1 | <p align="center"><img src ="https://github.com/bokuweb/re-resizable/blob/master/logo.png?raw=true" /></p>
|
2 |
|
3 | <p align="center">📏 A resizable component for React.</p>
|
4 |
|
5 | <p align="center"><img src="https://github.com/bokuweb/re-resizable/workflows/Continuous%20Integration/badge.svg" alt="Build Status" />
|
6 | <a href="https://www.npmjs.com/package/re-resizable">
|
7 | <img src="https://img.shields.io/npm/v/re-resizable.svg" alt="Build Status" /></a>
|
8 | <a href="https://www.npmjs.com/package/re-resizable">
|
9 | <img src="https://img.shields.io/npm/dm/re-resizable.svg" /></a>
|
10 | <a href="https://renovatebot.com/">
|
11 | <img src="https://img.shields.io/badge/renovate-enabled-brightgreen.svg" /></a>
|
12 | <a href="https://github.com/prettier/prettier">
|
13 | <img src="https://img.shields.io/badge/styled_with-prettier-ff69b4.svg" /></a>
|
14 | </p>
|
15 |
|
16 | ## Table of Contents
|
17 |
|
18 | - [Screenshot](#Screenshot)
|
19 | - [Live Demo](#live-demo)
|
20 | - [Storybook](#storybook)
|
21 | - [CodeSandbox](#codesandbox)
|
22 | - [Install](#install)
|
23 | - [Usage](#usage)
|
24 | - [Props](#props)
|
25 | - [Instance API](#instance-api)
|
26 | - [updateSize(size: { width: number | string, height: number | string }): void](#updateSize-void)
|
27 | - [Test](#test)
|
28 | - [Related](#related)
|
29 |
|
30 | ## Screenshot
|
31 |
|
32 | 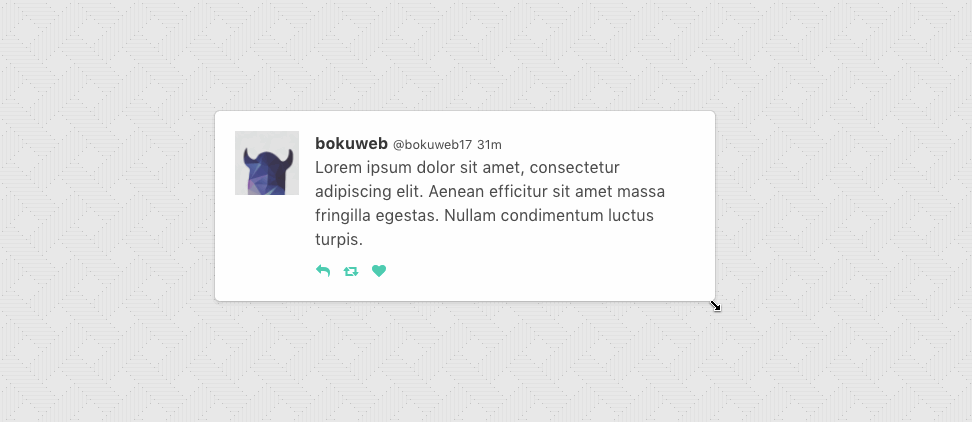
|
33 |
|
34 | ## Live Demo
|
35 |
|
36 | ### Storybook
|
37 |
|
38 | [Storybook](http://bokuweb.github.io/re-resizable/)
|
39 |
|
40 | ### CodeSandbox
|
41 |
|
42 | [](https://codesandbox.io/s/xp9p7272m4)
|
43 | [CodeSandbox](https://codesandbox.io/s/xp9p7272m4)
|
44 | [CodeSandbox(TypeScript)](https://codesandbox.io/s/1vwo2p4l64)
|
45 | [CodeSandbox(With hooks)](https://codesandbox.io/s/blissful-joliot-d3unx)
|
46 |
|
47 | ## Install
|
48 |
|
49 | ```sh
|
50 | $ npm install --save re-resizable
|
51 | ```
|
52 |
|
53 | ## Usage
|
54 |
|
55 | ### Example with `defaultSize`
|
56 |
|
57 | ```javascript
|
58 | import { Resizable } from 're-resizable';
|
59 |
|
60 | <Resizable
|
61 | defaultSize={{
|
62 | width: 320,
|
63 | height: 200,
|
64 | }}
|
65 | >
|
66 | Sample with default size
|
67 | </Resizable>
|
68 | ```
|
69 |
|
70 | If you only want to set the width, you can do so by providing just the width property.
