1 | # react-i18next [](https://twitter.com/intent/tweet?text=Awesome%20react-i18next%20for%20react.js%20based%20on%20i18next%20internationalization%20ecosystem%20&url=https://github.com/i18next/react-i18next&via=jamuhl&hashtags=i18n,reactjs,js,dev)
|
2 |
|
3 | [](https://github.com/i18next/react-i18next/actions/workflows/CI.yml)
|
4 | [](https://codeclimate.com/github/i18next/react-i18next)
|
5 | [](https://coveralls.io/github/i18next/react-i18next)
|
6 | [![Quality][quality-badge]][quality-url]
|
7 | [![npm][npm-dl-badge]][npm-url]
|
8 |
|
9 | [npm-icon]: https://nodei.co/npm/react-i18next.png?downloads=true
|
10 | [npm-url]: https://npmjs.org/package/react-i18next
|
11 | [quality-badge]: https://npm.packagequality.com/shield/react-i18next.svg
|
12 | [quality-url]: https://packagequality.com/#?package=react-i18next
|
13 | [npm-dl-badge]: https://img.shields.io/npm/dw/react-i18next
|
14 |
|
15 | ### IMPORTANT:
|
16 |
|
17 | Master Branch is the newest version using hooks (>= v10).
|
18 |
|
19 | ```bash
|
20 | $ >=v10.0.0
|
21 | npm i react-i18next
|
22 | ```
|
23 |
|
24 | **react-native: To use hooks within react-native, you must use react-native v0.59.0 or higher**
|
25 |
|
26 | For the legacy version please use the [v9.x.x Branch](https://github.com/i18next/react-i18next/tree/v9.x.x)
|
27 |
|
28 | ```bash
|
29 | $ v9.0.10 (legacy)
|
30 | npm i react-i18next@legacy
|
31 | ```
|
32 |
|
33 | ### Documentation
|
34 |
|
35 | The documentation is published on [react.i18next.com](https://react.i18next.com) and PR changes can be supplied [here](https://github.com/i18next/react-i18next-gitbook).
|
36 |
|
37 | The general i18next documentation is published on [www.i18next.com](https://www.i18next.com) and PR changes can be supplied [here](https://github.com/i18next/i18next-gitbook).
|
38 |
|
39 | ### What will my code look like?
|
40 |
|
41 | **Before:** Your react code would have looked something like:
|
42 |
|
43 | ```jsx
|
44 | ...
|
45 | <div>Just simple content</div>
|
46 | <div>
|
47 | Hello <strong title="this is your name">{name}</strong>, you have {count} unread message(s). <Link to="/msgs">Go to messages</Link>.
|
48 | </div>
|
49 | ...
|
50 | ```
|
51 |
|
52 | **After:** With the trans component just change it to:
|
53 |
|
54 | ```jsx
|
55 | ...
|
56 | <div>{t('simpleContent')}</div>
|
57 | <Trans i18nKey="userMessagesUnread" count={count}>
|
58 | Hello <strong title={t('nameTitle')}>{{name}}</strong>, you have {{count}} unread message. <Link to="/msgs">Go to messages</Link>.
|
59 | </Trans>
|
60 | ...
|
61 | ```
|
62 |
|
63 | ### 📖 What others say
|
64 |
|
65 | - [How to properly internationalize a React application using i18next](https://locize.com/blog/react-i18next/) by Adriano Raiano
|
66 | - [I18n with React and i18next](https://alligator.io/react/i18n-with-react-and-i18next) via Alligator.io by Danny Hurlburt
|
67 | - [Ultimate Localization of React (Mobx) App with i18next](https://itnext.io/ultimate-localization-of-react-mobx-app-with-i18next-efab77712149) via itnext.io by Viktor Shevchenko
|
68 | - [Internationalization for react done right Using the i18next i18n ecosystem](https://reactjsexample.com/internationalization-for-react-done-right-using-the-i18next-i18n-ecosystem/) via reactjsexample.com
|
69 | - [How to translate React application with react-i18next](https://codetain.com/blog/how-to-translate-react-application-with-react-i18next/) via codetain.co by Norbert Suski
|
70 | - [Building i18n with Gatsby](https://www.gatsbyjs.org/blog/2017-10-17-building-i18n-with-gatsby/) via gatsbyjs.org by Samuel Goudie
|
71 | - [Get your react.js application translated with style](https://medium.com/@jamuhl/get-your-react-js-application-translated-with-style-4ad090aefc2c) by Jan Mühlemann
|
72 | - [Translate your expo.io / react-native mobile application](https://medium.com/@jamuhl/translate-your-expo-io-react-native-mobile-application-aa220b2362d2) by Jan Mühlemann
|
73 | - You're welcome to share your story...
|
74 |
|
75 | ### Why i18next?
|
76 |
|
77 | - **Simplicity:** no need to change your webpack configuration or add additional babel transpilers, just use create-react-app and go.
