1 | # react-google-button
|
2 |
|
3 | [![NPM version][npm-image]][npm-url]
|
4 | [![Build Status][build-status-image]][build-status-url]
|
5 | [![Coverage][coverage-image]][coverage-url]
|
6 | [![License][license-image]][license-url]
|
7 | [![Code Style][code-style-image]][code-style-url]
|
8 |
|
9 | > Simple Google sign in button for React that follows Google's style guidelines (https://developers.google.com/identity/sign-in/web/build-button)
|
10 |
|
11 | ## [Codepen Demo](https://codepen.io/prescottprue/pen/NjmeKM)
|
12 |
|
13 | ## Rendered Preview
|
14 |
|
15 | 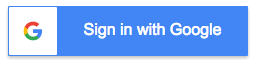
|
16 |
|
17 | ## Getting Started
|
18 |
|
19 | `react-google-button` is universal, so it can be used client-side or server-side.
|
20 |
|
21 | 1. Install through: `npm install --save react-google-button`
|
22 |
|
23 | 2. Import `GoogleButton` from `react-google-button`:
|
24 |
|
25 | ```javascript
|
26 | import GoogleButton from 'react-google-button'
|
27 | ```
|
28 |
|
29 | 3. Use `GoogleButton` component:
|
30 |
|
31 | ```javascript
|
32 | <GoogleButton
|
33 | onClick={() => { console.log('Google button clicked') }}
|
34 | />
|
35 | ```
|
36 |
|
37 | ## Props
|
38 |
|
39 | ### type
|
40 | ##### PropType
|
41 | ```js
|
42 | oneOf([ 'light', 'dark' ])
|
43 | ```
|
44 |
|
45 | ##### Default
|
46 | ```js
|
47 | 'dark'
|
48 | ```
|
49 |
|
50 | ##### Example
|
51 |
|
52 | ```js
|
53 | <GoogleButton
|
54 | type="light" // can be light or dark
|
55 | onClick={() => { console.log('Google button clicked') }}
|
56 | />
|
57 | ```
|
58 |
|
59 | ##### Description
|
60 | `'light'` or `'dark'` for the different google styles (defaults to `dark`)
|
61 |
|
62 |
|
63 | ### disabled
|
64 | `disabled` - whether or not button is disabled
|
65 |
|
66 | ##### PropType
|
67 | ```js
|
68 | Boolean
|
69 | ```
|
70 |
|
71 | ##### Default
|
72 | ```js
|
73 | false
|
74 | ```
|
75 |
|
76 | ##### Example
|
77 |
|
78 | ```javascript
|
79 | <GoogleButton
|
80 | disabled // can also be written as disabled={true} for clarity
|
81 | onClick={() => { console.log('this will not run on click since it is disabled') }}
|
82 | />
|
83 | ```
|
84 |
|
85 | ### label
|
86 | ##### PropType
|
87 | ```js
|
88 | String
|
89 | ```
|
90 | ##### Default
|
91 | ```js
|
92 | 'Sign in with Google'
|
93 | ```
|
94 |
|
95 | ##### Example
|
96 |
|
97 | ```javascript
|
98 | <GoogleButton
|
99 | label='Be Cool'
|
100 | onClick={() => { console.log('Google button clicked') }}
|
101 | />
|
102 | ```
|
103 |
|
104 | ##### Description
|
105 | Override the 'Sign in with Google' words with another string.
|
106 |
|
107 | **Note**: [Google's branding guidelines](https://developers.google.com/identity/branding-guidelines) states you should not to do this
|
108 |
|
109 |
|
110 | ## Builds
|
111 |
|
112 | Most commonly people consume `react-google-button` as a [CommonJS module](http://webpack.github.io/docs/commonjs.html). This module is what you get when you import redux in a Webpack, Browserify, or a Node environment.
|
113 |
|
114 | If you don't use a module bundler, it's also fine. The `react-google-button` npm package includes precompiled production and development [UMD builds](https://github.com/umdjs/umd) in the [dist folder](https://unpkg.com/react-google-button@latest/dist/). They can be used directly without a bundler and are thus compatible with many popular JavaScript module loaders and environments. For example, you can drop a UMD build as a `<script>` tag on the page. The UMD builds make Redux Firestore available as a `window.ReduxFirestore` global variable.
|
115 |
|
116 | It can be imported like so:
|
117 |
|
118 | ```html
|
119 | <script src="../node_modules/react-google-button/dist/react-google-button.min.js"></script>
|
120 | <!-- or through cdn: <script src="https://unpkg.com/react-google-button@latest/dist/react-google-button.min.js"></script> -->
|
121 | <script>console.log('redux firestore:', window.ReactGoogleButton)</script>
|
122 | ```
|
123 |
|
124 | Note: In an effort to keep things simple, the wording from this explanation was modeled after [the installation section of the Redux Docs](https://redux.js.org/#installation).
|
125 |
|
126 | [npm-image]: https://img.shields.io/npm/v/react-google-button.svg?style=flat-square
|
127 | [npm-url]: https://npmjs.org/package/react-google-button
|
128 | [build-status-image]: https://img.shields.io/github/workflow/status/prescottprue/react-google-button/NPM%20Package%20Publish?style=flat-square&logo=github
|
129 | [build-status-url]: https://github.com/prescottprue/react-google-button/actions
|
130 | [coverage-image]: https://img.shields.io/codeclimate/coverage/github/prescottprue/react-google-button.svg?style=flat-square&logo=codecov
|
131 | [coverage-url]: https://codeclimate.com/github/prescottprue/react-google-button
|
132 | [license-image]: https://img.shields.io/npm/l/react-google-button.svg?style=flat-square
|
133 | [license-url]: https://github.com/prescottprue/react-google-button/blob/master/LICENSE
|
134 | [code-style-image]: https://img.shields.io/badge/code%20style-standard-brightgreen.svg?style=flat-square
|
135 | [code-style-url]: http://standardjs.com/
|