|
71 | The height property will automatically be set to auto, which means it will adjust 100% of its parent's height:
|
72 |
|
73 | ```javascript
|
74 | import { Resizable } from 're-resizable';
|
75 |
|
76 | <Resizable
|
77 | defaultSize={{
|
78 | width: 320
|
79 | }}
|
80 | >
|
81 | Sample with default size
|
82 | </Resizable>
|
83 | ```
|
84 | ### Example with `size`
|
85 |
|
86 | If you use `size` props, please manage state by yourself.
|
87 |
|
88 | ```javascript
|
89 | import { Resizable } from 're-resizable';
|
90 |
|
91 | <Resizable
|
92 | size={{ width: this.state.width, height: this.state.height }}
|
93 | onResizeStop={(e, direction, ref, d) => {
|
94 | this.setState({
|
95 | width: this.state.width + d.width,
|
96 | height: this.state.height + d.height,
|
97 | });
|
98 | }}
|
99 | >
|
100 | Sample with size
|
101 | </Resizable>
|
102 | ```
|
103 |
|
104 | ## Props
|
105 |
|
106 | #### `defaultSize?: { width?: (number | string), height?: (number | string) };`
|
107 |
|
108 | Specifies the `width` and `height` that the dragged item should start at.
|
109 | For example, you can set `300`, `'300px'`, `50%`.
|
110 | If both `defaultSize` and `size` omitted, set `'auto'`.
|
111 |
|
112 | `defaultSize` will be ignored when `size` set.
|
113 |
|
114 | #### `size?: { width?: (number | string), height?: (number | string) };`
|
115 |
|
116 | The `size` property is used to set the size of the component.
|
117 | For example, you can set `300`, `'300px'`, `50%`.
|
118 |
|
119 | Use `size` if you need to control size state by yourself.
|
120 |
|
121 | #### `className?: string;`
|
122 |
|
123 | The `className` property is used to set the custom `className` of a resizable component.
|
124 |
|
125 | #### `style?: { [key: string]: string };`
|
126 |
|
127 | The `style` property is used to set the custom `style` of a resizable component.
|
128 |
|
129 | #### `minWidth?: number | string;`
|
130 |
|
131 | The `minWidth` property is used to set the minimum width of a resizable component. Defaults to 10px.
|
132 |
|
133 | It accepts viewport as well as parent relative units. For example, you can set `300`, `50%`, `50vw` or `50vh`.
|
134 |
|
135 | Same type of values can be applied to `minHeight`, `maxWidth` and `maxHeight`.
|
136 |
|
137 | #### `minHeight?: number | string;`
|
138 |
|
139 | The `minHeight` property is used to set the minimum height of a resizable component. Defaults to 10px.
|
140 |
|
141 | #### `maxWidth?: number | string;`
|
142 |
|
143 | The `maxWidth` property is used to set the maximum width of a resizable component.
|
144 |
|
145 | #### `maxHeight?: number | string`;
|
146 |
|
147 | The `maxHeight` property is used to set the maximum height of a resizable component.
|
148 |
|
149 | #### `grid?: [number, number];`
|
150 |
|
151 | The `grid` property is used to specify the increments that resizing should snap to. Defaults to `[1, 1]`.
|
152 |
|
153 | #### `gridGap?: [number, number];`
|
154 |
|
155 | The `gridGap` property is used to specify any gaps between your grid cells that should be accounted for when resizing. Defaults to `[0, 0]`.
|
156 | The value provided for each axis will always add the grid gap amount times grid cells spanned minus one.
