1 | # React Native Router (v4.x) [](#backers) [](#sponsors) [](https://gitter.im/aksonov/react-native-router-flux?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.codacy.com/app/aksonov-github/react-native-router-flux) [](http://badge.fury.io/js/react-native-router-flux) [](https://circleci.com/gh/aksonov/react-native-router-flux)
|
2 |
|
3 | [Follow author @PAksonov](https://twitter.com/PAksonov)
|
4 |
|
5 | `react-native-router-flux` is a different API over `react-navigation`. It helps users to define all the routes in one central place and navigate and communicate between different screens in an easy way. But it also means that `react-native-router-flux` inherits all [limitations](https://reactnavigation.org/docs/en/limitations.html) and changes from updated versions.
|
6 |
|
7 | ### IMPORTANT NOTES
|
8 | #### v5.0.alpha.x is based on React Navigation v5.x (very early alpha, development in progress, help wanted!)
|
9 |
|
10 | #### v4.2.x is based on [React Navigation v4.x](https://reactnavigation.org/docs/4.x/getting-started)
|
11 |
|
12 | #### v4.1.0-beta.x is based on [React Navigation v3.x](https://reactnavigation.org/docs/en/3.x/getting-started.html)
|
13 |
|
14 | #### v4.0.x is based on [React Navigation v2.x]. See [this branch](https://github.com/aksonov/react-native-router-flux/tree/v3) and [docs](https://github.com/aksonov/react-native-router-flux/blob/master/README3.md) for v3 based on deprecated React Native Experimental Navigation API. It is not supported and may not work with latest React Native versions.
|
15 |
|
16 | #### v4.0.0-beta.x is based on [React Navigation v1.5.x](https://reactnavigation.org/). See [this branch](https://github.com/aksonov/react-native-router-flux/tree/v4.0.0-beta) for this version. It is also not supported and may not work with the latest React Native versions.
|
17 |
|
18 | ---
|
19 |
|
20 | - [Examples](#try-the-example-apps)
|
21 | - [Motivation](https://gist.github.com/aksonov/e2d7454421e44b1c4c72214d14053410)
|
22 | - [v4 Features](#v4-features)
|
23 | - [API](/docs/API.md)
|
24 | - [Migrating from v3](/docs/MIGRATION.md)
|
25 | - [Sponsors/Backers/Contributors](#contributors)
|
26 |
|
27 | ## Getting Started
|
28 |
|
29 | 1. Install native dependencies used by RNRF (see below, https://reactnavigation.org/docs/en/getting-started.html)
|
30 | 2. Install this component
|
31 |
|
32 | ```sh
|
33 | yarn add react-native-router-flux
|
34 | ```
|
35 |
|
36 | ## install the following libraries first
|
37 | 1. react-native-screens by ( ``` npm install react-native-screens || yarn add react-native-screens ```)
|
38 | 2. react-native-gesture-handler ( ``` npm install react-native-gesture-handler || yarn add react-native-gesture-handler ```)
|
39 | 3. react-native-reanimated (``` npm install react-native-reanimated || yarn add react-native-reanimated ```)
|
40 | 4. react-native-safe-area-context (``` npm install react-native-safe-area-context || yarn add react-native-react-native-safe-area-context ```)
|
41 | 5. @react-native-community/masked-view (``` npm install @react-native-community/masked-view || yarn add @react-native-community/masked-view ```)
|
42 |
|
43 |
|
44 |
|
45 | ## Usage
|
46 |
|
47 | Define all your routes in one React component...
|
48 |
|
49 | ```jsx
|
50 | const App = () => (
|
51 | <Router>
|
52 | <Stack key="root">
|
53 | <Scene key="login" component={Login} title="Login" />
|
54 | <Scene key="register" component={Register} title="Register" />
|
55 | <Scene key="home" component={Home} />
|
56 | </Stack>
|
57 | </Router>
|
58 | );
|
59 | ```
|
60 |
|
61 | ...and navigate from one scene to another scene with a simple and powerful API.
