 |
|
186 | See example exports written to [CSS](test/export-properties.css),
|
187 | [JS](test/export-properties.js), [MJS](test/export-properties.mjs),
|
188 | [JSON](test/export-properties.json) and [SCSS](test/export-properties.scss).
|
189 |
|
190 | ### disableDeprecationNotice
|
191 |
|
192 | Silence the deprecation notice that is printed to the console when using `importFrom` or `exportTo`.
|
193 |
|
194 | > "importFrom" and "exportTo" will be removed in a future version of postcss-custom-properties.
|
195 | > Check the discussion on github for more details. https://github.com/csstools/postcss-plugins/discussions/192
|
196 |
|
197 | [cli-img]: https://github.com/csstools/postcss-plugins/actions/workflows/test.yml/badge.svg
|
198 | [cli-url]: https://github.com/csstools/postcss-plugins/actions/workflows/test.yml?query=workflow/test
|
199 | [css-img]: https://cssdb.org/images/badges/custom-properties.svg
|
200 | [css-url]: https://cssdb.org/#custom-properties
|
201 | [discord]: https://discord.gg/bUadyRwkJS
|
202 | [npm-img]: https://img.shields.io/npm/v/postcss-custom-properties.svg
|
203 | [npm-url]: https://www.npmjs.com/package/postcss-custom-properties
|
204 |
|
205 | [CSS Custom Properties]: https://www.w3.org/TR/css-variables-1/
|
206 | [PostCSS]: https://github.com/postcss/postcss
|
207 | [PostCSS Custom Properties]: https://github.com/csstools/postcss-plugins/tree/main/plugins/postcss-custom-properties
|