|
78 | - **Production ready** we know there are more needs for production than just doing i18n on the clientside, so we offer wider support on [serverside](https://www.i18next.com/overview/supported-frameworks) too (nodejs, php, ruby, .net, ...). **Learn once - translate everywhere**.
|
79 | - **Beyond i18n** comes with [locize](https://locize.com) bridging the gap between development and translations - covering the whole translation process.
|
80 |
|
81 | 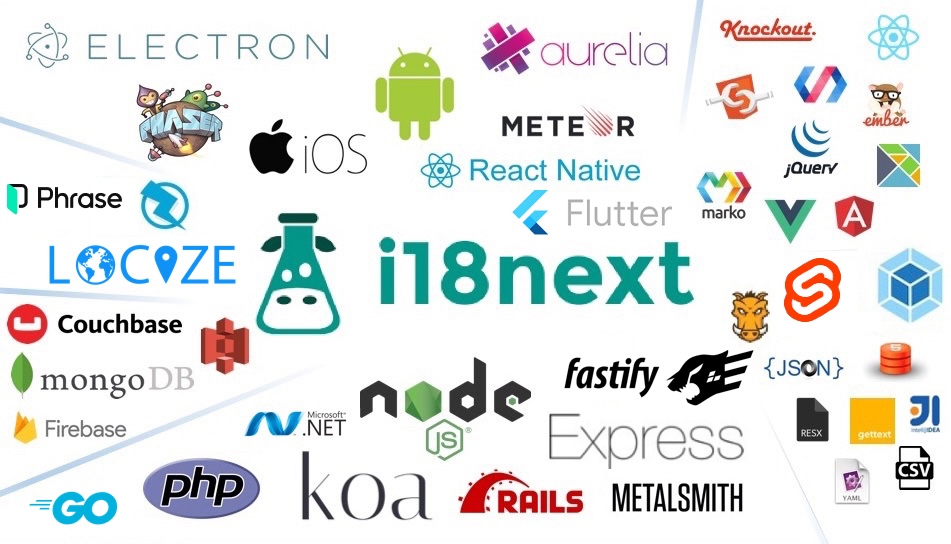
|
82 |
|
83 | ### Localization workflow
|
84 |
|
85 | Want to learn more about how seamless your internationalization and translation process can be?
|
86 |
|
87 | [](https://youtu.be/osScyaGMVqo)
|
88 |
|
89 | [watch the video](https://youtu.be/osScyaGMVqo)
|
90 |
|
91 | ### Installation
|
92 |
|
93 | Source can be loaded via [npm](https://www.npmjs.com/package/react-i18next) or [downloaded](https://github.com/i18next/react-i18next/blob/master/react-i18next.min.js) from this repo.
|
94 |
|
95 | ```
|
96 | # npm package
|
97 | $ npm install react-i18next
|
98 | ```
|
99 |
|
100 | - If you don't use a module loader it will be added to `window.reactI18next`
|
101 |
|
102 | ### Do you like to read a more complete step by step tutorial?
|
103 |
|
104 | [Here](https://locize.com/blog/react-i18next/) you'll find a simple tutorial on how to best use react-i18next.
|
105 | Some basics of i18next and some cool possibilities on how to optimize your localization workflow.
|
106 |
|
107 | ### Examples
|
108 |
|
109 | - [Example react](https://github.com/i18next/react-i18next/tree/master/example/react)
|
110 | - [React examples with typescript](https://github.com/i18next/react-i18next/tree/master/example/react-typescript)
|
111 | - [Example locize.com](https://github.com/i18next/react-i18next/tree/master/example/locize)
|
112 |
|
113 | #### v9 samples
|
114 |
|
115 | - [Example react](https://github.com/i18next/react-i18next/tree/v9.x.x/example/react)
|
116 | - [Example preact](https://github.com/i18next/react-i18next/tree/v9.x.x/example/preact)
|
117 | - [Example react-native](https://github.com/i18next/react-i18next/tree/v9.x.x/example/reactnative-expo)
|
118 | - [Example expo.io](https://github.com/i18next/react-i18next/tree/v9.x.x/example/reactnative-expo)
|
119 | - [Example next.js](https://github.com/i18next/react-i18next/tree/v9.x.x/example/nextjs)
|
120 | - [Example razzle](https://github.com/i18next/react-i18next/tree/v9.x.x/example/razzle-ssr)
|
121 | - [Example hashbase / beaker browser](https://github.com/i18next/react-i18next/tree/v9.x.x/example/dat)
|
122 | - [Example storybook](https://github.com/i18next/react-i18next/tree/v9.x.x/example/storybook)
|
123 | - [Example locize.com](https://github.com/i18next/react-i18next/tree/v9.x.x/example/locize)
|
124 | - [Example test with jest](https://github.com/i18next/react-i18next/tree/v9.x.x/example/test-jest)
|
125 |
|
126 | ### Requirements
|
127 |
|
128 | - react >= **16.8.0**
|
129 | - react-dom >= **16.8.0**
|
130 | - react-native >= **0.59.0**
|
131 | - i18next >= **10.0.0** (typescript users: >=17.0.9)
|
132 |
|
133 | #### v9
|
134 |
|
135 | - react >= **0.14.0** (in case of < v16 or preact you will need to define parent in [Trans component](https://react.i18next.com/legacy-v9/trans-component#trans-props) or globally in [i18next.react options](https://react.i18next.com/legacy-v9/trans-component#additional-options-on-i-18-next-init))
|
136 | - i18next >= **2.0.0**
|
137 |