|
62 |
|
63 | ```jsx
|
64 | // Login.js
|
65 |
|
66 | // navigate to 'home' as defined in your top-level router
|
67 | Actions.home(PARAMS);
|
68 |
|
69 | // go back (i.e. pop the current screen off the nav stack)
|
70 | Actions.pop();
|
71 |
|
72 | // refresh the current Scene with the specified props
|
73 | Actions.refresh({ param1: 'hello', param2: 'world' });
|
74 | ```
|
75 |
|
76 | ## API
|
77 |
|
78 | For a full listing of the API, [view the API docs](https://github.com/aksonov/react-native-router-flux/blob/master/docs/API.md).
|
79 |
|
80 | ## Try the [example apps](https://github.com/aksonov/react-native-router-flux/tree/master/examples)
|
81 |
|
82 | 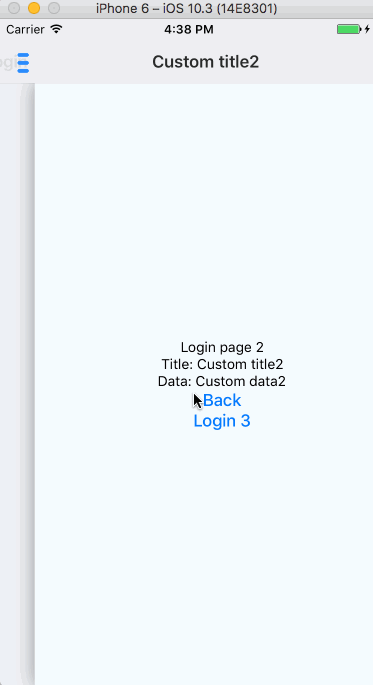
|
83 |
|
84 | ```sh
|
85 | # Get the code
|
86 | git clone https://github.com/aksonov/react-native-router-flux.git
|
87 | cd react-native-router-flux/examples/[expo|react-native|redux]
|
88 |
|
89 | # Installing dependencies
|
90 | yarn
|
91 |
|
92 | # Run it
|
93 | yarn start
|
94 | ```
|
95 |
|
96 | ## v4 Features
|
97 |
|
98 | - Based on latest [React Navigation](https://reactnavigation.org) API
|
99 | - Separate navigation logic from presentation. You may now change navigation state directly from your business logic code - stores/reducers/etc. navigationStore
|
100 | - Built-in state machine (v3 `Switch` replacement)
|
101 | - Each `Scene` with `component` defined can have `onEnter`/`onExit`/`on` handlers.
|
102 | - `onEnter`/`on` handler can be async.
|
103 | - For 'truthy' return of `onEnter`/`on`, `success` handler (if defined) will be executed
|
104 | - if `success` is a string then router will navigate to the `Scene` with that key
|
105 | - in case of handler's failure, `failure` prop (if defined) will be run.
|
106 | - Combining `onEnter`, `onExit`, `success`, and `failure` makes patterns like authentication, data validation, and conditional transitions simple and intuitive.
|
107 | - [MobX](https://mobx.js.org/)-friendly: all scenes are wrapped with `observer`. You may subscribe to `navigationStore` (`Actions` in v3) and observe current navigation state. Not applicable to Redux.
|
108 | - Flexible Nav bar customization, currently not allowed by React Navigation:
|
109 | https://github.com/react-community/react-navigation/issues/779
|
110 | - Drawer support (provided by React Navigation)
|
111 | - Inheritance of scene attributes allow you to avoid any code/attribute duplications. Adding `rightTitle` to a scene will apply to all child scenes simultaneously. See example app.
|
112 | - Access to your app navigations state as simple as `Actions.state`.
|
113 | - Use `Actions.currentScene` to get name of current scene.