|
157 |
|
158 | #### `snap?: { x?: Array<number>, y?: Array<number> };`
|
159 |
|
160 | The `snap` property is used to specify absolute pixel values that resizing should snap to. `x` and `y` are both optional, allowing you to only include the axis you want to define. Defaults to `null`.
|
161 |
|
162 | #### `snapGap?: number`
|
163 |
|
164 | The `snapGap` property is used to specify the minimum gap required in order to move to the next snapping target. Defaults to `0` which means that snap targets are always used.
|
165 |
|
166 | #### `resizeRatio?: number | [number, number];`
|
167 |
|
168 | The `resizeRatio` property is used to set the number of pixels the resizable component scales by compared to the number of pixels the mouse/touch moves. Defaults to `1` (for a 1:1 ratio). The number set is the left side of the ratio, `2` will give a 2:1 ratio.
|
169 |
|
170 | For [number, number] means [resizeRatioX, resizeRatioY], more precise control.
|
171 |
|
172 | #### `lockAspectRatio?: boolean | number;`
|
173 |
|
174 | The `lockAspectRatio` property is used to lock aspect ratio.
|
175 | Set to `true` to lock the aspect ratio based on the initial size.
|
176 | Set to a numeric value to lock a specific aspect ratio (such as `16/9`).
|
177 | If set to numeric, make sure to set initial height/width to values with correct aspect ratio.
|
178 | If omitted, set `false`.
|
179 |
|
180 | #### `lockAspectRatioExtraWidth?: number;`
|
181 |
|
182 | The `lockAspectRatioExtraWidth` property enables a resizable component to maintain an aspect ratio plus extra width.
|
183 | For instance, a video could be displayed 16:9 with a 50px side bar.
|
184 | If omitted, set `0`.
|
185 |
|
186 | #### `lockAspectRatioExtraHeight?: number;`
|
187 |
|
188 | The `lockAspectRatioExtraHeight` property enables a resizable component to maintain an aspect ratio plus extra height.
|
189 | For instance, a video could be displayed 16:9 with a 50px header bar.
|
190 | If omitted, set `0`.
|
191 |
|
192 | #### `bounds?: ('window' | 'parent' | HTMLElement);`
|
193 |
|
194 | Specifies resize boundaries.
|
195 |
|
196 | #### `boundsByDirection?: boolean;`
|
197 |
|
198 | By default max dimensions based on left and top element position.
|
199 | Width grow to right side, height grow to bottom side.
|
200 | Set `true` for detect max dimensions by direction.
|
201 | For example: enable `boundsByDirection` when resizable component stick on right side of screen and you want resize by left handler;
|
202 |
|
203 | `false` by default.
|
204 |
|
205 | #### `handleStyles?: HandleStyles;`
|
206 |
|
207 | The `handleStyles` property is used to override the style of one or more resize handles.
|
208 | Only the axis you specify will have its handle style replaced.
|
209 | If you specify a value for `right` it will completely replace the styles for the `right` resize handle,
|
210 | but other handle will still use the default styles.
|
211 |
|
212 | #### `handleClasses?: HandleClassName;`
|
213 |
|
214 | The `handleClasses` property is used to set the className of one or more resize handles.
|
215 |
|
216 | #### `handleComponent?: HandleComponent;`
|
217 |
|
218 | The `handleComponent` property is used to pass a React Component to be rendered as one or more resize handle. For example, this could be used to use an arrow icon as a handle..
|
219 |
|
220 | #### `handleWrapperStyle?: { [key: string]: string };`
|
221 |
|
222 | The `handleWrapperStyle` property is used to override the style of resize handles wrapper.
|
223 |
|
224 | #### `handleWrapperClass?: string;`
|
225 |
|
226 | The `handleWrapperClass` property is used to override the className of resize handles wrapper.
|
227 |
|
228 | #### `enable?: ?Enable | false;`
|
229 |
|
230 | The `enable` property is used to set the resizable permission of a resizable component.