|
138 | ## Core Contributors
|
139 |
|
140 | Thanks goes to these wonderful people ([emoji key](https://github.com/kentcdodds/all-contributors#emoji-key)):
|
141 |
|
142 |
|
143 |
|
144 |
|
145 | <table>
|
146 | <tr>
|
147 | <td align="center"><a href="http://twitter.com/jamuhl"><img src="https://avatars3.githubusercontent.com/u/977772?v=4?s=100" width="100px;" alt=""/><br /><sub><b>Jan Mühlemann</b></sub></a><br /><a href="https://github.com/i18next/react-i18next/commits?author=jamuhl" title="Code">💻</a> <a href="#example-jamuhl" title="Examples">💡</a> <a href="https://github.com/i18next/react-i18next/pulls?q=is%3Apr+reviewed-by%3Ajamuhl+" title="Reviewed Pull Requests">👀</a> <a href="https://github.com/i18next/react-i18next/commits?author=jamuhl" title="Documentation">📖</a> <a href="#question-jamuhl" title="Answering Questions">💬</a></td>
|
148 | <td align="center"><a href="http://twitter.com/#!/adrirai"><img src="https://avatars0.githubusercontent.com/u/1086194?v=4?s=100" width="100px;" alt=""/><br /><sub><b>Adriano Raiano</b></sub></a><br /><a href="https://github.com/i18next/react-i18next/commits?author=adrai" title="Code">💻</a> <a href="#example-adrai" title="Examples">💡</a> <a href="https://github.com/i18next/react-i18next/pulls?q=is%3Apr+reviewed-by%3Aadrai+" title="Reviewed Pull Requests">👀</a> <a href="https://github.com/i18next/react-i18next/commits?author=adrai" title="Documentation">📖</a> <a href="#question-adrai" title="Answering Questions">💬</a></td>
|
149 | <td align="center"><a href="https://github.com/pedrodurek"><img src="https://avatars1.githubusercontent.com/u/12190482?v=4?s=100" width="100px;" alt=""/><br /><sub><b>Pedro Durek</b></sub></a><br /><a href="https://github.com/i18next/react-i18next/commits?author=pedrodurek" title="Code">💻</a> <a href="#example-pedrodurek" title="Examples">💡</a> <a href="https://github.com/i18next/react-i18next/pulls?q=is%3Apr+reviewed-by%3Apedrodurek+" title="Reviewed Pull Requests">👀</a> <a href="#question-pedrodurek" title="Answering Questions">💬</a></td>
|
150 | <td align="center"><a href="https://tigerabrodi.dev/"><img src="https://avatars1.githubusercontent.com/u/49603590?v=4?s=100" width="100px;" alt=""/><br /><sub><b>Tiger Abrodi</b></sub></a><br /><a href="https://github.com/i18next/react-i18next/commits?author=tigerabrodi" title="Code">💻</a> <a href="https://github.com/i18next/react-i18next/pulls?q=is%3Apr+reviewed-by%3Atigerabrodi" title="Reviewed Pull Requests">👀</a></td>
|
151 | </tr>
|
152 | </table>
|
153 |
|
154 |
|
155 |
|
156 |
|
157 |
|
158 |
|
159 | This project follows the [all-contributors](https://github.com/kentcdodds/all-contributors) specification. Contributions of any kind are welcome!
|
160 |
|
161 | ---
|
162 |
|
163 | <h3 align="center">Gold Sponsors</h3>
|
164 |
|
165 | <p align="center">
|
166 | <a href="https://locize.com/" target="_blank">
|
167 | <img src="https://raw.githubusercontent.com/i18next/i18next/master/assets/locize_sponsor_240.gif" width="240px">
|
168 | </a>
|
169 | </p>
|
170 |
|
171 | ---
|
172 |
|
173 | **localization as a service - locize.com**
|
174 |
|
175 | Needing a translation management? Want to edit your translations with an InContext Editor? Use the original provided to you by the maintainers of i18next!
|
176 |
|
177 | 
|
178 |
|
179 | By using [locize](http://locize.com/?utm_source=react_i18next_readme&utm_medium=github) you directly support the future of i18next and react-i18next.
|
180 |
|
181 | ---
|