|
114 |
|
115 | ### Helpful tips if you run into some gotchas
|
116 |
|
117 | ## Using Static on Methods with HOCs
|
118 |
|
119 | - This is just a helpful tip for anyone who use the onExit/onEnter methods as a static method in their Component Class. Please refer to this link https://reactjs.org/docs/higher-order-components.html.
|
120 |
|
121 | - If your Scene Components are Wrapped in Custom HOCs/ Decorators, then the static onExit/onEnter methods will not work as your Custom HOCs will not copy the static methods over to your Enhanced Component.Use the npm package called hoist-non-react-statics to copy your Component level static methods over to your Enhanced Component.
|
122 |
|
123 | ## Implement onBack from your Scene after declaring it
|
124 |
|
125 | - If you have a Scene where in you want to make some changes to your Component State when Back button is pressed, then doing this
|
126 |
|
127 | ```jsx
|
128 | <Scene key={...} component={...} onBack={()=>{/*code*/}}/>
|
129 | ```
|
130 |
|
131 | will not help.
|
132 |
|
133 | ```jsx
|
134 | <Scene key={...} component={...} onBack={()=>{/*code*/}} back={true}/>
|
135 | ```
|
136 |
|
137 | Make sure back = true is passed to your scene, now in your Component's lifecycle add this
|
138 |
|
139 | ```jsx
|
140 | componentDidMount(){
|
141 | InteractionManager.runAfterInteractions(()=> {
|
142 | Actions.refresh({onBack:()=>this.changeSomethingInYourComponent()})
|
143 | })
|
144 | }
|
145 | ```
|
146 |
|
147 | ## Contributors
|
148 |
|
149 | This project exists thanks to all the people who contribute. [[Contribute]](CONTRIBUTING.md).
|
150 | <a href="https://github.com/aksonov/react-native-router-flux/graphs/contributors"><img src="https://opencollective.com/react-native-router-flux/contributors.svg?width=890" /></a>
|
151 |
|
152 | ## Backers
|
153 |
|
154 | Thank you to all our backers! 🙏 [[Become a backer](https://opencollective.com/react-native-router-flux#backer)]
|
155 |
|
156 | <a href="https://opencollective.com/react-native-router-flux#backers" target="_blank"><img src="https://opencollective.com/react-native-router-flux/backers.svg?width=890"></a>
|
157 |
|
158 | ## Sponsors
|
159 |
|
160 | Support this project by becoming a sponsor. Your logo will show up here with a link to your website. [[Become a sponsor](https://opencollective.com/react-native-router-flux#sponsor)]
|
161 |
|
162 | <a href="https://opencollective.com/react-native-router-flux/sponsor/0/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/0/avatar.svg"></a>
|
163 | <a href="https://opencollective.com/react-native-router-flux/sponsor/1/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/1/avatar.svg"></a>
|
164 | <a href="https://opencollective.com/react-native-router-flux/sponsor/2/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/2/avatar.svg"></a>
|
165 | <a href="https://opencollective.com/react-native-router-flux/sponsor/3/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/3/avatar.svg"></a>
|
166 | <a href="https://opencollective.com/react-native-router-flux/sponsor/4/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/4/avatar.svg"></a>
|
167 | <a href="https://opencollective.com/react-native-router-flux/sponsor/5/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/5/avatar.svg"></a>
|
168 | <a href="https://opencollective.com/react-native-router-flux/sponsor/6/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/6/avatar.svg"></a>
|
169 | <a href="https://opencollective.com/react-native-router-flux/sponsor/7/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/7/avatar.svg"></a>
|
170 | <a href="https://opencollective.com/react-native-router-flux/sponsor/8/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/8/avatar.svg"></a>
|
171 | <a href="https://opencollective.com/react-native-router-flux/sponsor/9/website" target="_blank"><img src="https://opencollective.com/react-native-router-flux/sponsor/9/avatar.svg"></a>
|