|
231 |
|
232 | The permission of `top`, `right`, `bottom`, `left`, `topRight`, `bottomRight`, `bottomLeft`, `topLeft` direction resizing.
|
233 | If omitted, all resizer are enabled.
|
234 | If you want to permit only right direction resizing, set `{ top:false, right:true, bottom:false, left:false, topRight:false, bottomRight:false, bottomLeft:false, topLeft:false }`.
|
235 |
|
236 | #### `onResizeStart?: ResizeStartCallBack;`
|
237 |
|
238 | `ResizeStartCallBack` type is below.
|
239 |
|
240 | ```javascript
|
241 | type ResizeStartCallback = (
|
242 | e: SyntheticMouseEvent<HTMLDivElement> | SyntheticTouchEvent<HTMLDivElement>,
|
243 | dir: ResizableDirection,
|
244 | refToElement: HTMLDivElement,
|
245 | ) => void;
|
246 | ```
|
247 |
|
248 | Calls when resizable component resize start.
|
249 |
|
250 | #### `onResize?: ResizeCallback;`
|
251 |
|
252 | #### `scale?: number`;
|
253 |
|
254 | The `scale` property is used in the scenario where the resizable element is a descendent of an element using css scaling (e.g. - `transform: scale(0.5)`).
|
255 |
|
256 | #### `as?: string | React.ComponentType`;
|
257 |
|
258 | By default the `Resizable` component will render a `div` as a wrapper. The `as` property is used to change the element used.
|
259 |
|
260 | ### Basic
|
261 |
|
262 | `ResizeCallback` type is below.
|
263 |
|
264 | ```javascript
|
265 | type ResizeCallback = (
|
266 | event: MouseEvent | TouchEvent,
|
267 | direction: ResizableDirection,
|
268 | refToElement: HTMLDivElement,
|
269 | delta: NumberSize,
|
270 | ) => void;
|
271 | ```
|
272 |
|
273 | Calls when resizable component resizing.
|
274 |
|
275 | #### `onResizeStop?: ResizeCallback;`
|
276 |
|
277 | `ResizeCallback` type is below.
|
278 |
|
279 | ```javascript
|
280 | type ResizeCallback = (
|
281 | event: MouseEvent | TouchEvent,
|
282 | direction: ResizableDirection,
|
283 | refToElement: HTMLDivElement,
|
284 | delta: NumberSize,
|
285 | ) => void;
|
286 | ```
|
287 |
|
288 | Calls when resizable component resize stop.
|
289 |
|
290 | ## Instance API
|
291 |
|
292 | #### * `updateSize(size: { width: number | string, height: number | string }): void`
|
293 |
|
294 | Update component size.
|
295 |
|
296 | `grid`, `snap`, `max/minWidth`, `max/minHeight` props is ignored, when this method called.
|
297 |
|
298 | - for example
|
299 |
|
300 | ```javascript
|
301 | class YourComponent extends Component {
|
302 |
|
303 | // ...
|
304 |
|
305 | update() {
|
306 | this.resizable.updateSize({ width: 200, height: 300 });
|
307 | }
|
308 |
|
309 | render() {
|
310 | return (
|
311 | <Resizable ref={c => { this.resizable = c; }}>
|
312 | example
|
313 | </Resizable>
|
314 | );
|
315 | }
|
316 |
|
317 | // ...
|
318 | }
|
319 | ```
|
320 |
|
321 | ## Contribute
|
322 |
|
323 | If you have a feature request, please add it as an issue or make a pull request.
|
324 |
|
325 | If you have a bug to report, please reproduce the bug in [CodeSandbox](https://codesandbox.io/s/ll587k677z) to help us easily isolate it.
|
326 |
|
327 | ## Test
|
328 |
|
329 | ``` sh
|
330 | npm test
|
331 | ```
|
332 |
|
333 | ## Related
|
334 |
|
335 | - [react-rnd](https://github.com/bokuweb/react-rnd)
|
336 | - [react-sortable-pane](https://github.com/bokuweb/react-sortable-pane)
|