1 | # React-Hookz
|
2 |
|
3 | [](https://travis-ci.com/garywenneker/react-hookz)
|
4 | [](https://badge.fury.io/js/react-hookz)
|
5 | [](https://bundlephobia.com/result?p=react-hookz)
|
6 | [](https://img.shields.io/npm/dt/react-hookz.svg)
|
7 |
|
8 | 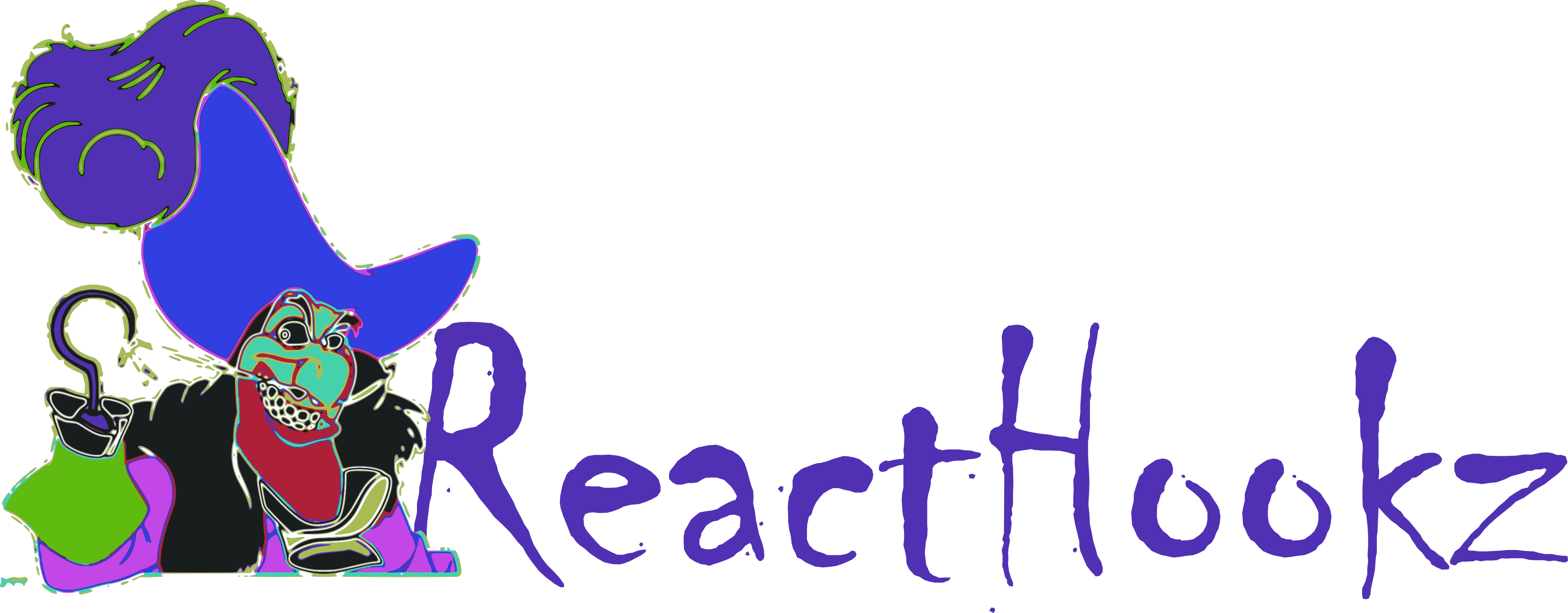
|
9 |
|
10 | React Global Hookz, a simple global state for React with the Hooks API in less than 1kb written in TypeScript
|
11 |
|
12 | ---
|
13 |
|
14 | Table of Contents
|
15 |
|
16 | - [Installation](#installation)
|
17 | - [Usage](#usage)
|
18 | - [Examples](#examples)
|
19 |
|
20 | ### Installation
|
21 |
|
22 | ```
|
23 | npm install react-hookz
|
24 | ```
|
25 |
|
26 | ## Usage
|
27 |
|
28 | ### Minimal example:
|
29 |
|
30 | #### Actions
|
31 |
|
32 | ```javascript
|
33 | export const addToCounter = (store: any, amount: number) => {
|
34 | const counter = store.state.counter + amount;
|
35 | store.setState({ counter });
|
36 | };
|
37 | ```
|
38 |
|
39 | #### HOC
|
40 |
|
41 | ```javascript
|
42 | import React from "react";
|
43 | import ReactHookz from "react-hookz";
|
44 |
|
45 | import * as actions from "../actions/index";
|
46 |
|
47 | export interface GlobalState {
|
48 | counter: number;
|
49 | }
|
50 | const initialState: GlobalState = {
|
51 | counter: 1
|
52 | };
|
53 |
|
54 | const useReactHookz = ReactHookz(React, initialState, actions);
|
55 | export const connect = Component => {
|
56 | return props => {
|
57 | let [state, actions] = useReactHookz();
|
58 | let _props = { ...props, state, actions };
|
59 | return <Component {..._props} />;
|
60 | };
|
61 | };
|
62 |
|
63 | export default useReactHookz;
|
64 | ```
|
65 |
|
66 | #### Component
|
67 |
|
68 | ```javascript
|
69 | import React from "react";
|
70 | import { connect } from "../store";
|
71 |
|
72 | interface Props {
|
73 | state: any;
|
74 | actions: any;
|
75 | }
|
76 |
|
77 | const Counter: React.FC<Props> = props => {
|
78 | const { state, actions } = props;
|
79 | return (
|
80 | <div className="Counter">
|
81 | <p>
|
82 | FC Counter:
|
83 | {state.counter}
|
84 | </p>
|
85 | <button type="button" onClick={() => actions.addToCounter(1)}>
|
86 | +1 to global
|
87 | </button>
|
88 | </div>
|
89 | );
|
90 | };
|
91 |
|
92 | export default connect(Counter);
|
93 | ```
|
94 |
|
95 | ## Examples
|
96 |
|
97 | - [React Hookz minimal example (like usage above)](https://codesandbox.io/s/react-hookz-global-state-vl5x7)
|
98 | - [React Hookz HOC](https://codesandbox.io/s/react-hookz-hoc-112fy)
|
99 | - [React Hookz Fetch Action](https://codesandbox.io/s/react-hookz-fetch-action-demo-ellw3)
|
100 | - [Using React Hookz in a class component](https://codesandbox.io/s/react-hookz-class-component-hfimj) |
\ | No newline at end of file |